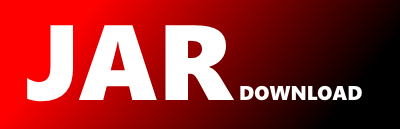
org.apache.royale.compiler.internal.mxml.MXMLDialect Maven / Gradle / Ivy
Show all versions of compiler-common Show documentation
/*
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.royale.compiler.internal.mxml;
import java.util.EnumSet;
import java.util.List;
import java.util.Map;
import com.google.common.collect.ImmutableMap;
import org.apache.royale.compiler.common.PrefixMap;
import org.apache.royale.compiler.common.XMLName;
import org.apache.royale.compiler.projects.ICompilerProject;
import org.apache.royale.compiler.mxml.IMXMLLanguageConstants;
/**
* This is the abstract base class for classes representing dialects of MXML,
* such as MXML 2006 or MXML 2009.
*
* Each MXML dialect has a unique language namespace, such as
* "http://ns.adobe.com/mxml/2009"
for MXML 2009.
*
* You can use an MXML dialect to get the XML name for various core tags of the
* language, such as ("http://ns.adobe.com/mxml/2009", "Library")
* for the {@code } tag of MXML 2009. The name will be
* null
if the tag is not supported in the dialect. For example,
* the {@code } tag does not exist in MXML 2006.
*
* You can also use an MXML dialect to perform core MXML parsing, such as
* parsing ActionScript values of type Boolean
, int
,
* etc. from attribute values and character data.
*
* Static methods on this class allow you to determine whether a URI is language
* namespace for a dialect of MXML, and to get the dialect object for that
* namespace.
*/
public abstract class MXMLDialect
{
/**
* The MXMLDialect
representing MXML 2012 (experimental).
*/
public static final MXMLDialect MXML_2012 = MXMLDialect2012.getInstance();
/**
* The MXMLDialect
representing MXML 2009.
*/
public static final MXMLDialect MXML_2009 = MXMLDialect2009.getInstance();
/**
* The MXMLDialect
representing MXML 2006.
*/
public static final MXMLDialect MXML_2006 = MXMLDialect2006.getInstance();
/**
* The MXMLDialect
representing the default dialect of MXML. It
* is used when the dialect is not explicitly expressed.
*/
public static final MXMLDialect DEFAULT = MXML_2009;
/*
* A map of language namespace to dialect, such as
* "http://ns.adobe.com/mxml/2009" -> MXMLDialect.MXML_2009.
*/
private static final Map DIALECT_MAP =
new ImmutableMap.Builder()
.put(MXML_2006.getLanguageNamespace(), MXML_2006)
.put(MXML_2009.getLanguageNamespace(), MXML_2009)
.put(MXML_2012.getLanguageNamespace(), MXML_2012)
.build();
/**
* A map of entities to characters, such as "lt" -> '<'.
* TODO HTML 4 supports about 250 named characters
* HTML 5 supports about 2500. How many should MXML support
* beyond these required 5? What did Xerces/mxmlc support?
*/
private static final Map NAMED_ENTITY_MAP =
new ImmutableMap.Builder()
.put("amp", '&')
.put("apos", '\'')
.put("gt", '>')
.put("lt", '<')
.put("quot", '"')
.put("nbsp", ' ')
.build();
/**
* Determines whether a specified URI is the language namespace for a
* supported dialect of MXML.
*
* The supported URIs are:
*
* "http://www.adobe.com/2006/mxml"
for MXML 2006
* "http://ns.adobe.com/mxml/2009"
for MXML 2009
* "http://ns.adobe.com/mxml/2012"
for MXML 2012
*
*
* @param uri A URI specifying a language namespace.
* @return true
if the URI is a supported language namespace.
*/
public static boolean isLanguageNamespace(String uri)
{
return DIALECT_MAP.containsKey(uri);
}
/**
* Gets the MXMLDialect
object corresponding to a specified
* language namespace.
*
* @param uri A URI string specifying a langauge namespace.
* @return An MXMLDialect
or null
.
*/
public static MXMLDialect getDialectForLanguageNamespace(String uri)
{
return DIALECT_MAP.get(uri);
}
/**
* Given a PrefixMap
representing the xmlns
* attributes on the root tag, determines which dialect of MXML is being
* used.
*
* @param rootPrefixMap A {@link PrefixMap}.
* @return An {@link MXMLDialect}.
*/
public static MXMLDialect getMXMLDialect(PrefixMap rootPrefixMap)
{
for (String prefix : rootPrefixMap.getAllPrefixes())
{
String ns = rootPrefixMap.getNamespaceForPrefix(prefix);
if (isLanguageNamespace(ns))
return getDialectForLanguageNamespace(ns);
}
// When we can't find anything, use the default dialect.
return MXMLDialect.DEFAULT;
}
/**
* Constructor.
*
* @param languageNamespace The language namespace URI that identifies this
* dialect of MXML, such as "http://ns.adobe.com/mxml/2009"
for
* MXML 2009.
* @param year The numeric value (such as 2009
) used for
* comparing different dialects of MXML.
*/
protected MXMLDialect(String languageNamespace, int year)
{
this.languageNamespace = languageNamespace;
this.year = year;
// Names of language tags for builtin types.
arrayXMLName = new XMLName(languageNamespace, IMXMLLanguageConstants.ARRAY);
booleanXMLName = new XMLName(languageNamespace, IMXMLLanguageConstants.BOOLEAN);
classXMLName = new XMLName(languageNamespace, IMXMLLanguageConstants.CLASS);
dateXMLName = new XMLName(languageNamespace, IMXMLLanguageConstants.DATE);
functionXMLName = new XMLName(languageNamespace, IMXMLLanguageConstants.FUNCTION);
intXMLName = new XMLName(languageNamespace, IMXMLLanguageConstants.INT);
numberXMLName = new XMLName(languageNamespace, IMXMLLanguageConstants.NUMBER);
objectXMLName = new XMLName(languageNamespace, IMXMLLanguageConstants.OBJECT);
stringXMLName = new XMLName(languageNamespace, IMXMLLanguageConstants.STRING);
uintXMLName = new XMLName(languageNamespace, IMXMLLanguageConstants.UINT);
xmlXMLName = new XMLName(languageNamespace, IMXMLLanguageConstants.XML);
xmlListXMLName = new XMLName(languageNamespace, IMXMLLanguageConstants.XML_LIST);
}
private final String languageNamespace;
private final int year;
// Names of language tags that represent builtin ActionScript types.
private final XMLName arrayXMLName;
private final XMLName booleanXMLName;
private final XMLName classXMLName;
private final XMLName dateXMLName;
private final XMLName functionXMLName;
private final XMLName intXMLName;
private final XMLName numberXMLName;
private final XMLName objectXMLName;
private final XMLName stringXMLName;
private final XMLName uintXMLName;
private final XMLName xmlXMLName;
private final XMLName xmlListXMLName;
// Names of special language tags that don't represent builtiln ActionScript types.
protected XMLName bindingXMLName;
protected XMLName componentXMLName;
protected XMLName declarationsXMLName;
protected XMLName definitionXMLName;
protected XMLName libraryXMLName;
protected XMLName metadataXMLName;
protected XMLName modelXMLName;
protected XMLName privateXMLName;
protected XMLName reparentXMLName;
protected XMLName scriptXMLName;
protected XMLName styleXMLName;
/**
* Gets the language namespace for this dialect of MXML.
*
* For MXML 2009, for example, this is
* "http://ns.adobe.com/mxml/2009"
.
*
* @return The language namespace as a String.
*/
public String getLanguageNamespace()
{
return languageNamespace;
}
/**
* Determines whether this dialect is equal to, or later than, another
* dialect.
*
* @param other Another MXMLDialect
.
* @return true
= other.year;
}
/**
* Determines whether this dialect is equal to, or earlier than, another
* dialect.
*
* @param other Another MXMLDialect
.
* @return true
} tag in this dialect of MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveArray()
{
return arrayXMLName;
}
/**
* Gets the XML name of the {@code } tag in this dialect of MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveBinding()
{
return bindingXMLName;
}
/**
* Gets the XML name of the {@code } tag in this dialect of MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveBoolean()
{
return booleanXMLName;
}
/**
* Gets the XML name of the {@code } tag in this dialect of MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveClass()
{
return classXMLName;
}
/**
* Gets the XML name of the {@code } tag in this dialect of MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveComponent()
{
return componentXMLName;
}
/**
* Gets the XML name of the {@code } tag in this dialect of
* MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveDeclarations()
{
return declarationsXMLName;
}
/**
* Gets the XML name of the {@code } tag in this dialect of MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveDate()
{
return dateXMLName;
}
/**
* Gets the XML name of the {@code } tag in this dialect of
* MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveDefinition()
{
return definitionXMLName;
}
/**
* Gets the XML name of the {@code } tag in this dialect of MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveFunction()
{
return functionXMLName;
}
/**
* Gets the XML name of the {@code } tag in this dialect of MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveInt()
{
return intXMLName;
}
/**
* Gets the XML name of the {@code } tag in this dialect of MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveLibrary()
{
return libraryXMLName;
}
/**
* Gets the XML name of the {@code } tag in this dialect of MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveMetadata()
{
return metadataXMLName;
}
/**
* Gets the XML name of the {@code } tag in this dialect of MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveModel()
{
return modelXMLName;
}
/**
* Gets the XML name of the {@code } tag in this dialect of MXML.
*
* @return An {@link XMLName} or null
if this tag is not
* supported.
*/
public XMLName resolveNumber()
{
return numberXMLName;
}
/**
* Gets the XML name of the {@code