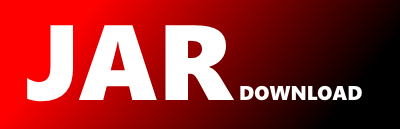
redis.clients.jedis.csc.CacheKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.apache.servicemix.bundles.jedis
Show all versions of org.apache.servicemix.bundles.jedis
This OSGi bundle wraps the ${pkgArtifactId} ${pkgVersion} jar file.
The newest version!
package redis.clients.jedis.csc;
import java.util.List;
import java.util.Objects;
import redis.clients.jedis.CommandObject;
import redis.clients.jedis.commands.ProtocolCommand;
public class CacheKey {
private final CommandObject command;
public CacheKey(CommandObject command) {
this.command = Objects.requireNonNull(command);
}
@Override
public int hashCode() {
return command.hashCode();
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
final CacheKey other = (CacheKey) obj;
return Objects.equals(this.command, other.command);
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy