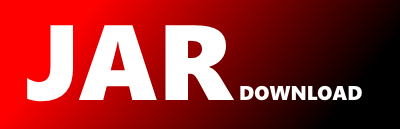
org.apache.shardingsphere.distsql.parser.autogen.ShadowDistSQLStatementParser Maven / Gradle / Ivy
// Generated from org/apache/shardingsphere/distsql/parser/autogen/ShadowDistSQLStatement.g4 by ANTLR 4.9.2
package org.apache.shardingsphere.distsql.parser.autogen;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class ShadowDistSQLStatementParser extends Parser {
static { RuntimeMetaData.checkVersion("4.9.2", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
AND=1, OR=2, NOT=3, TILDE=4, VERTICALBAR=5, AMPERSAND=6, SIGNEDLEFTSHIFT=7,
SIGNEDRIGHTSHIFT=8, CARET=9, MOD=10, COLON=11, PLUS=12, MINUS=13, ASTERISK=14,
SLASH=15, BACKSLASH=16, DOT=17, DOTASTERISK=18, SAFEEQ=19, DEQ=20, EQ=21,
NEQ=22, GT=23, GTE=24, LT=25, LTE=26, POUND=27, LP=28, RP=29, LBE=30,
RBE=31, LBT=32, RBT=33, COMMA=34, DQ=35, SQ=36, BQ=37, QUESTION=38, AT=39,
SEMI=40, JSONSEPARATOR=41, UL=42, WS=43, CREATE=44, ALTER=45, DROP=46,
SHOW=47, SHADOW=48, SOURCE=49, RULE=50, FROM=51, RESOURCES=52, TABLE=53,
TYPE=54, NAME=55, PROPERTIES=56, RULES=57, ALGORITHM=58, ALGORITHMS=59,
SET=60, ADD=61, DATABASE_VALUE=62, TABLE_VALUE=63, STATUS=64, CLEAR=65,
DEFAULT=66, IF=67, EXISTS=68, FOR_GENERATOR=69, STRING=70, IDENTIFIER=71,
INT=72, HEX=73, NUMBER=74, HEXDIGIT=75, BITNUM=76, BOOL=77;
public static final int
RULE_execute = 0, RULE_createShadowRule = 1, RULE_alterShadowRule = 2,
RULE_dropShadowRule = 3, RULE_createShadowAlgorithm = 4, RULE_alterShadowAlgorithm = 5,
RULE_dropShadowAlgorithm = 6, RULE_createDefaultShadowAlgorithm = 7, RULE_dropDefaultShadowAlgorithm = 8,
RULE_shadowRuleDefinition = 9, RULE_shadowTableRule = 10, RULE_source = 11,
RULE_shadow = 12, RULE_tableName = 13, RULE_shadowAlgorithmDefinition = 14,
RULE_algorithmName = 15, RULE_shadowAlgorithmType = 16, RULE_algorithmProperties = 17,
RULE_algorithmProperty = 18, RULE_existClause = 19, RULE_ruleName = 20,
RULE_showShadowRules = 21, RULE_showShadowTableRules = 22, RULE_showShadowAlgorithms = 23,
RULE_shadowRule = 24, RULE_databaseName = 25;
private static String[] makeRuleNames() {
return new String[] {
"execute", "createShadowRule", "alterShadowRule", "dropShadowRule", "createShadowAlgorithm",
"alterShadowAlgorithm", "dropShadowAlgorithm", "createDefaultShadowAlgorithm",
"dropDefaultShadowAlgorithm", "shadowRuleDefinition", "shadowTableRule",
"source", "shadow", "tableName", "shadowAlgorithmDefinition", "algorithmName",
"shadowAlgorithmType", "algorithmProperties", "algorithmProperty", "existClause",
"ruleName", "showShadowRules", "showShadowTableRules", "showShadowAlgorithms",
"shadowRule", "databaseName"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, "'&&'", "'||'", "'!'", "'~'", "'|'", "'&'", "'<<'", "'>>'", "'^'",
"'%'", "':'", "'+'", "'-'", "'*'", "'/'", "'\\'", "'.'", "'.*'", "'<=>'",
"'=='", "'='", null, "'>'", "'>='", "'<'", "'<='", "'#'", "'('", "')'",
"'{'", "'}'", "'['", "']'", "','", "'\"'", "'''", "'`'", "'?'", "'@'",
"';'", "'->>'", "'_'", null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, "'DO NOT MATCH ANY THING, JUST FOR GENERATOR'"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, "AND", "OR", "NOT", "TILDE", "VERTICALBAR", "AMPERSAND", "SIGNEDLEFTSHIFT",
"SIGNEDRIGHTSHIFT", "CARET", "MOD", "COLON", "PLUS", "MINUS", "ASTERISK",
"SLASH", "BACKSLASH", "DOT", "DOTASTERISK", "SAFEEQ", "DEQ", "EQ", "NEQ",
"GT", "GTE", "LT", "LTE", "POUND", "LP", "RP", "LBE", "RBE", "LBT", "RBT",
"COMMA", "DQ", "SQ", "BQ", "QUESTION", "AT", "SEMI", "JSONSEPARATOR",
"UL", "WS", "CREATE", "ALTER", "DROP", "SHOW", "SHADOW", "SOURCE", "RULE",
"FROM", "RESOURCES", "TABLE", "TYPE", "NAME", "PROPERTIES", "RULES",
"ALGORITHM", "ALGORITHMS", "SET", "ADD", "DATABASE_VALUE", "TABLE_VALUE",
"STATUS", "CLEAR", "DEFAULT", "IF", "EXISTS", "FOR_GENERATOR", "STRING",
"IDENTIFIER", "INT", "HEX", "NUMBER", "HEXDIGIT", "BITNUM", "BOOL"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "ShadowDistSQLStatement.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public ShadowDistSQLStatementParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class ExecuteContext extends ParserRuleContext {
public CreateShadowRuleContext createShadowRule() {
return getRuleContext(CreateShadowRuleContext.class,0);
}
public AlterShadowRuleContext alterShadowRule() {
return getRuleContext(AlterShadowRuleContext.class,0);
}
public DropShadowRuleContext dropShadowRule() {
return getRuleContext(DropShadowRuleContext.class,0);
}
public ShowShadowRulesContext showShadowRules() {
return getRuleContext(ShowShadowRulesContext.class,0);
}
public ShowShadowTableRulesContext showShadowTableRules() {
return getRuleContext(ShowShadowTableRulesContext.class,0);
}
public ShowShadowAlgorithmsContext showShadowAlgorithms() {
return getRuleContext(ShowShadowAlgorithmsContext.class,0);
}
public DropShadowAlgorithmContext dropShadowAlgorithm() {
return getRuleContext(DropShadowAlgorithmContext.class,0);
}
public DropDefaultShadowAlgorithmContext dropDefaultShadowAlgorithm() {
return getRuleContext(DropDefaultShadowAlgorithmContext.class,0);
}
public CreateDefaultShadowAlgorithmContext createDefaultShadowAlgorithm() {
return getRuleContext(CreateDefaultShadowAlgorithmContext.class,0);
}
public AlterShadowAlgorithmContext alterShadowAlgorithm() {
return getRuleContext(AlterShadowAlgorithmContext.class,0);
}
public CreateShadowAlgorithmContext createShadowAlgorithm() {
return getRuleContext(CreateShadowAlgorithmContext.class,0);
}
public TerminalNode SEMI() { return getToken(ShadowDistSQLStatementParser.SEMI, 0); }
public ExecuteContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_execute; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitExecute(this);
else return visitor.visitChildren(this);
}
}
public final ExecuteContext execute() throws RecognitionException {
ExecuteContext _localctx = new ExecuteContext(_ctx, getState());
enterRule(_localctx, 0, RULE_execute);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(63);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,0,_ctx) ) {
case 1:
{
setState(52);
createShadowRule();
}
break;
case 2:
{
setState(53);
alterShadowRule();
}
break;
case 3:
{
setState(54);
dropShadowRule();
}
break;
case 4:
{
setState(55);
showShadowRules();
}
break;
case 5:
{
setState(56);
showShadowTableRules();
}
break;
case 6:
{
setState(57);
showShadowAlgorithms();
}
break;
case 7:
{
setState(58);
dropShadowAlgorithm();
}
break;
case 8:
{
setState(59);
dropDefaultShadowAlgorithm();
}
break;
case 9:
{
setState(60);
createDefaultShadowAlgorithm();
}
break;
case 10:
{
setState(61);
alterShadowAlgorithm();
}
break;
case 11:
{
setState(62);
createShadowAlgorithm();
}
break;
}
setState(66);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==SEMI) {
{
setState(65);
match(SEMI);
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class CreateShadowRuleContext extends ParserRuleContext {
public TerminalNode CREATE() { return getToken(ShadowDistSQLStatementParser.CREATE, 0); }
public TerminalNode SHADOW() { return getToken(ShadowDistSQLStatementParser.SHADOW, 0); }
public TerminalNode RULE() { return getToken(ShadowDistSQLStatementParser.RULE, 0); }
public List shadowRuleDefinition() {
return getRuleContexts(ShadowRuleDefinitionContext.class);
}
public ShadowRuleDefinitionContext shadowRuleDefinition(int i) {
return getRuleContext(ShadowRuleDefinitionContext.class,i);
}
public List COMMA() { return getTokens(ShadowDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ShadowDistSQLStatementParser.COMMA, i);
}
public CreateShadowRuleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createShadowRule; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitCreateShadowRule(this);
else return visitor.visitChildren(this);
}
}
public final CreateShadowRuleContext createShadowRule() throws RecognitionException {
CreateShadowRuleContext _localctx = new CreateShadowRuleContext(_ctx, getState());
enterRule(_localctx, 2, RULE_createShadowRule);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(68);
match(CREATE);
setState(69);
match(SHADOW);
setState(70);
match(RULE);
setState(71);
shadowRuleDefinition();
setState(76);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(72);
match(COMMA);
setState(73);
shadowRuleDefinition();
}
}
setState(78);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AlterShadowRuleContext extends ParserRuleContext {
public TerminalNode ALTER() { return getToken(ShadowDistSQLStatementParser.ALTER, 0); }
public TerminalNode SHADOW() { return getToken(ShadowDistSQLStatementParser.SHADOW, 0); }
public TerminalNode RULE() { return getToken(ShadowDistSQLStatementParser.RULE, 0); }
public List shadowRuleDefinition() {
return getRuleContexts(ShadowRuleDefinitionContext.class);
}
public ShadowRuleDefinitionContext shadowRuleDefinition(int i) {
return getRuleContext(ShadowRuleDefinitionContext.class,i);
}
public List COMMA() { return getTokens(ShadowDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ShadowDistSQLStatementParser.COMMA, i);
}
public AlterShadowRuleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterShadowRule; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitAlterShadowRule(this);
else return visitor.visitChildren(this);
}
}
public final AlterShadowRuleContext alterShadowRule() throws RecognitionException {
AlterShadowRuleContext _localctx = new AlterShadowRuleContext(_ctx, getState());
enterRule(_localctx, 4, RULE_alterShadowRule);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(79);
match(ALTER);
setState(80);
match(SHADOW);
setState(81);
match(RULE);
setState(82);
shadowRuleDefinition();
setState(87);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(83);
match(COMMA);
setState(84);
shadowRuleDefinition();
}
}
setState(89);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DropShadowRuleContext extends ParserRuleContext {
public TerminalNode DROP() { return getToken(ShadowDistSQLStatementParser.DROP, 0); }
public TerminalNode SHADOW() { return getToken(ShadowDistSQLStatementParser.SHADOW, 0); }
public TerminalNode RULE() { return getToken(ShadowDistSQLStatementParser.RULE, 0); }
public List ruleName() {
return getRuleContexts(RuleNameContext.class);
}
public RuleNameContext ruleName(int i) {
return getRuleContext(RuleNameContext.class,i);
}
public ExistClauseContext existClause() {
return getRuleContext(ExistClauseContext.class,0);
}
public List COMMA() { return getTokens(ShadowDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ShadowDistSQLStatementParser.COMMA, i);
}
public DropShadowRuleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dropShadowRule; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitDropShadowRule(this);
else return visitor.visitChildren(this);
}
}
public final DropShadowRuleContext dropShadowRule() throws RecognitionException {
DropShadowRuleContext _localctx = new DropShadowRuleContext(_ctx, getState());
enterRule(_localctx, 6, RULE_dropShadowRule);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(90);
match(DROP);
setState(91);
match(SHADOW);
setState(92);
match(RULE);
setState(94);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(93);
existClause();
}
}
setState(96);
ruleName();
setState(101);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(97);
match(COMMA);
setState(98);
ruleName();
}
}
setState(103);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class CreateShadowAlgorithmContext extends ParserRuleContext {
public TerminalNode CREATE() { return getToken(ShadowDistSQLStatementParser.CREATE, 0); }
public TerminalNode SHADOW() { return getToken(ShadowDistSQLStatementParser.SHADOW, 0); }
public TerminalNode ALGORITHM() { return getToken(ShadowDistSQLStatementParser.ALGORITHM, 0); }
public List shadowAlgorithmDefinition() {
return getRuleContexts(ShadowAlgorithmDefinitionContext.class);
}
public ShadowAlgorithmDefinitionContext shadowAlgorithmDefinition(int i) {
return getRuleContext(ShadowAlgorithmDefinitionContext.class,i);
}
public List COMMA() { return getTokens(ShadowDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ShadowDistSQLStatementParser.COMMA, i);
}
public CreateShadowAlgorithmContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createShadowAlgorithm; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitCreateShadowAlgorithm(this);
else return visitor.visitChildren(this);
}
}
public final CreateShadowAlgorithmContext createShadowAlgorithm() throws RecognitionException {
CreateShadowAlgorithmContext _localctx = new CreateShadowAlgorithmContext(_ctx, getState());
enterRule(_localctx, 8, RULE_createShadowAlgorithm);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(104);
match(CREATE);
setState(105);
match(SHADOW);
setState(106);
match(ALGORITHM);
setState(107);
shadowAlgorithmDefinition();
setState(112);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(108);
match(COMMA);
setState(109);
shadowAlgorithmDefinition();
}
}
setState(114);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AlterShadowAlgorithmContext extends ParserRuleContext {
public TerminalNode ALTER() { return getToken(ShadowDistSQLStatementParser.ALTER, 0); }
public TerminalNode SHADOW() { return getToken(ShadowDistSQLStatementParser.SHADOW, 0); }
public TerminalNode ALGORITHM() { return getToken(ShadowDistSQLStatementParser.ALGORITHM, 0); }
public List shadowAlgorithmDefinition() {
return getRuleContexts(ShadowAlgorithmDefinitionContext.class);
}
public ShadowAlgorithmDefinitionContext shadowAlgorithmDefinition(int i) {
return getRuleContext(ShadowAlgorithmDefinitionContext.class,i);
}
public List COMMA() { return getTokens(ShadowDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ShadowDistSQLStatementParser.COMMA, i);
}
public AlterShadowAlgorithmContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterShadowAlgorithm; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitAlterShadowAlgorithm(this);
else return visitor.visitChildren(this);
}
}
public final AlterShadowAlgorithmContext alterShadowAlgorithm() throws RecognitionException {
AlterShadowAlgorithmContext _localctx = new AlterShadowAlgorithmContext(_ctx, getState());
enterRule(_localctx, 10, RULE_alterShadowAlgorithm);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(115);
match(ALTER);
setState(116);
match(SHADOW);
setState(117);
match(ALGORITHM);
setState(118);
shadowAlgorithmDefinition();
setState(123);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(119);
match(COMMA);
setState(120);
shadowAlgorithmDefinition();
}
}
setState(125);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DropShadowAlgorithmContext extends ParserRuleContext {
public TerminalNode DROP() { return getToken(ShadowDistSQLStatementParser.DROP, 0); }
public TerminalNode SHADOW() { return getToken(ShadowDistSQLStatementParser.SHADOW, 0); }
public TerminalNode ALGORITHM() { return getToken(ShadowDistSQLStatementParser.ALGORITHM, 0); }
public List algorithmName() {
return getRuleContexts(AlgorithmNameContext.class);
}
public AlgorithmNameContext algorithmName(int i) {
return getRuleContext(AlgorithmNameContext.class,i);
}
public ExistClauseContext existClause() {
return getRuleContext(ExistClauseContext.class,0);
}
public List COMMA() { return getTokens(ShadowDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ShadowDistSQLStatementParser.COMMA, i);
}
public DropShadowAlgorithmContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dropShadowAlgorithm; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitDropShadowAlgorithm(this);
else return visitor.visitChildren(this);
}
}
public final DropShadowAlgorithmContext dropShadowAlgorithm() throws RecognitionException {
DropShadowAlgorithmContext _localctx = new DropShadowAlgorithmContext(_ctx, getState());
enterRule(_localctx, 12, RULE_dropShadowAlgorithm);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(126);
match(DROP);
setState(127);
match(SHADOW);
setState(128);
match(ALGORITHM);
setState(130);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(129);
existClause();
}
}
setState(132);
algorithmName();
setState(137);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(133);
match(COMMA);
setState(134);
algorithmName();
}
}
setState(139);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class CreateDefaultShadowAlgorithmContext extends ParserRuleContext {
public TerminalNode CREATE() { return getToken(ShadowDistSQLStatementParser.CREATE, 0); }
public TerminalNode DEFAULT() { return getToken(ShadowDistSQLStatementParser.DEFAULT, 0); }
public TerminalNode SHADOW() { return getToken(ShadowDistSQLStatementParser.SHADOW, 0); }
public TerminalNode ALGORITHM() { return getToken(ShadowDistSQLStatementParser.ALGORITHM, 0); }
public TerminalNode NAME() { return getToken(ShadowDistSQLStatementParser.NAME, 0); }
public TerminalNode EQ() { return getToken(ShadowDistSQLStatementParser.EQ, 0); }
public AlgorithmNameContext algorithmName() {
return getRuleContext(AlgorithmNameContext.class,0);
}
public CreateDefaultShadowAlgorithmContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createDefaultShadowAlgorithm; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitCreateDefaultShadowAlgorithm(this);
else return visitor.visitChildren(this);
}
}
public final CreateDefaultShadowAlgorithmContext createDefaultShadowAlgorithm() throws RecognitionException {
CreateDefaultShadowAlgorithmContext _localctx = new CreateDefaultShadowAlgorithmContext(_ctx, getState());
enterRule(_localctx, 14, RULE_createDefaultShadowAlgorithm);
try {
enterOuterAlt(_localctx, 1);
{
setState(140);
match(CREATE);
setState(141);
match(DEFAULT);
setState(142);
match(SHADOW);
setState(143);
match(ALGORITHM);
setState(144);
match(NAME);
setState(145);
match(EQ);
setState(146);
algorithmName();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DropDefaultShadowAlgorithmContext extends ParserRuleContext {
public TerminalNode DROP() { return getToken(ShadowDistSQLStatementParser.DROP, 0); }
public TerminalNode DEFAULT() { return getToken(ShadowDistSQLStatementParser.DEFAULT, 0); }
public TerminalNode SHADOW() { return getToken(ShadowDistSQLStatementParser.SHADOW, 0); }
public TerminalNode ALGORITHM() { return getToken(ShadowDistSQLStatementParser.ALGORITHM, 0); }
public ExistClauseContext existClause() {
return getRuleContext(ExistClauseContext.class,0);
}
public DropDefaultShadowAlgorithmContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dropDefaultShadowAlgorithm; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitDropDefaultShadowAlgorithm(this);
else return visitor.visitChildren(this);
}
}
public final DropDefaultShadowAlgorithmContext dropDefaultShadowAlgorithm() throws RecognitionException {
DropDefaultShadowAlgorithmContext _localctx = new DropDefaultShadowAlgorithmContext(_ctx, getState());
enterRule(_localctx, 16, RULE_dropDefaultShadowAlgorithm);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(148);
match(DROP);
setState(149);
match(DEFAULT);
setState(150);
match(SHADOW);
setState(151);
match(ALGORITHM);
setState(153);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(152);
existClause();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ShadowRuleDefinitionContext extends ParserRuleContext {
public RuleNameContext ruleName() {
return getRuleContext(RuleNameContext.class,0);
}
public TerminalNode LP() { return getToken(ShadowDistSQLStatementParser.LP, 0); }
public TerminalNode SOURCE() { return getToken(ShadowDistSQLStatementParser.SOURCE, 0); }
public List EQ() { return getTokens(ShadowDistSQLStatementParser.EQ); }
public TerminalNode EQ(int i) {
return getToken(ShadowDistSQLStatementParser.EQ, i);
}
public SourceContext source() {
return getRuleContext(SourceContext.class,0);
}
public List COMMA() { return getTokens(ShadowDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ShadowDistSQLStatementParser.COMMA, i);
}
public TerminalNode SHADOW() { return getToken(ShadowDistSQLStatementParser.SHADOW, 0); }
public ShadowContext shadow() {
return getRuleContext(ShadowContext.class,0);
}
public List shadowTableRule() {
return getRuleContexts(ShadowTableRuleContext.class);
}
public ShadowTableRuleContext shadowTableRule(int i) {
return getRuleContext(ShadowTableRuleContext.class,i);
}
public TerminalNode RP() { return getToken(ShadowDistSQLStatementParser.RP, 0); }
public ShadowRuleDefinitionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_shadowRuleDefinition; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitShadowRuleDefinition(this);
else return visitor.visitChildren(this);
}
}
public final ShadowRuleDefinitionContext shadowRuleDefinition() throws RecognitionException {
ShadowRuleDefinitionContext _localctx = new ShadowRuleDefinitionContext(_ctx, getState());
enterRule(_localctx, 18, RULE_shadowRuleDefinition);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(155);
ruleName();
setState(156);
match(LP);
setState(157);
match(SOURCE);
setState(158);
match(EQ);
setState(159);
source();
setState(160);
match(COMMA);
setState(161);
match(SHADOW);
setState(162);
match(EQ);
setState(163);
shadow();
setState(164);
match(COMMA);
setState(165);
shadowTableRule();
setState(170);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(166);
match(COMMA);
setState(167);
shadowTableRule();
}
}
setState(172);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(173);
match(RP);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ShadowTableRuleContext extends ParserRuleContext {
public TableNameContext tableName() {
return getRuleContext(TableNameContext.class,0);
}
public TerminalNode LP() { return getToken(ShadowDistSQLStatementParser.LP, 0); }
public List shadowAlgorithmDefinition() {
return getRuleContexts(ShadowAlgorithmDefinitionContext.class);
}
public ShadowAlgorithmDefinitionContext shadowAlgorithmDefinition(int i) {
return getRuleContext(ShadowAlgorithmDefinitionContext.class,i);
}
public TerminalNode RP() { return getToken(ShadowDistSQLStatementParser.RP, 0); }
public List COMMA() { return getTokens(ShadowDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ShadowDistSQLStatementParser.COMMA, i);
}
public ShadowTableRuleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_shadowTableRule; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitShadowTableRule(this);
else return visitor.visitChildren(this);
}
}
public final ShadowTableRuleContext shadowTableRule() throws RecognitionException {
ShadowTableRuleContext _localctx = new ShadowTableRuleContext(_ctx, getState());
enterRule(_localctx, 20, RULE_shadowTableRule);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(175);
tableName();
setState(176);
match(LP);
setState(177);
shadowAlgorithmDefinition();
setState(182);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(178);
match(COMMA);
setState(179);
shadowAlgorithmDefinition();
}
}
setState(184);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(185);
match(RP);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SourceContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(ShadowDistSQLStatementParser.IDENTIFIER, 0); }
public TerminalNode STRING() { return getToken(ShadowDistSQLStatementParser.STRING, 0); }
public SourceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_source; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitSource(this);
else return visitor.visitChildren(this);
}
}
public final SourceContext source() throws RecognitionException {
SourceContext _localctx = new SourceContext(_ctx, getState());
enterRule(_localctx, 22, RULE_source);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(187);
_la = _input.LA(1);
if ( !(_la==STRING || _la==IDENTIFIER) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ShadowContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(ShadowDistSQLStatementParser.IDENTIFIER, 0); }
public TerminalNode STRING() { return getToken(ShadowDistSQLStatementParser.STRING, 0); }
public ShadowContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_shadow; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitShadow(this);
else return visitor.visitChildren(this);
}
}
public final ShadowContext shadow() throws RecognitionException {
ShadowContext _localctx = new ShadowContext(_ctx, getState());
enterRule(_localctx, 24, RULE_shadow);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(189);
_la = _input.LA(1);
if ( !(_la==STRING || _la==IDENTIFIER) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TableNameContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(ShadowDistSQLStatementParser.IDENTIFIER, 0); }
public TableNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_tableName; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitTableName(this);
else return visitor.visitChildren(this);
}
}
public final TableNameContext tableName() throws RecognitionException {
TableNameContext _localctx = new TableNameContext(_ctx, getState());
enterRule(_localctx, 26, RULE_tableName);
try {
enterOuterAlt(_localctx, 1);
{
setState(191);
match(IDENTIFIER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ShadowAlgorithmDefinitionContext extends ParserRuleContext {
public List LP() { return getTokens(ShadowDistSQLStatementParser.LP); }
public TerminalNode LP(int i) {
return getToken(ShadowDistSQLStatementParser.LP, i);
}
public TerminalNode TYPE() { return getToken(ShadowDistSQLStatementParser.TYPE, 0); }
public TerminalNode NAME() { return getToken(ShadowDistSQLStatementParser.NAME, 0); }
public TerminalNode EQ() { return getToken(ShadowDistSQLStatementParser.EQ, 0); }
public ShadowAlgorithmTypeContext shadowAlgorithmType() {
return getRuleContext(ShadowAlgorithmTypeContext.class,0);
}
public List COMMA() { return getTokens(ShadowDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ShadowDistSQLStatementParser.COMMA, i);
}
public TerminalNode PROPERTIES() { return getToken(ShadowDistSQLStatementParser.PROPERTIES, 0); }
public AlgorithmPropertiesContext algorithmProperties() {
return getRuleContext(AlgorithmPropertiesContext.class,0);
}
public List RP() { return getTokens(ShadowDistSQLStatementParser.RP); }
public TerminalNode RP(int i) {
return getToken(ShadowDistSQLStatementParser.RP, i);
}
public AlgorithmNameContext algorithmName() {
return getRuleContext(AlgorithmNameContext.class,0);
}
public ShadowAlgorithmDefinitionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_shadowAlgorithmDefinition; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitShadowAlgorithmDefinition(this);
else return visitor.visitChildren(this);
}
}
public final ShadowAlgorithmDefinitionContext shadowAlgorithmDefinition() throws RecognitionException {
ShadowAlgorithmDefinitionContext _localctx = new ShadowAlgorithmDefinitionContext(_ctx, getState());
enterRule(_localctx, 28, RULE_shadowAlgorithmDefinition);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(193);
match(LP);
setState(197);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IDENTIFIER) {
{
setState(194);
algorithmName();
setState(195);
match(COMMA);
}
}
setState(199);
match(TYPE);
setState(200);
match(LP);
setState(201);
match(NAME);
setState(202);
match(EQ);
setState(203);
shadowAlgorithmType();
setState(204);
match(COMMA);
setState(205);
match(PROPERTIES);
setState(206);
match(LP);
setState(207);
algorithmProperties();
setState(208);
match(RP);
setState(209);
match(RP);
setState(210);
match(RP);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AlgorithmNameContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(ShadowDistSQLStatementParser.IDENTIFIER, 0); }
public AlgorithmNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_algorithmName; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitAlgorithmName(this);
else return visitor.visitChildren(this);
}
}
public final AlgorithmNameContext algorithmName() throws RecognitionException {
AlgorithmNameContext _localctx = new AlgorithmNameContext(_ctx, getState());
enterRule(_localctx, 30, RULE_algorithmName);
try {
enterOuterAlt(_localctx, 1);
{
setState(212);
match(IDENTIFIER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ShadowAlgorithmTypeContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(ShadowDistSQLStatementParser.IDENTIFIER, 0); }
public ShadowAlgorithmTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_shadowAlgorithmType; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitShadowAlgorithmType(this);
else return visitor.visitChildren(this);
}
}
public final ShadowAlgorithmTypeContext shadowAlgorithmType() throws RecognitionException {
ShadowAlgorithmTypeContext _localctx = new ShadowAlgorithmTypeContext(_ctx, getState());
enterRule(_localctx, 32, RULE_shadowAlgorithmType);
try {
enterOuterAlt(_localctx, 1);
{
setState(214);
match(IDENTIFIER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AlgorithmPropertiesContext extends ParserRuleContext {
public List algorithmProperty() {
return getRuleContexts(AlgorithmPropertyContext.class);
}
public AlgorithmPropertyContext algorithmProperty(int i) {
return getRuleContext(AlgorithmPropertyContext.class,i);
}
public List COMMA() { return getTokens(ShadowDistSQLStatementParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(ShadowDistSQLStatementParser.COMMA, i);
}
public AlgorithmPropertiesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_algorithmProperties; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitAlgorithmProperties(this);
else return visitor.visitChildren(this);
}
}
public final AlgorithmPropertiesContext algorithmProperties() throws RecognitionException {
AlgorithmPropertiesContext _localctx = new AlgorithmPropertiesContext(_ctx, getState());
enterRule(_localctx, 34, RULE_algorithmProperties);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(216);
algorithmProperty();
setState(221);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(217);
match(COMMA);
setState(218);
algorithmProperty();
}
}
setState(223);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AlgorithmPropertyContext extends ParserRuleContext {
public Token key;
public Token value;
public TerminalNode EQ() { return getToken(ShadowDistSQLStatementParser.EQ, 0); }
public TerminalNode SHADOW() { return getToken(ShadowDistSQLStatementParser.SHADOW, 0); }
public TerminalNode IDENTIFIER() { return getToken(ShadowDistSQLStatementParser.IDENTIFIER, 0); }
public List STRING() { return getTokens(ShadowDistSQLStatementParser.STRING); }
public TerminalNode STRING(int i) {
return getToken(ShadowDistSQLStatementParser.STRING, i);
}
public TerminalNode NUMBER() { return getToken(ShadowDistSQLStatementParser.NUMBER, 0); }
public TerminalNode INT() { return getToken(ShadowDistSQLStatementParser.INT, 0); }
public AlgorithmPropertyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_algorithmProperty; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitAlgorithmProperty(this);
else return visitor.visitChildren(this);
}
}
public final AlgorithmPropertyContext algorithmProperty() throws RecognitionException {
AlgorithmPropertyContext _localctx = new AlgorithmPropertyContext(_ctx, getState());
enterRule(_localctx, 36, RULE_algorithmProperty);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(224);
((AlgorithmPropertyContext)_localctx).key = _input.LT(1);
_la = _input.LA(1);
if ( !(((((_la - 48)) & ~0x3f) == 0 && ((1L << (_la - 48)) & ((1L << (SHADOW - 48)) | (1L << (STRING - 48)) | (1L << (IDENTIFIER - 48)))) != 0)) ) {
((AlgorithmPropertyContext)_localctx).key = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(225);
match(EQ);
setState(226);
((AlgorithmPropertyContext)_localctx).value = _input.LT(1);
_la = _input.LA(1);
if ( !(((((_la - 70)) & ~0x3f) == 0 && ((1L << (_la - 70)) & ((1L << (STRING - 70)) | (1L << (INT - 70)) | (1L << (NUMBER - 70)))) != 0)) ) {
((AlgorithmPropertyContext)_localctx).value = (Token)_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExistClauseContext extends ParserRuleContext {
public TerminalNode IF() { return getToken(ShadowDistSQLStatementParser.IF, 0); }
public TerminalNode EXISTS() { return getToken(ShadowDistSQLStatementParser.EXISTS, 0); }
public ExistClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_existClause; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitExistClause(this);
else return visitor.visitChildren(this);
}
}
public final ExistClauseContext existClause() throws RecognitionException {
ExistClauseContext _localctx = new ExistClauseContext(_ctx, getState());
enterRule(_localctx, 38, RULE_existClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(228);
match(IF);
setState(229);
match(EXISTS);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class RuleNameContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(ShadowDistSQLStatementParser.IDENTIFIER, 0); }
public RuleNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_ruleName; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitRuleName(this);
else return visitor.visitChildren(this);
}
}
public final RuleNameContext ruleName() throws RecognitionException {
RuleNameContext _localctx = new RuleNameContext(_ctx, getState());
enterRule(_localctx, 40, RULE_ruleName);
try {
enterOuterAlt(_localctx, 1);
{
setState(231);
match(IDENTIFIER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ShowShadowRulesContext extends ParserRuleContext {
public TerminalNode SHOW() { return getToken(ShadowDistSQLStatementParser.SHOW, 0); }
public TerminalNode SHADOW() { return getToken(ShadowDistSQLStatementParser.SHADOW, 0); }
public ShadowRuleContext shadowRule() {
return getRuleContext(ShadowRuleContext.class,0);
}
public TerminalNode RULES() { return getToken(ShadowDistSQLStatementParser.RULES, 0); }
public TerminalNode FROM() { return getToken(ShadowDistSQLStatementParser.FROM, 0); }
public DatabaseNameContext databaseName() {
return getRuleContext(DatabaseNameContext.class,0);
}
public ShowShadowRulesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showShadowRules; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitShowShadowRules(this);
else return visitor.visitChildren(this);
}
}
public final ShowShadowRulesContext showShadowRules() throws RecognitionException {
ShowShadowRulesContext _localctx = new ShowShadowRulesContext(_ctx, getState());
enterRule(_localctx, 42, RULE_showShadowRules);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(233);
match(SHOW);
setState(234);
match(SHADOW);
setState(237);
_errHandler.sync(this);
switch (_input.LA(1)) {
case RULE:
{
setState(235);
shadowRule();
}
break;
case RULES:
{
setState(236);
match(RULES);
}
break;
default:
throw new NoViableAltException(this);
}
setState(241);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==FROM) {
{
setState(239);
match(FROM);
setState(240);
databaseName();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ShowShadowTableRulesContext extends ParserRuleContext {
public TerminalNode SHOW() { return getToken(ShadowDistSQLStatementParser.SHOW, 0); }
public TerminalNode SHADOW() { return getToken(ShadowDistSQLStatementParser.SHADOW, 0); }
public TerminalNode TABLE() { return getToken(ShadowDistSQLStatementParser.TABLE, 0); }
public TerminalNode RULES() { return getToken(ShadowDistSQLStatementParser.RULES, 0); }
public TerminalNode FROM() { return getToken(ShadowDistSQLStatementParser.FROM, 0); }
public DatabaseNameContext databaseName() {
return getRuleContext(DatabaseNameContext.class,0);
}
public ShowShadowTableRulesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showShadowTableRules; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitShowShadowTableRules(this);
else return visitor.visitChildren(this);
}
}
public final ShowShadowTableRulesContext showShadowTableRules() throws RecognitionException {
ShowShadowTableRulesContext _localctx = new ShowShadowTableRulesContext(_ctx, getState());
enterRule(_localctx, 44, RULE_showShadowTableRules);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(243);
match(SHOW);
setState(244);
match(SHADOW);
setState(245);
match(TABLE);
setState(246);
match(RULES);
setState(249);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==FROM) {
{
setState(247);
match(FROM);
setState(248);
databaseName();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ShowShadowAlgorithmsContext extends ParserRuleContext {
public TerminalNode SHOW() { return getToken(ShadowDistSQLStatementParser.SHOW, 0); }
public TerminalNode SHADOW() { return getToken(ShadowDistSQLStatementParser.SHADOW, 0); }
public TerminalNode ALGORITHMS() { return getToken(ShadowDistSQLStatementParser.ALGORITHMS, 0); }
public TerminalNode FROM() { return getToken(ShadowDistSQLStatementParser.FROM, 0); }
public DatabaseNameContext databaseName() {
return getRuleContext(DatabaseNameContext.class,0);
}
public ShowShadowAlgorithmsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showShadowAlgorithms; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitShowShadowAlgorithms(this);
else return visitor.visitChildren(this);
}
}
public final ShowShadowAlgorithmsContext showShadowAlgorithms() throws RecognitionException {
ShowShadowAlgorithmsContext _localctx = new ShowShadowAlgorithmsContext(_ctx, getState());
enterRule(_localctx, 46, RULE_showShadowAlgorithms);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(251);
match(SHOW);
setState(252);
match(SHADOW);
setState(253);
match(ALGORITHMS);
setState(256);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==FROM) {
{
setState(254);
match(FROM);
setState(255);
databaseName();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ShadowRuleContext extends ParserRuleContext {
public TerminalNode RULE() { return getToken(ShadowDistSQLStatementParser.RULE, 0); }
public RuleNameContext ruleName() {
return getRuleContext(RuleNameContext.class,0);
}
public ShadowRuleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_shadowRule; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitShadowRule(this);
else return visitor.visitChildren(this);
}
}
public final ShadowRuleContext shadowRule() throws RecognitionException {
ShadowRuleContext _localctx = new ShadowRuleContext(_ctx, getState());
enterRule(_localctx, 48, RULE_shadowRule);
try {
enterOuterAlt(_localctx, 1);
{
setState(258);
match(RULE);
setState(259);
ruleName();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DatabaseNameContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(ShadowDistSQLStatementParser.IDENTIFIER, 0); }
public DatabaseNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_databaseName; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof ShadowDistSQLStatementVisitor ) return ((ShadowDistSQLStatementVisitor extends T>)visitor).visitDatabaseName(this);
else return visitor.visitChildren(this);
}
}
public final DatabaseNameContext databaseName() throws RecognitionException {
DatabaseNameContext _localctx = new DatabaseNameContext(_ctx, getState());
enterRule(_localctx, 50, RULE_databaseName);
try {
enterOuterAlt(_localctx, 1);
{
setState(261);
match(IDENTIFIER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static final String _serializedATN =
"\3\u608b\ua72a\u8133\ub9ed\u417c\u3be7\u7786\u5964\3O\u010a\4\2\t\2\4"+
"\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13\t"+
"\13\4\f\t\f\4\r\t\r\4\16\t\16\4\17\t\17\4\20\t\20\4\21\t\21\4\22\t\22"+
"\4\23\t\23\4\24\t\24\4\25\t\25\4\26\t\26\4\27\t\27\4\30\t\30\4\31\t\31"+
"\4\32\t\32\4\33\t\33\3\2\3\2\3\2\3\2\3\2\3\2\3\2\3\2\3\2\3\2\3\2\5\2B"+
"\n\2\3\2\5\2E\n\2\3\3\3\3\3\3\3\3\3\3\3\3\7\3M\n\3\f\3\16\3P\13\3\3\4"+
"\3\4\3\4\3\4\3\4\3\4\7\4X\n\4\f\4\16\4[\13\4\3\5\3\5\3\5\3\5\5\5a\n\5"+
"\3\5\3\5\3\5\7\5f\n\5\f\5\16\5i\13\5\3\6\3\6\3\6\3\6\3\6\3\6\7\6q\n\6"+
"\f\6\16\6t\13\6\3\7\3\7\3\7\3\7\3\7\3\7\7\7|\n\7\f\7\16\7\177\13\7\3\b"+
"\3\b\3\b\3\b\5\b\u0085\n\b\3\b\3\b\3\b\7\b\u008a\n\b\f\b\16\b\u008d\13"+
"\b\3\t\3\t\3\t\3\t\3\t\3\t\3\t\3\t\3\n\3\n\3\n\3\n\3\n\5\n\u009c\n\n\3"+
"\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\7\13\u00ab"+
"\n\13\f\13\16\13\u00ae\13\13\3\13\3\13\3\f\3\f\3\f\3\f\3\f\7\f\u00b7\n"+
"\f\f\f\16\f\u00ba\13\f\3\f\3\f\3\r\3\r\3\16\3\16\3\17\3\17\3\20\3\20\3"+
"\20\3\20\5\20\u00c8\n\20\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3\20"+
"\3\20\3\20\3\20\3\20\3\21\3\21\3\22\3\22\3\23\3\23\3\23\7\23\u00de\n\23"+
"\f\23\16\23\u00e1\13\23\3\24\3\24\3\24\3\24\3\25\3\25\3\25\3\26\3\26\3"+
"\27\3\27\3\27\3\27\5\27\u00f0\n\27\3\27\3\27\5\27\u00f4\n\27\3\30\3\30"+
"\3\30\3\30\3\30\3\30\5\30\u00fc\n\30\3\31\3\31\3\31\3\31\3\31\5\31\u0103"+
"\n\31\3\32\3\32\3\32\3\33\3\33\3\33\2\2\34\2\4\6\b\n\f\16\20\22\24\26"+
"\30\32\34\36 \"$&(*,.\60\62\64\2\5\3\2HI\4\2\62\62HI\5\2HHJJLL\2\u010b"+
"\2A\3\2\2\2\4F\3\2\2\2\6Q\3\2\2\2\b\\\3\2\2\2\nj\3\2\2\2\fu\3\2\2\2\16"+
"\u0080\3\2\2\2\20\u008e\3\2\2\2\22\u0096\3\2\2\2\24\u009d\3\2\2\2\26\u00b1"+
"\3\2\2\2\30\u00bd\3\2\2\2\32\u00bf\3\2\2\2\34\u00c1\3\2\2\2\36\u00c3\3"+
"\2\2\2 \u00d6\3\2\2\2\"\u00d8\3\2\2\2$\u00da\3\2\2\2&\u00e2\3\2\2\2(\u00e6"+
"\3\2\2\2*\u00e9\3\2\2\2,\u00eb\3\2\2\2.\u00f5\3\2\2\2\60\u00fd\3\2\2\2"+
"\62\u0104\3\2\2\2\64\u0107\3\2\2\2\66B\5\4\3\2\67B\5\6\4\28B\5\b\5\29"+
"B\5,\27\2:B\5.\30\2;B\5\60\31\2B\5\20\t\2?B\5\f"+
"\7\2@B\5\n\6\2A\66\3\2\2\2A\67\3\2\2\2A8\3\2\2\2A9\3\2\2\2A:\3\2\2\2A"+
";\3\2\2\2A<\3\2\2\2A=\3\2\2\2A>\3\2\2\2A?\3\2\2\2A@\3\2\2\2BD\3\2\2\2"+
"CE\7*\2\2DC\3\2\2\2DE\3\2\2\2E\3\3\2\2\2FG\7.\2\2GH\7\62\2\2HI\7\64\2"+
"\2IN\5\24\13\2JK\7$\2\2KM\5\24\13\2LJ\3\2\2\2MP\3\2\2\2NL\3\2\2\2NO\3"+
"\2\2\2O\5\3\2\2\2PN\3\2\2\2QR\7/\2\2RS\7\62\2\2ST\7\64\2\2TY\5\24\13\2"+
"UV\7$\2\2VX\5\24\13\2WU\3\2\2\2X[\3\2\2\2YW\3\2\2\2YZ\3\2\2\2Z\7\3\2\2"+
"\2[Y\3\2\2\2\\]\7\60\2\2]^\7\62\2\2^`\7\64\2\2_a\5(\25\2`_\3\2\2\2`a\3"+
"\2\2\2ab\3\2\2\2bg\5*\26\2cd\7$\2\2df\5*\26\2ec\3\2\2\2fi\3\2\2\2ge\3"+
"\2\2\2gh\3\2\2\2h\t\3\2\2\2ig\3\2\2\2jk\7.\2\2kl\7\62\2\2lm\7<\2\2mr\5"+
"\36\20\2no\7$\2\2oq\5\36\20\2pn\3\2\2\2qt\3\2\2\2rp\3\2\2\2rs\3\2\2\2"+
"s\13\3\2\2\2tr\3\2\2\2uv\7/\2\2vw\7\62\2\2wx\7<\2\2x}\5\36\20\2yz\7$\2"+
"\2z|\5\36\20\2{y\3\2\2\2|\177\3\2\2\2}{\3\2\2\2}~\3\2\2\2~\r\3\2\2\2\177"+
"}\3\2\2\2\u0080\u0081\7\60\2\2\u0081\u0082\7\62\2\2\u0082\u0084\7<\2\2"+
"\u0083\u0085\5(\25\2\u0084\u0083\3\2\2\2\u0084\u0085\3\2\2\2\u0085\u0086"+
"\3\2\2\2\u0086\u008b\5 \21\2\u0087\u0088\7$\2\2\u0088\u008a\5 \21\2\u0089"+
"\u0087\3\2\2\2\u008a\u008d\3\2\2\2\u008b\u0089\3\2\2\2\u008b\u008c\3\2"+
"\2\2\u008c\17\3\2\2\2\u008d\u008b\3\2\2\2\u008e\u008f\7.\2\2\u008f\u0090"+
"\7D\2\2\u0090\u0091\7\62\2\2\u0091\u0092\7<\2\2\u0092\u0093\79\2\2\u0093"+
"\u0094\7\27\2\2\u0094\u0095\5 \21\2\u0095\21\3\2\2\2\u0096\u0097\7\60"+
"\2\2\u0097\u0098\7D\2\2\u0098\u0099\7\62\2\2\u0099\u009b\7<\2\2\u009a"+
"\u009c\5(\25\2\u009b\u009a\3\2\2\2\u009b\u009c\3\2\2\2\u009c\23\3\2\2"+
"\2\u009d\u009e\5*\26\2\u009e\u009f\7\36\2\2\u009f\u00a0\7\63\2\2\u00a0"+
"\u00a1\7\27\2\2\u00a1\u00a2\5\30\r\2\u00a2\u00a3\7$\2\2\u00a3\u00a4\7"+
"\62\2\2\u00a4\u00a5\7\27\2\2\u00a5\u00a6\5\32\16\2\u00a6\u00a7\7$\2\2"+
"\u00a7\u00ac\5\26\f\2\u00a8\u00a9\7$\2\2\u00a9\u00ab\5\26\f\2\u00aa\u00a8"+
"\3\2\2\2\u00ab\u00ae\3\2\2\2\u00ac\u00aa\3\2\2\2\u00ac\u00ad\3\2\2\2\u00ad"+
"\u00af\3\2\2\2\u00ae\u00ac\3\2\2\2\u00af\u00b0\7\37\2\2\u00b0\25\3\2\2"+
"\2\u00b1\u00b2\5\34\17\2\u00b2\u00b3\7\36\2\2\u00b3\u00b8\5\36\20\2\u00b4"+
"\u00b5\7$\2\2\u00b5\u00b7\5\36\20\2\u00b6\u00b4\3\2\2\2\u00b7\u00ba\3"+
"\2\2\2\u00b8\u00b6\3\2\2\2\u00b8\u00b9\3\2\2\2\u00b9\u00bb\3\2\2\2\u00ba"+
"\u00b8\3\2\2\2\u00bb\u00bc\7\37\2\2\u00bc\27\3\2\2\2\u00bd\u00be\t\2\2"+
"\2\u00be\31\3\2\2\2\u00bf\u00c0\t\2\2\2\u00c0\33\3\2\2\2\u00c1\u00c2\7"+
"I\2\2\u00c2\35\3\2\2\2\u00c3\u00c7\7\36\2\2\u00c4\u00c5\5 \21\2\u00c5"+
"\u00c6\7$\2\2\u00c6\u00c8\3\2\2\2\u00c7\u00c4\3\2\2\2\u00c7\u00c8\3\2"+
"\2\2\u00c8\u00c9\3\2\2\2\u00c9\u00ca\78\2\2\u00ca\u00cb\7\36\2\2\u00cb"+
"\u00cc\79\2\2\u00cc\u00cd\7\27\2\2\u00cd\u00ce\5\"\22\2\u00ce\u00cf\7"+
"$\2\2\u00cf\u00d0\7:\2\2\u00d0\u00d1\7\36\2\2\u00d1\u00d2\5$\23\2\u00d2"+
"\u00d3\7\37\2\2\u00d3\u00d4\7\37\2\2\u00d4\u00d5\7\37\2\2\u00d5\37\3\2"+
"\2\2\u00d6\u00d7\7I\2\2\u00d7!\3\2\2\2\u00d8\u00d9\7I\2\2\u00d9#\3\2\2"+
"\2\u00da\u00df\5&\24\2\u00db\u00dc\7$\2\2\u00dc\u00de\5&\24\2\u00dd\u00db"+
"\3\2\2\2\u00de\u00e1\3\2\2\2\u00df\u00dd\3\2\2\2\u00df\u00e0\3\2\2\2\u00e0"+
"%\3\2\2\2\u00e1\u00df\3\2\2\2\u00e2\u00e3\t\3\2\2\u00e3\u00e4\7\27\2\2"+
"\u00e4\u00e5\t\4\2\2\u00e5\'\3\2\2\2\u00e6\u00e7\7E\2\2\u00e7\u00e8\7"+
"F\2\2\u00e8)\3\2\2\2\u00e9\u00ea\7I\2\2\u00ea+\3\2\2\2\u00eb\u00ec\7\61"+
"\2\2\u00ec\u00ef\7\62\2\2\u00ed\u00f0\5\62\32\2\u00ee\u00f0\7;\2\2\u00ef"+
"\u00ed\3\2\2\2\u00ef\u00ee\3\2\2\2\u00f0\u00f3\3\2\2\2\u00f1\u00f2\7\65"+
"\2\2\u00f2\u00f4\5\64\33\2\u00f3\u00f1\3\2\2\2\u00f3\u00f4\3\2\2\2\u00f4"+
"-\3\2\2\2\u00f5\u00f6\7\61\2\2\u00f6\u00f7\7\62\2\2\u00f7\u00f8\7\67\2"+
"\2\u00f8\u00fb\7;\2\2\u00f9\u00fa\7\65\2\2\u00fa\u00fc\5\64\33\2\u00fb"+
"\u00f9\3\2\2\2\u00fb\u00fc\3\2\2\2\u00fc/\3\2\2\2\u00fd\u00fe\7\61\2\2"+
"\u00fe\u00ff\7\62\2\2\u00ff\u0102\7=\2\2\u0100\u0101\7\65\2\2\u0101\u0103"+
"\5\64\33\2\u0102\u0100\3\2\2\2\u0102\u0103\3\2\2\2\u0103\61\3\2\2\2\u0104"+
"\u0105\7\64\2\2\u0105\u0106\5*\26\2\u0106\63\3\2\2\2\u0107\u0108\7I\2"+
"\2\u0108\65\3\2\2\2\25ADNY`gr}\u0084\u008b\u009b\u00ac\u00b8\u00c7\u00df"+
"\u00ef\u00f3\u00fb\u0102";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy