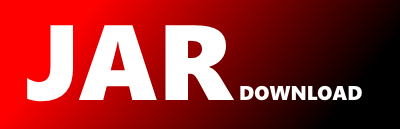
org.apache.tapestry.contrib.form.MultiplePropertySelection Maven / Gradle / Ivy
// Copyright 2004, 2005 The Apache Software Foundation
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package org.apache.tapestry.contrib.form;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import org.apache.tapestry.IMarkupWriter;
import org.apache.tapestry.IRequestCycle;
import org.apache.tapestry.Tapestry;
import org.apache.tapestry.form.AbstractFormComponent;
import org.apache.tapestry.form.IPropertySelectionModel;
import org.apache.tapestry.form.ValidatableField;
import org.apache.tapestry.form.ValidatableFieldSupport;
import org.apache.tapestry.valid.ValidatorException;
/**
* A component which uses <input type=checkbox> to set a property of some
* object. Typically, the values for the object are defined using an
* {@link java.lang.Enum}. A MultiplePropertySelection is
* dependent on an {link IPropertySelectionModel} to provide the list of
* possible values.
*
* Often, this is used to select one or more
* {@link java.lang.Enum} to assign to a property; the
* {@link org.apache.tapestry.form.EnumPropertySelectionModel}class simplifies
* this.
*
* The {@link org.apache.tapestry.contrib.palette.Palette}component is more
* powerful, but requires client-side JavaScript and is not fully cross-browser
* compatible.
*
*
*
* Parameter
* Type
* Direction
* Required
* Default
* Description
*
*
* selectedList
* java.util.List
* in-out
* yes
*
* The property to set. During rendering, this property is read, and sets
* the default value of the options in the select. When the form is submitted,
* list is cleared, then has each selected option added to it.
*
*
* renderer
* {@link IMultiplePropertySelectionRenderer}
* in
* no
* shared instance of {@link CheckBoxMultiplePropertySelectionRenderer}
* Defines the object used to render this component. The default renders a
* table of checkboxes.
*
*
* model
* {@link IPropertySelectionModel}
* in
* yes
*
* The model provides a list of possible labels, and matches those labels
* against possible values that can be assigned back to the property.
*
*
* disabled
* boolean
* in
* no
* false
* Controls whether the <select> is active or not. A disabled
* PropertySelection does not update its value parameter.
*
* Corresponds to the disabled
HTML attribute.
*
*
*
* Informal parameters are not allowed.
*
* As of 4.0, this component can be validated.
*
* @author Sanjay Munjal
*/
public abstract class MultiplePropertySelection extends AbstractFormComponent
implements ValidatableField
{
/**
* A shared instance of {@link CheckBoxMultiplePropertySelectionRenderer}.
*/
public static final IMultiplePropertySelectionRenderer DEFAULT_CHECKBOX_RENDERER = new CheckBoxMultiplePropertySelectionRenderer();
public abstract Collection getSelectedList();
public abstract void setSelectedList(Collection selectedList);
protected void finishLoad()
{
setRenderer(DEFAULT_CHECKBOX_RENDERER);
}
protected void renderFormComponent(IMarkupWriter writer, IRequestCycle cycle)
{
Collection selectedList = getSelectedList();
if (selectedList == null)
throw Tapestry.createRequiredParameterException(this,
"selectedList");
IPropertySelectionModel model = getModel();
if (model == null)
throw Tapestry.createRequiredParameterException(this, "model");
IMultiplePropertySelectionRenderer renderer = getRenderer();
// Start rendering
renderer.beginRender(this, writer, cycle);
int count = model.getOptionCount();
for(int i = 0; i < count; i++)
{
Object option = model.getOption(i);
// Try to find the option in the list and if yes, then it is
// checked.
boolean optionSelected = selectedList.contains(option);
renderer.renderOption(this, writer, cycle, model, option, i,
optionSelected);
}
// A PropertySelection doesn't allow a body, so no need to worry about
// wrapped components.
renderer.endRender(this, writer, cycle);
}
/**
* @see org.apache.tapestry.form.AbstractRequirableField#rewindFormComponent(org.apache.tapestry.IMarkupWriter,
* org.apache.tapestry.IRequestCycle)
*/
protected void rewindFormComponent(IMarkupWriter writer, IRequestCycle cycle)
{
// get all the values
String[] optionValues = cycle.getParameters(getName());
IPropertySelectionModel model = getModel();
List selectedList = new ArrayList(getModel().getOptionCount());
// Nothing was selected
if (optionValues != null)
{
// Go through the array and translate and put back in the list
for(int i = 0; i < optionValues.length; i++)
{
// Translate the new value
Object selectedValue = model.translateValue(optionValues[i]);
// Add this element in the list back
selectedList.add(selectedValue);
}
}
try
{
getValidatableFieldSupport().validate(this, writer, cycle,
selectedList);
setSelectedList(selectedList);
}
catch (ValidatorException e)
{
getForm().getDelegate().record(e);
}
}
public abstract IPropertySelectionModel getModel();
public abstract IMultiplePropertySelectionRenderer getRenderer();
public abstract void setRenderer(IMultiplePropertySelectionRenderer renderer);
public abstract ValidatableFieldSupport getValidatableFieldSupport();
/**
* @see org.apache.tapestry.form.AbstractFormComponent#isRequired()
*/
public boolean isRequired()
{
return getValidatableFieldSupport().isRequired(this);
}
}