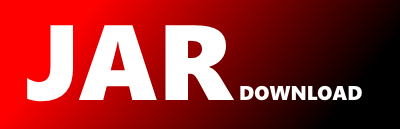
flex2.tools.oem.Report Maven / Gradle / Ivy
/*
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package flex2.tools.oem;
import java.io.IOException;
import java.io.Writer;
/**
* The Report
interface provides information about the composition
* of an Application
or a Library
.
*
* @see flex2.tools.oem.Builder#getReport()
* @version 2.0.1
* @author Clement Wong
*/
public interface Report
{
/**
* Use this constant when querying information from the compiler.
*/
Object COMPILER = "COMPILER";
/**
* Use this constant when querying information from the linker.
*/
Object LINKER = "LINKER";
/**
* Gets the name of all the sources that are involved in the Application
or Library
.
* The getSourceNames(Report.COMPILER)
method returns the name of all the source files
* that are involved in the compilation.
*
*
* The getSourceNames(Report.LINKER)
method returns the name of all the source files
* that are eventually output by the linker.
*
*
* The getSourceNames(Report.COMPILER)
* and getSourceNames(Report.LINKER)
methods can yield different results if the linker is
* instructed to exclude certain definitions from the final output.
*
* @see #COMPILER
* @see #LINKER
*
* @param report The COMPILER
or LINKER
.
*
* @return A list of source names.
*/
String[] getSourceNames(Object report);
/**
* Gets the names of all the assets that are involved in the Application
or Library
.
* The getAssetNames(Report.COMPILER)
method returns the names of all the asset files
* that are involved in the compilation.
*
*
* The getAssetNames(Report.LINKER)
method returns the names of all the asset files
* that are eventually output by the linker.
*
*
* The getAssetNames(Report.COMPILER)
* and getAssetNames(Report.LINKER)
methods can yield different results if the linker is
* instructed to exclude certain definitions from the final output.
*
* @see #COMPILER
* @see #LINKER
*
* @param report The COMPILER
or LINKER
.
*
* @return A list of asset names.
*/
String[] getAssetNames(Object report);
/**
* Gets the names of all the assets that are in the specified frame.
* The number of frames in the movie can be obtained by invoking getFrameCount()
.
*
*
* If the compilation did not generate a movie, this method returns null
.
*
* @see #getFrameCount()
* @param frame frame number. The number is 1-based.
* @return an array of asset file names
*/
String[] getAssetNames(int frame);
/**
* Gets the name of all the libraries that are involved in the Application
or Library
.
* The getLibraryNames(Report.COMPILER)
method returns the name of all the library files
* that are involved in the compilation.
*
*
* The getLibraryNames(Report.LINKER)
method returns the name of all the library files
* that are eventually output by the linker.
*
*
* The getLibraryNames(Report.COMPILER)
* and getLibraryNames(Report.LINKER)
methods can yield different results if the linker is
* instructed to exclude certain definitions from the final output.
*
* @see #COMPILER
* @see #LINKER
*
* @param report The COMPILER
or LINKER
.
*
* @return A list of library names.
*/
String[] getLibraryNames(Object report);
/**
* Gets the name of all the resource bundles that are involved in the Application/Library.
* The getResourceBundleNames()
method returns a list of names that
* can be passed to the Library.addResourceBundle()
method.
*
*
* The returned value should match the output from the resource-bundle-list
compiler option.
*
* @return A list of resource bundle names.
*/
String[] getResourceBundleNames();
/**
* Gets the list of all the top-level, externally-visible definitions in the specified
* source file. You can get the specified source file from the getSourceNames()
method.
*
*
* The output definition names are in the QName format; for example: mx.controls:Button
.
*
* @param sourceName Source file name.
* @return An array of definition names; null
if there is no definition in the source file.
*/
String[] getDefinitionNames(String sourceName);
/**
* Gets the list of all the top-level, externally-visible definitions in the specified frame.
* The sequence represents the order in which the definitions are exported to the frame.
* The number of frames in the movie can be obtained by invoking getFrameCount()
.
*
*
* If the compilation did not generate a movie, this method returns null
.
*
* @see #getFrameCount()
* @param frame frame number. The number is 1-based.
* @return an array of definition names
*/
String[] getDefinitionNames(int frame);
/**
* Gets the location of the specified definition.
*
*
* The specified definition name must be in the QName format; for example: mx.controls:Button
.
*
* @param definition A definition is a class, function, variable, or namespace.
* @return The location of the specified definition; null
if the definition is not found.
*/
String getLocation(String definition);
/**
* Gets the list of definitions that the specified definition depends on during initialization.
*
*
* The specified definition name must be in the QName format; for example: mx.controls:Button
.
*
* @param definition A class.
*
* @return An array of definition names; null
if there is no dependency.
*/
String[] getPrerequisites(String definition);
/**
* Gets the list of definitions that the specified definition depends on during run time.
*
*
* The specified definition name must be in the QName format; for example: mx.controls:Button
.
*
* @param definition A definition is a class, function, variable, or namespace.
*
* @return An array of definition names; null
if there is no dependency.
*/
String[] getDependencies(String definition);
/**
* Writes the linker report to the specified output. If this Report
was generated before linking,
* this method returns 0
. You should provide a BufferedWriter
, if possible.
* You should be sure to close the specified Writer
.
*
*
* To use this method, you must call the Configuration.keepLinkReport()
method
* before the compilation.
*
* @param out An instance of Writer
.
*
* @return The number of characters written out.
*
* @throws IOException Thrown when an I/O error occurs while the link report is being written.
*
* @see flex2.tools.oem.Configuration#keepLinkReport(boolean)
*/
long writeLinkReport(Writer out) throws IOException;
/**
* Writes the linker size report to the specified output. If this Report
was generated before linking,
* this method returns 0
. You should provide a BufferedWriter
, if possible.
* You should be sure to close the specified Writer
.
*
*
* To use this method, you must call the Configuration.keepSizeReport()
method
* before the compilation.
*
* @param out An instance of Writer
.
*
* @return The number of characters written out.
*
* @throws IOException Thrown when an I/O error occurs while the link report is being written.
*
* @see flex2.tools.oem.Configuration#keepSizeReport(boolean)
*/
long writeSizeReport(Writer out) throws IOException;
/**
* Writes the configuration report to the specified output.
* You should provide a BufferedWriter
, if possible.
* Be sure to close the specified Writer
.
*
*
* To use this method, you must call the Configuration.keepConfigurationReport()
method
* before the compilation.
*
* @param out An instance of Writer
.
*
* @return The number of characters written out.
*
* @throws IOException Thrown when an I/O error occurs during writing the configuration report.
*
* @see flex2.tools.oem.Configuration#keepConfigurationReport(boolean)
*/
long writeConfigurationReport(Writer out) throws IOException;
/**
* Gets the background color. The default value is 0x869CA7
.
* If the Report
was generated before linking, this method returns 0
.
*
* @return An RGB value.
*/
int getBackgroundColor();
/**
* Gets the page title.
* If the Report
was generated before linking, this method returns null
.
*
* @return Page title; null
if it was not specified.
*/
String getPageTitle();
/**
* Gets the default width of the application. The default value is 500
.
*
* @return The default width, in pixels.
*/
int getDefaultWidth();
/**
* Gets the default height of the application. The default value is 375
.
*
* @return The default height, in pixels.
*/
int getDefaultHeight();
/**
* Gets the user-defined width.
* If the Report
was generated before linking, this method returns 0
.
*
* @return Width of the application, in pixels; 0
if it was not specified.
*/
int getWidth();
/**
* Gets the user-defined height.
* If the Report
was generated before linking, this method returns 0
.
*
* @return Height, in pixels; 0
if it was not specified.
*/
int getHeight();
/**
* Gets the user-defined width percentage.
* If the Report
was generated before linking, this method returns 0.0
.
*
* @return Width percentage; 0.0
if it was not specified.
*/
double getWidthPercent();
/**
* Gets the user-defined height percentage.
* If the Report
was generated before linking, this method returns 0.0
.
*
* @return Height percentage; 0.0
if it was not specified.
*/
double getHeightPercent();
/**
* Outputs the compiler version.
*
* @return A string representing the compiler version.
*/
String getCompilerVersion();
/**
* Reports the errors and warnings that were generated during the compilation. The returned
* Message
objects are errors and warnings.
*
* @return An array of error and warning Message objects; null
if there were no errors or warnings.
*/
Message[] getMessages();
/**
* Gets the number of frames in the movie. For Application
, the returned
* value is the number of frames in the movie. For Library
, the returned
* value is the number of frames in library.swf.
*
*
* If the compilation did not generate a movie, the returned value will be 0
.
*
* @return number of frames
*/
int getFrameCount();
/**
* Checks whether the sources, assets and libraries have been updated since the report was created.
*
* @since 3.0
* @return
*/
boolean contentUpdated();
}