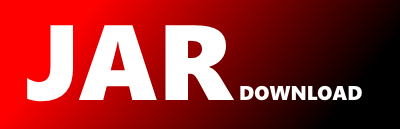
org.apache.sling.jcr.resource.JcrPropertyMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.apache.sling.jcr.resource Show documentation
Show all versions of org.apache.sling.jcr.resource Show documentation
This bundle provides the JCR based ResourceResolver.
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.sling.jcr.resource;
import java.util.Calendar;
import java.util.Collection;
import java.util.Collections;
import java.util.Date;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Set;
import javax.jcr.Node;
import javax.jcr.Property;
import javax.jcr.PropertyIterator;
import javax.jcr.RepositoryException;
import javax.jcr.Value;
import org.apache.jackrabbit.util.ISO9075;
import org.apache.jackrabbit.util.Text;
import org.apache.sling.api.resource.ValueMap;
import org.apache.sling.jcr.resource.internal.helper.JcrPropertyMapCacheEntry;
import org.slf4j.LoggerFactory;
/**
* An implementation of the value map based on a JCR node.
* @see JcrModifiablePropertyMap
*
* @deprecated A (JCR) resource should be adapted to a {@link ValueMap}.
*/
@Deprecated
public class JcrPropertyMap
implements ValueMap {
private static volatile boolean LOG_DEPRECATED = true;
/** The underlying node. */
private final Node node;
/** A cache for the properties. */
final Map cache;
/** A cache for the values. */
final Map valueCache;
/** Has the node been read completely? */
boolean fullyRead;
/** keep all prefixes for escaping */
private String[] namespacePrefixes;
private final ClassLoader dynamicClassLoader;
/**
* Create a new JCR property map based on a node.
* @param node The underlying node.
*/
public JcrPropertyMap(final Node node) {
this(node, null);
}
/**
* Create a new JCR property map based on a node.
* @param node The underlying node.
* @param dynamicCL Dynamic class loader for loading serialized objects.
* @since 2.0.8
*/
public JcrPropertyMap(final Node node, final ClassLoader dynamicCL) {
this.node = node;
this.cache = new LinkedHashMap();
this.valueCache = new LinkedHashMap();
this.fullyRead = false;
this.dynamicClassLoader = dynamicCL;
if ( LOG_DEPRECATED ) {
LOG_DEPRECATED = false;
LoggerFactory.getLogger(this.getClass()).warn("DEPRECATION WARNING: JcrPropertyMap is deprecated. Please switch to resource API.");
}
}
protected ClassLoader getDynamicClassLoader() {
return this.dynamicClassLoader;
}
/**
* Get the node.
*
* @return the node
*/
protected Node getNode() {
return node;
}
// ---------- ValueMap
String checkKey(final String key) {
if ( key == null ) {
throw new NullPointerException("Key must not be null.");
}
if ( key.startsWith("./") ) {
return key.substring(2);
}
return key;
}
/**
* @see org.apache.sling.api.resource.ValueMap#get(java.lang.String, java.lang.Class)
*/
@Override
@SuppressWarnings("unchecked")
public T get(final String aKey, final Class type) {
final String key = checkKey(aKey);
if (type == null) {
return (T) get(key);
}
final JcrPropertyMapCacheEntry entry = this.read(key);
if ( entry == null ) {
return null;
}
return entry.convertToType(type, this.node, this.getDynamicClassLoader());
}
/**
* @see org.apache.sling.api.resource.ValueMap#get(java.lang.String, java.lang.Object)
*/
@Override
@SuppressWarnings("unchecked")
public T get(final String aKey,final T defaultValue) {
final String key = checkKey(aKey);
if (defaultValue == null) {
return (T) get(key);
}
// special handling in case the default value implements one
// of the interface types supported by the convertToType method
Class type = (Class) normalizeClass(defaultValue.getClass());
T value = get(key, type);
if (value == null) {
value = defaultValue;
}
return value;
}
// ---------- Map
/**
* @see java.util.Map#get(java.lang.Object)
*/
@Override
public Object get(final Object aKey) {
final String key = checkKey(aKey.toString());
final JcrPropertyMapCacheEntry entry = this.read(key);
final Object value = (entry == null ? null : entry.getPropertyValueOrNull());
return value;
}
/**
* @see java.util.Map#containsKey(java.lang.Object)
*/
@Override
public boolean containsKey(final Object key) {
return get(key) != null;
}
/**
* @see java.util.Map#containsValue(java.lang.Object)
*/
@Override
public boolean containsValue(final Object value) {
readFully();
return valueCache.containsValue(value);
}
/**
* @see java.util.Map#isEmpty()
*/
@Override
public boolean isEmpty() {
return size() == 0;
}
/**
* @see java.util.Map#size()
*/
@Override
public int size() {
readFully();
return cache.size();
}
/**
* @see java.util.Map#entrySet()
*/
@Override
public Set> entrySet() {
readFully();
final Map sourceMap;
if (cache.size() == valueCache.size()) {
sourceMap = valueCache;
} else {
sourceMap = transformEntries(cache);
}
return Collections.unmodifiableSet(sourceMap.entrySet());
}
/**
* @see java.util.Map#keySet()
*/
@Override
public Set keySet() {
readFully();
return cache.keySet();
}
/**
* @see java.util.Map#values()
*/
@Override
public Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy