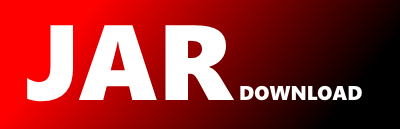
org.apache.royale.compiler.definitions.IDefinition Maven / Gradle / Ivy
Show all versions of compiler-common Show documentation
/*
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.royale.compiler.definitions;
import java.util.List;
import org.apache.royale.compiler.common.ASModifier;
import org.apache.royale.compiler.common.ModifiersSet;
import org.apache.royale.compiler.definitions.metadata.IDeprecationInfo;
import org.apache.royale.compiler.definitions.metadata.IMetaTag;
import org.apache.royale.compiler.definitions.references.INamespaceReference;
import org.apache.royale.compiler.definitions.references.IReference;
import org.apache.royale.compiler.projects.ICompilerProject;
import org.apache.royale.compiler.scopes.IASScope;
import org.apache.royale.compiler.tree.as.IDefinitionNode;
import org.apache.royale.compiler.tree.metadata.IMetaTagNode;
/**
* The base class for all definitions.
*
* A definition is something in source code that can be referred to by name:
* a package, namespace, class, interface, function, getter, setter,
* parameter, variable, constant, event, style, or effect.
*
*
* Each definition has
*
* - a source location;
* - an optional list of metadata annotations;
* - a namespace reference indicating its visibility,
* such as
public
;
* - a set of flags indicating modifiers such as
static
* or override
;
* - a base, or unqualified, name;
* - a type reference (which may be
null
* for some types of definitions.
*
* Specific types of definitions of course store additional information.
* For example, a class definition stores a reference to the class it extends
* and references to the interfaces that it implements.
*
* Definitions refer to other definitions indirectly, by name,
* using an IReference
. See the references
* subpackage for an explanation of this design.
*/
public interface IDefinition
{
/**
* Gets the local source path of the entire definition (not just the name).
* It is normalized.
*
* @return An String for the path.
*/
String getSourcePath();
/**
* Gets the local starting offset of the entire definition (not just the
* name). It is zero-based.
*
* @return An int
for the offset.
*/
int getStart();
/**
* Gets the local ending offset of this entire definition (not just the
* name). It is zero-based.
*
* @return An int
for the offset.
*/
int getEnd();
/**
* Gets the local line number of this entire definition (not just the name).
* It is zero-based.
*
* @return An int
for the line number.
*/
int getLine();
/**
* Gets the local column number of the entire definition (not just the
* name). It is zero-based.
*
* @return An int
for the column number.
*/
int getColumn();
/**
* Gets the local line number of the end of the entire definition (not just
* the name). It is zero-based.
*
* @return An int
for the line number.
*/
int getEndLine();
/**
* Gets the local column number of the end of the entire definition (not
* just the name). It is zero-based.
*
* @return An int
for the column number.
*/
int getEndColumn();
/**
* Gets the absolute starting offset of the entire definition (not just the
* name). It is zero-based.
*
* @return An int
for the offset.
*/
int getAbsoluteStart();
/**
* Gets the absolute ending offset of the entire definition (not just the
* name). It is zero-based.
*
* @return An int
for the offset.
*/
int getAbsoluteEnd();
/**
* Gets the base (i.e., unqualified) name of this definition.
*
* For example, the base name of the class definition
* for the class flash.display.Sprite
* is "Sprite"
.
*
* @return The base name as a String.
*/
String getBaseName();
/**
* Gets the fully-qualified package name for this definition.
*
* For example, the backage name of the class definition
* for the class flash.display.Sprite
* is "flash.display"
*
* @return The fully-qualified package name as a String.
*/
String getPackageName();
/**
* Gets the fully-qualified name of this definition.
*
* For example, the fully-qualified name of the class definition
* for the class flash.display.Sprite
is
* "flash.display.Sprite"
.
*
* @return The fully-qualified name as a String.
*/
String getQualifiedName();
/**
* Gets the local starting offset of the name of this definition. It is
* zero-based.
*
* @return An int
for the offset.
*/
int getNameStart();
/**
* Gets the local ending offset of the name of this definition. It is
* zero-based.
*
* @return An int
for the offset.
