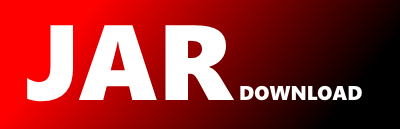
org.apache.solr.client.solrj.request.ReplicasApi Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.solr.client.solrj.request;
import org.apache.solr.client.api.model.ScaleCollectionRequestBody;
import org.apache.solr.client.api.model.SubResponseAccumulatingJerseyResponse;
import org.apache.solr.client.solrj.JacksonContentWriter;
import org.apache.solr.client.solrj.JacksonParsingResponse;
import org.apache.solr.client.solrj.ResponseParser;
import org.apache.solr.client.solrj.SolrClient;
import org.apache.solr.client.solrj.SolrRequest;
import org.apache.solr.client.solrj.impl.InputStreamResponseParser;
import org.apache.solr.common.params.ModifiableSolrParams;
import org.apache.solr.common.params.SolrParams;
// WARNING: This class is generated from a Mustache template; any intended
// changes should be made to the underlying template and not this file directly.
public class ReplicasApi {
public static class DeleteReplicaByNameResponse
extends JacksonParsingResponse {
public DeleteReplicaByNameResponse() {
super(SubResponseAccumulatingJerseyResponse.class);
}
}
public static class DeleteReplicaByName extends SolrRequest {
private final String collectionName;
private final String shardName;
private final String replicaName;
private Boolean followAliases;
private Boolean deleteInstanceDir;
private Boolean deleteDataDir;
private Boolean deleteIndex;
private Boolean onlyIfDown;
private String async;
/**
* Create a DeleteReplicaByName request object.
*
* @param collectionName Path param -
* @param shardName Path param -
* @param replicaName Path param -
*/
public DeleteReplicaByName(String collectionName, String shardName, String replicaName) {
super(
SolrRequest.METHOD.valueOf("DELETE"),
"/collections/{collectionName}/shards/{shardName}/replicas/{replicaName}"
.replace("{" + "collectionName" + "}", collectionName)
.replace("{" + "shardName" + "}", shardName)
.replace("{" + "replicaName" + "}", replicaName));
this.collectionName = collectionName;
this.shardName = shardName;
this.replicaName = replicaName;
}
public void setFollowAliases(Boolean followAliases) {
this.followAliases = followAliases;
}
public void setDeleteInstanceDir(Boolean deleteInstanceDir) {
this.deleteInstanceDir = deleteInstanceDir;
}
public void setDeleteDataDir(Boolean deleteDataDir) {
this.deleteDataDir = deleteDataDir;
}
public void setDeleteIndex(Boolean deleteIndex) {
this.deleteIndex = deleteIndex;
}
public void setOnlyIfDown(Boolean onlyIfDown) {
this.onlyIfDown = onlyIfDown;
}
public void setAsync(String async) {
this.async = async;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
if (followAliases != null) {
params.add("followAliases", followAliases.toString());
}
if (deleteInstanceDir != null) {
params.add("deleteInstanceDir", deleteInstanceDir.toString());
}
if (deleteDataDir != null) {
params.add("deleteDataDir", deleteDataDir.toString());
}
if (deleteIndex != null) {
params.add("deleteIndex", deleteIndex.toString());
}
if (onlyIfDown != null) {
params.add("onlyIfDown", onlyIfDown.toString());
}
if (async != null) {
params.add("async", async);
}
return params;
}
@Override
protected DeleteReplicaByNameResponse createResponse(SolrClient client) {
return new DeleteReplicaByNameResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class DeleteReplicasByCountResponse
extends JacksonParsingResponse {
public DeleteReplicasByCountResponse() {
super(SubResponseAccumulatingJerseyResponse.class);
}
}
public static class DeleteReplicasByCount extends SolrRequest {
private final String collectionName;
private final String shardName;
private Integer count;
private Boolean followAliases;
private Boolean deleteInstanceDir;
private Boolean deleteDataDir;
private Boolean deleteIndex;
private Boolean onlyIfDown;
private String async;
/**
* Create a DeleteReplicasByCount request object.
*
* @param collectionName Path param -
* @param shardName Path param -
*/
public DeleteReplicasByCount(String collectionName, String shardName) {
super(
SolrRequest.METHOD.valueOf("DELETE"),
"/collections/{collectionName}/shards/{shardName}/replicas"
.replace("{" + "collectionName" + "}", collectionName)
.replace("{" + "shardName" + "}", shardName));
this.collectionName = collectionName;
this.shardName = shardName;
}
public void setCount(Integer count) {
this.count = count;
}
public void setFollowAliases(Boolean followAliases) {
this.followAliases = followAliases;
}
public void setDeleteInstanceDir(Boolean deleteInstanceDir) {
this.deleteInstanceDir = deleteInstanceDir;
}
public void setDeleteDataDir(Boolean deleteDataDir) {
this.deleteDataDir = deleteDataDir;
}
public void setDeleteIndex(Boolean deleteIndex) {
this.deleteIndex = deleteIndex;
}
public void setOnlyIfDown(Boolean onlyIfDown) {
this.onlyIfDown = onlyIfDown;
}
public void setAsync(String async) {
this.async = async;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
if (count != null) {
params.add("count", count.toString());
}
if (followAliases != null) {
params.add("followAliases", followAliases.toString());
}
if (deleteInstanceDir != null) {
params.add("deleteInstanceDir", deleteInstanceDir.toString());
}
if (deleteDataDir != null) {
params.add("deleteDataDir", deleteDataDir.toString());
}
if (deleteIndex != null) {
params.add("deleteIndex", deleteIndex.toString());
}
if (onlyIfDown != null) {
params.add("onlyIfDown", onlyIfDown.toString());
}
if (async != null) {
params.add("async", async);
}
return params;
}
@Override
protected DeleteReplicasByCountResponse createResponse(SolrClient client) {
return new DeleteReplicasByCountResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class DeleteReplicasByCountAllShardsResponse
extends JacksonParsingResponse {
public DeleteReplicasByCountAllShardsResponse() {
super(SubResponseAccumulatingJerseyResponse.class);
}
}
public static class DeleteReplicasByCountAllShards
extends SolrRequest {
private final ScaleCollectionRequestBody requestBody;
private final String collectionName;
/**
* Create a DeleteReplicasByCountAllShards request object.
*
* @param collectionName Path param -
*/
public DeleteReplicasByCountAllShards(String collectionName) {
super(
SolrRequest.METHOD.valueOf("PUT"),
"/collections/{collectionName}/scale"
.replace("{" + "collectionName" + "}", collectionName));
this.collectionName = collectionName;
this.requestBody = new ScaleCollectionRequestBody();
addHeader("Content-type", "application/json");
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setNumToDelete(Integer numToDelete) {
this.requestBody.numToDelete = numToDelete;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setFollowAliases(Boolean followAliases) {
this.requestBody.followAliases = followAliases;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setDeleteInstanceDir(Boolean deleteInstanceDir) {
this.requestBody.deleteInstanceDir = deleteInstanceDir;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setDeleteDataDir(Boolean deleteDataDir) {
this.requestBody.deleteDataDir = deleteDataDir;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setDeleteIndex(Boolean deleteIndex) {
this.requestBody.deleteIndex = deleteIndex;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setOnlyIfDown(Boolean onlyIfDown) {
this.requestBody.onlyIfDown = onlyIfDown;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setAsync(String async) {
this.requestBody.async = async;
}
@Override
public RequestWriter.ContentWriter getContentWriter(String expectedType) {
return new JacksonContentWriter(expectedType, requestBody);
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected DeleteReplicasByCountAllShardsResponse createResponse(SolrClient client) {
return new DeleteReplicasByCountAllShardsResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy