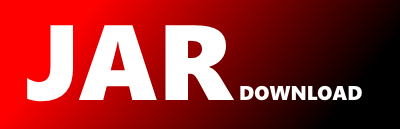
org.apache.solr.ltr.LTRThreadModule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of solr-ltr Show documentation
Show all versions of solr-ltr Show documentation
Apache Solr Learning to Rank Package
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.solr.ltr;
import java.util.Map;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Semaphore;
import org.apache.solr.common.util.NamedList;
import org.apache.solr.util.SolrPluginUtils;
import org.apache.solr.util.plugin.NamedListInitializedPlugin;
/**
* The LTRThreadModule is optionally used by the {@link org.apache.solr.ltr.search.LTRQParserPlugin}
* and {@link org.apache.solr.ltr.response.transform.LTRFeatureLoggerTransformerFactory
* LTRFeatureLoggerTransformerFactory} classes to parallelize the creation of {@link
* org.apache.solr.ltr.feature.Feature.FeatureWeight Feature.FeatureWeight} objects.
*
* Example configuration:
*
*
* <queryParser name="ltr" class="org.apache.solr.ltr.search.LTRQParserPlugin">
* <int name="threadModule.totalPoolThreads">10</int>
* <int name="threadModule.numThreadsPerRequest">5</int>
* </queryParser>
*
* <transformer name="features" class="org.apache.solr.ltr.response.transform.LTRFeatureLoggerTransformerFactory">
* <int name="threadModule.totalPoolThreads">10</int>
* <int name="threadModule.numThreadsPerRequest">5</int>
* </transformer>
*
*
* If an individual solr instance is expected to receive no more than one query at a time, it is
* best to set totalPoolThreads
and numThreadsPerRequest
to the same
* value.
*
* If multiple queries need to be serviced simultaneously then totalPoolThreads
and
* numThreadsPerRequest
can be adjusted based on the expected response times.
*
*
If the value of numThreadsPerRequest
is higher, the response time for a single
* query will be improved up to a point. If multiple queries are serviced simultaneously, the value
* of totalPoolThreads
imposes a contention between the queries if
* (totalPoolThreads < numThreadsPerRequest * total parallel queries)
.
*/
public final class LTRThreadModule implements NamedListInitializedPlugin {
public static LTRThreadModule getInstance(NamedList> args) {
final LTRThreadModule threadManager;
final NamedList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy