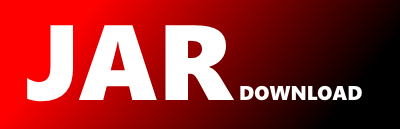
org.apache.solr.client.solrj.request.SchemaApi Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.solr.client.solrj.request;
import org.apache.solr.client.api.model.IndexType;
import org.apache.solr.client.solrj.JacksonParsingResponse;
import org.apache.solr.client.solrj.ResponseParser;
import org.apache.solr.client.solrj.SolrClient;
import org.apache.solr.client.solrj.SolrRequest;
import org.apache.solr.client.solrj.impl.InputStreamResponseParser;
import org.apache.solr.common.params.ModifiableSolrParams;
import org.apache.solr.common.params.SolrParams;
// WARNING: This class is generated from a Mustache template; any intended
// changes should be made to the underlying template and not this file directly.
/**
* Experimental SolrRequest's and SolrResponse's for schema, generated from an OAS.
*
* See individual request and response classes for more detailed and relevant information.
*
*
All SolrRequest implementations rely on v2 APIs which may require a SolrClient configured to
* use the '/api' path prefix, instead of '/solr'.
*
* @lucene.experimental
*/
public class SchemaApi {
public static class GetSchemaInfoResponse
extends JacksonParsingResponse {
public GetSchemaInfoResponse() {
super(org.apache.solr.client.api.model.SchemaInfoResponse.class);
}
}
public static class GetSchemaInfo extends SolrRequest {
private final IndexType indexType;
private final String indexName;
/**
* Create a GetSchemaInfo request object.
*
* @param indexType Path param -
* @param indexName Path param -
*/
public GetSchemaInfo(IndexType indexType, String indexName) {
super(
SolrRequest.METHOD.valueOf("GET"),
"/{indexType}/{indexName}/schema"
.replace("{" + "indexType" + "}", indexType.toString())
.replace("{" + "indexName" + "}", indexName));
this.indexType = indexType;
this.indexName = indexName;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected GetSchemaInfoResponse createResponse(SolrClient client) {
return new GetSchemaInfoResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class GetSchemaNameResponse
extends JacksonParsingResponse {
public GetSchemaNameResponse() {
super(org.apache.solr.client.api.model.SchemaNameResponse.class);
}
}
public static class GetSchemaName extends SolrRequest {
private final IndexType indexType;
private final String indexName;
/**
* Create a GetSchemaName request object.
*
* @param indexType Path param -
* @param indexName Path param -
*/
public GetSchemaName(IndexType indexType, String indexName) {
super(
SolrRequest.METHOD.valueOf("GET"),
"/{indexType}/{indexName}/schema/name"
.replace("{" + "indexType" + "}", indexType.toString())
.replace("{" + "indexName" + "}", indexName));
this.indexType = indexType;
this.indexName = indexName;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected GetSchemaNameResponse createResponse(SolrClient client) {
return new GetSchemaNameResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class GetSchemaSimilarityResponse
extends JacksonParsingResponse {
public GetSchemaSimilarityResponse() {
super(org.apache.solr.client.api.model.SchemaSimilarityResponse.class);
}
}
public static class GetSchemaSimilarity extends SolrRequest {
private final IndexType indexType;
private final String indexName;
/**
* Create a GetSchemaSimilarity request object.
*
* @param indexType Path param -
* @param indexName Path param -
*/
public GetSchemaSimilarity(IndexType indexType, String indexName) {
super(
SolrRequest.METHOD.valueOf("GET"),
"/{indexType}/{indexName}/schema/similarity"
.replace("{" + "indexType" + "}", indexType.toString())
.replace("{" + "indexName" + "}", indexName));
this.indexType = indexType;
this.indexName = indexName;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected GetSchemaSimilarityResponse createResponse(SolrClient client) {
return new GetSchemaSimilarityResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class GetSchemaUniqueKeyResponse
extends JacksonParsingResponse {
public GetSchemaUniqueKeyResponse() {
super(org.apache.solr.client.api.model.SchemaUniqueKeyResponse.class);
}
}
public static class GetSchemaUniqueKey extends SolrRequest {
private final IndexType indexType;
private final String indexName;
/**
* Create a GetSchemaUniqueKey request object.
*
* @param indexType Path param -
* @param indexName Path param -
*/
public GetSchemaUniqueKey(IndexType indexType, String indexName) {
super(
SolrRequest.METHOD.valueOf("GET"),
"/{indexType}/{indexName}/schema/uniquekey"
.replace("{" + "indexType" + "}", indexType.toString())
.replace("{" + "indexName" + "}", indexName));
this.indexType = indexType;
this.indexName = indexName;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected GetSchemaUniqueKeyResponse createResponse(SolrClient client) {
return new GetSchemaUniqueKeyResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class GetSchemaVersionResponse
extends JacksonParsingResponse {
public GetSchemaVersionResponse() {
super(org.apache.solr.client.api.model.SchemaVersionResponse.class);
}
}
public static class GetSchemaVersion extends SolrRequest {
private final IndexType indexType;
private final String indexName;
/**
* Create a GetSchemaVersion request object.
*
* @param indexType Path param -
* @param indexName Path param -
*/
public GetSchemaVersion(IndexType indexType, String indexName) {
super(
SolrRequest.METHOD.valueOf("GET"),
"/{indexType}/{indexName}/schema/version"
.replace("{" + "indexType" + "}", indexType.toString())
.replace("{" + "indexName" + "}", indexName));
this.indexType = indexType;
this.indexName = indexName;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected GetSchemaVersionResponse createResponse(SolrClient client) {
return new GetSchemaVersionResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class GetSchemaZkVersionResponse
extends JacksonParsingResponse {
public GetSchemaZkVersionResponse() {
super(org.apache.solr.client.api.model.SchemaZkVersionResponse.class);
}
}
public static class GetSchemaZkVersion extends SolrRequest {
private final IndexType indexType;
private final String indexName;
private Integer refreshIfBelowVersion;
/**
* Create a GetSchemaZkVersion request object.
*
* @param indexType Path param -
* @param indexName Path param -
*/
public GetSchemaZkVersion(IndexType indexType, String indexName) {
super(
SolrRequest.METHOD.valueOf("GET"),
"/{indexType}/{indexName}/schema/zkversion"
.replace("{" + "indexType" + "}", indexType.toString())
.replace("{" + "indexName" + "}", indexName));
this.indexType = indexType;
this.indexName = indexName;
}
public void setRefreshIfBelowVersion(Integer refreshIfBelowVersion) {
this.refreshIfBelowVersion = refreshIfBelowVersion;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
if (refreshIfBelowVersion != null) {
params.add("refreshIfBelowVersion", refreshIfBelowVersion.toString());
}
return params;
}
@Override
protected GetSchemaZkVersionResponse createResponse(SolrClient client) {
return new GetSchemaZkVersionResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
}