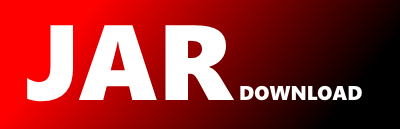
flex2.compiler.common.MetadataConfiguration Maven / Gradle / Ivy
/*
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package flex2.compiler.common;
import flex2.compiler.config.ConfigurationValue;
import flex2.compiler.config.ConfigurationInfo;
import java.util.Map;
import java.util.HashMap;
import java.util.Set;
import java.util.TreeSet;
import java.util.Iterator;
import java.util.Date;
import java.text.DateFormat;
/**
* This class handles metadata specific configuration options.
*
* @author Roger Gonzalez
*/
public class MetadataConfiguration
{
public boolean isSet()
{
return ((localizedTitles.size() > 0) || (localizedDescriptions.size() > 0) ||
(publishers.size() > 0) || (creators.size() > 0) || (contributors.size() > 0) || (langs.size() > 0) ||
(date != null));
}
private static String f( String in )
{
in = in.trim();
return ((in.indexOf('<') == -1) && (in.indexOf( '>') == -1))? in : ("");
}
public String toString()
{
if (!isSet())
{
return null;
}
StringBuilder sb = new StringBuilder();
sb.append("");
sb.append("");
sb.append("application/x-shockwave-flash ");
if (localizedTitles.size() > 0)
{
if ((localizedTitles.size() == 1) && (localizedTitles.get("x-default") != null))
{
sb.append("" + f(localizedTitles.get("x-default")) + " ");
}
else
{
sb.append("");
for (Iterator it = localizedTitles.entrySet().iterator(); it.hasNext();)
{
Map.Entry e = (Map.Entry) it.next();
sb.append("" + f((String) e.getValue()) + " ");
}
sb.append(" ");
}
}
if (localizedDescriptions.size() > 0)
{
if ((localizedDescriptions.size() == 1) && (localizedDescriptions.get("x-default") != null))
{
sb.append("" + f(localizedDescriptions.get("x-default")) + " ");
}
else
{
sb.append("");
for (Iterator it = localizedDescriptions.entrySet().iterator(); it.hasNext();)
{
Map.Entry e = (Map.Entry) it.next();
sb.append("" + f((String) e.getValue()) + " ");
}
sb.append(" ");
}
}
// FIXME - I suspect we need rdf:Bag for these when there are more than one? --rg
for (Iterator it = publishers.iterator(); it.hasNext();)
{
sb.append("" + f(it.next()) + " ");
}
for (Iterator it = creators.iterator(); it.hasNext();)
{
sb.append("" + f(it.next()) + " ");
}
for (Iterator it = contributors.iterator(); it.hasNext();)
{
sb.append("" + f(it.next()) + " ");
}
for (Iterator it = langs.iterator(); it.hasNext();)
{
sb.append("" + f(it.next()) + " ");
}
if (date == null)
{
date = DateFormat.getDateInstance().format(new Date());
}
sb.append("" + f(date) + " ");
sb.append(" ");
return sb.toString();
}
//
// 'metadata.contributor' option
//
private Set contributors = new TreeSet();
public void cfgContributor( ConfigurationValue cv, String name )
{
contributors.add( name );
}
public static ConfigurationInfo getContributorInfo()
{
return new ConfigurationInfo( 1, "name" )
{
public boolean isAdvanced()
{
return false;
}
public boolean allowMultiple()
{
return true;
}
};
}
//
// 'metadata.creator' option
//
private Set creators = new TreeSet();
public void cfgCreator( ConfigurationValue cv, String name )
{
creators.add( name );
}
public static ConfigurationInfo getCreatorInfo()
{
return new ConfigurationInfo( 1, "name" )
{
public boolean isAdvanced()
{
return false;
}
public boolean allowMultiple()
{
return true;
}
};
}
//
// 'metadata.date' option
//
public String date = null;
public void cfgDate( ConfigurationValue cv, String text)
{
date = text;
}
public static ConfigurationInfo getDateInfo()
{
return new ConfigurationInfo( 1, "text" )
{
public boolean isAdvanced()
{
return false;
}
};
}
//
// 'metadata.description' option
//
private Map localizedDescriptions = new HashMap();
public void cfgDescription( ConfigurationValue cv, String text )
{
localizedDescriptions.put( "x-default", text );
}
public static ConfigurationInfo getDescriptionInfo()
{
return new ConfigurationInfo( 1, "text" )
{
public boolean isAdvanced()
{
return false;
}
};
}
//
// 'metadata.language' option
//
public TreeSet langs = new TreeSet();
public void cfgLanguage( ConfigurationValue cv, String code )
{
langs.add( code );
}
public static ConfigurationInfo getLanguageInfo()
{
return new ConfigurationInfo( 1, "code" )
{
public boolean isAdvanced()
{
return false;
}
public boolean allowMultiple()
{
return true;
}
};
}
//
// 'metadata.localized-description' option
//
public void cfgLocalizedDescription( ConfigurationValue cv, String text, String lang )
{
localizedDescriptions.put( lang, text );
}
public static ConfigurationInfo getLocalizedDescriptionInfo()
{
return new ConfigurationInfo( 2, new String[] {"text", "lang"} )
{
public boolean isAdvanced()
{
return false;
}
public boolean allowMultiple()
{
return true;
}
};
}
//
// 'metadata.localized-title' option
//
public void cfgLocalizedTitle( ConfigurationValue cv, String title, String lang )
{
localizedTitles.put( lang, title );
}
public static ConfigurationInfo getLocalizedTitleInfo()
{
return new ConfigurationInfo( 2, new String[] {"title", "lang"} )
{
public boolean isAdvanced()
{
return false;
}
public boolean allowMultiple()
{
return true;
}
};
}
//
// 'metadata.publisher' option
//
private Set publishers = new TreeSet();
public void cfgPublisher( ConfigurationValue cv, String name )
{
publishers.add( name );
}
public static ConfigurationInfo getPublisherInfo()
{
return new ConfigurationInfo( 1, "name" )
{
public boolean isAdvanced()
{
return false;
}
public boolean allowMultiple()
{
return true;
}
};
}
//
// 'metadata.title' option
//
private Map localizedTitles = new HashMap();
public void cfgTitle( ConfigurationValue cv, String title )
{
localizedTitles.put( "x-default", title );
}
public static ConfigurationInfo getTitleInfo()
{
return new ConfigurationInfo( 1, "text" )
{
public boolean isAdvanced()
{
return false;
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy