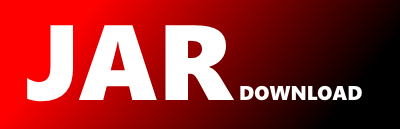
org.apache.rave.portal.model.JpaOrganization Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rave-jpa Show documentation
Show all versions of rave-jpa Show documentation
Apache Rave JPA Persistence Implementation
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.rave.portal.model;
import org.apache.rave.model.Address;
import org.apache.rave.model.Organization;
import org.apache.rave.portal.model.conversion.JpaConverter;
import javax.persistence.*;
import java.util.Date;
/**
*/
@Entity
@Access(AccessType.FIELD)
@NamedQueries(value = {
@NamedQuery(name = JpaOrganization.FIND_BY_NAME, query = "select o from JpaOrganization o where o.name like :name")
})
@Table(name = "organization")
public class JpaOrganization implements BasicEntity, Organization {
public static final String FIND_BY_NAME = "findByName";
public static final String NAME_PARAM = "name";
/**
* The internal object ID used for references to this object. Should be generated by the
* underlying storage mechanism
*/
@Id
@Column(name = "entity_id")
@GeneratedValue(strategy = GenerationType.TABLE, generator = "organizationIdGenerator")
@TableGenerator(name = "organizationIdGenerator", table = "RAVE_PORTAL_SEQUENCES", pkColumnName = "SEQ_NAME",
valueColumnName = "SEQ_COUNT", pkColumnValue = "organization", allocationSize = 1, initialValue = 1)
private Long entityId;
@OneToOne
private JpaAddress address;
@Basic
@Column(name = "description", length = 255)
private String description;
@Basic
@Column(name = "endDate")
@Temporal(TemporalType.DATE)
private Date endDate;
@Basic
@Column(name = "field", length = 255)
private String field;
@Basic
@Column(name = "name", length = 255)
private String name;
@Basic
@Column(name = "start_date")
@Temporal(TemporalType.DATE)
private Date startDate;
@Basic
@Column(name = "sub_field", length = 255)
private String subField;
@Basic
@Column(name = "title", length = 255)
private String title;
@Basic
@Column(name = "webpage", length = 255)
private String webpage;
@Basic
@Column(name = "type", length = 255)
private String qualifier;
@Basic
@Column(name = "primary_organization")
private Boolean primary;
/**
*/
public Long getEntityId() {
return entityId;
}
public void setEntityId(Long entityId) {
this.entityId = entityId;
}
@Override
public Address getAddress() {
return address;
}
@Override
public void setAddress(Address address) {
this.address = JpaConverter.getInstance().convert(address, Address.class);
}
@Override
public String getDescription() {
return description;
}
@Override
public void setDescription(String description) {
this.description = description;
}
@Override
public Date getEndDate() {
return endDate;
}
@Override
public void setEndDate(Date endDate) {
this.endDate = endDate;
}
@Override
public String getField() {
return field;
}
@Override
public void setField(String field) {
this.field = field;
}
@Override
public String getName() {
return name;
}
@Override
public void setName(String name) {
this.name = name;
}
@Override
public Date getStartDate() {
return startDate;
}
@Override
public void setStartDate(Date startDate) {
this.startDate = startDate;
}
@Override
public String getSubField() {
return subField;
}
@Override
public void setSubField(String subField) {
this.subField = subField;
}
@Override
public String getTitle() {
return title;
}
@Override
public void setTitle(String title) {
this.title = title;
}
@Override
public String getWebpage() {
return webpage;
}
@Override
public void setWebpage(String webpage) {
this.webpage = webpage;
}
@Override
public String getQualifier() {
return qualifier;
}
@Override
public void setQualifier(String qualifier) {
this.qualifier = qualifier;
}
@Override
public Boolean getPrimary() {
return primary;
}
@Override
public void setPrimary(Boolean primary) {
this.primary = primary;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
JpaOrganization that = (JpaOrganization) o;
if (entityId != null ? !entityId.equals(that.entityId) : that.entityId != null) return false;
return true;
}
@Override
public int hashCode() {
return entityId != null ? entityId.hashCode() : 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy