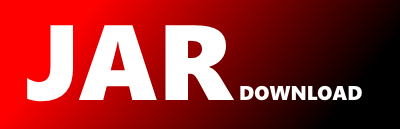
org.apache.rocketmq.test.clientinterface.MQCollector Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.rocketmq.test.clientinterface;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import org.apache.rocketmq.test.util.RandomUtil;
import org.apache.rocketmq.test.util.data.collect.DataCollector;
import org.apache.rocketmq.test.util.data.collect.DataCollectorManager;
public abstract class MQCollector {
protected DataCollector msgBodys = null;
protected DataCollector originMsgs = null;
protected DataCollector errorMsgs = null;
protected Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy