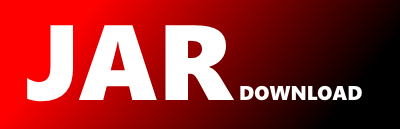
static.console-fe.src.pages.TransactionInfo.TransactionInfo.tsx Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of seata-console Show documentation
Show all versions of seata-console Show documentation
console for Seata built with Maven
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import React from 'react';
import { ConfigProvider, Table, Button, DatePicker, Form, Icon, Switch, Pagination, Dialog, Input, Select } from '@alicloud/console-components';
import Actions, { LinkButton } from '@alicloud/console-components-actions';
import { withRouter } from 'react-router-dom';
import Page from '@/components/Page';
import { GlobalProps } from '@/module';
import styled, { css } from 'styled-components';
import getData, { GlobalSessionParam } from '@/service/transactionInfo';
import PropTypes from 'prop-types';
import moment from 'moment';
import './index.scss';
const { RangePicker } = DatePicker;
const FormItem = Form.Item;
type StatusType = {
label: string,
value: number,
iconType: string,
iconColor: string,
}
type TransactionInfoState = {
list: Array;
total: number;
loading: boolean;
branchSessionDialogVisible: boolean;
currentBranchSession: Array;
globalSessionParam : GlobalSessionParam;
}
const statusList:Array = [
{
label: 'AsyncCommitting',
value: 8,
iconType: 'ellipsis',
iconColor: 'rgb(3, 193, 253)',
},
{
label: 'Begin',
value: 1,
iconType: 'ellipsis',
iconColor: 'rgb(3, 193, 253)',
},
{
label: 'Committing',
value: 2,
iconType: 'ellipsis',
iconColor: 'rgb(3, 193, 253)',
},
{
label: 'CommitRetrying',
value: 3,
iconType: 'ellipsis',
iconColor: 'rgb(3, 193, 253)',
},
{
label: 'Committed',
value: 9,
iconType: 'success',
iconColor: '#1DC11D',
},
{
label: 'CommitFailed',
value: 10,
iconType: 'error',
iconColor: '#FF3333',
},
{
label: 'CommitRetryTimeout',
value: 16,
iconType: 'error',
iconColor: '#FF3333',
},
{
label: 'Finished',
value: 15,
iconType: 'success',
iconColor: '#1DC11D',
},
{
label: 'Rollbacking',
value: 4,
iconType: 'ellipsis',
iconColor: 'rgb(3, 193, 253)',
},
{
label: 'RollbackRetrying',
value: 5,
iconType: 'ellipsis',
iconColor: 'rgb(3, 193, 253)',
},
{
label: 'Rollbacked',
value: 11,
iconType: 'error',
iconColor: '#FF3333',
},
{
label: 'RollbackFailed',
value: 12,
iconType: 'error',
iconColor: '#FF3333',
},
{
label: 'RollbackRetryTimeout',
value: 17,
iconType: 'error',
iconColor: '#FF3333',
},
{
label: 'TimeoutRollbacking',
value: 6,
iconType: 'ellipsis',
iconColor: 'rgb(3, 193, 253)',
},
{
label: 'TimeoutRollbackRetrying',
value: 7,
iconType: 'ellipsis',
iconColor: 'rgb(3, 193, 253)',
},
{
label: 'TimeoutRollbacked',
value: 13,
iconType: 'error',
iconColor: '#FF3333',
},
{
label: 'TimeoutRollbackFailed',
value: 14,
iconType: 'error',
iconColor: '#FF3333',
},
{
label: 'UnKnown',
value: 0,
iconType: 'warning',
iconColor: '#FFA003',
},
];
const branchSessionStatusList:Array = [
{
label: 'UnKnown',
value: 0,
iconType: 'warning',
iconColor: '#FFA003',
},
{
label: 'Registered',
value: 1,
iconType: 'ellipsis',
iconColor: 'rgb(3, 193, 253)',
},
{
label: 'PhaseOne_Done',
value: 2,
iconType: 'ellipsis',
iconColor: 'rgb(3, 193, 253)',
},
{
label: 'PhaseOne_Failed',
value: 3,
iconType: 'error',
iconColor: '#FF3333',
},
{
label: 'PhaseOne_Timeout',
value: 4,
iconType: 'error',
iconColor: '#FF3333',
},
{
label: 'PhaseTwo_Committed',
value: 5,
iconType: 'success',
iconColor: '#1DC11D',
},
{
label: 'PhaseTwo_CommitFailed_Retryable',
value: 6,
iconType: 'ellipsis',
iconColor: 'rgb(3, 193, 253)',
},
{
label: 'PhaseTwo_CommitFailed_Unretryable',
value: 7,
iconType: 'error',
iconColor: '#FF3333',
},
{
label: 'PhaseTwo_Rollbacked',
value: 8,
iconType: 'error',
iconColor: '#FF3333',
},
{
label: 'PhaseTwo_RollbackFailed_Retryable',
value: 9,
iconType: 'ellipsis',
iconColor: 'rgb(3, 193, 253)',
},
{
label: 'PhaseTwo_RollbackFailed_Unretryable',
value: 10,
iconType: 'error',
iconColor: '#FF3333',
},
{
label: 'PhaseOne_RDONLY',
value: 13,
iconType: 'ellipsis',
iconColor: 'rgb(3, 193, 253)',
},
];
class TransactionInfo extends React.Component {
static displayName = 'TransactionInfo';
static propTypes = {
locale: PropTypes.object,
history: PropTypes.object,
};
state: TransactionInfoState = {
list: [],
total: 0,
loading: false,
