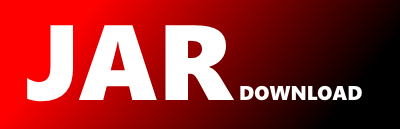
redis.clients.jedis.commands.HashBinaryCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.apache.servicemix.bundles.jedis
Show all versions of org.apache.servicemix.bundles.jedis
This OSGi bundle wraps the ${pkgArtifactId} ${pkgVersion} jar file.
The newest version!
package redis.clients.jedis.commands;
import java.util.List;
import java.util.Map;
import java.util.Set;
import redis.clients.jedis.args.ExpiryOption;
import redis.clients.jedis.params.ScanParams;
import redis.clients.jedis.resps.ScanResult;
public interface HashBinaryCommands {
long hset(byte[] key, byte[] field, byte[] value);
long hset(byte[] key, Map hash);
byte[] hget(byte[] key, byte[] field);
long hsetnx(byte[] key, byte[] field, byte[] value);
String hmset(byte[] key, Map hash);
List hmget(byte[] key, byte[]... fields);
long hincrBy(byte[] key, byte[] field, long value);
double hincrByFloat(byte[] key, byte[] field, double value);
boolean hexists(byte[] key, byte[] field);
long hdel(byte[] key, byte[]... field);
long hlen(byte[] key);
Set hkeys(byte[] key);
List hvals(byte[] key);
Map hgetAll(byte[] key);
byte[] hrandfield(byte[] key);
List hrandfield(byte[] key, long count);
List> hrandfieldWithValues(byte[] key, long count);
default ScanResult> hscan(byte[] key, byte[] cursor) {
return hscan(key, cursor, new ScanParams());
}
ScanResult> hscan(byte[] key, byte[] cursor, ScanParams params);
default ScanResult hscanNoValues(byte[] key, byte[] cursor) {
return hscanNoValues(key, cursor, new ScanParams());
}
ScanResult hscanNoValues(byte[] key, byte[] cursor, ScanParams params);
long hstrlen(byte[] key, byte[] field);
/**
* Set expiry for hash field using relative time to expire (seconds).
*
* @param key hash
* @param seconds time to expire
* @param fields
* @return integer-reply: 1 if the timeout was set, 0 otherwise
*/
List hexpire(byte[] key, long seconds, byte[]... fields);
/**
* Set expiry for hash field using relative time to expire (seconds).
*
* @param key hash
* @param seconds time to expire
* @param condition can be NX, XX, GT or LT
* @param fields
* @return integer-reply: 1 if the timeout was set, 0 otherwise
*/
List hexpire(byte[] key, long seconds, ExpiryOption condition, byte[]... fields);
/**
* Set expiry for hash field using relative time to expire (milliseconds).
*
* @param key hash
* @param milliseconds time to expire
* @param fields
* @return integer-reply: 1 if the timeout was set, 0 otherwise
*/
List hpexpire(byte[] key, long milliseconds, byte[]... fields);
/**
* Set expiry for hash field using relative time to expire (milliseconds).
*
* @param key hash
* @param milliseconds time to expire
* @param condition can be NX, XX, GT or LT
* @param fields
* @return integer-reply: 1 if the timeout was set, 0 otherwise
*/
List hpexpire(byte[] key, long milliseconds, ExpiryOption condition, byte[]... fields);
/**
* Set expiry for hash field using an absolute Unix timestamp (seconds).
*
* @param key hash
* @param unixTimeSeconds time to expire
* @param fields
* @return integer-reply: 1 if the timeout was set, 0 otherwise
*/
List hexpireAt(byte[] key, long unixTimeSeconds, byte[]... fields);
/**
* Set expiry for hash field using an absolute Unix timestamp (seconds).
*
* @param key hash
* @param unixTimeSeconds time to expire
* @param condition can be NX, XX, GT or LT
* @param fields
* @return integer-reply: 1 if the timeout was set, 0 otherwise
*/
List hexpireAt(byte[] key, long unixTimeSeconds, ExpiryOption condition, byte[]... fields);
/**
* Set expiry for hash field using an absolute Unix timestamp (milliseconds).
*
* @param key hash
* @param unixTimeMillis time to expire
* @param fields
* @return integer-reply: 1 if the timeout was set, 0 otherwise
*/
List hpexpireAt(byte[] key, long unixTimeMillis, byte[]... fields);
/**
* Set expiry for hash field using an absolute Unix timestamp (milliseconds).
*
* @param key hash
* @param unixTimeMillis time to expire
* @param condition can be NX, XX, GT or LT
* @param fields
* @return integer-reply: 1 if the timeout was set, 0 otherwise
*/
List hpexpireAt(byte[] key, long unixTimeMillis, ExpiryOption condition, byte[]... fields);
/**
* Returns the expiration time of a hash field as a Unix timestamp, in seconds.
*
* @param key hash
* @param fields
* @return Expiration Unix timestamp in seconds;
* or -1 if the field exists but has no associated expire or -2 if the field does not exist.
*/
List hexpireTime(byte[] key, byte[]... fields);
/**
* Returns the expiration time of a hash field as a Unix timestamp, in milliseconds.
*
* @param key hash
* @param fields
* @return Expiration Unix timestamp in milliseconds;
* or -1 if the field exists but has no associated expire or -2 if the field does not exist.
*/
List hpexpireTime(byte[] key, byte[]... fields);
/**
* Returns the TTL in seconds of a hash field.
*
* @param key hash
* @param fields
* @return TTL in seconds;
* or -1 if the field exists but has no associated expire or -2 if the field does not exist.
*/
List httl(byte[] key, byte[]... fields);
/**
* Returns the TTL in milliseconds of a hash field.
*
* @param key hash
* @param fields
* @return TTL in milliseconds;
* or -1 if the field exists but has no associated expire or -2 if the field does not exist.
*/
List hpttl(byte[] key, byte[]... fields);
/**
* Removes the expiration time for each specified field.
*
* @param key hash
* @param fields
* @return integer-reply: 1 if the expiration time was removed,
* or -1 if the field exists but has no associated expire or -2 if the field does not exist.
*/
List hpersist(byte[] key, byte[]... fields);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy