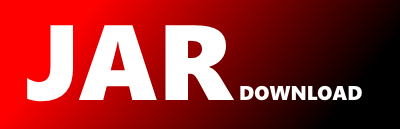
org.apache.solr.client.solrj.request.QueryingApi Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.solr.client.solrj.request;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import org.apache.solr.client.api.model.IndexType;
import org.apache.solr.client.solrj.JacksonContentWriter;
import org.apache.solr.client.solrj.JacksonParsingResponse;
import org.apache.solr.client.solrj.ResponseParser;
import org.apache.solr.client.solrj.SolrClient;
import org.apache.solr.client.solrj.SolrRequest;
import org.apache.solr.client.solrj.impl.InputStreamResponseParser;
import org.apache.solr.common.params.ModifiableSolrParams;
import org.apache.solr.common.params.SolrParams;
// WARNING: This class is generated from a Mustache template; any intended
// changes should be made to the underlying template and not this file directly.
/**
* Experimental SolrRequest's and SolrResponse's for querying, generated from an OAS.
*
* See individual request and response classes for more detailed and relevant information.
*
*
All SolrRequest implementations rely on v2 APIs which may require a SolrClient configured to
* use the '/api' path prefix, instead of '/solr'.
*
* @lucene.experimental
*/
public class QueryingApi {
public static class JsonQueryResponse
extends JacksonParsingResponse {
public JsonQueryResponse() {
super(org.apache.solr.client.api.model.FlexibleSolrJerseyResponse.class);
}
}
public static class JsonQuery extends SolrRequest {
private final Object requestBody;
private final IndexType indexType;
private final String indexName;
/**
* Create a JsonQuery request object.
*
* @param indexType Path param -
* @param indexName Path param -
*/
public JsonQuery(IndexType indexType, String indexName, Object requestBody) {
super(
SolrRequest.METHOD.valueOf("POST"),
"/{indexType}/{indexName}/select"
.replace("{" + "indexType" + "}", indexType.toString())
.replace("{" + "indexName" + "}", indexName));
this.indexType = indexType;
this.indexName = indexName;
this.requestBody = requestBody;
addHeader("Content-type", "application/json");
}
@Override
@SuppressWarnings("unchecked")
public RequestWriter.ContentWriter getContentWriter(String expectedType) {
if (requestBody instanceof String) {
return new RequestWriter.StringPayloadContentWriter((String) requestBody, expectedType);
} else if (requestBody instanceof InputStream) {
final var inputStream = (InputStream) requestBody;
return new RequestWriter.ContentWriter() {
@Override
public void write(OutputStream os) throws IOException {
inputStream.transferTo(os);
}
@Override
public String getContentType() {
return expectedType;
}
};
}
return new JacksonContentWriter(expectedType, requestBody);
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public ApiVersion getApiVersion() {
return ApiVersion.V2;
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected JsonQueryResponse createResponse(SolrClient client) {
return new JsonQueryResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class QueryResponse
extends JacksonParsingResponse {
public QueryResponse() {
super(org.apache.solr.client.api.model.FlexibleSolrJerseyResponse.class);
}
}
public static class Query extends SolrRequest {
private final IndexType indexType;
private final String indexName;
private String q;
private List fq;
private String fl;
private Integer rows;
/**
* Create a Query request object.
*
* @param indexType Path param -
* @param indexName Path param -
*/
public Query(IndexType indexType, String indexName) {
super(
SolrRequest.METHOD.valueOf("GET"),
"/{indexType}/{indexName}/select"
.replace("{" + "indexType" + "}", indexType.toString())
.replace("{" + "indexName" + "}", indexName));
this.indexType = indexType;
this.indexName = indexName;
}
public void setQ(String q) {
this.q = q;
}
public void setFq(List fq) {
this.fq = fq;
}
public void setFl(String fl) {
this.fl = fl;
}
public void setRows(Integer rows) {
this.rows = rows;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public ApiVersion getApiVersion() {
return ApiVersion.V2;
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
if (q != null) {
params.add("q", q);
}
if (fq != null) {
fq.stream().forEach(v -> params.add("fq", v));
}
if (fl != null) {
params.add("fl", fl);
}
if (rows != null) {
params.add("rows", rows.toString());
}
return params;
}
@Override
protected QueryResponse createResponse(SolrClient client) {
return new QueryResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
}