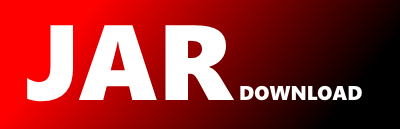
org.apache.tajo.ResourceProtos Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ResourceProtos.proto
package org.apache.tajo;
public final class ResourceProtos {
private ResourceProtos() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
/**
* Protobuf enum {@code ResponseCommand}
*/
public enum ResponseCommand
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NORMAL = 1;
*
*
*ping
*
*/
NORMAL(0, 1),
/**
* MEMBERSHIP = 2;
*
*
* request membership to worker node
*
*/
MEMBERSHIP(1, 2),
/**
* ABORT_QUERY = 3;
*
*
*query master failure
*
*/
ABORT_QUERY(2, 3),
/**
* SHUTDOWN = 4;
*
*
* black list
*
*/
SHUTDOWN(3, 4),
;
/**
* NORMAL = 1;
*
*
*ping
*
*/
public static final int NORMAL_VALUE = 1;
/**
* MEMBERSHIP = 2;
*
*
* request membership to worker node
*
*/
public static final int MEMBERSHIP_VALUE = 2;
/**
* ABORT_QUERY = 3;
*
*
*query master failure
*
*/
public static final int ABORT_QUERY_VALUE = 3;
/**
* SHUTDOWN = 4;
*
*
* black list
*
*/
public static final int SHUTDOWN_VALUE = 4;
public final int getNumber() { return value; }
public static ResponseCommand valueOf(int value) {
switch (value) {
case 1: return NORMAL;
case 2: return MEMBERSHIP;
case 3: return ABORT_QUERY;
case 4: return SHUTDOWN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ResponseCommand findValueByNumber(int number) {
return ResponseCommand.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.getDescriptor().getEnumTypes().get(0);
}
private static final ResponseCommand[] VALUES = values();
public static ResponseCommand valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private ResponseCommand(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:ResponseCommand)
}
/**
* Protobuf enum {@code ResourceType}
*/
public enum ResourceType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* LEAF = 1;
*/
LEAF(0, 1),
/**
* INTERMEDIATE = 2;
*/
INTERMEDIATE(1, 2),
/**
* QUERYMASTER = 3;
*/
QUERYMASTER(2, 3),
;
/**
* LEAF = 1;
*/
public static final int LEAF_VALUE = 1;
/**
* INTERMEDIATE = 2;
*/
public static final int INTERMEDIATE_VALUE = 2;
/**
* QUERYMASTER = 3;
*/
public static final int QUERYMASTER_VALUE = 3;
public final int getNumber() { return value; }
public static ResourceType valueOf(int value) {
switch (value) {
case 1: return LEAF;
case 2: return INTERMEDIATE;
case 3: return QUERYMASTER;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ResourceType findValueByNumber(int number) {
return ResourceType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.getDescriptor().getEnumTypes().get(1);
}
private static final ResourceType[] VALUES = values();
public static ResourceType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private ResourceType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:ResourceType)
}
/**
* Protobuf enum {@code CommandType}
*/
public enum CommandType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* PREPARE = 0;
*/
PREPARE(0, 0),
/**
* LAUNCH = 1;
*/
LAUNCH(1, 1),
/**
* STOP = 2;
*/
STOP(2, 2),
/**
* FINALIZE = 3;
*/
FINALIZE(3, 3),
;
/**
* PREPARE = 0;
*/
public static final int PREPARE_VALUE = 0;
/**
* LAUNCH = 1;
*/
public static final int LAUNCH_VALUE = 1;
/**
* STOP = 2;
*/
public static final int STOP_VALUE = 2;
/**
* FINALIZE = 3;
*/
public static final int FINALIZE_VALUE = 3;
public final int getNumber() { return value; }
public static CommandType valueOf(int value) {
switch (value) {
case 0: return PREPARE;
case 1: return LAUNCH;
case 2: return STOP;
case 3: return FINALIZE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public CommandType findValueByNumber(int number) {
return CommandType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.getDescriptor().getEnumTypes().get(2);
}
private static final CommandType[] VALUES = values();
public static CommandType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private CommandType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:CommandType)
}
public interface NodeStatusProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code NodeStatusProto}
*
*
*TODO add node health information
*
*/
public static final class NodeStatusProto extends
com.google.protobuf.GeneratedMessage
implements NodeStatusProtoOrBuilder {
// Use NodeStatusProto.newBuilder() to construct.
private NodeStatusProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private NodeStatusProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final NodeStatusProto defaultInstance;
public static NodeStatusProto getDefaultInstance() {
return defaultInstance;
}
public NodeStatusProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NodeStatusProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_NodeStatusProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_NodeStatusProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.NodeStatusProto.class, org.apache.tajo.ResourceProtos.NodeStatusProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public NodeStatusProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NodeStatusProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private void initFields() {
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.NodeStatusProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.NodeStatusProto other = (org.apache.tajo.ResourceProtos.NodeStatusProto) obj;
boolean result = true;
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.NodeStatusProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.NodeStatusProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.NodeStatusProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.NodeStatusProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.NodeStatusProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.NodeStatusProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.NodeStatusProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.NodeStatusProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.NodeStatusProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.NodeStatusProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.NodeStatusProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code NodeStatusProto}
*
*
*TODO add node health information
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.NodeStatusProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_NodeStatusProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_NodeStatusProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.NodeStatusProto.class, org.apache.tajo.ResourceProtos.NodeStatusProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.NodeStatusProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_NodeStatusProto_descriptor;
}
public org.apache.tajo.ResourceProtos.NodeStatusProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.NodeStatusProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.NodeStatusProto build() {
org.apache.tajo.ResourceProtos.NodeStatusProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.NodeStatusProto buildPartial() {
org.apache.tajo.ResourceProtos.NodeStatusProto result = new org.apache.tajo.ResourceProtos.NodeStatusProto(this);
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.NodeStatusProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.NodeStatusProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.NodeStatusProto other) {
if (other == org.apache.tajo.ResourceProtos.NodeStatusProto.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.NodeStatusProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.NodeStatusProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
// @@protoc_insertion_point(builder_scope:NodeStatusProto)
}
static {
defaultInstance = new NodeStatusProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:NodeStatusProto)
}
public interface AllocationResourceProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 workerId = 1;
/**
* required int32 workerId = 1;
*/
boolean hasWorkerId();
/**
* required int32 workerId = 1;
*/
int getWorkerId();
// required .NodeResourceProto resource = 2;
/**
* required .NodeResourceProto resource = 2;
*/
boolean hasResource();
/**
* required .NodeResourceProto resource = 2;
*/
org.apache.tajo.TajoProtos.NodeResourceProto getResource();
/**
* required .NodeResourceProto resource = 2;
*/
org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder getResourceOrBuilder();
}
/**
* Protobuf type {@code AllocationResourceProto}
*/
public static final class AllocationResourceProto extends
com.google.protobuf.GeneratedMessage
implements AllocationResourceProtoOrBuilder {
// Use AllocationResourceProto.newBuilder() to construct.
private AllocationResourceProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private AllocationResourceProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final AllocationResourceProto defaultInstance;
public static AllocationResourceProto getDefaultInstance() {
return defaultInstance;
}
public AllocationResourceProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AllocationResourceProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
workerId_ = input.readInt32();
break;
}
case 18: {
org.apache.tajo.TajoProtos.NodeResourceProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = resource_.toBuilder();
}
resource_ = input.readMessage(org.apache.tajo.TajoProtos.NodeResourceProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(resource_);
resource_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_AllocationResourceProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_AllocationResourceProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.AllocationResourceProto.class, org.apache.tajo.ResourceProtos.AllocationResourceProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public AllocationResourceProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AllocationResourceProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required int32 workerId = 1;
public static final int WORKERID_FIELD_NUMBER = 1;
private int workerId_;
/**
* required int32 workerId = 1;
*/
public boolean hasWorkerId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 workerId = 1;
*/
public int getWorkerId() {
return workerId_;
}
// required .NodeResourceProto resource = 2;
public static final int RESOURCE_FIELD_NUMBER = 2;
private org.apache.tajo.TajoProtos.NodeResourceProto resource_;
/**
* required .NodeResourceProto resource = 2;
*/
public boolean hasResource() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .NodeResourceProto resource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProto getResource() {
return resource_;
}
/**
* required .NodeResourceProto resource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder getResourceOrBuilder() {
return resource_;
}
private void initFields() {
workerId_ = 0;
resource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasWorkerId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasResource()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, workerId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, resource_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, workerId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, resource_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.AllocationResourceProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.AllocationResourceProto other = (org.apache.tajo.ResourceProtos.AllocationResourceProto) obj;
boolean result = true;
result = result && (hasWorkerId() == other.hasWorkerId());
if (hasWorkerId()) {
result = result && (getWorkerId()
== other.getWorkerId());
}
result = result && (hasResource() == other.hasResource());
if (hasResource()) {
result = result && getResource()
.equals(other.getResource());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasWorkerId()) {
hash = (37 * hash) + WORKERID_FIELD_NUMBER;
hash = (53 * hash) + getWorkerId();
}
if (hasResource()) {
hash = (37 * hash) + RESOURCE_FIELD_NUMBER;
hash = (53 * hash) + getResource().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.AllocationResourceProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.AllocationResourceProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.AllocationResourceProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.AllocationResourceProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.AllocationResourceProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.AllocationResourceProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.AllocationResourceProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.AllocationResourceProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.AllocationResourceProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.AllocationResourceProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.AllocationResourceProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code AllocationResourceProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.AllocationResourceProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_AllocationResourceProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_AllocationResourceProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.AllocationResourceProto.class, org.apache.tajo.ResourceProtos.AllocationResourceProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.AllocationResourceProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getResourceFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
workerId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
if (resourceBuilder_ == null) {
resource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
} else {
resourceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_AllocationResourceProto_descriptor;
}
public org.apache.tajo.ResourceProtos.AllocationResourceProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.AllocationResourceProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.AllocationResourceProto build() {
org.apache.tajo.ResourceProtos.AllocationResourceProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.AllocationResourceProto buildPartial() {
org.apache.tajo.ResourceProtos.AllocationResourceProto result = new org.apache.tajo.ResourceProtos.AllocationResourceProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.workerId_ = workerId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (resourceBuilder_ == null) {
result.resource_ = resource_;
} else {
result.resource_ = resourceBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.AllocationResourceProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.AllocationResourceProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.AllocationResourceProto other) {
if (other == org.apache.tajo.ResourceProtos.AllocationResourceProto.getDefaultInstance()) return this;
if (other.hasWorkerId()) {
setWorkerId(other.getWorkerId());
}
if (other.hasResource()) {
mergeResource(other.getResource());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasWorkerId()) {
return false;
}
if (!hasResource()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.AllocationResourceProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.AllocationResourceProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int32 workerId = 1;
private int workerId_ ;
/**
* required int32 workerId = 1;
*/
public boolean hasWorkerId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 workerId = 1;
*/
public int getWorkerId() {
return workerId_;
}
/**
* required int32 workerId = 1;
*/
public Builder setWorkerId(int value) {
bitField0_ |= 0x00000001;
workerId_ = value;
onChanged();
return this;
}
/**
* required int32 workerId = 1;
*/
public Builder clearWorkerId() {
bitField0_ = (bitField0_ & ~0x00000001);
workerId_ = 0;
onChanged();
return this;
}
// required .NodeResourceProto resource = 2;
private org.apache.tajo.TajoProtos.NodeResourceProto resource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.NodeResourceProto, org.apache.tajo.TajoProtos.NodeResourceProto.Builder, org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder> resourceBuilder_;
/**
* required .NodeResourceProto resource = 2;
*/
public boolean hasResource() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .NodeResourceProto resource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProto getResource() {
if (resourceBuilder_ == null) {
return resource_;
} else {
return resourceBuilder_.getMessage();
}
}
/**
* required .NodeResourceProto resource = 2;
*/
public Builder setResource(org.apache.tajo.TajoProtos.NodeResourceProto value) {
if (resourceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resource_ = value;
onChanged();
} else {
resourceBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .NodeResourceProto resource = 2;
*/
public Builder setResource(
org.apache.tajo.TajoProtos.NodeResourceProto.Builder builderForValue) {
if (resourceBuilder_ == null) {
resource_ = builderForValue.build();
onChanged();
} else {
resourceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .NodeResourceProto resource = 2;
*/
public Builder mergeResource(org.apache.tajo.TajoProtos.NodeResourceProto value) {
if (resourceBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
resource_ != org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance()) {
resource_ =
org.apache.tajo.TajoProtos.NodeResourceProto.newBuilder(resource_).mergeFrom(value).buildPartial();
} else {
resource_ = value;
}
onChanged();
} else {
resourceBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .NodeResourceProto resource = 2;
*/
public Builder clearResource() {
if (resourceBuilder_ == null) {
resource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
onChanged();
} else {
resourceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* required .NodeResourceProto resource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProto.Builder getResourceBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getResourceFieldBuilder().getBuilder();
}
/**
* required .NodeResourceProto resource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder getResourceOrBuilder() {
if (resourceBuilder_ != null) {
return resourceBuilder_.getMessageOrBuilder();
} else {
return resource_;
}
}
/**
* required .NodeResourceProto resource = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.NodeResourceProto, org.apache.tajo.TajoProtos.NodeResourceProto.Builder, org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder>
getResourceFieldBuilder() {
if (resourceBuilder_ == null) {
resourceBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.NodeResourceProto, org.apache.tajo.TajoProtos.NodeResourceProto.Builder, org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder>(
resource_,
getParentForChildren(),
isClean());
resource_ = null;
}
return resourceBuilder_;
}
// @@protoc_insertion_point(builder_scope:AllocationResourceProto)
}
static {
defaultInstance = new AllocationResourceProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:AllocationResourceProto)
}
public interface ExecutionBlockListProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// repeated .ExecutionBlockIdProto executionBlockId = 1;
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
java.util.List
getExecutionBlockIdList();
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto getExecutionBlockId(int index);
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
int getExecutionBlockIdCount();
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
java.util.List extends org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder>
getExecutionBlockIdOrBuilderList();
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder getExecutionBlockIdOrBuilder(
int index);
}
/**
* Protobuf type {@code ExecutionBlockListProto}
*/
public static final class ExecutionBlockListProto extends
com.google.protobuf.GeneratedMessage
implements ExecutionBlockListProtoOrBuilder {
// Use ExecutionBlockListProto.newBuilder() to construct.
private ExecutionBlockListProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ExecutionBlockListProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ExecutionBlockListProto defaultInstance;
public static ExecutionBlockListProto getDefaultInstance() {
return defaultInstance;
}
public ExecutionBlockListProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ExecutionBlockListProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
executionBlockId_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
executionBlockId_.add(input.readMessage(org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
executionBlockId_ = java.util.Collections.unmodifiableList(executionBlockId_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_ExecutionBlockListProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_ExecutionBlockListProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.ExecutionBlockListProto.class, org.apache.tajo.ResourceProtos.ExecutionBlockListProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ExecutionBlockListProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ExecutionBlockListProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
// repeated .ExecutionBlockIdProto executionBlockId = 1;
public static final int EXECUTIONBLOCKID_FIELD_NUMBER = 1;
private java.util.List executionBlockId_;
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public java.util.List getExecutionBlockIdList() {
return executionBlockId_;
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public java.util.List extends org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder>
getExecutionBlockIdOrBuilderList() {
return executionBlockId_;
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public int getExecutionBlockIdCount() {
return executionBlockId_.size();
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto getExecutionBlockId(int index) {
return executionBlockId_.get(index);
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder getExecutionBlockIdOrBuilder(
int index) {
return executionBlockId_.get(index);
}
private void initFields() {
executionBlockId_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
for (int i = 0; i < getExecutionBlockIdCount(); i++) {
if (!getExecutionBlockId(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < executionBlockId_.size(); i++) {
output.writeMessage(1, executionBlockId_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < executionBlockId_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, executionBlockId_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.ExecutionBlockListProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.ExecutionBlockListProto other = (org.apache.tajo.ResourceProtos.ExecutionBlockListProto) obj;
boolean result = true;
result = result && getExecutionBlockIdList()
.equals(other.getExecutionBlockIdList());
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (getExecutionBlockIdCount() > 0) {
hash = (37 * hash) + EXECUTIONBLOCKID_FIELD_NUMBER;
hash = (53 * hash) + getExecutionBlockIdList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockListProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockListProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockListProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockListProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockListProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockListProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockListProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockListProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockListProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockListProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.ExecutionBlockListProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ExecutionBlockListProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.ExecutionBlockListProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_ExecutionBlockListProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_ExecutionBlockListProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.ExecutionBlockListProto.class, org.apache.tajo.ResourceProtos.ExecutionBlockListProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.ExecutionBlockListProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getExecutionBlockIdFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (executionBlockIdBuilder_ == null) {
executionBlockId_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
executionBlockIdBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_ExecutionBlockListProto_descriptor;
}
public org.apache.tajo.ResourceProtos.ExecutionBlockListProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.ExecutionBlockListProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.ExecutionBlockListProto build() {
org.apache.tajo.ResourceProtos.ExecutionBlockListProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.ExecutionBlockListProto buildPartial() {
org.apache.tajo.ResourceProtos.ExecutionBlockListProto result = new org.apache.tajo.ResourceProtos.ExecutionBlockListProto(this);
int from_bitField0_ = bitField0_;
if (executionBlockIdBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
executionBlockId_ = java.util.Collections.unmodifiableList(executionBlockId_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.executionBlockId_ = executionBlockId_;
} else {
result.executionBlockId_ = executionBlockIdBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.ExecutionBlockListProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.ExecutionBlockListProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.ExecutionBlockListProto other) {
if (other == org.apache.tajo.ResourceProtos.ExecutionBlockListProto.getDefaultInstance()) return this;
if (executionBlockIdBuilder_ == null) {
if (!other.executionBlockId_.isEmpty()) {
if (executionBlockId_.isEmpty()) {
executionBlockId_ = other.executionBlockId_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureExecutionBlockIdIsMutable();
executionBlockId_.addAll(other.executionBlockId_);
}
onChanged();
}
} else {
if (!other.executionBlockId_.isEmpty()) {
if (executionBlockIdBuilder_.isEmpty()) {
executionBlockIdBuilder_.dispose();
executionBlockIdBuilder_ = null;
executionBlockId_ = other.executionBlockId_;
bitField0_ = (bitField0_ & ~0x00000001);
executionBlockIdBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getExecutionBlockIdFieldBuilder() : null;
} else {
executionBlockIdBuilder_.addAllMessages(other.executionBlockId_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getExecutionBlockIdCount(); i++) {
if (!getExecutionBlockId(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.ExecutionBlockListProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.ExecutionBlockListProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated .ExecutionBlockIdProto executionBlockId = 1;
private java.util.List executionBlockId_ =
java.util.Collections.emptyList();
private void ensureExecutionBlockIdIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
executionBlockId_ = new java.util.ArrayList(executionBlockId_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder> executionBlockIdBuilder_;
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public java.util.List getExecutionBlockIdList() {
if (executionBlockIdBuilder_ == null) {
return java.util.Collections.unmodifiableList(executionBlockId_);
} else {
return executionBlockIdBuilder_.getMessageList();
}
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public int getExecutionBlockIdCount() {
if (executionBlockIdBuilder_ == null) {
return executionBlockId_.size();
} else {
return executionBlockIdBuilder_.getCount();
}
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto getExecutionBlockId(int index) {
if (executionBlockIdBuilder_ == null) {
return executionBlockId_.get(index);
} else {
return executionBlockIdBuilder_.getMessage(index);
}
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public Builder setExecutionBlockId(
int index, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto value) {
if (executionBlockIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExecutionBlockIdIsMutable();
executionBlockId_.set(index, value);
onChanged();
} else {
executionBlockIdBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public Builder setExecutionBlockId(
int index, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder builderForValue) {
if (executionBlockIdBuilder_ == null) {
ensureExecutionBlockIdIsMutable();
executionBlockId_.set(index, builderForValue.build());
onChanged();
} else {
executionBlockIdBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public Builder addExecutionBlockId(org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto value) {
if (executionBlockIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExecutionBlockIdIsMutable();
executionBlockId_.add(value);
onChanged();
} else {
executionBlockIdBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public Builder addExecutionBlockId(
int index, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto value) {
if (executionBlockIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExecutionBlockIdIsMutable();
executionBlockId_.add(index, value);
onChanged();
} else {
executionBlockIdBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public Builder addExecutionBlockId(
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder builderForValue) {
if (executionBlockIdBuilder_ == null) {
ensureExecutionBlockIdIsMutable();
executionBlockId_.add(builderForValue.build());
onChanged();
} else {
executionBlockIdBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public Builder addExecutionBlockId(
int index, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder builderForValue) {
if (executionBlockIdBuilder_ == null) {
ensureExecutionBlockIdIsMutable();
executionBlockId_.add(index, builderForValue.build());
onChanged();
} else {
executionBlockIdBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public Builder addAllExecutionBlockId(
java.lang.Iterable extends org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto> values) {
if (executionBlockIdBuilder_ == null) {
ensureExecutionBlockIdIsMutable();
super.addAll(values, executionBlockId_);
onChanged();
} else {
executionBlockIdBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public Builder clearExecutionBlockId() {
if (executionBlockIdBuilder_ == null) {
executionBlockId_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
executionBlockIdBuilder_.clear();
}
return this;
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public Builder removeExecutionBlockId(int index) {
if (executionBlockIdBuilder_ == null) {
ensureExecutionBlockIdIsMutable();
executionBlockId_.remove(index);
onChanged();
} else {
executionBlockIdBuilder_.remove(index);
}
return this;
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder getExecutionBlockIdBuilder(
int index) {
return getExecutionBlockIdFieldBuilder().getBuilder(index);
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder getExecutionBlockIdOrBuilder(
int index) {
if (executionBlockIdBuilder_ == null) {
return executionBlockId_.get(index); } else {
return executionBlockIdBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public java.util.List extends org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder>
getExecutionBlockIdOrBuilderList() {
if (executionBlockIdBuilder_ != null) {
return executionBlockIdBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(executionBlockId_);
}
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder addExecutionBlockIdBuilder() {
return getExecutionBlockIdFieldBuilder().addBuilder(
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance());
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder addExecutionBlockIdBuilder(
int index) {
return getExecutionBlockIdFieldBuilder().addBuilder(
index, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance());
}
/**
* repeated .ExecutionBlockIdProto executionBlockId = 1;
*/
public java.util.List
getExecutionBlockIdBuilderList() {
return getExecutionBlockIdFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder>
getExecutionBlockIdFieldBuilder() {
if (executionBlockIdBuilder_ == null) {
executionBlockIdBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder>(
executionBlockId_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
executionBlockId_ = null;
}
return executionBlockIdBuilder_;
}
// @@protoc_insertion_point(builder_scope:ExecutionBlockListProto)
}
static {
defaultInstance = new ExecutionBlockListProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ExecutionBlockListProto)
}
public interface TaskAllocationProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required .TaskRequestProto taskRequest = 1;
/**
* required .TaskRequestProto taskRequest = 1;
*/
boolean hasTaskRequest();
/**
* required .TaskRequestProto taskRequest = 1;
*/
org.apache.tajo.ResourceProtos.TaskRequestProto getTaskRequest();
/**
* required .TaskRequestProto taskRequest = 1;
*/
org.apache.tajo.ResourceProtos.TaskRequestProtoOrBuilder getTaskRequestOrBuilder();
// required .NodeResourceProto resource = 2;
/**
* required .NodeResourceProto resource = 2;
*/
boolean hasResource();
/**
* required .NodeResourceProto resource = 2;
*/
org.apache.tajo.TajoProtos.NodeResourceProto getResource();
/**
* required .NodeResourceProto resource = 2;
*/
org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder getResourceOrBuilder();
}
/**
* Protobuf type {@code TaskAllocationProto}
*/
public static final class TaskAllocationProto extends
com.google.protobuf.GeneratedMessage
implements TaskAllocationProtoOrBuilder {
// Use TaskAllocationProto.newBuilder() to construct.
private TaskAllocationProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TaskAllocationProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TaskAllocationProto defaultInstance;
public static TaskAllocationProto getDefaultInstance() {
return defaultInstance;
}
public TaskAllocationProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TaskAllocationProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.tajo.ResourceProtos.TaskRequestProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = taskRequest_.toBuilder();
}
taskRequest_ = input.readMessage(org.apache.tajo.ResourceProtos.TaskRequestProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(taskRequest_);
taskRequest_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
org.apache.tajo.TajoProtos.NodeResourceProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = resource_.toBuilder();
}
resource_ = input.readMessage(org.apache.tajo.TajoProtos.NodeResourceProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(resource_);
resource_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TaskAllocationProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TaskAllocationProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TaskAllocationProto.class, org.apache.tajo.ResourceProtos.TaskAllocationProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TaskAllocationProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TaskAllocationProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .TaskRequestProto taskRequest = 1;
public static final int TASKREQUEST_FIELD_NUMBER = 1;
private org.apache.tajo.ResourceProtos.TaskRequestProto taskRequest_;
/**
* required .TaskRequestProto taskRequest = 1;
*/
public boolean hasTaskRequest() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .TaskRequestProto taskRequest = 1;
*/
public org.apache.tajo.ResourceProtos.TaskRequestProto getTaskRequest() {
return taskRequest_;
}
/**
* required .TaskRequestProto taskRequest = 1;
*/
public org.apache.tajo.ResourceProtos.TaskRequestProtoOrBuilder getTaskRequestOrBuilder() {
return taskRequest_;
}
// required .NodeResourceProto resource = 2;
public static final int RESOURCE_FIELD_NUMBER = 2;
private org.apache.tajo.TajoProtos.NodeResourceProto resource_;
/**
* required .NodeResourceProto resource = 2;
*/
public boolean hasResource() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .NodeResourceProto resource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProto getResource() {
return resource_;
}
/**
* required .NodeResourceProto resource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder getResourceOrBuilder() {
return resource_;
}
private void initFields() {
taskRequest_ = org.apache.tajo.ResourceProtos.TaskRequestProto.getDefaultInstance();
resource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasTaskRequest()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasResource()) {
memoizedIsInitialized = 0;
return false;
}
if (!getTaskRequest().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, taskRequest_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, resource_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, taskRequest_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, resource_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.TaskAllocationProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.TaskAllocationProto other = (org.apache.tajo.ResourceProtos.TaskAllocationProto) obj;
boolean result = true;
result = result && (hasTaskRequest() == other.hasTaskRequest());
if (hasTaskRequest()) {
result = result && getTaskRequest()
.equals(other.getTaskRequest());
}
result = result && (hasResource() == other.hasResource());
if (hasResource()) {
result = result && getResource()
.equals(other.getResource());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasTaskRequest()) {
hash = (37 * hash) + TASKREQUEST_FIELD_NUMBER;
hash = (53 * hash) + getTaskRequest().hashCode();
}
if (hasResource()) {
hash = (37 * hash) + RESOURCE_FIELD_NUMBER;
hash = (53 * hash) + getResource().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.TaskAllocationProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TaskAllocationProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskAllocationProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TaskAllocationProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskAllocationProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskAllocationProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskAllocationProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskAllocationProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskAllocationProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskAllocationProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.TaskAllocationProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TaskAllocationProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.TaskAllocationProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TaskAllocationProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TaskAllocationProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TaskAllocationProto.class, org.apache.tajo.ResourceProtos.TaskAllocationProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.TaskAllocationProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getTaskRequestFieldBuilder();
getResourceFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (taskRequestBuilder_ == null) {
taskRequest_ = org.apache.tajo.ResourceProtos.TaskRequestProto.getDefaultInstance();
} else {
taskRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (resourceBuilder_ == null) {
resource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
} else {
resourceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_TaskAllocationProto_descriptor;
}
public org.apache.tajo.ResourceProtos.TaskAllocationProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.TaskAllocationProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.TaskAllocationProto build() {
org.apache.tajo.ResourceProtos.TaskAllocationProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.TaskAllocationProto buildPartial() {
org.apache.tajo.ResourceProtos.TaskAllocationProto result = new org.apache.tajo.ResourceProtos.TaskAllocationProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (taskRequestBuilder_ == null) {
result.taskRequest_ = taskRequest_;
} else {
result.taskRequest_ = taskRequestBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (resourceBuilder_ == null) {
result.resource_ = resource_;
} else {
result.resource_ = resourceBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.TaskAllocationProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.TaskAllocationProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.TaskAllocationProto other) {
if (other == org.apache.tajo.ResourceProtos.TaskAllocationProto.getDefaultInstance()) return this;
if (other.hasTaskRequest()) {
mergeTaskRequest(other.getTaskRequest());
}
if (other.hasResource()) {
mergeResource(other.getResource());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasTaskRequest()) {
return false;
}
if (!hasResource()) {
return false;
}
if (!getTaskRequest().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.TaskAllocationProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.TaskAllocationProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .TaskRequestProto taskRequest = 1;
private org.apache.tajo.ResourceProtos.TaskRequestProto taskRequest_ = org.apache.tajo.ResourceProtos.TaskRequestProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.ResourceProtos.TaskRequestProto, org.apache.tajo.ResourceProtos.TaskRequestProto.Builder, org.apache.tajo.ResourceProtos.TaskRequestProtoOrBuilder> taskRequestBuilder_;
/**
* required .TaskRequestProto taskRequest = 1;
*/
public boolean hasTaskRequest() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .TaskRequestProto taskRequest = 1;
*/
public org.apache.tajo.ResourceProtos.TaskRequestProto getTaskRequest() {
if (taskRequestBuilder_ == null) {
return taskRequest_;
} else {
return taskRequestBuilder_.getMessage();
}
}
/**
* required .TaskRequestProto taskRequest = 1;
*/
public Builder setTaskRequest(org.apache.tajo.ResourceProtos.TaskRequestProto value) {
if (taskRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
taskRequest_ = value;
onChanged();
} else {
taskRequestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskRequestProto taskRequest = 1;
*/
public Builder setTaskRequest(
org.apache.tajo.ResourceProtos.TaskRequestProto.Builder builderForValue) {
if (taskRequestBuilder_ == null) {
taskRequest_ = builderForValue.build();
onChanged();
} else {
taskRequestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskRequestProto taskRequest = 1;
*/
public Builder mergeTaskRequest(org.apache.tajo.ResourceProtos.TaskRequestProto value) {
if (taskRequestBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
taskRequest_ != org.apache.tajo.ResourceProtos.TaskRequestProto.getDefaultInstance()) {
taskRequest_ =
org.apache.tajo.ResourceProtos.TaskRequestProto.newBuilder(taskRequest_).mergeFrom(value).buildPartial();
} else {
taskRequest_ = value;
}
onChanged();
} else {
taskRequestBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskRequestProto taskRequest = 1;
*/
public Builder clearTaskRequest() {
if (taskRequestBuilder_ == null) {
taskRequest_ = org.apache.tajo.ResourceProtos.TaskRequestProto.getDefaultInstance();
onChanged();
} else {
taskRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .TaskRequestProto taskRequest = 1;
*/
public org.apache.tajo.ResourceProtos.TaskRequestProto.Builder getTaskRequestBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getTaskRequestFieldBuilder().getBuilder();
}
/**
* required .TaskRequestProto taskRequest = 1;
*/
public org.apache.tajo.ResourceProtos.TaskRequestProtoOrBuilder getTaskRequestOrBuilder() {
if (taskRequestBuilder_ != null) {
return taskRequestBuilder_.getMessageOrBuilder();
} else {
return taskRequest_;
}
}
/**
* required .TaskRequestProto taskRequest = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.ResourceProtos.TaskRequestProto, org.apache.tajo.ResourceProtos.TaskRequestProto.Builder, org.apache.tajo.ResourceProtos.TaskRequestProtoOrBuilder>
getTaskRequestFieldBuilder() {
if (taskRequestBuilder_ == null) {
taskRequestBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.ResourceProtos.TaskRequestProto, org.apache.tajo.ResourceProtos.TaskRequestProto.Builder, org.apache.tajo.ResourceProtos.TaskRequestProtoOrBuilder>(
taskRequest_,
getParentForChildren(),
isClean());
taskRequest_ = null;
}
return taskRequestBuilder_;
}
// required .NodeResourceProto resource = 2;
private org.apache.tajo.TajoProtos.NodeResourceProto resource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.NodeResourceProto, org.apache.tajo.TajoProtos.NodeResourceProto.Builder, org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder> resourceBuilder_;
/**
* required .NodeResourceProto resource = 2;
*/
public boolean hasResource() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .NodeResourceProto resource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProto getResource() {
if (resourceBuilder_ == null) {
return resource_;
} else {
return resourceBuilder_.getMessage();
}
}
/**
* required .NodeResourceProto resource = 2;
*/
public Builder setResource(org.apache.tajo.TajoProtos.NodeResourceProto value) {
if (resourceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resource_ = value;
onChanged();
} else {
resourceBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .NodeResourceProto resource = 2;
*/
public Builder setResource(
org.apache.tajo.TajoProtos.NodeResourceProto.Builder builderForValue) {
if (resourceBuilder_ == null) {
resource_ = builderForValue.build();
onChanged();
} else {
resourceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .NodeResourceProto resource = 2;
*/
public Builder mergeResource(org.apache.tajo.TajoProtos.NodeResourceProto value) {
if (resourceBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
resource_ != org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance()) {
resource_ =
org.apache.tajo.TajoProtos.NodeResourceProto.newBuilder(resource_).mergeFrom(value).buildPartial();
} else {
resource_ = value;
}
onChanged();
} else {
resourceBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .NodeResourceProto resource = 2;
*/
public Builder clearResource() {
if (resourceBuilder_ == null) {
resource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
onChanged();
} else {
resourceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* required .NodeResourceProto resource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProto.Builder getResourceBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getResourceFieldBuilder().getBuilder();
}
/**
* required .NodeResourceProto resource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder getResourceOrBuilder() {
if (resourceBuilder_ != null) {
return resourceBuilder_.getMessageOrBuilder();
} else {
return resource_;
}
}
/**
* required .NodeResourceProto resource = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.NodeResourceProto, org.apache.tajo.TajoProtos.NodeResourceProto.Builder, org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder>
getResourceFieldBuilder() {
if (resourceBuilder_ == null) {
resourceBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.NodeResourceProto, org.apache.tajo.TajoProtos.NodeResourceProto.Builder, org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder>(
resource_,
getParentForChildren(),
isClean());
resource_ = null;
}
return resourceBuilder_;
}
// @@protoc_insertion_point(builder_scope:TaskAllocationProto)
}
static {
defaultInstance = new TaskAllocationProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TaskAllocationProto)
}
public interface TaskRequestProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string queryMasterHostAndPort = 1;
/**
* required string queryMasterHostAndPort = 1;
*/
boolean hasQueryMasterHostAndPort();
/**
* required string queryMasterHostAndPort = 1;
*/
java.lang.String getQueryMasterHostAndPort();
/**
* required string queryMasterHostAndPort = 1;
*/
com.google.protobuf.ByteString
getQueryMasterHostAndPortBytes();
// required .TaskAttemptIdProto id = 2;
/**
* required .TaskAttemptIdProto id = 2;
*/
boolean hasId();
/**
* required .TaskAttemptIdProto id = 2;
*/
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId();
/**
* required .TaskAttemptIdProto id = 2;
*/
org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder();
// repeated .FragmentProto fragments = 3;
/**
* repeated .FragmentProto fragments = 3;
*/
java.util.List
getFragmentsList();
/**
* repeated .FragmentProto fragments = 3;
*/
org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto getFragments(int index);
/**
* repeated .FragmentProto fragments = 3;
*/
int getFragmentsCount();
/**
* repeated .FragmentProto fragments = 3;
*/
java.util.List extends org.apache.tajo.catalog.proto.CatalogProtos.FragmentProtoOrBuilder>
getFragmentsOrBuilderList();
/**
* repeated .FragmentProto fragments = 3;
*/
org.apache.tajo.catalog.proto.CatalogProtos.FragmentProtoOrBuilder getFragmentsOrBuilder(
int index);
// required string outputTable = 4;
/**
* required string outputTable = 4;
*/
boolean hasOutputTable();
/**
* required string outputTable = 4;
*/
java.lang.String getOutputTable();
/**
* required string outputTable = 4;
*/
com.google.protobuf.ByteString
getOutputTableBytes();
// required bool clusteredOutput = 5;
/**
* required bool clusteredOutput = 5;
*/
boolean hasClusteredOutput();
/**
* required bool clusteredOutput = 5;
*/
boolean getClusteredOutput();
// required .LogicalNodeTree plan = 6;
/**
* required .LogicalNodeTree plan = 6;
*/
boolean hasPlan();
/**
* required .LogicalNodeTree plan = 6;
*/
org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree getPlan();
/**
* required .LogicalNodeTree plan = 6;
*/
org.apache.tajo.plan.serder.PlanProto.LogicalNodeTreeOrBuilder getPlanOrBuilder();
// optional bool interQuery = 7 [default = false];
/**
* optional bool interQuery = 7 [default = false];
*/
boolean hasInterQuery();
/**
* optional bool interQuery = 7 [default = false];
*/
boolean getInterQuery();
// repeated .FetchProto fetches = 8;
/**
* repeated .FetchProto fetches = 8;
*/
java.util.List
getFetchesList();
/**
* repeated .FetchProto fetches = 8;
*/
org.apache.tajo.ResourceProtos.FetchProto getFetches(int index);
/**
* repeated .FetchProto fetches = 8;
*/
int getFetchesCount();
/**
* repeated .FetchProto fetches = 8;
*/
java.util.List extends org.apache.tajo.ResourceProtos.FetchProtoOrBuilder>
getFetchesOrBuilderList();
/**
* repeated .FetchProto fetches = 8;
*/
org.apache.tajo.ResourceProtos.FetchProtoOrBuilder getFetchesOrBuilder(
int index);
// optional .KeyValueSetProto queryContext = 9;
/**
* optional .KeyValueSetProto queryContext = 9;
*/
boolean hasQueryContext();
/**
* optional .KeyValueSetProto queryContext = 9;
*/
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto getQueryContext();
/**
* optional .KeyValueSetProto queryContext = 9;
*/
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProtoOrBuilder getQueryContextOrBuilder();
// optional .DataChannelProto dataChannel = 10;
/**
* optional .DataChannelProto dataChannel = 10;
*/
boolean hasDataChannel();
/**
* optional .DataChannelProto dataChannel = 10;
*/
org.apache.tajo.plan.serder.PlanProto.DataChannelProto getDataChannel();
/**
* optional .DataChannelProto dataChannel = 10;
*/
org.apache.tajo.plan.serder.PlanProto.DataChannelProtoOrBuilder getDataChannelOrBuilder();
// optional .EnforcerProto enforcer = 11;
/**
* optional .EnforcerProto enforcer = 11;
*/
boolean hasEnforcer();
/**
* optional .EnforcerProto enforcer = 11;
*/
org.apache.tajo.plan.serder.PlanProto.EnforcerProto getEnforcer();
/**
* optional .EnforcerProto enforcer = 11;
*/
org.apache.tajo.plan.serder.PlanProto.EnforcerProtoOrBuilder getEnforcerOrBuilder();
}
/**
* Protobuf type {@code TaskRequestProto}
*/
public static final class TaskRequestProto extends
com.google.protobuf.GeneratedMessage
implements TaskRequestProtoOrBuilder {
// Use TaskRequestProto.newBuilder() to construct.
private TaskRequestProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TaskRequestProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TaskRequestProto defaultInstance;
public static TaskRequestProto getDefaultInstance() {
return defaultInstance;
}
public TaskRequestProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TaskRequestProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
queryMasterHostAndPort_ = input.readBytes();
break;
}
case 18: {
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = id_.toBuilder();
}
id_ = input.readMessage(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(id_);
id_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
fragments_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
fragments_.add(input.readMessage(org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto.PARSER, extensionRegistry));
break;
}
case 34: {
bitField0_ |= 0x00000004;
outputTable_ = input.readBytes();
break;
}
case 40: {
bitField0_ |= 0x00000008;
clusteredOutput_ = input.readBool();
break;
}
case 50: {
org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = plan_.toBuilder();
}
plan_ = input.readMessage(org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(plan_);
plan_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 56: {
bitField0_ |= 0x00000020;
interQuery_ = input.readBool();
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
fetches_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000080;
}
fetches_.add(input.readMessage(org.apache.tajo.ResourceProtos.FetchProto.PARSER, extensionRegistry));
break;
}
case 74: {
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
subBuilder = queryContext_.toBuilder();
}
queryContext_ = input.readMessage(org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(queryContext_);
queryContext_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
case 82: {
org.apache.tajo.plan.serder.PlanProto.DataChannelProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000080) == 0x00000080)) {
subBuilder = dataChannel_.toBuilder();
}
dataChannel_ = input.readMessage(org.apache.tajo.plan.serder.PlanProto.DataChannelProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(dataChannel_);
dataChannel_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000080;
break;
}
case 90: {
org.apache.tajo.plan.serder.PlanProto.EnforcerProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
subBuilder = enforcer_.toBuilder();
}
enforcer_ = input.readMessage(org.apache.tajo.plan.serder.PlanProto.EnforcerProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(enforcer_);
enforcer_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000100;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
fragments_ = java.util.Collections.unmodifiableList(fragments_);
}
if (((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
fetches_ = java.util.Collections.unmodifiableList(fetches_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TaskRequestProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TaskRequestProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TaskRequestProto.class, org.apache.tajo.ResourceProtos.TaskRequestProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TaskRequestProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TaskRequestProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string queryMasterHostAndPort = 1;
public static final int QUERYMASTERHOSTANDPORT_FIELD_NUMBER = 1;
private java.lang.Object queryMasterHostAndPort_;
/**
* required string queryMasterHostAndPort = 1;
*/
public boolean hasQueryMasterHostAndPort() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string queryMasterHostAndPort = 1;
*/
public java.lang.String getQueryMasterHostAndPort() {
java.lang.Object ref = queryMasterHostAndPort_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
queryMasterHostAndPort_ = s;
}
return s;
}
}
/**
* required string queryMasterHostAndPort = 1;
*/
public com.google.protobuf.ByteString
getQueryMasterHostAndPortBytes() {
java.lang.Object ref = queryMasterHostAndPort_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
queryMasterHostAndPort_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required .TaskAttemptIdProto id = 2;
public static final int ID_FIELD_NUMBER = 2;
private org.apache.tajo.TajoIdProtos.TaskAttemptIdProto id_;
/**
* required .TaskAttemptIdProto id = 2;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .TaskAttemptIdProto id = 2;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId() {
return id_;
}
/**
* required .TaskAttemptIdProto id = 2;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder() {
return id_;
}
// repeated .FragmentProto fragments = 3;
public static final int FRAGMENTS_FIELD_NUMBER = 3;
private java.util.List fragments_;
/**
* repeated .FragmentProto fragments = 3;
*/
public java.util.List getFragmentsList() {
return fragments_;
}
/**
* repeated .FragmentProto fragments = 3;
*/
public java.util.List extends org.apache.tajo.catalog.proto.CatalogProtos.FragmentProtoOrBuilder>
getFragmentsOrBuilderList() {
return fragments_;
}
/**
* repeated .FragmentProto fragments = 3;
*/
public int getFragmentsCount() {
return fragments_.size();
}
/**
* repeated .FragmentProto fragments = 3;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto getFragments(int index) {
return fragments_.get(index);
}
/**
* repeated .FragmentProto fragments = 3;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.FragmentProtoOrBuilder getFragmentsOrBuilder(
int index) {
return fragments_.get(index);
}
// required string outputTable = 4;
public static final int OUTPUTTABLE_FIELD_NUMBER = 4;
private java.lang.Object outputTable_;
/**
* required string outputTable = 4;
*/
public boolean hasOutputTable() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required string outputTable = 4;
*/
public java.lang.String getOutputTable() {
java.lang.Object ref = outputTable_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
outputTable_ = s;
}
return s;
}
}
/**
* required string outputTable = 4;
*/
public com.google.protobuf.ByteString
getOutputTableBytes() {
java.lang.Object ref = outputTable_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outputTable_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required bool clusteredOutput = 5;
public static final int CLUSTEREDOUTPUT_FIELD_NUMBER = 5;
private boolean clusteredOutput_;
/**
* required bool clusteredOutput = 5;
*/
public boolean hasClusteredOutput() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required bool clusteredOutput = 5;
*/
public boolean getClusteredOutput() {
return clusteredOutput_;
}
// required .LogicalNodeTree plan = 6;
public static final int PLAN_FIELD_NUMBER = 6;
private org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree plan_;
/**
* required .LogicalNodeTree plan = 6;
*/
public boolean hasPlan() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required .LogicalNodeTree plan = 6;
*/
public org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree getPlan() {
return plan_;
}
/**
* required .LogicalNodeTree plan = 6;
*/
public org.apache.tajo.plan.serder.PlanProto.LogicalNodeTreeOrBuilder getPlanOrBuilder() {
return plan_;
}
// optional bool interQuery = 7 [default = false];
public static final int INTERQUERY_FIELD_NUMBER = 7;
private boolean interQuery_;
/**
* optional bool interQuery = 7 [default = false];
*/
public boolean hasInterQuery() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bool interQuery = 7 [default = false];
*/
public boolean getInterQuery() {
return interQuery_;
}
// repeated .FetchProto fetches = 8;
public static final int FETCHES_FIELD_NUMBER = 8;
private java.util.List fetches_;
/**
* repeated .FetchProto fetches = 8;
*/
public java.util.List getFetchesList() {
return fetches_;
}
/**
* repeated .FetchProto fetches = 8;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.FetchProtoOrBuilder>
getFetchesOrBuilderList() {
return fetches_;
}
/**
* repeated .FetchProto fetches = 8;
*/
public int getFetchesCount() {
return fetches_.size();
}
/**
* repeated .FetchProto fetches = 8;
*/
public org.apache.tajo.ResourceProtos.FetchProto getFetches(int index) {
return fetches_.get(index);
}
/**
* repeated .FetchProto fetches = 8;
*/
public org.apache.tajo.ResourceProtos.FetchProtoOrBuilder getFetchesOrBuilder(
int index) {
return fetches_.get(index);
}
// optional .KeyValueSetProto queryContext = 9;
public static final int QUERYCONTEXT_FIELD_NUMBER = 9;
private org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto queryContext_;
/**
* optional .KeyValueSetProto queryContext = 9;
*/
public boolean hasQueryContext() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .KeyValueSetProto queryContext = 9;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto getQueryContext() {
return queryContext_;
}
/**
* optional .KeyValueSetProto queryContext = 9;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProtoOrBuilder getQueryContextOrBuilder() {
return queryContext_;
}
// optional .DataChannelProto dataChannel = 10;
public static final int DATACHANNEL_FIELD_NUMBER = 10;
private org.apache.tajo.plan.serder.PlanProto.DataChannelProto dataChannel_;
/**
* optional .DataChannelProto dataChannel = 10;
*/
public boolean hasDataChannel() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional .DataChannelProto dataChannel = 10;
*/
public org.apache.tajo.plan.serder.PlanProto.DataChannelProto getDataChannel() {
return dataChannel_;
}
/**
* optional .DataChannelProto dataChannel = 10;
*/
public org.apache.tajo.plan.serder.PlanProto.DataChannelProtoOrBuilder getDataChannelOrBuilder() {
return dataChannel_;
}
// optional .EnforcerProto enforcer = 11;
public static final int ENFORCER_FIELD_NUMBER = 11;
private org.apache.tajo.plan.serder.PlanProto.EnforcerProto enforcer_;
/**
* optional .EnforcerProto enforcer = 11;
*/
public boolean hasEnforcer() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .EnforcerProto enforcer = 11;
*/
public org.apache.tajo.plan.serder.PlanProto.EnforcerProto getEnforcer() {
return enforcer_;
}
/**
* optional .EnforcerProto enforcer = 11;
*/
public org.apache.tajo.plan.serder.PlanProto.EnforcerProtoOrBuilder getEnforcerOrBuilder() {
return enforcer_;
}
private void initFields() {
queryMasterHostAndPort_ = "";
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
fragments_ = java.util.Collections.emptyList();
outputTable_ = "";
clusteredOutput_ = false;
plan_ = org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree.getDefaultInstance();
interQuery_ = false;
fetches_ = java.util.Collections.emptyList();
queryContext_ = org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.getDefaultInstance();
dataChannel_ = org.apache.tajo.plan.serder.PlanProto.DataChannelProto.getDefaultInstance();
enforcer_ = org.apache.tajo.plan.serder.PlanProto.EnforcerProto.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasQueryMasterHostAndPort()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasOutputTable()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasClusteredOutput()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPlan()) {
memoizedIsInitialized = 0;
return false;
}
if (!getId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getFragmentsCount(); i++) {
if (!getFragments(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (!getPlan().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getFetchesCount(); i++) {
if (!getFetches(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasQueryContext()) {
if (!getQueryContext().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasDataChannel()) {
if (!getDataChannel().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasEnforcer()) {
if (!getEnforcer().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getQueryMasterHostAndPortBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, id_);
}
for (int i = 0; i < fragments_.size(); i++) {
output.writeMessage(3, fragments_.get(i));
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(4, getOutputTableBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(5, clusteredOutput_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(6, plan_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBool(7, interQuery_);
}
for (int i = 0; i < fetches_.size(); i++) {
output.writeMessage(8, fetches_.get(i));
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeMessage(9, queryContext_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeMessage(10, dataChannel_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeMessage(11, enforcer_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getQueryMasterHostAndPortBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, id_);
}
for (int i = 0; i < fragments_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, fragments_.get(i));
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getOutputTableBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, clusteredOutput_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, plan_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(7, interQuery_);
}
for (int i = 0; i < fetches_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, fetches_.get(i));
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, queryContext_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, dataChannel_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, enforcer_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.TaskRequestProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.TaskRequestProto other = (org.apache.tajo.ResourceProtos.TaskRequestProto) obj;
boolean result = true;
result = result && (hasQueryMasterHostAndPort() == other.hasQueryMasterHostAndPort());
if (hasQueryMasterHostAndPort()) {
result = result && getQueryMasterHostAndPort()
.equals(other.getQueryMasterHostAndPort());
}
result = result && (hasId() == other.hasId());
if (hasId()) {
result = result && getId()
.equals(other.getId());
}
result = result && getFragmentsList()
.equals(other.getFragmentsList());
result = result && (hasOutputTable() == other.hasOutputTable());
if (hasOutputTable()) {
result = result && getOutputTable()
.equals(other.getOutputTable());
}
result = result && (hasClusteredOutput() == other.hasClusteredOutput());
if (hasClusteredOutput()) {
result = result && (getClusteredOutput()
== other.getClusteredOutput());
}
result = result && (hasPlan() == other.hasPlan());
if (hasPlan()) {
result = result && getPlan()
.equals(other.getPlan());
}
result = result && (hasInterQuery() == other.hasInterQuery());
if (hasInterQuery()) {
result = result && (getInterQuery()
== other.getInterQuery());
}
result = result && getFetchesList()
.equals(other.getFetchesList());
result = result && (hasQueryContext() == other.hasQueryContext());
if (hasQueryContext()) {
result = result && getQueryContext()
.equals(other.getQueryContext());
}
result = result && (hasDataChannel() == other.hasDataChannel());
if (hasDataChannel()) {
result = result && getDataChannel()
.equals(other.getDataChannel());
}
result = result && (hasEnforcer() == other.hasEnforcer());
if (hasEnforcer()) {
result = result && getEnforcer()
.equals(other.getEnforcer());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasQueryMasterHostAndPort()) {
hash = (37 * hash) + QUERYMASTERHOSTANDPORT_FIELD_NUMBER;
hash = (53 * hash) + getQueryMasterHostAndPort().hashCode();
}
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (getFragmentsCount() > 0) {
hash = (37 * hash) + FRAGMENTS_FIELD_NUMBER;
hash = (53 * hash) + getFragmentsList().hashCode();
}
if (hasOutputTable()) {
hash = (37 * hash) + OUTPUTTABLE_FIELD_NUMBER;
hash = (53 * hash) + getOutputTable().hashCode();
}
if (hasClusteredOutput()) {
hash = (37 * hash) + CLUSTEREDOUTPUT_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getClusteredOutput());
}
if (hasPlan()) {
hash = (37 * hash) + PLAN_FIELD_NUMBER;
hash = (53 * hash) + getPlan().hashCode();
}
if (hasInterQuery()) {
hash = (37 * hash) + INTERQUERY_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getInterQuery());
}
if (getFetchesCount() > 0) {
hash = (37 * hash) + FETCHES_FIELD_NUMBER;
hash = (53 * hash) + getFetchesList().hashCode();
}
if (hasQueryContext()) {
hash = (37 * hash) + QUERYCONTEXT_FIELD_NUMBER;
hash = (53 * hash) + getQueryContext().hashCode();
}
if (hasDataChannel()) {
hash = (37 * hash) + DATACHANNEL_FIELD_NUMBER;
hash = (53 * hash) + getDataChannel().hashCode();
}
if (hasEnforcer()) {
hash = (37 * hash) + ENFORCER_FIELD_NUMBER;
hash = (53 * hash) + getEnforcer().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.TaskRequestProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TaskRequestProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskRequestProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TaskRequestProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskRequestProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskRequestProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskRequestProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskRequestProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskRequestProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskRequestProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.TaskRequestProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TaskRequestProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.TaskRequestProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TaskRequestProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TaskRequestProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TaskRequestProto.class, org.apache.tajo.ResourceProtos.TaskRequestProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.TaskRequestProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getIdFieldBuilder();
getFragmentsFieldBuilder();
getPlanFieldBuilder();
getFetchesFieldBuilder();
getQueryContextFieldBuilder();
getDataChannelFieldBuilder();
getEnforcerFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
queryMasterHostAndPort_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
if (idBuilder_ == null) {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (fragmentsBuilder_ == null) {
fragments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
fragmentsBuilder_.clear();
}
outputTable_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
clusteredOutput_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
if (planBuilder_ == null) {
plan_ = org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree.getDefaultInstance();
} else {
planBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
interQuery_ = false;
bitField0_ = (bitField0_ & ~0x00000040);
if (fetchesBuilder_ == null) {
fetches_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
} else {
fetchesBuilder_.clear();
}
if (queryContextBuilder_ == null) {
queryContext_ = org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.getDefaultInstance();
} else {
queryContextBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
if (dataChannelBuilder_ == null) {
dataChannel_ = org.apache.tajo.plan.serder.PlanProto.DataChannelProto.getDefaultInstance();
} else {
dataChannelBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
if (enforcerBuilder_ == null) {
enforcer_ = org.apache.tajo.plan.serder.PlanProto.EnforcerProto.getDefaultInstance();
} else {
enforcerBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_TaskRequestProto_descriptor;
}
public org.apache.tajo.ResourceProtos.TaskRequestProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.TaskRequestProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.TaskRequestProto build() {
org.apache.tajo.ResourceProtos.TaskRequestProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.TaskRequestProto buildPartial() {
org.apache.tajo.ResourceProtos.TaskRequestProto result = new org.apache.tajo.ResourceProtos.TaskRequestProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.queryMasterHostAndPort_ = queryMasterHostAndPort_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (idBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = idBuilder_.build();
}
if (fragmentsBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
fragments_ = java.util.Collections.unmodifiableList(fragments_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.fragments_ = fragments_;
} else {
result.fragments_ = fragmentsBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.outputTable_ = outputTable_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000008;
}
result.clusteredOutput_ = clusteredOutput_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000010;
}
if (planBuilder_ == null) {
result.plan_ = plan_;
} else {
result.plan_ = planBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000020;
}
result.interQuery_ = interQuery_;
if (fetchesBuilder_ == null) {
if (((bitField0_ & 0x00000080) == 0x00000080)) {
fetches_ = java.util.Collections.unmodifiableList(fetches_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.fetches_ = fetches_;
} else {
result.fetches_ = fetchesBuilder_.build();
}
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000040;
}
if (queryContextBuilder_ == null) {
result.queryContext_ = queryContext_;
} else {
result.queryContext_ = queryContextBuilder_.build();
}
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000080;
}
if (dataChannelBuilder_ == null) {
result.dataChannel_ = dataChannel_;
} else {
result.dataChannel_ = dataChannelBuilder_.build();
}
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000100;
}
if (enforcerBuilder_ == null) {
result.enforcer_ = enforcer_;
} else {
result.enforcer_ = enforcerBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.TaskRequestProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.TaskRequestProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.TaskRequestProto other) {
if (other == org.apache.tajo.ResourceProtos.TaskRequestProto.getDefaultInstance()) return this;
if (other.hasQueryMasterHostAndPort()) {
bitField0_ |= 0x00000001;
queryMasterHostAndPort_ = other.queryMasterHostAndPort_;
onChanged();
}
if (other.hasId()) {
mergeId(other.getId());
}
if (fragmentsBuilder_ == null) {
if (!other.fragments_.isEmpty()) {
if (fragments_.isEmpty()) {
fragments_ = other.fragments_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureFragmentsIsMutable();
fragments_.addAll(other.fragments_);
}
onChanged();
}
} else {
if (!other.fragments_.isEmpty()) {
if (fragmentsBuilder_.isEmpty()) {
fragmentsBuilder_.dispose();
fragmentsBuilder_ = null;
fragments_ = other.fragments_;
bitField0_ = (bitField0_ & ~0x00000004);
fragmentsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getFragmentsFieldBuilder() : null;
} else {
fragmentsBuilder_.addAllMessages(other.fragments_);
}
}
}
if (other.hasOutputTable()) {
bitField0_ |= 0x00000008;
outputTable_ = other.outputTable_;
onChanged();
}
if (other.hasClusteredOutput()) {
setClusteredOutput(other.getClusteredOutput());
}
if (other.hasPlan()) {
mergePlan(other.getPlan());
}
if (other.hasInterQuery()) {
setInterQuery(other.getInterQuery());
}
if (fetchesBuilder_ == null) {
if (!other.fetches_.isEmpty()) {
if (fetches_.isEmpty()) {
fetches_ = other.fetches_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureFetchesIsMutable();
fetches_.addAll(other.fetches_);
}
onChanged();
}
} else {
if (!other.fetches_.isEmpty()) {
if (fetchesBuilder_.isEmpty()) {
fetchesBuilder_.dispose();
fetchesBuilder_ = null;
fetches_ = other.fetches_;
bitField0_ = (bitField0_ & ~0x00000080);
fetchesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getFetchesFieldBuilder() : null;
} else {
fetchesBuilder_.addAllMessages(other.fetches_);
}
}
}
if (other.hasQueryContext()) {
mergeQueryContext(other.getQueryContext());
}
if (other.hasDataChannel()) {
mergeDataChannel(other.getDataChannel());
}
if (other.hasEnforcer()) {
mergeEnforcer(other.getEnforcer());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasQueryMasterHostAndPort()) {
return false;
}
if (!hasId()) {
return false;
}
if (!hasOutputTable()) {
return false;
}
if (!hasClusteredOutput()) {
return false;
}
if (!hasPlan()) {
return false;
}
if (!getId().isInitialized()) {
return false;
}
for (int i = 0; i < getFragmentsCount(); i++) {
if (!getFragments(i).isInitialized()) {
return false;
}
}
if (!getPlan().isInitialized()) {
return false;
}
for (int i = 0; i < getFetchesCount(); i++) {
if (!getFetches(i).isInitialized()) {
return false;
}
}
if (hasQueryContext()) {
if (!getQueryContext().isInitialized()) {
return false;
}
}
if (hasDataChannel()) {
if (!getDataChannel().isInitialized()) {
return false;
}
}
if (hasEnforcer()) {
if (!getEnforcer().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.TaskRequestProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.TaskRequestProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string queryMasterHostAndPort = 1;
private java.lang.Object queryMasterHostAndPort_ = "";
/**
* required string queryMasterHostAndPort = 1;
*/
public boolean hasQueryMasterHostAndPort() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string queryMasterHostAndPort = 1;
*/
public java.lang.String getQueryMasterHostAndPort() {
java.lang.Object ref = queryMasterHostAndPort_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
queryMasterHostAndPort_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string queryMasterHostAndPort = 1;
*/
public com.google.protobuf.ByteString
getQueryMasterHostAndPortBytes() {
java.lang.Object ref = queryMasterHostAndPort_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
queryMasterHostAndPort_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string queryMasterHostAndPort = 1;
*/
public Builder setQueryMasterHostAndPort(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
queryMasterHostAndPort_ = value;
onChanged();
return this;
}
/**
* required string queryMasterHostAndPort = 1;
*/
public Builder clearQueryMasterHostAndPort() {
bitField0_ = (bitField0_ & ~0x00000001);
queryMasterHostAndPort_ = getDefaultInstance().getQueryMasterHostAndPort();
onChanged();
return this;
}
/**
* required string queryMasterHostAndPort = 1;
*/
public Builder setQueryMasterHostAndPortBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
queryMasterHostAndPort_ = value;
onChanged();
return this;
}
// required .TaskAttemptIdProto id = 2;
private org.apache.tajo.TajoIdProtos.TaskAttemptIdProto id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder> idBuilder_;
/**
* required .TaskAttemptIdProto id = 2;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .TaskAttemptIdProto id = 2;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId() {
if (idBuilder_ == null) {
return id_;
} else {
return idBuilder_.getMessage();
}
}
/**
* required .TaskAttemptIdProto id = 2;
*/
public Builder setId(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto value) {
if (idBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
idBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .TaskAttemptIdProto id = 2;
*/
public Builder setId(
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder builderForValue) {
if (idBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
idBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .TaskAttemptIdProto id = 2;
*/
public Builder mergeId(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto value) {
if (idBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
id_ != org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance()) {
id_ =
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.newBuilder(id_).mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
idBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .TaskAttemptIdProto id = 2;
*/
public Builder clearId() {
if (idBuilder_ == null) {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
onChanged();
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* required .TaskAttemptIdProto id = 2;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder getIdBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getIdFieldBuilder().getBuilder();
}
/**
* required .TaskAttemptIdProto id = 2;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder() {
if (idBuilder_ != null) {
return idBuilder_.getMessageOrBuilder();
} else {
return id_;
}
}
/**
* required .TaskAttemptIdProto id = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>
getIdFieldBuilder() {
if (idBuilder_ == null) {
idBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>(
id_,
getParentForChildren(),
isClean());
id_ = null;
}
return idBuilder_;
}
// repeated .FragmentProto fragments = 3;
private java.util.List fragments_ =
java.util.Collections.emptyList();
private void ensureFragmentsIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
fragments_ = new java.util.ArrayList(fragments_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto, org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.FragmentProtoOrBuilder> fragmentsBuilder_;
/**
* repeated .FragmentProto fragments = 3;
*/
public java.util.List getFragmentsList() {
if (fragmentsBuilder_ == null) {
return java.util.Collections.unmodifiableList(fragments_);
} else {
return fragmentsBuilder_.getMessageList();
}
}
/**
* repeated .FragmentProto fragments = 3;
*/
public int getFragmentsCount() {
if (fragmentsBuilder_ == null) {
return fragments_.size();
} else {
return fragmentsBuilder_.getCount();
}
}
/**
* repeated .FragmentProto fragments = 3;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto getFragments(int index) {
if (fragmentsBuilder_ == null) {
return fragments_.get(index);
} else {
return fragmentsBuilder_.getMessage(index);
}
}
/**
* repeated .FragmentProto fragments = 3;
*/
public Builder setFragments(
int index, org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto value) {
if (fragmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFragmentsIsMutable();
fragments_.set(index, value);
onChanged();
} else {
fragmentsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .FragmentProto fragments = 3;
*/
public Builder setFragments(
int index, org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto.Builder builderForValue) {
if (fragmentsBuilder_ == null) {
ensureFragmentsIsMutable();
fragments_.set(index, builderForValue.build());
onChanged();
} else {
fragmentsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .FragmentProto fragments = 3;
*/
public Builder addFragments(org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto value) {
if (fragmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFragmentsIsMutable();
fragments_.add(value);
onChanged();
} else {
fragmentsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .FragmentProto fragments = 3;
*/
public Builder addFragments(
int index, org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto value) {
if (fragmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFragmentsIsMutable();
fragments_.add(index, value);
onChanged();
} else {
fragmentsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .FragmentProto fragments = 3;
*/
public Builder addFragments(
org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto.Builder builderForValue) {
if (fragmentsBuilder_ == null) {
ensureFragmentsIsMutable();
fragments_.add(builderForValue.build());
onChanged();
} else {
fragmentsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .FragmentProto fragments = 3;
*/
public Builder addFragments(
int index, org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto.Builder builderForValue) {
if (fragmentsBuilder_ == null) {
ensureFragmentsIsMutable();
fragments_.add(index, builderForValue.build());
onChanged();
} else {
fragmentsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .FragmentProto fragments = 3;
*/
public Builder addAllFragments(
java.lang.Iterable extends org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto> values) {
if (fragmentsBuilder_ == null) {
ensureFragmentsIsMutable();
super.addAll(values, fragments_);
onChanged();
} else {
fragmentsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .FragmentProto fragments = 3;
*/
public Builder clearFragments() {
if (fragmentsBuilder_ == null) {
fragments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
fragmentsBuilder_.clear();
}
return this;
}
/**
* repeated .FragmentProto fragments = 3;
*/
public Builder removeFragments(int index) {
if (fragmentsBuilder_ == null) {
ensureFragmentsIsMutable();
fragments_.remove(index);
onChanged();
} else {
fragmentsBuilder_.remove(index);
}
return this;
}
/**
* repeated .FragmentProto fragments = 3;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto.Builder getFragmentsBuilder(
int index) {
return getFragmentsFieldBuilder().getBuilder(index);
}
/**
* repeated .FragmentProto fragments = 3;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.FragmentProtoOrBuilder getFragmentsOrBuilder(
int index) {
if (fragmentsBuilder_ == null) {
return fragments_.get(index); } else {
return fragmentsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .FragmentProto fragments = 3;
*/
public java.util.List extends org.apache.tajo.catalog.proto.CatalogProtos.FragmentProtoOrBuilder>
getFragmentsOrBuilderList() {
if (fragmentsBuilder_ != null) {
return fragmentsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(fragments_);
}
}
/**
* repeated .FragmentProto fragments = 3;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto.Builder addFragmentsBuilder() {
return getFragmentsFieldBuilder().addBuilder(
org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto.getDefaultInstance());
}
/**
* repeated .FragmentProto fragments = 3;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto.Builder addFragmentsBuilder(
int index) {
return getFragmentsFieldBuilder().addBuilder(
index, org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto.getDefaultInstance());
}
/**
* repeated .FragmentProto fragments = 3;
*/
public java.util.List
getFragmentsBuilderList() {
return getFragmentsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto, org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.FragmentProtoOrBuilder>
getFragmentsFieldBuilder() {
if (fragmentsBuilder_ == null) {
fragmentsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto, org.apache.tajo.catalog.proto.CatalogProtos.FragmentProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.FragmentProtoOrBuilder>(
fragments_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
fragments_ = null;
}
return fragmentsBuilder_;
}
// required string outputTable = 4;
private java.lang.Object outputTable_ = "";
/**
* required string outputTable = 4;
*/
public boolean hasOutputTable() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required string outputTable = 4;
*/
public java.lang.String getOutputTable() {
java.lang.Object ref = outputTable_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
outputTable_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string outputTable = 4;
*/
public com.google.protobuf.ByteString
getOutputTableBytes() {
java.lang.Object ref = outputTable_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outputTable_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string outputTable = 4;
*/
public Builder setOutputTable(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
outputTable_ = value;
onChanged();
return this;
}
/**
* required string outputTable = 4;
*/
public Builder clearOutputTable() {
bitField0_ = (bitField0_ & ~0x00000008);
outputTable_ = getDefaultInstance().getOutputTable();
onChanged();
return this;
}
/**
* required string outputTable = 4;
*/
public Builder setOutputTableBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
outputTable_ = value;
onChanged();
return this;
}
// required bool clusteredOutput = 5;
private boolean clusteredOutput_ ;
/**
* required bool clusteredOutput = 5;
*/
public boolean hasClusteredOutput() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required bool clusteredOutput = 5;
*/
public boolean getClusteredOutput() {
return clusteredOutput_;
}
/**
* required bool clusteredOutput = 5;
*/
public Builder setClusteredOutput(boolean value) {
bitField0_ |= 0x00000010;
clusteredOutput_ = value;
onChanged();
return this;
}
/**
* required bool clusteredOutput = 5;
*/
public Builder clearClusteredOutput() {
bitField0_ = (bitField0_ & ~0x00000010);
clusteredOutput_ = false;
onChanged();
return this;
}
// required .LogicalNodeTree plan = 6;
private org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree plan_ = org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree, org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree.Builder, org.apache.tajo.plan.serder.PlanProto.LogicalNodeTreeOrBuilder> planBuilder_;
/**
* required .LogicalNodeTree plan = 6;
*/
public boolean hasPlan() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* required .LogicalNodeTree plan = 6;
*/
public org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree getPlan() {
if (planBuilder_ == null) {
return plan_;
} else {
return planBuilder_.getMessage();
}
}
/**
* required .LogicalNodeTree plan = 6;
*/
public Builder setPlan(org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree value) {
if (planBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
plan_ = value;
onChanged();
} else {
planBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* required .LogicalNodeTree plan = 6;
*/
public Builder setPlan(
org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree.Builder builderForValue) {
if (planBuilder_ == null) {
plan_ = builderForValue.build();
onChanged();
} else {
planBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
* required .LogicalNodeTree plan = 6;
*/
public Builder mergePlan(org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree value) {
if (planBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020) &&
plan_ != org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree.getDefaultInstance()) {
plan_ =
org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree.newBuilder(plan_).mergeFrom(value).buildPartial();
} else {
plan_ = value;
}
onChanged();
} else {
planBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* required .LogicalNodeTree plan = 6;
*/
public Builder clearPlan() {
if (planBuilder_ == null) {
plan_ = org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree.getDefaultInstance();
onChanged();
} else {
planBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
* required .LogicalNodeTree plan = 6;
*/
public org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree.Builder getPlanBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getPlanFieldBuilder().getBuilder();
}
/**
* required .LogicalNodeTree plan = 6;
*/
public org.apache.tajo.plan.serder.PlanProto.LogicalNodeTreeOrBuilder getPlanOrBuilder() {
if (planBuilder_ != null) {
return planBuilder_.getMessageOrBuilder();
} else {
return plan_;
}
}
/**
* required .LogicalNodeTree plan = 6;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree, org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree.Builder, org.apache.tajo.plan.serder.PlanProto.LogicalNodeTreeOrBuilder>
getPlanFieldBuilder() {
if (planBuilder_ == null) {
planBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree, org.apache.tajo.plan.serder.PlanProto.LogicalNodeTree.Builder, org.apache.tajo.plan.serder.PlanProto.LogicalNodeTreeOrBuilder>(
plan_,
getParentForChildren(),
isClean());
plan_ = null;
}
return planBuilder_;
}
// optional bool interQuery = 7 [default = false];
private boolean interQuery_ ;
/**
* optional bool interQuery = 7 [default = false];
*/
public boolean hasInterQuery() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool interQuery = 7 [default = false];
*/
public boolean getInterQuery() {
return interQuery_;
}
/**
* optional bool interQuery = 7 [default = false];
*/
public Builder setInterQuery(boolean value) {
bitField0_ |= 0x00000040;
interQuery_ = value;
onChanged();
return this;
}
/**
* optional bool interQuery = 7 [default = false];
*/
public Builder clearInterQuery() {
bitField0_ = (bitField0_ & ~0x00000040);
interQuery_ = false;
onChanged();
return this;
}
// repeated .FetchProto fetches = 8;
private java.util.List fetches_ =
java.util.Collections.emptyList();
private void ensureFetchesIsMutable() {
if (!((bitField0_ & 0x00000080) == 0x00000080)) {
fetches_ = new java.util.ArrayList(fetches_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.FetchProto, org.apache.tajo.ResourceProtos.FetchProto.Builder, org.apache.tajo.ResourceProtos.FetchProtoOrBuilder> fetchesBuilder_;
/**
* repeated .FetchProto fetches = 8;
*/
public java.util.List getFetchesList() {
if (fetchesBuilder_ == null) {
return java.util.Collections.unmodifiableList(fetches_);
} else {
return fetchesBuilder_.getMessageList();
}
}
/**
* repeated .FetchProto fetches = 8;
*/
public int getFetchesCount() {
if (fetchesBuilder_ == null) {
return fetches_.size();
} else {
return fetchesBuilder_.getCount();
}
}
/**
* repeated .FetchProto fetches = 8;
*/
public org.apache.tajo.ResourceProtos.FetchProto getFetches(int index) {
if (fetchesBuilder_ == null) {
return fetches_.get(index);
} else {
return fetchesBuilder_.getMessage(index);
}
}
/**
* repeated .FetchProto fetches = 8;
*/
public Builder setFetches(
int index, org.apache.tajo.ResourceProtos.FetchProto value) {
if (fetchesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFetchesIsMutable();
fetches_.set(index, value);
onChanged();
} else {
fetchesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .FetchProto fetches = 8;
*/
public Builder setFetches(
int index, org.apache.tajo.ResourceProtos.FetchProto.Builder builderForValue) {
if (fetchesBuilder_ == null) {
ensureFetchesIsMutable();
fetches_.set(index, builderForValue.build());
onChanged();
} else {
fetchesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .FetchProto fetches = 8;
*/
public Builder addFetches(org.apache.tajo.ResourceProtos.FetchProto value) {
if (fetchesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFetchesIsMutable();
fetches_.add(value);
onChanged();
} else {
fetchesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .FetchProto fetches = 8;
*/
public Builder addFetches(
int index, org.apache.tajo.ResourceProtos.FetchProto value) {
if (fetchesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFetchesIsMutable();
fetches_.add(index, value);
onChanged();
} else {
fetchesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .FetchProto fetches = 8;
*/
public Builder addFetches(
org.apache.tajo.ResourceProtos.FetchProto.Builder builderForValue) {
if (fetchesBuilder_ == null) {
ensureFetchesIsMutable();
fetches_.add(builderForValue.build());
onChanged();
} else {
fetchesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .FetchProto fetches = 8;
*/
public Builder addFetches(
int index, org.apache.tajo.ResourceProtos.FetchProto.Builder builderForValue) {
if (fetchesBuilder_ == null) {
ensureFetchesIsMutable();
fetches_.add(index, builderForValue.build());
onChanged();
} else {
fetchesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .FetchProto fetches = 8;
*/
public Builder addAllFetches(
java.lang.Iterable extends org.apache.tajo.ResourceProtos.FetchProto> values) {
if (fetchesBuilder_ == null) {
ensureFetchesIsMutable();
super.addAll(values, fetches_);
onChanged();
} else {
fetchesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .FetchProto fetches = 8;
*/
public Builder clearFetches() {
if (fetchesBuilder_ == null) {
fetches_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
fetchesBuilder_.clear();
}
return this;
}
/**
* repeated .FetchProto fetches = 8;
*/
public Builder removeFetches(int index) {
if (fetchesBuilder_ == null) {
ensureFetchesIsMutable();
fetches_.remove(index);
onChanged();
} else {
fetchesBuilder_.remove(index);
}
return this;
}
/**
* repeated .FetchProto fetches = 8;
*/
public org.apache.tajo.ResourceProtos.FetchProto.Builder getFetchesBuilder(
int index) {
return getFetchesFieldBuilder().getBuilder(index);
}
/**
* repeated .FetchProto fetches = 8;
*/
public org.apache.tajo.ResourceProtos.FetchProtoOrBuilder getFetchesOrBuilder(
int index) {
if (fetchesBuilder_ == null) {
return fetches_.get(index); } else {
return fetchesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .FetchProto fetches = 8;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.FetchProtoOrBuilder>
getFetchesOrBuilderList() {
if (fetchesBuilder_ != null) {
return fetchesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(fetches_);
}
}
/**
* repeated .FetchProto fetches = 8;
*/
public org.apache.tajo.ResourceProtos.FetchProto.Builder addFetchesBuilder() {
return getFetchesFieldBuilder().addBuilder(
org.apache.tajo.ResourceProtos.FetchProto.getDefaultInstance());
}
/**
* repeated .FetchProto fetches = 8;
*/
public org.apache.tajo.ResourceProtos.FetchProto.Builder addFetchesBuilder(
int index) {
return getFetchesFieldBuilder().addBuilder(
index, org.apache.tajo.ResourceProtos.FetchProto.getDefaultInstance());
}
/**
* repeated .FetchProto fetches = 8;
*/
public java.util.List
getFetchesBuilderList() {
return getFetchesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.FetchProto, org.apache.tajo.ResourceProtos.FetchProto.Builder, org.apache.tajo.ResourceProtos.FetchProtoOrBuilder>
getFetchesFieldBuilder() {
if (fetchesBuilder_ == null) {
fetchesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.FetchProto, org.apache.tajo.ResourceProtos.FetchProto.Builder, org.apache.tajo.ResourceProtos.FetchProtoOrBuilder>(
fetches_,
((bitField0_ & 0x00000080) == 0x00000080),
getParentForChildren(),
isClean());
fetches_ = null;
}
return fetchesBuilder_;
}
// optional .KeyValueSetProto queryContext = 9;
private org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto queryContext_ = org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.Builder, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProtoOrBuilder> queryContextBuilder_;
/**
* optional .KeyValueSetProto queryContext = 9;
*/
public boolean hasQueryContext() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .KeyValueSetProto queryContext = 9;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto getQueryContext() {
if (queryContextBuilder_ == null) {
return queryContext_;
} else {
return queryContextBuilder_.getMessage();
}
}
/**
* optional .KeyValueSetProto queryContext = 9;
*/
public Builder setQueryContext(org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto value) {
if (queryContextBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
queryContext_ = value;
onChanged();
} else {
queryContextBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .KeyValueSetProto queryContext = 9;
*/
public Builder setQueryContext(
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.Builder builderForValue) {
if (queryContextBuilder_ == null) {
queryContext_ = builderForValue.build();
onChanged();
} else {
queryContextBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .KeyValueSetProto queryContext = 9;
*/
public Builder mergeQueryContext(org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto value) {
if (queryContextBuilder_ == null) {
if (((bitField0_ & 0x00000100) == 0x00000100) &&
queryContext_ != org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.getDefaultInstance()) {
queryContext_ =
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.newBuilder(queryContext_).mergeFrom(value).buildPartial();
} else {
queryContext_ = value;
}
onChanged();
} else {
queryContextBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .KeyValueSetProto queryContext = 9;
*/
public Builder clearQueryContext() {
if (queryContextBuilder_ == null) {
queryContext_ = org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.getDefaultInstance();
onChanged();
} else {
queryContextBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
/**
* optional .KeyValueSetProto queryContext = 9;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.Builder getQueryContextBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getQueryContextFieldBuilder().getBuilder();
}
/**
* optional .KeyValueSetProto queryContext = 9;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProtoOrBuilder getQueryContextOrBuilder() {
if (queryContextBuilder_ != null) {
return queryContextBuilder_.getMessageOrBuilder();
} else {
return queryContext_;
}
}
/**
* optional .KeyValueSetProto queryContext = 9;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.Builder, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProtoOrBuilder>
getQueryContextFieldBuilder() {
if (queryContextBuilder_ == null) {
queryContextBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.Builder, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProtoOrBuilder>(
queryContext_,
getParentForChildren(),
isClean());
queryContext_ = null;
}
return queryContextBuilder_;
}
// optional .DataChannelProto dataChannel = 10;
private org.apache.tajo.plan.serder.PlanProto.DataChannelProto dataChannel_ = org.apache.tajo.plan.serder.PlanProto.DataChannelProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.plan.serder.PlanProto.DataChannelProto, org.apache.tajo.plan.serder.PlanProto.DataChannelProto.Builder, org.apache.tajo.plan.serder.PlanProto.DataChannelProtoOrBuilder> dataChannelBuilder_;
/**
* optional .DataChannelProto dataChannel = 10;
*/
public boolean hasDataChannel() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .DataChannelProto dataChannel = 10;
*/
public org.apache.tajo.plan.serder.PlanProto.DataChannelProto getDataChannel() {
if (dataChannelBuilder_ == null) {
return dataChannel_;
} else {
return dataChannelBuilder_.getMessage();
}
}
/**
* optional .DataChannelProto dataChannel = 10;
*/
public Builder setDataChannel(org.apache.tajo.plan.serder.PlanProto.DataChannelProto value) {
if (dataChannelBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dataChannel_ = value;
onChanged();
} else {
dataChannelBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .DataChannelProto dataChannel = 10;
*/
public Builder setDataChannel(
org.apache.tajo.plan.serder.PlanProto.DataChannelProto.Builder builderForValue) {
if (dataChannelBuilder_ == null) {
dataChannel_ = builderForValue.build();
onChanged();
} else {
dataChannelBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .DataChannelProto dataChannel = 10;
*/
public Builder mergeDataChannel(org.apache.tajo.plan.serder.PlanProto.DataChannelProto value) {
if (dataChannelBuilder_ == null) {
if (((bitField0_ & 0x00000200) == 0x00000200) &&
dataChannel_ != org.apache.tajo.plan.serder.PlanProto.DataChannelProto.getDefaultInstance()) {
dataChannel_ =
org.apache.tajo.plan.serder.PlanProto.DataChannelProto.newBuilder(dataChannel_).mergeFrom(value).buildPartial();
} else {
dataChannel_ = value;
}
onChanged();
} else {
dataChannelBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .DataChannelProto dataChannel = 10;
*/
public Builder clearDataChannel() {
if (dataChannelBuilder_ == null) {
dataChannel_ = org.apache.tajo.plan.serder.PlanProto.DataChannelProto.getDefaultInstance();
onChanged();
} else {
dataChannelBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
/**
* optional .DataChannelProto dataChannel = 10;
*/
public org.apache.tajo.plan.serder.PlanProto.DataChannelProto.Builder getDataChannelBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getDataChannelFieldBuilder().getBuilder();
}
/**
* optional .DataChannelProto dataChannel = 10;
*/
public org.apache.tajo.plan.serder.PlanProto.DataChannelProtoOrBuilder getDataChannelOrBuilder() {
if (dataChannelBuilder_ != null) {
return dataChannelBuilder_.getMessageOrBuilder();
} else {
return dataChannel_;
}
}
/**
* optional .DataChannelProto dataChannel = 10;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.plan.serder.PlanProto.DataChannelProto, org.apache.tajo.plan.serder.PlanProto.DataChannelProto.Builder, org.apache.tajo.plan.serder.PlanProto.DataChannelProtoOrBuilder>
getDataChannelFieldBuilder() {
if (dataChannelBuilder_ == null) {
dataChannelBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.plan.serder.PlanProto.DataChannelProto, org.apache.tajo.plan.serder.PlanProto.DataChannelProto.Builder, org.apache.tajo.plan.serder.PlanProto.DataChannelProtoOrBuilder>(
dataChannel_,
getParentForChildren(),
isClean());
dataChannel_ = null;
}
return dataChannelBuilder_;
}
// optional .EnforcerProto enforcer = 11;
private org.apache.tajo.plan.serder.PlanProto.EnforcerProto enforcer_ = org.apache.tajo.plan.serder.PlanProto.EnforcerProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.plan.serder.PlanProto.EnforcerProto, org.apache.tajo.plan.serder.PlanProto.EnforcerProto.Builder, org.apache.tajo.plan.serder.PlanProto.EnforcerProtoOrBuilder> enforcerBuilder_;
/**
* optional .EnforcerProto enforcer = 11;
*/
public boolean hasEnforcer() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional .EnforcerProto enforcer = 11;
*/
public org.apache.tajo.plan.serder.PlanProto.EnforcerProto getEnforcer() {
if (enforcerBuilder_ == null) {
return enforcer_;
} else {
return enforcerBuilder_.getMessage();
}
}
/**
* optional .EnforcerProto enforcer = 11;
*/
public Builder setEnforcer(org.apache.tajo.plan.serder.PlanProto.EnforcerProto value) {
if (enforcerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
enforcer_ = value;
onChanged();
} else {
enforcerBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .EnforcerProto enforcer = 11;
*/
public Builder setEnforcer(
org.apache.tajo.plan.serder.PlanProto.EnforcerProto.Builder builderForValue) {
if (enforcerBuilder_ == null) {
enforcer_ = builderForValue.build();
onChanged();
} else {
enforcerBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .EnforcerProto enforcer = 11;
*/
public Builder mergeEnforcer(org.apache.tajo.plan.serder.PlanProto.EnforcerProto value) {
if (enforcerBuilder_ == null) {
if (((bitField0_ & 0x00000400) == 0x00000400) &&
enforcer_ != org.apache.tajo.plan.serder.PlanProto.EnforcerProto.getDefaultInstance()) {
enforcer_ =
org.apache.tajo.plan.serder.PlanProto.EnforcerProto.newBuilder(enforcer_).mergeFrom(value).buildPartial();
} else {
enforcer_ = value;
}
onChanged();
} else {
enforcerBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .EnforcerProto enforcer = 11;
*/
public Builder clearEnforcer() {
if (enforcerBuilder_ == null) {
enforcer_ = org.apache.tajo.plan.serder.PlanProto.EnforcerProto.getDefaultInstance();
onChanged();
} else {
enforcerBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
/**
* optional .EnforcerProto enforcer = 11;
*/
public org.apache.tajo.plan.serder.PlanProto.EnforcerProto.Builder getEnforcerBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getEnforcerFieldBuilder().getBuilder();
}
/**
* optional .EnforcerProto enforcer = 11;
*/
public org.apache.tajo.plan.serder.PlanProto.EnforcerProtoOrBuilder getEnforcerOrBuilder() {
if (enforcerBuilder_ != null) {
return enforcerBuilder_.getMessageOrBuilder();
} else {
return enforcer_;
}
}
/**
* optional .EnforcerProto enforcer = 11;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.plan.serder.PlanProto.EnforcerProto, org.apache.tajo.plan.serder.PlanProto.EnforcerProto.Builder, org.apache.tajo.plan.serder.PlanProto.EnforcerProtoOrBuilder>
getEnforcerFieldBuilder() {
if (enforcerBuilder_ == null) {
enforcerBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.plan.serder.PlanProto.EnforcerProto, org.apache.tajo.plan.serder.PlanProto.EnforcerProto.Builder, org.apache.tajo.plan.serder.PlanProto.EnforcerProtoOrBuilder>(
enforcer_,
getParentForChildren(),
isClean());
enforcer_ = null;
}
return enforcerBuilder_;
}
// @@protoc_insertion_point(builder_scope:TaskRequestProto)
}
static {
defaultInstance = new TaskRequestProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TaskRequestProto)
}
public interface FetchProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string host = 1;
/**
* required string host = 1;
*/
boolean hasHost();
/**
* required string host = 1;
*/
java.lang.String getHost();
/**
* required string host = 1;
*/
com.google.protobuf.ByteString
getHostBytes();
// required int32 port = 2;
/**
* required int32 port = 2;
*/
boolean hasPort();
/**
* required int32 port = 2;
*/
int getPort();
// required .ShuffleType type = 3;
/**
* required .ShuffleType type = 3;
*/
boolean hasType();
/**
* required .ShuffleType type = 3;
*/
org.apache.tajo.plan.serder.PlanProto.ShuffleType getType();
// required .ExecutionBlockIdProto executionBlockId = 4;
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
boolean hasExecutionBlockId();
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto getExecutionBlockId();
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder getExecutionBlockIdOrBuilder();
// required int32 partitionId = 5;
/**
* required int32 partitionId = 5;
*/
boolean hasPartitionId();
/**
* required int32 partitionId = 5;
*/
int getPartitionId();
// required string name = 6;
/**
* required string name = 6;
*/
boolean hasName();
/**
* required string name = 6;
*/
java.lang.String getName();
/**
* required string name = 6;
*/
com.google.protobuf.ByteString
getNameBytes();
// optional bytes range_start = 7;
/**
* optional bytes range_start = 7;
*/
boolean hasRangeStart();
/**
* optional bytes range_start = 7;
*/
com.google.protobuf.ByteString getRangeStart();
// optional bytes range_end = 8;
/**
* optional bytes range_end = 8;
*/
boolean hasRangeEnd();
/**
* optional bytes range_end = 8;
*/
com.google.protobuf.ByteString getRangeEnd();
// optional bool range_last_inclusive = 9;
/**
* optional bool range_last_inclusive = 9;
*/
boolean hasRangeLastInclusive();
/**
* optional bool range_last_inclusive = 9;
*/
boolean getRangeLastInclusive();
// optional bool has_next = 10 [default = false];
/**
* optional bool has_next = 10 [default = false];
*/
boolean hasHasNext();
/**
* optional bool has_next = 10 [default = false];
*/
boolean getHasNext();
// repeated int32 task_id = 11 [packed = true];
/**
* repeated int32 task_id = 11 [packed = true];
*
*
* repeated part
*
*/
java.util.List getTaskIdList();
/**
* repeated int32 task_id = 11 [packed = true];
*
*
* repeated part
*
*/
int getTaskIdCount();
/**
* repeated int32 task_id = 11 [packed = true];
*
*
* repeated part
*
*/
int getTaskId(int index);
// repeated int32 attempt_id = 12 [packed = true];
/**
* repeated int32 attempt_id = 12 [packed = true];
*/
java.util.List getAttemptIdList();
/**
* repeated int32 attempt_id = 12 [packed = true];
*/
int getAttemptIdCount();
/**
* repeated int32 attempt_id = 12 [packed = true];
*/
int getAttemptId(int index);
// optional int64 offset = 13;
/**
* optional int64 offset = 13;
*/
boolean hasOffset();
/**
* optional int64 offset = 13;
*/
long getOffset();
// optional int64 length = 14;
/**
* optional int64 length = 14;
*/
boolean hasLength();
/**
* optional int64 length = 14;
*/
long getLength();
}
/**
* Protobuf type {@code FetchProto}
*/
public static final class FetchProto extends
com.google.protobuf.GeneratedMessage
implements FetchProtoOrBuilder {
// Use FetchProto.newBuilder() to construct.
private FetchProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private FetchProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final FetchProto defaultInstance;
public static FetchProto getDefaultInstance() {
return defaultInstance;
}
public FetchProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FetchProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
host_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
port_ = input.readInt32();
break;
}
case 24: {
int rawValue = input.readEnum();
org.apache.tajo.plan.serder.PlanProto.ShuffleType value = org.apache.tajo.plan.serder.PlanProto.ShuffleType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(3, rawValue);
} else {
bitField0_ |= 0x00000004;
type_ = value;
}
break;
}
case 34: {
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = executionBlockId_.toBuilder();
}
executionBlockId_ = input.readMessage(org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(executionBlockId_);
executionBlockId_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 40: {
bitField0_ |= 0x00000010;
partitionId_ = input.readInt32();
break;
}
case 50: {
bitField0_ |= 0x00000020;
name_ = input.readBytes();
break;
}
case 58: {
bitField0_ |= 0x00000040;
rangeStart_ = input.readBytes();
break;
}
case 66: {
bitField0_ |= 0x00000080;
rangeEnd_ = input.readBytes();
break;
}
case 72: {
bitField0_ |= 0x00000100;
rangeLastInclusive_ = input.readBool();
break;
}
case 80: {
bitField0_ |= 0x00000200;
hasNext_ = input.readBool();
break;
}
case 88: {
if (!((mutable_bitField0_ & 0x00000400) == 0x00000400)) {
taskId_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000400;
}
taskId_.add(input.readInt32());
break;
}
case 90: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000400) == 0x00000400) && input.getBytesUntilLimit() > 0) {
taskId_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000400;
}
while (input.getBytesUntilLimit() > 0) {
taskId_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
case 96: {
if (!((mutable_bitField0_ & 0x00000800) == 0x00000800)) {
attemptId_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000800;
}
attemptId_.add(input.readInt32());
break;
}
case 98: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000800) == 0x00000800) && input.getBytesUntilLimit() > 0) {
attemptId_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000800;
}
while (input.getBytesUntilLimit() > 0) {
attemptId_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
case 104: {
bitField0_ |= 0x00000400;
offset_ = input.readInt64();
break;
}
case 112: {
bitField0_ |= 0x00000800;
length_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000400) == 0x00000400)) {
taskId_ = java.util.Collections.unmodifiableList(taskId_);
}
if (((mutable_bitField0_ & 0x00000800) == 0x00000800)) {
attemptId_ = java.util.Collections.unmodifiableList(attemptId_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_FetchProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_FetchProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.FetchProto.class, org.apache.tajo.ResourceProtos.FetchProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public FetchProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FetchProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string host = 1;
public static final int HOST_FIELD_NUMBER = 1;
private java.lang.Object host_;
/**
* required string host = 1;
*/
public boolean hasHost() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string host = 1;
*/
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
host_ = s;
}
return s;
}
}
/**
* required string host = 1;
*/
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required int32 port = 2;
public static final int PORT_FIELD_NUMBER = 2;
private int port_;
/**
* required int32 port = 2;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 port = 2;
*/
public int getPort() {
return port_;
}
// required .ShuffleType type = 3;
public static final int TYPE_FIELD_NUMBER = 3;
private org.apache.tajo.plan.serder.PlanProto.ShuffleType type_;
/**
* required .ShuffleType type = 3;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required .ShuffleType type = 3;
*/
public org.apache.tajo.plan.serder.PlanProto.ShuffleType getType() {
return type_;
}
// required .ExecutionBlockIdProto executionBlockId = 4;
public static final int EXECUTIONBLOCKID_FIELD_NUMBER = 4;
private org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto executionBlockId_;
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
public boolean hasExecutionBlockId() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto getExecutionBlockId() {
return executionBlockId_;
}
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder getExecutionBlockIdOrBuilder() {
return executionBlockId_;
}
// required int32 partitionId = 5;
public static final int PARTITIONID_FIELD_NUMBER = 5;
private int partitionId_;
/**
* required int32 partitionId = 5;
*/
public boolean hasPartitionId() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required int32 partitionId = 5;
*/
public int getPartitionId() {
return partitionId_;
}
// required string name = 6;
public static final int NAME_FIELD_NUMBER = 6;
private java.lang.Object name_;
/**
* required string name = 6;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* required string name = 6;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 6;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional bytes range_start = 7;
public static final int RANGE_START_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString rangeStart_;
/**
* optional bytes range_start = 7;
*/
public boolean hasRangeStart() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bytes range_start = 7;
*/
public com.google.protobuf.ByteString getRangeStart() {
return rangeStart_;
}
// optional bytes range_end = 8;
public static final int RANGE_END_FIELD_NUMBER = 8;
private com.google.protobuf.ByteString rangeEnd_;
/**
* optional bytes range_end = 8;
*/
public boolean hasRangeEnd() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bytes range_end = 8;
*/
public com.google.protobuf.ByteString getRangeEnd() {
return rangeEnd_;
}
// optional bool range_last_inclusive = 9;
public static final int RANGE_LAST_INCLUSIVE_FIELD_NUMBER = 9;
private boolean rangeLastInclusive_;
/**
* optional bool range_last_inclusive = 9;
*/
public boolean hasRangeLastInclusive() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool range_last_inclusive = 9;
*/
public boolean getRangeLastInclusive() {
return rangeLastInclusive_;
}
// optional bool has_next = 10 [default = false];
public static final int HAS_NEXT_FIELD_NUMBER = 10;
private boolean hasNext_;
/**
* optional bool has_next = 10 [default = false];
*/
public boolean hasHasNext() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional bool has_next = 10 [default = false];
*/
public boolean getHasNext() {
return hasNext_;
}
// repeated int32 task_id = 11 [packed = true];
public static final int TASK_ID_FIELD_NUMBER = 11;
private java.util.List taskId_;
/**
* repeated int32 task_id = 11 [packed = true];
*
*
* repeated part
*
*/
public java.util.List
getTaskIdList() {
return taskId_;
}
/**
* repeated int32 task_id = 11 [packed = true];
*
*
* repeated part
*
*/
public int getTaskIdCount() {
return taskId_.size();
}
/**
* repeated int32 task_id = 11 [packed = true];
*
*
* repeated part
*
*/
public int getTaskId(int index) {
return taskId_.get(index);
}
private int taskIdMemoizedSerializedSize = -1;
// repeated int32 attempt_id = 12 [packed = true];
public static final int ATTEMPT_ID_FIELD_NUMBER = 12;
private java.util.List attemptId_;
/**
* repeated int32 attempt_id = 12 [packed = true];
*/
public java.util.List
getAttemptIdList() {
return attemptId_;
}
/**
* repeated int32 attempt_id = 12 [packed = true];
*/
public int getAttemptIdCount() {
return attemptId_.size();
}
/**
* repeated int32 attempt_id = 12 [packed = true];
*/
public int getAttemptId(int index) {
return attemptId_.get(index);
}
private int attemptIdMemoizedSerializedSize = -1;
// optional int64 offset = 13;
public static final int OFFSET_FIELD_NUMBER = 13;
private long offset_;
/**
* optional int64 offset = 13;
*/
public boolean hasOffset() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional int64 offset = 13;
*/
public long getOffset() {
return offset_;
}
// optional int64 length = 14;
public static final int LENGTH_FIELD_NUMBER = 14;
private long length_;
/**
* optional int64 length = 14;
*/
public boolean hasLength() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional int64 length = 14;
*/
public long getLength() {
return length_;
}
private void initFields() {
host_ = "";
port_ = 0;
type_ = org.apache.tajo.plan.serder.PlanProto.ShuffleType.NONE_SHUFFLE;
executionBlockId_ = org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance();
partitionId_ = 0;
name_ = "";
rangeStart_ = com.google.protobuf.ByteString.EMPTY;
rangeEnd_ = com.google.protobuf.ByteString.EMPTY;
rangeLastInclusive_ = false;
hasNext_ = false;
taskId_ = java.util.Collections.emptyList();
attemptId_ = java.util.Collections.emptyList();
offset_ = 0L;
length_ = 0L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasHost()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPort()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasExecutionBlockId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPartitionId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!getExecutionBlockId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getHostBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, port_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeEnum(3, type_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, executionBlockId_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt32(5, partitionId_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBytes(6, getNameBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBytes(7, rangeStart_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBytes(8, rangeEnd_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBool(9, rangeLastInclusive_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeBool(10, hasNext_);
}
if (getTaskIdList().size() > 0) {
output.writeRawVarint32(90);
output.writeRawVarint32(taskIdMemoizedSerializedSize);
}
for (int i = 0; i < taskId_.size(); i++) {
output.writeInt32NoTag(taskId_.get(i));
}
if (getAttemptIdList().size() > 0) {
output.writeRawVarint32(98);
output.writeRawVarint32(attemptIdMemoizedSerializedSize);
}
for (int i = 0; i < attemptId_.size(); i++) {
output.writeInt32NoTag(attemptId_.get(i));
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeInt64(13, offset_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeInt64(14, length_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getHostBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, port_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, type_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, executionBlockId_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, partitionId_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, getNameBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, rangeStart_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, rangeEnd_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, rangeLastInclusive_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, hasNext_);
}
{
int dataSize = 0;
for (int i = 0; i < taskId_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(taskId_.get(i));
}
size += dataSize;
if (!getTaskIdList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
taskIdMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < attemptId_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(attemptId_.get(i));
}
size += dataSize;
if (!getAttemptIdList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
attemptIdMemoizedSerializedSize = dataSize;
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(13, offset_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(14, length_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.FetchProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.FetchProto other = (org.apache.tajo.ResourceProtos.FetchProto) obj;
boolean result = true;
result = result && (hasHost() == other.hasHost());
if (hasHost()) {
result = result && getHost()
.equals(other.getHost());
}
result = result && (hasPort() == other.hasPort());
if (hasPort()) {
result = result && (getPort()
== other.getPort());
}
result = result && (hasType() == other.hasType());
if (hasType()) {
result = result &&
(getType() == other.getType());
}
result = result && (hasExecutionBlockId() == other.hasExecutionBlockId());
if (hasExecutionBlockId()) {
result = result && getExecutionBlockId()
.equals(other.getExecutionBlockId());
}
result = result && (hasPartitionId() == other.hasPartitionId());
if (hasPartitionId()) {
result = result && (getPartitionId()
== other.getPartitionId());
}
result = result && (hasName() == other.hasName());
if (hasName()) {
result = result && getName()
.equals(other.getName());
}
result = result && (hasRangeStart() == other.hasRangeStart());
if (hasRangeStart()) {
result = result && getRangeStart()
.equals(other.getRangeStart());
}
result = result && (hasRangeEnd() == other.hasRangeEnd());
if (hasRangeEnd()) {
result = result && getRangeEnd()
.equals(other.getRangeEnd());
}
result = result && (hasRangeLastInclusive() == other.hasRangeLastInclusive());
if (hasRangeLastInclusive()) {
result = result && (getRangeLastInclusive()
== other.getRangeLastInclusive());
}
result = result && (hasHasNext() == other.hasHasNext());
if (hasHasNext()) {
result = result && (getHasNext()
== other.getHasNext());
}
result = result && getTaskIdList()
.equals(other.getTaskIdList());
result = result && getAttemptIdList()
.equals(other.getAttemptIdList());
result = result && (hasOffset() == other.hasOffset());
if (hasOffset()) {
result = result && (getOffset()
== other.getOffset());
}
result = result && (hasLength() == other.hasLength());
if (hasLength()) {
result = result && (getLength()
== other.getLength());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasHost()) {
hash = (37 * hash) + HOST_FIELD_NUMBER;
hash = (53 * hash) + getHost().hashCode();
}
if (hasPort()) {
hash = (37 * hash) + PORT_FIELD_NUMBER;
hash = (53 * hash) + getPort();
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + hashEnum(getType());
}
if (hasExecutionBlockId()) {
hash = (37 * hash) + EXECUTIONBLOCKID_FIELD_NUMBER;
hash = (53 * hash) + getExecutionBlockId().hashCode();
}
if (hasPartitionId()) {
hash = (37 * hash) + PARTITIONID_FIELD_NUMBER;
hash = (53 * hash) + getPartitionId();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasRangeStart()) {
hash = (37 * hash) + RANGE_START_FIELD_NUMBER;
hash = (53 * hash) + getRangeStart().hashCode();
}
if (hasRangeEnd()) {
hash = (37 * hash) + RANGE_END_FIELD_NUMBER;
hash = (53 * hash) + getRangeEnd().hashCode();
}
if (hasRangeLastInclusive()) {
hash = (37 * hash) + RANGE_LAST_INCLUSIVE_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getRangeLastInclusive());
}
if (hasHasNext()) {
hash = (37 * hash) + HAS_NEXT_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getHasNext());
}
if (getTaskIdCount() > 0) {
hash = (37 * hash) + TASK_ID_FIELD_NUMBER;
hash = (53 * hash) + getTaskIdList().hashCode();
}
if (getAttemptIdCount() > 0) {
hash = (37 * hash) + ATTEMPT_ID_FIELD_NUMBER;
hash = (53 * hash) + getAttemptIdList().hashCode();
}
if (hasOffset()) {
hash = (37 * hash) + OFFSET_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getOffset());
}
if (hasLength()) {
hash = (37 * hash) + LENGTH_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getLength());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.FetchProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.FetchProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.FetchProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.FetchProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.FetchProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.FetchProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.FetchProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.FetchProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.FetchProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.FetchProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.FetchProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code FetchProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.FetchProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_FetchProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_FetchProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.FetchProto.class, org.apache.tajo.ResourceProtos.FetchProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.FetchProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getExecutionBlockIdFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
host_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
port_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
type_ = org.apache.tajo.plan.serder.PlanProto.ShuffleType.NONE_SHUFFLE;
bitField0_ = (bitField0_ & ~0x00000004);
if (executionBlockIdBuilder_ == null) {
executionBlockId_ = org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance();
} else {
executionBlockIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
partitionId_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
rangeStart_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
rangeEnd_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000080);
rangeLastInclusive_ = false;
bitField0_ = (bitField0_ & ~0x00000100);
hasNext_ = false;
bitField0_ = (bitField0_ & ~0x00000200);
taskId_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000400);
attemptId_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000800);
offset_ = 0L;
bitField0_ = (bitField0_ & ~0x00001000);
length_ = 0L;
bitField0_ = (bitField0_ & ~0x00002000);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_FetchProto_descriptor;
}
public org.apache.tajo.ResourceProtos.FetchProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.FetchProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.FetchProto build() {
org.apache.tajo.ResourceProtos.FetchProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.FetchProto buildPartial() {
org.apache.tajo.ResourceProtos.FetchProto result = new org.apache.tajo.ResourceProtos.FetchProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.host_ = host_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.port_ = port_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (executionBlockIdBuilder_ == null) {
result.executionBlockId_ = executionBlockId_;
} else {
result.executionBlockId_ = executionBlockIdBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.partitionId_ = partitionId_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.rangeStart_ = rangeStart_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.rangeEnd_ = rangeEnd_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.rangeLastInclusive_ = rangeLastInclusive_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.hasNext_ = hasNext_;
if (((bitField0_ & 0x00000400) == 0x00000400)) {
taskId_ = java.util.Collections.unmodifiableList(taskId_);
bitField0_ = (bitField0_ & ~0x00000400);
}
result.taskId_ = taskId_;
if (((bitField0_ & 0x00000800) == 0x00000800)) {
attemptId_ = java.util.Collections.unmodifiableList(attemptId_);
bitField0_ = (bitField0_ & ~0x00000800);
}
result.attemptId_ = attemptId_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00000400;
}
result.offset_ = offset_;
if (((from_bitField0_ & 0x00002000) == 0x00002000)) {
to_bitField0_ |= 0x00000800;
}
result.length_ = length_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.FetchProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.FetchProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.FetchProto other) {
if (other == org.apache.tajo.ResourceProtos.FetchProto.getDefaultInstance()) return this;
if (other.hasHost()) {
bitField0_ |= 0x00000001;
host_ = other.host_;
onChanged();
}
if (other.hasPort()) {
setPort(other.getPort());
}
if (other.hasType()) {
setType(other.getType());
}
if (other.hasExecutionBlockId()) {
mergeExecutionBlockId(other.getExecutionBlockId());
}
if (other.hasPartitionId()) {
setPartitionId(other.getPartitionId());
}
if (other.hasName()) {
bitField0_ |= 0x00000020;
name_ = other.name_;
onChanged();
}
if (other.hasRangeStart()) {
setRangeStart(other.getRangeStart());
}
if (other.hasRangeEnd()) {
setRangeEnd(other.getRangeEnd());
}
if (other.hasRangeLastInclusive()) {
setRangeLastInclusive(other.getRangeLastInclusive());
}
if (other.hasHasNext()) {
setHasNext(other.getHasNext());
}
if (!other.taskId_.isEmpty()) {
if (taskId_.isEmpty()) {
taskId_ = other.taskId_;
bitField0_ = (bitField0_ & ~0x00000400);
} else {
ensureTaskIdIsMutable();
taskId_.addAll(other.taskId_);
}
onChanged();
}
if (!other.attemptId_.isEmpty()) {
if (attemptId_.isEmpty()) {
attemptId_ = other.attemptId_;
bitField0_ = (bitField0_ & ~0x00000800);
} else {
ensureAttemptIdIsMutable();
attemptId_.addAll(other.attemptId_);
}
onChanged();
}
if (other.hasOffset()) {
setOffset(other.getOffset());
}
if (other.hasLength()) {
setLength(other.getLength());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasHost()) {
return false;
}
if (!hasPort()) {
return false;
}
if (!hasType()) {
return false;
}
if (!hasExecutionBlockId()) {
return false;
}
if (!hasPartitionId()) {
return false;
}
if (!hasName()) {
return false;
}
if (!getExecutionBlockId().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.FetchProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.FetchProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string host = 1;
private java.lang.Object host_ = "";
/**
* required string host = 1;
*/
public boolean hasHost() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string host = 1;
*/
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
host_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string host = 1;
*/
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string host = 1;
*/
public Builder setHost(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
host_ = value;
onChanged();
return this;
}
/**
* required string host = 1;
*/
public Builder clearHost() {
bitField0_ = (bitField0_ & ~0x00000001);
host_ = getDefaultInstance().getHost();
onChanged();
return this;
}
/**
* required string host = 1;
*/
public Builder setHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
host_ = value;
onChanged();
return this;
}
// required int32 port = 2;
private int port_ ;
/**
* required int32 port = 2;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 port = 2;
*/
public int getPort() {
return port_;
}
/**
* required int32 port = 2;
*/
public Builder setPort(int value) {
bitField0_ |= 0x00000002;
port_ = value;
onChanged();
return this;
}
/**
* required int32 port = 2;
*/
public Builder clearPort() {
bitField0_ = (bitField0_ & ~0x00000002);
port_ = 0;
onChanged();
return this;
}
// required .ShuffleType type = 3;
private org.apache.tajo.plan.serder.PlanProto.ShuffleType type_ = org.apache.tajo.plan.serder.PlanProto.ShuffleType.NONE_SHUFFLE;
/**
* required .ShuffleType type = 3;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required .ShuffleType type = 3;
*/
public org.apache.tajo.plan.serder.PlanProto.ShuffleType getType() {
return type_;
}
/**
* required .ShuffleType type = 3;
*/
public Builder setType(org.apache.tajo.plan.serder.PlanProto.ShuffleType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
type_ = value;
onChanged();
return this;
}
/**
* required .ShuffleType type = 3;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000004);
type_ = org.apache.tajo.plan.serder.PlanProto.ShuffleType.NONE_SHUFFLE;
onChanged();
return this;
}
// required .ExecutionBlockIdProto executionBlockId = 4;
private org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto executionBlockId_ = org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder> executionBlockIdBuilder_;
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
public boolean hasExecutionBlockId() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto getExecutionBlockId() {
if (executionBlockIdBuilder_ == null) {
return executionBlockId_;
} else {
return executionBlockIdBuilder_.getMessage();
}
}
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
public Builder setExecutionBlockId(org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto value) {
if (executionBlockIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
executionBlockId_ = value;
onChanged();
} else {
executionBlockIdBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
public Builder setExecutionBlockId(
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder builderForValue) {
if (executionBlockIdBuilder_ == null) {
executionBlockId_ = builderForValue.build();
onChanged();
} else {
executionBlockIdBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
public Builder mergeExecutionBlockId(org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto value) {
if (executionBlockIdBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
executionBlockId_ != org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance()) {
executionBlockId_ =
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.newBuilder(executionBlockId_).mergeFrom(value).buildPartial();
} else {
executionBlockId_ = value;
}
onChanged();
} else {
executionBlockIdBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
public Builder clearExecutionBlockId() {
if (executionBlockIdBuilder_ == null) {
executionBlockId_ = org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance();
onChanged();
} else {
executionBlockIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder getExecutionBlockIdBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getExecutionBlockIdFieldBuilder().getBuilder();
}
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder getExecutionBlockIdOrBuilder() {
if (executionBlockIdBuilder_ != null) {
return executionBlockIdBuilder_.getMessageOrBuilder();
} else {
return executionBlockId_;
}
}
/**
* required .ExecutionBlockIdProto executionBlockId = 4;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder>
getExecutionBlockIdFieldBuilder() {
if (executionBlockIdBuilder_ == null) {
executionBlockIdBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder>(
executionBlockId_,
getParentForChildren(),
isClean());
executionBlockId_ = null;
}
return executionBlockIdBuilder_;
}
// required int32 partitionId = 5;
private int partitionId_ ;
/**
* required int32 partitionId = 5;
*/
public boolean hasPartitionId() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required int32 partitionId = 5;
*/
public int getPartitionId() {
return partitionId_;
}
/**
* required int32 partitionId = 5;
*/
public Builder setPartitionId(int value) {
bitField0_ |= 0x00000010;
partitionId_ = value;
onChanged();
return this;
}
/**
* required int32 partitionId = 5;
*/
public Builder clearPartitionId() {
bitField0_ = (bitField0_ & ~0x00000010);
partitionId_ = 0;
onChanged();
return this;
}
// required string name = 6;
private java.lang.Object name_ = "";
/**
* required string name = 6;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* required string name = 6;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 6;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 6;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 6;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000020);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 6;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
name_ = value;
onChanged();
return this;
}
// optional bytes range_start = 7;
private com.google.protobuf.ByteString rangeStart_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes range_start = 7;
*/
public boolean hasRangeStart() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bytes range_start = 7;
*/
public com.google.protobuf.ByteString getRangeStart() {
return rangeStart_;
}
/**
* optional bytes range_start = 7;
*/
public Builder setRangeStart(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
rangeStart_ = value;
onChanged();
return this;
}
/**
* optional bytes range_start = 7;
*/
public Builder clearRangeStart() {
bitField0_ = (bitField0_ & ~0x00000040);
rangeStart_ = getDefaultInstance().getRangeStart();
onChanged();
return this;
}
// optional bytes range_end = 8;
private com.google.protobuf.ByteString rangeEnd_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes range_end = 8;
*/
public boolean hasRangeEnd() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bytes range_end = 8;
*/
public com.google.protobuf.ByteString getRangeEnd() {
return rangeEnd_;
}
/**
* optional bytes range_end = 8;
*/
public Builder setRangeEnd(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
rangeEnd_ = value;
onChanged();
return this;
}
/**
* optional bytes range_end = 8;
*/
public Builder clearRangeEnd() {
bitField0_ = (bitField0_ & ~0x00000080);
rangeEnd_ = getDefaultInstance().getRangeEnd();
onChanged();
return this;
}
// optional bool range_last_inclusive = 9;
private boolean rangeLastInclusive_ ;
/**
* optional bool range_last_inclusive = 9;
*/
public boolean hasRangeLastInclusive() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool range_last_inclusive = 9;
*/
public boolean getRangeLastInclusive() {
return rangeLastInclusive_;
}
/**
* optional bool range_last_inclusive = 9;
*/
public Builder setRangeLastInclusive(boolean value) {
bitField0_ |= 0x00000100;
rangeLastInclusive_ = value;
onChanged();
return this;
}
/**
* optional bool range_last_inclusive = 9;
*/
public Builder clearRangeLastInclusive() {
bitField0_ = (bitField0_ & ~0x00000100);
rangeLastInclusive_ = false;
onChanged();
return this;
}
// optional bool has_next = 10 [default = false];
private boolean hasNext_ ;
/**
* optional bool has_next = 10 [default = false];
*/
public boolean hasHasNext() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional bool has_next = 10 [default = false];
*/
public boolean getHasNext() {
return hasNext_;
}
/**
* optional bool has_next = 10 [default = false];
*/
public Builder setHasNext(boolean value) {
bitField0_ |= 0x00000200;
hasNext_ = value;
onChanged();
return this;
}
/**
* optional bool has_next = 10 [default = false];
*/
public Builder clearHasNext() {
bitField0_ = (bitField0_ & ~0x00000200);
hasNext_ = false;
onChanged();
return this;
}
// repeated int32 task_id = 11 [packed = true];
private java.util.List taskId_ = java.util.Collections.emptyList();
private void ensureTaskIdIsMutable() {
if (!((bitField0_ & 0x00000400) == 0x00000400)) {
taskId_ = new java.util.ArrayList(taskId_);
bitField0_ |= 0x00000400;
}
}
/**
* repeated int32 task_id = 11 [packed = true];
*
*
* repeated part
*
*/
public java.util.List
getTaskIdList() {
return java.util.Collections.unmodifiableList(taskId_);
}
/**
* repeated int32 task_id = 11 [packed = true];
*
*
* repeated part
*
*/
public int getTaskIdCount() {
return taskId_.size();
}
/**
* repeated int32 task_id = 11 [packed = true];
*
*
* repeated part
*
*/
public int getTaskId(int index) {
return taskId_.get(index);
}
/**
* repeated int32 task_id = 11 [packed = true];
*
*
* repeated part
*
*/
public Builder setTaskId(
int index, int value) {
ensureTaskIdIsMutable();
taskId_.set(index, value);
onChanged();
return this;
}
/**
* repeated int32 task_id = 11 [packed = true];
*
*
* repeated part
*
*/
public Builder addTaskId(int value) {
ensureTaskIdIsMutable();
taskId_.add(value);
onChanged();
return this;
}
/**
* repeated int32 task_id = 11 [packed = true];
*
*
* repeated part
*
*/
public Builder addAllTaskId(
java.lang.Iterable extends java.lang.Integer> values) {
ensureTaskIdIsMutable();
super.addAll(values, taskId_);
onChanged();
return this;
}
/**
* repeated int32 task_id = 11 [packed = true];
*
*
* repeated part
*
*/
public Builder clearTaskId() {
taskId_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
return this;
}
// repeated int32 attempt_id = 12 [packed = true];
private java.util.List attemptId_ = java.util.Collections.emptyList();
private void ensureAttemptIdIsMutable() {
if (!((bitField0_ & 0x00000800) == 0x00000800)) {
attemptId_ = new java.util.ArrayList(attemptId_);
bitField0_ |= 0x00000800;
}
}
/**
* repeated int32 attempt_id = 12 [packed = true];
*/
public java.util.List
getAttemptIdList() {
return java.util.Collections.unmodifiableList(attemptId_);
}
/**
* repeated int32 attempt_id = 12 [packed = true];
*/
public int getAttemptIdCount() {
return attemptId_.size();
}
/**
* repeated int32 attempt_id = 12 [packed = true];
*/
public int getAttemptId(int index) {
return attemptId_.get(index);
}
/**
* repeated int32 attempt_id = 12 [packed = true];
*/
public Builder setAttemptId(
int index, int value) {
ensureAttemptIdIsMutable();
attemptId_.set(index, value);
onChanged();
return this;
}
/**
* repeated int32 attempt_id = 12 [packed = true];
*/
public Builder addAttemptId(int value) {
ensureAttemptIdIsMutable();
attemptId_.add(value);
onChanged();
return this;
}
/**
* repeated int32 attempt_id = 12 [packed = true];
*/
public Builder addAllAttemptId(
java.lang.Iterable extends java.lang.Integer> values) {
ensureAttemptIdIsMutable();
super.addAll(values, attemptId_);
onChanged();
return this;
}
/**
* repeated int32 attempt_id = 12 [packed = true];
*/
public Builder clearAttemptId() {
attemptId_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000800);
onChanged();
return this;
}
// optional int64 offset = 13;
private long offset_ ;
/**
* optional int64 offset = 13;
*/
public boolean hasOffset() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional int64 offset = 13;
*/
public long getOffset() {
return offset_;
}
/**
* optional int64 offset = 13;
*/
public Builder setOffset(long value) {
bitField0_ |= 0x00001000;
offset_ = value;
onChanged();
return this;
}
/**
* optional int64 offset = 13;
*/
public Builder clearOffset() {
bitField0_ = (bitField0_ & ~0x00001000);
offset_ = 0L;
onChanged();
return this;
}
// optional int64 length = 14;
private long length_ ;
/**
* optional int64 length = 14;
*/
public boolean hasLength() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional int64 length = 14;
*/
public long getLength() {
return length_;
}
/**
* optional int64 length = 14;
*/
public Builder setLength(long value) {
bitField0_ |= 0x00002000;
length_ = value;
onChanged();
return this;
}
/**
* optional int64 length = 14;
*/
public Builder clearLength() {
bitField0_ = (bitField0_ & ~0x00002000);
length_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:FetchProto)
}
static {
defaultInstance = new FetchProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:FetchProto)
}
public interface TaskStatusProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required .TaskAttemptIdProto id = 1;
/**
* required .TaskAttemptIdProto id = 1;
*/
boolean hasId();
/**
* required .TaskAttemptIdProto id = 1;
*/
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId();
/**
* required .TaskAttemptIdProto id = 1;
*/
org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder();
// required string workerName = 2;
/**
* required string workerName = 2;
*/
boolean hasWorkerName();
/**
* required string workerName = 2;
*/
java.lang.String getWorkerName();
/**
* required string workerName = 2;
*/
com.google.protobuf.ByteString
getWorkerNameBytes();
// required float progress = 3;
/**
* required float progress = 3;
*/
boolean hasProgress();
/**
* required float progress = 3;
*/
float getProgress();
// required .TaskAttemptState state = 4;
/**
* required .TaskAttemptState state = 4;
*/
boolean hasState();
/**
* required .TaskAttemptState state = 4;
*/
org.apache.tajo.TajoProtos.TaskAttemptState getState();
// optional .StatSetProto stats = 5;
/**
* optional .StatSetProto stats = 5;
*/
boolean hasStats();
/**
* optional .StatSetProto stats = 5;
*/
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto getStats();
/**
* optional .StatSetProto stats = 5;
*/
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProtoOrBuilder getStatsOrBuilder();
// optional .TableStatsProto inputStats = 6;
/**
* optional .TableStatsProto inputStats = 6;
*/
boolean hasInputStats();
/**
* optional .TableStatsProto inputStats = 6;
*/
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto getInputStats();
/**
* optional .TableStatsProto inputStats = 6;
*/
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder getInputStatsOrBuilder();
// optional .TableStatsProto resultStats = 7;
/**
* optional .TableStatsProto resultStats = 7;
*/
boolean hasResultStats();
/**
* optional .TableStatsProto resultStats = 7;
*/
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto getResultStats();
/**
* optional .TableStatsProto resultStats = 7;
*/
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder getResultStatsOrBuilder();
// repeated .ShuffleFileOutput shuffleFileOutputs = 8;
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
java.util.List
getShuffleFileOutputsList();
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
org.apache.tajo.ResourceProtos.ShuffleFileOutput getShuffleFileOutputs(int index);
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
int getShuffleFileOutputsCount();
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
java.util.List extends org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder>
getShuffleFileOutputsOrBuilderList();
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder getShuffleFileOutputsOrBuilder(
int index);
}
/**
* Protobuf type {@code TaskStatusProto}
*/
public static final class TaskStatusProto extends
com.google.protobuf.GeneratedMessage
implements TaskStatusProtoOrBuilder {
// Use TaskStatusProto.newBuilder() to construct.
private TaskStatusProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TaskStatusProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TaskStatusProto defaultInstance;
public static TaskStatusProto getDefaultInstance() {
return defaultInstance;
}
public TaskStatusProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TaskStatusProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = id_.toBuilder();
}
id_ = input.readMessage(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(id_);
id_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
bitField0_ |= 0x00000002;
workerName_ = input.readBytes();
break;
}
case 29: {
bitField0_ |= 0x00000004;
progress_ = input.readFloat();
break;
}
case 32: {
int rawValue = input.readEnum();
org.apache.tajo.TajoProtos.TaskAttemptState value = org.apache.tajo.TajoProtos.TaskAttemptState.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(4, rawValue);
} else {
bitField0_ |= 0x00000008;
state_ = value;
}
break;
}
case 42: {
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = stats_.toBuilder();
}
stats_ = input.readMessage(org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(stats_);
stats_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 50: {
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
subBuilder = inputStats_.toBuilder();
}
inputStats_ = input.readMessage(org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(inputStats_);
inputStats_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
case 58: {
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
subBuilder = resultStats_.toBuilder();
}
resultStats_ = input.readMessage(org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(resultStats_);
resultStats_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
shuffleFileOutputs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000080;
}
shuffleFileOutputs_.add(input.readMessage(org.apache.tajo.ResourceProtos.ShuffleFileOutput.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
shuffleFileOutputs_ = java.util.Collections.unmodifiableList(shuffleFileOutputs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TaskStatusProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TaskStatusProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TaskStatusProto.class, org.apache.tajo.ResourceProtos.TaskStatusProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TaskStatusProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TaskStatusProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .TaskAttemptIdProto id = 1;
public static final int ID_FIELD_NUMBER = 1;
private org.apache.tajo.TajoIdProtos.TaskAttemptIdProto id_;
/**
* required .TaskAttemptIdProto id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId() {
return id_;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder() {
return id_;
}
// required string workerName = 2;
public static final int WORKERNAME_FIELD_NUMBER = 2;
private java.lang.Object workerName_;
/**
* required string workerName = 2;
*/
public boolean hasWorkerName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string workerName = 2;
*/
public java.lang.String getWorkerName() {
java.lang.Object ref = workerName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
workerName_ = s;
}
return s;
}
}
/**
* required string workerName = 2;
*/
public com.google.protobuf.ByteString
getWorkerNameBytes() {
java.lang.Object ref = workerName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workerName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required float progress = 3;
public static final int PROGRESS_FIELD_NUMBER = 3;
private float progress_;
/**
* required float progress = 3;
*/
public boolean hasProgress() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required float progress = 3;
*/
public float getProgress() {
return progress_;
}
// required .TaskAttemptState state = 4;
public static final int STATE_FIELD_NUMBER = 4;
private org.apache.tajo.TajoProtos.TaskAttemptState state_;
/**
* required .TaskAttemptState state = 4;
*/
public boolean hasState() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required .TaskAttemptState state = 4;
*/
public org.apache.tajo.TajoProtos.TaskAttemptState getState() {
return state_;
}
// optional .StatSetProto stats = 5;
public static final int STATS_FIELD_NUMBER = 5;
private org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto stats_;
/**
* optional .StatSetProto stats = 5;
*/
public boolean hasStats() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .StatSetProto stats = 5;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto getStats() {
return stats_;
}
/**
* optional .StatSetProto stats = 5;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.StatSetProtoOrBuilder getStatsOrBuilder() {
return stats_;
}
// optional .TableStatsProto inputStats = 6;
public static final int INPUTSTATS_FIELD_NUMBER = 6;
private org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto inputStats_;
/**
* optional .TableStatsProto inputStats = 6;
*/
public boolean hasInputStats() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .TableStatsProto inputStats = 6;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto getInputStats() {
return inputStats_;
}
/**
* optional .TableStatsProto inputStats = 6;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder getInputStatsOrBuilder() {
return inputStats_;
}
// optional .TableStatsProto resultStats = 7;
public static final int RESULTSTATS_FIELD_NUMBER = 7;
private org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto resultStats_;
/**
* optional .TableStatsProto resultStats = 7;
*/
public boolean hasResultStats() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .TableStatsProto resultStats = 7;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto getResultStats() {
return resultStats_;
}
/**
* optional .TableStatsProto resultStats = 7;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder getResultStatsOrBuilder() {
return resultStats_;
}
// repeated .ShuffleFileOutput shuffleFileOutputs = 8;
public static final int SHUFFLEFILEOUTPUTS_FIELD_NUMBER = 8;
private java.util.List shuffleFileOutputs_;
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public java.util.List getShuffleFileOutputsList() {
return shuffleFileOutputs_;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder>
getShuffleFileOutputsOrBuilderList() {
return shuffleFileOutputs_;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public int getShuffleFileOutputsCount() {
return shuffleFileOutputs_.size();
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutput getShuffleFileOutputs(int index) {
return shuffleFileOutputs_.get(index);
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder getShuffleFileOutputsOrBuilder(
int index) {
return shuffleFileOutputs_.get(index);
}
private void initFields() {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
workerName_ = "";
progress_ = 0F;
state_ = org.apache.tajo.TajoProtos.TaskAttemptState.TA_NEW;
stats_ = org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.getDefaultInstance();
inputStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
resultStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
shuffleFileOutputs_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasWorkerName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasProgress()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasState()) {
memoizedIsInitialized = 0;
return false;
}
if (!getId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (hasStats()) {
if (!getStats().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasInputStats()) {
if (!getInputStats().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasResultStats()) {
if (!getResultStats().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getShuffleFileOutputsCount(); i++) {
if (!getShuffleFileOutputs(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getWorkerNameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeFloat(3, progress_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeEnum(4, state_.getNumber());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(5, stats_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeMessage(6, inputStats_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeMessage(7, resultStats_);
}
for (int i = 0; i < shuffleFileOutputs_.size(); i++) {
output.writeMessage(8, shuffleFileOutputs_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getWorkerNameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(3, progress_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, state_.getNumber());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, stats_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, inputStats_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, resultStats_);
}
for (int i = 0; i < shuffleFileOutputs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, shuffleFileOutputs_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.TaskStatusProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.TaskStatusProto other = (org.apache.tajo.ResourceProtos.TaskStatusProto) obj;
boolean result = true;
result = result && (hasId() == other.hasId());
if (hasId()) {
result = result && getId()
.equals(other.getId());
}
result = result && (hasWorkerName() == other.hasWorkerName());
if (hasWorkerName()) {
result = result && getWorkerName()
.equals(other.getWorkerName());
}
result = result && (hasProgress() == other.hasProgress());
if (hasProgress()) {
result = result && (Float.floatToIntBits(getProgress()) == Float.floatToIntBits(other.getProgress()));
}
result = result && (hasState() == other.hasState());
if (hasState()) {
result = result &&
(getState() == other.getState());
}
result = result && (hasStats() == other.hasStats());
if (hasStats()) {
result = result && getStats()
.equals(other.getStats());
}
result = result && (hasInputStats() == other.hasInputStats());
if (hasInputStats()) {
result = result && getInputStats()
.equals(other.getInputStats());
}
result = result && (hasResultStats() == other.hasResultStats());
if (hasResultStats()) {
result = result && getResultStats()
.equals(other.getResultStats());
}
result = result && getShuffleFileOutputsList()
.equals(other.getShuffleFileOutputsList());
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasWorkerName()) {
hash = (37 * hash) + WORKERNAME_FIELD_NUMBER;
hash = (53 * hash) + getWorkerName().hashCode();
}
if (hasProgress()) {
hash = (37 * hash) + PROGRESS_FIELD_NUMBER;
hash = (53 * hash) + Float.floatToIntBits(
getProgress());
}
if (hasState()) {
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + hashEnum(getState());
}
if (hasStats()) {
hash = (37 * hash) + STATS_FIELD_NUMBER;
hash = (53 * hash) + getStats().hashCode();
}
if (hasInputStats()) {
hash = (37 * hash) + INPUTSTATS_FIELD_NUMBER;
hash = (53 * hash) + getInputStats().hashCode();
}
if (hasResultStats()) {
hash = (37 * hash) + RESULTSTATS_FIELD_NUMBER;
hash = (53 * hash) + getResultStats().hashCode();
}
if (getShuffleFileOutputsCount() > 0) {
hash = (37 * hash) + SHUFFLEFILEOUTPUTS_FIELD_NUMBER;
hash = (53 * hash) + getShuffleFileOutputsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.TaskStatusProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TaskStatusProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskStatusProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TaskStatusProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskStatusProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskStatusProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskStatusProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskStatusProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskStatusProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskStatusProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.TaskStatusProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TaskStatusProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.TaskStatusProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TaskStatusProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TaskStatusProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TaskStatusProto.class, org.apache.tajo.ResourceProtos.TaskStatusProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.TaskStatusProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getIdFieldBuilder();
getStatsFieldBuilder();
getInputStatsFieldBuilder();
getResultStatsFieldBuilder();
getShuffleFileOutputsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (idBuilder_ == null) {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
workerName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
progress_ = 0F;
bitField0_ = (bitField0_ & ~0x00000004);
state_ = org.apache.tajo.TajoProtos.TaskAttemptState.TA_NEW;
bitField0_ = (bitField0_ & ~0x00000008);
if (statsBuilder_ == null) {
stats_ = org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.getDefaultInstance();
} else {
statsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (inputStatsBuilder_ == null) {
inputStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
} else {
inputStatsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
if (resultStatsBuilder_ == null) {
resultStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
} else {
resultStatsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
if (shuffleFileOutputsBuilder_ == null) {
shuffleFileOutputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
} else {
shuffleFileOutputsBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_TaskStatusProto_descriptor;
}
public org.apache.tajo.ResourceProtos.TaskStatusProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.TaskStatusProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.TaskStatusProto build() {
org.apache.tajo.ResourceProtos.TaskStatusProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.TaskStatusProto buildPartial() {
org.apache.tajo.ResourceProtos.TaskStatusProto result = new org.apache.tajo.ResourceProtos.TaskStatusProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (idBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = idBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.workerName_ = workerName_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.progress_ = progress_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.state_ = state_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (statsBuilder_ == null) {
result.stats_ = stats_;
} else {
result.stats_ = statsBuilder_.build();
}
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
if (inputStatsBuilder_ == null) {
result.inputStats_ = inputStats_;
} else {
result.inputStats_ = inputStatsBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
if (resultStatsBuilder_ == null) {
result.resultStats_ = resultStats_;
} else {
result.resultStats_ = resultStatsBuilder_.build();
}
if (shuffleFileOutputsBuilder_ == null) {
if (((bitField0_ & 0x00000080) == 0x00000080)) {
shuffleFileOutputs_ = java.util.Collections.unmodifiableList(shuffleFileOutputs_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.shuffleFileOutputs_ = shuffleFileOutputs_;
} else {
result.shuffleFileOutputs_ = shuffleFileOutputsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.TaskStatusProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.TaskStatusProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.TaskStatusProto other) {
if (other == org.apache.tajo.ResourceProtos.TaskStatusProto.getDefaultInstance()) return this;
if (other.hasId()) {
mergeId(other.getId());
}
if (other.hasWorkerName()) {
bitField0_ |= 0x00000002;
workerName_ = other.workerName_;
onChanged();
}
if (other.hasProgress()) {
setProgress(other.getProgress());
}
if (other.hasState()) {
setState(other.getState());
}
if (other.hasStats()) {
mergeStats(other.getStats());
}
if (other.hasInputStats()) {
mergeInputStats(other.getInputStats());
}
if (other.hasResultStats()) {
mergeResultStats(other.getResultStats());
}
if (shuffleFileOutputsBuilder_ == null) {
if (!other.shuffleFileOutputs_.isEmpty()) {
if (shuffleFileOutputs_.isEmpty()) {
shuffleFileOutputs_ = other.shuffleFileOutputs_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.addAll(other.shuffleFileOutputs_);
}
onChanged();
}
} else {
if (!other.shuffleFileOutputs_.isEmpty()) {
if (shuffleFileOutputsBuilder_.isEmpty()) {
shuffleFileOutputsBuilder_.dispose();
shuffleFileOutputsBuilder_ = null;
shuffleFileOutputs_ = other.shuffleFileOutputs_;
bitField0_ = (bitField0_ & ~0x00000080);
shuffleFileOutputsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getShuffleFileOutputsFieldBuilder() : null;
} else {
shuffleFileOutputsBuilder_.addAllMessages(other.shuffleFileOutputs_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasId()) {
return false;
}
if (!hasWorkerName()) {
return false;
}
if (!hasProgress()) {
return false;
}
if (!hasState()) {
return false;
}
if (!getId().isInitialized()) {
return false;
}
if (hasStats()) {
if (!getStats().isInitialized()) {
return false;
}
}
if (hasInputStats()) {
if (!getInputStats().isInitialized()) {
return false;
}
}
if (hasResultStats()) {
if (!getResultStats().isInitialized()) {
return false;
}
}
for (int i = 0; i < getShuffleFileOutputsCount(); i++) {
if (!getShuffleFileOutputs(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.TaskStatusProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.TaskStatusProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .TaskAttemptIdProto id = 1;
private org.apache.tajo.TajoIdProtos.TaskAttemptIdProto id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder> idBuilder_;
/**
* required .TaskAttemptIdProto id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId() {
if (idBuilder_ == null) {
return id_;
} else {
return idBuilder_.getMessage();
}
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder setId(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto value) {
if (idBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
idBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder setId(
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder builderForValue) {
if (idBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
idBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder mergeId(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto value) {
if (idBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
id_ != org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance()) {
id_ =
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.newBuilder(id_).mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
idBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder clearId() {
if (idBuilder_ == null) {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
onChanged();
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder getIdBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getIdFieldBuilder().getBuilder();
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder() {
if (idBuilder_ != null) {
return idBuilder_.getMessageOrBuilder();
} else {
return id_;
}
}
/**
* required .TaskAttemptIdProto id = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>
getIdFieldBuilder() {
if (idBuilder_ == null) {
idBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>(
id_,
getParentForChildren(),
isClean());
id_ = null;
}
return idBuilder_;
}
// required string workerName = 2;
private java.lang.Object workerName_ = "";
/**
* required string workerName = 2;
*/
public boolean hasWorkerName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string workerName = 2;
*/
public java.lang.String getWorkerName() {
java.lang.Object ref = workerName_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
workerName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string workerName = 2;
*/
public com.google.protobuf.ByteString
getWorkerNameBytes() {
java.lang.Object ref = workerName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workerName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string workerName = 2;
*/
public Builder setWorkerName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
workerName_ = value;
onChanged();
return this;
}
/**
* required string workerName = 2;
*/
public Builder clearWorkerName() {
bitField0_ = (bitField0_ & ~0x00000002);
workerName_ = getDefaultInstance().getWorkerName();
onChanged();
return this;
}
/**
* required string workerName = 2;
*/
public Builder setWorkerNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
workerName_ = value;
onChanged();
return this;
}
// required float progress = 3;
private float progress_ ;
/**
* required float progress = 3;
*/
public boolean hasProgress() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required float progress = 3;
*/
public float getProgress() {
return progress_;
}
/**
* required float progress = 3;
*/
public Builder setProgress(float value) {
bitField0_ |= 0x00000004;
progress_ = value;
onChanged();
return this;
}
/**
* required float progress = 3;
*/
public Builder clearProgress() {
bitField0_ = (bitField0_ & ~0x00000004);
progress_ = 0F;
onChanged();
return this;
}
// required .TaskAttemptState state = 4;
private org.apache.tajo.TajoProtos.TaskAttemptState state_ = org.apache.tajo.TajoProtos.TaskAttemptState.TA_NEW;
/**
* required .TaskAttemptState state = 4;
*/
public boolean hasState() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required .TaskAttemptState state = 4;
*/
public org.apache.tajo.TajoProtos.TaskAttemptState getState() {
return state_;
}
/**
* required .TaskAttemptState state = 4;
*/
public Builder setState(org.apache.tajo.TajoProtos.TaskAttemptState value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
state_ = value;
onChanged();
return this;
}
/**
* required .TaskAttemptState state = 4;
*/
public Builder clearState() {
bitField0_ = (bitField0_ & ~0x00000008);
state_ = org.apache.tajo.TajoProtos.TaskAttemptState.TA_NEW;
onChanged();
return this;
}
// optional .StatSetProto stats = 5;
private org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto stats_ = org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto, org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.StatSetProtoOrBuilder> statsBuilder_;
/**
* optional .StatSetProto stats = 5;
*/
public boolean hasStats() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .StatSetProto stats = 5;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto getStats() {
if (statsBuilder_ == null) {
return stats_;
} else {
return statsBuilder_.getMessage();
}
}
/**
* optional .StatSetProto stats = 5;
*/
public Builder setStats(org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto value) {
if (statsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
stats_ = value;
onChanged();
} else {
statsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .StatSetProto stats = 5;
*/
public Builder setStats(
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.Builder builderForValue) {
if (statsBuilder_ == null) {
stats_ = builderForValue.build();
onChanged();
} else {
statsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .StatSetProto stats = 5;
*/
public Builder mergeStats(org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto value) {
if (statsBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
stats_ != org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.getDefaultInstance()) {
stats_ =
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.newBuilder(stats_).mergeFrom(value).buildPartial();
} else {
stats_ = value;
}
onChanged();
} else {
statsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .StatSetProto stats = 5;
*/
public Builder clearStats() {
if (statsBuilder_ == null) {
stats_ = org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.getDefaultInstance();
onChanged();
} else {
statsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* optional .StatSetProto stats = 5;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.Builder getStatsBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getStatsFieldBuilder().getBuilder();
}
/**
* optional .StatSetProto stats = 5;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.StatSetProtoOrBuilder getStatsOrBuilder() {
if (statsBuilder_ != null) {
return statsBuilder_.getMessageOrBuilder();
} else {
return stats_;
}
}
/**
* optional .StatSetProto stats = 5;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto, org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.StatSetProtoOrBuilder>
getStatsFieldBuilder() {
if (statsBuilder_ == null) {
statsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto, org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.StatSetProtoOrBuilder>(
stats_,
getParentForChildren(),
isClean());
stats_ = null;
}
return statsBuilder_;
}
// optional .TableStatsProto inputStats = 6;
private org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto inputStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder> inputStatsBuilder_;
/**
* optional .TableStatsProto inputStats = 6;
*/
public boolean hasInputStats() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .TableStatsProto inputStats = 6;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto getInputStats() {
if (inputStatsBuilder_ == null) {
return inputStats_;
} else {
return inputStatsBuilder_.getMessage();
}
}
/**
* optional .TableStatsProto inputStats = 6;
*/
public Builder setInputStats(org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto value) {
if (inputStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
inputStats_ = value;
onChanged();
} else {
inputStatsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .TableStatsProto inputStats = 6;
*/
public Builder setInputStats(
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder builderForValue) {
if (inputStatsBuilder_ == null) {
inputStats_ = builderForValue.build();
onChanged();
} else {
inputStatsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .TableStatsProto inputStats = 6;
*/
public Builder mergeInputStats(org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto value) {
if (inputStatsBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020) &&
inputStats_ != org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance()) {
inputStats_ =
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.newBuilder(inputStats_).mergeFrom(value).buildPartial();
} else {
inputStats_ = value;
}
onChanged();
} else {
inputStatsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .TableStatsProto inputStats = 6;
*/
public Builder clearInputStats() {
if (inputStatsBuilder_ == null) {
inputStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
onChanged();
} else {
inputStatsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
* optional .TableStatsProto inputStats = 6;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder getInputStatsBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getInputStatsFieldBuilder().getBuilder();
}
/**
* optional .TableStatsProto inputStats = 6;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder getInputStatsOrBuilder() {
if (inputStatsBuilder_ != null) {
return inputStatsBuilder_.getMessageOrBuilder();
} else {
return inputStats_;
}
}
/**
* optional .TableStatsProto inputStats = 6;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder>
getInputStatsFieldBuilder() {
if (inputStatsBuilder_ == null) {
inputStatsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder>(
inputStats_,
getParentForChildren(),
isClean());
inputStats_ = null;
}
return inputStatsBuilder_;
}
// optional .TableStatsProto resultStats = 7;
private org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto resultStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder> resultStatsBuilder_;
/**
* optional .TableStatsProto resultStats = 7;
*/
public boolean hasResultStats() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .TableStatsProto resultStats = 7;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto getResultStats() {
if (resultStatsBuilder_ == null) {
return resultStats_;
} else {
return resultStatsBuilder_.getMessage();
}
}
/**
* optional .TableStatsProto resultStats = 7;
*/
public Builder setResultStats(org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto value) {
if (resultStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resultStats_ = value;
onChanged();
} else {
resultStatsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .TableStatsProto resultStats = 7;
*/
public Builder setResultStats(
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder builderForValue) {
if (resultStatsBuilder_ == null) {
resultStats_ = builderForValue.build();
onChanged();
} else {
resultStatsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .TableStatsProto resultStats = 7;
*/
public Builder mergeResultStats(org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto value) {
if (resultStatsBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040) &&
resultStats_ != org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance()) {
resultStats_ =
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.newBuilder(resultStats_).mergeFrom(value).buildPartial();
} else {
resultStats_ = value;
}
onChanged();
} else {
resultStatsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .TableStatsProto resultStats = 7;
*/
public Builder clearResultStats() {
if (resultStatsBuilder_ == null) {
resultStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
onChanged();
} else {
resultStatsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
/**
* optional .TableStatsProto resultStats = 7;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder getResultStatsBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getResultStatsFieldBuilder().getBuilder();
}
/**
* optional .TableStatsProto resultStats = 7;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder getResultStatsOrBuilder() {
if (resultStatsBuilder_ != null) {
return resultStatsBuilder_.getMessageOrBuilder();
} else {
return resultStats_;
}
}
/**
* optional .TableStatsProto resultStats = 7;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder>
getResultStatsFieldBuilder() {
if (resultStatsBuilder_ == null) {
resultStatsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder>(
resultStats_,
getParentForChildren(),
isClean());
resultStats_ = null;
}
return resultStatsBuilder_;
}
// repeated .ShuffleFileOutput shuffleFileOutputs = 8;
private java.util.List shuffleFileOutputs_ =
java.util.Collections.emptyList();
private void ensureShuffleFileOutputsIsMutable() {
if (!((bitField0_ & 0x00000080) == 0x00000080)) {
shuffleFileOutputs_ = new java.util.ArrayList(shuffleFileOutputs_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.ShuffleFileOutput, org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder, org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder> shuffleFileOutputsBuilder_;
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public java.util.List getShuffleFileOutputsList() {
if (shuffleFileOutputsBuilder_ == null) {
return java.util.Collections.unmodifiableList(shuffleFileOutputs_);
} else {
return shuffleFileOutputsBuilder_.getMessageList();
}
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public int getShuffleFileOutputsCount() {
if (shuffleFileOutputsBuilder_ == null) {
return shuffleFileOutputs_.size();
} else {
return shuffleFileOutputsBuilder_.getCount();
}
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutput getShuffleFileOutputs(int index) {
if (shuffleFileOutputsBuilder_ == null) {
return shuffleFileOutputs_.get(index);
} else {
return shuffleFileOutputsBuilder_.getMessage(index);
}
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public Builder setShuffleFileOutputs(
int index, org.apache.tajo.ResourceProtos.ShuffleFileOutput value) {
if (shuffleFileOutputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.set(index, value);
onChanged();
} else {
shuffleFileOutputsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public Builder setShuffleFileOutputs(
int index, org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder builderForValue) {
if (shuffleFileOutputsBuilder_ == null) {
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.set(index, builderForValue.build());
onChanged();
} else {
shuffleFileOutputsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public Builder addShuffleFileOutputs(org.apache.tajo.ResourceProtos.ShuffleFileOutput value) {
if (shuffleFileOutputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.add(value);
onChanged();
} else {
shuffleFileOutputsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public Builder addShuffleFileOutputs(
int index, org.apache.tajo.ResourceProtos.ShuffleFileOutput value) {
if (shuffleFileOutputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.add(index, value);
onChanged();
} else {
shuffleFileOutputsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public Builder addShuffleFileOutputs(
org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder builderForValue) {
if (shuffleFileOutputsBuilder_ == null) {
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.add(builderForValue.build());
onChanged();
} else {
shuffleFileOutputsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public Builder addShuffleFileOutputs(
int index, org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder builderForValue) {
if (shuffleFileOutputsBuilder_ == null) {
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.add(index, builderForValue.build());
onChanged();
} else {
shuffleFileOutputsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public Builder addAllShuffleFileOutputs(
java.lang.Iterable extends org.apache.tajo.ResourceProtos.ShuffleFileOutput> values) {
if (shuffleFileOutputsBuilder_ == null) {
ensureShuffleFileOutputsIsMutable();
super.addAll(values, shuffleFileOutputs_);
onChanged();
} else {
shuffleFileOutputsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public Builder clearShuffleFileOutputs() {
if (shuffleFileOutputsBuilder_ == null) {
shuffleFileOutputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
shuffleFileOutputsBuilder_.clear();
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public Builder removeShuffleFileOutputs(int index) {
if (shuffleFileOutputsBuilder_ == null) {
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.remove(index);
onChanged();
} else {
shuffleFileOutputsBuilder_.remove(index);
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder getShuffleFileOutputsBuilder(
int index) {
return getShuffleFileOutputsFieldBuilder().getBuilder(index);
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder getShuffleFileOutputsOrBuilder(
int index) {
if (shuffleFileOutputsBuilder_ == null) {
return shuffleFileOutputs_.get(index); } else {
return shuffleFileOutputsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder>
getShuffleFileOutputsOrBuilderList() {
if (shuffleFileOutputsBuilder_ != null) {
return shuffleFileOutputsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(shuffleFileOutputs_);
}
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder addShuffleFileOutputsBuilder() {
return getShuffleFileOutputsFieldBuilder().addBuilder(
org.apache.tajo.ResourceProtos.ShuffleFileOutput.getDefaultInstance());
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder addShuffleFileOutputsBuilder(
int index) {
return getShuffleFileOutputsFieldBuilder().addBuilder(
index, org.apache.tajo.ResourceProtos.ShuffleFileOutput.getDefaultInstance());
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 8;
*/
public java.util.List
getShuffleFileOutputsBuilderList() {
return getShuffleFileOutputsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.ShuffleFileOutput, org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder, org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder>
getShuffleFileOutputsFieldBuilder() {
if (shuffleFileOutputsBuilder_ == null) {
shuffleFileOutputsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.ShuffleFileOutput, org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder, org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder>(
shuffleFileOutputs_,
((bitField0_ & 0x00000080) == 0x00000080),
getParentForChildren(),
isClean());
shuffleFileOutputs_ = null;
}
return shuffleFileOutputsBuilder_;
}
// @@protoc_insertion_point(builder_scope:TaskStatusProto)
}
static {
defaultInstance = new TaskStatusProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TaskStatusProto)
}
public interface TaskCompletionReportOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required .TaskAttemptIdProto id = 1;
/**
* required .TaskAttemptIdProto id = 1;
*/
boolean hasId();
/**
* required .TaskAttemptIdProto id = 1;
*/
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId();
/**
* required .TaskAttemptIdProto id = 1;
*/
org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder();
// optional .StatSetProto stats = 2;
/**
* optional .StatSetProto stats = 2;
*/
boolean hasStats();
/**
* optional .StatSetProto stats = 2;
*/
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto getStats();
/**
* optional .StatSetProto stats = 2;
*/
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProtoOrBuilder getStatsOrBuilder();
// optional .TableStatsProto inputStats = 3;
/**
* optional .TableStatsProto inputStats = 3;
*/
boolean hasInputStats();
/**
* optional .TableStatsProto inputStats = 3;
*/
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto getInputStats();
/**
* optional .TableStatsProto inputStats = 3;
*/
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder getInputStatsOrBuilder();
// optional .TableStatsProto resultStats = 4;
/**
* optional .TableStatsProto resultStats = 4;
*/
boolean hasResultStats();
/**
* optional .TableStatsProto resultStats = 4;
*/
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto getResultStats();
/**
* optional .TableStatsProto resultStats = 4;
*/
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder getResultStatsOrBuilder();
// repeated .ShuffleFileOutput shuffleFileOutputs = 5;
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
java.util.List
getShuffleFileOutputsList();
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
org.apache.tajo.ResourceProtos.ShuffleFileOutput getShuffleFileOutputs(int index);
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
int getShuffleFileOutputsCount();
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
java.util.List extends org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder>
getShuffleFileOutputsOrBuilderList();
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder getShuffleFileOutputsOrBuilder(
int index);
// repeated .PartitionDescProto partitions = 6;
/**
* repeated .PartitionDescProto partitions = 6;
*/
java.util.List
getPartitionsList();
/**
* repeated .PartitionDescProto partitions = 6;
*/
org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto getPartitions(int index);
/**
* repeated .PartitionDescProto partitions = 6;
*/
int getPartitionsCount();
/**
* repeated .PartitionDescProto partitions = 6;
*/
java.util.List extends org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProtoOrBuilder>
getPartitionsOrBuilderList();
/**
* repeated .PartitionDescProto partitions = 6;
*/
org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProtoOrBuilder getPartitionsOrBuilder(
int index);
}
/**
* Protobuf type {@code TaskCompletionReport}
*/
public static final class TaskCompletionReport extends
com.google.protobuf.GeneratedMessage
implements TaskCompletionReportOrBuilder {
// Use TaskCompletionReport.newBuilder() to construct.
private TaskCompletionReport(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TaskCompletionReport(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TaskCompletionReport defaultInstance;
public static TaskCompletionReport getDefaultInstance() {
return defaultInstance;
}
public TaskCompletionReport getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TaskCompletionReport(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = id_.toBuilder();
}
id_ = input.readMessage(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(id_);
id_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = stats_.toBuilder();
}
stats_ = input.readMessage(org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(stats_);
stats_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = inputStats_.toBuilder();
}
inputStats_ = input.readMessage(org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(inputStats_);
inputStats_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 34: {
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = resultStats_.toBuilder();
}
resultStats_ = input.readMessage(org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(resultStats_);
resultStats_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
shuffleFileOutputs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
shuffleFileOutputs_.add(input.readMessage(org.apache.tajo.ResourceProtos.ShuffleFileOutput.PARSER, extensionRegistry));
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
partitions_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
partitions_.add(input.readMessage(org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
shuffleFileOutputs_ = java.util.Collections.unmodifiableList(shuffleFileOutputs_);
}
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
partitions_ = java.util.Collections.unmodifiableList(partitions_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TaskCompletionReport_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TaskCompletionReport_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TaskCompletionReport.class, org.apache.tajo.ResourceProtos.TaskCompletionReport.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TaskCompletionReport parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TaskCompletionReport(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .TaskAttemptIdProto id = 1;
public static final int ID_FIELD_NUMBER = 1;
private org.apache.tajo.TajoIdProtos.TaskAttemptIdProto id_;
/**
* required .TaskAttemptIdProto id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId() {
return id_;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder() {
return id_;
}
// optional .StatSetProto stats = 2;
public static final int STATS_FIELD_NUMBER = 2;
private org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto stats_;
/**
* optional .StatSetProto stats = 2;
*/
public boolean hasStats() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .StatSetProto stats = 2;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto getStats() {
return stats_;
}
/**
* optional .StatSetProto stats = 2;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.StatSetProtoOrBuilder getStatsOrBuilder() {
return stats_;
}
// optional .TableStatsProto inputStats = 3;
public static final int INPUTSTATS_FIELD_NUMBER = 3;
private org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto inputStats_;
/**
* optional .TableStatsProto inputStats = 3;
*/
public boolean hasInputStats() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .TableStatsProto inputStats = 3;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto getInputStats() {
return inputStats_;
}
/**
* optional .TableStatsProto inputStats = 3;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder getInputStatsOrBuilder() {
return inputStats_;
}
// optional .TableStatsProto resultStats = 4;
public static final int RESULTSTATS_FIELD_NUMBER = 4;
private org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto resultStats_;
/**
* optional .TableStatsProto resultStats = 4;
*/
public boolean hasResultStats() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .TableStatsProto resultStats = 4;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto getResultStats() {
return resultStats_;
}
/**
* optional .TableStatsProto resultStats = 4;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder getResultStatsOrBuilder() {
return resultStats_;
}
// repeated .ShuffleFileOutput shuffleFileOutputs = 5;
public static final int SHUFFLEFILEOUTPUTS_FIELD_NUMBER = 5;
private java.util.List shuffleFileOutputs_;
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public java.util.List getShuffleFileOutputsList() {
return shuffleFileOutputs_;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder>
getShuffleFileOutputsOrBuilderList() {
return shuffleFileOutputs_;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public int getShuffleFileOutputsCount() {
return shuffleFileOutputs_.size();
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutput getShuffleFileOutputs(int index) {
return shuffleFileOutputs_.get(index);
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder getShuffleFileOutputsOrBuilder(
int index) {
return shuffleFileOutputs_.get(index);
}
// repeated .PartitionDescProto partitions = 6;
public static final int PARTITIONS_FIELD_NUMBER = 6;
private java.util.List partitions_;
/**
* repeated .PartitionDescProto partitions = 6;
*/
public java.util.List getPartitionsList() {
return partitions_;
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public java.util.List extends org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProtoOrBuilder>
getPartitionsOrBuilderList() {
return partitions_;
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public int getPartitionsCount() {
return partitions_.size();
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto getPartitions(int index) {
return partitions_.get(index);
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProtoOrBuilder getPartitionsOrBuilder(
int index) {
return partitions_.get(index);
}
private void initFields() {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
stats_ = org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.getDefaultInstance();
inputStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
resultStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
shuffleFileOutputs_ = java.util.Collections.emptyList();
partitions_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasId()) {
memoizedIsInitialized = 0;
return false;
}
if (!getId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (hasStats()) {
if (!getStats().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasInputStats()) {
if (!getInputStats().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasResultStats()) {
if (!getResultStats().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getShuffleFileOutputsCount(); i++) {
if (!getShuffleFileOutputs(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getPartitionsCount(); i++) {
if (!getPartitions(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, stats_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, inputStats_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, resultStats_);
}
for (int i = 0; i < shuffleFileOutputs_.size(); i++) {
output.writeMessage(5, shuffleFileOutputs_.get(i));
}
for (int i = 0; i < partitions_.size(); i++) {
output.writeMessage(6, partitions_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, stats_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, inputStats_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, resultStats_);
}
for (int i = 0; i < shuffleFileOutputs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, shuffleFileOutputs_.get(i));
}
for (int i = 0; i < partitions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, partitions_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.TaskCompletionReport)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.TaskCompletionReport other = (org.apache.tajo.ResourceProtos.TaskCompletionReport) obj;
boolean result = true;
result = result && (hasId() == other.hasId());
if (hasId()) {
result = result && getId()
.equals(other.getId());
}
result = result && (hasStats() == other.hasStats());
if (hasStats()) {
result = result && getStats()
.equals(other.getStats());
}
result = result && (hasInputStats() == other.hasInputStats());
if (hasInputStats()) {
result = result && getInputStats()
.equals(other.getInputStats());
}
result = result && (hasResultStats() == other.hasResultStats());
if (hasResultStats()) {
result = result && getResultStats()
.equals(other.getResultStats());
}
result = result && getShuffleFileOutputsList()
.equals(other.getShuffleFileOutputsList());
result = result && getPartitionsList()
.equals(other.getPartitionsList());
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasStats()) {
hash = (37 * hash) + STATS_FIELD_NUMBER;
hash = (53 * hash) + getStats().hashCode();
}
if (hasInputStats()) {
hash = (37 * hash) + INPUTSTATS_FIELD_NUMBER;
hash = (53 * hash) + getInputStats().hashCode();
}
if (hasResultStats()) {
hash = (37 * hash) + RESULTSTATS_FIELD_NUMBER;
hash = (53 * hash) + getResultStats().hashCode();
}
if (getShuffleFileOutputsCount() > 0) {
hash = (37 * hash) + SHUFFLEFILEOUTPUTS_FIELD_NUMBER;
hash = (53 * hash) + getShuffleFileOutputsList().hashCode();
}
if (getPartitionsCount() > 0) {
hash = (37 * hash) + PARTITIONS_FIELD_NUMBER;
hash = (53 * hash) + getPartitionsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.TaskCompletionReport parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TaskCompletionReport parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskCompletionReport parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TaskCompletionReport parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskCompletionReport parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskCompletionReport parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskCompletionReport parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskCompletionReport parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskCompletionReport parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskCompletionReport parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.TaskCompletionReport prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TaskCompletionReport}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.TaskCompletionReportOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TaskCompletionReport_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TaskCompletionReport_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TaskCompletionReport.class, org.apache.tajo.ResourceProtos.TaskCompletionReport.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.TaskCompletionReport.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getIdFieldBuilder();
getStatsFieldBuilder();
getInputStatsFieldBuilder();
getResultStatsFieldBuilder();
getShuffleFileOutputsFieldBuilder();
getPartitionsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (idBuilder_ == null) {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (statsBuilder_ == null) {
stats_ = org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.getDefaultInstance();
} else {
statsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (inputStatsBuilder_ == null) {
inputStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
} else {
inputStatsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (resultStatsBuilder_ == null) {
resultStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
} else {
resultStatsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
if (shuffleFileOutputsBuilder_ == null) {
shuffleFileOutputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
shuffleFileOutputsBuilder_.clear();
}
if (partitionsBuilder_ == null) {
partitions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
partitionsBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_TaskCompletionReport_descriptor;
}
public org.apache.tajo.ResourceProtos.TaskCompletionReport getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.TaskCompletionReport.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.TaskCompletionReport build() {
org.apache.tajo.ResourceProtos.TaskCompletionReport result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.TaskCompletionReport buildPartial() {
org.apache.tajo.ResourceProtos.TaskCompletionReport result = new org.apache.tajo.ResourceProtos.TaskCompletionReport(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (idBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = idBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (statsBuilder_ == null) {
result.stats_ = stats_;
} else {
result.stats_ = statsBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (inputStatsBuilder_ == null) {
result.inputStats_ = inputStats_;
} else {
result.inputStats_ = inputStatsBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (resultStatsBuilder_ == null) {
result.resultStats_ = resultStats_;
} else {
result.resultStats_ = resultStatsBuilder_.build();
}
if (shuffleFileOutputsBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010)) {
shuffleFileOutputs_ = java.util.Collections.unmodifiableList(shuffleFileOutputs_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.shuffleFileOutputs_ = shuffleFileOutputs_;
} else {
result.shuffleFileOutputs_ = shuffleFileOutputsBuilder_.build();
}
if (partitionsBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
partitions_ = java.util.Collections.unmodifiableList(partitions_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.partitions_ = partitions_;
} else {
result.partitions_ = partitionsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.TaskCompletionReport) {
return mergeFrom((org.apache.tajo.ResourceProtos.TaskCompletionReport)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.TaskCompletionReport other) {
if (other == org.apache.tajo.ResourceProtos.TaskCompletionReport.getDefaultInstance()) return this;
if (other.hasId()) {
mergeId(other.getId());
}
if (other.hasStats()) {
mergeStats(other.getStats());
}
if (other.hasInputStats()) {
mergeInputStats(other.getInputStats());
}
if (other.hasResultStats()) {
mergeResultStats(other.getResultStats());
}
if (shuffleFileOutputsBuilder_ == null) {
if (!other.shuffleFileOutputs_.isEmpty()) {
if (shuffleFileOutputs_.isEmpty()) {
shuffleFileOutputs_ = other.shuffleFileOutputs_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.addAll(other.shuffleFileOutputs_);
}
onChanged();
}
} else {
if (!other.shuffleFileOutputs_.isEmpty()) {
if (shuffleFileOutputsBuilder_.isEmpty()) {
shuffleFileOutputsBuilder_.dispose();
shuffleFileOutputsBuilder_ = null;
shuffleFileOutputs_ = other.shuffleFileOutputs_;
bitField0_ = (bitField0_ & ~0x00000010);
shuffleFileOutputsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getShuffleFileOutputsFieldBuilder() : null;
} else {
shuffleFileOutputsBuilder_.addAllMessages(other.shuffleFileOutputs_);
}
}
}
if (partitionsBuilder_ == null) {
if (!other.partitions_.isEmpty()) {
if (partitions_.isEmpty()) {
partitions_ = other.partitions_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensurePartitionsIsMutable();
partitions_.addAll(other.partitions_);
}
onChanged();
}
} else {
if (!other.partitions_.isEmpty()) {
if (partitionsBuilder_.isEmpty()) {
partitionsBuilder_.dispose();
partitionsBuilder_ = null;
partitions_ = other.partitions_;
bitField0_ = (bitField0_ & ~0x00000020);
partitionsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getPartitionsFieldBuilder() : null;
} else {
partitionsBuilder_.addAllMessages(other.partitions_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasId()) {
return false;
}
if (!getId().isInitialized()) {
return false;
}
if (hasStats()) {
if (!getStats().isInitialized()) {
return false;
}
}
if (hasInputStats()) {
if (!getInputStats().isInitialized()) {
return false;
}
}
if (hasResultStats()) {
if (!getResultStats().isInitialized()) {
return false;
}
}
for (int i = 0; i < getShuffleFileOutputsCount(); i++) {
if (!getShuffleFileOutputs(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getPartitionsCount(); i++) {
if (!getPartitions(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.TaskCompletionReport parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.TaskCompletionReport) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .TaskAttemptIdProto id = 1;
private org.apache.tajo.TajoIdProtos.TaskAttemptIdProto id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder> idBuilder_;
/**
* required .TaskAttemptIdProto id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId() {
if (idBuilder_ == null) {
return id_;
} else {
return idBuilder_.getMessage();
}
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder setId(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto value) {
if (idBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
idBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder setId(
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder builderForValue) {
if (idBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
idBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder mergeId(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto value) {
if (idBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
id_ != org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance()) {
id_ =
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.newBuilder(id_).mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
idBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder clearId() {
if (idBuilder_ == null) {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
onChanged();
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder getIdBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getIdFieldBuilder().getBuilder();
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder() {
if (idBuilder_ != null) {
return idBuilder_.getMessageOrBuilder();
} else {
return id_;
}
}
/**
* required .TaskAttemptIdProto id = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>
getIdFieldBuilder() {
if (idBuilder_ == null) {
idBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>(
id_,
getParentForChildren(),
isClean());
id_ = null;
}
return idBuilder_;
}
// optional .StatSetProto stats = 2;
private org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto stats_ = org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto, org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.StatSetProtoOrBuilder> statsBuilder_;
/**
* optional .StatSetProto stats = 2;
*/
public boolean hasStats() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .StatSetProto stats = 2;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto getStats() {
if (statsBuilder_ == null) {
return stats_;
} else {
return statsBuilder_.getMessage();
}
}
/**
* optional .StatSetProto stats = 2;
*/
public Builder setStats(org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto value) {
if (statsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
stats_ = value;
onChanged();
} else {
statsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .StatSetProto stats = 2;
*/
public Builder setStats(
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.Builder builderForValue) {
if (statsBuilder_ == null) {
stats_ = builderForValue.build();
onChanged();
} else {
statsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .StatSetProto stats = 2;
*/
public Builder mergeStats(org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto value) {
if (statsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
stats_ != org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.getDefaultInstance()) {
stats_ =
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.newBuilder(stats_).mergeFrom(value).buildPartial();
} else {
stats_ = value;
}
onChanged();
} else {
statsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .StatSetProto stats = 2;
*/
public Builder clearStats() {
if (statsBuilder_ == null) {
stats_ = org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.getDefaultInstance();
onChanged();
} else {
statsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .StatSetProto stats = 2;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.Builder getStatsBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getStatsFieldBuilder().getBuilder();
}
/**
* optional .StatSetProto stats = 2;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.StatSetProtoOrBuilder getStatsOrBuilder() {
if (statsBuilder_ != null) {
return statsBuilder_.getMessageOrBuilder();
} else {
return stats_;
}
}
/**
* optional .StatSetProto stats = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto, org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.StatSetProtoOrBuilder>
getStatsFieldBuilder() {
if (statsBuilder_ == null) {
statsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto, org.apache.tajo.catalog.proto.CatalogProtos.StatSetProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.StatSetProtoOrBuilder>(
stats_,
getParentForChildren(),
isClean());
stats_ = null;
}
return statsBuilder_;
}
// optional .TableStatsProto inputStats = 3;
private org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto inputStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder> inputStatsBuilder_;
/**
* optional .TableStatsProto inputStats = 3;
*/
public boolean hasInputStats() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .TableStatsProto inputStats = 3;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto getInputStats() {
if (inputStatsBuilder_ == null) {
return inputStats_;
} else {
return inputStatsBuilder_.getMessage();
}
}
/**
* optional .TableStatsProto inputStats = 3;
*/
public Builder setInputStats(org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto value) {
if (inputStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
inputStats_ = value;
onChanged();
} else {
inputStatsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .TableStatsProto inputStats = 3;
*/
public Builder setInputStats(
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder builderForValue) {
if (inputStatsBuilder_ == null) {
inputStats_ = builderForValue.build();
onChanged();
} else {
inputStatsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .TableStatsProto inputStats = 3;
*/
public Builder mergeInputStats(org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto value) {
if (inputStatsBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
inputStats_ != org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance()) {
inputStats_ =
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.newBuilder(inputStats_).mergeFrom(value).buildPartial();
} else {
inputStats_ = value;
}
onChanged();
} else {
inputStatsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .TableStatsProto inputStats = 3;
*/
public Builder clearInputStats() {
if (inputStatsBuilder_ == null) {
inputStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
onChanged();
} else {
inputStatsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .TableStatsProto inputStats = 3;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder getInputStatsBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getInputStatsFieldBuilder().getBuilder();
}
/**
* optional .TableStatsProto inputStats = 3;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder getInputStatsOrBuilder() {
if (inputStatsBuilder_ != null) {
return inputStatsBuilder_.getMessageOrBuilder();
} else {
return inputStats_;
}
}
/**
* optional .TableStatsProto inputStats = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder>
getInputStatsFieldBuilder() {
if (inputStatsBuilder_ == null) {
inputStatsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder>(
inputStats_,
getParentForChildren(),
isClean());
inputStats_ = null;
}
return inputStatsBuilder_;
}
// optional .TableStatsProto resultStats = 4;
private org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto resultStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder> resultStatsBuilder_;
/**
* optional .TableStatsProto resultStats = 4;
*/
public boolean hasResultStats() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .TableStatsProto resultStats = 4;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto getResultStats() {
if (resultStatsBuilder_ == null) {
return resultStats_;
} else {
return resultStatsBuilder_.getMessage();
}
}
/**
* optional .TableStatsProto resultStats = 4;
*/
public Builder setResultStats(org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto value) {
if (resultStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resultStats_ = value;
onChanged();
} else {
resultStatsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .TableStatsProto resultStats = 4;
*/
public Builder setResultStats(
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder builderForValue) {
if (resultStatsBuilder_ == null) {
resultStats_ = builderForValue.build();
onChanged();
} else {
resultStatsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .TableStatsProto resultStats = 4;
*/
public Builder mergeResultStats(org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto value) {
if (resultStatsBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
resultStats_ != org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance()) {
resultStats_ =
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.newBuilder(resultStats_).mergeFrom(value).buildPartial();
} else {
resultStats_ = value;
}
onChanged();
} else {
resultStatsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .TableStatsProto resultStats = 4;
*/
public Builder clearResultStats() {
if (resultStatsBuilder_ == null) {
resultStats_ = org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.getDefaultInstance();
onChanged();
} else {
resultStatsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* optional .TableStatsProto resultStats = 4;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder getResultStatsBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getResultStatsFieldBuilder().getBuilder();
}
/**
* optional .TableStatsProto resultStats = 4;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder getResultStatsOrBuilder() {
if (resultStatsBuilder_ != null) {
return resultStatsBuilder_.getMessageOrBuilder();
} else {
return resultStats_;
}
}
/**
* optional .TableStatsProto resultStats = 4;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder>
getResultStatsFieldBuilder() {
if (resultStatsBuilder_ == null) {
resultStatsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableStatsProtoOrBuilder>(
resultStats_,
getParentForChildren(),
isClean());
resultStats_ = null;
}
return resultStatsBuilder_;
}
// repeated .ShuffleFileOutput shuffleFileOutputs = 5;
private java.util.List shuffleFileOutputs_ =
java.util.Collections.emptyList();
private void ensureShuffleFileOutputsIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
shuffleFileOutputs_ = new java.util.ArrayList(shuffleFileOutputs_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.ShuffleFileOutput, org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder, org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder> shuffleFileOutputsBuilder_;
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public java.util.List getShuffleFileOutputsList() {
if (shuffleFileOutputsBuilder_ == null) {
return java.util.Collections.unmodifiableList(shuffleFileOutputs_);
} else {
return shuffleFileOutputsBuilder_.getMessageList();
}
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public int getShuffleFileOutputsCount() {
if (shuffleFileOutputsBuilder_ == null) {
return shuffleFileOutputs_.size();
} else {
return shuffleFileOutputsBuilder_.getCount();
}
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutput getShuffleFileOutputs(int index) {
if (shuffleFileOutputsBuilder_ == null) {
return shuffleFileOutputs_.get(index);
} else {
return shuffleFileOutputsBuilder_.getMessage(index);
}
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public Builder setShuffleFileOutputs(
int index, org.apache.tajo.ResourceProtos.ShuffleFileOutput value) {
if (shuffleFileOutputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.set(index, value);
onChanged();
} else {
shuffleFileOutputsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public Builder setShuffleFileOutputs(
int index, org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder builderForValue) {
if (shuffleFileOutputsBuilder_ == null) {
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.set(index, builderForValue.build());
onChanged();
} else {
shuffleFileOutputsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public Builder addShuffleFileOutputs(org.apache.tajo.ResourceProtos.ShuffleFileOutput value) {
if (shuffleFileOutputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.add(value);
onChanged();
} else {
shuffleFileOutputsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public Builder addShuffleFileOutputs(
int index, org.apache.tajo.ResourceProtos.ShuffleFileOutput value) {
if (shuffleFileOutputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.add(index, value);
onChanged();
} else {
shuffleFileOutputsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public Builder addShuffleFileOutputs(
org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder builderForValue) {
if (shuffleFileOutputsBuilder_ == null) {
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.add(builderForValue.build());
onChanged();
} else {
shuffleFileOutputsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public Builder addShuffleFileOutputs(
int index, org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder builderForValue) {
if (shuffleFileOutputsBuilder_ == null) {
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.add(index, builderForValue.build());
onChanged();
} else {
shuffleFileOutputsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public Builder addAllShuffleFileOutputs(
java.lang.Iterable extends org.apache.tajo.ResourceProtos.ShuffleFileOutput> values) {
if (shuffleFileOutputsBuilder_ == null) {
ensureShuffleFileOutputsIsMutable();
super.addAll(values, shuffleFileOutputs_);
onChanged();
} else {
shuffleFileOutputsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public Builder clearShuffleFileOutputs() {
if (shuffleFileOutputsBuilder_ == null) {
shuffleFileOutputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
shuffleFileOutputsBuilder_.clear();
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public Builder removeShuffleFileOutputs(int index) {
if (shuffleFileOutputsBuilder_ == null) {
ensureShuffleFileOutputsIsMutable();
shuffleFileOutputs_.remove(index);
onChanged();
} else {
shuffleFileOutputsBuilder_.remove(index);
}
return this;
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder getShuffleFileOutputsBuilder(
int index) {
return getShuffleFileOutputsFieldBuilder().getBuilder(index);
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder getShuffleFileOutputsOrBuilder(
int index) {
if (shuffleFileOutputsBuilder_ == null) {
return shuffleFileOutputs_.get(index); } else {
return shuffleFileOutputsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder>
getShuffleFileOutputsOrBuilderList() {
if (shuffleFileOutputsBuilder_ != null) {
return shuffleFileOutputsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(shuffleFileOutputs_);
}
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder addShuffleFileOutputsBuilder() {
return getShuffleFileOutputsFieldBuilder().addBuilder(
org.apache.tajo.ResourceProtos.ShuffleFileOutput.getDefaultInstance());
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder addShuffleFileOutputsBuilder(
int index) {
return getShuffleFileOutputsFieldBuilder().addBuilder(
index, org.apache.tajo.ResourceProtos.ShuffleFileOutput.getDefaultInstance());
}
/**
* repeated .ShuffleFileOutput shuffleFileOutputs = 5;
*/
public java.util.List
getShuffleFileOutputsBuilderList() {
return getShuffleFileOutputsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.ShuffleFileOutput, org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder, org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder>
getShuffleFileOutputsFieldBuilder() {
if (shuffleFileOutputsBuilder_ == null) {
shuffleFileOutputsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.ShuffleFileOutput, org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder, org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder>(
shuffleFileOutputs_,
((bitField0_ & 0x00000010) == 0x00000010),
getParentForChildren(),
isClean());
shuffleFileOutputs_ = null;
}
return shuffleFileOutputsBuilder_;
}
// repeated .PartitionDescProto partitions = 6;
private java.util.List partitions_ =
java.util.Collections.emptyList();
private void ensurePartitionsIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
partitions_ = new java.util.ArrayList(partitions_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto, org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProtoOrBuilder> partitionsBuilder_;
/**
* repeated .PartitionDescProto partitions = 6;
*/
public java.util.List getPartitionsList() {
if (partitionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(partitions_);
} else {
return partitionsBuilder_.getMessageList();
}
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public int getPartitionsCount() {
if (partitionsBuilder_ == null) {
return partitions_.size();
} else {
return partitionsBuilder_.getCount();
}
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto getPartitions(int index) {
if (partitionsBuilder_ == null) {
return partitions_.get(index);
} else {
return partitionsBuilder_.getMessage(index);
}
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public Builder setPartitions(
int index, org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto value) {
if (partitionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePartitionsIsMutable();
partitions_.set(index, value);
onChanged();
} else {
partitionsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public Builder setPartitions(
int index, org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto.Builder builderForValue) {
if (partitionsBuilder_ == null) {
ensurePartitionsIsMutable();
partitions_.set(index, builderForValue.build());
onChanged();
} else {
partitionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public Builder addPartitions(org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto value) {
if (partitionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePartitionsIsMutable();
partitions_.add(value);
onChanged();
} else {
partitionsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public Builder addPartitions(
int index, org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto value) {
if (partitionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePartitionsIsMutable();
partitions_.add(index, value);
onChanged();
} else {
partitionsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public Builder addPartitions(
org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto.Builder builderForValue) {
if (partitionsBuilder_ == null) {
ensurePartitionsIsMutable();
partitions_.add(builderForValue.build());
onChanged();
} else {
partitionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public Builder addPartitions(
int index, org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto.Builder builderForValue) {
if (partitionsBuilder_ == null) {
ensurePartitionsIsMutable();
partitions_.add(index, builderForValue.build());
onChanged();
} else {
partitionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public Builder addAllPartitions(
java.lang.Iterable extends org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto> values) {
if (partitionsBuilder_ == null) {
ensurePartitionsIsMutable();
super.addAll(values, partitions_);
onChanged();
} else {
partitionsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public Builder clearPartitions() {
if (partitionsBuilder_ == null) {
partitions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
partitionsBuilder_.clear();
}
return this;
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public Builder removePartitions(int index) {
if (partitionsBuilder_ == null) {
ensurePartitionsIsMutable();
partitions_.remove(index);
onChanged();
} else {
partitionsBuilder_.remove(index);
}
return this;
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto.Builder getPartitionsBuilder(
int index) {
return getPartitionsFieldBuilder().getBuilder(index);
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProtoOrBuilder getPartitionsOrBuilder(
int index) {
if (partitionsBuilder_ == null) {
return partitions_.get(index); } else {
return partitionsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public java.util.List extends org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProtoOrBuilder>
getPartitionsOrBuilderList() {
if (partitionsBuilder_ != null) {
return partitionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(partitions_);
}
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto.Builder addPartitionsBuilder() {
return getPartitionsFieldBuilder().addBuilder(
org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto.getDefaultInstance());
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto.Builder addPartitionsBuilder(
int index) {
return getPartitionsFieldBuilder().addBuilder(
index, org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto.getDefaultInstance());
}
/**
* repeated .PartitionDescProto partitions = 6;
*/
public java.util.List
getPartitionsBuilderList() {
return getPartitionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto, org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProtoOrBuilder>
getPartitionsFieldBuilder() {
if (partitionsBuilder_ == null) {
partitionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto, org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.PartitionDescProtoOrBuilder>(
partitions_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
partitions_ = null;
}
return partitionsBuilder_;
}
// @@protoc_insertion_point(builder_scope:TaskCompletionReport)
}
static {
defaultInstance = new TaskCompletionReport(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TaskCompletionReport)
}
public interface TaskFatalErrorReportOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required .TaskAttemptIdProto id = 1;
/**
* required .TaskAttemptIdProto id = 1;
*/
boolean hasId();
/**
* required .TaskAttemptIdProto id = 1;
*/
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId();
/**
* required .TaskAttemptIdProto id = 1;
*/
org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder();
// required .tajo.error.SerializedException error = 2;
/**
* required .tajo.error.SerializedException error = 2;
*/
boolean hasError();
/**
* required .tajo.error.SerializedException error = 2;
*/
org.apache.tajo.error.Errors.SerializedException getError();
/**
* required .tajo.error.SerializedException error = 2;
*/
org.apache.tajo.error.Errors.SerializedExceptionOrBuilder getErrorOrBuilder();
}
/**
* Protobuf type {@code TaskFatalErrorReport}
*/
public static final class TaskFatalErrorReport extends
com.google.protobuf.GeneratedMessage
implements TaskFatalErrorReportOrBuilder {
// Use TaskFatalErrorReport.newBuilder() to construct.
private TaskFatalErrorReport(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TaskFatalErrorReport(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TaskFatalErrorReport defaultInstance;
public static TaskFatalErrorReport getDefaultInstance() {
return defaultInstance;
}
public TaskFatalErrorReport getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TaskFatalErrorReport(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = id_.toBuilder();
}
id_ = input.readMessage(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(id_);
id_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
org.apache.tajo.error.Errors.SerializedException.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = error_.toBuilder();
}
error_ = input.readMessage(org.apache.tajo.error.Errors.SerializedException.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(error_);
error_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TaskFatalErrorReport_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TaskFatalErrorReport_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TaskFatalErrorReport.class, org.apache.tajo.ResourceProtos.TaskFatalErrorReport.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TaskFatalErrorReport parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TaskFatalErrorReport(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .TaskAttemptIdProto id = 1;
public static final int ID_FIELD_NUMBER = 1;
private org.apache.tajo.TajoIdProtos.TaskAttemptIdProto id_;
/**
* required .TaskAttemptIdProto id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId() {
return id_;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder() {
return id_;
}
// required .tajo.error.SerializedException error = 2;
public static final int ERROR_FIELD_NUMBER = 2;
private org.apache.tajo.error.Errors.SerializedException error_;
/**
* required .tajo.error.SerializedException error = 2;
*/
public boolean hasError() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .tajo.error.SerializedException error = 2;
*/
public org.apache.tajo.error.Errors.SerializedException getError() {
return error_;
}
/**
* required .tajo.error.SerializedException error = 2;
*/
public org.apache.tajo.error.Errors.SerializedExceptionOrBuilder getErrorOrBuilder() {
return error_;
}
private void initFields() {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
error_ = org.apache.tajo.error.Errors.SerializedException.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasError()) {
memoizedIsInitialized = 0;
return false;
}
if (!getId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (!getError().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, error_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, error_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.TaskFatalErrorReport)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.TaskFatalErrorReport other = (org.apache.tajo.ResourceProtos.TaskFatalErrorReport) obj;
boolean result = true;
result = result && (hasId() == other.hasId());
if (hasId()) {
result = result && getId()
.equals(other.getId());
}
result = result && (hasError() == other.hasError());
if (hasError()) {
result = result && getError()
.equals(other.getError());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasError()) {
hash = (37 * hash) + ERROR_FIELD_NUMBER;
hash = (53 * hash) + getError().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.TaskFatalErrorReport parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TaskFatalErrorReport parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskFatalErrorReport parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TaskFatalErrorReport parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskFatalErrorReport parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskFatalErrorReport parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskFatalErrorReport parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskFatalErrorReport parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskFatalErrorReport parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskFatalErrorReport parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.TaskFatalErrorReport prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TaskFatalErrorReport}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.TaskFatalErrorReportOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TaskFatalErrorReport_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TaskFatalErrorReport_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TaskFatalErrorReport.class, org.apache.tajo.ResourceProtos.TaskFatalErrorReport.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.TaskFatalErrorReport.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getIdFieldBuilder();
getErrorFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (idBuilder_ == null) {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (errorBuilder_ == null) {
error_ = org.apache.tajo.error.Errors.SerializedException.getDefaultInstance();
} else {
errorBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_TaskFatalErrorReport_descriptor;
}
public org.apache.tajo.ResourceProtos.TaskFatalErrorReport getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.TaskFatalErrorReport.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.TaskFatalErrorReport build() {
org.apache.tajo.ResourceProtos.TaskFatalErrorReport result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.TaskFatalErrorReport buildPartial() {
org.apache.tajo.ResourceProtos.TaskFatalErrorReport result = new org.apache.tajo.ResourceProtos.TaskFatalErrorReport(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (idBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = idBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (errorBuilder_ == null) {
result.error_ = error_;
} else {
result.error_ = errorBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.TaskFatalErrorReport) {
return mergeFrom((org.apache.tajo.ResourceProtos.TaskFatalErrorReport)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.TaskFatalErrorReport other) {
if (other == org.apache.tajo.ResourceProtos.TaskFatalErrorReport.getDefaultInstance()) return this;
if (other.hasId()) {
mergeId(other.getId());
}
if (other.hasError()) {
mergeError(other.getError());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasId()) {
return false;
}
if (!hasError()) {
return false;
}
if (!getId().isInitialized()) {
return false;
}
if (!getError().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.TaskFatalErrorReport parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.TaskFatalErrorReport) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .TaskAttemptIdProto id = 1;
private org.apache.tajo.TajoIdProtos.TaskAttemptIdProto id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder> idBuilder_;
/**
* required .TaskAttemptIdProto id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId() {
if (idBuilder_ == null) {
return id_;
} else {
return idBuilder_.getMessage();
}
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder setId(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto value) {
if (idBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
idBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder setId(
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder builderForValue) {
if (idBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
idBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder mergeId(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto value) {
if (idBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
id_ != org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance()) {
id_ =
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.newBuilder(id_).mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
idBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder clearId() {
if (idBuilder_ == null) {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
onChanged();
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder getIdBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getIdFieldBuilder().getBuilder();
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder() {
if (idBuilder_ != null) {
return idBuilder_.getMessageOrBuilder();
} else {
return id_;
}
}
/**
* required .TaskAttemptIdProto id = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>
getIdFieldBuilder() {
if (idBuilder_ == null) {
idBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>(
id_,
getParentForChildren(),
isClean());
id_ = null;
}
return idBuilder_;
}
// required .tajo.error.SerializedException error = 2;
private org.apache.tajo.error.Errors.SerializedException error_ = org.apache.tajo.error.Errors.SerializedException.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.error.Errors.SerializedException, org.apache.tajo.error.Errors.SerializedException.Builder, org.apache.tajo.error.Errors.SerializedExceptionOrBuilder> errorBuilder_;
/**
* required .tajo.error.SerializedException error = 2;
*/
public boolean hasError() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .tajo.error.SerializedException error = 2;
*/
public org.apache.tajo.error.Errors.SerializedException getError() {
if (errorBuilder_ == null) {
return error_;
} else {
return errorBuilder_.getMessage();
}
}
/**
* required .tajo.error.SerializedException error = 2;
*/
public Builder setError(org.apache.tajo.error.Errors.SerializedException value) {
if (errorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
error_ = value;
onChanged();
} else {
errorBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .tajo.error.SerializedException error = 2;
*/
public Builder setError(
org.apache.tajo.error.Errors.SerializedException.Builder builderForValue) {
if (errorBuilder_ == null) {
error_ = builderForValue.build();
onChanged();
} else {
errorBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .tajo.error.SerializedException error = 2;
*/
public Builder mergeError(org.apache.tajo.error.Errors.SerializedException value) {
if (errorBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
error_ != org.apache.tajo.error.Errors.SerializedException.getDefaultInstance()) {
error_ =
org.apache.tajo.error.Errors.SerializedException.newBuilder(error_).mergeFrom(value).buildPartial();
} else {
error_ = value;
}
onChanged();
} else {
errorBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .tajo.error.SerializedException error = 2;
*/
public Builder clearError() {
if (errorBuilder_ == null) {
error_ = org.apache.tajo.error.Errors.SerializedException.getDefaultInstance();
onChanged();
} else {
errorBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* required .tajo.error.SerializedException error = 2;
*/
public org.apache.tajo.error.Errors.SerializedException.Builder getErrorBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getErrorFieldBuilder().getBuilder();
}
/**
* required .tajo.error.SerializedException error = 2;
*/
public org.apache.tajo.error.Errors.SerializedExceptionOrBuilder getErrorOrBuilder() {
if (errorBuilder_ != null) {
return errorBuilder_.getMessageOrBuilder();
} else {
return error_;
}
}
/**
* required .tajo.error.SerializedException error = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.error.Errors.SerializedException, org.apache.tajo.error.Errors.SerializedException.Builder, org.apache.tajo.error.Errors.SerializedExceptionOrBuilder>
getErrorFieldBuilder() {
if (errorBuilder_ == null) {
errorBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.error.Errors.SerializedException, org.apache.tajo.error.Errors.SerializedException.Builder, org.apache.tajo.error.Errors.SerializedExceptionOrBuilder>(
error_,
getParentForChildren(),
isClean());
error_ = null;
}
return errorBuilder_;
}
// @@protoc_insertion_point(builder_scope:TaskFatalErrorReport)
}
static {
defaultInstance = new TaskFatalErrorReport(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TaskFatalErrorReport)
}
public interface FailureIntermediateProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int64 pagePos = 1;
/**
* required int64 pagePos = 1;
*/
boolean hasPagePos();
/**
* required int64 pagePos = 1;
*/
long getPagePos();
// required int32 startRowNum = 2;
/**
* required int32 startRowNum = 2;
*/
boolean hasStartRowNum();
/**
* required int32 startRowNum = 2;
*/
int getStartRowNum();
// required int32 endRowNum = 3;
/**
* required int32 endRowNum = 3;
*/
boolean hasEndRowNum();
/**
* required int32 endRowNum = 3;
*/
int getEndRowNum();
}
/**
* Protobuf type {@code FailureIntermediateProto}
*/
public static final class FailureIntermediateProto extends
com.google.protobuf.GeneratedMessage
implements FailureIntermediateProtoOrBuilder {
// Use FailureIntermediateProto.newBuilder() to construct.
private FailureIntermediateProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private FailureIntermediateProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final FailureIntermediateProto defaultInstance;
public static FailureIntermediateProto getDefaultInstance() {
return defaultInstance;
}
public FailureIntermediateProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FailureIntermediateProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
pagePos_ = input.readInt64();
break;
}
case 16: {
bitField0_ |= 0x00000002;
startRowNum_ = input.readInt32();
break;
}
case 24: {
bitField0_ |= 0x00000004;
endRowNum_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_FailureIntermediateProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_FailureIntermediateProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.FailureIntermediateProto.class, org.apache.tajo.ResourceProtos.FailureIntermediateProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public FailureIntermediateProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FailureIntermediateProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required int64 pagePos = 1;
public static final int PAGEPOS_FIELD_NUMBER = 1;
private long pagePos_;
/**
* required int64 pagePos = 1;
*/
public boolean hasPagePos() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 pagePos = 1;
*/
public long getPagePos() {
return pagePos_;
}
// required int32 startRowNum = 2;
public static final int STARTROWNUM_FIELD_NUMBER = 2;
private int startRowNum_;
/**
* required int32 startRowNum = 2;
*/
public boolean hasStartRowNum() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 startRowNum = 2;
*/
public int getStartRowNum() {
return startRowNum_;
}
// required int32 endRowNum = 3;
public static final int ENDROWNUM_FIELD_NUMBER = 3;
private int endRowNum_;
/**
* required int32 endRowNum = 3;
*/
public boolean hasEndRowNum() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required int32 endRowNum = 3;
*/
public int getEndRowNum() {
return endRowNum_;
}
private void initFields() {
pagePos_ = 0L;
startRowNum_ = 0;
endRowNum_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasPagePos()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasStartRowNum()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasEndRowNum()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, pagePos_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, startRowNum_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, endRowNum_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, pagePos_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, startRowNum_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, endRowNum_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.FailureIntermediateProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.FailureIntermediateProto other = (org.apache.tajo.ResourceProtos.FailureIntermediateProto) obj;
boolean result = true;
result = result && (hasPagePos() == other.hasPagePos());
if (hasPagePos()) {
result = result && (getPagePos()
== other.getPagePos());
}
result = result && (hasStartRowNum() == other.hasStartRowNum());
if (hasStartRowNum()) {
result = result && (getStartRowNum()
== other.getStartRowNum());
}
result = result && (hasEndRowNum() == other.hasEndRowNum());
if (hasEndRowNum()) {
result = result && (getEndRowNum()
== other.getEndRowNum());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasPagePos()) {
hash = (37 * hash) + PAGEPOS_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getPagePos());
}
if (hasStartRowNum()) {
hash = (37 * hash) + STARTROWNUM_FIELD_NUMBER;
hash = (53 * hash) + getStartRowNum();
}
if (hasEndRowNum()) {
hash = (37 * hash) + ENDROWNUM_FIELD_NUMBER;
hash = (53 * hash) + getEndRowNum();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.FailureIntermediateProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.FailureIntermediateProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.FailureIntermediateProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.FailureIntermediateProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.FailureIntermediateProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.FailureIntermediateProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.FailureIntermediateProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.FailureIntermediateProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.FailureIntermediateProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.FailureIntermediateProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.FailureIntermediateProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code FailureIntermediateProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.FailureIntermediateProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_FailureIntermediateProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_FailureIntermediateProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.FailureIntermediateProto.class, org.apache.tajo.ResourceProtos.FailureIntermediateProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.FailureIntermediateProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
pagePos_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
startRowNum_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
endRowNum_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_FailureIntermediateProto_descriptor;
}
public org.apache.tajo.ResourceProtos.FailureIntermediateProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.FailureIntermediateProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.FailureIntermediateProto build() {
org.apache.tajo.ResourceProtos.FailureIntermediateProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.FailureIntermediateProto buildPartial() {
org.apache.tajo.ResourceProtos.FailureIntermediateProto result = new org.apache.tajo.ResourceProtos.FailureIntermediateProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.pagePos_ = pagePos_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.startRowNum_ = startRowNum_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.endRowNum_ = endRowNum_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.FailureIntermediateProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.FailureIntermediateProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.FailureIntermediateProto other) {
if (other == org.apache.tajo.ResourceProtos.FailureIntermediateProto.getDefaultInstance()) return this;
if (other.hasPagePos()) {
setPagePos(other.getPagePos());
}
if (other.hasStartRowNum()) {
setStartRowNum(other.getStartRowNum());
}
if (other.hasEndRowNum()) {
setEndRowNum(other.getEndRowNum());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasPagePos()) {
return false;
}
if (!hasStartRowNum()) {
return false;
}
if (!hasEndRowNum()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.FailureIntermediateProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.FailureIntermediateProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int64 pagePos = 1;
private long pagePos_ ;
/**
* required int64 pagePos = 1;
*/
public boolean hasPagePos() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 pagePos = 1;
*/
public long getPagePos() {
return pagePos_;
}
/**
* required int64 pagePos = 1;
*/
public Builder setPagePos(long value) {
bitField0_ |= 0x00000001;
pagePos_ = value;
onChanged();
return this;
}
/**
* required int64 pagePos = 1;
*/
public Builder clearPagePos() {
bitField0_ = (bitField0_ & ~0x00000001);
pagePos_ = 0L;
onChanged();
return this;
}
// required int32 startRowNum = 2;
private int startRowNum_ ;
/**
* required int32 startRowNum = 2;
*/
public boolean hasStartRowNum() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 startRowNum = 2;
*/
public int getStartRowNum() {
return startRowNum_;
}
/**
* required int32 startRowNum = 2;
*/
public Builder setStartRowNum(int value) {
bitField0_ |= 0x00000002;
startRowNum_ = value;
onChanged();
return this;
}
/**
* required int32 startRowNum = 2;
*/
public Builder clearStartRowNum() {
bitField0_ = (bitField0_ & ~0x00000002);
startRowNum_ = 0;
onChanged();
return this;
}
// required int32 endRowNum = 3;
private int endRowNum_ ;
/**
* required int32 endRowNum = 3;
*/
public boolean hasEndRowNum() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required int32 endRowNum = 3;
*/
public int getEndRowNum() {
return endRowNum_;
}
/**
* required int32 endRowNum = 3;
*/
public Builder setEndRowNum(int value) {
bitField0_ |= 0x00000004;
endRowNum_ = value;
onChanged();
return this;
}
/**
* required int32 endRowNum = 3;
*/
public Builder clearEndRowNum() {
bitField0_ = (bitField0_ & ~0x00000004);
endRowNum_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:FailureIntermediateProto)
}
static {
defaultInstance = new FailureIntermediateProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:FailureIntermediateProto)
}
public interface IntermediateEntryProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required .ExecutionBlockIdProto ebId = 1;
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
boolean hasEbId();
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto getEbId();
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder getEbIdOrBuilder();
// required int32 taskId = 2;
/**
* required int32 taskId = 2;
*/
boolean hasTaskId();
/**
* required int32 taskId = 2;
*/
int getTaskId();
// required int32 attemptId = 3;
/**
* required int32 attemptId = 3;
*/
boolean hasAttemptId();
/**
* required int32 attemptId = 3;
*/
int getAttemptId();
// required int32 partId = 4;
/**
* required int32 partId = 4;
*/
boolean hasPartId();
/**
* required int32 partId = 4;
*/
int getPartId();
// required string host = 5;
/**
* required string host = 5;
*/
boolean hasHost();
/**
* required string host = 5;
*/
java.lang.String getHost();
/**
* required string host = 5;
*/
com.google.protobuf.ByteString
getHostBytes();
// required int64 volume = 6;
/**
* required int64 volume = 6;
*/
boolean hasVolume();
/**
* required int64 volume = 6;
*/
long getVolume();
// repeated .IntermediateEntryProto.PageProto pages = 7;
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
java.util.List
getPagesList();
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto getPages(int index);
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
int getPagesCount();
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
java.util.List extends org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProtoOrBuilder>
getPagesOrBuilderList();
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProtoOrBuilder getPagesOrBuilder(
int index);
// repeated .FailureIntermediateProto failures = 8;
/**
* repeated .FailureIntermediateProto failures = 8;
*/
java.util.List
getFailuresList();
/**
* repeated .FailureIntermediateProto failures = 8;
*/
org.apache.tajo.ResourceProtos.FailureIntermediateProto getFailures(int index);
/**
* repeated .FailureIntermediateProto failures = 8;
*/
int getFailuresCount();
/**
* repeated .FailureIntermediateProto failures = 8;
*/
java.util.List extends org.apache.tajo.ResourceProtos.FailureIntermediateProtoOrBuilder>
getFailuresOrBuilderList();
/**
* repeated .FailureIntermediateProto failures = 8;
*/
org.apache.tajo.ResourceProtos.FailureIntermediateProtoOrBuilder getFailuresOrBuilder(
int index);
}
/**
* Protobuf type {@code IntermediateEntryProto}
*/
public static final class IntermediateEntryProto extends
com.google.protobuf.GeneratedMessage
implements IntermediateEntryProtoOrBuilder {
// Use IntermediateEntryProto.newBuilder() to construct.
private IntermediateEntryProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private IntermediateEntryProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final IntermediateEntryProto defaultInstance;
public static IntermediateEntryProto getDefaultInstance() {
return defaultInstance;
}
public IntermediateEntryProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private IntermediateEntryProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = ebId_.toBuilder();
}
ebId_ = input.readMessage(org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(ebId_);
ebId_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 16: {
bitField0_ |= 0x00000002;
taskId_ = input.readInt32();
break;
}
case 24: {
bitField0_ |= 0x00000004;
attemptId_ = input.readInt32();
break;
}
case 32: {
bitField0_ |= 0x00000008;
partId_ = input.readInt32();
break;
}
case 42: {
bitField0_ |= 0x00000010;
host_ = input.readBytes();
break;
}
case 48: {
bitField0_ |= 0x00000020;
volume_ = input.readInt64();
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
pages_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
pages_.add(input.readMessage(org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.PARSER, extensionRegistry));
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
failures_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000080;
}
failures_.add(input.readMessage(org.apache.tajo.ResourceProtos.FailureIntermediateProto.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
pages_ = java.util.Collections.unmodifiableList(pages_);
}
if (((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
failures_ = java.util.Collections.unmodifiableList(failures_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_IntermediateEntryProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_IntermediateEntryProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.IntermediateEntryProto.class, org.apache.tajo.ResourceProtos.IntermediateEntryProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public IntermediateEntryProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new IntermediateEntryProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public interface PageProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int64 pos = 1;
/**
* required int64 pos = 1;
*/
boolean hasPos();
/**
* required int64 pos = 1;
*/
long getPos();
// required int32 length = 2;
/**
* required int32 length = 2;
*/
boolean hasLength();
/**
* required int32 length = 2;
*/
int getLength();
}
/**
* Protobuf type {@code IntermediateEntryProto.PageProto}
*/
public static final class PageProto extends
com.google.protobuf.GeneratedMessage
implements PageProtoOrBuilder {
// Use PageProto.newBuilder() to construct.
private PageProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private PageProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final PageProto defaultInstance;
public static PageProto getDefaultInstance() {
return defaultInstance;
}
public PageProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PageProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
pos_ = input.readInt64();
break;
}
case 16: {
bitField0_ |= 0x00000002;
length_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_IntermediateEntryProto_PageProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_IntermediateEntryProto_PageProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.class, org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public PageProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PageProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required int64 pos = 1;
public static final int POS_FIELD_NUMBER = 1;
private long pos_;
/**
* required int64 pos = 1;
*/
public boolean hasPos() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 pos = 1;
*/
public long getPos() {
return pos_;
}
// required int32 length = 2;
public static final int LENGTH_FIELD_NUMBER = 2;
private int length_;
/**
* required int32 length = 2;
*/
public boolean hasLength() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 length = 2;
*/
public int getLength() {
return length_;
}
private void initFields() {
pos_ = 0L;
length_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasPos()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasLength()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, pos_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, length_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, pos_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, length_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto other = (org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto) obj;
boolean result = true;
result = result && (hasPos() == other.hasPos());
if (hasPos()) {
result = result && (getPos()
== other.getPos());
}
result = result && (hasLength() == other.hasLength());
if (hasLength()) {
result = result && (getLength()
== other.getLength());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasPos()) {
hash = (37 * hash) + POS_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getPos());
}
if (hasLength()) {
hash = (37 * hash) + LENGTH_FIELD_NUMBER;
hash = (53 * hash) + getLength();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code IntermediateEntryProto.PageProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_IntermediateEntryProto_PageProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_IntermediateEntryProto_PageProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.class, org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
pos_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
length_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_IntermediateEntryProto_PageProto_descriptor;
}
public org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto build() {
org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto buildPartial() {
org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto result = new org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.pos_ = pos_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.length_ = length_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto other) {
if (other == org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.getDefaultInstance()) return this;
if (other.hasPos()) {
setPos(other.getPos());
}
if (other.hasLength()) {
setLength(other.getLength());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasPos()) {
return false;
}
if (!hasLength()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int64 pos = 1;
private long pos_ ;
/**
* required int64 pos = 1;
*/
public boolean hasPos() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 pos = 1;
*/
public long getPos() {
return pos_;
}
/**
* required int64 pos = 1;
*/
public Builder setPos(long value) {
bitField0_ |= 0x00000001;
pos_ = value;
onChanged();
return this;
}
/**
* required int64 pos = 1;
*/
public Builder clearPos() {
bitField0_ = (bitField0_ & ~0x00000001);
pos_ = 0L;
onChanged();
return this;
}
// required int32 length = 2;
private int length_ ;
/**
* required int32 length = 2;
*/
public boolean hasLength() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 length = 2;
*/
public int getLength() {
return length_;
}
/**
* required int32 length = 2;
*/
public Builder setLength(int value) {
bitField0_ |= 0x00000002;
length_ = value;
onChanged();
return this;
}
/**
* required int32 length = 2;
*/
public Builder clearLength() {
bitField0_ = (bitField0_ & ~0x00000002);
length_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:IntermediateEntryProto.PageProto)
}
static {
defaultInstance = new PageProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:IntermediateEntryProto.PageProto)
}
private int bitField0_;
// required .ExecutionBlockIdProto ebId = 1;
public static final int EBID_FIELD_NUMBER = 1;
private org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto ebId_;
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public boolean hasEbId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto getEbId() {
return ebId_;
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder getEbIdOrBuilder() {
return ebId_;
}
// required int32 taskId = 2;
public static final int TASKID_FIELD_NUMBER = 2;
private int taskId_;
/**
* required int32 taskId = 2;
*/
public boolean hasTaskId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 taskId = 2;
*/
public int getTaskId() {
return taskId_;
}
// required int32 attemptId = 3;
public static final int ATTEMPTID_FIELD_NUMBER = 3;
private int attemptId_;
/**
* required int32 attemptId = 3;
*/
public boolean hasAttemptId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required int32 attemptId = 3;
*/
public int getAttemptId() {
return attemptId_;
}
// required int32 partId = 4;
public static final int PARTID_FIELD_NUMBER = 4;
private int partId_;
/**
* required int32 partId = 4;
*/
public boolean hasPartId() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required int32 partId = 4;
*/
public int getPartId() {
return partId_;
}
// required string host = 5;
public static final int HOST_FIELD_NUMBER = 5;
private java.lang.Object host_;
/**
* required string host = 5;
*/
public boolean hasHost() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required string host = 5;
*/
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
host_ = s;
}
return s;
}
}
/**
* required string host = 5;
*/
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required int64 volume = 6;
public static final int VOLUME_FIELD_NUMBER = 6;
private long volume_;
/**
* required int64 volume = 6;
*/
public boolean hasVolume() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* required int64 volume = 6;
*/
public long getVolume() {
return volume_;
}
// repeated .IntermediateEntryProto.PageProto pages = 7;
public static final int PAGES_FIELD_NUMBER = 7;
private java.util.List pages_;
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public java.util.List getPagesList() {
return pages_;
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProtoOrBuilder>
getPagesOrBuilderList() {
return pages_;
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public int getPagesCount() {
return pages_.size();
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto getPages(int index) {
return pages_.get(index);
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProtoOrBuilder getPagesOrBuilder(
int index) {
return pages_.get(index);
}
// repeated .FailureIntermediateProto failures = 8;
public static final int FAILURES_FIELD_NUMBER = 8;
private java.util.List failures_;
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public java.util.List getFailuresList() {
return failures_;
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.FailureIntermediateProtoOrBuilder>
getFailuresOrBuilderList() {
return failures_;
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public int getFailuresCount() {
return failures_.size();
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public org.apache.tajo.ResourceProtos.FailureIntermediateProto getFailures(int index) {
return failures_.get(index);
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public org.apache.tajo.ResourceProtos.FailureIntermediateProtoOrBuilder getFailuresOrBuilder(
int index) {
return failures_.get(index);
}
private void initFields() {
ebId_ = org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance();
taskId_ = 0;
attemptId_ = 0;
partId_ = 0;
host_ = "";
volume_ = 0L;
pages_ = java.util.Collections.emptyList();
failures_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasEbId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasTaskId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasAttemptId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPartId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasHost()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasVolume()) {
memoizedIsInitialized = 0;
return false;
}
if (!getEbId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getPagesCount(); i++) {
if (!getPages(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getFailuresCount(); i++) {
if (!getFailures(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, ebId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, taskId_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, attemptId_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt32(4, partId_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(5, getHostBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeInt64(6, volume_);
}
for (int i = 0; i < pages_.size(); i++) {
output.writeMessage(7, pages_.get(i));
}
for (int i = 0; i < failures_.size(); i++) {
output.writeMessage(8, failures_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, ebId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, taskId_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, attemptId_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, partId_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, getHostBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(6, volume_);
}
for (int i = 0; i < pages_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, pages_.get(i));
}
for (int i = 0; i < failures_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, failures_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.IntermediateEntryProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.IntermediateEntryProto other = (org.apache.tajo.ResourceProtos.IntermediateEntryProto) obj;
boolean result = true;
result = result && (hasEbId() == other.hasEbId());
if (hasEbId()) {
result = result && getEbId()
.equals(other.getEbId());
}
result = result && (hasTaskId() == other.hasTaskId());
if (hasTaskId()) {
result = result && (getTaskId()
== other.getTaskId());
}
result = result && (hasAttemptId() == other.hasAttemptId());
if (hasAttemptId()) {
result = result && (getAttemptId()
== other.getAttemptId());
}
result = result && (hasPartId() == other.hasPartId());
if (hasPartId()) {
result = result && (getPartId()
== other.getPartId());
}
result = result && (hasHost() == other.hasHost());
if (hasHost()) {
result = result && getHost()
.equals(other.getHost());
}
result = result && (hasVolume() == other.hasVolume());
if (hasVolume()) {
result = result && (getVolume()
== other.getVolume());
}
result = result && getPagesList()
.equals(other.getPagesList());
result = result && getFailuresList()
.equals(other.getFailuresList());
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasEbId()) {
hash = (37 * hash) + EBID_FIELD_NUMBER;
hash = (53 * hash) + getEbId().hashCode();
}
if (hasTaskId()) {
hash = (37 * hash) + TASKID_FIELD_NUMBER;
hash = (53 * hash) + getTaskId();
}
if (hasAttemptId()) {
hash = (37 * hash) + ATTEMPTID_FIELD_NUMBER;
hash = (53 * hash) + getAttemptId();
}
if (hasPartId()) {
hash = (37 * hash) + PARTID_FIELD_NUMBER;
hash = (53 * hash) + getPartId();
}
if (hasHost()) {
hash = (37 * hash) + HOST_FIELD_NUMBER;
hash = (53 * hash) + getHost().hashCode();
}
if (hasVolume()) {
hash = (37 * hash) + VOLUME_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getVolume());
}
if (getPagesCount() > 0) {
hash = (37 * hash) + PAGES_FIELD_NUMBER;
hash = (53 * hash) + getPagesList().hashCode();
}
if (getFailuresCount() > 0) {
hash = (37 * hash) + FAILURES_FIELD_NUMBER;
hash = (53 * hash) + getFailuresList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.IntermediateEntryProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.IntermediateEntryProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code IntermediateEntryProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.IntermediateEntryProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_IntermediateEntryProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_IntermediateEntryProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.IntermediateEntryProto.class, org.apache.tajo.ResourceProtos.IntermediateEntryProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.IntermediateEntryProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getEbIdFieldBuilder();
getPagesFieldBuilder();
getFailuresFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (ebIdBuilder_ == null) {
ebId_ = org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance();
} else {
ebIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
taskId_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
attemptId_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
partId_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
host_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
volume_ = 0L;
bitField0_ = (bitField0_ & ~0x00000020);
if (pagesBuilder_ == null) {
pages_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
pagesBuilder_.clear();
}
if (failuresBuilder_ == null) {
failures_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
} else {
failuresBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_IntermediateEntryProto_descriptor;
}
public org.apache.tajo.ResourceProtos.IntermediateEntryProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.IntermediateEntryProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.IntermediateEntryProto build() {
org.apache.tajo.ResourceProtos.IntermediateEntryProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.IntermediateEntryProto buildPartial() {
org.apache.tajo.ResourceProtos.IntermediateEntryProto result = new org.apache.tajo.ResourceProtos.IntermediateEntryProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (ebIdBuilder_ == null) {
result.ebId_ = ebId_;
} else {
result.ebId_ = ebIdBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.taskId_ = taskId_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.attemptId_ = attemptId_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.partId_ = partId_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.host_ = host_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.volume_ = volume_;
if (pagesBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040)) {
pages_ = java.util.Collections.unmodifiableList(pages_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.pages_ = pages_;
} else {
result.pages_ = pagesBuilder_.build();
}
if (failuresBuilder_ == null) {
if (((bitField0_ & 0x00000080) == 0x00000080)) {
failures_ = java.util.Collections.unmodifiableList(failures_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.failures_ = failures_;
} else {
result.failures_ = failuresBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.IntermediateEntryProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.IntermediateEntryProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.IntermediateEntryProto other) {
if (other == org.apache.tajo.ResourceProtos.IntermediateEntryProto.getDefaultInstance()) return this;
if (other.hasEbId()) {
mergeEbId(other.getEbId());
}
if (other.hasTaskId()) {
setTaskId(other.getTaskId());
}
if (other.hasAttemptId()) {
setAttemptId(other.getAttemptId());
}
if (other.hasPartId()) {
setPartId(other.getPartId());
}
if (other.hasHost()) {
bitField0_ |= 0x00000010;
host_ = other.host_;
onChanged();
}
if (other.hasVolume()) {
setVolume(other.getVolume());
}
if (pagesBuilder_ == null) {
if (!other.pages_.isEmpty()) {
if (pages_.isEmpty()) {
pages_ = other.pages_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensurePagesIsMutable();
pages_.addAll(other.pages_);
}
onChanged();
}
} else {
if (!other.pages_.isEmpty()) {
if (pagesBuilder_.isEmpty()) {
pagesBuilder_.dispose();
pagesBuilder_ = null;
pages_ = other.pages_;
bitField0_ = (bitField0_ & ~0x00000040);
pagesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getPagesFieldBuilder() : null;
} else {
pagesBuilder_.addAllMessages(other.pages_);
}
}
}
if (failuresBuilder_ == null) {
if (!other.failures_.isEmpty()) {
if (failures_.isEmpty()) {
failures_ = other.failures_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureFailuresIsMutable();
failures_.addAll(other.failures_);
}
onChanged();
}
} else {
if (!other.failures_.isEmpty()) {
if (failuresBuilder_.isEmpty()) {
failuresBuilder_.dispose();
failuresBuilder_ = null;
failures_ = other.failures_;
bitField0_ = (bitField0_ & ~0x00000080);
failuresBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getFailuresFieldBuilder() : null;
} else {
failuresBuilder_.addAllMessages(other.failures_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasEbId()) {
return false;
}
if (!hasTaskId()) {
return false;
}
if (!hasAttemptId()) {
return false;
}
if (!hasPartId()) {
return false;
}
if (!hasHost()) {
return false;
}
if (!hasVolume()) {
return false;
}
if (!getEbId().isInitialized()) {
return false;
}
for (int i = 0; i < getPagesCount(); i++) {
if (!getPages(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getFailuresCount(); i++) {
if (!getFailures(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.IntermediateEntryProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.IntermediateEntryProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .ExecutionBlockIdProto ebId = 1;
private org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto ebId_ = org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder> ebIdBuilder_;
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public boolean hasEbId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto getEbId() {
if (ebIdBuilder_ == null) {
return ebId_;
} else {
return ebIdBuilder_.getMessage();
}
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public Builder setEbId(org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto value) {
if (ebIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ebId_ = value;
onChanged();
} else {
ebIdBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public Builder setEbId(
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder builderForValue) {
if (ebIdBuilder_ == null) {
ebId_ = builderForValue.build();
onChanged();
} else {
ebIdBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public Builder mergeEbId(org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto value) {
if (ebIdBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
ebId_ != org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance()) {
ebId_ =
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.newBuilder(ebId_).mergeFrom(value).buildPartial();
} else {
ebId_ = value;
}
onChanged();
} else {
ebIdBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public Builder clearEbId() {
if (ebIdBuilder_ == null) {
ebId_ = org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance();
onChanged();
} else {
ebIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder getEbIdBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getEbIdFieldBuilder().getBuilder();
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder getEbIdOrBuilder() {
if (ebIdBuilder_ != null) {
return ebIdBuilder_.getMessageOrBuilder();
} else {
return ebId_;
}
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder>
getEbIdFieldBuilder() {
if (ebIdBuilder_ == null) {
ebIdBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder>(
ebId_,
getParentForChildren(),
isClean());
ebId_ = null;
}
return ebIdBuilder_;
}
// required int32 taskId = 2;
private int taskId_ ;
/**
* required int32 taskId = 2;
*/
public boolean hasTaskId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 taskId = 2;
*/
public int getTaskId() {
return taskId_;
}
/**
* required int32 taskId = 2;
*/
public Builder setTaskId(int value) {
bitField0_ |= 0x00000002;
taskId_ = value;
onChanged();
return this;
}
/**
* required int32 taskId = 2;
*/
public Builder clearTaskId() {
bitField0_ = (bitField0_ & ~0x00000002);
taskId_ = 0;
onChanged();
return this;
}
// required int32 attemptId = 3;
private int attemptId_ ;
/**
* required int32 attemptId = 3;
*/
public boolean hasAttemptId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required int32 attemptId = 3;
*/
public int getAttemptId() {
return attemptId_;
}
/**
* required int32 attemptId = 3;
*/
public Builder setAttemptId(int value) {
bitField0_ |= 0x00000004;
attemptId_ = value;
onChanged();
return this;
}
/**
* required int32 attemptId = 3;
*/
public Builder clearAttemptId() {
bitField0_ = (bitField0_ & ~0x00000004);
attemptId_ = 0;
onChanged();
return this;
}
// required int32 partId = 4;
private int partId_ ;
/**
* required int32 partId = 4;
*/
public boolean hasPartId() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required int32 partId = 4;
*/
public int getPartId() {
return partId_;
}
/**
* required int32 partId = 4;
*/
public Builder setPartId(int value) {
bitField0_ |= 0x00000008;
partId_ = value;
onChanged();
return this;
}
/**
* required int32 partId = 4;
*/
public Builder clearPartId() {
bitField0_ = (bitField0_ & ~0x00000008);
partId_ = 0;
onChanged();
return this;
}
// required string host = 5;
private java.lang.Object host_ = "";
/**
* required string host = 5;
*/
public boolean hasHost() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required string host = 5;
*/
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
host_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string host = 5;
*/
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string host = 5;
*/
public Builder setHost(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
host_ = value;
onChanged();
return this;
}
/**
* required string host = 5;
*/
public Builder clearHost() {
bitField0_ = (bitField0_ & ~0x00000010);
host_ = getDefaultInstance().getHost();
onChanged();
return this;
}
/**
* required string host = 5;
*/
public Builder setHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
host_ = value;
onChanged();
return this;
}
// required int64 volume = 6;
private long volume_ ;
/**
* required int64 volume = 6;
*/
public boolean hasVolume() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* required int64 volume = 6;
*/
public long getVolume() {
return volume_;
}
/**
* required int64 volume = 6;
*/
public Builder setVolume(long value) {
bitField0_ |= 0x00000020;
volume_ = value;
onChanged();
return this;
}
/**
* required int64 volume = 6;
*/
public Builder clearVolume() {
bitField0_ = (bitField0_ & ~0x00000020);
volume_ = 0L;
onChanged();
return this;
}
// repeated .IntermediateEntryProto.PageProto pages = 7;
private java.util.List pages_ =
java.util.Collections.emptyList();
private void ensurePagesIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
pages_ = new java.util.ArrayList(pages_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto, org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.Builder, org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProtoOrBuilder> pagesBuilder_;
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public java.util.List getPagesList() {
if (pagesBuilder_ == null) {
return java.util.Collections.unmodifiableList(pages_);
} else {
return pagesBuilder_.getMessageList();
}
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public int getPagesCount() {
if (pagesBuilder_ == null) {
return pages_.size();
} else {
return pagesBuilder_.getCount();
}
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto getPages(int index) {
if (pagesBuilder_ == null) {
return pages_.get(index);
} else {
return pagesBuilder_.getMessage(index);
}
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public Builder setPages(
int index, org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto value) {
if (pagesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePagesIsMutable();
pages_.set(index, value);
onChanged();
} else {
pagesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public Builder setPages(
int index, org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.Builder builderForValue) {
if (pagesBuilder_ == null) {
ensurePagesIsMutable();
pages_.set(index, builderForValue.build());
onChanged();
} else {
pagesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public Builder addPages(org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto value) {
if (pagesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePagesIsMutable();
pages_.add(value);
onChanged();
} else {
pagesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public Builder addPages(
int index, org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto value) {
if (pagesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePagesIsMutable();
pages_.add(index, value);
onChanged();
} else {
pagesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public Builder addPages(
org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.Builder builderForValue) {
if (pagesBuilder_ == null) {
ensurePagesIsMutable();
pages_.add(builderForValue.build());
onChanged();
} else {
pagesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public Builder addPages(
int index, org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.Builder builderForValue) {
if (pagesBuilder_ == null) {
ensurePagesIsMutable();
pages_.add(index, builderForValue.build());
onChanged();
} else {
pagesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public Builder addAllPages(
java.lang.Iterable extends org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto> values) {
if (pagesBuilder_ == null) {
ensurePagesIsMutable();
super.addAll(values, pages_);
onChanged();
} else {
pagesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public Builder clearPages() {
if (pagesBuilder_ == null) {
pages_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
pagesBuilder_.clear();
}
return this;
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public Builder removePages(int index) {
if (pagesBuilder_ == null) {
ensurePagesIsMutable();
pages_.remove(index);
onChanged();
} else {
pagesBuilder_.remove(index);
}
return this;
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.Builder getPagesBuilder(
int index) {
return getPagesFieldBuilder().getBuilder(index);
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProtoOrBuilder getPagesOrBuilder(
int index) {
if (pagesBuilder_ == null) {
return pages_.get(index); } else {
return pagesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProtoOrBuilder>
getPagesOrBuilderList() {
if (pagesBuilder_ != null) {
return pagesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(pages_);
}
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.Builder addPagesBuilder() {
return getPagesFieldBuilder().addBuilder(
org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.getDefaultInstance());
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.Builder addPagesBuilder(
int index) {
return getPagesFieldBuilder().addBuilder(
index, org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.getDefaultInstance());
}
/**
* repeated .IntermediateEntryProto.PageProto pages = 7;
*/
public java.util.List
getPagesBuilderList() {
return getPagesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto, org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.Builder, org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProtoOrBuilder>
getPagesFieldBuilder() {
if (pagesBuilder_ == null) {
pagesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto, org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProto.Builder, org.apache.tajo.ResourceProtos.IntermediateEntryProto.PageProtoOrBuilder>(
pages_,
((bitField0_ & 0x00000040) == 0x00000040),
getParentForChildren(),
isClean());
pages_ = null;
}
return pagesBuilder_;
}
// repeated .FailureIntermediateProto failures = 8;
private java.util.List failures_ =
java.util.Collections.emptyList();
private void ensureFailuresIsMutable() {
if (!((bitField0_ & 0x00000080) == 0x00000080)) {
failures_ = new java.util.ArrayList(failures_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.FailureIntermediateProto, org.apache.tajo.ResourceProtos.FailureIntermediateProto.Builder, org.apache.tajo.ResourceProtos.FailureIntermediateProtoOrBuilder> failuresBuilder_;
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public java.util.List getFailuresList() {
if (failuresBuilder_ == null) {
return java.util.Collections.unmodifiableList(failures_);
} else {
return failuresBuilder_.getMessageList();
}
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public int getFailuresCount() {
if (failuresBuilder_ == null) {
return failures_.size();
} else {
return failuresBuilder_.getCount();
}
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public org.apache.tajo.ResourceProtos.FailureIntermediateProto getFailures(int index) {
if (failuresBuilder_ == null) {
return failures_.get(index);
} else {
return failuresBuilder_.getMessage(index);
}
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public Builder setFailures(
int index, org.apache.tajo.ResourceProtos.FailureIntermediateProto value) {
if (failuresBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFailuresIsMutable();
failures_.set(index, value);
onChanged();
} else {
failuresBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public Builder setFailures(
int index, org.apache.tajo.ResourceProtos.FailureIntermediateProto.Builder builderForValue) {
if (failuresBuilder_ == null) {
ensureFailuresIsMutable();
failures_.set(index, builderForValue.build());
onChanged();
} else {
failuresBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public Builder addFailures(org.apache.tajo.ResourceProtos.FailureIntermediateProto value) {
if (failuresBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFailuresIsMutable();
failures_.add(value);
onChanged();
} else {
failuresBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public Builder addFailures(
int index, org.apache.tajo.ResourceProtos.FailureIntermediateProto value) {
if (failuresBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFailuresIsMutable();
failures_.add(index, value);
onChanged();
} else {
failuresBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public Builder addFailures(
org.apache.tajo.ResourceProtos.FailureIntermediateProto.Builder builderForValue) {
if (failuresBuilder_ == null) {
ensureFailuresIsMutable();
failures_.add(builderForValue.build());
onChanged();
} else {
failuresBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public Builder addFailures(
int index, org.apache.tajo.ResourceProtos.FailureIntermediateProto.Builder builderForValue) {
if (failuresBuilder_ == null) {
ensureFailuresIsMutable();
failures_.add(index, builderForValue.build());
onChanged();
} else {
failuresBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public Builder addAllFailures(
java.lang.Iterable extends org.apache.tajo.ResourceProtos.FailureIntermediateProto> values) {
if (failuresBuilder_ == null) {
ensureFailuresIsMutable();
super.addAll(values, failures_);
onChanged();
} else {
failuresBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public Builder clearFailures() {
if (failuresBuilder_ == null) {
failures_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
failuresBuilder_.clear();
}
return this;
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public Builder removeFailures(int index) {
if (failuresBuilder_ == null) {
ensureFailuresIsMutable();
failures_.remove(index);
onChanged();
} else {
failuresBuilder_.remove(index);
}
return this;
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public org.apache.tajo.ResourceProtos.FailureIntermediateProto.Builder getFailuresBuilder(
int index) {
return getFailuresFieldBuilder().getBuilder(index);
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public org.apache.tajo.ResourceProtos.FailureIntermediateProtoOrBuilder getFailuresOrBuilder(
int index) {
if (failuresBuilder_ == null) {
return failures_.get(index); } else {
return failuresBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.FailureIntermediateProtoOrBuilder>
getFailuresOrBuilderList() {
if (failuresBuilder_ != null) {
return failuresBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(failures_);
}
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public org.apache.tajo.ResourceProtos.FailureIntermediateProto.Builder addFailuresBuilder() {
return getFailuresFieldBuilder().addBuilder(
org.apache.tajo.ResourceProtos.FailureIntermediateProto.getDefaultInstance());
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public org.apache.tajo.ResourceProtos.FailureIntermediateProto.Builder addFailuresBuilder(
int index) {
return getFailuresFieldBuilder().addBuilder(
index, org.apache.tajo.ResourceProtos.FailureIntermediateProto.getDefaultInstance());
}
/**
* repeated .FailureIntermediateProto failures = 8;
*/
public java.util.List
getFailuresBuilderList() {
return getFailuresFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.FailureIntermediateProto, org.apache.tajo.ResourceProtos.FailureIntermediateProto.Builder, org.apache.tajo.ResourceProtos.FailureIntermediateProtoOrBuilder>
getFailuresFieldBuilder() {
if (failuresBuilder_ == null) {
failuresBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.FailureIntermediateProto, org.apache.tajo.ResourceProtos.FailureIntermediateProto.Builder, org.apache.tajo.ResourceProtos.FailureIntermediateProtoOrBuilder>(
failures_,
((bitField0_ & 0x00000080) == 0x00000080),
getParentForChildren(),
isClean());
failures_ = null;
}
return failuresBuilder_;
}
// @@protoc_insertion_point(builder_scope:IntermediateEntryProto)
}
static {
defaultInstance = new IntermediateEntryProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:IntermediateEntryProto)
}
public interface ExecutionBlockReportOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required .ExecutionBlockIdProto ebId = 1;
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
boolean hasEbId();
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto getEbId();
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder getEbIdOrBuilder();
// required bool reportSuccess = 2;
/**
* required bool reportSuccess = 2;
*/
boolean hasReportSuccess();
/**
* required bool reportSuccess = 2;
*/
boolean getReportSuccess();
// optional string reportErrorMessage = 3;
/**
* optional string reportErrorMessage = 3;
*/
boolean hasReportErrorMessage();
/**
* optional string reportErrorMessage = 3;
*/
java.lang.String getReportErrorMessage();
/**
* optional string reportErrorMessage = 3;
*/
com.google.protobuf.ByteString
getReportErrorMessageBytes();
// required int32 succeededTasks = 4;
/**
* required int32 succeededTasks = 4;
*/
boolean hasSucceededTasks();
/**
* required int32 succeededTasks = 4;
*/
int getSucceededTasks();
// repeated .IntermediateEntryProto intermediateEntries = 5;
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
java.util.List
getIntermediateEntriesList();
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
org.apache.tajo.ResourceProtos.IntermediateEntryProto getIntermediateEntries(int index);
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
int getIntermediateEntriesCount();
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
java.util.List extends org.apache.tajo.ResourceProtos.IntermediateEntryProtoOrBuilder>
getIntermediateEntriesOrBuilderList();
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
org.apache.tajo.ResourceProtos.IntermediateEntryProtoOrBuilder getIntermediateEntriesOrBuilder(
int index);
}
/**
* Protobuf type {@code ExecutionBlockReport}
*/
public static final class ExecutionBlockReport extends
com.google.protobuf.GeneratedMessage
implements ExecutionBlockReportOrBuilder {
// Use ExecutionBlockReport.newBuilder() to construct.
private ExecutionBlockReport(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ExecutionBlockReport(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ExecutionBlockReport defaultInstance;
public static ExecutionBlockReport getDefaultInstance() {
return defaultInstance;
}
public ExecutionBlockReport getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ExecutionBlockReport(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = ebId_.toBuilder();
}
ebId_ = input.readMessage(org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(ebId_);
ebId_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 16: {
bitField0_ |= 0x00000002;
reportSuccess_ = input.readBool();
break;
}
case 26: {
bitField0_ |= 0x00000004;
reportErrorMessage_ = input.readBytes();
break;
}
case 32: {
bitField0_ |= 0x00000008;
succeededTasks_ = input.readInt32();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
intermediateEntries_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
intermediateEntries_.add(input.readMessage(org.apache.tajo.ResourceProtos.IntermediateEntryProto.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
intermediateEntries_ = java.util.Collections.unmodifiableList(intermediateEntries_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_ExecutionBlockReport_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_ExecutionBlockReport_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.ExecutionBlockReport.class, org.apache.tajo.ResourceProtos.ExecutionBlockReport.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ExecutionBlockReport parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ExecutionBlockReport(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .ExecutionBlockIdProto ebId = 1;
public static final int EBID_FIELD_NUMBER = 1;
private org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto ebId_;
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public boolean hasEbId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto getEbId() {
return ebId_;
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder getEbIdOrBuilder() {
return ebId_;
}
// required bool reportSuccess = 2;
public static final int REPORTSUCCESS_FIELD_NUMBER = 2;
private boolean reportSuccess_;
/**
* required bool reportSuccess = 2;
*/
public boolean hasReportSuccess() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bool reportSuccess = 2;
*/
public boolean getReportSuccess() {
return reportSuccess_;
}
// optional string reportErrorMessage = 3;
public static final int REPORTERRORMESSAGE_FIELD_NUMBER = 3;
private java.lang.Object reportErrorMessage_;
/**
* optional string reportErrorMessage = 3;
*/
public boolean hasReportErrorMessage() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string reportErrorMessage = 3;
*/
public java.lang.String getReportErrorMessage() {
java.lang.Object ref = reportErrorMessage_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
reportErrorMessage_ = s;
}
return s;
}
}
/**
* optional string reportErrorMessage = 3;
*/
public com.google.protobuf.ByteString
getReportErrorMessageBytes() {
java.lang.Object ref = reportErrorMessage_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reportErrorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required int32 succeededTasks = 4;
public static final int SUCCEEDEDTASKS_FIELD_NUMBER = 4;
private int succeededTasks_;
/**
* required int32 succeededTasks = 4;
*/
public boolean hasSucceededTasks() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required int32 succeededTasks = 4;
*/
public int getSucceededTasks() {
return succeededTasks_;
}
// repeated .IntermediateEntryProto intermediateEntries = 5;
public static final int INTERMEDIATEENTRIES_FIELD_NUMBER = 5;
private java.util.List intermediateEntries_;
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public java.util.List getIntermediateEntriesList() {
return intermediateEntries_;
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.IntermediateEntryProtoOrBuilder>
getIntermediateEntriesOrBuilderList() {
return intermediateEntries_;
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public int getIntermediateEntriesCount() {
return intermediateEntries_.size();
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProto getIntermediateEntries(int index) {
return intermediateEntries_.get(index);
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProtoOrBuilder getIntermediateEntriesOrBuilder(
int index) {
return intermediateEntries_.get(index);
}
private void initFields() {
ebId_ = org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance();
reportSuccess_ = false;
reportErrorMessage_ = "";
succeededTasks_ = 0;
intermediateEntries_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasEbId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasReportSuccess()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasSucceededTasks()) {
memoizedIsInitialized = 0;
return false;
}
if (!getEbId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getIntermediateEntriesCount(); i++) {
if (!getIntermediateEntries(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, ebId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBool(2, reportSuccess_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getReportErrorMessageBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt32(4, succeededTasks_);
}
for (int i = 0; i < intermediateEntries_.size(); i++) {
output.writeMessage(5, intermediateEntries_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, ebId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, reportSuccess_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getReportErrorMessageBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, succeededTasks_);
}
for (int i = 0; i < intermediateEntries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, intermediateEntries_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.ExecutionBlockReport)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.ExecutionBlockReport other = (org.apache.tajo.ResourceProtos.ExecutionBlockReport) obj;
boolean result = true;
result = result && (hasEbId() == other.hasEbId());
if (hasEbId()) {
result = result && getEbId()
.equals(other.getEbId());
}
result = result && (hasReportSuccess() == other.hasReportSuccess());
if (hasReportSuccess()) {
result = result && (getReportSuccess()
== other.getReportSuccess());
}
result = result && (hasReportErrorMessage() == other.hasReportErrorMessage());
if (hasReportErrorMessage()) {
result = result && getReportErrorMessage()
.equals(other.getReportErrorMessage());
}
result = result && (hasSucceededTasks() == other.hasSucceededTasks());
if (hasSucceededTasks()) {
result = result && (getSucceededTasks()
== other.getSucceededTasks());
}
result = result && getIntermediateEntriesList()
.equals(other.getIntermediateEntriesList());
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasEbId()) {
hash = (37 * hash) + EBID_FIELD_NUMBER;
hash = (53 * hash) + getEbId().hashCode();
}
if (hasReportSuccess()) {
hash = (37 * hash) + REPORTSUCCESS_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getReportSuccess());
}
if (hasReportErrorMessage()) {
hash = (37 * hash) + REPORTERRORMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getReportErrorMessage().hashCode();
}
if (hasSucceededTasks()) {
hash = (37 * hash) + SUCCEEDEDTASKS_FIELD_NUMBER;
hash = (53 * hash) + getSucceededTasks();
}
if (getIntermediateEntriesCount() > 0) {
hash = (37 * hash) + INTERMEDIATEENTRIES_FIELD_NUMBER;
hash = (53 * hash) + getIntermediateEntriesList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockReport parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockReport parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockReport parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockReport parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockReport parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockReport parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockReport parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockReport parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockReport parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.ExecutionBlockReport parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.ExecutionBlockReport prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ExecutionBlockReport}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.ExecutionBlockReportOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_ExecutionBlockReport_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_ExecutionBlockReport_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.ExecutionBlockReport.class, org.apache.tajo.ResourceProtos.ExecutionBlockReport.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.ExecutionBlockReport.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getEbIdFieldBuilder();
getIntermediateEntriesFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (ebIdBuilder_ == null) {
ebId_ = org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance();
} else {
ebIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
reportSuccess_ = false;
bitField0_ = (bitField0_ & ~0x00000002);
reportErrorMessage_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
succeededTasks_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
if (intermediateEntriesBuilder_ == null) {
intermediateEntries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
intermediateEntriesBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_ExecutionBlockReport_descriptor;
}
public org.apache.tajo.ResourceProtos.ExecutionBlockReport getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.ExecutionBlockReport.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.ExecutionBlockReport build() {
org.apache.tajo.ResourceProtos.ExecutionBlockReport result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.ExecutionBlockReport buildPartial() {
org.apache.tajo.ResourceProtos.ExecutionBlockReport result = new org.apache.tajo.ResourceProtos.ExecutionBlockReport(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (ebIdBuilder_ == null) {
result.ebId_ = ebId_;
} else {
result.ebId_ = ebIdBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.reportSuccess_ = reportSuccess_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.reportErrorMessage_ = reportErrorMessage_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.succeededTasks_ = succeededTasks_;
if (intermediateEntriesBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010)) {
intermediateEntries_ = java.util.Collections.unmodifiableList(intermediateEntries_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.intermediateEntries_ = intermediateEntries_;
} else {
result.intermediateEntries_ = intermediateEntriesBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.ExecutionBlockReport) {
return mergeFrom((org.apache.tajo.ResourceProtos.ExecutionBlockReport)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.ExecutionBlockReport other) {
if (other == org.apache.tajo.ResourceProtos.ExecutionBlockReport.getDefaultInstance()) return this;
if (other.hasEbId()) {
mergeEbId(other.getEbId());
}
if (other.hasReportSuccess()) {
setReportSuccess(other.getReportSuccess());
}
if (other.hasReportErrorMessage()) {
bitField0_ |= 0x00000004;
reportErrorMessage_ = other.reportErrorMessage_;
onChanged();
}
if (other.hasSucceededTasks()) {
setSucceededTasks(other.getSucceededTasks());
}
if (intermediateEntriesBuilder_ == null) {
if (!other.intermediateEntries_.isEmpty()) {
if (intermediateEntries_.isEmpty()) {
intermediateEntries_ = other.intermediateEntries_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureIntermediateEntriesIsMutable();
intermediateEntries_.addAll(other.intermediateEntries_);
}
onChanged();
}
} else {
if (!other.intermediateEntries_.isEmpty()) {
if (intermediateEntriesBuilder_.isEmpty()) {
intermediateEntriesBuilder_.dispose();
intermediateEntriesBuilder_ = null;
intermediateEntries_ = other.intermediateEntries_;
bitField0_ = (bitField0_ & ~0x00000010);
intermediateEntriesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getIntermediateEntriesFieldBuilder() : null;
} else {
intermediateEntriesBuilder_.addAllMessages(other.intermediateEntries_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasEbId()) {
return false;
}
if (!hasReportSuccess()) {
return false;
}
if (!hasSucceededTasks()) {
return false;
}
if (!getEbId().isInitialized()) {
return false;
}
for (int i = 0; i < getIntermediateEntriesCount(); i++) {
if (!getIntermediateEntries(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.ExecutionBlockReport parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.ExecutionBlockReport) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .ExecutionBlockIdProto ebId = 1;
private org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto ebId_ = org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder> ebIdBuilder_;
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public boolean hasEbId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto getEbId() {
if (ebIdBuilder_ == null) {
return ebId_;
} else {
return ebIdBuilder_.getMessage();
}
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public Builder setEbId(org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto value) {
if (ebIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ebId_ = value;
onChanged();
} else {
ebIdBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public Builder setEbId(
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder builderForValue) {
if (ebIdBuilder_ == null) {
ebId_ = builderForValue.build();
onChanged();
} else {
ebIdBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public Builder mergeEbId(org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto value) {
if (ebIdBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
ebId_ != org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance()) {
ebId_ =
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.newBuilder(ebId_).mergeFrom(value).buildPartial();
} else {
ebId_ = value;
}
onChanged();
} else {
ebIdBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public Builder clearEbId() {
if (ebIdBuilder_ == null) {
ebId_ = org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.getDefaultInstance();
onChanged();
} else {
ebIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder getEbIdBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getEbIdFieldBuilder().getBuilder();
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
public org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder getEbIdOrBuilder() {
if (ebIdBuilder_ != null) {
return ebIdBuilder_.getMessageOrBuilder();
} else {
return ebId_;
}
}
/**
* required .ExecutionBlockIdProto ebId = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder>
getEbIdFieldBuilder() {
if (ebIdBuilder_ == null) {
ebIdBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProto.Builder, org.apache.tajo.TajoIdProtos.ExecutionBlockIdProtoOrBuilder>(
ebId_,
getParentForChildren(),
isClean());
ebId_ = null;
}
return ebIdBuilder_;
}
// required bool reportSuccess = 2;
private boolean reportSuccess_ ;
/**
* required bool reportSuccess = 2;
*/
public boolean hasReportSuccess() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bool reportSuccess = 2;
*/
public boolean getReportSuccess() {
return reportSuccess_;
}
/**
* required bool reportSuccess = 2;
*/
public Builder setReportSuccess(boolean value) {
bitField0_ |= 0x00000002;
reportSuccess_ = value;
onChanged();
return this;
}
/**
* required bool reportSuccess = 2;
*/
public Builder clearReportSuccess() {
bitField0_ = (bitField0_ & ~0x00000002);
reportSuccess_ = false;
onChanged();
return this;
}
// optional string reportErrorMessage = 3;
private java.lang.Object reportErrorMessage_ = "";
/**
* optional string reportErrorMessage = 3;
*/
public boolean hasReportErrorMessage() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string reportErrorMessage = 3;
*/
public java.lang.String getReportErrorMessage() {
java.lang.Object ref = reportErrorMessage_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
reportErrorMessage_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string reportErrorMessage = 3;
*/
public com.google.protobuf.ByteString
getReportErrorMessageBytes() {
java.lang.Object ref = reportErrorMessage_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reportErrorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string reportErrorMessage = 3;
*/
public Builder setReportErrorMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
reportErrorMessage_ = value;
onChanged();
return this;
}
/**
* optional string reportErrorMessage = 3;
*/
public Builder clearReportErrorMessage() {
bitField0_ = (bitField0_ & ~0x00000004);
reportErrorMessage_ = getDefaultInstance().getReportErrorMessage();
onChanged();
return this;
}
/**
* optional string reportErrorMessage = 3;
*/
public Builder setReportErrorMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
reportErrorMessage_ = value;
onChanged();
return this;
}
// required int32 succeededTasks = 4;
private int succeededTasks_ ;
/**
* required int32 succeededTasks = 4;
*/
public boolean hasSucceededTasks() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required int32 succeededTasks = 4;
*/
public int getSucceededTasks() {
return succeededTasks_;
}
/**
* required int32 succeededTasks = 4;
*/
public Builder setSucceededTasks(int value) {
bitField0_ |= 0x00000008;
succeededTasks_ = value;
onChanged();
return this;
}
/**
* required int32 succeededTasks = 4;
*/
public Builder clearSucceededTasks() {
bitField0_ = (bitField0_ & ~0x00000008);
succeededTasks_ = 0;
onChanged();
return this;
}
// repeated .IntermediateEntryProto intermediateEntries = 5;
private java.util.List intermediateEntries_ =
java.util.Collections.emptyList();
private void ensureIntermediateEntriesIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
intermediateEntries_ = new java.util.ArrayList(intermediateEntries_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.IntermediateEntryProto, org.apache.tajo.ResourceProtos.IntermediateEntryProto.Builder, org.apache.tajo.ResourceProtos.IntermediateEntryProtoOrBuilder> intermediateEntriesBuilder_;
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public java.util.List getIntermediateEntriesList() {
if (intermediateEntriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(intermediateEntries_);
} else {
return intermediateEntriesBuilder_.getMessageList();
}
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public int getIntermediateEntriesCount() {
if (intermediateEntriesBuilder_ == null) {
return intermediateEntries_.size();
} else {
return intermediateEntriesBuilder_.getCount();
}
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProto getIntermediateEntries(int index) {
if (intermediateEntriesBuilder_ == null) {
return intermediateEntries_.get(index);
} else {
return intermediateEntriesBuilder_.getMessage(index);
}
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public Builder setIntermediateEntries(
int index, org.apache.tajo.ResourceProtos.IntermediateEntryProto value) {
if (intermediateEntriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIntermediateEntriesIsMutable();
intermediateEntries_.set(index, value);
onChanged();
} else {
intermediateEntriesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public Builder setIntermediateEntries(
int index, org.apache.tajo.ResourceProtos.IntermediateEntryProto.Builder builderForValue) {
if (intermediateEntriesBuilder_ == null) {
ensureIntermediateEntriesIsMutable();
intermediateEntries_.set(index, builderForValue.build());
onChanged();
} else {
intermediateEntriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public Builder addIntermediateEntries(org.apache.tajo.ResourceProtos.IntermediateEntryProto value) {
if (intermediateEntriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIntermediateEntriesIsMutable();
intermediateEntries_.add(value);
onChanged();
} else {
intermediateEntriesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public Builder addIntermediateEntries(
int index, org.apache.tajo.ResourceProtos.IntermediateEntryProto value) {
if (intermediateEntriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIntermediateEntriesIsMutable();
intermediateEntries_.add(index, value);
onChanged();
} else {
intermediateEntriesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public Builder addIntermediateEntries(
org.apache.tajo.ResourceProtos.IntermediateEntryProto.Builder builderForValue) {
if (intermediateEntriesBuilder_ == null) {
ensureIntermediateEntriesIsMutable();
intermediateEntries_.add(builderForValue.build());
onChanged();
} else {
intermediateEntriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public Builder addIntermediateEntries(
int index, org.apache.tajo.ResourceProtos.IntermediateEntryProto.Builder builderForValue) {
if (intermediateEntriesBuilder_ == null) {
ensureIntermediateEntriesIsMutable();
intermediateEntries_.add(index, builderForValue.build());
onChanged();
} else {
intermediateEntriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public Builder addAllIntermediateEntries(
java.lang.Iterable extends org.apache.tajo.ResourceProtos.IntermediateEntryProto> values) {
if (intermediateEntriesBuilder_ == null) {
ensureIntermediateEntriesIsMutable();
super.addAll(values, intermediateEntries_);
onChanged();
} else {
intermediateEntriesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public Builder clearIntermediateEntries() {
if (intermediateEntriesBuilder_ == null) {
intermediateEntries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
intermediateEntriesBuilder_.clear();
}
return this;
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public Builder removeIntermediateEntries(int index) {
if (intermediateEntriesBuilder_ == null) {
ensureIntermediateEntriesIsMutable();
intermediateEntries_.remove(index);
onChanged();
} else {
intermediateEntriesBuilder_.remove(index);
}
return this;
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProto.Builder getIntermediateEntriesBuilder(
int index) {
return getIntermediateEntriesFieldBuilder().getBuilder(index);
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProtoOrBuilder getIntermediateEntriesOrBuilder(
int index) {
if (intermediateEntriesBuilder_ == null) {
return intermediateEntries_.get(index); } else {
return intermediateEntriesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.IntermediateEntryProtoOrBuilder>
getIntermediateEntriesOrBuilderList() {
if (intermediateEntriesBuilder_ != null) {
return intermediateEntriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(intermediateEntries_);
}
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProto.Builder addIntermediateEntriesBuilder() {
return getIntermediateEntriesFieldBuilder().addBuilder(
org.apache.tajo.ResourceProtos.IntermediateEntryProto.getDefaultInstance());
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public org.apache.tajo.ResourceProtos.IntermediateEntryProto.Builder addIntermediateEntriesBuilder(
int index) {
return getIntermediateEntriesFieldBuilder().addBuilder(
index, org.apache.tajo.ResourceProtos.IntermediateEntryProto.getDefaultInstance());
}
/**
* repeated .IntermediateEntryProto intermediateEntries = 5;
*/
public java.util.List
getIntermediateEntriesBuilderList() {
return getIntermediateEntriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.IntermediateEntryProto, org.apache.tajo.ResourceProtos.IntermediateEntryProto.Builder, org.apache.tajo.ResourceProtos.IntermediateEntryProtoOrBuilder>
getIntermediateEntriesFieldBuilder() {
if (intermediateEntriesBuilder_ == null) {
intermediateEntriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.IntermediateEntryProto, org.apache.tajo.ResourceProtos.IntermediateEntryProto.Builder, org.apache.tajo.ResourceProtos.IntermediateEntryProtoOrBuilder>(
intermediateEntries_,
((bitField0_ & 0x00000010) == 0x00000010),
getParentForChildren(),
isClean());
intermediateEntries_ = null;
}
return intermediateEntriesBuilder_;
}
// @@protoc_insertion_point(builder_scope:ExecutionBlockReport)
}
static {
defaultInstance = new ExecutionBlockReport(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ExecutionBlockReport)
}
public interface TaskResponseProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string id = 1;
/**
* required string id = 1;
*/
boolean hasId();
/**
* required string id = 1;
*/
java.lang.String getId();
/**
* required string id = 1;
*/
com.google.protobuf.ByteString
getIdBytes();
// required .QueryState status = 2;
/**
* required .QueryState status = 2;
*/
boolean hasStatus();
/**
* required .QueryState status = 2;
*/
org.apache.tajo.TajoProtos.QueryState getStatus();
}
/**
* Protobuf type {@code TaskResponseProto}
*
*
* deprecated
*
*/
public static final class TaskResponseProto extends
com.google.protobuf.GeneratedMessage
implements TaskResponseProtoOrBuilder {
// Use TaskResponseProto.newBuilder() to construct.
private TaskResponseProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TaskResponseProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TaskResponseProto defaultInstance;
public static TaskResponseProto getDefaultInstance() {
return defaultInstance;
}
public TaskResponseProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TaskResponseProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
id_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
org.apache.tajo.TajoProtos.QueryState value = org.apache.tajo.TajoProtos.QueryState.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
status_ = value;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TaskResponseProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TaskResponseProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TaskResponseProto.class, org.apache.tajo.ResourceProtos.TaskResponseProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TaskResponseProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TaskResponseProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string id = 1;
public static final int ID_FIELD_NUMBER = 1;
private java.lang.Object id_;
/**
* required string id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
}
}
/**
* required string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required .QueryState status = 2;
public static final int STATUS_FIELD_NUMBER = 2;
private org.apache.tajo.TajoProtos.QueryState status_;
/**
* required .QueryState status = 2;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .QueryState status = 2;
*/
public org.apache.tajo.TajoProtos.QueryState getStatus() {
return status_;
}
private void initFields() {
id_ = "";
status_ = org.apache.tajo.TajoProtos.QueryState.QUERY_MASTER_INIT;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasStatus()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getIdBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, status_.getNumber());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getIdBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, status_.getNumber());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.TaskResponseProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.TaskResponseProto other = (org.apache.tajo.ResourceProtos.TaskResponseProto) obj;
boolean result = true;
result = result && (hasId() == other.hasId());
if (hasId()) {
result = result && getId()
.equals(other.getId());
}
result = result && (hasStatus() == other.hasStatus());
if (hasStatus()) {
result = result &&
(getStatus() == other.getStatus());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + hashEnum(getStatus());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.TaskResponseProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TaskResponseProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskResponseProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TaskResponseProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskResponseProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskResponseProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskResponseProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskResponseProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TaskResponseProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TaskResponseProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.TaskResponseProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TaskResponseProto}
*
*
* deprecated
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.TaskResponseProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TaskResponseProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TaskResponseProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TaskResponseProto.class, org.apache.tajo.ResourceProtos.TaskResponseProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.TaskResponseProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
id_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
status_ = org.apache.tajo.TajoProtos.QueryState.QUERY_MASTER_INIT;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_TaskResponseProto_descriptor;
}
public org.apache.tajo.ResourceProtos.TaskResponseProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.TaskResponseProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.TaskResponseProto build() {
org.apache.tajo.ResourceProtos.TaskResponseProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.TaskResponseProto buildPartial() {
org.apache.tajo.ResourceProtos.TaskResponseProto result = new org.apache.tajo.ResourceProtos.TaskResponseProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.status_ = status_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.TaskResponseProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.TaskResponseProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.TaskResponseProto other) {
if (other == org.apache.tajo.ResourceProtos.TaskResponseProto.getDefaultInstance()) return this;
if (other.hasId()) {
bitField0_ |= 0x00000001;
id_ = other.id_;
onChanged();
}
if (other.hasStatus()) {
setStatus(other.getStatus());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasId()) {
return false;
}
if (!hasStatus()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.TaskResponseProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.TaskResponseProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string id = 1;
private java.lang.Object id_ = "";
/**
* required string id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string id = 1;
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* required string id = 1;
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* required string id = 1;
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
// required .QueryState status = 2;
private org.apache.tajo.TajoProtos.QueryState status_ = org.apache.tajo.TajoProtos.QueryState.QUERY_MASTER_INIT;
/**
* required .QueryState status = 2;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .QueryState status = 2;
*/
public org.apache.tajo.TajoProtos.QueryState getStatus() {
return status_;
}
/**
* required .QueryState status = 2;
*/
public Builder setStatus(org.apache.tajo.TajoProtos.QueryState value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
status_ = value;
onChanged();
return this;
}
/**
* required .QueryState status = 2;
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000002);
status_ = org.apache.tajo.TajoProtos.QueryState.QUERY_MASTER_INIT;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:TaskResponseProto)
}
static {
defaultInstance = new TaskResponseProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TaskResponseProto)
}
public interface StatusReportProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int64 timestamp = 1;
/**
* required int64 timestamp = 1;
*/
boolean hasTimestamp();
/**
* required int64 timestamp = 1;
*/
long getTimestamp();
// required string serverName = 2;
/**
* required string serverName = 2;
*/
boolean hasServerName();
/**
* required string serverName = 2;
*/
java.lang.String getServerName();
/**
* required string serverName = 2;
*/
com.google.protobuf.ByteString
getServerNameBytes();
// repeated .TaskStatusProto status = 3;
/**
* repeated .TaskStatusProto status = 3;
*/
java.util.List
getStatusList();
/**
* repeated .TaskStatusProto status = 3;
*/
org.apache.tajo.ResourceProtos.TaskStatusProto getStatus(int index);
/**
* repeated .TaskStatusProto status = 3;
*/
int getStatusCount();
/**
* repeated .TaskStatusProto status = 3;
*/
java.util.List extends org.apache.tajo.ResourceProtos.TaskStatusProtoOrBuilder>
getStatusOrBuilderList();
/**
* repeated .TaskStatusProto status = 3;
*/
org.apache.tajo.ResourceProtos.TaskStatusProtoOrBuilder getStatusOrBuilder(
int index);
// repeated .TaskAttemptIdProto pings = 4;
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
java.util.List
getPingsList();
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getPings(int index);
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
int getPingsCount();
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
java.util.List extends org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>
getPingsOrBuilderList();
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getPingsOrBuilder(
int index);
}
/**
* Protobuf type {@code StatusReportProto}
*/
public static final class StatusReportProto extends
com.google.protobuf.GeneratedMessage
implements StatusReportProtoOrBuilder {
// Use StatusReportProto.newBuilder() to construct.
private StatusReportProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private StatusReportProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final StatusReportProto defaultInstance;
public static StatusReportProto getDefaultInstance() {
return defaultInstance;
}
public StatusReportProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StatusReportProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
timestamp_ = input.readInt64();
break;
}
case 18: {
bitField0_ |= 0x00000002;
serverName_ = input.readBytes();
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
status_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
status_.add(input.readMessage(org.apache.tajo.ResourceProtos.TaskStatusProto.PARSER, extensionRegistry));
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
pings_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
pings_.add(input.readMessage(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
status_ = java.util.Collections.unmodifiableList(status_);
}
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
pings_ = java.util.Collections.unmodifiableList(pings_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_StatusReportProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_StatusReportProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.StatusReportProto.class, org.apache.tajo.ResourceProtos.StatusReportProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public StatusReportProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StatusReportProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required int64 timestamp = 1;
public static final int TIMESTAMP_FIELD_NUMBER = 1;
private long timestamp_;
/**
* required int64 timestamp = 1;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 timestamp = 1;
*/
public long getTimestamp() {
return timestamp_;
}
// required string serverName = 2;
public static final int SERVERNAME_FIELD_NUMBER = 2;
private java.lang.Object serverName_;
/**
* required string serverName = 2;
*/
public boolean hasServerName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string serverName = 2;
*/
public java.lang.String getServerName() {
java.lang.Object ref = serverName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
serverName_ = s;
}
return s;
}
}
/**
* required string serverName = 2;
*/
public com.google.protobuf.ByteString
getServerNameBytes() {
java.lang.Object ref = serverName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serverName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// repeated .TaskStatusProto status = 3;
public static final int STATUS_FIELD_NUMBER = 3;
private java.util.List status_;
/**
* repeated .TaskStatusProto status = 3;
*/
public java.util.List getStatusList() {
return status_;
}
/**
* repeated .TaskStatusProto status = 3;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.TaskStatusProtoOrBuilder>
getStatusOrBuilderList() {
return status_;
}
/**
* repeated .TaskStatusProto status = 3;
*/
public int getStatusCount() {
return status_.size();
}
/**
* repeated .TaskStatusProto status = 3;
*/
public org.apache.tajo.ResourceProtos.TaskStatusProto getStatus(int index) {
return status_.get(index);
}
/**
* repeated .TaskStatusProto status = 3;
*/
public org.apache.tajo.ResourceProtos.TaskStatusProtoOrBuilder getStatusOrBuilder(
int index) {
return status_.get(index);
}
// repeated .TaskAttemptIdProto pings = 4;
public static final int PINGS_FIELD_NUMBER = 4;
private java.util.List pings_;
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public java.util.List getPingsList() {
return pings_;
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public java.util.List extends org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>
getPingsOrBuilderList() {
return pings_;
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public int getPingsCount() {
return pings_.size();
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getPings(int index) {
return pings_.get(index);
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getPingsOrBuilder(
int index) {
return pings_.get(index);
}
private void initFields() {
timestamp_ = 0L;
serverName_ = "";
status_ = java.util.Collections.emptyList();
pings_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasTimestamp()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasServerName()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getStatusCount(); i++) {
if (!getStatus(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getPingsCount(); i++) {
if (!getPings(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, timestamp_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getServerNameBytes());
}
for (int i = 0; i < status_.size(); i++) {
output.writeMessage(3, status_.get(i));
}
for (int i = 0; i < pings_.size(); i++) {
output.writeMessage(4, pings_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, timestamp_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getServerNameBytes());
}
for (int i = 0; i < status_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, status_.get(i));
}
for (int i = 0; i < pings_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, pings_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.StatusReportProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.StatusReportProto other = (org.apache.tajo.ResourceProtos.StatusReportProto) obj;
boolean result = true;
result = result && (hasTimestamp() == other.hasTimestamp());
if (hasTimestamp()) {
result = result && (getTimestamp()
== other.getTimestamp());
}
result = result && (hasServerName() == other.hasServerName());
if (hasServerName()) {
result = result && getServerName()
.equals(other.getServerName());
}
result = result && getStatusList()
.equals(other.getStatusList());
result = result && getPingsList()
.equals(other.getPingsList());
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasTimestamp()) {
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getTimestamp());
}
if (hasServerName()) {
hash = (37 * hash) + SERVERNAME_FIELD_NUMBER;
hash = (53 * hash) + getServerName().hashCode();
}
if (getStatusCount() > 0) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatusList().hashCode();
}
if (getPingsCount() > 0) {
hash = (37 * hash) + PINGS_FIELD_NUMBER;
hash = (53 * hash) + getPingsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.StatusReportProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.StatusReportProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.StatusReportProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.StatusReportProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.StatusReportProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.StatusReportProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.StatusReportProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.StatusReportProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.StatusReportProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.StatusReportProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.StatusReportProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code StatusReportProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.StatusReportProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_StatusReportProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_StatusReportProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.StatusReportProto.class, org.apache.tajo.ResourceProtos.StatusReportProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.StatusReportProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getStatusFieldBuilder();
getPingsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
timestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
serverName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
if (statusBuilder_ == null) {
status_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
statusBuilder_.clear();
}
if (pingsBuilder_ == null) {
pings_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
pingsBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_StatusReportProto_descriptor;
}
public org.apache.tajo.ResourceProtos.StatusReportProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.StatusReportProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.StatusReportProto build() {
org.apache.tajo.ResourceProtos.StatusReportProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.StatusReportProto buildPartial() {
org.apache.tajo.ResourceProtos.StatusReportProto result = new org.apache.tajo.ResourceProtos.StatusReportProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.timestamp_ = timestamp_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.serverName_ = serverName_;
if (statusBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
status_ = java.util.Collections.unmodifiableList(status_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
if (pingsBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008)) {
pings_ = java.util.Collections.unmodifiableList(pings_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.pings_ = pings_;
} else {
result.pings_ = pingsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.StatusReportProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.StatusReportProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.StatusReportProto other) {
if (other == org.apache.tajo.ResourceProtos.StatusReportProto.getDefaultInstance()) return this;
if (other.hasTimestamp()) {
setTimestamp(other.getTimestamp());
}
if (other.hasServerName()) {
bitField0_ |= 0x00000002;
serverName_ = other.serverName_;
onChanged();
}
if (statusBuilder_ == null) {
if (!other.status_.isEmpty()) {
if (status_.isEmpty()) {
status_ = other.status_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureStatusIsMutable();
status_.addAll(other.status_);
}
onChanged();
}
} else {
if (!other.status_.isEmpty()) {
if (statusBuilder_.isEmpty()) {
statusBuilder_.dispose();
statusBuilder_ = null;
status_ = other.status_;
bitField0_ = (bitField0_ & ~0x00000004);
statusBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getStatusFieldBuilder() : null;
} else {
statusBuilder_.addAllMessages(other.status_);
}
}
}
if (pingsBuilder_ == null) {
if (!other.pings_.isEmpty()) {
if (pings_.isEmpty()) {
pings_ = other.pings_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensurePingsIsMutable();
pings_.addAll(other.pings_);
}
onChanged();
}
} else {
if (!other.pings_.isEmpty()) {
if (pingsBuilder_.isEmpty()) {
pingsBuilder_.dispose();
pingsBuilder_ = null;
pings_ = other.pings_;
bitField0_ = (bitField0_ & ~0x00000008);
pingsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getPingsFieldBuilder() : null;
} else {
pingsBuilder_.addAllMessages(other.pings_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasTimestamp()) {
return false;
}
if (!hasServerName()) {
return false;
}
for (int i = 0; i < getStatusCount(); i++) {
if (!getStatus(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getPingsCount(); i++) {
if (!getPings(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.StatusReportProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.StatusReportProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int64 timestamp = 1;
private long timestamp_ ;
/**
* required int64 timestamp = 1;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 timestamp = 1;
*/
public long getTimestamp() {
return timestamp_;
}
/**
* required int64 timestamp = 1;
*/
public Builder setTimestamp(long value) {
bitField0_ |= 0x00000001;
timestamp_ = value;
onChanged();
return this;
}
/**
* required int64 timestamp = 1;
*/
public Builder clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00000001);
timestamp_ = 0L;
onChanged();
return this;
}
// required string serverName = 2;
private java.lang.Object serverName_ = "";
/**
* required string serverName = 2;
*/
public boolean hasServerName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string serverName = 2;
*/
public java.lang.String getServerName() {
java.lang.Object ref = serverName_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
serverName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string serverName = 2;
*/
public com.google.protobuf.ByteString
getServerNameBytes() {
java.lang.Object ref = serverName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serverName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string serverName = 2;
*/
public Builder setServerName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
serverName_ = value;
onChanged();
return this;
}
/**
* required string serverName = 2;
*/
public Builder clearServerName() {
bitField0_ = (bitField0_ & ~0x00000002);
serverName_ = getDefaultInstance().getServerName();
onChanged();
return this;
}
/**
* required string serverName = 2;
*/
public Builder setServerNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
serverName_ = value;
onChanged();
return this;
}
// repeated .TaskStatusProto status = 3;
private java.util.List status_ =
java.util.Collections.emptyList();
private void ensureStatusIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
status_ = new java.util.ArrayList(status_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.TaskStatusProto, org.apache.tajo.ResourceProtos.TaskStatusProto.Builder, org.apache.tajo.ResourceProtos.TaskStatusProtoOrBuilder> statusBuilder_;
/**
* repeated .TaskStatusProto status = 3;
*/
public java.util.List getStatusList() {
if (statusBuilder_ == null) {
return java.util.Collections.unmodifiableList(status_);
} else {
return statusBuilder_.getMessageList();
}
}
/**
* repeated .TaskStatusProto status = 3;
*/
public int getStatusCount() {
if (statusBuilder_ == null) {
return status_.size();
} else {
return statusBuilder_.getCount();
}
}
/**
* repeated .TaskStatusProto status = 3;
*/
public org.apache.tajo.ResourceProtos.TaskStatusProto getStatus(int index) {
if (statusBuilder_ == null) {
return status_.get(index);
} else {
return statusBuilder_.getMessage(index);
}
}
/**
* repeated .TaskStatusProto status = 3;
*/
public Builder setStatus(
int index, org.apache.tajo.ResourceProtos.TaskStatusProto value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStatusIsMutable();
status_.set(index, value);
onChanged();
} else {
statusBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TaskStatusProto status = 3;
*/
public Builder setStatus(
int index, org.apache.tajo.ResourceProtos.TaskStatusProto.Builder builderForValue) {
if (statusBuilder_ == null) {
ensureStatusIsMutable();
status_.set(index, builderForValue.build());
onChanged();
} else {
statusBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TaskStatusProto status = 3;
*/
public Builder addStatus(org.apache.tajo.ResourceProtos.TaskStatusProto value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStatusIsMutable();
status_.add(value);
onChanged();
} else {
statusBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TaskStatusProto status = 3;
*/
public Builder addStatus(
int index, org.apache.tajo.ResourceProtos.TaskStatusProto value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStatusIsMutable();
status_.add(index, value);
onChanged();
} else {
statusBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TaskStatusProto status = 3;
*/
public Builder addStatus(
org.apache.tajo.ResourceProtos.TaskStatusProto.Builder builderForValue) {
if (statusBuilder_ == null) {
ensureStatusIsMutable();
status_.add(builderForValue.build());
onChanged();
} else {
statusBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TaskStatusProto status = 3;
*/
public Builder addStatus(
int index, org.apache.tajo.ResourceProtos.TaskStatusProto.Builder builderForValue) {
if (statusBuilder_ == null) {
ensureStatusIsMutable();
status_.add(index, builderForValue.build());
onChanged();
} else {
statusBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TaskStatusProto status = 3;
*/
public Builder addAllStatus(
java.lang.Iterable extends org.apache.tajo.ResourceProtos.TaskStatusProto> values) {
if (statusBuilder_ == null) {
ensureStatusIsMutable();
super.addAll(values, status_);
onChanged();
} else {
statusBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TaskStatusProto status = 3;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
statusBuilder_.clear();
}
return this;
}
/**
* repeated .TaskStatusProto status = 3;
*/
public Builder removeStatus(int index) {
if (statusBuilder_ == null) {
ensureStatusIsMutable();
status_.remove(index);
onChanged();
} else {
statusBuilder_.remove(index);
}
return this;
}
/**
* repeated .TaskStatusProto status = 3;
*/
public org.apache.tajo.ResourceProtos.TaskStatusProto.Builder getStatusBuilder(
int index) {
return getStatusFieldBuilder().getBuilder(index);
}
/**
* repeated .TaskStatusProto status = 3;
*/
public org.apache.tajo.ResourceProtos.TaskStatusProtoOrBuilder getStatusOrBuilder(
int index) {
if (statusBuilder_ == null) {
return status_.get(index); } else {
return statusBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TaskStatusProto status = 3;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.TaskStatusProtoOrBuilder>
getStatusOrBuilderList() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(status_);
}
}
/**
* repeated .TaskStatusProto status = 3;
*/
public org.apache.tajo.ResourceProtos.TaskStatusProto.Builder addStatusBuilder() {
return getStatusFieldBuilder().addBuilder(
org.apache.tajo.ResourceProtos.TaskStatusProto.getDefaultInstance());
}
/**
* repeated .TaskStatusProto status = 3;
*/
public org.apache.tajo.ResourceProtos.TaskStatusProto.Builder addStatusBuilder(
int index) {
return getStatusFieldBuilder().addBuilder(
index, org.apache.tajo.ResourceProtos.TaskStatusProto.getDefaultInstance());
}
/**
* repeated .TaskStatusProto status = 3;
*/
public java.util.List
getStatusBuilderList() {
return getStatusFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.TaskStatusProto, org.apache.tajo.ResourceProtos.TaskStatusProto.Builder, org.apache.tajo.ResourceProtos.TaskStatusProtoOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.TaskStatusProto, org.apache.tajo.ResourceProtos.TaskStatusProto.Builder, org.apache.tajo.ResourceProtos.TaskStatusProtoOrBuilder>(
status_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
// repeated .TaskAttemptIdProto pings = 4;
private java.util.List pings_ =
java.util.Collections.emptyList();
private void ensurePingsIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
pings_ = new java.util.ArrayList(pings_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder> pingsBuilder_;
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public java.util.List getPingsList() {
if (pingsBuilder_ == null) {
return java.util.Collections.unmodifiableList(pings_);
} else {
return pingsBuilder_.getMessageList();
}
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public int getPingsCount() {
if (pingsBuilder_ == null) {
return pings_.size();
} else {
return pingsBuilder_.getCount();
}
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getPings(int index) {
if (pingsBuilder_ == null) {
return pings_.get(index);
} else {
return pingsBuilder_.getMessage(index);
}
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public Builder setPings(
int index, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto value) {
if (pingsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePingsIsMutable();
pings_.set(index, value);
onChanged();
} else {
pingsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public Builder setPings(
int index, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder builderForValue) {
if (pingsBuilder_ == null) {
ensurePingsIsMutable();
pings_.set(index, builderForValue.build());
onChanged();
} else {
pingsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public Builder addPings(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto value) {
if (pingsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePingsIsMutable();
pings_.add(value);
onChanged();
} else {
pingsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public Builder addPings(
int index, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto value) {
if (pingsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePingsIsMutable();
pings_.add(index, value);
onChanged();
} else {
pingsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public Builder addPings(
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder builderForValue) {
if (pingsBuilder_ == null) {
ensurePingsIsMutable();
pings_.add(builderForValue.build());
onChanged();
} else {
pingsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public Builder addPings(
int index, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder builderForValue) {
if (pingsBuilder_ == null) {
ensurePingsIsMutable();
pings_.add(index, builderForValue.build());
onChanged();
} else {
pingsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public Builder addAllPings(
java.lang.Iterable extends org.apache.tajo.TajoIdProtos.TaskAttemptIdProto> values) {
if (pingsBuilder_ == null) {
ensurePingsIsMutable();
super.addAll(values, pings_);
onChanged();
} else {
pingsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public Builder clearPings() {
if (pingsBuilder_ == null) {
pings_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
pingsBuilder_.clear();
}
return this;
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public Builder removePings(int index) {
if (pingsBuilder_ == null) {
ensurePingsIsMutable();
pings_.remove(index);
onChanged();
} else {
pingsBuilder_.remove(index);
}
return this;
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder getPingsBuilder(
int index) {
return getPingsFieldBuilder().getBuilder(index);
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getPingsOrBuilder(
int index) {
if (pingsBuilder_ == null) {
return pings_.get(index); } else {
return pingsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public java.util.List extends org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>
getPingsOrBuilderList() {
if (pingsBuilder_ != null) {
return pingsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(pings_);
}
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder addPingsBuilder() {
return getPingsFieldBuilder().addBuilder(
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance());
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder addPingsBuilder(
int index) {
return getPingsFieldBuilder().addBuilder(
index, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance());
}
/**
* repeated .TaskAttemptIdProto pings = 4;
*/
public java.util.List
getPingsBuilderList() {
return getPingsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>
getPingsFieldBuilder() {
if (pingsBuilder_ == null) {
pingsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>(
pings_,
((bitField0_ & 0x00000008) == 0x00000008),
getParentForChildren(),
isClean());
pings_ = null;
}
return pingsBuilder_;
}
// @@protoc_insertion_point(builder_scope:StatusReportProto)
}
static {
defaultInstance = new StatusReportProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:StatusReportProto)
}
public interface CommandRequestProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// repeated .Command command = 1;
/**
* repeated .Command command = 1;
*/
java.util.List
getCommandList();
/**
* repeated .Command command = 1;
*/
org.apache.tajo.ResourceProtos.Command getCommand(int index);
/**
* repeated .Command command = 1;
*/
int getCommandCount();
/**
* repeated .Command command = 1;
*/
java.util.List extends org.apache.tajo.ResourceProtos.CommandOrBuilder>
getCommandOrBuilderList();
/**
* repeated .Command command = 1;
*/
org.apache.tajo.ResourceProtos.CommandOrBuilder getCommandOrBuilder(
int index);
}
/**
* Protobuf type {@code CommandRequestProto}
*/
public static final class CommandRequestProto extends
com.google.protobuf.GeneratedMessage
implements CommandRequestProtoOrBuilder {
// Use CommandRequestProto.newBuilder() to construct.
private CommandRequestProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private CommandRequestProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final CommandRequestProto defaultInstance;
public static CommandRequestProto getDefaultInstance() {
return defaultInstance;
}
public CommandRequestProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommandRequestProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
command_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
command_.add(input.readMessage(org.apache.tajo.ResourceProtos.Command.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
command_ = java.util.Collections.unmodifiableList(command_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_CommandRequestProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_CommandRequestProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.CommandRequestProto.class, org.apache.tajo.ResourceProtos.CommandRequestProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public CommandRequestProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommandRequestProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
// repeated .Command command = 1;
public static final int COMMAND_FIELD_NUMBER = 1;
private java.util.List command_;
/**
* repeated .Command command = 1;
*/
public java.util.List getCommandList() {
return command_;
}
/**
* repeated .Command command = 1;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.CommandOrBuilder>
getCommandOrBuilderList() {
return command_;
}
/**
* repeated .Command command = 1;
*/
public int getCommandCount() {
return command_.size();
}
/**
* repeated .Command command = 1;
*/
public org.apache.tajo.ResourceProtos.Command getCommand(int index) {
return command_.get(index);
}
/**
* repeated .Command command = 1;
*/
public org.apache.tajo.ResourceProtos.CommandOrBuilder getCommandOrBuilder(
int index) {
return command_.get(index);
}
private void initFields() {
command_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
for (int i = 0; i < getCommandCount(); i++) {
if (!getCommand(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < command_.size(); i++) {
output.writeMessage(1, command_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < command_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, command_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.CommandRequestProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.CommandRequestProto other = (org.apache.tajo.ResourceProtos.CommandRequestProto) obj;
boolean result = true;
result = result && getCommandList()
.equals(other.getCommandList());
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (getCommandCount() > 0) {
hash = (37 * hash) + COMMAND_FIELD_NUMBER;
hash = (53 * hash) + getCommandList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.CommandRequestProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.CommandRequestProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.CommandRequestProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.CommandRequestProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.CommandRequestProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.CommandRequestProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.CommandRequestProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.CommandRequestProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.CommandRequestProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.CommandRequestProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.CommandRequestProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code CommandRequestProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.CommandRequestProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_CommandRequestProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_CommandRequestProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.CommandRequestProto.class, org.apache.tajo.ResourceProtos.CommandRequestProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.CommandRequestProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getCommandFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (commandBuilder_ == null) {
command_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
commandBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_CommandRequestProto_descriptor;
}
public org.apache.tajo.ResourceProtos.CommandRequestProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.CommandRequestProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.CommandRequestProto build() {
org.apache.tajo.ResourceProtos.CommandRequestProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.CommandRequestProto buildPartial() {
org.apache.tajo.ResourceProtos.CommandRequestProto result = new org.apache.tajo.ResourceProtos.CommandRequestProto(this);
int from_bitField0_ = bitField0_;
if (commandBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
command_ = java.util.Collections.unmodifiableList(command_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.command_ = command_;
} else {
result.command_ = commandBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.CommandRequestProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.CommandRequestProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.CommandRequestProto other) {
if (other == org.apache.tajo.ResourceProtos.CommandRequestProto.getDefaultInstance()) return this;
if (commandBuilder_ == null) {
if (!other.command_.isEmpty()) {
if (command_.isEmpty()) {
command_ = other.command_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureCommandIsMutable();
command_.addAll(other.command_);
}
onChanged();
}
} else {
if (!other.command_.isEmpty()) {
if (commandBuilder_.isEmpty()) {
commandBuilder_.dispose();
commandBuilder_ = null;
command_ = other.command_;
bitField0_ = (bitField0_ & ~0x00000001);
commandBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getCommandFieldBuilder() : null;
} else {
commandBuilder_.addAllMessages(other.command_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getCommandCount(); i++) {
if (!getCommand(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.CommandRequestProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.CommandRequestProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated .Command command = 1;
private java.util.List command_ =
java.util.Collections.emptyList();
private void ensureCommandIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
command_ = new java.util.ArrayList(command_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.Command, org.apache.tajo.ResourceProtos.Command.Builder, org.apache.tajo.ResourceProtos.CommandOrBuilder> commandBuilder_;
/**
* repeated .Command command = 1;
*/
public java.util.List getCommandList() {
if (commandBuilder_ == null) {
return java.util.Collections.unmodifiableList(command_);
} else {
return commandBuilder_.getMessageList();
}
}
/**
* repeated .Command command = 1;
*/
public int getCommandCount() {
if (commandBuilder_ == null) {
return command_.size();
} else {
return commandBuilder_.getCount();
}
}
/**
* repeated .Command command = 1;
*/
public org.apache.tajo.ResourceProtos.Command getCommand(int index) {
if (commandBuilder_ == null) {
return command_.get(index);
} else {
return commandBuilder_.getMessage(index);
}
}
/**
* repeated .Command command = 1;
*/
public Builder setCommand(
int index, org.apache.tajo.ResourceProtos.Command value) {
if (commandBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommandIsMutable();
command_.set(index, value);
onChanged();
} else {
commandBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .Command command = 1;
*/
public Builder setCommand(
int index, org.apache.tajo.ResourceProtos.Command.Builder builderForValue) {
if (commandBuilder_ == null) {
ensureCommandIsMutable();
command_.set(index, builderForValue.build());
onChanged();
} else {
commandBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Command command = 1;
*/
public Builder addCommand(org.apache.tajo.ResourceProtos.Command value) {
if (commandBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommandIsMutable();
command_.add(value);
onChanged();
} else {
commandBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .Command command = 1;
*/
public Builder addCommand(
int index, org.apache.tajo.ResourceProtos.Command value) {
if (commandBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommandIsMutable();
command_.add(index, value);
onChanged();
} else {
commandBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .Command command = 1;
*/
public Builder addCommand(
org.apache.tajo.ResourceProtos.Command.Builder builderForValue) {
if (commandBuilder_ == null) {
ensureCommandIsMutable();
command_.add(builderForValue.build());
onChanged();
} else {
commandBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .Command command = 1;
*/
public Builder addCommand(
int index, org.apache.tajo.ResourceProtos.Command.Builder builderForValue) {
if (commandBuilder_ == null) {
ensureCommandIsMutable();
command_.add(index, builderForValue.build());
onChanged();
} else {
commandBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Command command = 1;
*/
public Builder addAllCommand(
java.lang.Iterable extends org.apache.tajo.ResourceProtos.Command> values) {
if (commandBuilder_ == null) {
ensureCommandIsMutable();
super.addAll(values, command_);
onChanged();
} else {
commandBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .Command command = 1;
*/
public Builder clearCommand() {
if (commandBuilder_ == null) {
command_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
commandBuilder_.clear();
}
return this;
}
/**
* repeated .Command command = 1;
*/
public Builder removeCommand(int index) {
if (commandBuilder_ == null) {
ensureCommandIsMutable();
command_.remove(index);
onChanged();
} else {
commandBuilder_.remove(index);
}
return this;
}
/**
* repeated .Command command = 1;
*/
public org.apache.tajo.ResourceProtos.Command.Builder getCommandBuilder(
int index) {
return getCommandFieldBuilder().getBuilder(index);
}
/**
* repeated .Command command = 1;
*/
public org.apache.tajo.ResourceProtos.CommandOrBuilder getCommandOrBuilder(
int index) {
if (commandBuilder_ == null) {
return command_.get(index); } else {
return commandBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .Command command = 1;
*/
public java.util.List extends org.apache.tajo.ResourceProtos.CommandOrBuilder>
getCommandOrBuilderList() {
if (commandBuilder_ != null) {
return commandBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(command_);
}
}
/**
* repeated .Command command = 1;
*/
public org.apache.tajo.ResourceProtos.Command.Builder addCommandBuilder() {
return getCommandFieldBuilder().addBuilder(
org.apache.tajo.ResourceProtos.Command.getDefaultInstance());
}
/**
* repeated .Command command = 1;
*/
public org.apache.tajo.ResourceProtos.Command.Builder addCommandBuilder(
int index) {
return getCommandFieldBuilder().addBuilder(
index, org.apache.tajo.ResourceProtos.Command.getDefaultInstance());
}
/**
* repeated .Command command = 1;
*/
public java.util.List
getCommandBuilderList() {
return getCommandFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.Command, org.apache.tajo.ResourceProtos.Command.Builder, org.apache.tajo.ResourceProtos.CommandOrBuilder>
getCommandFieldBuilder() {
if (commandBuilder_ == null) {
commandBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.ResourceProtos.Command, org.apache.tajo.ResourceProtos.Command.Builder, org.apache.tajo.ResourceProtos.CommandOrBuilder>(
command_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
command_ = null;
}
return commandBuilder_;
}
// @@protoc_insertion_point(builder_scope:CommandRequestProto)
}
static {
defaultInstance = new CommandRequestProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:CommandRequestProto)
}
public interface CommandResponseProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code CommandResponseProto}
*/
public static final class CommandResponseProto extends
com.google.protobuf.GeneratedMessage
implements CommandResponseProtoOrBuilder {
// Use CommandResponseProto.newBuilder() to construct.
private CommandResponseProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private CommandResponseProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final CommandResponseProto defaultInstance;
public static CommandResponseProto getDefaultInstance() {
return defaultInstance;
}
public CommandResponseProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommandResponseProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_CommandResponseProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_CommandResponseProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.CommandResponseProto.class, org.apache.tajo.ResourceProtos.CommandResponseProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public CommandResponseProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommandResponseProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private void initFields() {
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.CommandResponseProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.CommandResponseProto other = (org.apache.tajo.ResourceProtos.CommandResponseProto) obj;
boolean result = true;
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.CommandResponseProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.CommandResponseProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.CommandResponseProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.CommandResponseProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.CommandResponseProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.CommandResponseProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.CommandResponseProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.CommandResponseProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.CommandResponseProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.CommandResponseProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.CommandResponseProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code CommandResponseProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.CommandResponseProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_CommandResponseProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_CommandResponseProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.CommandResponseProto.class, org.apache.tajo.ResourceProtos.CommandResponseProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.CommandResponseProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_CommandResponseProto_descriptor;
}
public org.apache.tajo.ResourceProtos.CommandResponseProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.CommandResponseProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.CommandResponseProto build() {
org.apache.tajo.ResourceProtos.CommandResponseProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.CommandResponseProto buildPartial() {
org.apache.tajo.ResourceProtos.CommandResponseProto result = new org.apache.tajo.ResourceProtos.CommandResponseProto(this);
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.CommandResponseProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.CommandResponseProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.CommandResponseProto other) {
if (other == org.apache.tajo.ResourceProtos.CommandResponseProto.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.CommandResponseProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.CommandResponseProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
// @@protoc_insertion_point(builder_scope:CommandResponseProto)
}
static {
defaultInstance = new CommandResponseProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:CommandResponseProto)
}
public interface CommandOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required .TaskAttemptIdProto id = 1;
/**
* required .TaskAttemptIdProto id = 1;
*/
boolean hasId();
/**
* required .TaskAttemptIdProto id = 1;
*/
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId();
/**
* required .TaskAttemptIdProto id = 1;
*/
org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder();
// required .CommandType type = 2;
/**
* required .CommandType type = 2;
*/
boolean hasType();
/**
* required .CommandType type = 2;
*/
org.apache.tajo.ResourceProtos.CommandType getType();
}
/**
* Protobuf type {@code Command}
*/
public static final class Command extends
com.google.protobuf.GeneratedMessage
implements CommandOrBuilder {
// Use Command.newBuilder() to construct.
private Command(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Command(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Command defaultInstance;
public static Command getDefaultInstance() {
return defaultInstance;
}
public Command getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Command(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = id_.toBuilder();
}
id_ = input.readMessage(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(id_);
id_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 16: {
int rawValue = input.readEnum();
org.apache.tajo.ResourceProtos.CommandType value = org.apache.tajo.ResourceProtos.CommandType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
type_ = value;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_Command_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_Command_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.Command.class, org.apache.tajo.ResourceProtos.Command.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Command parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Command(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .TaskAttemptIdProto id = 1;
public static final int ID_FIELD_NUMBER = 1;
private org.apache.tajo.TajoIdProtos.TaskAttemptIdProto id_;
/**
* required .TaskAttemptIdProto id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId() {
return id_;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder() {
return id_;
}
// required .CommandType type = 2;
public static final int TYPE_FIELD_NUMBER = 2;
private org.apache.tajo.ResourceProtos.CommandType type_;
/**
* required .CommandType type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .CommandType type = 2;
*/
public org.apache.tajo.ResourceProtos.CommandType getType() {
return type_;
}
private void initFields() {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
type_ = org.apache.tajo.ResourceProtos.CommandType.PREPARE;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
if (!getId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, type_.getNumber());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, type_.getNumber());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.Command)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.Command other = (org.apache.tajo.ResourceProtos.Command) obj;
boolean result = true;
result = result && (hasId() == other.hasId());
if (hasId()) {
result = result && getId()
.equals(other.getId());
}
result = result && (hasType() == other.hasType());
if (hasType()) {
result = result &&
(getType() == other.getType());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + hashEnum(getType());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.Command parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.Command parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.Command parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.Command parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.Command parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.Command parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.Command parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.Command parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.Command parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.Command parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.Command prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Command}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.CommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_Command_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_Command_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.Command.class, org.apache.tajo.ResourceProtos.Command.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.Command.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getIdFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (idBuilder_ == null) {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
type_ = org.apache.tajo.ResourceProtos.CommandType.PREPARE;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_Command_descriptor;
}
public org.apache.tajo.ResourceProtos.Command getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.Command.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.Command build() {
org.apache.tajo.ResourceProtos.Command result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.Command buildPartial() {
org.apache.tajo.ResourceProtos.Command result = new org.apache.tajo.ResourceProtos.Command(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (idBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = idBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.type_ = type_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.Command) {
return mergeFrom((org.apache.tajo.ResourceProtos.Command)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.Command other) {
if (other == org.apache.tajo.ResourceProtos.Command.getDefaultInstance()) return this;
if (other.hasId()) {
mergeId(other.getId());
}
if (other.hasType()) {
setType(other.getType());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasId()) {
return false;
}
if (!hasType()) {
return false;
}
if (!getId().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.Command parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.Command) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .TaskAttemptIdProto id = 1;
private org.apache.tajo.TajoIdProtos.TaskAttemptIdProto id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder> idBuilder_;
/**
* required .TaskAttemptIdProto id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto getId() {
if (idBuilder_ == null) {
return id_;
} else {
return idBuilder_.getMessage();
}
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder setId(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto value) {
if (idBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
idBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder setId(
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder builderForValue) {
if (idBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
idBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder mergeId(org.apache.tajo.TajoIdProtos.TaskAttemptIdProto value) {
if (idBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
id_ != org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance()) {
id_ =
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.newBuilder(id_).mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
idBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public Builder clearId() {
if (idBuilder_ == null) {
id_ = org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.getDefaultInstance();
onChanged();
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder getIdBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getIdFieldBuilder().getBuilder();
}
/**
* required .TaskAttemptIdProto id = 1;
*/
public org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder getIdOrBuilder() {
if (idBuilder_ != null) {
return idBuilder_.getMessageOrBuilder();
} else {
return id_;
}
}
/**
* required .TaskAttemptIdProto id = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>
getIdFieldBuilder() {
if (idBuilder_ == null) {
idBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.TaskAttemptIdProto, org.apache.tajo.TajoIdProtos.TaskAttemptIdProto.Builder, org.apache.tajo.TajoIdProtos.TaskAttemptIdProtoOrBuilder>(
id_,
getParentForChildren(),
isClean());
id_ = null;
}
return idBuilder_;
}
// required .CommandType type = 2;
private org.apache.tajo.ResourceProtos.CommandType type_ = org.apache.tajo.ResourceProtos.CommandType.PREPARE;
/**
* required .CommandType type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .CommandType type = 2;
*/
public org.apache.tajo.ResourceProtos.CommandType getType() {
return type_;
}
/**
* required .CommandType type = 2;
*/
public Builder setType(org.apache.tajo.ResourceProtos.CommandType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
/**
* required .CommandType type = 2;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000002);
type_ = org.apache.tajo.ResourceProtos.CommandType.PREPARE;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:Command)
}
static {
defaultInstance = new Command(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Command)
}
public interface ShuffleFileOutputOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 partId = 1;
/**
* required int32 partId = 1;
*/
boolean hasPartId();
/**
* required int32 partId = 1;
*/
int getPartId();
// optional string fileName = 2;
/**
* optional string fileName = 2;
*/
boolean hasFileName();
/**
* optional string fileName = 2;
*/
java.lang.String getFileName();
/**
* optional string fileName = 2;
*/
com.google.protobuf.ByteString
getFileNameBytes();
// optional int64 volume = 3;
/**
* optional int64 volume = 3;
*/
boolean hasVolume();
/**
* optional int64 volume = 3;
*/
long getVolume();
}
/**
* Protobuf type {@code ShuffleFileOutput}
*/
public static final class ShuffleFileOutput extends
com.google.protobuf.GeneratedMessage
implements ShuffleFileOutputOrBuilder {
// Use ShuffleFileOutput.newBuilder() to construct.
private ShuffleFileOutput(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ShuffleFileOutput(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ShuffleFileOutput defaultInstance;
public static ShuffleFileOutput getDefaultInstance() {
return defaultInstance;
}
public ShuffleFileOutput getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ShuffleFileOutput(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
partId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
fileName_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000004;
volume_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_ShuffleFileOutput_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_ShuffleFileOutput_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.ShuffleFileOutput.class, org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ShuffleFileOutput parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ShuffleFileOutput(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required int32 partId = 1;
public static final int PARTID_FIELD_NUMBER = 1;
private int partId_;
/**
* required int32 partId = 1;
*/
public boolean hasPartId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 partId = 1;
*/
public int getPartId() {
return partId_;
}
// optional string fileName = 2;
public static final int FILENAME_FIELD_NUMBER = 2;
private java.lang.Object fileName_;
/**
* optional string fileName = 2;
*/
public boolean hasFileName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string fileName = 2;
*/
public java.lang.String getFileName() {
java.lang.Object ref = fileName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fileName_ = s;
}
return s;
}
}
/**
* optional string fileName = 2;
*/
public com.google.protobuf.ByteString
getFileNameBytes() {
java.lang.Object ref = fileName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional int64 volume = 3;
public static final int VOLUME_FIELD_NUMBER = 3;
private long volume_;
/**
* optional int64 volume = 3;
*/
public boolean hasVolume() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 volume = 3;
*/
public long getVolume() {
return volume_;
}
private void initFields() {
partId_ = 0;
fileName_ = "";
volume_ = 0L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasPartId()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, partId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getFileNameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt64(3, volume_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, partId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getFileNameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, volume_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.ShuffleFileOutput)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.ShuffleFileOutput other = (org.apache.tajo.ResourceProtos.ShuffleFileOutput) obj;
boolean result = true;
result = result && (hasPartId() == other.hasPartId());
if (hasPartId()) {
result = result && (getPartId()
== other.getPartId());
}
result = result && (hasFileName() == other.hasFileName());
if (hasFileName()) {
result = result && getFileName()
.equals(other.getFileName());
}
result = result && (hasVolume() == other.hasVolume());
if (hasVolume()) {
result = result && (getVolume()
== other.getVolume());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasPartId()) {
hash = (37 * hash) + PARTID_FIELD_NUMBER;
hash = (53 * hash) + getPartId();
}
if (hasFileName()) {
hash = (37 * hash) + FILENAME_FIELD_NUMBER;
hash = (53 * hash) + getFileName().hashCode();
}
if (hasVolume()) {
hash = (37 * hash) + VOLUME_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getVolume());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.ShuffleFileOutput parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.ShuffleFileOutput parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.ShuffleFileOutput parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.ShuffleFileOutput parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.ShuffleFileOutput parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.ShuffleFileOutput parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.ShuffleFileOutput parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.ShuffleFileOutput parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.ShuffleFileOutput parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.ShuffleFileOutput parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.ShuffleFileOutput prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ShuffleFileOutput}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.ShuffleFileOutputOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_ShuffleFileOutput_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_ShuffleFileOutput_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.ShuffleFileOutput.class, org.apache.tajo.ResourceProtos.ShuffleFileOutput.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.ShuffleFileOutput.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
partId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
fileName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
volume_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_ShuffleFileOutput_descriptor;
}
public org.apache.tajo.ResourceProtos.ShuffleFileOutput getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.ShuffleFileOutput.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.ShuffleFileOutput build() {
org.apache.tajo.ResourceProtos.ShuffleFileOutput result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.ShuffleFileOutput buildPartial() {
org.apache.tajo.ResourceProtos.ShuffleFileOutput result = new org.apache.tajo.ResourceProtos.ShuffleFileOutput(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.partId_ = partId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.fileName_ = fileName_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.volume_ = volume_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.ShuffleFileOutput) {
return mergeFrom((org.apache.tajo.ResourceProtos.ShuffleFileOutput)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.ShuffleFileOutput other) {
if (other == org.apache.tajo.ResourceProtos.ShuffleFileOutput.getDefaultInstance()) return this;
if (other.hasPartId()) {
setPartId(other.getPartId());
}
if (other.hasFileName()) {
bitField0_ |= 0x00000002;
fileName_ = other.fileName_;
onChanged();
}
if (other.hasVolume()) {
setVolume(other.getVolume());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasPartId()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.ShuffleFileOutput parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.ShuffleFileOutput) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int32 partId = 1;
private int partId_ ;
/**
* required int32 partId = 1;
*/
public boolean hasPartId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 partId = 1;
*/
public int getPartId() {
return partId_;
}
/**
* required int32 partId = 1;
*/
public Builder setPartId(int value) {
bitField0_ |= 0x00000001;
partId_ = value;
onChanged();
return this;
}
/**
* required int32 partId = 1;
*/
public Builder clearPartId() {
bitField0_ = (bitField0_ & ~0x00000001);
partId_ = 0;
onChanged();
return this;
}
// optional string fileName = 2;
private java.lang.Object fileName_ = "";
/**
* optional string fileName = 2;
*/
public boolean hasFileName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string fileName = 2;
*/
public java.lang.String getFileName() {
java.lang.Object ref = fileName_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
fileName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string fileName = 2;
*/
public com.google.protobuf.ByteString
getFileNameBytes() {
java.lang.Object ref = fileName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string fileName = 2;
*/
public Builder setFileName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
fileName_ = value;
onChanged();
return this;
}
/**
* optional string fileName = 2;
*/
public Builder clearFileName() {
bitField0_ = (bitField0_ & ~0x00000002);
fileName_ = getDefaultInstance().getFileName();
onChanged();
return this;
}
/**
* optional string fileName = 2;
*/
public Builder setFileNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
fileName_ = value;
onChanged();
return this;
}
// optional int64 volume = 3;
private long volume_ ;
/**
* optional int64 volume = 3;
*/
public boolean hasVolume() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 volume = 3;
*/
public long getVolume() {
return volume_;
}
/**
* optional int64 volume = 3;
*/
public Builder setVolume(long value) {
bitField0_ |= 0x00000004;
volume_ = value;
onChanged();
return this;
}
/**
* optional int64 volume = 3;
*/
public Builder clearVolume() {
bitField0_ = (bitField0_ & ~0x00000004);
volume_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:ShuffleFileOutput)
}
static {
defaultInstance = new ShuffleFileOutput(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ShuffleFileOutput)
}
public interface SessionProtoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string session_id = 1;
/**
* required string session_id = 1;
*/
boolean hasSessionId();
/**
* required string session_id = 1;
*/
java.lang.String getSessionId();
/**
* required string session_id = 1;
*/
com.google.protobuf.ByteString
getSessionIdBytes();
// required string username = 2;
/**
* required string username = 2;
*/
boolean hasUsername();
/**
* required string username = 2;
*/
java.lang.String getUsername();
/**
* required string username = 2;
*/
com.google.protobuf.ByteString
getUsernameBytes();
// required string current_database = 3;
/**
* required string current_database = 3;
*/
boolean hasCurrentDatabase();
/**
* required string current_database = 3;
*/
java.lang.String getCurrentDatabase();
/**
* required string current_database = 3;
*/
com.google.protobuf.ByteString
getCurrentDatabaseBytes();
// required int64 last_access_time = 4;
/**
* required int64 last_access_time = 4;
*/
boolean hasLastAccessTime();
/**
* required int64 last_access_time = 4;
*/
long getLastAccessTime();
// required .KeyValueSetProto variables = 5;
/**
* required .KeyValueSetProto variables = 5;
*/
boolean hasVariables();
/**
* required .KeyValueSetProto variables = 5;
*/
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto getVariables();
/**
* required .KeyValueSetProto variables = 5;
*/
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProtoOrBuilder getVariablesOrBuilder();
}
/**
* Protobuf type {@code SessionProto}
*/
public static final class SessionProto extends
com.google.protobuf.GeneratedMessage
implements SessionProtoOrBuilder {
// Use SessionProto.newBuilder() to construct.
private SessionProto(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private SessionProto(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final SessionProto defaultInstance;
public static SessionProto getDefaultInstance() {
return defaultInstance;
}
public SessionProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SessionProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
sessionId_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
username_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
currentDatabase_ = input.readBytes();
break;
}
case 32: {
bitField0_ |= 0x00000008;
lastAccessTime_ = input.readInt64();
break;
}
case 42: {
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = variables_.toBuilder();
}
variables_ = input.readMessage(org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(variables_);
variables_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_SessionProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_SessionProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.SessionProto.class, org.apache.tajo.ResourceProtos.SessionProto.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public SessionProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SessionProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string session_id = 1;
public static final int SESSION_ID_FIELD_NUMBER = 1;
private java.lang.Object sessionId_;
/**
* required string session_id = 1;
*/
public boolean hasSessionId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string session_id = 1;
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
sessionId_ = s;
}
return s;
}
}
/**
* required string session_id = 1;
*/
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required string username = 2;
public static final int USERNAME_FIELD_NUMBER = 2;
private java.lang.Object username_;
/**
* required string username = 2;
*/
public boolean hasUsername() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string username = 2;
*/
public java.lang.String getUsername() {
java.lang.Object ref = username_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
username_ = s;
}
return s;
}
}
/**
* required string username = 2;
*/
public com.google.protobuf.ByteString
getUsernameBytes() {
java.lang.Object ref = username_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
username_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required string current_database = 3;
public static final int CURRENT_DATABASE_FIELD_NUMBER = 3;
private java.lang.Object currentDatabase_;
/**
* required string current_database = 3;
*/
public boolean hasCurrentDatabase() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required string current_database = 3;
*/
public java.lang.String getCurrentDatabase() {
java.lang.Object ref = currentDatabase_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
currentDatabase_ = s;
}
return s;
}
}
/**
* required string current_database = 3;
*/
public com.google.protobuf.ByteString
getCurrentDatabaseBytes() {
java.lang.Object ref = currentDatabase_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currentDatabase_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required int64 last_access_time = 4;
public static final int LAST_ACCESS_TIME_FIELD_NUMBER = 4;
private long lastAccessTime_;
/**
* required int64 last_access_time = 4;
*/
public boolean hasLastAccessTime() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required int64 last_access_time = 4;
*/
public long getLastAccessTime() {
return lastAccessTime_;
}
// required .KeyValueSetProto variables = 5;
public static final int VARIABLES_FIELD_NUMBER = 5;
private org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto variables_;
/**
* required .KeyValueSetProto variables = 5;
*/
public boolean hasVariables() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required .KeyValueSetProto variables = 5;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto getVariables() {
return variables_;
}
/**
* required .KeyValueSetProto variables = 5;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProtoOrBuilder getVariablesOrBuilder() {
return variables_;
}
private void initFields() {
sessionId_ = "";
username_ = "";
currentDatabase_ = "";
lastAccessTime_ = 0L;
variables_ = org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasSessionId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasUsername()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasCurrentDatabase()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasLastAccessTime()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasVariables()) {
memoizedIsInitialized = 0;
return false;
}
if (!getVariables().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getSessionIdBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getUsernameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getCurrentDatabaseBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt64(4, lastAccessTime_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(5, variables_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getSessionIdBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getUsernameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getCurrentDatabaseBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, lastAccessTime_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, variables_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.SessionProto)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.SessionProto other = (org.apache.tajo.ResourceProtos.SessionProto) obj;
boolean result = true;
result = result && (hasSessionId() == other.hasSessionId());
if (hasSessionId()) {
result = result && getSessionId()
.equals(other.getSessionId());
}
result = result && (hasUsername() == other.hasUsername());
if (hasUsername()) {
result = result && getUsername()
.equals(other.getUsername());
}
result = result && (hasCurrentDatabase() == other.hasCurrentDatabase());
if (hasCurrentDatabase()) {
result = result && getCurrentDatabase()
.equals(other.getCurrentDatabase());
}
result = result && (hasLastAccessTime() == other.hasLastAccessTime());
if (hasLastAccessTime()) {
result = result && (getLastAccessTime()
== other.getLastAccessTime());
}
result = result && (hasVariables() == other.hasVariables());
if (hasVariables()) {
result = result && getVariables()
.equals(other.getVariables());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasSessionId()) {
hash = (37 * hash) + SESSION_ID_FIELD_NUMBER;
hash = (53 * hash) + getSessionId().hashCode();
}
if (hasUsername()) {
hash = (37 * hash) + USERNAME_FIELD_NUMBER;
hash = (53 * hash) + getUsername().hashCode();
}
if (hasCurrentDatabase()) {
hash = (37 * hash) + CURRENT_DATABASE_FIELD_NUMBER;
hash = (53 * hash) + getCurrentDatabase().hashCode();
}
if (hasLastAccessTime()) {
hash = (37 * hash) + LAST_ACCESS_TIME_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getLastAccessTime());
}
if (hasVariables()) {
hash = (37 * hash) + VARIABLES_FIELD_NUMBER;
hash = (53 * hash) + getVariables().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.SessionProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.SessionProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.SessionProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.SessionProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.SessionProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.SessionProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.SessionProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.SessionProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.SessionProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.SessionProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.SessionProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code SessionProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.SessionProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_SessionProto_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_SessionProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.SessionProto.class, org.apache.tajo.ResourceProtos.SessionProto.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.SessionProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getVariablesFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
sessionId_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
username_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
currentDatabase_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
lastAccessTime_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
if (variablesBuilder_ == null) {
variables_ = org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.getDefaultInstance();
} else {
variablesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_SessionProto_descriptor;
}
public org.apache.tajo.ResourceProtos.SessionProto getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.SessionProto.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.SessionProto build() {
org.apache.tajo.ResourceProtos.SessionProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.SessionProto buildPartial() {
org.apache.tajo.ResourceProtos.SessionProto result = new org.apache.tajo.ResourceProtos.SessionProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.sessionId_ = sessionId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.username_ = username_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.currentDatabase_ = currentDatabase_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.lastAccessTime_ = lastAccessTime_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (variablesBuilder_ == null) {
result.variables_ = variables_;
} else {
result.variables_ = variablesBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.SessionProto) {
return mergeFrom((org.apache.tajo.ResourceProtos.SessionProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.SessionProto other) {
if (other == org.apache.tajo.ResourceProtos.SessionProto.getDefaultInstance()) return this;
if (other.hasSessionId()) {
bitField0_ |= 0x00000001;
sessionId_ = other.sessionId_;
onChanged();
}
if (other.hasUsername()) {
bitField0_ |= 0x00000002;
username_ = other.username_;
onChanged();
}
if (other.hasCurrentDatabase()) {
bitField0_ |= 0x00000004;
currentDatabase_ = other.currentDatabase_;
onChanged();
}
if (other.hasLastAccessTime()) {
setLastAccessTime(other.getLastAccessTime());
}
if (other.hasVariables()) {
mergeVariables(other.getVariables());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasSessionId()) {
return false;
}
if (!hasUsername()) {
return false;
}
if (!hasCurrentDatabase()) {
return false;
}
if (!hasLastAccessTime()) {
return false;
}
if (!hasVariables()) {
return false;
}
if (!getVariables().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.SessionProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.SessionProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string session_id = 1;
private java.lang.Object sessionId_ = "";
/**
* required string session_id = 1;
*/
public boolean hasSessionId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string session_id = 1;
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
sessionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string session_id = 1;
*/
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string session_id = 1;
*/
public Builder setSessionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
sessionId_ = value;
onChanged();
return this;
}
/**
* required string session_id = 1;
*/
public Builder clearSessionId() {
bitField0_ = (bitField0_ & ~0x00000001);
sessionId_ = getDefaultInstance().getSessionId();
onChanged();
return this;
}
/**
* required string session_id = 1;
*/
public Builder setSessionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
sessionId_ = value;
onChanged();
return this;
}
// required string username = 2;
private java.lang.Object username_ = "";
/**
* required string username = 2;
*/
public boolean hasUsername() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string username = 2;
*/
public java.lang.String getUsername() {
java.lang.Object ref = username_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
username_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string username = 2;
*/
public com.google.protobuf.ByteString
getUsernameBytes() {
java.lang.Object ref = username_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
username_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string username = 2;
*/
public Builder setUsername(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
username_ = value;
onChanged();
return this;
}
/**
* required string username = 2;
*/
public Builder clearUsername() {
bitField0_ = (bitField0_ & ~0x00000002);
username_ = getDefaultInstance().getUsername();
onChanged();
return this;
}
/**
* required string username = 2;
*/
public Builder setUsernameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
username_ = value;
onChanged();
return this;
}
// required string current_database = 3;
private java.lang.Object currentDatabase_ = "";
/**
* required string current_database = 3;
*/
public boolean hasCurrentDatabase() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required string current_database = 3;
*/
public java.lang.String getCurrentDatabase() {
java.lang.Object ref = currentDatabase_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
currentDatabase_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string current_database = 3;
*/
public com.google.protobuf.ByteString
getCurrentDatabaseBytes() {
java.lang.Object ref = currentDatabase_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currentDatabase_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string current_database = 3;
*/
public Builder setCurrentDatabase(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
currentDatabase_ = value;
onChanged();
return this;
}
/**
* required string current_database = 3;
*/
public Builder clearCurrentDatabase() {
bitField0_ = (bitField0_ & ~0x00000004);
currentDatabase_ = getDefaultInstance().getCurrentDatabase();
onChanged();
return this;
}
/**
* required string current_database = 3;
*/
public Builder setCurrentDatabaseBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
currentDatabase_ = value;
onChanged();
return this;
}
// required int64 last_access_time = 4;
private long lastAccessTime_ ;
/**
* required int64 last_access_time = 4;
*/
public boolean hasLastAccessTime() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required int64 last_access_time = 4;
*/
public long getLastAccessTime() {
return lastAccessTime_;
}
/**
* required int64 last_access_time = 4;
*/
public Builder setLastAccessTime(long value) {
bitField0_ |= 0x00000008;
lastAccessTime_ = value;
onChanged();
return this;
}
/**
* required int64 last_access_time = 4;
*/
public Builder clearLastAccessTime() {
bitField0_ = (bitField0_ & ~0x00000008);
lastAccessTime_ = 0L;
onChanged();
return this;
}
// required .KeyValueSetProto variables = 5;
private org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto variables_ = org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.Builder, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProtoOrBuilder> variablesBuilder_;
/**
* required .KeyValueSetProto variables = 5;
*/
public boolean hasVariables() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required .KeyValueSetProto variables = 5;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto getVariables() {
if (variablesBuilder_ == null) {
return variables_;
} else {
return variablesBuilder_.getMessage();
}
}
/**
* required .KeyValueSetProto variables = 5;
*/
public Builder setVariables(org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto value) {
if (variablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
variables_ = value;
onChanged();
} else {
variablesBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* required .KeyValueSetProto variables = 5;
*/
public Builder setVariables(
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.Builder builderForValue) {
if (variablesBuilder_ == null) {
variables_ = builderForValue.build();
onChanged();
} else {
variablesBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
* required .KeyValueSetProto variables = 5;
*/
public Builder mergeVariables(org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto value) {
if (variablesBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
variables_ != org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.getDefaultInstance()) {
variables_ =
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.newBuilder(variables_).mergeFrom(value).buildPartial();
} else {
variables_ = value;
}
onChanged();
} else {
variablesBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* required .KeyValueSetProto variables = 5;
*/
public Builder clearVariables() {
if (variablesBuilder_ == null) {
variables_ = org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.getDefaultInstance();
onChanged();
} else {
variablesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* required .KeyValueSetProto variables = 5;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.Builder getVariablesBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getVariablesFieldBuilder().getBuilder();
}
/**
* required .KeyValueSetProto variables = 5;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProtoOrBuilder getVariablesOrBuilder() {
if (variablesBuilder_ != null) {
return variablesBuilder_.getMessageOrBuilder();
} else {
return variables_;
}
}
/**
* required .KeyValueSetProto variables = 5;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.Builder, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProtoOrBuilder>
getVariablesFieldBuilder() {
if (variablesBuilder_ == null) {
variablesBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProto.Builder, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.KeyValueSetProtoOrBuilder>(
variables_,
getParentForChildren(),
isClean());
variables_ = null;
}
return variablesBuilder_;
}
// @@protoc_insertion_point(builder_scope:SessionProto)
}
static {
defaultInstance = new SessionProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:SessionProto)
}
public interface NodeHeartbeatRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 workerId = 1;
/**
* required int32 workerId = 1;
*/
boolean hasWorkerId();
/**
* required int32 workerId = 1;
*/
int getWorkerId();
// optional .NodeResourceProto totalResource = 2;
/**
* optional .NodeResourceProto totalResource = 2;
*/
boolean hasTotalResource();
/**
* optional .NodeResourceProto totalResource = 2;
*/
org.apache.tajo.TajoProtos.NodeResourceProto getTotalResource();
/**
* optional .NodeResourceProto totalResource = 2;
*/
org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder getTotalResourceOrBuilder();
// optional .NodeResourceProto availableResource = 3;
/**
* optional .NodeResourceProto availableResource = 3;
*/
boolean hasAvailableResource();
/**
* optional .NodeResourceProto availableResource = 3;
*/
org.apache.tajo.TajoProtos.NodeResourceProto getAvailableResource();
/**
* optional .NodeResourceProto availableResource = 3;
*/
org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder getAvailableResourceOrBuilder();
// optional int32 runningTasks = 4;
/**
* optional int32 runningTasks = 4;
*/
boolean hasRunningTasks();
/**
* optional int32 runningTasks = 4;
*/
int getRunningTasks();
// optional int32 runningQueryMasters = 5;
/**
* optional int32 runningQueryMasters = 5;
*/
boolean hasRunningQueryMasters();
/**
* optional int32 runningQueryMasters = 5;
*/
int getRunningQueryMasters();
// optional .WorkerConnectionInfoProto connectionInfo = 6;
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
boolean hasConnectionInfo();
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto getConnectionInfo();
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder getConnectionInfoOrBuilder();
// optional .NodeStatusProto status = 7;
/**
* optional .NodeStatusProto status = 7;
*/
boolean hasStatus();
/**
* optional .NodeStatusProto status = 7;
*/
org.apache.tajo.ResourceProtos.NodeStatusProto getStatus();
/**
* optional .NodeStatusProto status = 7;
*/
org.apache.tajo.ResourceProtos.NodeStatusProtoOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code NodeHeartbeatRequest}
*/
public static final class NodeHeartbeatRequest extends
com.google.protobuf.GeneratedMessage
implements NodeHeartbeatRequestOrBuilder {
// Use NodeHeartbeatRequest.newBuilder() to construct.
private NodeHeartbeatRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private NodeHeartbeatRequest(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final NodeHeartbeatRequest defaultInstance;
public static NodeHeartbeatRequest getDefaultInstance() {
return defaultInstance;
}
public NodeHeartbeatRequest getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NodeHeartbeatRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
workerId_ = input.readInt32();
break;
}
case 18: {
org.apache.tajo.TajoProtos.NodeResourceProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = totalResource_.toBuilder();
}
totalResource_ = input.readMessage(org.apache.tajo.TajoProtos.NodeResourceProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(totalResource_);
totalResource_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
org.apache.tajo.TajoProtos.NodeResourceProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = availableResource_.toBuilder();
}
availableResource_ = input.readMessage(org.apache.tajo.TajoProtos.NodeResourceProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(availableResource_);
availableResource_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 32: {
bitField0_ |= 0x00000008;
runningTasks_ = input.readInt32();
break;
}
case 40: {
bitField0_ |= 0x00000010;
runningQueryMasters_ = input.readInt32();
break;
}
case 50: {
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
subBuilder = connectionInfo_.toBuilder();
}
connectionInfo_ = input.readMessage(org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(connectionInfo_);
connectionInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
case 58: {
org.apache.tajo.ResourceProtos.NodeStatusProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(org.apache.tajo.ResourceProtos.NodeStatusProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_NodeHeartbeatRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_NodeHeartbeatRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.NodeHeartbeatRequest.class, org.apache.tajo.ResourceProtos.NodeHeartbeatRequest.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public NodeHeartbeatRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NodeHeartbeatRequest(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required int32 workerId = 1;
public static final int WORKERID_FIELD_NUMBER = 1;
private int workerId_;
/**
* required int32 workerId = 1;
*/
public boolean hasWorkerId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 workerId = 1;
*/
public int getWorkerId() {
return workerId_;
}
// optional .NodeResourceProto totalResource = 2;
public static final int TOTALRESOURCE_FIELD_NUMBER = 2;
private org.apache.tajo.TajoProtos.NodeResourceProto totalResource_;
/**
* optional .NodeResourceProto totalResource = 2;
*/
public boolean hasTotalResource() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .NodeResourceProto totalResource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProto getTotalResource() {
return totalResource_;
}
/**
* optional .NodeResourceProto totalResource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder getTotalResourceOrBuilder() {
return totalResource_;
}
// optional .NodeResourceProto availableResource = 3;
public static final int AVAILABLERESOURCE_FIELD_NUMBER = 3;
private org.apache.tajo.TajoProtos.NodeResourceProto availableResource_;
/**
* optional .NodeResourceProto availableResource = 3;
*/
public boolean hasAvailableResource() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .NodeResourceProto availableResource = 3;
*/
public org.apache.tajo.TajoProtos.NodeResourceProto getAvailableResource() {
return availableResource_;
}
/**
* optional .NodeResourceProto availableResource = 3;
*/
public org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder getAvailableResourceOrBuilder() {
return availableResource_;
}
// optional int32 runningTasks = 4;
public static final int RUNNINGTASKS_FIELD_NUMBER = 4;
private int runningTasks_;
/**
* optional int32 runningTasks = 4;
*/
public boolean hasRunningTasks() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int32 runningTasks = 4;
*/
public int getRunningTasks() {
return runningTasks_;
}
// optional int32 runningQueryMasters = 5;
public static final int RUNNINGQUERYMASTERS_FIELD_NUMBER = 5;
private int runningQueryMasters_;
/**
* optional int32 runningQueryMasters = 5;
*/
public boolean hasRunningQueryMasters() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 runningQueryMasters = 5;
*/
public int getRunningQueryMasters() {
return runningQueryMasters_;
}
// optional .WorkerConnectionInfoProto connectionInfo = 6;
public static final int CONNECTIONINFO_FIELD_NUMBER = 6;
private org.apache.tajo.TajoProtos.WorkerConnectionInfoProto connectionInfo_;
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
public boolean hasConnectionInfo() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProto getConnectionInfo() {
return connectionInfo_;
}
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder getConnectionInfoOrBuilder() {
return connectionInfo_;
}
// optional .NodeStatusProto status = 7;
public static final int STATUS_FIELD_NUMBER = 7;
private org.apache.tajo.ResourceProtos.NodeStatusProto status_;
/**
* optional .NodeStatusProto status = 7;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .NodeStatusProto status = 7;
*/
public org.apache.tajo.ResourceProtos.NodeStatusProto getStatus() {
return status_;
}
/**
* optional .NodeStatusProto status = 7;
*/
public org.apache.tajo.ResourceProtos.NodeStatusProtoOrBuilder getStatusOrBuilder() {
return status_;
}
private void initFields() {
workerId_ = 0;
totalResource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
availableResource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
runningTasks_ = 0;
runningQueryMasters_ = 0;
connectionInfo_ = org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.getDefaultInstance();
status_ = org.apache.tajo.ResourceProtos.NodeStatusProto.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasWorkerId()) {
memoizedIsInitialized = 0;
return false;
}
if (hasConnectionInfo()) {
if (!getConnectionInfo().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, workerId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, totalResource_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, availableResource_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt32(4, runningTasks_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt32(5, runningQueryMasters_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeMessage(6, connectionInfo_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeMessage(7, status_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, workerId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, totalResource_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, availableResource_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, runningTasks_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, runningQueryMasters_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, connectionInfo_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, status_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.NodeHeartbeatRequest)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.NodeHeartbeatRequest other = (org.apache.tajo.ResourceProtos.NodeHeartbeatRequest) obj;
boolean result = true;
result = result && (hasWorkerId() == other.hasWorkerId());
if (hasWorkerId()) {
result = result && (getWorkerId()
== other.getWorkerId());
}
result = result && (hasTotalResource() == other.hasTotalResource());
if (hasTotalResource()) {
result = result && getTotalResource()
.equals(other.getTotalResource());
}
result = result && (hasAvailableResource() == other.hasAvailableResource());
if (hasAvailableResource()) {
result = result && getAvailableResource()
.equals(other.getAvailableResource());
}
result = result && (hasRunningTasks() == other.hasRunningTasks());
if (hasRunningTasks()) {
result = result && (getRunningTasks()
== other.getRunningTasks());
}
result = result && (hasRunningQueryMasters() == other.hasRunningQueryMasters());
if (hasRunningQueryMasters()) {
result = result && (getRunningQueryMasters()
== other.getRunningQueryMasters());
}
result = result && (hasConnectionInfo() == other.hasConnectionInfo());
if (hasConnectionInfo()) {
result = result && getConnectionInfo()
.equals(other.getConnectionInfo());
}
result = result && (hasStatus() == other.hasStatus());
if (hasStatus()) {
result = result && getStatus()
.equals(other.getStatus());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasWorkerId()) {
hash = (37 * hash) + WORKERID_FIELD_NUMBER;
hash = (53 * hash) + getWorkerId();
}
if (hasTotalResource()) {
hash = (37 * hash) + TOTALRESOURCE_FIELD_NUMBER;
hash = (53 * hash) + getTotalResource().hashCode();
}
if (hasAvailableResource()) {
hash = (37 * hash) + AVAILABLERESOURCE_FIELD_NUMBER;
hash = (53 * hash) + getAvailableResource().hashCode();
}
if (hasRunningTasks()) {
hash = (37 * hash) + RUNNINGTASKS_FIELD_NUMBER;
hash = (53 * hash) + getRunningTasks();
}
if (hasRunningQueryMasters()) {
hash = (37 * hash) + RUNNINGQUERYMASTERS_FIELD_NUMBER;
hash = (53 * hash) + getRunningQueryMasters();
}
if (hasConnectionInfo()) {
hash = (37 * hash) + CONNECTIONINFO_FIELD_NUMBER;
hash = (53 * hash) + getConnectionInfo().hashCode();
}
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.NodeHeartbeatRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code NodeHeartbeatRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.NodeHeartbeatRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_NodeHeartbeatRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_NodeHeartbeatRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.NodeHeartbeatRequest.class, org.apache.tajo.ResourceProtos.NodeHeartbeatRequest.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.NodeHeartbeatRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getTotalResourceFieldBuilder();
getAvailableResourceFieldBuilder();
getConnectionInfoFieldBuilder();
getStatusFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
workerId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
if (totalResourceBuilder_ == null) {
totalResource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
} else {
totalResourceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (availableResourceBuilder_ == null) {
availableResource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
} else {
availableResourceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
runningTasks_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
runningQueryMasters_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
if (connectionInfoBuilder_ == null) {
connectionInfo_ = org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.getDefaultInstance();
} else {
connectionInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
if (statusBuilder_ == null) {
status_ = org.apache.tajo.ResourceProtos.NodeStatusProto.getDefaultInstance();
} else {
statusBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_NodeHeartbeatRequest_descriptor;
}
public org.apache.tajo.ResourceProtos.NodeHeartbeatRequest getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.NodeHeartbeatRequest.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.NodeHeartbeatRequest build() {
org.apache.tajo.ResourceProtos.NodeHeartbeatRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.NodeHeartbeatRequest buildPartial() {
org.apache.tajo.ResourceProtos.NodeHeartbeatRequest result = new org.apache.tajo.ResourceProtos.NodeHeartbeatRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.workerId_ = workerId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (totalResourceBuilder_ == null) {
result.totalResource_ = totalResource_;
} else {
result.totalResource_ = totalResourceBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (availableResourceBuilder_ == null) {
result.availableResource_ = availableResource_;
} else {
result.availableResource_ = availableResourceBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.runningTasks_ = runningTasks_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.runningQueryMasters_ = runningQueryMasters_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
if (connectionInfoBuilder_ == null) {
result.connectionInfo_ = connectionInfo_;
} else {
result.connectionInfo_ = connectionInfoBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.NodeHeartbeatRequest) {
return mergeFrom((org.apache.tajo.ResourceProtos.NodeHeartbeatRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.NodeHeartbeatRequest other) {
if (other == org.apache.tajo.ResourceProtos.NodeHeartbeatRequest.getDefaultInstance()) return this;
if (other.hasWorkerId()) {
setWorkerId(other.getWorkerId());
}
if (other.hasTotalResource()) {
mergeTotalResource(other.getTotalResource());
}
if (other.hasAvailableResource()) {
mergeAvailableResource(other.getAvailableResource());
}
if (other.hasRunningTasks()) {
setRunningTasks(other.getRunningTasks());
}
if (other.hasRunningQueryMasters()) {
setRunningQueryMasters(other.getRunningQueryMasters());
}
if (other.hasConnectionInfo()) {
mergeConnectionInfo(other.getConnectionInfo());
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasWorkerId()) {
return false;
}
if (hasConnectionInfo()) {
if (!getConnectionInfo().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.NodeHeartbeatRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.NodeHeartbeatRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int32 workerId = 1;
private int workerId_ ;
/**
* required int32 workerId = 1;
*/
public boolean hasWorkerId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 workerId = 1;
*/
public int getWorkerId() {
return workerId_;
}
/**
* required int32 workerId = 1;
*/
public Builder setWorkerId(int value) {
bitField0_ |= 0x00000001;
workerId_ = value;
onChanged();
return this;
}
/**
* required int32 workerId = 1;
*/
public Builder clearWorkerId() {
bitField0_ = (bitField0_ & ~0x00000001);
workerId_ = 0;
onChanged();
return this;
}
// optional .NodeResourceProto totalResource = 2;
private org.apache.tajo.TajoProtos.NodeResourceProto totalResource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.NodeResourceProto, org.apache.tajo.TajoProtos.NodeResourceProto.Builder, org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder> totalResourceBuilder_;
/**
* optional .NodeResourceProto totalResource = 2;
*/
public boolean hasTotalResource() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .NodeResourceProto totalResource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProto getTotalResource() {
if (totalResourceBuilder_ == null) {
return totalResource_;
} else {
return totalResourceBuilder_.getMessage();
}
}
/**
* optional .NodeResourceProto totalResource = 2;
*/
public Builder setTotalResource(org.apache.tajo.TajoProtos.NodeResourceProto value) {
if (totalResourceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
totalResource_ = value;
onChanged();
} else {
totalResourceBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .NodeResourceProto totalResource = 2;
*/
public Builder setTotalResource(
org.apache.tajo.TajoProtos.NodeResourceProto.Builder builderForValue) {
if (totalResourceBuilder_ == null) {
totalResource_ = builderForValue.build();
onChanged();
} else {
totalResourceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .NodeResourceProto totalResource = 2;
*/
public Builder mergeTotalResource(org.apache.tajo.TajoProtos.NodeResourceProto value) {
if (totalResourceBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
totalResource_ != org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance()) {
totalResource_ =
org.apache.tajo.TajoProtos.NodeResourceProto.newBuilder(totalResource_).mergeFrom(value).buildPartial();
} else {
totalResource_ = value;
}
onChanged();
} else {
totalResourceBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .NodeResourceProto totalResource = 2;
*/
public Builder clearTotalResource() {
if (totalResourceBuilder_ == null) {
totalResource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
onChanged();
} else {
totalResourceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .NodeResourceProto totalResource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProto.Builder getTotalResourceBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getTotalResourceFieldBuilder().getBuilder();
}
/**
* optional .NodeResourceProto totalResource = 2;
*/
public org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder getTotalResourceOrBuilder() {
if (totalResourceBuilder_ != null) {
return totalResourceBuilder_.getMessageOrBuilder();
} else {
return totalResource_;
}
}
/**
* optional .NodeResourceProto totalResource = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.NodeResourceProto, org.apache.tajo.TajoProtos.NodeResourceProto.Builder, org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder>
getTotalResourceFieldBuilder() {
if (totalResourceBuilder_ == null) {
totalResourceBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.NodeResourceProto, org.apache.tajo.TajoProtos.NodeResourceProto.Builder, org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder>(
totalResource_,
getParentForChildren(),
isClean());
totalResource_ = null;
}
return totalResourceBuilder_;
}
// optional .NodeResourceProto availableResource = 3;
private org.apache.tajo.TajoProtos.NodeResourceProto availableResource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.NodeResourceProto, org.apache.tajo.TajoProtos.NodeResourceProto.Builder, org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder> availableResourceBuilder_;
/**
* optional .NodeResourceProto availableResource = 3;
*/
public boolean hasAvailableResource() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .NodeResourceProto availableResource = 3;
*/
public org.apache.tajo.TajoProtos.NodeResourceProto getAvailableResource() {
if (availableResourceBuilder_ == null) {
return availableResource_;
} else {
return availableResourceBuilder_.getMessage();
}
}
/**
* optional .NodeResourceProto availableResource = 3;
*/
public Builder setAvailableResource(org.apache.tajo.TajoProtos.NodeResourceProto value) {
if (availableResourceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
availableResource_ = value;
onChanged();
} else {
availableResourceBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .NodeResourceProto availableResource = 3;
*/
public Builder setAvailableResource(
org.apache.tajo.TajoProtos.NodeResourceProto.Builder builderForValue) {
if (availableResourceBuilder_ == null) {
availableResource_ = builderForValue.build();
onChanged();
} else {
availableResourceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .NodeResourceProto availableResource = 3;
*/
public Builder mergeAvailableResource(org.apache.tajo.TajoProtos.NodeResourceProto value) {
if (availableResourceBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
availableResource_ != org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance()) {
availableResource_ =
org.apache.tajo.TajoProtos.NodeResourceProto.newBuilder(availableResource_).mergeFrom(value).buildPartial();
} else {
availableResource_ = value;
}
onChanged();
} else {
availableResourceBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .NodeResourceProto availableResource = 3;
*/
public Builder clearAvailableResource() {
if (availableResourceBuilder_ == null) {
availableResource_ = org.apache.tajo.TajoProtos.NodeResourceProto.getDefaultInstance();
onChanged();
} else {
availableResourceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .NodeResourceProto availableResource = 3;
*/
public org.apache.tajo.TajoProtos.NodeResourceProto.Builder getAvailableResourceBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getAvailableResourceFieldBuilder().getBuilder();
}
/**
* optional .NodeResourceProto availableResource = 3;
*/
public org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder getAvailableResourceOrBuilder() {
if (availableResourceBuilder_ != null) {
return availableResourceBuilder_.getMessageOrBuilder();
} else {
return availableResource_;
}
}
/**
* optional .NodeResourceProto availableResource = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.NodeResourceProto, org.apache.tajo.TajoProtos.NodeResourceProto.Builder, org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder>
getAvailableResourceFieldBuilder() {
if (availableResourceBuilder_ == null) {
availableResourceBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.NodeResourceProto, org.apache.tajo.TajoProtos.NodeResourceProto.Builder, org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder>(
availableResource_,
getParentForChildren(),
isClean());
availableResource_ = null;
}
return availableResourceBuilder_;
}
// optional int32 runningTasks = 4;
private int runningTasks_ ;
/**
* optional int32 runningTasks = 4;
*/
public boolean hasRunningTasks() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int32 runningTasks = 4;
*/
public int getRunningTasks() {
return runningTasks_;
}
/**
* optional int32 runningTasks = 4;
*/
public Builder setRunningTasks(int value) {
bitField0_ |= 0x00000008;
runningTasks_ = value;
onChanged();
return this;
}
/**
* optional int32 runningTasks = 4;
*/
public Builder clearRunningTasks() {
bitField0_ = (bitField0_ & ~0x00000008);
runningTasks_ = 0;
onChanged();
return this;
}
// optional int32 runningQueryMasters = 5;
private int runningQueryMasters_ ;
/**
* optional int32 runningQueryMasters = 5;
*/
public boolean hasRunningQueryMasters() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 runningQueryMasters = 5;
*/
public int getRunningQueryMasters() {
return runningQueryMasters_;
}
/**
* optional int32 runningQueryMasters = 5;
*/
public Builder setRunningQueryMasters(int value) {
bitField0_ |= 0x00000010;
runningQueryMasters_ = value;
onChanged();
return this;
}
/**
* optional int32 runningQueryMasters = 5;
*/
public Builder clearRunningQueryMasters() {
bitField0_ = (bitField0_ & ~0x00000010);
runningQueryMasters_ = 0;
onChanged();
return this;
}
// optional .WorkerConnectionInfoProto connectionInfo = 6;
private org.apache.tajo.TajoProtos.WorkerConnectionInfoProto connectionInfo_ = org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder, org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder> connectionInfoBuilder_;
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
public boolean hasConnectionInfo() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProto getConnectionInfo() {
if (connectionInfoBuilder_ == null) {
return connectionInfo_;
} else {
return connectionInfoBuilder_.getMessage();
}
}
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
public Builder setConnectionInfo(org.apache.tajo.TajoProtos.WorkerConnectionInfoProto value) {
if (connectionInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
connectionInfo_ = value;
onChanged();
} else {
connectionInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
public Builder setConnectionInfo(
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder builderForValue) {
if (connectionInfoBuilder_ == null) {
connectionInfo_ = builderForValue.build();
onChanged();
} else {
connectionInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
public Builder mergeConnectionInfo(org.apache.tajo.TajoProtos.WorkerConnectionInfoProto value) {
if (connectionInfoBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020) &&
connectionInfo_ != org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.getDefaultInstance()) {
connectionInfo_ =
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.newBuilder(connectionInfo_).mergeFrom(value).buildPartial();
} else {
connectionInfo_ = value;
}
onChanged();
} else {
connectionInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
public Builder clearConnectionInfo() {
if (connectionInfoBuilder_ == null) {
connectionInfo_ = org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.getDefaultInstance();
onChanged();
} else {
connectionInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder getConnectionInfoBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getConnectionInfoFieldBuilder().getBuilder();
}
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder getConnectionInfoOrBuilder() {
if (connectionInfoBuilder_ != null) {
return connectionInfoBuilder_.getMessageOrBuilder();
} else {
return connectionInfo_;
}
}
/**
* optional .WorkerConnectionInfoProto connectionInfo = 6;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder, org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder>
getConnectionInfoFieldBuilder() {
if (connectionInfoBuilder_ == null) {
connectionInfoBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder, org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder>(
connectionInfo_,
getParentForChildren(),
isClean());
connectionInfo_ = null;
}
return connectionInfoBuilder_;
}
// optional .NodeStatusProto status = 7;
private org.apache.tajo.ResourceProtos.NodeStatusProto status_ = org.apache.tajo.ResourceProtos.NodeStatusProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.ResourceProtos.NodeStatusProto, org.apache.tajo.ResourceProtos.NodeStatusProto.Builder, org.apache.tajo.ResourceProtos.NodeStatusProtoOrBuilder> statusBuilder_;
/**
* optional .NodeStatusProto status = 7;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .NodeStatusProto status = 7;
*/
public org.apache.tajo.ResourceProtos.NodeStatusProto getStatus() {
if (statusBuilder_ == null) {
return status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* optional .NodeStatusProto status = 7;
*/
public Builder setStatus(org.apache.tajo.ResourceProtos.NodeStatusProto value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .NodeStatusProto status = 7;
*/
public Builder setStatus(
org.apache.tajo.ResourceProtos.NodeStatusProto.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .NodeStatusProto status = 7;
*/
public Builder mergeStatus(org.apache.tajo.ResourceProtos.NodeStatusProto value) {
if (statusBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040) &&
status_ != org.apache.tajo.ResourceProtos.NodeStatusProto.getDefaultInstance()) {
status_ =
org.apache.tajo.ResourceProtos.NodeStatusProto.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .NodeStatusProto status = 7;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = org.apache.tajo.ResourceProtos.NodeStatusProto.getDefaultInstance();
onChanged();
} else {
statusBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
/**
* optional .NodeStatusProto status = 7;
*/
public org.apache.tajo.ResourceProtos.NodeStatusProto.Builder getStatusBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* optional .NodeStatusProto status = 7;
*/
public org.apache.tajo.ResourceProtos.NodeStatusProtoOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_;
}
}
/**
* optional .NodeStatusProto status = 7;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.ResourceProtos.NodeStatusProto, org.apache.tajo.ResourceProtos.NodeStatusProto.Builder, org.apache.tajo.ResourceProtos.NodeStatusProtoOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.ResourceProtos.NodeStatusProto, org.apache.tajo.ResourceProtos.NodeStatusProto.Builder, org.apache.tajo.ResourceProtos.NodeStatusProtoOrBuilder>(
status_,
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
// @@protoc_insertion_point(builder_scope:NodeHeartbeatRequest)
}
static {
defaultInstance = new NodeHeartbeatRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:NodeHeartbeatRequest)
}
public interface NodeHeartbeatResponseOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required .ResponseCommand command = 1 [default = NORMAL];
/**
* required .ResponseCommand command = 1 [default = NORMAL];
*/
boolean hasCommand();
/**
* required .ResponseCommand command = 1 [default = NORMAL];
*/
org.apache.tajo.ResourceProtos.ResponseCommand getCommand();
// optional int32 heartBeatInterval = 2;
/**
* optional int32 heartBeatInterval = 2;
*/
boolean hasHeartBeatInterval();
/**
* optional int32 heartBeatInterval = 2;
*/
int getHeartBeatInterval();
// repeated .QueryIdProto queryId = 3;
/**
* repeated .QueryIdProto queryId = 3;
*/
java.util.List
getQueryIdList();
/**
* repeated .QueryIdProto queryId = 3;
*/
org.apache.tajo.TajoIdProtos.QueryIdProto getQueryId(int index);
/**
* repeated .QueryIdProto queryId = 3;
*/
int getQueryIdCount();
/**
* repeated .QueryIdProto queryId = 3;
*/
java.util.List extends org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder>
getQueryIdOrBuilderList();
/**
* repeated .QueryIdProto queryId = 3;
*/
org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder getQueryIdOrBuilder(
int index);
}
/**
* Protobuf type {@code NodeHeartbeatResponse}
*/
public static final class NodeHeartbeatResponse extends
com.google.protobuf.GeneratedMessage
implements NodeHeartbeatResponseOrBuilder {
// Use NodeHeartbeatResponse.newBuilder() to construct.
private NodeHeartbeatResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private NodeHeartbeatResponse(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final NodeHeartbeatResponse defaultInstance;
public static NodeHeartbeatResponse getDefaultInstance() {
return defaultInstance;
}
public NodeHeartbeatResponse getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NodeHeartbeatResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
org.apache.tajo.ResourceProtos.ResponseCommand value = org.apache.tajo.ResourceProtos.ResponseCommand.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
command_ = value;
}
break;
}
case 16: {
bitField0_ |= 0x00000002;
heartBeatInterval_ = input.readInt32();
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
queryId_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
queryId_.add(input.readMessage(org.apache.tajo.TajoIdProtos.QueryIdProto.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
queryId_ = java.util.Collections.unmodifiableList(queryId_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_NodeHeartbeatResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_NodeHeartbeatResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.NodeHeartbeatResponse.class, org.apache.tajo.ResourceProtos.NodeHeartbeatResponse.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public NodeHeartbeatResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NodeHeartbeatResponse(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .ResponseCommand command = 1 [default = NORMAL];
public static final int COMMAND_FIELD_NUMBER = 1;
private org.apache.tajo.ResourceProtos.ResponseCommand command_;
/**
* required .ResponseCommand command = 1 [default = NORMAL];
*/
public boolean hasCommand() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .ResponseCommand command = 1 [default = NORMAL];
*/
public org.apache.tajo.ResourceProtos.ResponseCommand getCommand() {
return command_;
}
// optional int32 heartBeatInterval = 2;
public static final int HEARTBEATINTERVAL_FIELD_NUMBER = 2;
private int heartBeatInterval_;
/**
* optional int32 heartBeatInterval = 2;
*/
public boolean hasHeartBeatInterval() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 heartBeatInterval = 2;
*/
public int getHeartBeatInterval() {
return heartBeatInterval_;
}
// repeated .QueryIdProto queryId = 3;
public static final int QUERYID_FIELD_NUMBER = 3;
private java.util.List queryId_;
/**
* repeated .QueryIdProto queryId = 3;
*/
public java.util.List getQueryIdList() {
return queryId_;
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public java.util.List extends org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder>
getQueryIdOrBuilderList() {
return queryId_;
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public int getQueryIdCount() {
return queryId_.size();
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public org.apache.tajo.TajoIdProtos.QueryIdProto getQueryId(int index) {
return queryId_.get(index);
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder getQueryIdOrBuilder(
int index) {
return queryId_.get(index);
}
private void initFields() {
command_ = org.apache.tajo.ResourceProtos.ResponseCommand.NORMAL;
heartBeatInterval_ = 0;
queryId_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCommand()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getQueryIdCount(); i++) {
if (!getQueryId(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, command_.getNumber());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, heartBeatInterval_);
}
for (int i = 0; i < queryId_.size(); i++) {
output.writeMessage(3, queryId_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, command_.getNumber());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, heartBeatInterval_);
}
for (int i = 0; i < queryId_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, queryId_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.NodeHeartbeatResponse)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.NodeHeartbeatResponse other = (org.apache.tajo.ResourceProtos.NodeHeartbeatResponse) obj;
boolean result = true;
result = result && (hasCommand() == other.hasCommand());
if (hasCommand()) {
result = result &&
(getCommand() == other.getCommand());
}
result = result && (hasHeartBeatInterval() == other.hasHeartBeatInterval());
if (hasHeartBeatInterval()) {
result = result && (getHeartBeatInterval()
== other.getHeartBeatInterval());
}
result = result && getQueryIdList()
.equals(other.getQueryIdList());
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasCommand()) {
hash = (37 * hash) + COMMAND_FIELD_NUMBER;
hash = (53 * hash) + hashEnum(getCommand());
}
if (hasHeartBeatInterval()) {
hash = (37 * hash) + HEARTBEATINTERVAL_FIELD_NUMBER;
hash = (53 * hash) + getHeartBeatInterval();
}
if (getQueryIdCount() > 0) {
hash = (37 * hash) + QUERYID_FIELD_NUMBER;
hash = (53 * hash) + getQueryIdList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.NodeHeartbeatResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.NodeHeartbeatResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code NodeHeartbeatResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.NodeHeartbeatResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_NodeHeartbeatResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_NodeHeartbeatResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.NodeHeartbeatResponse.class, org.apache.tajo.ResourceProtos.NodeHeartbeatResponse.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.NodeHeartbeatResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getQueryIdFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
command_ = org.apache.tajo.ResourceProtos.ResponseCommand.NORMAL;
bitField0_ = (bitField0_ & ~0x00000001);
heartBeatInterval_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
if (queryIdBuilder_ == null) {
queryId_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
queryIdBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_NodeHeartbeatResponse_descriptor;
}
public org.apache.tajo.ResourceProtos.NodeHeartbeatResponse getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.NodeHeartbeatResponse.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.NodeHeartbeatResponse build() {
org.apache.tajo.ResourceProtos.NodeHeartbeatResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.NodeHeartbeatResponse buildPartial() {
org.apache.tajo.ResourceProtos.NodeHeartbeatResponse result = new org.apache.tajo.ResourceProtos.NodeHeartbeatResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.command_ = command_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.heartBeatInterval_ = heartBeatInterval_;
if (queryIdBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
queryId_ = java.util.Collections.unmodifiableList(queryId_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.queryId_ = queryId_;
} else {
result.queryId_ = queryIdBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.NodeHeartbeatResponse) {
return mergeFrom((org.apache.tajo.ResourceProtos.NodeHeartbeatResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.NodeHeartbeatResponse other) {
if (other == org.apache.tajo.ResourceProtos.NodeHeartbeatResponse.getDefaultInstance()) return this;
if (other.hasCommand()) {
setCommand(other.getCommand());
}
if (other.hasHeartBeatInterval()) {
setHeartBeatInterval(other.getHeartBeatInterval());
}
if (queryIdBuilder_ == null) {
if (!other.queryId_.isEmpty()) {
if (queryId_.isEmpty()) {
queryId_ = other.queryId_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureQueryIdIsMutable();
queryId_.addAll(other.queryId_);
}
onChanged();
}
} else {
if (!other.queryId_.isEmpty()) {
if (queryIdBuilder_.isEmpty()) {
queryIdBuilder_.dispose();
queryIdBuilder_ = null;
queryId_ = other.queryId_;
bitField0_ = (bitField0_ & ~0x00000004);
queryIdBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getQueryIdFieldBuilder() : null;
} else {
queryIdBuilder_.addAllMessages(other.queryId_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCommand()) {
return false;
}
for (int i = 0; i < getQueryIdCount(); i++) {
if (!getQueryId(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.NodeHeartbeatResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.NodeHeartbeatResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .ResponseCommand command = 1 [default = NORMAL];
private org.apache.tajo.ResourceProtos.ResponseCommand command_ = org.apache.tajo.ResourceProtos.ResponseCommand.NORMAL;
/**
* required .ResponseCommand command = 1 [default = NORMAL];
*/
public boolean hasCommand() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .ResponseCommand command = 1 [default = NORMAL];
*/
public org.apache.tajo.ResourceProtos.ResponseCommand getCommand() {
return command_;
}
/**
* required .ResponseCommand command = 1 [default = NORMAL];
*/
public Builder setCommand(org.apache.tajo.ResourceProtos.ResponseCommand value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
command_ = value;
onChanged();
return this;
}
/**
* required .ResponseCommand command = 1 [default = NORMAL];
*/
public Builder clearCommand() {
bitField0_ = (bitField0_ & ~0x00000001);
command_ = org.apache.tajo.ResourceProtos.ResponseCommand.NORMAL;
onChanged();
return this;
}
// optional int32 heartBeatInterval = 2;
private int heartBeatInterval_ ;
/**
* optional int32 heartBeatInterval = 2;
*/
public boolean hasHeartBeatInterval() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 heartBeatInterval = 2;
*/
public int getHeartBeatInterval() {
return heartBeatInterval_;
}
/**
* optional int32 heartBeatInterval = 2;
*/
public Builder setHeartBeatInterval(int value) {
bitField0_ |= 0x00000002;
heartBeatInterval_ = value;
onChanged();
return this;
}
/**
* optional int32 heartBeatInterval = 2;
*/
public Builder clearHeartBeatInterval() {
bitField0_ = (bitField0_ & ~0x00000002);
heartBeatInterval_ = 0;
onChanged();
return this;
}
// repeated .QueryIdProto queryId = 3;
private java.util.List queryId_ =
java.util.Collections.emptyList();
private void ensureQueryIdIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
queryId_ = new java.util.ArrayList(queryId_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.TajoIdProtos.QueryIdProto, org.apache.tajo.TajoIdProtos.QueryIdProto.Builder, org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder> queryIdBuilder_;
/**
* repeated .QueryIdProto queryId = 3;
*/
public java.util.List getQueryIdList() {
if (queryIdBuilder_ == null) {
return java.util.Collections.unmodifiableList(queryId_);
} else {
return queryIdBuilder_.getMessageList();
}
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public int getQueryIdCount() {
if (queryIdBuilder_ == null) {
return queryId_.size();
} else {
return queryIdBuilder_.getCount();
}
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public org.apache.tajo.TajoIdProtos.QueryIdProto getQueryId(int index) {
if (queryIdBuilder_ == null) {
return queryId_.get(index);
} else {
return queryIdBuilder_.getMessage(index);
}
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public Builder setQueryId(
int index, org.apache.tajo.TajoIdProtos.QueryIdProto value) {
if (queryIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryIdIsMutable();
queryId_.set(index, value);
onChanged();
} else {
queryIdBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public Builder setQueryId(
int index, org.apache.tajo.TajoIdProtos.QueryIdProto.Builder builderForValue) {
if (queryIdBuilder_ == null) {
ensureQueryIdIsMutable();
queryId_.set(index, builderForValue.build());
onChanged();
} else {
queryIdBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public Builder addQueryId(org.apache.tajo.TajoIdProtos.QueryIdProto value) {
if (queryIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryIdIsMutable();
queryId_.add(value);
onChanged();
} else {
queryIdBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public Builder addQueryId(
int index, org.apache.tajo.TajoIdProtos.QueryIdProto value) {
if (queryIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryIdIsMutable();
queryId_.add(index, value);
onChanged();
} else {
queryIdBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public Builder addQueryId(
org.apache.tajo.TajoIdProtos.QueryIdProto.Builder builderForValue) {
if (queryIdBuilder_ == null) {
ensureQueryIdIsMutable();
queryId_.add(builderForValue.build());
onChanged();
} else {
queryIdBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public Builder addQueryId(
int index, org.apache.tajo.TajoIdProtos.QueryIdProto.Builder builderForValue) {
if (queryIdBuilder_ == null) {
ensureQueryIdIsMutable();
queryId_.add(index, builderForValue.build());
onChanged();
} else {
queryIdBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public Builder addAllQueryId(
java.lang.Iterable extends org.apache.tajo.TajoIdProtos.QueryIdProto> values) {
if (queryIdBuilder_ == null) {
ensureQueryIdIsMutable();
super.addAll(values, queryId_);
onChanged();
} else {
queryIdBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public Builder clearQueryId() {
if (queryIdBuilder_ == null) {
queryId_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
queryIdBuilder_.clear();
}
return this;
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public Builder removeQueryId(int index) {
if (queryIdBuilder_ == null) {
ensureQueryIdIsMutable();
queryId_.remove(index);
onChanged();
} else {
queryIdBuilder_.remove(index);
}
return this;
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public org.apache.tajo.TajoIdProtos.QueryIdProto.Builder getQueryIdBuilder(
int index) {
return getQueryIdFieldBuilder().getBuilder(index);
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder getQueryIdOrBuilder(
int index) {
if (queryIdBuilder_ == null) {
return queryId_.get(index); } else {
return queryIdBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public java.util.List extends org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder>
getQueryIdOrBuilderList() {
if (queryIdBuilder_ != null) {
return queryIdBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(queryId_);
}
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public org.apache.tajo.TajoIdProtos.QueryIdProto.Builder addQueryIdBuilder() {
return getQueryIdFieldBuilder().addBuilder(
org.apache.tajo.TajoIdProtos.QueryIdProto.getDefaultInstance());
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public org.apache.tajo.TajoIdProtos.QueryIdProto.Builder addQueryIdBuilder(
int index) {
return getQueryIdFieldBuilder().addBuilder(
index, org.apache.tajo.TajoIdProtos.QueryIdProto.getDefaultInstance());
}
/**
* repeated .QueryIdProto queryId = 3;
*/
public java.util.List
getQueryIdBuilderList() {
return getQueryIdFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.TajoIdProtos.QueryIdProto, org.apache.tajo.TajoIdProtos.QueryIdProto.Builder, org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder>
getQueryIdFieldBuilder() {
if (queryIdBuilder_ == null) {
queryIdBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.TajoIdProtos.QueryIdProto, org.apache.tajo.TajoIdProtos.QueryIdProto.Builder, org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder>(
queryId_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
queryId_ = null;
}
return queryIdBuilder_;
}
// @@protoc_insertion_point(builder_scope:NodeHeartbeatResponse)
}
static {
defaultInstance = new NodeHeartbeatResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:NodeHeartbeatResponse)
}
public interface TajoHeartbeatRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required .WorkerConnectionInfoProto connectionInfo = 1;
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
boolean hasConnectionInfo();
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto getConnectionInfo();
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder getConnectionInfoOrBuilder();
// optional .QueryIdProto queryId = 2;
/**
* optional .QueryIdProto queryId = 2;
*/
boolean hasQueryId();
/**
* optional .QueryIdProto queryId = 2;
*/
org.apache.tajo.TajoIdProtos.QueryIdProto getQueryId();
/**
* optional .QueryIdProto queryId = 2;
*/
org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder getQueryIdOrBuilder();
// optional .QueryState state = 3;
/**
* optional .QueryState state = 3;
*/
boolean hasState();
/**
* optional .QueryState state = 3;
*/
org.apache.tajo.TajoProtos.QueryState getState();
// optional .TableDescProto result_desc = 4;
/**
* optional .TableDescProto result_desc = 4;
*/
boolean hasResultDesc();
/**
* optional .TableDescProto result_desc = 4;
*/
org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto getResultDesc();
/**
* optional .TableDescProto result_desc = 4;
*/
org.apache.tajo.catalog.proto.CatalogProtos.TableDescProtoOrBuilder getResultDescOrBuilder();
// optional .tajo.error.SerializedException error = 5;
/**
* optional .tajo.error.SerializedException error = 5;
*/
boolean hasError();
/**
* optional .tajo.error.SerializedException error = 5;
*/
org.apache.tajo.error.Errors.SerializedException getError();
/**
* optional .tajo.error.SerializedException error = 5;
*/
org.apache.tajo.error.Errors.SerializedExceptionOrBuilder getErrorOrBuilder();
// optional float query_progress = 6;
/**
* optional float query_progress = 6;
*/
boolean hasQueryProgress();
/**
* optional float query_progress = 6;
*/
float getQueryProgress();
}
/**
* Protobuf type {@code TajoHeartbeatRequest}
*
*
* deprecated
*
*/
public static final class TajoHeartbeatRequest extends
com.google.protobuf.GeneratedMessage
implements TajoHeartbeatRequestOrBuilder {
// Use TajoHeartbeatRequest.newBuilder() to construct.
private TajoHeartbeatRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TajoHeartbeatRequest(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TajoHeartbeatRequest defaultInstance;
public static TajoHeartbeatRequest getDefaultInstance() {
return defaultInstance;
}
public TajoHeartbeatRequest getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TajoHeartbeatRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = connectionInfo_.toBuilder();
}
connectionInfo_ = input.readMessage(org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(connectionInfo_);
connectionInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
org.apache.tajo.TajoIdProtos.QueryIdProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = queryId_.toBuilder();
}
queryId_ = input.readMessage(org.apache.tajo.TajoIdProtos.QueryIdProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(queryId_);
queryId_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 24: {
int rawValue = input.readEnum();
org.apache.tajo.TajoProtos.QueryState value = org.apache.tajo.TajoProtos.QueryState.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(3, rawValue);
} else {
bitField0_ |= 0x00000004;
state_ = value;
}
break;
}
case 34: {
org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = resultDesc_.toBuilder();
}
resultDesc_ = input.readMessage(org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(resultDesc_);
resultDesc_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 42: {
org.apache.tajo.error.Errors.SerializedException.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = error_.toBuilder();
}
error_ = input.readMessage(org.apache.tajo.error.Errors.SerializedException.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(error_);
error_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 53: {
bitField0_ |= 0x00000020;
queryProgress_ = input.readFloat();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TajoHeartbeatRequest.class, org.apache.tajo.ResourceProtos.TajoHeartbeatRequest.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TajoHeartbeatRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TajoHeartbeatRequest(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .WorkerConnectionInfoProto connectionInfo = 1;
public static final int CONNECTIONINFO_FIELD_NUMBER = 1;
private org.apache.tajo.TajoProtos.WorkerConnectionInfoProto connectionInfo_;
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
public boolean hasConnectionInfo() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProto getConnectionInfo() {
return connectionInfo_;
}
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder getConnectionInfoOrBuilder() {
return connectionInfo_;
}
// optional .QueryIdProto queryId = 2;
public static final int QUERYID_FIELD_NUMBER = 2;
private org.apache.tajo.TajoIdProtos.QueryIdProto queryId_;
/**
* optional .QueryIdProto queryId = 2;
*/
public boolean hasQueryId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .QueryIdProto queryId = 2;
*/
public org.apache.tajo.TajoIdProtos.QueryIdProto getQueryId() {
return queryId_;
}
/**
* optional .QueryIdProto queryId = 2;
*/
public org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder getQueryIdOrBuilder() {
return queryId_;
}
// optional .QueryState state = 3;
public static final int STATE_FIELD_NUMBER = 3;
private org.apache.tajo.TajoProtos.QueryState state_;
/**
* optional .QueryState state = 3;
*/
public boolean hasState() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .QueryState state = 3;
*/
public org.apache.tajo.TajoProtos.QueryState getState() {
return state_;
}
// optional .TableDescProto result_desc = 4;
public static final int RESULT_DESC_FIELD_NUMBER = 4;
private org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto resultDesc_;
/**
* optional .TableDescProto result_desc = 4;
*/
public boolean hasResultDesc() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .TableDescProto result_desc = 4;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto getResultDesc() {
return resultDesc_;
}
/**
* optional .TableDescProto result_desc = 4;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableDescProtoOrBuilder getResultDescOrBuilder() {
return resultDesc_;
}
// optional .tajo.error.SerializedException error = 5;
public static final int ERROR_FIELD_NUMBER = 5;
private org.apache.tajo.error.Errors.SerializedException error_;
/**
* optional .tajo.error.SerializedException error = 5;
*/
public boolean hasError() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .tajo.error.SerializedException error = 5;
*/
public org.apache.tajo.error.Errors.SerializedException getError() {
return error_;
}
/**
* optional .tajo.error.SerializedException error = 5;
*/
public org.apache.tajo.error.Errors.SerializedExceptionOrBuilder getErrorOrBuilder() {
return error_;
}
// optional float query_progress = 6;
public static final int QUERY_PROGRESS_FIELD_NUMBER = 6;
private float queryProgress_;
/**
* optional float query_progress = 6;
*/
public boolean hasQueryProgress() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional float query_progress = 6;
*/
public float getQueryProgress() {
return queryProgress_;
}
private void initFields() {
connectionInfo_ = org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.getDefaultInstance();
queryId_ = org.apache.tajo.TajoIdProtos.QueryIdProto.getDefaultInstance();
state_ = org.apache.tajo.TajoProtos.QueryState.QUERY_MASTER_INIT;
resultDesc_ = org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto.getDefaultInstance();
error_ = org.apache.tajo.error.Errors.SerializedException.getDefaultInstance();
queryProgress_ = 0F;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasConnectionInfo()) {
memoizedIsInitialized = 0;
return false;
}
if (!getConnectionInfo().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (hasQueryId()) {
if (!getQueryId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasResultDesc()) {
if (!getResultDesc().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasError()) {
if (!getError().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, connectionInfo_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, queryId_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeEnum(3, state_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, resultDesc_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(5, error_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeFloat(6, queryProgress_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, connectionInfo_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, queryId_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, state_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, resultDesc_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, error_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(6, queryProgress_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.TajoHeartbeatRequest)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.TajoHeartbeatRequest other = (org.apache.tajo.ResourceProtos.TajoHeartbeatRequest) obj;
boolean result = true;
result = result && (hasConnectionInfo() == other.hasConnectionInfo());
if (hasConnectionInfo()) {
result = result && getConnectionInfo()
.equals(other.getConnectionInfo());
}
result = result && (hasQueryId() == other.hasQueryId());
if (hasQueryId()) {
result = result && getQueryId()
.equals(other.getQueryId());
}
result = result && (hasState() == other.hasState());
if (hasState()) {
result = result &&
(getState() == other.getState());
}
result = result && (hasResultDesc() == other.hasResultDesc());
if (hasResultDesc()) {
result = result && getResultDesc()
.equals(other.getResultDesc());
}
result = result && (hasError() == other.hasError());
if (hasError()) {
result = result && getError()
.equals(other.getError());
}
result = result && (hasQueryProgress() == other.hasQueryProgress());
if (hasQueryProgress()) {
result = result && (Float.floatToIntBits(getQueryProgress()) == Float.floatToIntBits(other.getQueryProgress()));
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasConnectionInfo()) {
hash = (37 * hash) + CONNECTIONINFO_FIELD_NUMBER;
hash = (53 * hash) + getConnectionInfo().hashCode();
}
if (hasQueryId()) {
hash = (37 * hash) + QUERYID_FIELD_NUMBER;
hash = (53 * hash) + getQueryId().hashCode();
}
if (hasState()) {
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + hashEnum(getState());
}
if (hasResultDesc()) {
hash = (37 * hash) + RESULT_DESC_FIELD_NUMBER;
hash = (53 * hash) + getResultDesc().hashCode();
}
if (hasError()) {
hash = (37 * hash) + ERROR_FIELD_NUMBER;
hash = (53 * hash) + getError().hashCode();
}
if (hasQueryProgress()) {
hash = (37 * hash) + QUERY_PROGRESS_FIELD_NUMBER;
hash = (53 * hash) + Float.floatToIntBits(
getQueryProgress());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.TajoHeartbeatRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TajoHeartbeatRequest}
*
*
* deprecated
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.TajoHeartbeatRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TajoHeartbeatRequest.class, org.apache.tajo.ResourceProtos.TajoHeartbeatRequest.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.TajoHeartbeatRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getConnectionInfoFieldBuilder();
getQueryIdFieldBuilder();
getResultDescFieldBuilder();
getErrorFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (connectionInfoBuilder_ == null) {
connectionInfo_ = org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.getDefaultInstance();
} else {
connectionInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (queryIdBuilder_ == null) {
queryId_ = org.apache.tajo.TajoIdProtos.QueryIdProto.getDefaultInstance();
} else {
queryIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
state_ = org.apache.tajo.TajoProtos.QueryState.QUERY_MASTER_INIT;
bitField0_ = (bitField0_ & ~0x00000004);
if (resultDescBuilder_ == null) {
resultDesc_ = org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto.getDefaultInstance();
} else {
resultDescBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
if (errorBuilder_ == null) {
error_ = org.apache.tajo.error.Errors.SerializedException.getDefaultInstance();
} else {
errorBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
queryProgress_ = 0F;
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatRequest_descriptor;
}
public org.apache.tajo.ResourceProtos.TajoHeartbeatRequest getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.TajoHeartbeatRequest.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.TajoHeartbeatRequest build() {
org.apache.tajo.ResourceProtos.TajoHeartbeatRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.TajoHeartbeatRequest buildPartial() {
org.apache.tajo.ResourceProtos.TajoHeartbeatRequest result = new org.apache.tajo.ResourceProtos.TajoHeartbeatRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (connectionInfoBuilder_ == null) {
result.connectionInfo_ = connectionInfo_;
} else {
result.connectionInfo_ = connectionInfoBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (queryIdBuilder_ == null) {
result.queryId_ = queryId_;
} else {
result.queryId_ = queryIdBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.state_ = state_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (resultDescBuilder_ == null) {
result.resultDesc_ = resultDesc_;
} else {
result.resultDesc_ = resultDescBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (errorBuilder_ == null) {
result.error_ = error_;
} else {
result.error_ = errorBuilder_.build();
}
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.queryProgress_ = queryProgress_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.TajoHeartbeatRequest) {
return mergeFrom((org.apache.tajo.ResourceProtos.TajoHeartbeatRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.TajoHeartbeatRequest other) {
if (other == org.apache.tajo.ResourceProtos.TajoHeartbeatRequest.getDefaultInstance()) return this;
if (other.hasConnectionInfo()) {
mergeConnectionInfo(other.getConnectionInfo());
}
if (other.hasQueryId()) {
mergeQueryId(other.getQueryId());
}
if (other.hasState()) {
setState(other.getState());
}
if (other.hasResultDesc()) {
mergeResultDesc(other.getResultDesc());
}
if (other.hasError()) {
mergeError(other.getError());
}
if (other.hasQueryProgress()) {
setQueryProgress(other.getQueryProgress());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasConnectionInfo()) {
return false;
}
if (!getConnectionInfo().isInitialized()) {
return false;
}
if (hasQueryId()) {
if (!getQueryId().isInitialized()) {
return false;
}
}
if (hasResultDesc()) {
if (!getResultDesc().isInitialized()) {
return false;
}
}
if (hasError()) {
if (!getError().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.TajoHeartbeatRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.TajoHeartbeatRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .WorkerConnectionInfoProto connectionInfo = 1;
private org.apache.tajo.TajoProtos.WorkerConnectionInfoProto connectionInfo_ = org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder, org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder> connectionInfoBuilder_;
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
public boolean hasConnectionInfo() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProto getConnectionInfo() {
if (connectionInfoBuilder_ == null) {
return connectionInfo_;
} else {
return connectionInfoBuilder_.getMessage();
}
}
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
public Builder setConnectionInfo(org.apache.tajo.TajoProtos.WorkerConnectionInfoProto value) {
if (connectionInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
connectionInfo_ = value;
onChanged();
} else {
connectionInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
public Builder setConnectionInfo(
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder builderForValue) {
if (connectionInfoBuilder_ == null) {
connectionInfo_ = builderForValue.build();
onChanged();
} else {
connectionInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
public Builder mergeConnectionInfo(org.apache.tajo.TajoProtos.WorkerConnectionInfoProto value) {
if (connectionInfoBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
connectionInfo_ != org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.getDefaultInstance()) {
connectionInfo_ =
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.newBuilder(connectionInfo_).mergeFrom(value).buildPartial();
} else {
connectionInfo_ = value;
}
onChanged();
} else {
connectionInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
public Builder clearConnectionInfo() {
if (connectionInfoBuilder_ == null) {
connectionInfo_ = org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.getDefaultInstance();
onChanged();
} else {
connectionInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder getConnectionInfoBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getConnectionInfoFieldBuilder().getBuilder();
}
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder getConnectionInfoOrBuilder() {
if (connectionInfoBuilder_ != null) {
return connectionInfoBuilder_.getMessageOrBuilder();
} else {
return connectionInfo_;
}
}
/**
* required .WorkerConnectionInfoProto connectionInfo = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder, org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder>
getConnectionInfoFieldBuilder() {
if (connectionInfoBuilder_ == null) {
connectionInfoBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder, org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder>(
connectionInfo_,
getParentForChildren(),
isClean());
connectionInfo_ = null;
}
return connectionInfoBuilder_;
}
// optional .QueryIdProto queryId = 2;
private org.apache.tajo.TajoIdProtos.QueryIdProto queryId_ = org.apache.tajo.TajoIdProtos.QueryIdProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.QueryIdProto, org.apache.tajo.TajoIdProtos.QueryIdProto.Builder, org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder> queryIdBuilder_;
/**
* optional .QueryIdProto queryId = 2;
*/
public boolean hasQueryId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .QueryIdProto queryId = 2;
*/
public org.apache.tajo.TajoIdProtos.QueryIdProto getQueryId() {
if (queryIdBuilder_ == null) {
return queryId_;
} else {
return queryIdBuilder_.getMessage();
}
}
/**
* optional .QueryIdProto queryId = 2;
*/
public Builder setQueryId(org.apache.tajo.TajoIdProtos.QueryIdProto value) {
if (queryIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
queryId_ = value;
onChanged();
} else {
queryIdBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .QueryIdProto queryId = 2;
*/
public Builder setQueryId(
org.apache.tajo.TajoIdProtos.QueryIdProto.Builder builderForValue) {
if (queryIdBuilder_ == null) {
queryId_ = builderForValue.build();
onChanged();
} else {
queryIdBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .QueryIdProto queryId = 2;
*/
public Builder mergeQueryId(org.apache.tajo.TajoIdProtos.QueryIdProto value) {
if (queryIdBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
queryId_ != org.apache.tajo.TajoIdProtos.QueryIdProto.getDefaultInstance()) {
queryId_ =
org.apache.tajo.TajoIdProtos.QueryIdProto.newBuilder(queryId_).mergeFrom(value).buildPartial();
} else {
queryId_ = value;
}
onChanged();
} else {
queryIdBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .QueryIdProto queryId = 2;
*/
public Builder clearQueryId() {
if (queryIdBuilder_ == null) {
queryId_ = org.apache.tajo.TajoIdProtos.QueryIdProto.getDefaultInstance();
onChanged();
} else {
queryIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .QueryIdProto queryId = 2;
*/
public org.apache.tajo.TajoIdProtos.QueryIdProto.Builder getQueryIdBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getQueryIdFieldBuilder().getBuilder();
}
/**
* optional .QueryIdProto queryId = 2;
*/
public org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder getQueryIdOrBuilder() {
if (queryIdBuilder_ != null) {
return queryIdBuilder_.getMessageOrBuilder();
} else {
return queryId_;
}
}
/**
* optional .QueryIdProto queryId = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.QueryIdProto, org.apache.tajo.TajoIdProtos.QueryIdProto.Builder, org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder>
getQueryIdFieldBuilder() {
if (queryIdBuilder_ == null) {
queryIdBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.TajoIdProtos.QueryIdProto, org.apache.tajo.TajoIdProtos.QueryIdProto.Builder, org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder>(
queryId_,
getParentForChildren(),
isClean());
queryId_ = null;
}
return queryIdBuilder_;
}
// optional .QueryState state = 3;
private org.apache.tajo.TajoProtos.QueryState state_ = org.apache.tajo.TajoProtos.QueryState.QUERY_MASTER_INIT;
/**
* optional .QueryState state = 3;
*/
public boolean hasState() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .QueryState state = 3;
*/
public org.apache.tajo.TajoProtos.QueryState getState() {
return state_;
}
/**
* optional .QueryState state = 3;
*/
public Builder setState(org.apache.tajo.TajoProtos.QueryState value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
state_ = value;
onChanged();
return this;
}
/**
* optional .QueryState state = 3;
*/
public Builder clearState() {
bitField0_ = (bitField0_ & ~0x00000004);
state_ = org.apache.tajo.TajoProtos.QueryState.QUERY_MASTER_INIT;
onChanged();
return this;
}
// optional .TableDescProto result_desc = 4;
private org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto resultDesc_ = org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto, org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableDescProtoOrBuilder> resultDescBuilder_;
/**
* optional .TableDescProto result_desc = 4;
*/
public boolean hasResultDesc() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .TableDescProto result_desc = 4;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto getResultDesc() {
if (resultDescBuilder_ == null) {
return resultDesc_;
} else {
return resultDescBuilder_.getMessage();
}
}
/**
* optional .TableDescProto result_desc = 4;
*/
public Builder setResultDesc(org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto value) {
if (resultDescBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resultDesc_ = value;
onChanged();
} else {
resultDescBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .TableDescProto result_desc = 4;
*/
public Builder setResultDesc(
org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto.Builder builderForValue) {
if (resultDescBuilder_ == null) {
resultDesc_ = builderForValue.build();
onChanged();
} else {
resultDescBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .TableDescProto result_desc = 4;
*/
public Builder mergeResultDesc(org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto value) {
if (resultDescBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
resultDesc_ != org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto.getDefaultInstance()) {
resultDesc_ =
org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto.newBuilder(resultDesc_).mergeFrom(value).buildPartial();
} else {
resultDesc_ = value;
}
onChanged();
} else {
resultDescBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .TableDescProto result_desc = 4;
*/
public Builder clearResultDesc() {
if (resultDescBuilder_ == null) {
resultDesc_ = org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto.getDefaultInstance();
onChanged();
} else {
resultDescBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* optional .TableDescProto result_desc = 4;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto.Builder getResultDescBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getResultDescFieldBuilder().getBuilder();
}
/**
* optional .TableDescProto result_desc = 4;
*/
public org.apache.tajo.catalog.proto.CatalogProtos.TableDescProtoOrBuilder getResultDescOrBuilder() {
if (resultDescBuilder_ != null) {
return resultDescBuilder_.getMessageOrBuilder();
} else {
return resultDesc_;
}
}
/**
* optional .TableDescProto result_desc = 4;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto, org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableDescProtoOrBuilder>
getResultDescFieldBuilder() {
if (resultDescBuilder_ == null) {
resultDescBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto, org.apache.tajo.catalog.proto.CatalogProtos.TableDescProto.Builder, org.apache.tajo.catalog.proto.CatalogProtos.TableDescProtoOrBuilder>(
resultDesc_,
getParentForChildren(),
isClean());
resultDesc_ = null;
}
return resultDescBuilder_;
}
// optional .tajo.error.SerializedException error = 5;
private org.apache.tajo.error.Errors.SerializedException error_ = org.apache.tajo.error.Errors.SerializedException.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.error.Errors.SerializedException, org.apache.tajo.error.Errors.SerializedException.Builder, org.apache.tajo.error.Errors.SerializedExceptionOrBuilder> errorBuilder_;
/**
* optional .tajo.error.SerializedException error = 5;
*/
public boolean hasError() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .tajo.error.SerializedException error = 5;
*/
public org.apache.tajo.error.Errors.SerializedException getError() {
if (errorBuilder_ == null) {
return error_;
} else {
return errorBuilder_.getMessage();
}
}
/**
* optional .tajo.error.SerializedException error = 5;
*/
public Builder setError(org.apache.tajo.error.Errors.SerializedException value) {
if (errorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
error_ = value;
onChanged();
} else {
errorBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .tajo.error.SerializedException error = 5;
*/
public Builder setError(
org.apache.tajo.error.Errors.SerializedException.Builder builderForValue) {
if (errorBuilder_ == null) {
error_ = builderForValue.build();
onChanged();
} else {
errorBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .tajo.error.SerializedException error = 5;
*/
public Builder mergeError(org.apache.tajo.error.Errors.SerializedException value) {
if (errorBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
error_ != org.apache.tajo.error.Errors.SerializedException.getDefaultInstance()) {
error_ =
org.apache.tajo.error.Errors.SerializedException.newBuilder(error_).mergeFrom(value).buildPartial();
} else {
error_ = value;
}
onChanged();
} else {
errorBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .tajo.error.SerializedException error = 5;
*/
public Builder clearError() {
if (errorBuilder_ == null) {
error_ = org.apache.tajo.error.Errors.SerializedException.getDefaultInstance();
onChanged();
} else {
errorBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* optional .tajo.error.SerializedException error = 5;
*/
public org.apache.tajo.error.Errors.SerializedException.Builder getErrorBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getErrorFieldBuilder().getBuilder();
}
/**
* optional .tajo.error.SerializedException error = 5;
*/
public org.apache.tajo.error.Errors.SerializedExceptionOrBuilder getErrorOrBuilder() {
if (errorBuilder_ != null) {
return errorBuilder_.getMessageOrBuilder();
} else {
return error_;
}
}
/**
* optional .tajo.error.SerializedException error = 5;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.error.Errors.SerializedException, org.apache.tajo.error.Errors.SerializedException.Builder, org.apache.tajo.error.Errors.SerializedExceptionOrBuilder>
getErrorFieldBuilder() {
if (errorBuilder_ == null) {
errorBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.error.Errors.SerializedException, org.apache.tajo.error.Errors.SerializedException.Builder, org.apache.tajo.error.Errors.SerializedExceptionOrBuilder>(
error_,
getParentForChildren(),
isClean());
error_ = null;
}
return errorBuilder_;
}
// optional float query_progress = 6;
private float queryProgress_ ;
/**
* optional float query_progress = 6;
*/
public boolean hasQueryProgress() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional float query_progress = 6;
*/
public float getQueryProgress() {
return queryProgress_;
}
/**
* optional float query_progress = 6;
*/
public Builder setQueryProgress(float value) {
bitField0_ |= 0x00000020;
queryProgress_ = value;
onChanged();
return this;
}
/**
* optional float query_progress = 6;
*/
public Builder clearQueryProgress() {
bitField0_ = (bitField0_ & ~0x00000020);
queryProgress_ = 0F;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:TajoHeartbeatRequest)
}
static {
defaultInstance = new TajoHeartbeatRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TajoHeartbeatRequest)
}
public interface TajoHeartbeatResponseOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required .BoolProto heartbeatResult = 1;
/**
* required .BoolProto heartbeatResult = 1;
*/
boolean hasHeartbeatResult();
/**
* required .BoolProto heartbeatResult = 1;
*/
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto getHeartbeatResult();
/**
* required .BoolProto heartbeatResult = 1;
*/
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProtoOrBuilder getHeartbeatResultOrBuilder();
// optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
boolean hasResponseCommand();
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand getResponseCommand();
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommandOrBuilder getResponseCommandOrBuilder();
}
/**
* Protobuf type {@code TajoHeartbeatResponse}
*
*
* deprecated
*
*/
public static final class TajoHeartbeatResponse extends
com.google.protobuf.GeneratedMessage
implements TajoHeartbeatResponseOrBuilder {
// Use TajoHeartbeatResponse.newBuilder() to construct.
private TajoHeartbeatResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TajoHeartbeatResponse(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TajoHeartbeatResponse defaultInstance;
public static TajoHeartbeatResponse getDefaultInstance() {
return defaultInstance;
}
public TajoHeartbeatResponse getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TajoHeartbeatResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = heartbeatResult_.toBuilder();
}
heartbeatResult_ = input.readMessage(org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(heartbeatResult_);
heartbeatResult_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 26: {
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = responseCommand_.toBuilder();
}
responseCommand_ = input.readMessage(org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(responseCommand_);
responseCommand_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.class, org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TajoHeartbeatResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TajoHeartbeatResponse(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public interface ResponseCommandOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string command = 1;
/**
* required string command = 1;
*/
boolean hasCommand();
/**
* required string command = 1;
*/
java.lang.String getCommand();
/**
* required string command = 1;
*/
com.google.protobuf.ByteString
getCommandBytes();
// repeated string params = 2;
/**
* repeated string params = 2;
*/
java.util.List
getParamsList();
/**
* repeated string params = 2;
*/
int getParamsCount();
/**
* repeated string params = 2;
*/
java.lang.String getParams(int index);
/**
* repeated string params = 2;
*/
com.google.protobuf.ByteString
getParamsBytes(int index);
}
/**
* Protobuf type {@code TajoHeartbeatResponse.ResponseCommand}
*/
public static final class ResponseCommand extends
com.google.protobuf.GeneratedMessage
implements ResponseCommandOrBuilder {
// Use ResponseCommand.newBuilder() to construct.
private ResponseCommand(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ResponseCommand(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ResponseCommand defaultInstance;
public static ResponseCommand getDefaultInstance() {
return defaultInstance;
}
public ResponseCommand getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ResponseCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
command_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
params_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
params_.add(input.readBytes());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
params_ = new com.google.protobuf.UnmodifiableLazyStringList(params_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatResponse_ResponseCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatResponse_ResponseCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.class, org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ResponseCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ResponseCommand(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string command = 1;
public static final int COMMAND_FIELD_NUMBER = 1;
private java.lang.Object command_;
/**
* required string command = 1;
*/
public boolean hasCommand() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string command = 1;
*/
public java.lang.String getCommand() {
java.lang.Object ref = command_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
command_ = s;
}
return s;
}
}
/**
* required string command = 1;
*/
public com.google.protobuf.ByteString
getCommandBytes() {
java.lang.Object ref = command_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
command_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// repeated string params = 2;
public static final int PARAMS_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList params_;
/**
* repeated string params = 2;
*/
public java.util.List
getParamsList() {
return params_;
}
/**
* repeated string params = 2;
*/
public int getParamsCount() {
return params_.size();
}
/**
* repeated string params = 2;
*/
public java.lang.String getParams(int index) {
return params_.get(index);
}
/**
* repeated string params = 2;
*/
public com.google.protobuf.ByteString
getParamsBytes(int index) {
return params_.getByteString(index);
}
private void initFields() {
command_ = "";
params_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCommand()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getCommandBytes());
}
for (int i = 0; i < params_.size(); i++) {
output.writeBytes(2, params_.getByteString(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getCommandBytes());
}
{
int dataSize = 0;
for (int i = 0; i < params_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(params_.getByteString(i));
}
size += dataSize;
size += 1 * getParamsList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand other = (org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand) obj;
boolean result = true;
result = result && (hasCommand() == other.hasCommand());
if (hasCommand()) {
result = result && getCommand()
.equals(other.getCommand());
}
result = result && getParamsList()
.equals(other.getParamsList());
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasCommand()) {
hash = (37 * hash) + COMMAND_FIELD_NUMBER;
hash = (53 * hash) + getCommand().hashCode();
}
if (getParamsCount() > 0) {
hash = (37 * hash) + PARAMS_FIELD_NUMBER;
hash = (53 * hash) + getParamsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TajoHeartbeatResponse.ResponseCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatResponse_ResponseCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatResponse_ResponseCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.class, org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
command_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
params_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatResponse_ResponseCommand_descriptor;
}
public org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand build() {
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand buildPartial() {
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand result = new org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.command_ = command_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
params_ = new com.google.protobuf.UnmodifiableLazyStringList(
params_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.params_ = params_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand) {
return mergeFrom((org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand other) {
if (other == org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.getDefaultInstance()) return this;
if (other.hasCommand()) {
bitField0_ |= 0x00000001;
command_ = other.command_;
onChanged();
}
if (!other.params_.isEmpty()) {
if (params_.isEmpty()) {
params_ = other.params_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureParamsIsMutable();
params_.addAll(other.params_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCommand()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string command = 1;
private java.lang.Object command_ = "";
/**
* required string command = 1;
*/
public boolean hasCommand() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string command = 1;
*/
public java.lang.String getCommand() {
java.lang.Object ref = command_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
command_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string command = 1;
*/
public com.google.protobuf.ByteString
getCommandBytes() {
java.lang.Object ref = command_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
command_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string command = 1;
*/
public Builder setCommand(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
command_ = value;
onChanged();
return this;
}
/**
* required string command = 1;
*/
public Builder clearCommand() {
bitField0_ = (bitField0_ & ~0x00000001);
command_ = getDefaultInstance().getCommand();
onChanged();
return this;
}
/**
* required string command = 1;
*/
public Builder setCommandBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
command_ = value;
onChanged();
return this;
}
// repeated string params = 2;
private com.google.protobuf.LazyStringList params_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureParamsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
params_ = new com.google.protobuf.LazyStringArrayList(params_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated string params = 2;
*/
public java.util.List
getParamsList() {
return java.util.Collections.unmodifiableList(params_);
}
/**
* repeated string params = 2;
*/
public int getParamsCount() {
return params_.size();
}
/**
* repeated string params = 2;
*/
public java.lang.String getParams(int index) {
return params_.get(index);
}
/**
* repeated string params = 2;
*/
public com.google.protobuf.ByteString
getParamsBytes(int index) {
return params_.getByteString(index);
}
/**
* repeated string params = 2;
*/
public Builder setParams(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureParamsIsMutable();
params_.set(index, value);
onChanged();
return this;
}
/**
* repeated string params = 2;
*/
public Builder addParams(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureParamsIsMutable();
params_.add(value);
onChanged();
return this;
}
/**
* repeated string params = 2;
*/
public Builder addAllParams(
java.lang.Iterable values) {
ensureParamsIsMutable();
super.addAll(values, params_);
onChanged();
return this;
}
/**
* repeated string params = 2;
*/
public Builder clearParams() {
params_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* repeated string params = 2;
*/
public Builder addParamsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureParamsIsMutable();
params_.add(value);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:TajoHeartbeatResponse.ResponseCommand)
}
static {
defaultInstance = new ResponseCommand(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TajoHeartbeatResponse.ResponseCommand)
}
private int bitField0_;
// required .BoolProto heartbeatResult = 1;
public static final int HEARTBEATRESULT_FIELD_NUMBER = 1;
private org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto heartbeatResult_;
/**
* required .BoolProto heartbeatResult = 1;
*/
public boolean hasHeartbeatResult() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .BoolProto heartbeatResult = 1;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto getHeartbeatResult() {
return heartbeatResult_;
}
/**
* required .BoolProto heartbeatResult = 1;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProtoOrBuilder getHeartbeatResultOrBuilder() {
return heartbeatResult_;
}
// optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
public static final int RESPONSECOMMAND_FIELD_NUMBER = 3;
private org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand responseCommand_;
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
public boolean hasResponseCommand() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
public org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand getResponseCommand() {
return responseCommand_;
}
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
public org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommandOrBuilder getResponseCommandOrBuilder() {
return responseCommand_;
}
private void initFields() {
heartbeatResult_ = org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto.getDefaultInstance();
responseCommand_ = org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasHeartbeatResult()) {
memoizedIsInitialized = 0;
return false;
}
if (!getHeartbeatResult().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (hasResponseCommand()) {
if (!getResponseCommand().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, heartbeatResult_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(3, responseCommand_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, heartbeatResult_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, responseCommand_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.TajoHeartbeatResponse)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse other = (org.apache.tajo.ResourceProtos.TajoHeartbeatResponse) obj;
boolean result = true;
result = result && (hasHeartbeatResult() == other.hasHeartbeatResult());
if (hasHeartbeatResult()) {
result = result && getHeartbeatResult()
.equals(other.getHeartbeatResult());
}
result = result && (hasResponseCommand() == other.hasResponseCommand());
if (hasResponseCommand()) {
result = result && getResponseCommand()
.equals(other.getResponseCommand());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasHeartbeatResult()) {
hash = (37 * hash) + HEARTBEATRESULT_FIELD_NUMBER;
hash = (53 * hash) + getHeartbeatResult().hashCode();
}
if (hasResponseCommand()) {
hash = (37 * hash) + RESPONSECOMMAND_FIELD_NUMBER;
hash = (53 * hash) + getResponseCommand().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.TajoHeartbeatResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.TajoHeartbeatResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TajoHeartbeatResponse}
*
*
* deprecated
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.TajoHeartbeatResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.class, org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getHeartbeatResultFieldBuilder();
getResponseCommandFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (heartbeatResultBuilder_ == null) {
heartbeatResult_ = org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto.getDefaultInstance();
} else {
heartbeatResultBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (responseCommandBuilder_ == null) {
responseCommand_ = org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.getDefaultInstance();
} else {
responseCommandBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_TajoHeartbeatResponse_descriptor;
}
public org.apache.tajo.ResourceProtos.TajoHeartbeatResponse getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.TajoHeartbeatResponse build() {
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.TajoHeartbeatResponse buildPartial() {
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse result = new org.apache.tajo.ResourceProtos.TajoHeartbeatResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (heartbeatResultBuilder_ == null) {
result.heartbeatResult_ = heartbeatResult_;
} else {
result.heartbeatResult_ = heartbeatResultBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (responseCommandBuilder_ == null) {
result.responseCommand_ = responseCommand_;
} else {
result.responseCommand_ = responseCommandBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.TajoHeartbeatResponse) {
return mergeFrom((org.apache.tajo.ResourceProtos.TajoHeartbeatResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.TajoHeartbeatResponse other) {
if (other == org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.getDefaultInstance()) return this;
if (other.hasHeartbeatResult()) {
mergeHeartbeatResult(other.getHeartbeatResult());
}
if (other.hasResponseCommand()) {
mergeResponseCommand(other.getResponseCommand());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasHeartbeatResult()) {
return false;
}
if (!getHeartbeatResult().isInitialized()) {
return false;
}
if (hasResponseCommand()) {
if (!getResponseCommand().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.TajoHeartbeatResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .BoolProto heartbeatResult = 1;
private org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto heartbeatResult_ = org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto.Builder, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProtoOrBuilder> heartbeatResultBuilder_;
/**
* required .BoolProto heartbeatResult = 1;
*/
public boolean hasHeartbeatResult() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .BoolProto heartbeatResult = 1;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto getHeartbeatResult() {
if (heartbeatResultBuilder_ == null) {
return heartbeatResult_;
} else {
return heartbeatResultBuilder_.getMessage();
}
}
/**
* required .BoolProto heartbeatResult = 1;
*/
public Builder setHeartbeatResult(org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto value) {
if (heartbeatResultBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
heartbeatResult_ = value;
onChanged();
} else {
heartbeatResultBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .BoolProto heartbeatResult = 1;
*/
public Builder setHeartbeatResult(
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto.Builder builderForValue) {
if (heartbeatResultBuilder_ == null) {
heartbeatResult_ = builderForValue.build();
onChanged();
} else {
heartbeatResultBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .BoolProto heartbeatResult = 1;
*/
public Builder mergeHeartbeatResult(org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto value) {
if (heartbeatResultBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
heartbeatResult_ != org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto.getDefaultInstance()) {
heartbeatResult_ =
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto.newBuilder(heartbeatResult_).mergeFrom(value).buildPartial();
} else {
heartbeatResult_ = value;
}
onChanged();
} else {
heartbeatResultBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .BoolProto heartbeatResult = 1;
*/
public Builder clearHeartbeatResult() {
if (heartbeatResultBuilder_ == null) {
heartbeatResult_ = org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto.getDefaultInstance();
onChanged();
} else {
heartbeatResultBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .BoolProto heartbeatResult = 1;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto.Builder getHeartbeatResultBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getHeartbeatResultFieldBuilder().getBuilder();
}
/**
* required .BoolProto heartbeatResult = 1;
*/
public org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProtoOrBuilder getHeartbeatResultOrBuilder() {
if (heartbeatResultBuilder_ != null) {
return heartbeatResultBuilder_.getMessageOrBuilder();
} else {
return heartbeatResult_;
}
}
/**
* required .BoolProto heartbeatResult = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto.Builder, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProtoOrBuilder>
getHeartbeatResultFieldBuilder() {
if (heartbeatResultBuilder_ == null) {
heartbeatResultBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProto.Builder, org.apache.tajo.rpc.protocolrecords.PrimitiveProtos.BoolProtoOrBuilder>(
heartbeatResult_,
getParentForChildren(),
isClean());
heartbeatResult_ = null;
}
return heartbeatResultBuilder_;
}
// optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
private org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand responseCommand_ = org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand, org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.Builder, org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommandOrBuilder> responseCommandBuilder_;
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
public boolean hasResponseCommand() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
public org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand getResponseCommand() {
if (responseCommandBuilder_ == null) {
return responseCommand_;
} else {
return responseCommandBuilder_.getMessage();
}
}
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
public Builder setResponseCommand(org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand value) {
if (responseCommandBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
responseCommand_ = value;
onChanged();
} else {
responseCommandBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
public Builder setResponseCommand(
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.Builder builderForValue) {
if (responseCommandBuilder_ == null) {
responseCommand_ = builderForValue.build();
onChanged();
} else {
responseCommandBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
public Builder mergeResponseCommand(org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand value) {
if (responseCommandBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
responseCommand_ != org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.getDefaultInstance()) {
responseCommand_ =
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.newBuilder(responseCommand_).mergeFrom(value).buildPartial();
} else {
responseCommand_ = value;
}
onChanged();
} else {
responseCommandBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
public Builder clearResponseCommand() {
if (responseCommandBuilder_ == null) {
responseCommand_ = org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.getDefaultInstance();
onChanged();
} else {
responseCommandBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
public org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.Builder getResponseCommandBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getResponseCommandFieldBuilder().getBuilder();
}
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
public org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommandOrBuilder getResponseCommandOrBuilder() {
if (responseCommandBuilder_ != null) {
return responseCommandBuilder_.getMessageOrBuilder();
} else {
return responseCommand_;
}
}
/**
* optional .TajoHeartbeatResponse.ResponseCommand responseCommand = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand, org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.Builder, org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommandOrBuilder>
getResponseCommandFieldBuilder() {
if (responseCommandBuilder_ == null) {
responseCommandBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand, org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommand.Builder, org.apache.tajo.ResourceProtos.TajoHeartbeatResponse.ResponseCommandOrBuilder>(
responseCommand_,
getParentForChildren(),
isClean());
responseCommand_ = null;
}
return responseCommandBuilder_;
}
// @@protoc_insertion_point(builder_scope:TajoHeartbeatResponse)
}
static {
defaultInstance = new TajoHeartbeatResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TajoHeartbeatResponse)
}
public interface WorkerConnectionsResponseOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// repeated .WorkerConnectionInfoProto worker = 1;
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
java.util.List
getWorkerList();
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto getWorker(int index);
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
int getWorkerCount();
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
java.util.List extends org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder>
getWorkerOrBuilderList();
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder getWorkerOrBuilder(
int index);
}
/**
* Protobuf type {@code WorkerConnectionsResponse}
*/
public static final class WorkerConnectionsResponse extends
com.google.protobuf.GeneratedMessage
implements WorkerConnectionsResponseOrBuilder {
// Use WorkerConnectionsResponse.newBuilder() to construct.
private WorkerConnectionsResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private WorkerConnectionsResponse(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final WorkerConnectionsResponse defaultInstance;
public static WorkerConnectionsResponse getDefaultInstance() {
return defaultInstance;
}
public WorkerConnectionsResponse getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private WorkerConnectionsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
worker_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
worker_.add(input.readMessage(org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
worker_ = java.util.Collections.unmodifiableList(worker_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_WorkerConnectionsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_WorkerConnectionsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.WorkerConnectionsResponse.class, org.apache.tajo.ResourceProtos.WorkerConnectionsResponse.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public WorkerConnectionsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new WorkerConnectionsResponse(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
// repeated .WorkerConnectionInfoProto worker = 1;
public static final int WORKER_FIELD_NUMBER = 1;
private java.util.List worker_;
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public java.util.List getWorkerList() {
return worker_;
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public java.util.List extends org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder>
getWorkerOrBuilderList() {
return worker_;
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public int getWorkerCount() {
return worker_.size();
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProto getWorker(int index) {
return worker_.get(index);
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder getWorkerOrBuilder(
int index) {
return worker_.get(index);
}
private void initFields() {
worker_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
for (int i = 0; i < getWorkerCount(); i++) {
if (!getWorker(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < worker_.size(); i++) {
output.writeMessage(1, worker_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < worker_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, worker_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tajo.ResourceProtos.WorkerConnectionsResponse)) {
return super.equals(obj);
}
org.apache.tajo.ResourceProtos.WorkerConnectionsResponse other = (org.apache.tajo.ResourceProtos.WorkerConnectionsResponse) obj;
boolean result = true;
result = result && getWorkerList()
.equals(other.getWorkerList());
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (getWorkerCount() > 0) {
hash = (37 * hash) + WORKER_FIELD_NUMBER;
hash = (53 * hash) + getWorkerList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tajo.ResourceProtos.WorkerConnectionsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.WorkerConnectionsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.WorkerConnectionsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tajo.ResourceProtos.WorkerConnectionsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.WorkerConnectionsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.WorkerConnectionsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.WorkerConnectionsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.tajo.ResourceProtos.WorkerConnectionsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.tajo.ResourceProtos.WorkerConnectionsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.tajo.ResourceProtos.WorkerConnectionsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.tajo.ResourceProtos.WorkerConnectionsResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code WorkerConnectionsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.tajo.ResourceProtos.WorkerConnectionsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_WorkerConnectionsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_WorkerConnectionsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.WorkerConnectionsResponse.class, org.apache.tajo.ResourceProtos.WorkerConnectionsResponse.Builder.class);
}
// Construct using org.apache.tajo.ResourceProtos.WorkerConnectionsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getWorkerFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (workerBuilder_ == null) {
worker_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
workerBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tajo.ResourceProtos.internal_static_WorkerConnectionsResponse_descriptor;
}
public org.apache.tajo.ResourceProtos.WorkerConnectionsResponse getDefaultInstanceForType() {
return org.apache.tajo.ResourceProtos.WorkerConnectionsResponse.getDefaultInstance();
}
public org.apache.tajo.ResourceProtos.WorkerConnectionsResponse build() {
org.apache.tajo.ResourceProtos.WorkerConnectionsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.tajo.ResourceProtos.WorkerConnectionsResponse buildPartial() {
org.apache.tajo.ResourceProtos.WorkerConnectionsResponse result = new org.apache.tajo.ResourceProtos.WorkerConnectionsResponse(this);
int from_bitField0_ = bitField0_;
if (workerBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
worker_ = java.util.Collections.unmodifiableList(worker_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.worker_ = worker_;
} else {
result.worker_ = workerBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tajo.ResourceProtos.WorkerConnectionsResponse) {
return mergeFrom((org.apache.tajo.ResourceProtos.WorkerConnectionsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tajo.ResourceProtos.WorkerConnectionsResponse other) {
if (other == org.apache.tajo.ResourceProtos.WorkerConnectionsResponse.getDefaultInstance()) return this;
if (workerBuilder_ == null) {
if (!other.worker_.isEmpty()) {
if (worker_.isEmpty()) {
worker_ = other.worker_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureWorkerIsMutable();
worker_.addAll(other.worker_);
}
onChanged();
}
} else {
if (!other.worker_.isEmpty()) {
if (workerBuilder_.isEmpty()) {
workerBuilder_.dispose();
workerBuilder_ = null;
worker_ = other.worker_;
bitField0_ = (bitField0_ & ~0x00000001);
workerBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getWorkerFieldBuilder() : null;
} else {
workerBuilder_.addAllMessages(other.worker_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getWorkerCount(); i++) {
if (!getWorker(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tajo.ResourceProtos.WorkerConnectionsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tajo.ResourceProtos.WorkerConnectionsResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated .WorkerConnectionInfoProto worker = 1;
private java.util.List worker_ =
java.util.Collections.emptyList();
private void ensureWorkerIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
worker_ = new java.util.ArrayList(worker_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder, org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder> workerBuilder_;
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public java.util.List getWorkerList() {
if (workerBuilder_ == null) {
return java.util.Collections.unmodifiableList(worker_);
} else {
return workerBuilder_.getMessageList();
}
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public int getWorkerCount() {
if (workerBuilder_ == null) {
return worker_.size();
} else {
return workerBuilder_.getCount();
}
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProto getWorker(int index) {
if (workerBuilder_ == null) {
return worker_.get(index);
} else {
return workerBuilder_.getMessage(index);
}
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public Builder setWorker(
int index, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto value) {
if (workerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureWorkerIsMutable();
worker_.set(index, value);
onChanged();
} else {
workerBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public Builder setWorker(
int index, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder builderForValue) {
if (workerBuilder_ == null) {
ensureWorkerIsMutable();
worker_.set(index, builderForValue.build());
onChanged();
} else {
workerBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public Builder addWorker(org.apache.tajo.TajoProtos.WorkerConnectionInfoProto value) {
if (workerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureWorkerIsMutable();
worker_.add(value);
onChanged();
} else {
workerBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public Builder addWorker(
int index, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto value) {
if (workerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureWorkerIsMutable();
worker_.add(index, value);
onChanged();
} else {
workerBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public Builder addWorker(
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder builderForValue) {
if (workerBuilder_ == null) {
ensureWorkerIsMutable();
worker_.add(builderForValue.build());
onChanged();
} else {
workerBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public Builder addWorker(
int index, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder builderForValue) {
if (workerBuilder_ == null) {
ensureWorkerIsMutable();
worker_.add(index, builderForValue.build());
onChanged();
} else {
workerBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public Builder addAllWorker(
java.lang.Iterable extends org.apache.tajo.TajoProtos.WorkerConnectionInfoProto> values) {
if (workerBuilder_ == null) {
ensureWorkerIsMutable();
super.addAll(values, worker_);
onChanged();
} else {
workerBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public Builder clearWorker() {
if (workerBuilder_ == null) {
worker_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
workerBuilder_.clear();
}
return this;
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public Builder removeWorker(int index) {
if (workerBuilder_ == null) {
ensureWorkerIsMutable();
worker_.remove(index);
onChanged();
} else {
workerBuilder_.remove(index);
}
return this;
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder getWorkerBuilder(
int index) {
return getWorkerFieldBuilder().getBuilder(index);
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder getWorkerOrBuilder(
int index) {
if (workerBuilder_ == null) {
return worker_.get(index); } else {
return workerBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public java.util.List extends org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder>
getWorkerOrBuilderList() {
if (workerBuilder_ != null) {
return workerBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(worker_);
}
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder addWorkerBuilder() {
return getWorkerFieldBuilder().addBuilder(
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.getDefaultInstance());
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder addWorkerBuilder(
int index) {
return getWorkerFieldBuilder().addBuilder(
index, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.getDefaultInstance());
}
/**
* repeated .WorkerConnectionInfoProto worker = 1;
*/
public java.util.List
getWorkerBuilderList() {
return getWorkerFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder, org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder>
getWorkerFieldBuilder() {
if (workerBuilder_ == null) {
workerBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.tajo.TajoProtos.WorkerConnectionInfoProto, org.apache.tajo.TajoProtos.WorkerConnectionInfoProto.Builder, org.apache.tajo.TajoProtos.WorkerConnectionInfoProtoOrBuilder>(
worker_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
worker_ = null;
}
return workerBuilder_;
}
// @@protoc_insertion_point(builder_scope:WorkerConnectionsResponse)
}
static {
defaultInstance = new WorkerConnectionsResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:WorkerConnectionsResponse)
}
public interface NodeResourceRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string queue = 1;
/**
* optional string queue = 1;
*/
boolean hasQueue();
/**
* optional string queue = 1;
*/
java.lang.String getQueue();
/**
* optional string queue = 1;
*/
com.google.protobuf.ByteString
getQueueBytes();
// required string userId = 2;
/**
* required string userId = 2;
*/
boolean hasUserId();
/**
* required string userId = 2;
*/
java.lang.String getUserId();
/**
* required string userId = 2;
*/
com.google.protobuf.ByteString
getUserIdBytes();
// required .ResourceType type = 3;
/**
* required .ResourceType type = 3;
*/
boolean hasType();
/**
* required .ResourceType type = 3;
*/
org.apache.tajo.ResourceProtos.ResourceType getType();
// required int32 priority = 4;
/**
* required int32 priority = 4;
*/
boolean hasPriority();
/**
* required int32 priority = 4;
*/
int getPriority();
// required .QueryIdProto queryId = 5;
/**
* required .QueryIdProto queryId = 5;
*/
boolean hasQueryId();
/**
* required .QueryIdProto queryId = 5;
*/
org.apache.tajo.TajoIdProtos.QueryIdProto getQueryId();
/**
* required .QueryIdProto queryId = 5;
*/
org.apache.tajo.TajoIdProtos.QueryIdProtoOrBuilder getQueryIdOrBuilder();
// required int32 numContainers = 6;
/**
* required int32 numContainers = 6;
*/
boolean hasNumContainers();
/**
* required int32 numContainers = 6;
*/
int getNumContainers();
// required .NodeResourceProto capacity = 7;
/**
* required .NodeResourceProto capacity = 7;
*/
boolean hasCapacity();
/**
* required .NodeResourceProto capacity = 7;
*/
org.apache.tajo.TajoProtos.NodeResourceProto getCapacity();
/**
* required .NodeResourceProto capacity = 7;
*/
org.apache.tajo.TajoProtos.NodeResourceProtoOrBuilder getCapacityOrBuilder();
// required int32 runningTasks = 8;
/**
* required int32 runningTasks = 8;
*/
boolean hasRunningTasks();
/**
* required int32 runningTasks = 8;
*/
int getRunningTasks();
// repeated int32 candidateNodes = 9;
/**
* repeated int32 candidateNodes = 9;
*/
java.util.List getCandidateNodesList();
/**
* repeated int32 candidateNodes = 9;
*/
int getCandidateNodesCount();
/**
* repeated int32 candidateNodes = 9;
*/
int getCandidateNodes(int index);
}
/**
* Protobuf type {@code NodeResourceRequest}
*/
public static final class NodeResourceRequest extends
com.google.protobuf.GeneratedMessage
implements NodeResourceRequestOrBuilder {
// Use NodeResourceRequest.newBuilder() to construct.
private NodeResourceRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private NodeResourceRequest(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final NodeResourceRequest defaultInstance;
public static NodeResourceRequest getDefaultInstance() {
return defaultInstance;
}
public NodeResourceRequest getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NodeResourceRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
queue_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
userId_ = input.readBytes();
break;
}
case 24: {
int rawValue = input.readEnum();
org.apache.tajo.ResourceProtos.ResourceType value = org.apache.tajo.ResourceProtos.ResourceType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(3, rawValue);
} else {
bitField0_ |= 0x00000004;
type_ = value;
}
break;
}
case 32: {
bitField0_ |= 0x00000008;
priority_ = input.readInt32();
break;
}
case 42: {
org.apache.tajo.TajoIdProtos.QueryIdProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = queryId_.toBuilder();
}
queryId_ = input.readMessage(org.apache.tajo.TajoIdProtos.QueryIdProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(queryId_);
queryId_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 48: {
bitField0_ |= 0x00000020;
numContainers_ = input.readInt32();
break;
}
case 58: {
org.apache.tajo.TajoProtos.NodeResourceProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
subBuilder = capacity_.toBuilder();
}
capacity_ = input.readMessage(org.apache.tajo.TajoProtos.NodeResourceProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(capacity_);
capacity_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
case 64: {
bitField0_ |= 0x00000080;
runningTasks_ = input.readInt32();
break;
}
case 72: {
if (!((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
candidateNodes_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000100;
}
candidateNodes_.add(input.readInt32());
break;
}
case 74: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000100) == 0x00000100) && input.getBytesUntilLimit() > 0) {
candidateNodes_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000100;
}
while (input.getBytesUntilLimit() > 0) {
candidateNodes_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
candidateNodes_ = java.util.Collections.unmodifiableList(candidateNodes_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tajo.ResourceProtos.internal_static_NodeResourceRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tajo.ResourceProtos.internal_static_NodeResourceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tajo.ResourceProtos.NodeResourceRequest.class, org.apache.tajo.ResourceProtos.NodeResourceRequest.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public NodeResourceRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NodeResourceRequest(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser