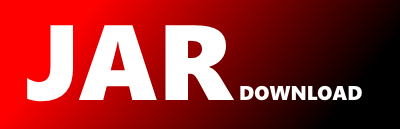
org.apache.shindig.gadgets.rewrite.RewriteModule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shindig-gadgets Show documentation
Show all versions of shindig-gadgets Show documentation
Renders gadgets, provides the gadget metadata service, and serves
all javascript required by the OpenSocial specification.
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.shindig.gadgets.rewrite;
import com.google.common.collect.ImmutableList;
import com.google.inject.AbstractModule;
import com.google.inject.Key;
import com.google.inject.Provider;
import com.google.inject.Provides;
import com.google.inject.Singleton;
import com.google.inject.TypeLiteral;
import com.google.inject.multibindings.MapBinder;
import com.google.inject.multibindings.Multibinder;
import com.google.inject.name.Named;
import com.google.inject.name.Names;
import org.apache.shindig.config.ContainerConfig;
import org.apache.shindig.gadgets.parse.GadgetHtmlParser;
import org.apache.shindig.gadgets.render.CajaResponseRewriter;
import org.apache.shindig.gadgets.render.OpenSocialI18NGadgetRewriter;
import org.apache.shindig.gadgets.render.RenderingGadgetRewriter;
import org.apache.shindig.gadgets.render.SanitizingGadgetRewriter;
import org.apache.shindig.gadgets.render.SanitizingResponseRewriter;
import org.apache.shindig.gadgets.rewrite.ResponseRewriterList.RewriteFlow;
import org.apache.shindig.gadgets.rewrite.image.BasicImageRewriter;
import org.apache.shindig.gadgets.servlet.CajaContentRewriter;
import org.apache.shindig.gadgets.uri.AccelUriManager;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* Guice bindings for the rewrite package.
*/
public class RewriteModule extends AbstractModule {
public static final String ACCEL_CONTAINER = AccelUriManager.CONTAINER;
public static final String DEFAULT_CONTAINER = ContainerConfig.DEFAULT_CONTAINER;
// Mapbinder for the map from
// RewritePath -> [ List of response rewriters ].
protected MapBinder> mapbinder;
@Override
protected void configure() {
configureGadgetRewriters();
provideResponseRewriters();
}
protected void provideResponseRewriters() {
mapbinder = MapBinder.newMapBinder(binder(), new TypeLiteral(){},
new TypeLiteral>() {});
Provider> accelRewriterList = getResponseRewriters(
ACCEL_CONTAINER, RewriteFlow.ACCELERATE);
Provider> requestPipelineRewriterList = getResponseRewriters(
DEFAULT_CONTAINER, RewriteFlow.REQUEST_PIPELINE);
addBindingForRewritePath(DEFAULT_CONTAINER, RewriteFlow.REQUEST_PIPELINE);
addBindingForRewritePath(DEFAULT_CONTAINER, RewriteFlow.DEFAULT);
addBindingForRewritePath(ACCEL_CONTAINER, RewriteFlow.ACCELERATE);
addBindingForRewritePath(ACCEL_CONTAINER, RewriteFlow.REQUEST_PIPELINE,
requestPipelineRewriterList);
addBindingForRewritePath(ACCEL_CONTAINER, RewriteFlow.DEFAULT, accelRewriterList);
}
protected void addBindingForRewritePath(String container, RewriteFlow rewriteFlow,
Provider> list) {
RewritePath rewritePath = new RewritePath(container, rewriteFlow);
mapbinder.addBinding(rewritePath).toProvider(list);
}
protected void addBindingForRewritePath(String container, RewriteFlow rewriteFlow) {
addBindingForRewritePath(container, rewriteFlow, binder().getProvider(
getKey(container, rewriteFlow)));
}
protected Provider> getResponseRewriters(String container,
RewriteFlow flow) {
return binder().getProvider(getKey(container, flow));
}
protected Key> getKey(String container, RewriteFlow flow) {
return Key.get(new TypeLiteral>() {},
new RewritePath(container, flow));
}
// Provides ResponseRewriterRegistry for DEFAULT flow.
@Provides
@Singleton
@RewriterRegistry(rewriteFlow = RewriteFlow.DEFAULT)
public ResponseRewriterRegistry provideDefaultList(GadgetHtmlParser parser,
Provider
© 2015 - 2024 Weber Informatics LLC | Privacy Policy