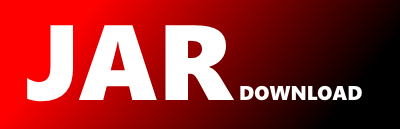
org.apache.reef.webserver.AvroHttpRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reef-webserver Show documentation
Show all versions of reef-webserver Show documentation
HTTP Server component to implement a REST API for the Driver or Evaluators.
The newest version!
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.apache.reef.webserver;
import org.apache.avro.specific.SpecificData;
@SuppressWarnings("all")
@org.apache.avro.specific.AvroGenerated
public class AvroHttpRequest extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
private static final long serialVersionUID = -3314313793478582565L;
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"AvroHttpRequest\",\"namespace\":\"org.apache.reef.webserver\",\"fields\":[{\"name\":\"header\",\"type\":{\"type\":\"array\",\"items\":{\"type\":\"record\",\"name\":\"HeaderEntry\",\"fields\":[{\"name\":\"key\",\"type\":\"string\"},{\"name\":\"value\",\"type\":\"string\"}]}}},{\"name\":\"requestUrl\",\"type\":\"string\"},{\"name\":\"pathInfo\",\"type\":\"string\"},{\"name\":\"queryString\",\"type\":\"string\"},{\"name\":\"httpMethod\",\"type\":\"string\"},{\"name\":\"inputStream\",\"type\":\"bytes\"}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
@Deprecated public java.util.List header;
@Deprecated public java.lang.CharSequence requestUrl;
@Deprecated public java.lang.CharSequence pathInfo;
@Deprecated public java.lang.CharSequence queryString;
@Deprecated public java.lang.CharSequence httpMethod;
@Deprecated public java.nio.ByteBuffer inputStream;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public AvroHttpRequest() {}
/**
* All-args constructor.
* @param header The new value for header
* @param requestUrl The new value for requestUrl
* @param pathInfo The new value for pathInfo
* @param queryString The new value for queryString
* @param httpMethod The new value for httpMethod
* @param inputStream The new value for inputStream
*/
public AvroHttpRequest(java.util.List header, java.lang.CharSequence requestUrl, java.lang.CharSequence pathInfo, java.lang.CharSequence queryString, java.lang.CharSequence httpMethod, java.nio.ByteBuffer inputStream) {
this.header = header;
this.requestUrl = requestUrl;
this.pathInfo = pathInfo;
this.queryString = queryString;
this.httpMethod = httpMethod;
this.inputStream = inputStream;
}
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return header;
case 1: return requestUrl;
case 2: return pathInfo;
case 3: return queryString;
case 4: return httpMethod;
case 5: return inputStream;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: header = (java.util.List)value$; break;
case 1: requestUrl = (java.lang.CharSequence)value$; break;
case 2: pathInfo = (java.lang.CharSequence)value$; break;
case 3: queryString = (java.lang.CharSequence)value$; break;
case 4: httpMethod = (java.lang.CharSequence)value$; break;
case 5: inputStream = (java.nio.ByteBuffer)value$; break;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'header' field.
* @return The value of the 'header' field.
*/
public java.util.List getHeader() {
return header;
}
/**
* Sets the value of the 'header' field.
* @param value the value to set.
*/
public void setHeader(java.util.List value) {
this.header = value;
}
/**
* Gets the value of the 'requestUrl' field.
* @return The value of the 'requestUrl' field.
*/
public java.lang.CharSequence getRequestUrl() {
return requestUrl;
}
/**
* Sets the value of the 'requestUrl' field.
* @param value the value to set.
*/
public void setRequestUrl(java.lang.CharSequence value) {
this.requestUrl = value;
}
/**
* Gets the value of the 'pathInfo' field.
* @return The value of the 'pathInfo' field.
*/
public java.lang.CharSequence getPathInfo() {
return pathInfo;
}
/**
* Sets the value of the 'pathInfo' field.
* @param value the value to set.
*/
public void setPathInfo(java.lang.CharSequence value) {
this.pathInfo = value;
}
/**
* Gets the value of the 'queryString' field.
* @return The value of the 'queryString' field.
*/
public java.lang.CharSequence getQueryString() {
return queryString;
}
/**
* Sets the value of the 'queryString' field.
* @param value the value to set.
*/
public void setQueryString(java.lang.CharSequence value) {
this.queryString = value;
}
/**
* Gets the value of the 'httpMethod' field.
* @return The value of the 'httpMethod' field.
*/
public java.lang.CharSequence getHttpMethod() {
return httpMethod;
}
/**
* Sets the value of the 'httpMethod' field.
* @param value the value to set.
*/
public void setHttpMethod(java.lang.CharSequence value) {
this.httpMethod = value;
}
/**
* Gets the value of the 'inputStream' field.
* @return The value of the 'inputStream' field.
*/
public java.nio.ByteBuffer getInputStream() {
return inputStream;
}
/**
* Sets the value of the 'inputStream' field.
* @param value the value to set.
*/
public void setInputStream(java.nio.ByteBuffer value) {
this.inputStream = value;
}
/**
* Creates a new AvroHttpRequest RecordBuilder.
* @return A new AvroHttpRequest RecordBuilder
*/
public static org.apache.reef.webserver.AvroHttpRequest.Builder newBuilder() {
return new org.apache.reef.webserver.AvroHttpRequest.Builder();
}
/**
* Creates a new AvroHttpRequest RecordBuilder by copying an existing Builder.
* @param other The existing builder to copy.
* @return A new AvroHttpRequest RecordBuilder
*/
public static org.apache.reef.webserver.AvroHttpRequest.Builder newBuilder(org.apache.reef.webserver.AvroHttpRequest.Builder other) {
return new org.apache.reef.webserver.AvroHttpRequest.Builder(other);
}
/**
* Creates a new AvroHttpRequest RecordBuilder by copying an existing AvroHttpRequest instance.
* @param other The existing instance to copy.
* @return A new AvroHttpRequest RecordBuilder
*/
public static org.apache.reef.webserver.AvroHttpRequest.Builder newBuilder(org.apache.reef.webserver.AvroHttpRequest other) {
return new org.apache.reef.webserver.AvroHttpRequest.Builder(other);
}
/**
* RecordBuilder for AvroHttpRequest instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.util.List header;
private java.lang.CharSequence requestUrl;
private java.lang.CharSequence pathInfo;
private java.lang.CharSequence queryString;
private java.lang.CharSequence httpMethod;
private java.nio.ByteBuffer inputStream;
/** Creates a new Builder */
private Builder() {
super(SCHEMA$);
}
/**
* Creates a Builder by copying an existing Builder.
* @param other The existing Builder to copy.
*/
private Builder(org.apache.reef.webserver.AvroHttpRequest.Builder other) {
super(other);
if (isValidValue(fields()[0], other.header)) {
this.header = data().deepCopy(fields()[0].schema(), other.header);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.requestUrl)) {
this.requestUrl = data().deepCopy(fields()[1].schema(), other.requestUrl);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.pathInfo)) {
this.pathInfo = data().deepCopy(fields()[2].schema(), other.pathInfo);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.queryString)) {
this.queryString = data().deepCopy(fields()[3].schema(), other.queryString);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.httpMethod)) {
this.httpMethod = data().deepCopy(fields()[4].schema(), other.httpMethod);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.inputStream)) {
this.inputStream = data().deepCopy(fields()[5].schema(), other.inputStream);
fieldSetFlags()[5] = true;
}
}
/**
* Creates a Builder by copying an existing AvroHttpRequest instance
* @param other The existing instance to copy.
*/
private Builder(org.apache.reef.webserver.AvroHttpRequest other) {
super(SCHEMA$);
if (isValidValue(fields()[0], other.header)) {
this.header = data().deepCopy(fields()[0].schema(), other.header);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.requestUrl)) {
this.requestUrl = data().deepCopy(fields()[1].schema(), other.requestUrl);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.pathInfo)) {
this.pathInfo = data().deepCopy(fields()[2].schema(), other.pathInfo);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.queryString)) {
this.queryString = data().deepCopy(fields()[3].schema(), other.queryString);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.httpMethod)) {
this.httpMethod = data().deepCopy(fields()[4].schema(), other.httpMethod);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.inputStream)) {
this.inputStream = data().deepCopy(fields()[5].schema(), other.inputStream);
fieldSetFlags()[5] = true;
}
}
/**
* Gets the value of the 'header' field.
* @return The value.
*/
public java.util.List getHeader() {
return header;
}
/**
* Sets the value of the 'header' field.
* @param value The value of 'header'.
* @return This builder.
*/
public org.apache.reef.webserver.AvroHttpRequest.Builder setHeader(java.util.List value) {
validate(fields()[0], value);
this.header = value;
fieldSetFlags()[0] = true;
return this;
}
/**
* Checks whether the 'header' field has been set.
* @return True if the 'header' field has been set, false otherwise.
*/
public boolean hasHeader() {
return fieldSetFlags()[0];
}
/**
* Clears the value of the 'header' field.
* @return This builder.
*/
public org.apache.reef.webserver.AvroHttpRequest.Builder clearHeader() {
header = null;
fieldSetFlags()[0] = false;
return this;
}
/**
* Gets the value of the 'requestUrl' field.
* @return The value.
*/
public java.lang.CharSequence getRequestUrl() {
return requestUrl;
}
/**
* Sets the value of the 'requestUrl' field.
* @param value The value of 'requestUrl'.
* @return This builder.
*/
public org.apache.reef.webserver.AvroHttpRequest.Builder setRequestUrl(java.lang.CharSequence value) {
validate(fields()[1], value);
this.requestUrl = value;
fieldSetFlags()[1] = true;
return this;
}
/**
* Checks whether the 'requestUrl' field has been set.
* @return True if the 'requestUrl' field has been set, false otherwise.
*/
public boolean hasRequestUrl() {
return fieldSetFlags()[1];
}
/**
* Clears the value of the 'requestUrl' field.
* @return This builder.
*/
public org.apache.reef.webserver.AvroHttpRequest.Builder clearRequestUrl() {
requestUrl = null;
fieldSetFlags()[1] = false;
return this;
}
/**
* Gets the value of the 'pathInfo' field.
* @return The value.
*/
public java.lang.CharSequence getPathInfo() {
return pathInfo;
}
/**
* Sets the value of the 'pathInfo' field.
* @param value The value of 'pathInfo'.
* @return This builder.
*/
public org.apache.reef.webserver.AvroHttpRequest.Builder setPathInfo(java.lang.CharSequence value) {
validate(fields()[2], value);
this.pathInfo = value;
fieldSetFlags()[2] = true;
return this;
}
/**
* Checks whether the 'pathInfo' field has been set.
* @return True if the 'pathInfo' field has been set, false otherwise.
*/
public boolean hasPathInfo() {
return fieldSetFlags()[2];
}
/**
* Clears the value of the 'pathInfo' field.
* @return This builder.
*/
public org.apache.reef.webserver.AvroHttpRequest.Builder clearPathInfo() {
pathInfo = null;
fieldSetFlags()[2] = false;
return this;
}
/**
* Gets the value of the 'queryString' field.
* @return The value.
*/
public java.lang.CharSequence getQueryString() {
return queryString;
}
/**
* Sets the value of the 'queryString' field.
* @param value The value of 'queryString'.
* @return This builder.
*/
public org.apache.reef.webserver.AvroHttpRequest.Builder setQueryString(java.lang.CharSequence value) {
validate(fields()[3], value);
this.queryString = value;
fieldSetFlags()[3] = true;
return this;
}
/**
* Checks whether the 'queryString' field has been set.
* @return True if the 'queryString' field has been set, false otherwise.
*/
public boolean hasQueryString() {
return fieldSetFlags()[3];
}
/**
* Clears the value of the 'queryString' field.
* @return This builder.
*/
public org.apache.reef.webserver.AvroHttpRequest.Builder clearQueryString() {
queryString = null;
fieldSetFlags()[3] = false;
return this;
}
/**
* Gets the value of the 'httpMethod' field.
* @return The value.
*/
public java.lang.CharSequence getHttpMethod() {
return httpMethod;
}
/**
* Sets the value of the 'httpMethod' field.
* @param value The value of 'httpMethod'.
* @return This builder.
*/
public org.apache.reef.webserver.AvroHttpRequest.Builder setHttpMethod(java.lang.CharSequence value) {
validate(fields()[4], value);
this.httpMethod = value;
fieldSetFlags()[4] = true;
return this;
}
/**
* Checks whether the 'httpMethod' field has been set.
* @return True if the 'httpMethod' field has been set, false otherwise.
*/
public boolean hasHttpMethod() {
return fieldSetFlags()[4];
}
/**
* Clears the value of the 'httpMethod' field.
* @return This builder.
*/
public org.apache.reef.webserver.AvroHttpRequest.Builder clearHttpMethod() {
httpMethod = null;
fieldSetFlags()[4] = false;
return this;
}
/**
* Gets the value of the 'inputStream' field.
* @return The value.
*/
public java.nio.ByteBuffer getInputStream() {
return inputStream;
}
/**
* Sets the value of the 'inputStream' field.
* @param value The value of 'inputStream'.
* @return This builder.
*/
public org.apache.reef.webserver.AvroHttpRequest.Builder setInputStream(java.nio.ByteBuffer value) {
validate(fields()[5], value);
this.inputStream = value;
fieldSetFlags()[5] = true;
return this;
}
/**
* Checks whether the 'inputStream' field has been set.
* @return True if the 'inputStream' field has been set, false otherwise.
*/
public boolean hasInputStream() {
return fieldSetFlags()[5];
}
/**
* Clears the value of the 'inputStream' field.
* @return This builder.
*/
public org.apache.reef.webserver.AvroHttpRequest.Builder clearInputStream() {
inputStream = null;
fieldSetFlags()[5] = false;
return this;
}
@Override
public AvroHttpRequest build() {
try {
AvroHttpRequest record = new AvroHttpRequest();
record.header = fieldSetFlags()[0] ? this.header : (java.util.List) defaultValue(fields()[0]);
record.requestUrl = fieldSetFlags()[1] ? this.requestUrl : (java.lang.CharSequence) defaultValue(fields()[1]);
record.pathInfo = fieldSetFlags()[2] ? this.pathInfo : (java.lang.CharSequence) defaultValue(fields()[2]);
record.queryString = fieldSetFlags()[3] ? this.queryString : (java.lang.CharSequence) defaultValue(fields()[3]);
record.httpMethod = fieldSetFlags()[4] ? this.httpMethod : (java.lang.CharSequence) defaultValue(fields()[4]);
record.inputStream = fieldSetFlags()[5] ? this.inputStream : (java.nio.ByteBuffer) defaultValue(fields()[5]);
return record;
} catch (Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
private static final org.apache.avro.io.DatumWriter
WRITER$ = new org.apache.avro.specific.SpecificDatumWriter(SCHEMA$);
@Override public void writeExternal(java.io.ObjectOutput out)
throws java.io.IOException {
WRITER$.write(this, SpecificData.getEncoder(out));
}
private static final org.apache.avro.io.DatumReader
READER$ = new org.apache.avro.specific.SpecificDatumReader(SCHEMA$);
@Override public void readExternal(java.io.ObjectInput in)
throws java.io.IOException {
READER$.read(this, SpecificData.getDecoder(in));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy