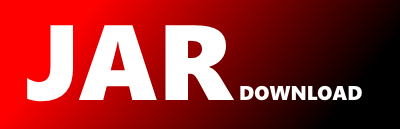
org.apache.solr.client.solrj.request.ReplicaPropertiesApi Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.solr.client.solrj.request;
import org.apache.solr.client.api.model.AddReplicaPropertyRequestBody;
import org.apache.solr.client.solrj.JacksonContentWriter;
import org.apache.solr.client.solrj.JacksonParsingResponse;
import org.apache.solr.client.solrj.ResponseParser;
import org.apache.solr.client.solrj.SolrClient;
import org.apache.solr.client.solrj.SolrRequest;
import org.apache.solr.client.solrj.impl.InputStreamResponseParser;
import org.apache.solr.common.params.ModifiableSolrParams;
import org.apache.solr.common.params.SolrParams;
// WARNING: This class is generated from a Mustache template; any intended
// changes should be made to the underlying template and not this file directly.
/**
* Experimental SolrRequest's and SolrResponse's for replicaProperties, generated from an OAS.
*
* See individual request and response classes for more detailed and relevant information.
*
*
All SolrRequest implementations rely on v2 APIs which may require a SolrClient configured to
* use the '/api' path prefix, instead of '/solr'.
*
* @lucene.experimental
*/
public class ReplicaPropertiesApi {
public static class AddReplicaPropertyResponse
extends JacksonParsingResponse {
public AddReplicaPropertyResponse() {
super(org.apache.solr.client.api.model.SolrJerseyResponse.class);
}
}
public static class AddReplicaProperty extends SolrRequest {
private final AddReplicaPropertyRequestBody requestBody;
private final String collName;
private final String shardName;
private final String replicaName;
private final String propName;
/**
* Create a AddReplicaProperty request object.
*
* @param collName Path param - The name of the collection the replica belongs to.
* @param shardName Path param - The name of the shard the replica belongs to.
* @param replicaName Path param - The replica, e.g., `core_node1`.
* @param propName Path param - The name of the property to add.
*/
public AddReplicaProperty(
String collName, String shardName, String replicaName, String propName) {
super(
SolrRequest.METHOD.valueOf("PUT"),
"/collections/{collName}/shards/{shardName}/replicas/{replicaName}/properties/{propName}"
.replace("{" + "collName" + "}", collName)
.replace("{" + "shardName" + "}", shardName)
.replace("{" + "replicaName" + "}", replicaName)
.replace("{" + "propName" + "}", propName));
this.collName = collName;
this.shardName = shardName;
this.replicaName = replicaName;
this.propName = propName;
this.requestBody = new AddReplicaPropertyRequestBody();
addHeader("Content-type", "application/json");
}
// TODO find a way to add required parameters in the request body to the class constructor
/**
* @param value The value to assign to the property.
*/
public void setValue(String value) {
this.requestBody.value = value;
}
// TODO find a way to add required parameters in the request body to the class constructor
/**
* @param shardUnique If `true`, then setting this property in one replica will remove
* the property from all other replicas in that shard. The default is
* `false`.\\nThere is one pre-defined property `preferredLeader` for
* which `shardUnique` is forced to `true` and an error returned if
* `shardUnique` is explicitly set to `false`.
*/
public void setShardUnique(Boolean shardUnique) {
this.requestBody.shardUnique = shardUnique;
}
@Override
@SuppressWarnings("unchecked")
public RequestWriter.ContentWriter getContentWriter(String expectedType) {
return new JacksonContentWriter(expectedType, requestBody);
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected AddReplicaPropertyResponse createResponse(SolrClient client) {
return new AddReplicaPropertyResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class DeleteReplicaPropertyResponse
extends JacksonParsingResponse {
public DeleteReplicaPropertyResponse() {
super(org.apache.solr.client.api.model.SolrJerseyResponse.class);
}
}
public static class DeleteReplicaProperty extends SolrRequest {
private final String collName;
private final String shardName;
private final String replicaName;
private final String propName;
/**
* Create a DeleteReplicaProperty request object.
*
* @param collName Path param - The name of the collection the replica belongs to.
* @param shardName Path param - The name of the shard the replica belongs to.
* @param replicaName Path param - The replica, e.g., `core_node1`.
* @param propName Path param - The name of the property to delete.
*/
public DeleteReplicaProperty(
String collName, String shardName, String replicaName, String propName) {
super(
SolrRequest.METHOD.valueOf("DELETE"),
"/collections/{collName}/shards/{shardName}/replicas/{replicaName}/properties/{propName}"
.replace("{" + "collName" + "}", collName)
.replace("{" + "shardName" + "}", shardName)
.replace("{" + "replicaName" + "}", replicaName)
.replace("{" + "propName" + "}", propName));
this.collName = collName;
this.shardName = shardName;
this.replicaName = replicaName;
this.propName = propName;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected DeleteReplicaPropertyResponse createResponse(SolrClient client) {
return new DeleteReplicaPropertyResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
}