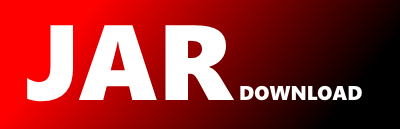
flex2.tools.oem.VirtualLocalFileSystem Maven / Gradle / Ivy
/*
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package flex2.tools.oem;
import java.io.File;
import java.util.HashMap;
import java.util.Map;
import flex2.compiler.io.FileUtil;
import flex2.compiler.io.LocalFile;
import flex2.compiler.io.VirtualFile;
/**
* The VirtualLocalFileSystem
class serves as a factory for creating VirtualLocalFile
instances.
* It also helps VirtualLocalFile
instances resolve relative paths that might point to other
* VirtualLocalFile
instances or "real" files in the file system.
*
* @see flex2.tools.oem.VirtualLocalFile
* @version 2.0.1
* @author Clement Wong
*/
public class VirtualLocalFileSystem
{
/**
* Constructor.
*/
public VirtualLocalFileSystem()
{
files = new HashMap();
}
private final Map files;
/**
* Creates a VirtualLocalFile
instance.
*
* @param name A canonical path. The name must end with a file extension; for example: .mxml
or .as
.
* @param text Source code.
* @param parent The parent directory of this VirtualLocalFile
object.
* @param lastModified The last modified time for the virtual local file.
* @return A VirtualLocalFile
.
*/
public final VirtualLocalFile create(String name, String text, File parent, long lastModified)
{
VirtualLocalFile f = new VirtualLocalFile(name, text, parent, lastModified, this);
files.put(name, f);
return f;
}
/**
* Updates a VirtualLocalFile
with the specified text and timestamp.
*
* @param name A canonical path. The name must end with a file extension; for example: .mxml
or .as
.
* @param text Source code.
* @param lastModified The last modified time.
* @return true
if the VirtualLocalFile
was successfully updated; false
if a
* VirtualLocalFile
was not found.
*/
public final boolean update(String name, String text, long lastModified)
{
VirtualLocalFile f = files.get(name);
if (f != null)
{
f.text = text;
f.lastModified = lastModified;
return true;
}
else
{
return false;
}
}
final VirtualFile resolve(VirtualLocalFile base, String name)
{
// if 'name' is a full name and the VirtualFile instance exists, return it.
VirtualLocalFile f = files.get(name);
if (f != null)
{
return f;
}
// if 'name' is relative to 'base', resolve 'name' into a full name.
String fullName = constructName(base, name);
// try to lookup again.
f = files.get(fullName);
if (f != null)
{
return f;
}
// it's not in the HashMap. let's locate it in the file system.
File absolute = FileUtil.openFile(name);
if (absolute != null && absolute.exists())
{
return new LocalFile(absolute);
}
File relative = FileUtil.openFile(fullName);
if (relative != null && relative.exists())
{
return new LocalFile(relative);
}
return null;
}
private String constructName(VirtualLocalFile base, String relativeName)
{
return FileUtil.getCanonicalPath(FileUtil.openFile(base.getParent() + File.separator + relativeName));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy