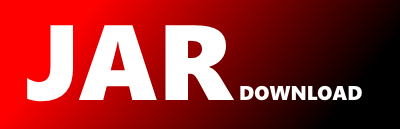
org.mozilla.javascript.Hashtable Maven / Gradle / Ivy
Show all versions of org.apache.servicemix.bundles.rhino
/* -*- Mode: java; tab-width: 8; indent-tabs-mode: nil; c-basic-offset: 4 -*-
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
package org.mozilla.javascript;
import java.io.Serializable;
import java.math.BigInteger;
import java.util.HashMap;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* This generic hash table class is used by Set and Map. It uses a standard HashMap for storing keys
* and values so that we can handle lots of hash collisions if necessary, and a doubly-linked list
* to support the iterator capability.
*
* This second one is important because JavaScript handling of the iterator is completely
* different from the way that Java does it. In Java an attempt to modify a collection on a HashMap
* or LinkedHashMap while iterating through it (except by using the "remove" method on the Iterator
* object itself) results in a ConcurrentModificationException. JavaScript Maps and Sets explicitly
* allow the collection to be modified, or even cleared completely, while iterators exist, and even
* lets an iterator keep on iterating on a collection that was empty when it was created..
*/
public class Hashtable implements Serializable, Iterable {
private static final long serialVersionUID = -7151554912419543747L;
private final HashMap