branchSessionDialogVisible: false,
currentBranchSession: [],
globalSessionParam: {
withBranch: false,
pageSize: 10,
pageNum: 1,
},
};
componentDidMount = () => {
// search once by default
this.search();
}
resetSearchFilter = () => {
this.setState({
globalSessionParam: {
withBranch: false,
// pagination info don`t reset
pageSize: this.state.globalSessionParam.pageSize,
pageNum: this.state.globalSessionParam.pageNum,
},
});
}
search = () => {
this.setState({ loading: true });
getData(this.state.globalSessionParam).then(data => {
// if the result set is empty, set the page number to go back to the first page
if (data.total === 0) {
this.setState({
list: [],
total: 0,
loading: false,
globalSessionParam: Object.assign(this.state.globalSessionParam,
{ pageNum: 1 }),
});
return;
}
// format time
data.data.forEach((element: any) => {
element.beginTime = (element.beginTime == null || element.beginTime === '') ? null : moment(Number(element.beginTime)).format('YYYY-MM-DD HH:mm:ss');
});
this.setState({
list: data.data,
total: data.total,
loading: false,
});
}).catch(err => {
this.setState({ loading: false });
});
}
searchFilterOnChange = (key:string, val:string) => {
this.setState({
globalSessionParam: Object.assign(this.state.globalSessionParam,
{ [key]: val }),
});
}
branchSessionSwitchOnChange = (checked: boolean, e: any) => {
this.setState({
globalSessionParam: Object.assign(this.state.globalSessionParam,
{ withBranch: checked }),
});
if (checked) {
// if checked, do search for load branch sessions
this.search();
}
}
createTimeOnChange = (value: Array) => {
// timestamp(milliseconds)
const timeStart = value[0] == null ? null : moment(value[0]).unix() * 1000;
const timeEnd = value[1] == null ? null : moment(value[1]).unix() * 1000;
this.setState({
globalSessionParam: Object.assign(this.state.globalSessionParam,
{ timeStart, timeEnd }),
});
}
statusCell = (val: number, index: number, record: any) => {
let icon;
statusList.forEach((status: StatusType) => {
if (status.value === val) {
icon = (
{status.label}
);
}
});
// Unmatched
if (icon === undefined) {
icon = ({val});
}
return icon;
}
branchSessionStatusCell = (val: number, index: number, record: any) => {
let icon;
branchSessionStatusList.forEach((status: StatusType) => {
if (status.value === val) {
icon = (
{status.label}
);
}
});
// Unmatched
if (icon === undefined) {
icon = ({val});
}
return icon;
}
operateCell = (val: string, index: number, record: any) => {
const { locale = {}, history } = this.props;
const {
showBranchSessionTitle,
showGlobalLockTitle,
} = locale;
return (
{/* {when withBranch false, hide 'View branch session' button} */}
{this.state.globalSessionParam.withBranch ? (
{showBranchSessionTitle}
) : null}
{
history.push({
pathname: '/globallock/list',
// @ts-ignore
query: { xid: record.xid },
});
}}
>
{showGlobalLockTitle}
);
}
branchSessionDialogOperateCell = (val: string, index: number, record: any) => {
const { locale = {}, history } = this.props;
const {
showGlobalLockTitle,
} = locale;
return (
{
history.push({
pathname: '/globallock/list',
// @ts-ignore
query: { xid: record.xid },
});
}}
>
{showGlobalLockTitle}
);
}
paginationOnChange = (current: number, e: {}) => {
this.setState({
globalSessionParam: Object.assign(this.state.globalSessionParam,
{ pageNum: current }),
});
this.search();
}
paginationOnPageSizeChange = (pageSize: number) => {
this.setState({
globalSessionParam: Object.assign(this.state.globalSessionParam,
{ pageSize }),
});
this.search();
}
showBranchSessionDialog = (val: string, index: number, record: any) => () => {
this.setState({
branchSessionDialogVisible: true,
currentBranchSession: record.branchSessionVOs,
});
}
closeBranchSessionDialog = () => {
this.setState({
branchSessionDialogVisible: false,
currentBranchSession: [],
});
}
render() {
const { locale = {} } = this.props;
const { title, subTitle, createTimeLabel,
selectFilerPlaceholder,
inputFilterPlaceholder,
branchSessionSwitchLabel,
resetButtonLabel,
searchButtonLabel,
operateTitle,
branchSessionDialogTitle,
} = locale;
return (
{/* search form */}
{/* global session table */}
{/* branch session dialog */}
);
}
}
export default withRouter(ConfigProvider.config(TransactionInfo, {}));
© 2015 - 2024 Weber Informatics LLC | Privacy Policy