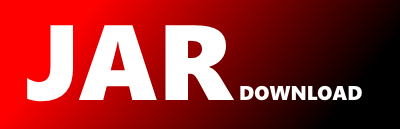
org.apache.shardingsphere.distsql.parser.autogen.ReadwriteSplittingDistSQLStatementParser Maven / Gradle / Ivy
// Generated from org/apache/shardingsphere/distsql/parser/autogen/ReadwriteSplittingDistSQLStatement.g4 by ANTLR 4.9.2
package org.apache.shardingsphere.distsql.parser.autogen;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class ReadwriteSplittingDistSQLStatementParser extends Parser {
static { RuntimeMetaData.checkVersion("4.9.2", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
AND=1, OR=2, NOT=3, TILDE=4, VERTICALBAR=5, AMPERSAND=6, SIGNEDLEFTSHIFT=7,
SIGNEDRIGHTSHIFT=8, CARET=9, MOD=10, COLON=11, PLUS=12, MINUS=13, ASTERISK=14,
SLASH=15, BACKSLASH=16, DOT=17, DOTASTERISK=18, SAFEEQ=19, DEQ=20, EQ=21,
NEQ=22, GT=23, GTE=24, LT=25, LTE=26, POUND=27, LP=28, RP=29, LBE=30,
RBE=31, LBT=32, RBT=33, COMMA=34, DQ=35, SQ=36, BQ=37, QUESTION=38, AT=39,
SEMI=40, JSONSEPARATOR=41, UL=42, WS=43, CREATE=44, ALTER=45, DROP=46,
SET=47, SHOW=48, RULE=49, FROM=50, READWRITE_SPLITTING=51, WRITE_RESOURCE=52,
READ_RESOURCES=53, AUTO_AWARE_RESOURCE=54, TYPE=55, NAME=56, PROPERTIES=57,
RULES=58, SOURCE=59, RESOURCES=60, STATUS=61, HINT=62, CLEAR=63, ENABLE=64,
DISABLE=65, READ=66, FOR_GENERATOR=67, IDENTIFIER=68, STRING=69, INT=70,
HEX=71, NUMBER=72, HEXDIGIT=73, BITNUM=74;
public static final int
RULE_execute = 0, RULE_setReadwriteSplittingHintSource = 1, RULE_showReadwriteSplittingHintStatus = 2,
RULE_clearReadwriteSplittingHint = 3, RULE_enableReadDataSource = 4, RULE_disableReadDataSource = 5,
RULE_showReadwriteSplittingReadResources = 6, RULE_sourceValue = 7, RULE_resourceName = 8,
RULE_schemaName = 9, RULE_createReadwriteSplittingRule = 10, RULE_alterReadwriteSplittingRule = 11,
RULE_dropReadwriteSplittingRule = 12, RULE_readwriteSplittingRuleDefinition = 13,
RULE_staticReadwriteSplittingRuleDefinition = 14, RULE_dynamicReadwriteSplittingRuleDefinition = 15,
RULE_ruleName = 16, RULE_writeResourceName = 17, RULE_readResourceNames = 18,
RULE_algorithmDefinition = 19, RULE_algorithmName = 20, RULE_algorithmProperties = 21,
RULE_algorithmProperty = 22, RULE_showReadwriteSplittingRules = 23;
private static String[] makeRuleNames() {
return new String[] {
"execute", "setReadwriteSplittingHintSource", "showReadwriteSplittingHintStatus",
"clearReadwriteSplittingHint", "enableReadDataSource", "disableReadDataSource",
"showReadwriteSplittingReadResources", "sourceValue", "resourceName",
"schemaName", "createReadwriteSplittingRule", "alterReadwriteSplittingRule",
"dropReadwriteSplittingRule", "readwriteSplittingRuleDefinition", "staticReadwriteSplittingRuleDefinition",
"dynamicReadwriteSplittingRuleDefinition", "ruleName", "writeResourceName",
"readResourceNames", "algorithmDefinition", "algorithmName", "algorithmProperties",
"algorithmProperty", "showReadwriteSplittingRules"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, "'&&'", "'||'", "'!'", "'~'", "'|'", "'&'", "'<<'", "'>>'", "'^'",
"'%'", "':'", "'+'", "'-'", "'*'", "'/'", "'\\'", "'.'", "'.*'", "'<=>'",
"'=='", "'='", null, "'>'", "'>='", "'<'", "'<='", "'#'", "'('", "')'",
"'{'", "'}'", "'['", "']'", "','", "'\"'", "'''", "'`'", "'?'", "'@'",
"';'", "'->>'", "'_'", null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, "'DO NOT MATCH ANY THING, JUST FOR GENERATOR'"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, "AND", "OR", "NOT", "TILDE", "VERTICALBAR", "AMPERSAND", "SIGNEDLEFTSHIFT",
"SIGNEDRIGHTSHIFT", "CARET", "MOD", "COLON", "PLUS", "MINUS", "ASTERISK",
"SLASH", "BACKSLASH", "DOT", "DOTASTERISK", "SAFEEQ", "DEQ", "EQ", "NEQ",
"GT", "GTE", "LT", "LTE", "POUND", "LP", "RP", "LBE", "RBE", "LBT", "RBT",
"COMMA", "DQ", "SQ", "BQ", "QUESTION", "AT", "SEMI", "JSONSEPARATOR",
"UL", "WS", "CREATE", "ALTER", "DROP", "SET", "SHOW", "RULE", "FROM",
"READWRITE_SPLITTING", "WRITE_RESOURCE", "READ_RESOURCES", "AUTO_AWARE_RESOURCE",
"TYPE", "NAME", "PROPERTIES", "RULES", "SOURCE", "RESOURCES", "STATUS",
"HINT", "CLEAR", "ENABLE", "DISABLE", "READ", "FOR_GENERATOR", "IDENTIFIER",
"STRING", "INT", "HEX", "NUMBER", "HEXDIGIT", "BITNUM"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "ReadwriteSplittingDistSQLStatement.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public ReadwriteSplittingDistSQLStatementParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class ExecuteContext extends ParserRuleContext {
public CreateReadwriteSplittingRuleContext createReadwriteSplittingRule() {
return getRuleContext(CreateReadwriteSplittingRuleContext.class,0);
}
public AlterReadwriteSplittingRuleContext alterReadwriteSplittingRule() {
return getRuleContext(AlterReadwriteSplittingRuleContext.class,0);
}
public DropReadwriteSplittingRuleContext dropReadwriteSplittingRule() {
return getRuleContext(DropReadwriteSplittingRuleContext.class,0);
}
public ShowReadwriteSplittingRulesContext showReadwriteSplittingRules() {
return getRuleContext(ShowReadwriteSplittingRulesContext.class,0);
}
public SetReadwriteSplittingHintSourceContext setReadwriteSplittingHintSource() {
return getRuleContext(SetReadwriteSplittingHintSourceContext.class,0);
}
public ShowReadwriteSplittingHintStatusContext showReadwriteSplittingHintStatus() {
return getRuleContext(ShowReadwriteSplittingHintStatusContext.class,0);
}
public ClearReadwriteSplittingHintContext clearReadwriteSplittingHint() {
return getRuleContext(ClearReadwriteSplittingHintContext.class,0);
}
public EnableReadDataSourceContext enableReadDataSource() {
return getRuleContext(EnableReadDataSourceContext.class,0);
}
public DisableReadDataSourceContext disableReadDataSource() {
return getRuleContext(DisableReadDataSourceContext.class,0);
}
public ShowReadwriteSplittingReadResourcesContext showReadwriteSplittingReadResources() {
return getRuleContext(ShowReadwriteSplittingReadResourcesContext.class,0);
}
public TerminalNode SEMI() { return getToken(ReadwriteSplittingDistSQLStatementParser.SEMI, 0); }
public ExecuteContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_execute; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitExecute(this);
else return visitor.visitChildren(this);
}
}
public final ExecuteContext execute() throws RecognitionException {
ExecuteContext _localctx = new ExecuteContext(_ctx, getState());
enterRule(_localctx, 0, RULE_execute);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(58);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,0,_ctx) ) {
case 1:
{
setState(48);
createReadwriteSplittingRule();
}
break;
case 2:
{
setState(49);
alterReadwriteSplittingRule();
}
break;
case 3:
{
setState(50);
dropReadwriteSplittingRule();
}
break;
case 4:
{
setState(51);
showReadwriteSplittingRules();
}
break;
case 5:
{
setState(52);
setReadwriteSplittingHintSource();
}
break;
case 6:
{
setState(53);
showReadwriteSplittingHintStatus();
}
break;
case 7:
{
setState(54);
clearReadwriteSplittingHint();
}
break;
case 8:
{
setState(55);
enableReadDataSource();
}
break;
case 9:
{
setState(56);
disableReadDataSource();
}
break;
case 10:
{
setState(57);
showReadwriteSplittingReadResources();
}
break;
}
setState(61);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==SEMI) {
{
setState(60);
match(SEMI);
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SetReadwriteSplittingHintSourceContext extends ParserRuleContext {
public TerminalNode SET() { return getToken(ReadwriteSplittingDistSQLStatementParser.SET, 0); }
public TerminalNode READWRITE_SPLITTING() { return getToken(ReadwriteSplittingDistSQLStatementParser.READWRITE_SPLITTING, 0); }
public TerminalNode HINT() { return getToken(ReadwriteSplittingDistSQLStatementParser.HINT, 0); }
public TerminalNode SOURCE() { return getToken(ReadwriteSplittingDistSQLStatementParser.SOURCE, 0); }
public TerminalNode EQ() { return getToken(ReadwriteSplittingDistSQLStatementParser.EQ, 0); }
public SourceValueContext sourceValue() {
return getRuleContext(SourceValueContext.class,0);
}
public SetReadwriteSplittingHintSourceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_setReadwriteSplittingHintSource; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitSetReadwriteSplittingHintSource(this);
else return visitor.visitChildren(this);
}
}
public final SetReadwriteSplittingHintSourceContext setReadwriteSplittingHintSource() throws RecognitionException {
SetReadwriteSplittingHintSourceContext _localctx = new SetReadwriteSplittingHintSourceContext(_ctx, getState());
enterRule(_localctx, 2, RULE_setReadwriteSplittingHintSource);
try {
enterOuterAlt(_localctx, 1);
{
setState(63);
match(SET);
setState(64);
match(READWRITE_SPLITTING);
setState(65);
match(HINT);
setState(66);
match(SOURCE);
setState(67);
match(EQ);
setState(68);
sourceValue();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ShowReadwriteSplittingHintStatusContext extends ParserRuleContext {
public TerminalNode SHOW() { return getToken(ReadwriteSplittingDistSQLStatementParser.SHOW, 0); }
public TerminalNode READWRITE_SPLITTING() { return getToken(ReadwriteSplittingDistSQLStatementParser.READWRITE_SPLITTING, 0); }
public TerminalNode HINT() { return getToken(ReadwriteSplittingDistSQLStatementParser.HINT, 0); }
public TerminalNode STATUS() { return getToken(ReadwriteSplittingDistSQLStatementParser.STATUS, 0); }
public ShowReadwriteSplittingHintStatusContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showReadwriteSplittingHintStatus; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitShowReadwriteSplittingHintStatus(this);
else return visitor.visitChildren(this);
}
}
public final ShowReadwriteSplittingHintStatusContext showReadwriteSplittingHintStatus() throws RecognitionException {
ShowReadwriteSplittingHintStatusContext _localctx = new ShowReadwriteSplittingHintStatusContext(_ctx, getState());
enterRule(_localctx, 4, RULE_showReadwriteSplittingHintStatus);
try {
enterOuterAlt(_localctx, 1);
{
setState(70);
match(SHOW);
setState(71);
match(READWRITE_SPLITTING);
setState(72);
match(HINT);
setState(73);
match(STATUS);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ClearReadwriteSplittingHintContext extends ParserRuleContext {
public TerminalNode CLEAR() { return getToken(ReadwriteSplittingDistSQLStatementParser.CLEAR, 0); }
public TerminalNode READWRITE_SPLITTING() { return getToken(ReadwriteSplittingDistSQLStatementParser.READWRITE_SPLITTING, 0); }
public TerminalNode HINT() { return getToken(ReadwriteSplittingDistSQLStatementParser.HINT, 0); }
public ClearReadwriteSplittingHintContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_clearReadwriteSplittingHint; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitClearReadwriteSplittingHint(this);
else return visitor.visitChildren(this);
}
}
public final ClearReadwriteSplittingHintContext clearReadwriteSplittingHint() throws RecognitionException {
ClearReadwriteSplittingHintContext _localctx = new ClearReadwriteSplittingHintContext(_ctx, getState());
enterRule(_localctx, 6, RULE_clearReadwriteSplittingHint);
try {
enterOuterAlt(_localctx, 1);
{
setState(75);
match(CLEAR);
setState(76);
match(READWRITE_SPLITTING);
setState(77);
match(HINT);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class EnableReadDataSourceContext extends ParserRuleContext {
public TerminalNode ENABLE() { return getToken(ReadwriteSplittingDistSQLStatementParser.ENABLE, 0); }
public TerminalNode READWRITE_SPLITTING() { return getToken(ReadwriteSplittingDistSQLStatementParser.READWRITE_SPLITTING, 0); }
public TerminalNode READ() { return getToken(ReadwriteSplittingDistSQLStatementParser.READ, 0); }
public ResourceNameContext resourceName() {
return getRuleContext(ResourceNameContext.class,0);
}
public TerminalNode FROM() { return getToken(ReadwriteSplittingDistSQLStatementParser.FROM, 0); }
public SchemaNameContext schemaName() {
return getRuleContext(SchemaNameContext.class,0);
}
public EnableReadDataSourceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_enableReadDataSource; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitEnableReadDataSource(this);
else return visitor.visitChildren(this);
}
}
public final EnableReadDataSourceContext enableReadDataSource() throws RecognitionException {
EnableReadDataSourceContext _localctx = new EnableReadDataSourceContext(_ctx, getState());
enterRule(_localctx, 8, RULE_enableReadDataSource);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(79);
match(ENABLE);
setState(80);
match(READWRITE_SPLITTING);
setState(81);
match(READ);
setState(82);
resourceName();
setState(85);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==FROM) {
{
setState(83);
match(FROM);
setState(84);
schemaName();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DisableReadDataSourceContext extends ParserRuleContext {
public TerminalNode DISABLE() { return getToken(ReadwriteSplittingDistSQLStatementParser.DISABLE, 0); }
public TerminalNode READWRITE_SPLITTING() { return getToken(ReadwriteSplittingDistSQLStatementParser.READWRITE_SPLITTING, 0); }
public TerminalNode READ() { return getToken(ReadwriteSplittingDistSQLStatementParser.READ, 0); }
public ResourceNameContext resourceName() {
return getRuleContext(ResourceNameContext.class,0);
}
public TerminalNode FROM() { return getToken(ReadwriteSplittingDistSQLStatementParser.FROM, 0); }
public SchemaNameContext schemaName() {
return getRuleContext(SchemaNameContext.class,0);
}
public DisableReadDataSourceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_disableReadDataSource; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitDisableReadDataSource(this);
else return visitor.visitChildren(this);
}
}
public final DisableReadDataSourceContext disableReadDataSource() throws RecognitionException {
DisableReadDataSourceContext _localctx = new DisableReadDataSourceContext(_ctx, getState());
enterRule(_localctx, 10, RULE_disableReadDataSource);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(87);
match(DISABLE);
setState(88);
match(READWRITE_SPLITTING);
setState(89);
match(READ);
setState(90);
resourceName();
setState(93);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==FROM) {
{
setState(91);
match(FROM);
setState(92);
schemaName();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ShowReadwriteSplittingReadResourcesContext extends ParserRuleContext {
public TerminalNode SHOW() { return getToken(ReadwriteSplittingDistSQLStatementParser.SHOW, 0); }
public TerminalNode READWRITE_SPLITTING() { return getToken(ReadwriteSplittingDistSQLStatementParser.READWRITE_SPLITTING, 0); }
public TerminalNode READ() { return getToken(ReadwriteSplittingDistSQLStatementParser.READ, 0); }
public TerminalNode RESOURCES() { return getToken(ReadwriteSplittingDistSQLStatementParser.RESOURCES, 0); }
public TerminalNode FROM() { return getToken(ReadwriteSplittingDistSQLStatementParser.FROM, 0); }
public SchemaNameContext schemaName() {
return getRuleContext(SchemaNameContext.class,0);
}
public ShowReadwriteSplittingReadResourcesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showReadwriteSplittingReadResources; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitShowReadwriteSplittingReadResources(this);
else return visitor.visitChildren(this);
}
}
public final ShowReadwriteSplittingReadResourcesContext showReadwriteSplittingReadResources() throws RecognitionException {
ShowReadwriteSplittingReadResourcesContext _localctx = new ShowReadwriteSplittingReadResourcesContext(_ctx, getState());
enterRule(_localctx, 12, RULE_showReadwriteSplittingReadResources);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(95);
match(SHOW);
setState(96);
match(READWRITE_SPLITTING);
setState(97);
match(READ);
setState(98);
match(RESOURCES);
setState(101);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==FROM) {
{
setState(99);
match(FROM);
setState(100);
schemaName();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SourceValueContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(ReadwriteSplittingDistSQLStatementParser.IDENTIFIER, 0); }
public SourceValueContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_sourceValue; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitSourceValue(this);
else return visitor.visitChildren(this);
}
}
public final SourceValueContext sourceValue() throws RecognitionException {
SourceValueContext _localctx = new SourceValueContext(_ctx, getState());
enterRule(_localctx, 14, RULE_sourceValue);
try {
enterOuterAlt(_localctx, 1);
{
setState(103);
match(IDENTIFIER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ResourceNameContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(ReadwriteSplittingDistSQLStatementParser.IDENTIFIER, 0); }
public ResourceNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_resourceName; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitResourceName(this);
else return visitor.visitChildren(this);
}
}
public final ResourceNameContext resourceName() throws RecognitionException {
ResourceNameContext _localctx = new ResourceNameContext(_ctx, getState());
enterRule(_localctx, 16, RULE_resourceName);
try {
enterOuterAlt(_localctx, 1);
{
setState(105);
match(IDENTIFIER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SchemaNameContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(ReadwriteSplittingDistSQLStatementParser.IDENTIFIER, 0); }
public SchemaNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_schemaName; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitSchemaName(this);
else return visitor.visitChildren(this);
}
}
public final SchemaNameContext schemaName() throws RecognitionException {
SchemaNameContext _localctx = new SchemaNameContext(_ctx, getState());
enterRule(_localctx, 18, RULE_schemaName);
try {
enterOuterAlt(_localctx, 1);
{
setState(107);
match(IDENTIFIER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class CreateReadwriteSplittingRuleContext extends ParserRuleContext {
public TerminalNode CREATE() { return getToken(ReadwriteSplittingDistSQLStatementParser.CREATE, 0); }
public TerminalNode READWRITE_SPLITTING() { return getToken(ReadwriteSplittingDistSQLStatementParser.READWRITE_SPLITTING, 0); }
public TerminalNode RULE() { return getToken(ReadwriteSplittingDistSQLStatementParser.RULE, 0); }
public List readwriteSplittingRuleDefinition() {
return getRuleContexts(ReadwriteSplittingRuleDefinitionContext.class);
}
public ReadwriteSplittingRuleDefinitionContext readwriteSplittingRuleDefinition(int i) {
return getRuleContext(ReadwriteSplittingRuleDefinitionContext.class,i);
}
public List COMMA() { return getTokens(ReadwriteSplittingDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ReadwriteSplittingDistSQLStatementParser.COMMA, i);
}
public CreateReadwriteSplittingRuleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createReadwriteSplittingRule; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitCreateReadwriteSplittingRule(this);
else return visitor.visitChildren(this);
}
}
public final CreateReadwriteSplittingRuleContext createReadwriteSplittingRule() throws RecognitionException {
CreateReadwriteSplittingRuleContext _localctx = new CreateReadwriteSplittingRuleContext(_ctx, getState());
enterRule(_localctx, 20, RULE_createReadwriteSplittingRule);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(109);
match(CREATE);
setState(110);
match(READWRITE_SPLITTING);
setState(111);
match(RULE);
setState(112);
readwriteSplittingRuleDefinition();
setState(117);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(113);
match(COMMA);
setState(114);
readwriteSplittingRuleDefinition();
}
}
setState(119);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AlterReadwriteSplittingRuleContext extends ParserRuleContext {
public TerminalNode ALTER() { return getToken(ReadwriteSplittingDistSQLStatementParser.ALTER, 0); }
public TerminalNode READWRITE_SPLITTING() { return getToken(ReadwriteSplittingDistSQLStatementParser.READWRITE_SPLITTING, 0); }
public TerminalNode RULE() { return getToken(ReadwriteSplittingDistSQLStatementParser.RULE, 0); }
public List readwriteSplittingRuleDefinition() {
return getRuleContexts(ReadwriteSplittingRuleDefinitionContext.class);
}
public ReadwriteSplittingRuleDefinitionContext readwriteSplittingRuleDefinition(int i) {
return getRuleContext(ReadwriteSplittingRuleDefinitionContext.class,i);
}
public List COMMA() { return getTokens(ReadwriteSplittingDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ReadwriteSplittingDistSQLStatementParser.COMMA, i);
}
public AlterReadwriteSplittingRuleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterReadwriteSplittingRule; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitAlterReadwriteSplittingRule(this);
else return visitor.visitChildren(this);
}
}
public final AlterReadwriteSplittingRuleContext alterReadwriteSplittingRule() throws RecognitionException {
AlterReadwriteSplittingRuleContext _localctx = new AlterReadwriteSplittingRuleContext(_ctx, getState());
enterRule(_localctx, 22, RULE_alterReadwriteSplittingRule);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(120);
match(ALTER);
setState(121);
match(READWRITE_SPLITTING);
setState(122);
match(RULE);
setState(123);
readwriteSplittingRuleDefinition();
setState(128);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(124);
match(COMMA);
setState(125);
readwriteSplittingRuleDefinition();
}
}
setState(130);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DropReadwriteSplittingRuleContext extends ParserRuleContext {
public TerminalNode DROP() { return getToken(ReadwriteSplittingDistSQLStatementParser.DROP, 0); }
public TerminalNode READWRITE_SPLITTING() { return getToken(ReadwriteSplittingDistSQLStatementParser.READWRITE_SPLITTING, 0); }
public TerminalNode RULE() { return getToken(ReadwriteSplittingDistSQLStatementParser.RULE, 0); }
public List ruleName() {
return getRuleContexts(RuleNameContext.class);
}
public RuleNameContext ruleName(int i) {
return getRuleContext(RuleNameContext.class,i);
}
public List COMMA() { return getTokens(ReadwriteSplittingDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ReadwriteSplittingDistSQLStatementParser.COMMA, i);
}
public DropReadwriteSplittingRuleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dropReadwriteSplittingRule; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitDropReadwriteSplittingRule(this);
else return visitor.visitChildren(this);
}
}
public final DropReadwriteSplittingRuleContext dropReadwriteSplittingRule() throws RecognitionException {
DropReadwriteSplittingRuleContext _localctx = new DropReadwriteSplittingRuleContext(_ctx, getState());
enterRule(_localctx, 24, RULE_dropReadwriteSplittingRule);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(131);
match(DROP);
setState(132);
match(READWRITE_SPLITTING);
setState(133);
match(RULE);
setState(134);
ruleName();
setState(139);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(135);
match(COMMA);
setState(136);
ruleName();
}
}
setState(141);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ReadwriteSplittingRuleDefinitionContext extends ParserRuleContext {
public RuleNameContext ruleName() {
return getRuleContext(RuleNameContext.class,0);
}
public TerminalNode LP() { return getToken(ReadwriteSplittingDistSQLStatementParser.LP, 0); }
public TerminalNode RP() { return getToken(ReadwriteSplittingDistSQLStatementParser.RP, 0); }
public StaticReadwriteSplittingRuleDefinitionContext staticReadwriteSplittingRuleDefinition() {
return getRuleContext(StaticReadwriteSplittingRuleDefinitionContext.class,0);
}
public DynamicReadwriteSplittingRuleDefinitionContext dynamicReadwriteSplittingRuleDefinition() {
return getRuleContext(DynamicReadwriteSplittingRuleDefinitionContext.class,0);
}
public TerminalNode COMMA() { return getToken(ReadwriteSplittingDistSQLStatementParser.COMMA, 0); }
public AlgorithmDefinitionContext algorithmDefinition() {
return getRuleContext(AlgorithmDefinitionContext.class,0);
}
public ReadwriteSplittingRuleDefinitionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_readwriteSplittingRuleDefinition; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitReadwriteSplittingRuleDefinition(this);
else return visitor.visitChildren(this);
}
}
public final ReadwriteSplittingRuleDefinitionContext readwriteSplittingRuleDefinition() throws RecognitionException {
ReadwriteSplittingRuleDefinitionContext _localctx = new ReadwriteSplittingRuleDefinitionContext(_ctx, getState());
enterRule(_localctx, 26, RULE_readwriteSplittingRuleDefinition);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(142);
ruleName();
setState(143);
match(LP);
setState(146);
_errHandler.sync(this);
switch (_input.LA(1)) {
case WRITE_RESOURCE:
{
setState(144);
staticReadwriteSplittingRuleDefinition();
}
break;
case AUTO_AWARE_RESOURCE:
{
setState(145);
dynamicReadwriteSplittingRuleDefinition();
}
break;
default:
throw new NoViableAltException(this);
}
setState(150);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA) {
{
setState(148);
match(COMMA);
setState(149);
algorithmDefinition();
}
}
setState(152);
match(RP);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class StaticReadwriteSplittingRuleDefinitionContext extends ParserRuleContext {
public TerminalNode WRITE_RESOURCE() { return getToken(ReadwriteSplittingDistSQLStatementParser.WRITE_RESOURCE, 0); }
public TerminalNode EQ() { return getToken(ReadwriteSplittingDistSQLStatementParser.EQ, 0); }
public WriteResourceNameContext writeResourceName() {
return getRuleContext(WriteResourceNameContext.class,0);
}
public TerminalNode COMMA() { return getToken(ReadwriteSplittingDistSQLStatementParser.COMMA, 0); }
public TerminalNode READ_RESOURCES() { return getToken(ReadwriteSplittingDistSQLStatementParser.READ_RESOURCES, 0); }
public TerminalNode LP() { return getToken(ReadwriteSplittingDistSQLStatementParser.LP, 0); }
public ReadResourceNamesContext readResourceNames() {
return getRuleContext(ReadResourceNamesContext.class,0);
}
public TerminalNode RP() { return getToken(ReadwriteSplittingDistSQLStatementParser.RP, 0); }
public StaticReadwriteSplittingRuleDefinitionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_staticReadwriteSplittingRuleDefinition; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitStaticReadwriteSplittingRuleDefinition(this);
else return visitor.visitChildren(this);
}
}
public final StaticReadwriteSplittingRuleDefinitionContext staticReadwriteSplittingRuleDefinition() throws RecognitionException {
StaticReadwriteSplittingRuleDefinitionContext _localctx = new StaticReadwriteSplittingRuleDefinitionContext(_ctx, getState());
enterRule(_localctx, 28, RULE_staticReadwriteSplittingRuleDefinition);
try {
enterOuterAlt(_localctx, 1);
{
setState(154);
match(WRITE_RESOURCE);
setState(155);
match(EQ);
setState(156);
writeResourceName();
setState(157);
match(COMMA);
setState(158);
match(READ_RESOURCES);
setState(159);
match(LP);
setState(160);
readResourceNames();
setState(161);
match(RP);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DynamicReadwriteSplittingRuleDefinitionContext extends ParserRuleContext {
public TerminalNode AUTO_AWARE_RESOURCE() { return getToken(ReadwriteSplittingDistSQLStatementParser.AUTO_AWARE_RESOURCE, 0); }
public TerminalNode EQ() { return getToken(ReadwriteSplittingDistSQLStatementParser.EQ, 0); }
public ResourceNameContext resourceName() {
return getRuleContext(ResourceNameContext.class,0);
}
public DynamicReadwriteSplittingRuleDefinitionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dynamicReadwriteSplittingRuleDefinition; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitDynamicReadwriteSplittingRuleDefinition(this);
else return visitor.visitChildren(this);
}
}
public final DynamicReadwriteSplittingRuleDefinitionContext dynamicReadwriteSplittingRuleDefinition() throws RecognitionException {
DynamicReadwriteSplittingRuleDefinitionContext _localctx = new DynamicReadwriteSplittingRuleDefinitionContext(_ctx, getState());
enterRule(_localctx, 30, RULE_dynamicReadwriteSplittingRuleDefinition);
try {
enterOuterAlt(_localctx, 1);
{
setState(163);
match(AUTO_AWARE_RESOURCE);
setState(164);
match(EQ);
setState(165);
resourceName();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class RuleNameContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(ReadwriteSplittingDistSQLStatementParser.IDENTIFIER, 0); }
public RuleNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_ruleName; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitRuleName(this);
else return visitor.visitChildren(this);
}
}
public final RuleNameContext ruleName() throws RecognitionException {
RuleNameContext _localctx = new RuleNameContext(_ctx, getState());
enterRule(_localctx, 32, RULE_ruleName);
try {
enterOuterAlt(_localctx, 1);
{
setState(167);
match(IDENTIFIER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class WriteResourceNameContext extends ParserRuleContext {
public ResourceNameContext resourceName() {
return getRuleContext(ResourceNameContext.class,0);
}
public WriteResourceNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_writeResourceName; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitWriteResourceName(this);
else return visitor.visitChildren(this);
}
}
public final WriteResourceNameContext writeResourceName() throws RecognitionException {
WriteResourceNameContext _localctx = new WriteResourceNameContext(_ctx, getState());
enterRule(_localctx, 34, RULE_writeResourceName);
try {
enterOuterAlt(_localctx, 1);
{
setState(169);
resourceName();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ReadResourceNamesContext extends ParserRuleContext {
public List resourceName() {
return getRuleContexts(ResourceNameContext.class);
}
public ResourceNameContext resourceName(int i) {
return getRuleContext(ResourceNameContext.class,i);
}
public List COMMA() { return getTokens(ReadwriteSplittingDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ReadwriteSplittingDistSQLStatementParser.COMMA, i);
}
public ReadResourceNamesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_readResourceNames; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitReadResourceNames(this);
else return visitor.visitChildren(this);
}
}
public final ReadResourceNamesContext readResourceNames() throws RecognitionException {
ReadResourceNamesContext _localctx = new ReadResourceNamesContext(_ctx, getState());
enterRule(_localctx, 36, RULE_readResourceNames);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(171);
resourceName();
setState(176);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(172);
match(COMMA);
setState(173);
resourceName();
}
}
setState(178);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AlgorithmDefinitionContext extends ParserRuleContext {
public TerminalNode TYPE() { return getToken(ReadwriteSplittingDistSQLStatementParser.TYPE, 0); }
public List LP() { return getTokens(ReadwriteSplittingDistSQLStatementParser.LP); }
public TerminalNode LP(int i) {
return getToken(ReadwriteSplittingDistSQLStatementParser.LP, i);
}
public TerminalNode NAME() { return getToken(ReadwriteSplittingDistSQLStatementParser.NAME, 0); }
public TerminalNode EQ() { return getToken(ReadwriteSplittingDistSQLStatementParser.EQ, 0); }
public AlgorithmNameContext algorithmName() {
return getRuleContext(AlgorithmNameContext.class,0);
}
public List RP() { return getTokens(ReadwriteSplittingDistSQLStatementParser.RP); }
public TerminalNode RP(int i) {
return getToken(ReadwriteSplittingDistSQLStatementParser.RP, i);
}
public TerminalNode COMMA() { return getToken(ReadwriteSplittingDistSQLStatementParser.COMMA, 0); }
public TerminalNode PROPERTIES() { return getToken(ReadwriteSplittingDistSQLStatementParser.PROPERTIES, 0); }
public AlgorithmPropertiesContext algorithmProperties() {
return getRuleContext(AlgorithmPropertiesContext.class,0);
}
public AlgorithmDefinitionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_algorithmDefinition; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitAlgorithmDefinition(this);
else return visitor.visitChildren(this);
}
}
public final AlgorithmDefinitionContext algorithmDefinition() throws RecognitionException {
AlgorithmDefinitionContext _localctx = new AlgorithmDefinitionContext(_ctx, getState());
enterRule(_localctx, 38, RULE_algorithmDefinition);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(179);
match(TYPE);
setState(180);
match(LP);
setState(181);
match(NAME);
setState(182);
match(EQ);
setState(183);
algorithmName();
setState(191);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA) {
{
setState(184);
match(COMMA);
setState(185);
match(PROPERTIES);
setState(186);
match(LP);
setState(188);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IDENTIFIER || _la==STRING) {
{
setState(187);
algorithmProperties();
}
}
setState(190);
match(RP);
}
}
setState(193);
match(RP);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AlgorithmNameContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(ReadwriteSplittingDistSQLStatementParser.IDENTIFIER, 0); }
public AlgorithmNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_algorithmName; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitAlgorithmName(this);
else return visitor.visitChildren(this);
}
}
public final AlgorithmNameContext algorithmName() throws RecognitionException {
AlgorithmNameContext _localctx = new AlgorithmNameContext(_ctx, getState());
enterRule(_localctx, 40, RULE_algorithmName);
try {
enterOuterAlt(_localctx, 1);
{
setState(195);
match(IDENTIFIER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AlgorithmPropertiesContext extends ParserRuleContext {
public List algorithmProperty() {
return getRuleContexts(AlgorithmPropertyContext.class);
}
public AlgorithmPropertyContext algorithmProperty(int i) {
return getRuleContext(AlgorithmPropertyContext.class,i);
}
public List COMMA() { return getTokens(ReadwriteSplittingDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ReadwriteSplittingDistSQLStatementParser.COMMA, i);
}
public AlgorithmPropertiesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_algorithmProperties; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitAlgorithmProperties(this);
else return visitor.visitChildren(this);
}
}
public final AlgorithmPropertiesContext algorithmProperties() throws RecognitionException {
AlgorithmPropertiesContext _localctx = new AlgorithmPropertiesContext(_ctx, getState());
enterRule(_localctx, 42, RULE_algorithmProperties);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(197);
algorithmProperty();
setState(202);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(198);
match(COMMA);
setState(199);
algorithmProperty();
}
}
setState(204);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AlgorithmPropertyContext extends ParserRuleContext {
public Token key;
public Token value;
public TerminalNode EQ() { return getToken(ReadwriteSplittingDistSQLStatementParser.EQ, 0); }
public TerminalNode IDENTIFIER() { return getToken(ReadwriteSplittingDistSQLStatementParser.IDENTIFIER, 0); }
public List STRING() { return getTokens(ReadwriteSplittingDistSQLStatementParser.STRING); }
public TerminalNode STRING(int i) {
return getToken(ReadwriteSplittingDistSQLStatementParser.STRING, i);
}
public TerminalNode NUMBER() { return getToken(ReadwriteSplittingDistSQLStatementParser.NUMBER, 0); }
public TerminalNode INT() { return getToken(ReadwriteSplittingDistSQLStatementParser.INT, 0); }
public AlgorithmPropertyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_algorithmProperty; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitAlgorithmProperty(this);
else return visitor.visitChildren(this);
}
}
public final AlgorithmPropertyContext algorithmProperty() throws RecognitionException {
AlgorithmPropertyContext _localctx = new AlgorithmPropertyContext(_ctx, getState());
enterRule(_localctx, 44, RULE_algorithmProperty);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(205);
((AlgorithmPropertyContext)_localctx).key = _input.LT(1);
_la = _input.LA(1);
if ( !(_la==IDENTIFIER || _la==STRING) ) {
((AlgorithmPropertyContext)_localctx).key = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(206);
match(EQ);
setState(207);
((AlgorithmPropertyContext)_localctx).value = _input.LT(1);
_la = _input.LA(1);
if ( !(((((_la - 69)) & ~0x3f) == 0 && ((1L << (_la - 69)) & ((1L << (STRING - 69)) | (1L << (INT - 69)) | (1L << (NUMBER - 69)))) != 0)) ) {
((AlgorithmPropertyContext)_localctx).value = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ShowReadwriteSplittingRulesContext extends ParserRuleContext {
public TerminalNode SHOW() { return getToken(ReadwriteSplittingDistSQLStatementParser.SHOW, 0); }
public TerminalNode READWRITE_SPLITTING() { return getToken(ReadwriteSplittingDistSQLStatementParser.READWRITE_SPLITTING, 0); }
public TerminalNode RULES() { return getToken(ReadwriteSplittingDistSQLStatementParser.RULES, 0); }
public TerminalNode FROM() { return getToken(ReadwriteSplittingDistSQLStatementParser.FROM, 0); }
public SchemaNameContext schemaName() {
return getRuleContext(SchemaNameContext.class,0);
}
public ShowReadwriteSplittingRulesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showReadwriteSplittingRules; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ReadwriteSplittingDistSQLStatementVisitor ) return ((ReadwriteSplittingDistSQLStatementVisitor extends T>)visitor).visitShowReadwriteSplittingRules(this);
else return visitor.visitChildren(this);
}
}
public final ShowReadwriteSplittingRulesContext showReadwriteSplittingRules() throws RecognitionException {
ShowReadwriteSplittingRulesContext _localctx = new ShowReadwriteSplittingRulesContext(_ctx, getState());
enterRule(_localctx, 46, RULE_showReadwriteSplittingRules);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(209);
match(SHOW);
setState(210);
match(READWRITE_SPLITTING);
setState(211);
match(RULES);
setState(214);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==FROM) {
{
setState(212);
match(FROM);
setState(213);
schemaName();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static final String _serializedATN =
"\3\u608b\ua72a\u8133\ub9ed\u417c\u3be7\u7786\u5964\3L\u00db\4\2\t\2\4"+
"\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13\t"+
"\13\4\f\t\f\4\r\t\r\4\16\t\16\4\17\t\17\4\20\t\20\4\21\t\21\4\22\t\22"+
"\4\23\t\23\4\24\t\24\4\25\t\25\4\26\t\26\4\27\t\27\4\30\t\30\4\31\t\31"+
"\3\2\3\2\3\2\3\2\3\2\3\2\3\2\3\2\3\2\3\2\5\2=\n\2\3\2\5\2@\n\2\3\3\3\3"+
"\3\3\3\3\3\3\3\3\3\3\3\4\3\4\3\4\3\4\3\4\3\5\3\5\3\5\3\5\3\6\3\6\3\6\3"+
"\6\3\6\3\6\5\6X\n\6\3\7\3\7\3\7\3\7\3\7\3\7\5\7`\n\7\3\b\3\b\3\b\3\b\3"+
"\b\3\b\5\bh\n\b\3\t\3\t\3\n\3\n\3\13\3\13\3\f\3\f\3\f\3\f\3\f\3\f\7\f"+
"v\n\f\f\f\16\fy\13\f\3\r\3\r\3\r\3\r\3\r\3\r\7\r\u0081\n\r\f\r\16\r\u0084"+
"\13\r\3\16\3\16\3\16\3\16\3\16\3\16\7\16\u008c\n\16\f\16\16\16\u008f\13"+
"\16\3\17\3\17\3\17\3\17\5\17\u0095\n\17\3\17\3\17\5\17\u0099\n\17\3\17"+
"\3\17\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3\21\3\21\3\21\3\21"+
"\3\22\3\22\3\23\3\23\3\24\3\24\3\24\7\24\u00b1\n\24\f\24\16\24\u00b4\13"+
"\24\3\25\3\25\3\25\3\25\3\25\3\25\3\25\3\25\3\25\5\25\u00bf\n\25\3\25"+
"\5\25\u00c2\n\25\3\25\3\25\3\26\3\26\3\27\3\27\3\27\7\27\u00cb\n\27\f"+
"\27\16\27\u00ce\13\27\3\30\3\30\3\30\3\30\3\31\3\31\3\31\3\31\3\31\5\31"+
"\u00d9\n\31\3\31\2\2\32\2\4\6\b\n\f\16\20\22\24\26\30\32\34\36 \"$&(*"+
",.\60\2\4\3\2FG\4\2GHJJ\2\u00d9\2<\3\2\2\2\4A\3\2\2\2\6H\3\2\2\2\bM\3"+
"\2\2\2\nQ\3\2\2\2\fY\3\2\2\2\16a\3\2\2\2\20i\3\2\2\2\22k\3\2\2\2\24m\3"+
"\2\2\2\26o\3\2\2\2\30z\3\2\2\2\32\u0085\3\2\2\2\34\u0090\3\2\2\2\36\u009c"+
"\3\2\2\2 \u00a5\3\2\2\2\"\u00a9\3\2\2\2$\u00ab\3\2\2\2&\u00ad\3\2\2\2"+
"(\u00b5\3\2\2\2*\u00c5\3\2\2\2,\u00c7\3\2\2\2.\u00cf\3\2\2\2\60\u00d3"+
"\3\2\2\2\62=\5\26\f\2\63=\5\30\r\2\64=\5\32\16\2\65=\5\60\31\2\66=\5\4"+
"\3\2\67=\5\6\4\28=\5\b\5\29=\5\n\6\2:=\5\f\7\2;=\5\16\b\2<\62\3\2\2\2"+
"<\63\3\2\2\2<\64\3\2\2\2<\65\3\2\2\2<\66\3\2\2\2<\67\3\2\2\2<8\3\2\2\2"+
"<9\3\2\2\2<:\3\2\2\2<;\3\2\2\2=?\3\2\2\2>@\7*\2\2?>\3\2\2\2?@\3\2\2\2"+
"@\3\3\2\2\2AB\7\61\2\2BC\7\65\2\2CD\7@\2\2DE\7=\2\2EF\7\27\2\2FG\5\20"+
"\t\2G\5\3\2\2\2HI\7\62\2\2IJ\7\65\2\2JK\7@\2\2KL\7?\2\2L\7\3\2\2\2MN\7"+
"A\2\2NO\7\65\2\2OP\7@\2\2P\t\3\2\2\2QR\7B\2\2RS\7\65\2\2ST\7D\2\2TW\5"+
"\22\n\2UV\7\64\2\2VX\5\24\13\2WU\3\2\2\2WX\3\2\2\2X\13\3\2\2\2YZ\7C\2"+
"\2Z[\7\65\2\2[\\\7D\2\2\\_\5\22\n\2]^\7\64\2\2^`\5\24\13\2_]\3\2\2\2_"+
"`\3\2\2\2`\r\3\2\2\2ab\7\62\2\2bc\7\65\2\2cd\7D\2\2dg\7>\2\2ef\7\64\2"+
"\2fh\5\24\13\2ge\3\2\2\2gh\3\2\2\2h\17\3\2\2\2ij\7F\2\2j\21\3\2\2\2kl"+
"\7F\2\2l\23\3\2\2\2mn\7F\2\2n\25\3\2\2\2op\7.\2\2pq\7\65\2\2qr\7\63\2"+
"\2rw\5\34\17\2st\7$\2\2tv\5\34\17\2us\3\2\2\2vy\3\2\2\2wu\3\2\2\2wx\3"+
"\2\2\2x\27\3\2\2\2yw\3\2\2\2z{\7/\2\2{|\7\65\2\2|}\7\63\2\2}\u0082\5\34"+
"\17\2~\177\7$\2\2\177\u0081\5\34\17\2\u0080~\3\2\2\2\u0081\u0084\3\2\2"+
"\2\u0082\u0080\3\2\2\2\u0082\u0083\3\2\2\2\u0083\31\3\2\2\2\u0084\u0082"+
"\3\2\2\2\u0085\u0086\7\60\2\2\u0086\u0087\7\65\2\2\u0087\u0088\7\63\2"+
"\2\u0088\u008d\5\"\22\2\u0089\u008a\7$\2\2\u008a\u008c\5\"\22\2\u008b"+
"\u0089\3\2\2\2\u008c\u008f\3\2\2\2\u008d\u008b\3\2\2\2\u008d\u008e\3\2"+
"\2\2\u008e\33\3\2\2\2\u008f\u008d\3\2\2\2\u0090\u0091\5\"\22\2\u0091\u0094"+
"\7\36\2\2\u0092\u0095\5\36\20\2\u0093\u0095\5 \21\2\u0094\u0092\3\2\2"+
"\2\u0094\u0093\3\2\2\2\u0095\u0098\3\2\2\2\u0096\u0097\7$\2\2\u0097\u0099"+
"\5(\25\2\u0098\u0096\3\2\2\2\u0098\u0099\3\2\2\2\u0099\u009a\3\2\2\2\u009a"+
"\u009b\7\37\2\2\u009b\35\3\2\2\2\u009c\u009d\7\66\2\2\u009d\u009e\7\27"+
"\2\2\u009e\u009f\5$\23\2\u009f\u00a0\7$\2\2\u00a0\u00a1\7\67\2\2\u00a1"+
"\u00a2\7\36\2\2\u00a2\u00a3\5&\24\2\u00a3\u00a4\7\37\2\2\u00a4\37\3\2"+
"\2\2\u00a5\u00a6\78\2\2\u00a6\u00a7\7\27\2\2\u00a7\u00a8\5\22\n\2\u00a8"+
"!\3\2\2\2\u00a9\u00aa\7F\2\2\u00aa#\3\2\2\2\u00ab\u00ac\5\22\n\2\u00ac"+
"%\3\2\2\2\u00ad\u00b2\5\22\n\2\u00ae\u00af\7$\2\2\u00af\u00b1\5\22\n\2"+
"\u00b0\u00ae\3\2\2\2\u00b1\u00b4\3\2\2\2\u00b2\u00b0\3\2\2\2\u00b2\u00b3"+
"\3\2\2\2\u00b3\'\3\2\2\2\u00b4\u00b2\3\2\2\2\u00b5\u00b6\79\2\2\u00b6"+
"\u00b7\7\36\2\2\u00b7\u00b8\7:\2\2\u00b8\u00b9\7\27\2\2\u00b9\u00c1\5"+
"*\26\2\u00ba\u00bb\7$\2\2\u00bb\u00bc\7;\2\2\u00bc\u00be\7\36\2\2\u00bd"+
"\u00bf\5,\27\2\u00be\u00bd\3\2\2\2\u00be\u00bf\3\2\2\2\u00bf\u00c0\3\2"+
"\2\2\u00c0\u00c2\7\37\2\2\u00c1\u00ba\3\2\2\2\u00c1\u00c2\3\2\2\2\u00c2"+
"\u00c3\3\2\2\2\u00c3\u00c4\7\37\2\2\u00c4)\3\2\2\2\u00c5\u00c6\7F\2\2"+
"\u00c6+\3\2\2\2\u00c7\u00cc\5.\30\2\u00c8\u00c9\7$\2\2\u00c9\u00cb\5."+
"\30\2\u00ca\u00c8\3\2\2\2\u00cb\u00ce\3\2\2\2\u00cc\u00ca\3\2\2\2\u00cc"+
"\u00cd\3\2\2\2\u00cd-\3\2\2\2\u00ce\u00cc\3\2\2\2\u00cf\u00d0\t\2\2\2"+
"\u00d0\u00d1\7\27\2\2\u00d1\u00d2\t\3\2\2\u00d2/\3\2\2\2\u00d3\u00d4\7"+
"\62\2\2\u00d4\u00d5\7\65\2\2\u00d5\u00d8\7<\2\2\u00d6\u00d7\7\64\2\2\u00d7"+
"\u00d9\5\24\13\2\u00d8\u00d6\3\2\2\2\u00d8\u00d9\3\2\2\2\u00d9\61\3\2"+
"\2\2\21
© 2015 - 2025 Weber Informatics LLC | Privacy Policy