*/
int getNameEnd();
/**
* Gets the local line number of the name of this definition. It is
* zero-based.
*
* @return An int
for the line number.
*/
int getNameLine();
/**
* Gets the local column number of the name of this definition. It is
* zero-based.
*
* @return An int
for the column number.
*/
int getNameColumn();
/**
* Gets the scope in which this definition exists.
*
* @return An IASScope for the containing scope, or null
if
* this definition has not yet been added to a scope.
*/
IASScope getContainingScope();
/**
* Gets the parent definition of this definition.
*
* @return An IDefinition for the parent, or null
if there is
* no parent.
*/
IDefinition getParent();
/**
* Gets an ancestor definition of this definition.
*
* This method walks up the chain of parent definitions and returns the
* first one having the specified type.
*
* @param ancestorType A Class specifying the desired type of ancestor.
* @return An IDefinition for the ancestor.
*/
IDefinition getAncestorOfType(Class extends IDefinition> ancestorType);
/**
* Gets the file path in which this definition is defined.
*
* @return A String for the file path.
*/
String getContainingFilePath();
/**
* Gets the source file path in which this definition is defined.
*
* For definitions from a source file (like *.as), the result is the same as
* {@link #getContainingFilePath()}.
*
* If the definition is from a SWC library, and the library source path was
* set, the result is the file path in the source directory. If the source
* path was not set, the result is null.
*
* @param project the containing project
* @return A String for the file path.
*/
String getContainingSourceFilePath(ICompilerProject project);
/**
* Is this definition marked as dynamic
?
*
* @return true
if the definition is dynamic
.
*/
boolean isDynamic();
/**
* Is this definition marked as final
?
*
* @return true
if the definition is final
.
*/
boolean isFinal();
/**
* Is this definition marked as native
?
*
* @return true
if the definition is native
.
*/
boolean isNative();
/**
* Is this definition marked as override
?
*
* @return true
if the definition is override
.
*/
boolean isOverride();
/**
* Is this definition marked as static
?
*
* @return true
if the definition is static
.
*/
boolean isStatic();
/**
* Is this definition marked as abstract
?
*
* @return true
if the definition is abstract
.
*/
boolean isAbstract();
/**
* Determines whether the specified modifier is present on this definition.
* See {@link ASModifier} for the list of modifiers.
*
* @return true
if the modifier is present.
*/
boolean hasModifier(ASModifier modifier);
ModifiersSet getModifiers();
/**
* Determines whether the specified namespace is present on this definition.
*
* Namespaces include public
, private
,
* protected
, and internal
, plus any custom
* namespaces available in the definition's scope.
*
* The namespace is specified by an INamespaceReference, which can represent
* not only a custom namespace like ns1
but also
* ns1::ns2
, (ns1::ns2)::ns3
, etc.
*
* @param namespace An INamespaceReference specifying the namespace to check
* for.
* @return true
if the namespace is present.
*/
boolean hasNamespace(INamespaceReference namespace, ICompilerProject project);
/**
* Gets the namespace that this definition belongs to.
*
* @return An INamespaceReference representing the namespace. For some
* built-in namespaces, you can:
* == NamespaceDefinition.getPublic()
,
* instanceof IPublicNamespaceDefinition
,
* instanceof IProtectedNamespaceDefinition
, or For a custom
* namespace, the INamespaceReference will represent an expression of the
* form ns1
, ns1::ns2
,
* (ns1::ns2)::ns3
, etc.
*/
INamespaceReference getNamespaceReference();
/**
* Resolves the namespace specified for this definition to its
* INamespaceDefinition. Built-in namespaces will return null
.
*
* @return An {@link INamespaceDefinition} or null
.
*/
INamespaceDefinition resolveNamespace(ICompilerProject project);
/**
* Determines whether this definition is in a public namespace.
*
* @return true
if it is.
*/
boolean isPublic();
/**
* Determines whether this definition is in a private namespace.
*
* @return true
if it is.
*/
boolean isPrivate();
/**
* Determines whether this definition is in a protected namespace.
*
* @return true
if it is.
*/
boolean isProtected();
/**
* Determines whether this definition is in an internal namespace.
*
* @return true
if it is.
*/
boolean isInternal();
/**
* Gets a reference to the type of this definition.
*
* For a constant, variable, parameter, getter, or setter definition,
* the type reference is determined by the type annotation.
* For example, for a variable declaration like var i:int
,
* the type reference is a reference to the int
class.
* (For a setter, the relevant type annotation is on the parameter,
* not the return type, which is supposed to be void
.)
* If the type annotation is missing, the reference is null
.
*
* For a function definition, the type reference is always
* to the Function
class. You must use the
* getReturnTypeReference()
method of
* FunctionDefinition
to get the function's return type.
*
* For a rest parameter, the type reference is always
* to the Array
class.
*
* For a package, class, or interface definition,
* the type reference is null
.
*
* For style and event definitions, the type reference
* is determined by the type
attribute in the metadata.
*
* @return An {@link IReference} to a class or interface.
*/
IReference getTypeReference();
/**
* Resolves the type of this definition to an {@link ITypeDefinition}.
*
* @param project The {@link ICompilerProject} within which references are
* to be resolved.
* @return An {@link ITypeDefinition} or null
*/
ITypeDefinition resolveType(ICompilerProject project);
/**
* Converts this definition's type reference to a human-readable String.
*
* If the definition does not have a type reference,
* this method returns the empty string.
*
* This method should only be used for displaying types in problems,
* and not for making semantic decisions.
*
* @return The result of calling {@link IReference#getDisplayString}()
* on the {@link IReference} returned by {@link #getTypeReference}().
*/
String getTypeAsDisplayString();
/**
* Is this definition an implicit definition that doesn't actually appear in
* the source file? Examples include this
, super
,
* default constructors, and cast functions.
*/
boolean isImplicit();
/**
* Gets all the {@link IMetaTagNode} objects that match the given name
*
* @param name the name to match, such as Event, Style, IconFile, etc
* @return an array of {@link IMetaTagNode} objects, or empty array (never
* null)
*/
IMetaTag[] getMetaTagsByName(String name);
/**
* Returns all the {@link IMetaTagNode} objects as an array
*
* @return an array of objects, or an empty array
*/
IMetaTag[] getAllMetaTags();
/**
* Determines if a specific {@link IMetaTagNode} exists in this collection
*
* @param name the name of the tag
* @return true if it exists
*/
boolean hasMetaTagByName(String name);
/**
* Returns the first {@link IMetaTagNode} matching the given name
*
* @param name the name to search for
* @return an {@link IMetaTagNode} or null
*/
IMetaTag getMetaTagByName(String name);
/**
* Returns the {@link IDefinitionNode} from which this definition was
* created, if the definition came from the AST for a source file.
*
* This method may require the AST to be reloaded or regenerated and
* therefore may be slow.
*
* More specific definition interfaces such as {@link IClassDefinition}
* redeclare this method to return a more specific node interface such as
* IClassNode.
*/
IDefinitionNode getNode();
/**
* Whether this definition was specified as "Bindable" in its metadata
*
* @return true if this definition is Bindable.
*/
boolean isBindable();
/**
* @return a collection of Bindable event names, if any were specified in
* the metadata
*/
List getBindableEventNames();
/**
* @return true if this definition has metadata [Bindable(style="true")]
*/
boolean isBindableStyle();
/**
* @return true if contingent definition
*/
boolean isContingent();
/**
* @return true
if this definition is for an auto-generated
* embed class (i.e., one created for a var
with
* [Embed(...)]
metadata).
*/
boolean isGeneratedEmbedClass();
/**
* Check if the contingent definition is needed
*
* @param project
* @return true is the contingent definition is needed
*/
boolean isContingentNeeded(ICompilerProject project);
/**
* Checks if this definition is deprecated.
*
* @return true
if it has [Deprecated]
metadata.
*/
boolean isDeprecated();
/**
* Gets the information in the definition's [Deprecated]
metadata.
*
* @return An {@link IDeprecationInfo} object.
*/
IDeprecationInfo getDeprecationInfo();
/**
* Debugging method that can be used to assert that a definition is a specified project.
* @param project
* @return true if this definition is in the specified project.
*/
boolean isInProject(ICompilerProject project);
}