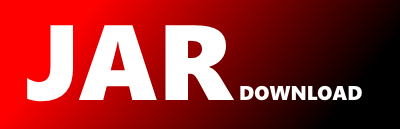
org.apache.solr.client.solrj.request.CollectionBackupsApi Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.solr.client.solrj.request;
import java.util.Map;
import org.apache.solr.client.api.model.CreateCollectionBackupRequestBody;
import org.apache.solr.client.api.model.CreateCollectionRequestBody;
import org.apache.solr.client.api.model.PurgeUnusedFilesRequestBody;
import org.apache.solr.client.api.model.RestoreCollectionRequestBody;
import org.apache.solr.client.solrj.JacksonContentWriter;
import org.apache.solr.client.solrj.JacksonParsingResponse;
import org.apache.solr.client.solrj.ResponseParser;
import org.apache.solr.client.solrj.SolrClient;
import org.apache.solr.client.solrj.SolrRequest;
import org.apache.solr.client.solrj.impl.InputStreamResponseParser;
import org.apache.solr.common.params.ModifiableSolrParams;
import org.apache.solr.common.params.SolrParams;
// WARNING: This class is generated from a Mustache template; any intended
// changes should be made to the underlying template and not this file directly.
/**
* Experimental SolrRequest's and SolrResponse's for collectionBackups, generated from an OAS.
*
* See individual request and response classes for more detailed and relevant information.
*
*
All SolrRequest implementations rely on v2 APIs which may require a SolrClient configured to
* use the '/api' path prefix, instead of '/solr'.
*
* @lucene.experimental
*/
public class CollectionBackupsApi {
public static class CreateCollectionBackupResponse
extends JacksonParsingResponse {
public CreateCollectionBackupResponse() {
super(org.apache.solr.client.api.model.SolrJerseyResponse.class);
}
}
public static class CreateCollectionBackup extends SolrRequest {
private final CreateCollectionBackupRequestBody requestBody;
private final String collectionName;
private final String backupName;
/**
* Create a CreateCollectionBackup request object.
*
* @param collectionName Path param -
* @param backupName Path param -
*/
public CreateCollectionBackup(String collectionName, String backupName) {
super(
SolrRequest.METHOD.valueOf("POST"),
"/collections/{collectionName}/backups/{backupName}/versions"
.replace("{" + "collectionName" + "}", collectionName)
.replace("{" + "backupName" + "}", backupName));
this.collectionName = collectionName;
this.backupName = backupName;
this.requestBody = new CreateCollectionBackupRequestBody();
addHeader("Content-type", "application/json");
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setLocation(String location) {
this.requestBody.location = location;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setRepository(String repository) {
this.requestBody.repository = repository;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setFollowAliases(Boolean followAliases) {
this.requestBody.followAliases = followAliases;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setBackupStrategy(String backupStrategy) {
this.requestBody.backupStrategy = backupStrategy;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setSnapshotName(String snapshotName) {
this.requestBody.snapshotName = snapshotName;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setIncremental(Boolean incremental) {
this.requestBody.incremental = incremental;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setBackupConfigset(Boolean backupConfigset) {
this.requestBody.backupConfigset = backupConfigset;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setMaxNumBackupPoints(Integer maxNumBackupPoints) {
this.requestBody.maxNumBackupPoints = maxNumBackupPoints;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setAsync(String async) {
this.requestBody.async = async;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setExtraProperties(Map extraProperties) {
this.requestBody.extraProperties = extraProperties;
}
@Override
@SuppressWarnings("unchecked")
public RequestWriter.ContentWriter getContentWriter(String expectedType) {
return new JacksonContentWriter(expectedType, requestBody);
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public ApiVersion getApiVersion() {
return ApiVersion.V2;
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected CreateCollectionBackupResponse createResponse(SolrClient client) {
return new CreateCollectionBackupResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class DeleteMultipleBackupsByRecencyResponse
extends JacksonParsingResponse {
public DeleteMultipleBackupsByRecencyResponse() {
super(org.apache.solr.client.api.model.BackupDeletionResponseBody.class);
}
}
public static class DeleteMultipleBackupsByRecency
extends SolrRequest {
private final String backupName;
private Integer retainLatest;
private String location;
private String repository;
private String async;
/**
* Create a DeleteMultipleBackupsByRecency request object.
*
* @param backupName Path param -
*/
public DeleteMultipleBackupsByRecency(String backupName) {
super(
SolrRequest.METHOD.valueOf("DELETE"),
"/backups/{backupName}/versions".replace("{" + "backupName" + "}", backupName));
this.backupName = backupName;
}
public void setRetainLatest(Integer retainLatest) {
this.retainLatest = retainLatest;
}
public void setLocation(String location) {
this.location = location;
}
public void setRepository(String repository) {
this.repository = repository;
}
public void setAsync(String async) {
this.async = async;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public ApiVersion getApiVersion() {
return ApiVersion.V2;
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
if (retainLatest != null) {
params.add("retainLatest", retainLatest.toString());
}
if (location != null) {
params.add("location", location);
}
if (repository != null) {
params.add("repository", repository);
}
if (async != null) {
params.add("async", async);
}
return params;
}
@Override
protected DeleteMultipleBackupsByRecencyResponse createResponse(SolrClient client) {
return new DeleteMultipleBackupsByRecencyResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class DeleteSingleBackupByIdResponse
extends JacksonParsingResponse {
public DeleteSingleBackupByIdResponse() {
super(org.apache.solr.client.api.model.BackupDeletionResponseBody.class);
}
}
public static class DeleteSingleBackupById extends SolrRequest {
private final String backupName;
private final String backupId;
private String location;
private String repository;
private String async;
/**
* Create a DeleteSingleBackupById request object.
*
* @param backupName Path param -
* @param backupId Path param -
*/
public DeleteSingleBackupById(String backupName, String backupId) {
super(
SolrRequest.METHOD.valueOf("DELETE"),
"/backups/{backupName}/versions/{backupId}"
.replace("{" + "backupName" + "}", backupName)
.replace("{" + "backupId" + "}", backupId));
this.backupName = backupName;
this.backupId = backupId;
}
public void setLocation(String location) {
this.location = location;
}
public void setRepository(String repository) {
this.repository = repository;
}
public void setAsync(String async) {
this.async = async;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public ApiVersion getApiVersion() {
return ApiVersion.V2;
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
if (location != null) {
params.add("location", location);
}
if (repository != null) {
params.add("repository", repository);
}
if (async != null) {
params.add("async", async);
}
return params;
}
@Override
protected DeleteSingleBackupByIdResponse createResponse(SolrClient client) {
return new DeleteSingleBackupByIdResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class GarbageCollectUnusedBackupFilesResponse
extends JacksonParsingResponse {
public GarbageCollectUnusedBackupFilesResponse() {
super(org.apache.solr.client.api.model.PurgeUnusedResponse.class);
}
}
public static class GarbageCollectUnusedBackupFiles
extends SolrRequest {
private final PurgeUnusedFilesRequestBody requestBody;
private final String backupName;
/**
* Create a GarbageCollectUnusedBackupFiles request object.
*
* @param backupName Path param -
*/
public GarbageCollectUnusedBackupFiles(String backupName) {
super(
SolrRequest.METHOD.valueOf("PUT"),
"/backups/{backupName}/purgeUnused".replace("{" + "backupName" + "}", backupName));
this.backupName = backupName;
this.requestBody = new PurgeUnusedFilesRequestBody();
addHeader("Content-type", "application/json");
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setLocation(String location) {
this.requestBody.location = location;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setRepositoryName(String repositoryName) {
this.requestBody.repositoryName = repositoryName;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setAsync(String async) {
this.requestBody.async = async;
}
@Override
@SuppressWarnings("unchecked")
public RequestWriter.ContentWriter getContentWriter(String expectedType) {
return new JacksonContentWriter(expectedType, requestBody);
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public ApiVersion getApiVersion() {
return ApiVersion.V2;
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected GarbageCollectUnusedBackupFilesResponse createResponse(SolrClient client) {
return new GarbageCollectUnusedBackupFilesResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class ListBackupsAtLocationResponse
extends JacksonParsingResponse<
org.apache.solr.client.api.model.ListCollectionBackupsResponse> {
public ListBackupsAtLocationResponse() {
super(org.apache.solr.client.api.model.ListCollectionBackupsResponse.class);
}
}
public static class ListBackupsAtLocation extends SolrRequest {
private final String backupName;
private String location;
private String repository;
/**
* Create a ListBackupsAtLocation request object.
*
* @param backupName Path param -
*/
public ListBackupsAtLocation(String backupName) {
super(
SolrRequest.METHOD.valueOf("GET"),
"/backups/{backupName}/versions".replace("{" + "backupName" + "}", backupName));
this.backupName = backupName;
}
public void setLocation(String location) {
this.location = location;
}
public void setRepository(String repository) {
this.repository = repository;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public ApiVersion getApiVersion() {
return ApiVersion.V2;
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
if (location != null) {
params.add("location", location);
}
if (repository != null) {
params.add("repository", repository);
}
return params;
}
@Override
protected ListBackupsAtLocationResponse createResponse(SolrClient client) {
return new ListBackupsAtLocationResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class RestoreCollectionResponse
extends JacksonParsingResponse<
org.apache.solr.client.api.model.SubResponseAccumulatingJerseyResponse> {
public RestoreCollectionResponse() {
super(org.apache.solr.client.api.model.SubResponseAccumulatingJerseyResponse.class);
}
}
public static class RestoreCollection extends SolrRequest {
private final RestoreCollectionRequestBody requestBody;
private final String backupName;
/**
* Create a RestoreCollection request object.
*
* @param backupName Path param -
*/
public RestoreCollection(String backupName) {
super(
SolrRequest.METHOD.valueOf("POST"),
"/backups/{backupName}/restore".replace("{" + "backupName" + "}", backupName));
this.backupName = backupName;
this.requestBody = new RestoreCollectionRequestBody();
addHeader("Content-type", "application/json");
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setCollection(String collection) {
this.requestBody.collection = collection;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setLocation(String location) {
this.requestBody.location = location;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setRepository(String repository) {
this.requestBody.repository = repository;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setBackupId(Integer backupId) {
this.requestBody.backupId = backupId;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setAsync(String async) {
this.requestBody.async = async;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setCreateCollectionParams(CreateCollectionRequestBody createCollectionParams) {
this.requestBody.createCollectionParams = createCollectionParams;
}
@Override
@SuppressWarnings("unchecked")
public RequestWriter.ContentWriter getContentWriter(String expectedType) {
return new JacksonContentWriter(expectedType, requestBody);
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public ApiVersion getApiVersion() {
return ApiVersion.V2;
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected RestoreCollectionResponse createResponse(SolrClient client) {
return new RestoreCollectionResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
}