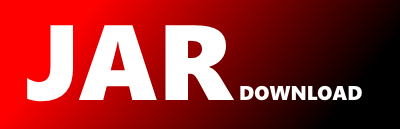
org.apache.solr.client.solrj.request.ShardsApi Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.solr.client.solrj.request;
import java.util.List;
import java.util.Map;
import org.apache.solr.client.api.model.CreateShardRequestBody;
import org.apache.solr.client.api.model.InstallShardDataRequestBody;
import org.apache.solr.client.solrj.JacksonContentWriter;
import org.apache.solr.client.solrj.JacksonParsingResponse;
import org.apache.solr.client.solrj.ResponseParser;
import org.apache.solr.client.solrj.SolrClient;
import org.apache.solr.client.solrj.SolrRequest;
import org.apache.solr.client.solrj.impl.InputStreamResponseParser;
import org.apache.solr.common.params.ModifiableSolrParams;
import org.apache.solr.common.params.SolrParams;
// WARNING: This class is generated from a Mustache template; any intended
// changes should be made to the underlying template and not this file directly.
/**
* Experimental SolrRequest's and SolrResponse's for shards, generated from an OAS.
*
* See individual request and response classes for more detailed and relevant information.
*
*
All SolrRequest implementations rely on v2 APIs which may require a SolrClient configured to
* use the '/api' path prefix, instead of '/solr'.
*
* @lucene.experimental
*/
public class ShardsApi {
public static class CreateShardResponse
extends JacksonParsingResponse<
org.apache.solr.client.api.model.SubResponseAccumulatingJerseyResponse> {
public CreateShardResponse() {
super(org.apache.solr.client.api.model.SubResponseAccumulatingJerseyResponse.class);
}
}
public static class CreateShard extends SolrRequest {
private final CreateShardRequestBody requestBody;
private final String collectionName;
/**
* Create a CreateShard request object.
*
* @param collectionName Path param -
*/
public CreateShard(String collectionName) {
super(
SolrRequest.METHOD.valueOf("POST"),
"/collections/{collectionName}/shards"
.replace("{" + "collectionName" + "}", collectionName));
this.collectionName = collectionName;
this.requestBody = new CreateShardRequestBody();
addHeader("Content-type", "application/json");
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setReplicationFactor(Integer replicationFactor) {
this.requestBody.replicationFactor = replicationFactor;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setNrtReplicas(Integer nrtReplicas) {
this.requestBody.nrtReplicas = nrtReplicas;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setTlogReplicas(Integer tlogReplicas) {
this.requestBody.tlogReplicas = tlogReplicas;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setPullReplicas(Integer pullReplicas) {
this.requestBody.pullReplicas = pullReplicas;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setWaitForFinalState(Boolean waitForFinalState) {
this.requestBody.waitForFinalState = waitForFinalState;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setFollowAliases(Boolean followAliases) {
this.requestBody.followAliases = followAliases;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setAsync(String async) {
this.requestBody.async = async;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setProperties(Map properties) {
this.requestBody.properties = properties;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setShardName(String shardName) {
this.requestBody.shardName = shardName;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setCreateReplicas(Boolean createReplicas) {
this.requestBody.createReplicas = createReplicas;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setNodeSet(List nodeSet) {
this.requestBody.nodeSet = nodeSet;
}
@Override
@SuppressWarnings("unchecked")
public RequestWriter.ContentWriter getContentWriter(String expectedType) {
return new JacksonContentWriter(expectedType, requestBody);
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public ApiVersion getApiVersion() {
return ApiVersion.V2;
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected CreateShardResponse createResponse(SolrClient client) {
return new CreateShardResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class DeleteShardResponse
extends JacksonParsingResponse<
org.apache.solr.client.api.model.SubResponseAccumulatingJerseyResponse> {
public DeleteShardResponse() {
super(org.apache.solr.client.api.model.SubResponseAccumulatingJerseyResponse.class);
}
}
public static class DeleteShard extends SolrRequest {
private final String collectionName;
private final String shardName;
private Boolean deleteInstanceDir;
private Boolean deleteDataDir;
private Boolean deleteIndex;
private Boolean followAliases;
private String async;
/**
* Create a DeleteShard request object.
*
* @param collectionName Path param -
* @param shardName Path param -
*/
public DeleteShard(String collectionName, String shardName) {
super(
SolrRequest.METHOD.valueOf("DELETE"),
"/collections/{collectionName}/shards/{shardName}"
.replace("{" + "collectionName" + "}", collectionName)
.replace("{" + "shardName" + "}", shardName));
this.collectionName = collectionName;
this.shardName = shardName;
}
public void setDeleteInstanceDir(Boolean deleteInstanceDir) {
this.deleteInstanceDir = deleteInstanceDir;
}
public void setDeleteDataDir(Boolean deleteDataDir) {
this.deleteDataDir = deleteDataDir;
}
public void setDeleteIndex(Boolean deleteIndex) {
this.deleteIndex = deleteIndex;
}
public void setFollowAliases(Boolean followAliases) {
this.followAliases = followAliases;
}
public void setAsync(String async) {
this.async = async;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public ApiVersion getApiVersion() {
return ApiVersion.V2;
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
if (deleteInstanceDir != null) {
params.add("deleteInstanceDir", deleteInstanceDir.toString());
}
if (deleteDataDir != null) {
params.add("deleteDataDir", deleteDataDir.toString());
}
if (deleteIndex != null) {
params.add("deleteIndex", deleteIndex.toString());
}
if (followAliases != null) {
params.add("followAliases", followAliases.toString());
}
if (async != null) {
params.add("async", async);
}
return params;
}
@Override
protected DeleteShardResponse createResponse(SolrClient client) {
return new DeleteShardResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class ForceShardLeaderResponse
extends JacksonParsingResponse {
public ForceShardLeaderResponse() {
super(org.apache.solr.client.api.model.SolrJerseyResponse.class);
}
}
public static class ForceShardLeader extends SolrRequest {
private final String collectionName;
private final String shardName;
/**
* Create a ForceShardLeader request object.
*
* @param collectionName Path param -
* @param shardName Path param -
*/
public ForceShardLeader(String collectionName, String shardName) {
super(
SolrRequest.METHOD.valueOf("POST"),
"/collections/{collectionName}/shards/{shardName}/force-leader"
.replace("{" + "collectionName" + "}", collectionName)
.replace("{" + "shardName" + "}", shardName));
this.collectionName = collectionName;
this.shardName = shardName;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public ApiVersion getApiVersion() {
return ApiVersion.V2;
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected ForceShardLeaderResponse createResponse(SolrClient client) {
return new ForceShardLeaderResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class InstallShardDataResponse
extends JacksonParsingResponse {
public InstallShardDataResponse() {
super(org.apache.solr.client.api.model.SolrJerseyResponse.class);
}
}
public static class InstallShardData extends SolrRequest {
private final InstallShardDataRequestBody requestBody;
private final String collName;
private final String shardName;
/**
* Create a InstallShardData request object.
*
* @param collName Path param -
* @param shardName Path param -
*/
public InstallShardData(String collName, String shardName) {
super(
SolrRequest.METHOD.valueOf("POST"),
"/collections/{collName}/shards/{shardName}/install"
.replace("{" + "collName" + "}", collName)
.replace("{" + "shardName" + "}", shardName));
this.collName = collName;
this.shardName = shardName;
this.requestBody = new InstallShardDataRequestBody();
addHeader("Content-type", "application/json");
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setRepository(String repository) {
this.requestBody.repository = repository;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setAsync(String async) {
this.requestBody.async = async;
}
// TODO find a way to add required parameters in the request body to the class constructor
public void setLocation(String location) {
this.requestBody.location = location;
}
@Override
@SuppressWarnings("unchecked")
public RequestWriter.ContentWriter getContentWriter(String expectedType) {
return new JacksonContentWriter(expectedType, requestBody);
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public ApiVersion getApiVersion() {
return ApiVersion.V2;
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected InstallShardDataResponse createResponse(SolrClient client) {
return new InstallShardDataResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
public static class SyncShardResponse
extends JacksonParsingResponse {
public SyncShardResponse() {
super(org.apache.solr.client.api.model.SolrJerseyResponse.class);
}
}
public static class SyncShard extends SolrRequest {
private final String collectionName;
private final String shardName;
/**
* Create a SyncShard request object.
*
* @param collectionName Path param -
* @param shardName Path param -
*/
public SyncShard(String collectionName, String shardName) {
super(
SolrRequest.METHOD.valueOf("POST"),
"/collections/{collectionName}/shards/{shardName}/sync"
.replace("{" + "collectionName" + "}", collectionName)
.replace("{" + "shardName" + "}", shardName));
this.collectionName = collectionName;
this.shardName = shardName;
}
// TODO Hardcode this for now, but in reality we'll want to parse this out of the Operation data
// somehow
@Override
public String getRequestType() {
return SolrRequestType.ADMIN.toString();
}
@Override
public ApiVersion getApiVersion() {
return ApiVersion.V2;
}
@Override
public SolrParams getParams() {
final ModifiableSolrParams params = new ModifiableSolrParams();
return params;
}
@Override
protected SyncShardResponse createResponse(SolrClient client) {
return new SyncShardResponse();
}
@Override
public ResponseParser getResponseParser() {
return new InputStreamResponseParser("json");
}
}
}