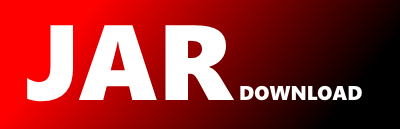
org.apache.royale.compiler.mxml.IMXMLLanguageConstants Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of compiler-common Show documentation
Show all versions of compiler-common Show documentation
The Apache Royale Compiler Common classes
The newest version!
/*
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.royale.compiler.mxml;
import org.apache.royale.compiler.constants.IASLanguageConstants;
/**
* Core MXML constants.
*/
public interface IMXMLLanguageConstants
{
//
// Language namespaces
//
/**
* The langauge namespace for MXML 2006.
*/
static final String NAMESPACE_MXML_2006 = "http://www.adobe.com/2006/mxml";
/**
* The language namespace for MXML 2009.
*/
static final String NAMESPACE_MXML_2009 = "http://ns.adobe.com/mxml/2009";
/**
* The language namespace for MXML 2012 (experimental).
*/
static final String NAMESPACE_MXML_2012 = "http://ns.adobe.com/mxml/2012";
//
// Short names of tags for builtin ActionScript types
//
/**
* The short name of the tag representing the ActionScript
* Array
type.
*/
static final String ARRAY = IASLanguageConstants.Array;
/**
* The short name of the tag representing the ActionScript
* Boolean
type.
*/
static final String BOOLEAN = IASLanguageConstants.Boolean;
/**
* The short name of the tag representing the ActionScript
* Class
type.
*/
static final String CLASS = IASLanguageConstants.Class;
/**
* The short name of the tag representing the ActionScript Date
* type.
*/
static final String DATE = IASLanguageConstants.Date;
/**
* The short name of the tag representing the ActionScript
* Function
type.
*/
static final String FUNCTION = IASLanguageConstants.Function;
/**
* The short name of the tag representing the ActionScript int
* type.
*/
static final String INT = IASLanguageConstants._int;
/**
* The short name of the tag representing the ActionScript
* Number
type.
*/
static final String NUMBER = IASLanguageConstants.Number;
/**
* The short name of the tag representing the ActionScript
* Object
type.
*/
static final String OBJECT = IASLanguageConstants.Object;
/**
* The short name of the tag representing the ActionScript uint
* type.
*/
static final String STRING = IASLanguageConstants.String;
/**
* The short name of the tag representing the ActionScript uint
* type.
*/
static final String UINT = IASLanguageConstants.uint;
/**
* The short name of the tag representing the ActionScript XML
* type.
*/
static final String XML = IASLanguageConstants.XML;
/**
* The short name of the tag representing the ActionScript
* XMLList
type.
*/
static final String XML_LIST = IASLanguageConstants.XMLList;
//
// Short names of special language tags
//
/**
* The short name of the special {@code } tag.
*/
static final String BINDING = "Binding";
/**
* The short name of the special {@code } tag.
*/
static final String COMPONENT = "Component";
/**
* The short name of the special {@code } tag.
*/
static final String DECLARATIONS = "Declarations";
/**
* The short name of the special {@code } tag.
*/
static final String DEFINITION = "Definition";
/**
* The short name of the special {@code } tag.
*/
static final String DESIGN_LAYER = "DesignLayer";
/**
* The short name of the special {@code } tag.
*/
static final String LIBRARY = "Library";
/**
* The short name of the special {@code } tag.
*/
static final String METADATA = "Metadata";
/**
* The short name of the special {@code } tag.
*/
static final String MODEL = "Model";
/**
* The short name of the special {@code } tag.
*/
static final String PRIVATE = "Private";
/**
* The short name of the special {@code } tag.
*/
static final String REPARENT = "Reparent";
/**
* The short name of the special {@code } tag.
*/
static final String REPEATER = "Repeater";
/**
* The short name of the special {@code