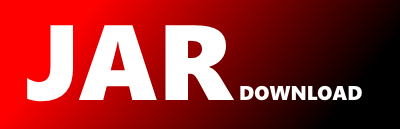
org.apache.tez.runtime.api.events.EventProtos Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: Events.proto
package org.apache.tez.runtime.api.events;
public final class EventProtos {
private EventProtos() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface DataMovementEventProtoOrBuilder extends
// @@protoc_insertion_point(interface_extends:DataMovementEventProto)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 source_index = 1;
* @return Whether the sourceIndex field is set.
*/
boolean hasSourceIndex();
/**
* optional int32 source_index = 1;
* @return The sourceIndex.
*/
int getSourceIndex();
/**
* optional int32 target_index = 2;
* @return Whether the targetIndex field is set.
*/
boolean hasTargetIndex();
/**
* optional int32 target_index = 2;
* @return The targetIndex.
*/
int getTargetIndex();
/**
* optional bytes user_payload = 3;
* @return Whether the userPayload field is set.
*/
boolean hasUserPayload();
/**
* optional bytes user_payload = 3;
* @return The userPayload.
*/
com.google.protobuf.ByteString getUserPayload();
/**
* optional int32 version = 4;
* @return Whether the version field is set.
*/
boolean hasVersion();
/**
* optional int32 version = 4;
* @return The version.
*/
int getVersion();
}
/**
* Protobuf type {@code DataMovementEventProto}
*/
public static final class DataMovementEventProto extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:DataMovementEventProto)
DataMovementEventProtoOrBuilder {
private static final long serialVersionUID = 0L;
// Use DataMovementEventProto.newBuilder() to construct.
private DataMovementEventProto(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DataMovementEventProto() {
userPayload_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DataMovementEventProto();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DataMovementEventProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
sourceIndex_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
targetIndex_ = input.readInt32();
break;
}
case 26: {
bitField0_ |= 0x00000004;
userPayload_ = input.readBytes();
break;
}
case 32: {
bitField0_ |= 0x00000008;
version_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_DataMovementEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_DataMovementEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto.class, org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto.Builder.class);
}
private int bitField0_;
public static final int SOURCE_INDEX_FIELD_NUMBER = 1;
private int sourceIndex_;
/**
* optional int32 source_index = 1;
* @return Whether the sourceIndex field is set.
*/
@java.lang.Override
public boolean hasSourceIndex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 source_index = 1;
* @return The sourceIndex.
*/
@java.lang.Override
public int getSourceIndex() {
return sourceIndex_;
}
public static final int TARGET_INDEX_FIELD_NUMBER = 2;
private int targetIndex_;
/**
* optional int32 target_index = 2;
* @return Whether the targetIndex field is set.
*/
@java.lang.Override
public boolean hasTargetIndex() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 target_index = 2;
* @return The targetIndex.
*/
@java.lang.Override
public int getTargetIndex() {
return targetIndex_;
}
public static final int USER_PAYLOAD_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString userPayload_;
/**
* optional bytes user_payload = 3;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes user_payload = 3;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
public static final int VERSION_FIELD_NUMBER = 4;
private int version_;
/**
* optional int32 version = 4;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional int32 version = 4;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, sourceIndex_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, targetIndex_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(3, userPayload_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt32(4, version_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, sourceIndex_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, targetIndex_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, userPayload_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, version_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto)) {
return super.equals(obj);
}
org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto other = (org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto) obj;
if (hasSourceIndex() != other.hasSourceIndex()) return false;
if (hasSourceIndex()) {
if (getSourceIndex()
!= other.getSourceIndex()) return false;
}
if (hasTargetIndex() != other.hasTargetIndex()) return false;
if (hasTargetIndex()) {
if (getTargetIndex()
!= other.getTargetIndex()) return false;
}
if (hasUserPayload() != other.hasUserPayload()) return false;
if (hasUserPayload()) {
if (!getUserPayload()
.equals(other.getUserPayload())) return false;
}
if (hasVersion() != other.hasVersion()) return false;
if (hasVersion()) {
if (getVersion()
!= other.getVersion()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasSourceIndex()) {
hash = (37 * hash) + SOURCE_INDEX_FIELD_NUMBER;
hash = (53 * hash) + getSourceIndex();
}
if (hasTargetIndex()) {
hash = (37 * hash) + TARGET_INDEX_FIELD_NUMBER;
hash = (53 * hash) + getTargetIndex();
}
if (hasUserPayload()) {
hash = (37 * hash) + USER_PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getUserPayload().hashCode();
}
if (hasVersion()) {
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code DataMovementEventProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:DataMovementEventProto)
org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_DataMovementEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_DataMovementEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto.class, org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto.Builder.class);
}
// Construct using org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
sourceIndex_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
targetIndex_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
userPayload_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
version_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_DataMovementEventProto_descriptor;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto getDefaultInstanceForType() {
return org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto.getDefaultInstance();
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto build() {
org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto buildPartial() {
org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto result = new org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.sourceIndex_ = sourceIndex_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.targetIndex_ = targetIndex_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.userPayload_ = userPayload_;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.version_ = version_;
to_bitField0_ |= 0x00000008;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto) {
return mergeFrom((org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto other) {
if (other == org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto.getDefaultInstance()) return this;
if (other.hasSourceIndex()) {
setSourceIndex(other.getSourceIndex());
}
if (other.hasTargetIndex()) {
setTargetIndex(other.getTargetIndex());
}
if (other.hasUserPayload()) {
setUserPayload(other.getUserPayload());
}
if (other.hasVersion()) {
setVersion(other.getVersion());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int sourceIndex_ ;
/**
* optional int32 source_index = 1;
* @return Whether the sourceIndex field is set.
*/
@java.lang.Override
public boolean hasSourceIndex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 source_index = 1;
* @return The sourceIndex.
*/
@java.lang.Override
public int getSourceIndex() {
return sourceIndex_;
}
/**
* optional int32 source_index = 1;
* @param value The sourceIndex to set.
* @return This builder for chaining.
*/
public Builder setSourceIndex(int value) {
bitField0_ |= 0x00000001;
sourceIndex_ = value;
onChanged();
return this;
}
/**
* optional int32 source_index = 1;
* @return This builder for chaining.
*/
public Builder clearSourceIndex() {
bitField0_ = (bitField0_ & ~0x00000001);
sourceIndex_ = 0;
onChanged();
return this;
}
private int targetIndex_ ;
/**
* optional int32 target_index = 2;
* @return Whether the targetIndex field is set.
*/
@java.lang.Override
public boolean hasTargetIndex() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 target_index = 2;
* @return The targetIndex.
*/
@java.lang.Override
public int getTargetIndex() {
return targetIndex_;
}
/**
* optional int32 target_index = 2;
* @param value The targetIndex to set.
* @return This builder for chaining.
*/
public Builder setTargetIndex(int value) {
bitField0_ |= 0x00000002;
targetIndex_ = value;
onChanged();
return this;
}
/**
* optional int32 target_index = 2;
* @return This builder for chaining.
*/
public Builder clearTargetIndex() {
bitField0_ = (bitField0_ & ~0x00000002);
targetIndex_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString userPayload_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes user_payload = 3;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes user_payload = 3;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
/**
* optional bytes user_payload = 3;
* @param value The userPayload to set.
* @return This builder for chaining.
*/
public Builder setUserPayload(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
userPayload_ = value;
onChanged();
return this;
}
/**
* optional bytes user_payload = 3;
* @return This builder for chaining.
*/
public Builder clearUserPayload() {
bitField0_ = (bitField0_ & ~0x00000004);
userPayload_ = getDefaultInstance().getUserPayload();
onChanged();
return this;
}
private int version_ ;
/**
* optional int32 version = 4;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional int32 version = 4;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
/**
* optional int32 version = 4;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(int value) {
bitField0_ |= 0x00000008;
version_ = value;
onChanged();
return this;
}
/**
* optional int32 version = 4;
* @return This builder for chaining.
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000008);
version_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:DataMovementEventProto)
}
// @@protoc_insertion_point(class_scope:DataMovementEventProto)
private static final org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto();
}
public static org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DataMovementEventProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DataMovementEventProto(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.DataMovementEventProto getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CompositeRoutedDataMovementEventProtoOrBuilder extends
// @@protoc_insertion_point(interface_extends:CompositeRoutedDataMovementEventProto)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 source_index = 1;
* @return Whether the sourceIndex field is set.
*/
boolean hasSourceIndex();
/**
* optional int32 source_index = 1;
* @return The sourceIndex.
*/
int getSourceIndex();
/**
* optional int32 target_index = 2;
* @return Whether the targetIndex field is set.
*/
boolean hasTargetIndex();
/**
* optional int32 target_index = 2;
* @return The targetIndex.
*/
int getTargetIndex();
/**
* optional int32 count = 3;
* @return Whether the count field is set.
*/
boolean hasCount();
/**
* optional int32 count = 3;
* @return The count.
*/
int getCount();
/**
* optional bytes user_payload = 4;
* @return Whether the userPayload field is set.
*/
boolean hasUserPayload();
/**
* optional bytes user_payload = 4;
* @return The userPayload.
*/
com.google.protobuf.ByteString getUserPayload();
/**
* optional int32 version = 5;
* @return Whether the version field is set.
*/
boolean hasVersion();
/**
* optional int32 version = 5;
* @return The version.
*/
int getVersion();
}
/**
* Protobuf type {@code CompositeRoutedDataMovementEventProto}
*/
public static final class CompositeRoutedDataMovementEventProto extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:CompositeRoutedDataMovementEventProto)
CompositeRoutedDataMovementEventProtoOrBuilder {
private static final long serialVersionUID = 0L;
// Use CompositeRoutedDataMovementEventProto.newBuilder() to construct.
private CompositeRoutedDataMovementEventProto(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CompositeRoutedDataMovementEventProto() {
userPayload_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CompositeRoutedDataMovementEventProto();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CompositeRoutedDataMovementEventProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
sourceIndex_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
targetIndex_ = input.readInt32();
break;
}
case 24: {
bitField0_ |= 0x00000004;
count_ = input.readInt32();
break;
}
case 34: {
bitField0_ |= 0x00000008;
userPayload_ = input.readBytes();
break;
}
case 40: {
bitField0_ |= 0x00000010;
version_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CompositeRoutedDataMovementEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CompositeRoutedDataMovementEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto.class, org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto.Builder.class);
}
private int bitField0_;
public static final int SOURCE_INDEX_FIELD_NUMBER = 1;
private int sourceIndex_;
/**
* optional int32 source_index = 1;
* @return Whether the sourceIndex field is set.
*/
@java.lang.Override
public boolean hasSourceIndex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 source_index = 1;
* @return The sourceIndex.
*/
@java.lang.Override
public int getSourceIndex() {
return sourceIndex_;
}
public static final int TARGET_INDEX_FIELD_NUMBER = 2;
private int targetIndex_;
/**
* optional int32 target_index = 2;
* @return Whether the targetIndex field is set.
*/
@java.lang.Override
public boolean hasTargetIndex() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 target_index = 2;
* @return The targetIndex.
*/
@java.lang.Override
public int getTargetIndex() {
return targetIndex_;
}
public static final int COUNT_FIELD_NUMBER = 3;
private int count_;
/**
* optional int32 count = 3;
* @return Whether the count field is set.
*/
@java.lang.Override
public boolean hasCount() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional int32 count = 3;
* @return The count.
*/
@java.lang.Override
public int getCount() {
return count_;
}
public static final int USER_PAYLOAD_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString userPayload_;
/**
* optional bytes user_payload = 4;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bytes user_payload = 4;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
public static final int VERSION_FIELD_NUMBER = 5;
private int version_;
/**
* optional int32 version = 5;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional int32 version = 5;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, sourceIndex_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, targetIndex_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt32(3, count_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeBytes(4, userPayload_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt32(5, version_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, sourceIndex_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, targetIndex_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, count_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, userPayload_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, version_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto)) {
return super.equals(obj);
}
org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto other = (org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto) obj;
if (hasSourceIndex() != other.hasSourceIndex()) return false;
if (hasSourceIndex()) {
if (getSourceIndex()
!= other.getSourceIndex()) return false;
}
if (hasTargetIndex() != other.hasTargetIndex()) return false;
if (hasTargetIndex()) {
if (getTargetIndex()
!= other.getTargetIndex()) return false;
}
if (hasCount() != other.hasCount()) return false;
if (hasCount()) {
if (getCount()
!= other.getCount()) return false;
}
if (hasUserPayload() != other.hasUserPayload()) return false;
if (hasUserPayload()) {
if (!getUserPayload()
.equals(other.getUserPayload())) return false;
}
if (hasVersion() != other.hasVersion()) return false;
if (hasVersion()) {
if (getVersion()
!= other.getVersion()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasSourceIndex()) {
hash = (37 * hash) + SOURCE_INDEX_FIELD_NUMBER;
hash = (53 * hash) + getSourceIndex();
}
if (hasTargetIndex()) {
hash = (37 * hash) + TARGET_INDEX_FIELD_NUMBER;
hash = (53 * hash) + getTargetIndex();
}
if (hasCount()) {
hash = (37 * hash) + COUNT_FIELD_NUMBER;
hash = (53 * hash) + getCount();
}
if (hasUserPayload()) {
hash = (37 * hash) + USER_PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getUserPayload().hashCode();
}
if (hasVersion()) {
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code CompositeRoutedDataMovementEventProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:CompositeRoutedDataMovementEventProto)
org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CompositeRoutedDataMovementEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CompositeRoutedDataMovementEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto.class, org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto.Builder.class);
}
// Construct using org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
sourceIndex_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
targetIndex_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
count_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
userPayload_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
version_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CompositeRoutedDataMovementEventProto_descriptor;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto getDefaultInstanceForType() {
return org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto.getDefaultInstance();
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto build() {
org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto buildPartial() {
org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto result = new org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.sourceIndex_ = sourceIndex_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.targetIndex_ = targetIndex_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.count_ = count_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.userPayload_ = userPayload_;
if (((from_bitField0_ & 0x00000010) != 0)) {
result.version_ = version_;
to_bitField0_ |= 0x00000010;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto) {
return mergeFrom((org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto other) {
if (other == org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto.getDefaultInstance()) return this;
if (other.hasSourceIndex()) {
setSourceIndex(other.getSourceIndex());
}
if (other.hasTargetIndex()) {
setTargetIndex(other.getTargetIndex());
}
if (other.hasCount()) {
setCount(other.getCount());
}
if (other.hasUserPayload()) {
setUserPayload(other.getUserPayload());
}
if (other.hasVersion()) {
setVersion(other.getVersion());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int sourceIndex_ ;
/**
* optional int32 source_index = 1;
* @return Whether the sourceIndex field is set.
*/
@java.lang.Override
public boolean hasSourceIndex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 source_index = 1;
* @return The sourceIndex.
*/
@java.lang.Override
public int getSourceIndex() {
return sourceIndex_;
}
/**
* optional int32 source_index = 1;
* @param value The sourceIndex to set.
* @return This builder for chaining.
*/
public Builder setSourceIndex(int value) {
bitField0_ |= 0x00000001;
sourceIndex_ = value;
onChanged();
return this;
}
/**
* optional int32 source_index = 1;
* @return This builder for chaining.
*/
public Builder clearSourceIndex() {
bitField0_ = (bitField0_ & ~0x00000001);
sourceIndex_ = 0;
onChanged();
return this;
}
private int targetIndex_ ;
/**
* optional int32 target_index = 2;
* @return Whether the targetIndex field is set.
*/
@java.lang.Override
public boolean hasTargetIndex() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 target_index = 2;
* @return The targetIndex.
*/
@java.lang.Override
public int getTargetIndex() {
return targetIndex_;
}
/**
* optional int32 target_index = 2;
* @param value The targetIndex to set.
* @return This builder for chaining.
*/
public Builder setTargetIndex(int value) {
bitField0_ |= 0x00000002;
targetIndex_ = value;
onChanged();
return this;
}
/**
* optional int32 target_index = 2;
* @return This builder for chaining.
*/
public Builder clearTargetIndex() {
bitField0_ = (bitField0_ & ~0x00000002);
targetIndex_ = 0;
onChanged();
return this;
}
private int count_ ;
/**
* optional int32 count = 3;
* @return Whether the count field is set.
*/
@java.lang.Override
public boolean hasCount() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional int32 count = 3;
* @return The count.
*/
@java.lang.Override
public int getCount() {
return count_;
}
/**
* optional int32 count = 3;
* @param value The count to set.
* @return This builder for chaining.
*/
public Builder setCount(int value) {
bitField0_ |= 0x00000004;
count_ = value;
onChanged();
return this;
}
/**
* optional int32 count = 3;
* @return This builder for chaining.
*/
public Builder clearCount() {
bitField0_ = (bitField0_ & ~0x00000004);
count_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString userPayload_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes user_payload = 4;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bytes user_payload = 4;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
/**
* optional bytes user_payload = 4;
* @param value The userPayload to set.
* @return This builder for chaining.
*/
public Builder setUserPayload(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
userPayload_ = value;
onChanged();
return this;
}
/**
* optional bytes user_payload = 4;
* @return This builder for chaining.
*/
public Builder clearUserPayload() {
bitField0_ = (bitField0_ & ~0x00000008);
userPayload_ = getDefaultInstance().getUserPayload();
onChanged();
return this;
}
private int version_ ;
/**
* optional int32 version = 5;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional int32 version = 5;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
/**
* optional int32 version = 5;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(int value) {
bitField0_ |= 0x00000010;
version_ = value;
onChanged();
return this;
}
/**
* optional int32 version = 5;
* @return This builder for chaining.
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000010);
version_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:CompositeRoutedDataMovementEventProto)
}
// @@protoc_insertion_point(class_scope:CompositeRoutedDataMovementEventProto)
private static final org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto();
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CompositeRoutedDataMovementEventProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CompositeRoutedDataMovementEventProto(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.CompositeRoutedDataMovementEventProto getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InputReadErrorEventProtoOrBuilder extends
// @@protoc_insertion_point(interface_extends:InputReadErrorEventProto)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 index = 1;
* @return Whether the index field is set.
*/
boolean hasIndex();
/**
* optional int32 index = 1;
* @return The index.
*/
int getIndex();
/**
* optional string diagnostics = 2;
* @return Whether the diagnostics field is set.
*/
boolean hasDiagnostics();
/**
* optional string diagnostics = 2;
* @return The diagnostics.
*/
java.lang.String getDiagnostics();
/**
* optional string diagnostics = 2;
* @return The bytes for diagnostics.
*/
com.google.protobuf.ByteString
getDiagnosticsBytes();
/**
* optional int32 version = 3;
* @return Whether the version field is set.
*/
boolean hasVersion();
/**
* optional int32 version = 3;
* @return The version.
*/
int getVersion();
/**
* optional bool is_local_fetch = 4;
* @return Whether the isLocalFetch field is set.
*/
boolean hasIsLocalFetch();
/**
* optional bool is_local_fetch = 4;
* @return The isLocalFetch.
*/
boolean getIsLocalFetch();
/**
* optional bool is_disk_error_at_source = 5;
* @return Whether the isDiskErrorAtSource field is set.
*/
boolean hasIsDiskErrorAtSource();
/**
* optional bool is_disk_error_at_source = 5;
* @return The isDiskErrorAtSource.
*/
boolean getIsDiskErrorAtSource();
/**
* optional string destination_localhost_name = 6;
* @return Whether the destinationLocalhostName field is set.
*/
boolean hasDestinationLocalhostName();
/**
* optional string destination_localhost_name = 6;
* @return The destinationLocalhostName.
*/
java.lang.String getDestinationLocalhostName();
/**
* optional string destination_localhost_name = 6;
* @return The bytes for destinationLocalhostName.
*/
com.google.protobuf.ByteString
getDestinationLocalhostNameBytes();
}
/**
* Protobuf type {@code InputReadErrorEventProto}
*/
public static final class InputReadErrorEventProto extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:InputReadErrorEventProto)
InputReadErrorEventProtoOrBuilder {
private static final long serialVersionUID = 0L;
// Use InputReadErrorEventProto.newBuilder() to construct.
private InputReadErrorEventProto(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InputReadErrorEventProto() {
diagnostics_ = "";
destinationLocalhostName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new InputReadErrorEventProto();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private InputReadErrorEventProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
index_ = input.readInt32();
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
diagnostics_ = bs;
break;
}
case 24: {
bitField0_ |= 0x00000004;
version_ = input.readInt32();
break;
}
case 32: {
bitField0_ |= 0x00000008;
isLocalFetch_ = input.readBool();
break;
}
case 40: {
bitField0_ |= 0x00000010;
isDiskErrorAtSource_ = input.readBool();
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
destinationLocalhostName_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_InputReadErrorEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_InputReadErrorEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto.class, org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto.Builder.class);
}
private int bitField0_;
public static final int INDEX_FIELD_NUMBER = 1;
private int index_;
/**
* optional int32 index = 1;
* @return Whether the index field is set.
*/
@java.lang.Override
public boolean hasIndex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 index = 1;
* @return The index.
*/
@java.lang.Override
public int getIndex() {
return index_;
}
public static final int DIAGNOSTICS_FIELD_NUMBER = 2;
private volatile java.lang.Object diagnostics_;
/**
* optional string diagnostics = 2;
* @return Whether the diagnostics field is set.
*/
@java.lang.Override
public boolean hasDiagnostics() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string diagnostics = 2;
* @return The diagnostics.
*/
@java.lang.Override
public java.lang.String getDiagnostics() {
java.lang.Object ref = diagnostics_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
diagnostics_ = s;
}
return s;
}
}
/**
* optional string diagnostics = 2;
* @return The bytes for diagnostics.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDiagnosticsBytes() {
java.lang.Object ref = diagnostics_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
diagnostics_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VERSION_FIELD_NUMBER = 3;
private int version_;
/**
* optional int32 version = 3;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional int32 version = 3;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
public static final int IS_LOCAL_FETCH_FIELD_NUMBER = 4;
private boolean isLocalFetch_;
/**
* optional bool is_local_fetch = 4;
* @return Whether the isLocalFetch field is set.
*/
@java.lang.Override
public boolean hasIsLocalFetch() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bool is_local_fetch = 4;
* @return The isLocalFetch.
*/
@java.lang.Override
public boolean getIsLocalFetch() {
return isLocalFetch_;
}
public static final int IS_DISK_ERROR_AT_SOURCE_FIELD_NUMBER = 5;
private boolean isDiskErrorAtSource_;
/**
* optional bool is_disk_error_at_source = 5;
* @return Whether the isDiskErrorAtSource field is set.
*/
@java.lang.Override
public boolean hasIsDiskErrorAtSource() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional bool is_disk_error_at_source = 5;
* @return The isDiskErrorAtSource.
*/
@java.lang.Override
public boolean getIsDiskErrorAtSource() {
return isDiskErrorAtSource_;
}
public static final int DESTINATION_LOCALHOST_NAME_FIELD_NUMBER = 6;
private volatile java.lang.Object destinationLocalhostName_;
/**
* optional string destination_localhost_name = 6;
* @return Whether the destinationLocalhostName field is set.
*/
@java.lang.Override
public boolean hasDestinationLocalhostName() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional string destination_localhost_name = 6;
* @return The destinationLocalhostName.
*/
@java.lang.Override
public java.lang.String getDestinationLocalhostName() {
java.lang.Object ref = destinationLocalhostName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
destinationLocalhostName_ = s;
}
return s;
}
}
/**
* optional string destination_localhost_name = 6;
* @return The bytes for destinationLocalhostName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDestinationLocalhostNameBytes() {
java.lang.Object ref = destinationLocalhostName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
destinationLocalhostName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, index_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, diagnostics_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt32(3, version_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeBool(4, isLocalFetch_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeBool(5, isDiskErrorAtSource_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, destinationLocalhostName_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, index_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, diagnostics_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, version_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, isLocalFetch_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, isDiskErrorAtSource_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, destinationLocalhostName_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto)) {
return super.equals(obj);
}
org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto other = (org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto) obj;
if (hasIndex() != other.hasIndex()) return false;
if (hasIndex()) {
if (getIndex()
!= other.getIndex()) return false;
}
if (hasDiagnostics() != other.hasDiagnostics()) return false;
if (hasDiagnostics()) {
if (!getDiagnostics()
.equals(other.getDiagnostics())) return false;
}
if (hasVersion() != other.hasVersion()) return false;
if (hasVersion()) {
if (getVersion()
!= other.getVersion()) return false;
}
if (hasIsLocalFetch() != other.hasIsLocalFetch()) return false;
if (hasIsLocalFetch()) {
if (getIsLocalFetch()
!= other.getIsLocalFetch()) return false;
}
if (hasIsDiskErrorAtSource() != other.hasIsDiskErrorAtSource()) return false;
if (hasIsDiskErrorAtSource()) {
if (getIsDiskErrorAtSource()
!= other.getIsDiskErrorAtSource()) return false;
}
if (hasDestinationLocalhostName() != other.hasDestinationLocalhostName()) return false;
if (hasDestinationLocalhostName()) {
if (!getDestinationLocalhostName()
.equals(other.getDestinationLocalhostName())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasIndex()) {
hash = (37 * hash) + INDEX_FIELD_NUMBER;
hash = (53 * hash) + getIndex();
}
if (hasDiagnostics()) {
hash = (37 * hash) + DIAGNOSTICS_FIELD_NUMBER;
hash = (53 * hash) + getDiagnostics().hashCode();
}
if (hasVersion()) {
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion();
}
if (hasIsLocalFetch()) {
hash = (37 * hash) + IS_LOCAL_FETCH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsLocalFetch());
}
if (hasIsDiskErrorAtSource()) {
hash = (37 * hash) + IS_DISK_ERROR_AT_SOURCE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsDiskErrorAtSource());
}
if (hasDestinationLocalhostName()) {
hash = (37 * hash) + DESTINATION_LOCALHOST_NAME_FIELD_NUMBER;
hash = (53 * hash) + getDestinationLocalhostName().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code InputReadErrorEventProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:InputReadErrorEventProto)
org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_InputReadErrorEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_InputReadErrorEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto.class, org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto.Builder.class);
}
// Construct using org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
index_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
diagnostics_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
version_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
isLocalFetch_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
isDiskErrorAtSource_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
destinationLocalhostName_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_InputReadErrorEventProto_descriptor;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto getDefaultInstanceForType() {
return org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto.getDefaultInstance();
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto build() {
org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto buildPartial() {
org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto result = new org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.index_ = index_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.diagnostics_ = diagnostics_;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.version_ = version_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.isLocalFetch_ = isLocalFetch_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.isDiskErrorAtSource_ = isDiskErrorAtSource_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.destinationLocalhostName_ = destinationLocalhostName_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto) {
return mergeFrom((org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto other) {
if (other == org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto.getDefaultInstance()) return this;
if (other.hasIndex()) {
setIndex(other.getIndex());
}
if (other.hasDiagnostics()) {
bitField0_ |= 0x00000002;
diagnostics_ = other.diagnostics_;
onChanged();
}
if (other.hasVersion()) {
setVersion(other.getVersion());
}
if (other.hasIsLocalFetch()) {
setIsLocalFetch(other.getIsLocalFetch());
}
if (other.hasIsDiskErrorAtSource()) {
setIsDiskErrorAtSource(other.getIsDiskErrorAtSource());
}
if (other.hasDestinationLocalhostName()) {
bitField0_ |= 0x00000020;
destinationLocalhostName_ = other.destinationLocalhostName_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int index_ ;
/**
* optional int32 index = 1;
* @return Whether the index field is set.
*/
@java.lang.Override
public boolean hasIndex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 index = 1;
* @return The index.
*/
@java.lang.Override
public int getIndex() {
return index_;
}
/**
* optional int32 index = 1;
* @param value The index to set.
* @return This builder for chaining.
*/
public Builder setIndex(int value) {
bitField0_ |= 0x00000001;
index_ = value;
onChanged();
return this;
}
/**
* optional int32 index = 1;
* @return This builder for chaining.
*/
public Builder clearIndex() {
bitField0_ = (bitField0_ & ~0x00000001);
index_ = 0;
onChanged();
return this;
}
private java.lang.Object diagnostics_ = "";
/**
* optional string diagnostics = 2;
* @return Whether the diagnostics field is set.
*/
public boolean hasDiagnostics() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string diagnostics = 2;
* @return The diagnostics.
*/
public java.lang.String getDiagnostics() {
java.lang.Object ref = diagnostics_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
diagnostics_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string diagnostics = 2;
* @return The bytes for diagnostics.
*/
public com.google.protobuf.ByteString
getDiagnosticsBytes() {
java.lang.Object ref = diagnostics_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
diagnostics_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string diagnostics = 2;
* @param value The diagnostics to set.
* @return This builder for chaining.
*/
public Builder setDiagnostics(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
diagnostics_ = value;
onChanged();
return this;
}
/**
* optional string diagnostics = 2;
* @return This builder for chaining.
*/
public Builder clearDiagnostics() {
bitField0_ = (bitField0_ & ~0x00000002);
diagnostics_ = getDefaultInstance().getDiagnostics();
onChanged();
return this;
}
/**
* optional string diagnostics = 2;
* @param value The bytes for diagnostics to set.
* @return This builder for chaining.
*/
public Builder setDiagnosticsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
diagnostics_ = value;
onChanged();
return this;
}
private int version_ ;
/**
* optional int32 version = 3;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional int32 version = 3;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
/**
* optional int32 version = 3;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(int value) {
bitField0_ |= 0x00000004;
version_ = value;
onChanged();
return this;
}
/**
* optional int32 version = 3;
* @return This builder for chaining.
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000004);
version_ = 0;
onChanged();
return this;
}
private boolean isLocalFetch_ ;
/**
* optional bool is_local_fetch = 4;
* @return Whether the isLocalFetch field is set.
*/
@java.lang.Override
public boolean hasIsLocalFetch() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bool is_local_fetch = 4;
* @return The isLocalFetch.
*/
@java.lang.Override
public boolean getIsLocalFetch() {
return isLocalFetch_;
}
/**
* optional bool is_local_fetch = 4;
* @param value The isLocalFetch to set.
* @return This builder for chaining.
*/
public Builder setIsLocalFetch(boolean value) {
bitField0_ |= 0x00000008;
isLocalFetch_ = value;
onChanged();
return this;
}
/**
* optional bool is_local_fetch = 4;
* @return This builder for chaining.
*/
public Builder clearIsLocalFetch() {
bitField0_ = (bitField0_ & ~0x00000008);
isLocalFetch_ = false;
onChanged();
return this;
}
private boolean isDiskErrorAtSource_ ;
/**
* optional bool is_disk_error_at_source = 5;
* @return Whether the isDiskErrorAtSource field is set.
*/
@java.lang.Override
public boolean hasIsDiskErrorAtSource() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional bool is_disk_error_at_source = 5;
* @return The isDiskErrorAtSource.
*/
@java.lang.Override
public boolean getIsDiskErrorAtSource() {
return isDiskErrorAtSource_;
}
/**
* optional bool is_disk_error_at_source = 5;
* @param value The isDiskErrorAtSource to set.
* @return This builder for chaining.
*/
public Builder setIsDiskErrorAtSource(boolean value) {
bitField0_ |= 0x00000010;
isDiskErrorAtSource_ = value;
onChanged();
return this;
}
/**
* optional bool is_disk_error_at_source = 5;
* @return This builder for chaining.
*/
public Builder clearIsDiskErrorAtSource() {
bitField0_ = (bitField0_ & ~0x00000010);
isDiskErrorAtSource_ = false;
onChanged();
return this;
}
private java.lang.Object destinationLocalhostName_ = "";
/**
* optional string destination_localhost_name = 6;
* @return Whether the destinationLocalhostName field is set.
*/
public boolean hasDestinationLocalhostName() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional string destination_localhost_name = 6;
* @return The destinationLocalhostName.
*/
public java.lang.String getDestinationLocalhostName() {
java.lang.Object ref = destinationLocalhostName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
destinationLocalhostName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string destination_localhost_name = 6;
* @return The bytes for destinationLocalhostName.
*/
public com.google.protobuf.ByteString
getDestinationLocalhostNameBytes() {
java.lang.Object ref = destinationLocalhostName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
destinationLocalhostName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string destination_localhost_name = 6;
* @param value The destinationLocalhostName to set.
* @return This builder for chaining.
*/
public Builder setDestinationLocalhostName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
destinationLocalhostName_ = value;
onChanged();
return this;
}
/**
* optional string destination_localhost_name = 6;
* @return This builder for chaining.
*/
public Builder clearDestinationLocalhostName() {
bitField0_ = (bitField0_ & ~0x00000020);
destinationLocalhostName_ = getDefaultInstance().getDestinationLocalhostName();
onChanged();
return this;
}
/**
* optional string destination_localhost_name = 6;
* @param value The bytes for destinationLocalhostName to set.
* @return This builder for chaining.
*/
public Builder setDestinationLocalhostNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
destinationLocalhostName_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:InputReadErrorEventProto)
}
// @@protoc_insertion_point(class_scope:InputReadErrorEventProto)
private static final org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto();
}
public static org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public InputReadErrorEventProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InputReadErrorEventProto(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.InputReadErrorEventProto getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InputFailedEventProtoOrBuilder extends
// @@protoc_insertion_point(interface_extends:InputFailedEventProto)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 target_index = 1;
* @return Whether the targetIndex field is set.
*/
boolean hasTargetIndex();
/**
* optional int32 target_index = 1;
* @return The targetIndex.
*/
int getTargetIndex();
/**
* optional int32 version = 2;
* @return Whether the version field is set.
*/
boolean hasVersion();
/**
* optional int32 version = 2;
* @return The version.
*/
int getVersion();
}
/**
* Protobuf type {@code InputFailedEventProto}
*/
public static final class InputFailedEventProto extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:InputFailedEventProto)
InputFailedEventProtoOrBuilder {
private static final long serialVersionUID = 0L;
// Use InputFailedEventProto.newBuilder() to construct.
private InputFailedEventProto(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InputFailedEventProto() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new InputFailedEventProto();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private InputFailedEventProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
targetIndex_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
version_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_InputFailedEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_InputFailedEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto.class, org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto.Builder.class);
}
private int bitField0_;
public static final int TARGET_INDEX_FIELD_NUMBER = 1;
private int targetIndex_;
/**
* optional int32 target_index = 1;
* @return Whether the targetIndex field is set.
*/
@java.lang.Override
public boolean hasTargetIndex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 target_index = 1;
* @return The targetIndex.
*/
@java.lang.Override
public int getTargetIndex() {
return targetIndex_;
}
public static final int VERSION_FIELD_NUMBER = 2;
private int version_;
/**
* optional int32 version = 2;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 version = 2;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, targetIndex_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, version_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, targetIndex_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, version_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto)) {
return super.equals(obj);
}
org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto other = (org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto) obj;
if (hasTargetIndex() != other.hasTargetIndex()) return false;
if (hasTargetIndex()) {
if (getTargetIndex()
!= other.getTargetIndex()) return false;
}
if (hasVersion() != other.hasVersion()) return false;
if (hasVersion()) {
if (getVersion()
!= other.getVersion()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTargetIndex()) {
hash = (37 * hash) + TARGET_INDEX_FIELD_NUMBER;
hash = (53 * hash) + getTargetIndex();
}
if (hasVersion()) {
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code InputFailedEventProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:InputFailedEventProto)
org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_InputFailedEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_InputFailedEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto.class, org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto.Builder.class);
}
// Construct using org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
targetIndex_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
version_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_InputFailedEventProto_descriptor;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto getDefaultInstanceForType() {
return org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto.getDefaultInstance();
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto build() {
org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto buildPartial() {
org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto result = new org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.targetIndex_ = targetIndex_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.version_ = version_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto) {
return mergeFrom((org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto other) {
if (other == org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto.getDefaultInstance()) return this;
if (other.hasTargetIndex()) {
setTargetIndex(other.getTargetIndex());
}
if (other.hasVersion()) {
setVersion(other.getVersion());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int targetIndex_ ;
/**
* optional int32 target_index = 1;
* @return Whether the targetIndex field is set.
*/
@java.lang.Override
public boolean hasTargetIndex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 target_index = 1;
* @return The targetIndex.
*/
@java.lang.Override
public int getTargetIndex() {
return targetIndex_;
}
/**
* optional int32 target_index = 1;
* @param value The targetIndex to set.
* @return This builder for chaining.
*/
public Builder setTargetIndex(int value) {
bitField0_ |= 0x00000001;
targetIndex_ = value;
onChanged();
return this;
}
/**
* optional int32 target_index = 1;
* @return This builder for chaining.
*/
public Builder clearTargetIndex() {
bitField0_ = (bitField0_ & ~0x00000001);
targetIndex_ = 0;
onChanged();
return this;
}
private int version_ ;
/**
* optional int32 version = 2;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 version = 2;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
/**
* optional int32 version = 2;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(int value) {
bitField0_ |= 0x00000002;
version_ = value;
onChanged();
return this;
}
/**
* optional int32 version = 2;
* @return This builder for chaining.
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000002);
version_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:InputFailedEventProto)
}
// @@protoc_insertion_point(class_scope:InputFailedEventProto)
private static final org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto();
}
public static org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public InputFailedEventProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InputFailedEventProto(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.InputFailedEventProto getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface VertexManagerEventProtoOrBuilder extends
// @@protoc_insertion_point(interface_extends:VertexManagerEventProto)
com.google.protobuf.MessageOrBuilder {
/**
* optional string target_vertex_name = 1;
* @return Whether the targetVertexName field is set.
*/
boolean hasTargetVertexName();
/**
* optional string target_vertex_name = 1;
* @return The targetVertexName.
*/
java.lang.String getTargetVertexName();
/**
* optional string target_vertex_name = 1;
* @return The bytes for targetVertexName.
*/
com.google.protobuf.ByteString
getTargetVertexNameBytes();
/**
* optional bytes user_payload = 2;
* @return Whether the userPayload field is set.
*/
boolean hasUserPayload();
/**
* optional bytes user_payload = 2;
* @return The userPayload.
*/
com.google.protobuf.ByteString getUserPayload();
}
/**
* Protobuf type {@code VertexManagerEventProto}
*/
public static final class VertexManagerEventProto extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:VertexManagerEventProto)
VertexManagerEventProtoOrBuilder {
private static final long serialVersionUID = 0L;
// Use VertexManagerEventProto.newBuilder() to construct.
private VertexManagerEventProto(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private VertexManagerEventProto() {
targetVertexName_ = "";
userPayload_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new VertexManagerEventProto();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private VertexManagerEventProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
targetVertexName_ = bs;
break;
}
case 18: {
bitField0_ |= 0x00000002;
userPayload_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_VertexManagerEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_VertexManagerEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto.class, org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto.Builder.class);
}
private int bitField0_;
public static final int TARGET_VERTEX_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object targetVertexName_;
/**
* optional string target_vertex_name = 1;
* @return Whether the targetVertexName field is set.
*/
@java.lang.Override
public boolean hasTargetVertexName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string target_vertex_name = 1;
* @return The targetVertexName.
*/
@java.lang.Override
public java.lang.String getTargetVertexName() {
java.lang.Object ref = targetVertexName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
targetVertexName_ = s;
}
return s;
}
}
/**
* optional string target_vertex_name = 1;
* @return The bytes for targetVertexName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTargetVertexNameBytes() {
java.lang.Object ref = targetVertexName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
targetVertexName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int USER_PAYLOAD_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString userPayload_;
/**
* optional bytes user_payload = 2;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes user_payload = 2;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, targetVertexName_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, userPayload_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, targetVertexName_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, userPayload_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto)) {
return super.equals(obj);
}
org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto other = (org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto) obj;
if (hasTargetVertexName() != other.hasTargetVertexName()) return false;
if (hasTargetVertexName()) {
if (!getTargetVertexName()
.equals(other.getTargetVertexName())) return false;
}
if (hasUserPayload() != other.hasUserPayload()) return false;
if (hasUserPayload()) {
if (!getUserPayload()
.equals(other.getUserPayload())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTargetVertexName()) {
hash = (37 * hash) + TARGET_VERTEX_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTargetVertexName().hashCode();
}
if (hasUserPayload()) {
hash = (37 * hash) + USER_PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getUserPayload().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code VertexManagerEventProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:VertexManagerEventProto)
org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_VertexManagerEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_VertexManagerEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto.class, org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto.Builder.class);
}
// Construct using org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
targetVertexName_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
userPayload_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_VertexManagerEventProto_descriptor;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto getDefaultInstanceForType() {
return org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto.getDefaultInstance();
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto build() {
org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto buildPartial() {
org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto result = new org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.targetVertexName_ = targetVertexName_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.userPayload_ = userPayload_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto) {
return mergeFrom((org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto other) {
if (other == org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto.getDefaultInstance()) return this;
if (other.hasTargetVertexName()) {
bitField0_ |= 0x00000001;
targetVertexName_ = other.targetVertexName_;
onChanged();
}
if (other.hasUserPayload()) {
setUserPayload(other.getUserPayload());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object targetVertexName_ = "";
/**
* optional string target_vertex_name = 1;
* @return Whether the targetVertexName field is set.
*/
public boolean hasTargetVertexName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string target_vertex_name = 1;
* @return The targetVertexName.
*/
public java.lang.String getTargetVertexName() {
java.lang.Object ref = targetVertexName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
targetVertexName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string target_vertex_name = 1;
* @return The bytes for targetVertexName.
*/
public com.google.protobuf.ByteString
getTargetVertexNameBytes() {
java.lang.Object ref = targetVertexName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
targetVertexName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string target_vertex_name = 1;
* @param value The targetVertexName to set.
* @return This builder for chaining.
*/
public Builder setTargetVertexName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
targetVertexName_ = value;
onChanged();
return this;
}
/**
* optional string target_vertex_name = 1;
* @return This builder for chaining.
*/
public Builder clearTargetVertexName() {
bitField0_ = (bitField0_ & ~0x00000001);
targetVertexName_ = getDefaultInstance().getTargetVertexName();
onChanged();
return this;
}
/**
* optional string target_vertex_name = 1;
* @param value The bytes for targetVertexName to set.
* @return This builder for chaining.
*/
public Builder setTargetVertexNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
targetVertexName_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString userPayload_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes user_payload = 2;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes user_payload = 2;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
/**
* optional bytes user_payload = 2;
* @param value The userPayload to set.
* @return This builder for chaining.
*/
public Builder setUserPayload(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
userPayload_ = value;
onChanged();
return this;
}
/**
* optional bytes user_payload = 2;
* @return This builder for chaining.
*/
public Builder clearUserPayload() {
bitField0_ = (bitField0_ & ~0x00000002);
userPayload_ = getDefaultInstance().getUserPayload();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:VertexManagerEventProto)
}
// @@protoc_insertion_point(class_scope:VertexManagerEventProto)
private static final org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto();
}
public static org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public VertexManagerEventProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new VertexManagerEventProto(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.VertexManagerEventProto getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RootInputDataInformationEventProtoOrBuilder extends
// @@protoc_insertion_point(interface_extends:RootInputDataInformationEventProto)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 source_index = 1;
* @return Whether the sourceIndex field is set.
*/
boolean hasSourceIndex();
/**
* optional int32 source_index = 1;
* @return The sourceIndex.
*/
int getSourceIndex();
/**
* optional int32 target_index = 2;
* @return Whether the targetIndex field is set.
*/
boolean hasTargetIndex();
/**
* optional int32 target_index = 2;
* @return The targetIndex.
*/
int getTargetIndex();
/**
* optional bytes user_payload = 3;
* @return Whether the userPayload field is set.
*/
boolean hasUserPayload();
/**
* optional bytes user_payload = 3;
* @return The userPayload.
*/
com.google.protobuf.ByteString getUserPayload();
}
/**
* Protobuf type {@code RootInputDataInformationEventProto}
*/
public static final class RootInputDataInformationEventProto extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:RootInputDataInformationEventProto)
RootInputDataInformationEventProtoOrBuilder {
private static final long serialVersionUID = 0L;
// Use RootInputDataInformationEventProto.newBuilder() to construct.
private RootInputDataInformationEventProto(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RootInputDataInformationEventProto() {
userPayload_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RootInputDataInformationEventProto();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RootInputDataInformationEventProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
sourceIndex_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
targetIndex_ = input.readInt32();
break;
}
case 26: {
bitField0_ |= 0x00000004;
userPayload_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_RootInputDataInformationEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_RootInputDataInformationEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto.class, org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto.Builder.class);
}
private int bitField0_;
public static final int SOURCE_INDEX_FIELD_NUMBER = 1;
private int sourceIndex_;
/**
* optional int32 source_index = 1;
* @return Whether the sourceIndex field is set.
*/
@java.lang.Override
public boolean hasSourceIndex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 source_index = 1;
* @return The sourceIndex.
*/
@java.lang.Override
public int getSourceIndex() {
return sourceIndex_;
}
public static final int TARGET_INDEX_FIELD_NUMBER = 2;
private int targetIndex_;
/**
* optional int32 target_index = 2;
* @return Whether the targetIndex field is set.
*/
@java.lang.Override
public boolean hasTargetIndex() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 target_index = 2;
* @return The targetIndex.
*/
@java.lang.Override
public int getTargetIndex() {
return targetIndex_;
}
public static final int USER_PAYLOAD_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString userPayload_;
/**
* optional bytes user_payload = 3;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes user_payload = 3;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, sourceIndex_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, targetIndex_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(3, userPayload_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, sourceIndex_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, targetIndex_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, userPayload_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto)) {
return super.equals(obj);
}
org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto other = (org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto) obj;
if (hasSourceIndex() != other.hasSourceIndex()) return false;
if (hasSourceIndex()) {
if (getSourceIndex()
!= other.getSourceIndex()) return false;
}
if (hasTargetIndex() != other.hasTargetIndex()) return false;
if (hasTargetIndex()) {
if (getTargetIndex()
!= other.getTargetIndex()) return false;
}
if (hasUserPayload() != other.hasUserPayload()) return false;
if (hasUserPayload()) {
if (!getUserPayload()
.equals(other.getUserPayload())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasSourceIndex()) {
hash = (37 * hash) + SOURCE_INDEX_FIELD_NUMBER;
hash = (53 * hash) + getSourceIndex();
}
if (hasTargetIndex()) {
hash = (37 * hash) + TARGET_INDEX_FIELD_NUMBER;
hash = (53 * hash) + getTargetIndex();
}
if (hasUserPayload()) {
hash = (37 * hash) + USER_PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getUserPayload().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RootInputDataInformationEventProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:RootInputDataInformationEventProto)
org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_RootInputDataInformationEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_RootInputDataInformationEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto.class, org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto.Builder.class);
}
// Construct using org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
sourceIndex_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
targetIndex_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
userPayload_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_RootInputDataInformationEventProto_descriptor;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto getDefaultInstanceForType() {
return org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto.getDefaultInstance();
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto build() {
org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto buildPartial() {
org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto result = new org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.sourceIndex_ = sourceIndex_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.targetIndex_ = targetIndex_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.userPayload_ = userPayload_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto) {
return mergeFrom((org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto other) {
if (other == org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto.getDefaultInstance()) return this;
if (other.hasSourceIndex()) {
setSourceIndex(other.getSourceIndex());
}
if (other.hasTargetIndex()) {
setTargetIndex(other.getTargetIndex());
}
if (other.hasUserPayload()) {
setUserPayload(other.getUserPayload());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int sourceIndex_ ;
/**
* optional int32 source_index = 1;
* @return Whether the sourceIndex field is set.
*/
@java.lang.Override
public boolean hasSourceIndex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 source_index = 1;
* @return The sourceIndex.
*/
@java.lang.Override
public int getSourceIndex() {
return sourceIndex_;
}
/**
* optional int32 source_index = 1;
* @param value The sourceIndex to set.
* @return This builder for chaining.
*/
public Builder setSourceIndex(int value) {
bitField0_ |= 0x00000001;
sourceIndex_ = value;
onChanged();
return this;
}
/**
* optional int32 source_index = 1;
* @return This builder for chaining.
*/
public Builder clearSourceIndex() {
bitField0_ = (bitField0_ & ~0x00000001);
sourceIndex_ = 0;
onChanged();
return this;
}
private int targetIndex_ ;
/**
* optional int32 target_index = 2;
* @return Whether the targetIndex field is set.
*/
@java.lang.Override
public boolean hasTargetIndex() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 target_index = 2;
* @return The targetIndex.
*/
@java.lang.Override
public int getTargetIndex() {
return targetIndex_;
}
/**
* optional int32 target_index = 2;
* @param value The targetIndex to set.
* @return This builder for chaining.
*/
public Builder setTargetIndex(int value) {
bitField0_ |= 0x00000002;
targetIndex_ = value;
onChanged();
return this;
}
/**
* optional int32 target_index = 2;
* @return This builder for chaining.
*/
public Builder clearTargetIndex() {
bitField0_ = (bitField0_ & ~0x00000002);
targetIndex_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString userPayload_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes user_payload = 3;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes user_payload = 3;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
/**
* optional bytes user_payload = 3;
* @param value The userPayload to set.
* @return This builder for chaining.
*/
public Builder setUserPayload(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
userPayload_ = value;
onChanged();
return this;
}
/**
* optional bytes user_payload = 3;
* @return This builder for chaining.
*/
public Builder clearUserPayload() {
bitField0_ = (bitField0_ & ~0x00000004);
userPayload_ = getDefaultInstance().getUserPayload();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:RootInputDataInformationEventProto)
}
// @@protoc_insertion_point(class_scope:RootInputDataInformationEventProto)
private static final org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto();
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RootInputDataInformationEventProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RootInputDataInformationEventProto(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.RootInputDataInformationEventProto getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CompositeEventProtoOrBuilder extends
// @@protoc_insertion_point(interface_extends:CompositeEventProto)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 start_index = 1;
* @return Whether the startIndex field is set.
*/
boolean hasStartIndex();
/**
* optional int32 start_index = 1;
* @return The startIndex.
*/
int getStartIndex();
/**
* optional int32 count = 2;
* @return Whether the count field is set.
*/
boolean hasCount();
/**
* optional int32 count = 2;
* @return The count.
*/
int getCount();
/**
* optional bytes user_payload = 3;
* @return Whether the userPayload field is set.
*/
boolean hasUserPayload();
/**
* optional bytes user_payload = 3;
* @return The userPayload.
*/
com.google.protobuf.ByteString getUserPayload();
/**
* optional int32 version = 4;
* @return Whether the version field is set.
*/
boolean hasVersion();
/**
* optional int32 version = 4;
* @return The version.
*/
int getVersion();
}
/**
* Protobuf type {@code CompositeEventProto}
*/
public static final class CompositeEventProto extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:CompositeEventProto)
CompositeEventProtoOrBuilder {
private static final long serialVersionUID = 0L;
// Use CompositeEventProto.newBuilder() to construct.
private CompositeEventProto(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CompositeEventProto() {
userPayload_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CompositeEventProto();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CompositeEventProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
startIndex_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
count_ = input.readInt32();
break;
}
case 26: {
bitField0_ |= 0x00000004;
userPayload_ = input.readBytes();
break;
}
case 32: {
bitField0_ |= 0x00000008;
version_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CompositeEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CompositeEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto.class, org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto.Builder.class);
}
private int bitField0_;
public static final int START_INDEX_FIELD_NUMBER = 1;
private int startIndex_;
/**
* optional int32 start_index = 1;
* @return Whether the startIndex field is set.
*/
@java.lang.Override
public boolean hasStartIndex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 start_index = 1;
* @return The startIndex.
*/
@java.lang.Override
public int getStartIndex() {
return startIndex_;
}
public static final int COUNT_FIELD_NUMBER = 2;
private int count_;
/**
* optional int32 count = 2;
* @return Whether the count field is set.
*/
@java.lang.Override
public boolean hasCount() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 count = 2;
* @return The count.
*/
@java.lang.Override
public int getCount() {
return count_;
}
public static final int USER_PAYLOAD_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString userPayload_;
/**
* optional bytes user_payload = 3;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes user_payload = 3;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
public static final int VERSION_FIELD_NUMBER = 4;
private int version_;
/**
* optional int32 version = 4;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional int32 version = 4;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, startIndex_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, count_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(3, userPayload_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt32(4, version_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, startIndex_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, count_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, userPayload_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, version_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto)) {
return super.equals(obj);
}
org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto other = (org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto) obj;
if (hasStartIndex() != other.hasStartIndex()) return false;
if (hasStartIndex()) {
if (getStartIndex()
!= other.getStartIndex()) return false;
}
if (hasCount() != other.hasCount()) return false;
if (hasCount()) {
if (getCount()
!= other.getCount()) return false;
}
if (hasUserPayload() != other.hasUserPayload()) return false;
if (hasUserPayload()) {
if (!getUserPayload()
.equals(other.getUserPayload())) return false;
}
if (hasVersion() != other.hasVersion()) return false;
if (hasVersion()) {
if (getVersion()
!= other.getVersion()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStartIndex()) {
hash = (37 * hash) + START_INDEX_FIELD_NUMBER;
hash = (53 * hash) + getStartIndex();
}
if (hasCount()) {
hash = (37 * hash) + COUNT_FIELD_NUMBER;
hash = (53 * hash) + getCount();
}
if (hasUserPayload()) {
hash = (37 * hash) + USER_PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getUserPayload().hashCode();
}
if (hasVersion()) {
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code CompositeEventProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:CompositeEventProto)
org.apache.tez.runtime.api.events.EventProtos.CompositeEventProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CompositeEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CompositeEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto.class, org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto.Builder.class);
}
// Construct using org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
startIndex_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
count_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
userPayload_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
version_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CompositeEventProto_descriptor;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto getDefaultInstanceForType() {
return org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto.getDefaultInstance();
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto build() {
org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto buildPartial() {
org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto result = new org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.startIndex_ = startIndex_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.count_ = count_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.userPayload_ = userPayload_;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.version_ = version_;
to_bitField0_ |= 0x00000008;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto) {
return mergeFrom((org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto other) {
if (other == org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto.getDefaultInstance()) return this;
if (other.hasStartIndex()) {
setStartIndex(other.getStartIndex());
}
if (other.hasCount()) {
setCount(other.getCount());
}
if (other.hasUserPayload()) {
setUserPayload(other.getUserPayload());
}
if (other.hasVersion()) {
setVersion(other.getVersion());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int startIndex_ ;
/**
* optional int32 start_index = 1;
* @return Whether the startIndex field is set.
*/
@java.lang.Override
public boolean hasStartIndex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 start_index = 1;
* @return The startIndex.
*/
@java.lang.Override
public int getStartIndex() {
return startIndex_;
}
/**
* optional int32 start_index = 1;
* @param value The startIndex to set.
* @return This builder for chaining.
*/
public Builder setStartIndex(int value) {
bitField0_ |= 0x00000001;
startIndex_ = value;
onChanged();
return this;
}
/**
* optional int32 start_index = 1;
* @return This builder for chaining.
*/
public Builder clearStartIndex() {
bitField0_ = (bitField0_ & ~0x00000001);
startIndex_ = 0;
onChanged();
return this;
}
private int count_ ;
/**
* optional int32 count = 2;
* @return Whether the count field is set.
*/
@java.lang.Override
public boolean hasCount() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 count = 2;
* @return The count.
*/
@java.lang.Override
public int getCount() {
return count_;
}
/**
* optional int32 count = 2;
* @param value The count to set.
* @return This builder for chaining.
*/
public Builder setCount(int value) {
bitField0_ |= 0x00000002;
count_ = value;
onChanged();
return this;
}
/**
* optional int32 count = 2;
* @return This builder for chaining.
*/
public Builder clearCount() {
bitField0_ = (bitField0_ & ~0x00000002);
count_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString userPayload_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes user_payload = 3;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes user_payload = 3;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
/**
* optional bytes user_payload = 3;
* @param value The userPayload to set.
* @return This builder for chaining.
*/
public Builder setUserPayload(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
userPayload_ = value;
onChanged();
return this;
}
/**
* optional bytes user_payload = 3;
* @return This builder for chaining.
*/
public Builder clearUserPayload() {
bitField0_ = (bitField0_ & ~0x00000004);
userPayload_ = getDefaultInstance().getUserPayload();
onChanged();
return this;
}
private int version_ ;
/**
* optional int32 version = 4;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional int32 version = 4;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
/**
* optional int32 version = 4;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(int value) {
bitField0_ |= 0x00000008;
version_ = value;
onChanged();
return this;
}
/**
* optional int32 version = 4;
* @return This builder for chaining.
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000008);
version_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:CompositeEventProto)
}
// @@protoc_insertion_point(class_scope:CompositeEventProto)
private static final org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto();
}
public static org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CompositeEventProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CompositeEventProto(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.CompositeEventProto getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RootInputInitializerEventProtoOrBuilder extends
// @@protoc_insertion_point(interface_extends:RootInputInitializerEventProto)
com.google.protobuf.MessageOrBuilder {
/**
* optional string target_vertex_name = 1;
* @return Whether the targetVertexName field is set.
*/
boolean hasTargetVertexName();
/**
* optional string target_vertex_name = 1;
* @return The targetVertexName.
*/
java.lang.String getTargetVertexName();
/**
* optional string target_vertex_name = 1;
* @return The bytes for targetVertexName.
*/
com.google.protobuf.ByteString
getTargetVertexNameBytes();
/**
* optional string target_input_name = 2;
* @return Whether the targetInputName field is set.
*/
boolean hasTargetInputName();
/**
* optional string target_input_name = 2;
* @return The targetInputName.
*/
java.lang.String getTargetInputName();
/**
* optional string target_input_name = 2;
* @return The bytes for targetInputName.
*/
com.google.protobuf.ByteString
getTargetInputNameBytes();
/**
* optional bytes user_payload = 3;
* @return Whether the userPayload field is set.
*/
boolean hasUserPayload();
/**
* optional bytes user_payload = 3;
* @return The userPayload.
*/
com.google.protobuf.ByteString getUserPayload();
}
/**
* Protobuf type {@code RootInputInitializerEventProto}
*/
public static final class RootInputInitializerEventProto extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:RootInputInitializerEventProto)
RootInputInitializerEventProtoOrBuilder {
private static final long serialVersionUID = 0L;
// Use RootInputInitializerEventProto.newBuilder() to construct.
private RootInputInitializerEventProto(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RootInputInitializerEventProto() {
targetVertexName_ = "";
targetInputName_ = "";
userPayload_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RootInputInitializerEventProto();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RootInputInitializerEventProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
targetVertexName_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
targetInputName_ = bs;
break;
}
case 26: {
bitField0_ |= 0x00000004;
userPayload_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_RootInputInitializerEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_RootInputInitializerEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto.class, org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto.Builder.class);
}
private int bitField0_;
public static final int TARGET_VERTEX_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object targetVertexName_;
/**
* optional string target_vertex_name = 1;
* @return Whether the targetVertexName field is set.
*/
@java.lang.Override
public boolean hasTargetVertexName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string target_vertex_name = 1;
* @return The targetVertexName.
*/
@java.lang.Override
public java.lang.String getTargetVertexName() {
java.lang.Object ref = targetVertexName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
targetVertexName_ = s;
}
return s;
}
}
/**
* optional string target_vertex_name = 1;
* @return The bytes for targetVertexName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTargetVertexNameBytes() {
java.lang.Object ref = targetVertexName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
targetVertexName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TARGET_INPUT_NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object targetInputName_;
/**
* optional string target_input_name = 2;
* @return Whether the targetInputName field is set.
*/
@java.lang.Override
public boolean hasTargetInputName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string target_input_name = 2;
* @return The targetInputName.
*/
@java.lang.Override
public java.lang.String getTargetInputName() {
java.lang.Object ref = targetInputName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
targetInputName_ = s;
}
return s;
}
}
/**
* optional string target_input_name = 2;
* @return The bytes for targetInputName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTargetInputNameBytes() {
java.lang.Object ref = targetInputName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
targetInputName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int USER_PAYLOAD_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString userPayload_;
/**
* optional bytes user_payload = 3;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes user_payload = 3;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, targetVertexName_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, targetInputName_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(3, userPayload_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, targetVertexName_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, targetInputName_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, userPayload_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto)) {
return super.equals(obj);
}
org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto other = (org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto) obj;
if (hasTargetVertexName() != other.hasTargetVertexName()) return false;
if (hasTargetVertexName()) {
if (!getTargetVertexName()
.equals(other.getTargetVertexName())) return false;
}
if (hasTargetInputName() != other.hasTargetInputName()) return false;
if (hasTargetInputName()) {
if (!getTargetInputName()
.equals(other.getTargetInputName())) return false;
}
if (hasUserPayload() != other.hasUserPayload()) return false;
if (hasUserPayload()) {
if (!getUserPayload()
.equals(other.getUserPayload())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTargetVertexName()) {
hash = (37 * hash) + TARGET_VERTEX_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTargetVertexName().hashCode();
}
if (hasTargetInputName()) {
hash = (37 * hash) + TARGET_INPUT_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTargetInputName().hashCode();
}
if (hasUserPayload()) {
hash = (37 * hash) + USER_PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getUserPayload().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RootInputInitializerEventProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:RootInputInitializerEventProto)
org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_RootInputInitializerEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_RootInputInitializerEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto.class, org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto.Builder.class);
}
// Construct using org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
targetVertexName_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
targetInputName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
userPayload_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_RootInputInitializerEventProto_descriptor;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto getDefaultInstanceForType() {
return org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto.getDefaultInstance();
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto build() {
org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto buildPartial() {
org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto result = new org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.targetVertexName_ = targetVertexName_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.targetInputName_ = targetInputName_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.userPayload_ = userPayload_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto) {
return mergeFrom((org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto other) {
if (other == org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto.getDefaultInstance()) return this;
if (other.hasTargetVertexName()) {
bitField0_ |= 0x00000001;
targetVertexName_ = other.targetVertexName_;
onChanged();
}
if (other.hasTargetInputName()) {
bitField0_ |= 0x00000002;
targetInputName_ = other.targetInputName_;
onChanged();
}
if (other.hasUserPayload()) {
setUserPayload(other.getUserPayload());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object targetVertexName_ = "";
/**
* optional string target_vertex_name = 1;
* @return Whether the targetVertexName field is set.
*/
public boolean hasTargetVertexName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string target_vertex_name = 1;
* @return The targetVertexName.
*/
public java.lang.String getTargetVertexName() {
java.lang.Object ref = targetVertexName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
targetVertexName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string target_vertex_name = 1;
* @return The bytes for targetVertexName.
*/
public com.google.protobuf.ByteString
getTargetVertexNameBytes() {
java.lang.Object ref = targetVertexName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
targetVertexName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string target_vertex_name = 1;
* @param value The targetVertexName to set.
* @return This builder for chaining.
*/
public Builder setTargetVertexName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
targetVertexName_ = value;
onChanged();
return this;
}
/**
* optional string target_vertex_name = 1;
* @return This builder for chaining.
*/
public Builder clearTargetVertexName() {
bitField0_ = (bitField0_ & ~0x00000001);
targetVertexName_ = getDefaultInstance().getTargetVertexName();
onChanged();
return this;
}
/**
* optional string target_vertex_name = 1;
* @param value The bytes for targetVertexName to set.
* @return This builder for chaining.
*/
public Builder setTargetVertexNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
targetVertexName_ = value;
onChanged();
return this;
}
private java.lang.Object targetInputName_ = "";
/**
* optional string target_input_name = 2;
* @return Whether the targetInputName field is set.
*/
public boolean hasTargetInputName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string target_input_name = 2;
* @return The targetInputName.
*/
public java.lang.String getTargetInputName() {
java.lang.Object ref = targetInputName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
targetInputName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string target_input_name = 2;
* @return The bytes for targetInputName.
*/
public com.google.protobuf.ByteString
getTargetInputNameBytes() {
java.lang.Object ref = targetInputName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
targetInputName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string target_input_name = 2;
* @param value The targetInputName to set.
* @return This builder for chaining.
*/
public Builder setTargetInputName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
targetInputName_ = value;
onChanged();
return this;
}
/**
* optional string target_input_name = 2;
* @return This builder for chaining.
*/
public Builder clearTargetInputName() {
bitField0_ = (bitField0_ & ~0x00000002);
targetInputName_ = getDefaultInstance().getTargetInputName();
onChanged();
return this;
}
/**
* optional string target_input_name = 2;
* @param value The bytes for targetInputName to set.
* @return This builder for chaining.
*/
public Builder setTargetInputNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
targetInputName_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString userPayload_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes user_payload = 3;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes user_payload = 3;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
/**
* optional bytes user_payload = 3;
* @param value The userPayload to set.
* @return This builder for chaining.
*/
public Builder setUserPayload(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
userPayload_ = value;
onChanged();
return this;
}
/**
* optional bytes user_payload = 3;
* @return This builder for chaining.
*/
public Builder clearUserPayload() {
bitField0_ = (bitField0_ & ~0x00000004);
userPayload_ = getDefaultInstance().getUserPayload();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:RootInputInitializerEventProto)
}
// @@protoc_insertion_point(class_scope:RootInputInitializerEventProto)
private static final org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto();
}
public static org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RootInputInitializerEventProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RootInputInitializerEventProto(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.RootInputInitializerEventProto getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CustomProcessorEventProtoOrBuilder extends
// @@protoc_insertion_point(interface_extends:CustomProcessorEventProto)
com.google.protobuf.MessageOrBuilder {
/**
* optional bytes user_payload = 1;
* @return Whether the userPayload field is set.
*/
boolean hasUserPayload();
/**
* optional bytes user_payload = 1;
* @return The userPayload.
*/
com.google.protobuf.ByteString getUserPayload();
/**
* required int32 version = 2;
* @return Whether the version field is set.
*/
boolean hasVersion();
/**
* required int32 version = 2;
* @return The version.
*/
int getVersion();
}
/**
* Protobuf type {@code CustomProcessorEventProto}
*/
public static final class CustomProcessorEventProto extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:CustomProcessorEventProto)
CustomProcessorEventProtoOrBuilder {
private static final long serialVersionUID = 0L;
// Use CustomProcessorEventProto.newBuilder() to construct.
private CustomProcessorEventProto(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CustomProcessorEventProto() {
userPayload_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CustomProcessorEventProto();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CustomProcessorEventProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
bitField0_ |= 0x00000001;
userPayload_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
version_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CustomProcessorEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CustomProcessorEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto.class, org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto.Builder.class);
}
private int bitField0_;
public static final int USER_PAYLOAD_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString userPayload_;
/**
* optional bytes user_payload = 1;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional bytes user_payload = 1;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
public static final int VERSION_FIELD_NUMBER = 2;
private int version_;
/**
* required int32 version = 2;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required int32 version = 2;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasVersion()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, userPayload_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, version_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, userPayload_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, version_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto)) {
return super.equals(obj);
}
org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto other = (org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto) obj;
if (hasUserPayload() != other.hasUserPayload()) return false;
if (hasUserPayload()) {
if (!getUserPayload()
.equals(other.getUserPayload())) return false;
}
if (hasVersion() != other.hasVersion()) return false;
if (hasVersion()) {
if (getVersion()
!= other.getVersion()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasUserPayload()) {
hash = (37 * hash) + USER_PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getUserPayload().hashCode();
}
if (hasVersion()) {
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code CustomProcessorEventProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:CustomProcessorEventProto)
org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CustomProcessorEventProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CustomProcessorEventProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto.class, org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto.Builder.class);
}
// Construct using org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
userPayload_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
version_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.tez.runtime.api.events.EventProtos.internal_static_CustomProcessorEventProto_descriptor;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto getDefaultInstanceForType() {
return org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto.getDefaultInstance();
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto build() {
org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto buildPartial() {
org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto result = new org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.userPayload_ = userPayload_;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.version_ = version_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto) {
return mergeFrom((org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto other) {
if (other == org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto.getDefaultInstance()) return this;
if (other.hasUserPayload()) {
setUserPayload(other.getUserPayload());
}
if (other.hasVersion()) {
setVersion(other.getVersion());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasVersion()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString userPayload_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes user_payload = 1;
* @return Whether the userPayload field is set.
*/
@java.lang.Override
public boolean hasUserPayload() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional bytes user_payload = 1;
* @return The userPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUserPayload() {
return userPayload_;
}
/**
* optional bytes user_payload = 1;
* @param value The userPayload to set.
* @return This builder for chaining.
*/
public Builder setUserPayload(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
userPayload_ = value;
onChanged();
return this;
}
/**
* optional bytes user_payload = 1;
* @return This builder for chaining.
*/
public Builder clearUserPayload() {
bitField0_ = (bitField0_ & ~0x00000001);
userPayload_ = getDefaultInstance().getUserPayload();
onChanged();
return this;
}
private int version_ ;
/**
* required int32 version = 2;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required int32 version = 2;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
/**
* required int32 version = 2;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(int value) {
bitField0_ |= 0x00000002;
version_ = value;
onChanged();
return this;
}
/**
* required int32 version = 2;
* @return This builder for chaining.
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000002);
version_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:CustomProcessorEventProto)
}
// @@protoc_insertion_point(class_scope:CustomProcessorEventProto)
private static final org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto();
}
public static org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CustomProcessorEventProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CustomProcessorEventProto(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.tez.runtime.api.events.EventProtos.CustomProcessorEventProto getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_DataMovementEventProto_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_DataMovementEventProto_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_CompositeRoutedDataMovementEventProto_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_CompositeRoutedDataMovementEventProto_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_InputReadErrorEventProto_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_InputReadErrorEventProto_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_InputFailedEventProto_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_InputFailedEventProto_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_VertexManagerEventProto_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_VertexManagerEventProto_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RootInputDataInformationEventProto_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_RootInputDataInformationEventProto_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_CompositeEventProto_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_CompositeEventProto_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RootInputInitializerEventProto_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_RootInputInitializerEventProto_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_CustomProcessorEventProto_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_CustomProcessorEventProto_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\014Events.proto\"k\n\026DataMovementEventProto" +
"\022\024\n\014source_index\030\001 \001(\005\022\024\n\014target_index\030\002" +
" \001(\005\022\024\n\014user_payload\030\003 \001(\014\022\017\n\007version\030\004 " +
"\001(\005\"\211\001\n%CompositeRoutedDataMovementEvent" +
"Proto\022\024\n\014source_index\030\001 \001(\005\022\024\n\014target_in" +
"dex\030\002 \001(\005\022\r\n\005count\030\003 \001(\005\022\024\n\014user_payload" +
"\030\004 \001(\014\022\017\n\007version\030\005 \001(\005\"\254\001\n\030InputReadErr" +
"orEventProto\022\r\n\005index\030\001 \001(\005\022\023\n\013diagnosti" +
"cs\030\002 \001(\t\022\017\n\007version\030\003 \001(\005\022\026\n\016is_local_fe" +
"tch\030\004 \001(\010\022\037\n\027is_disk_error_at_source\030\005 \001" +
"(\010\022\"\n\032destination_localhost_name\030\006 \001(\t\">" +
"\n\025InputFailedEventProto\022\024\n\014target_index\030" +
"\001 \001(\005\022\017\n\007version\030\002 \001(\005\"K\n\027VertexManagerE" +
"ventProto\022\032\n\022target_vertex_name\030\001 \001(\t\022\024\n" +
"\014user_payload\030\002 \001(\014\"f\n\"RootInputDataInfo" +
"rmationEventProto\022\024\n\014source_index\030\001 \001(\005\022" +
"\024\n\014target_index\030\002 \001(\005\022\024\n\014user_payload\030\003 " +
"\001(\014\"`\n\023CompositeEventProto\022\023\n\013start_inde" +
"x\030\001 \001(\005\022\r\n\005count\030\002 \001(\005\022\024\n\014user_payload\030\003" +
" \001(\014\022\017\n\007version\030\004 \001(\005\"m\n\036RootInputInitia" +
"lizerEventProto\022\032\n\022target_vertex_name\030\001 " +
"\001(\t\022\031\n\021target_input_name\030\002 \001(\t\022\024\n\014user_p" +
"ayload\030\003 \001(\014\"B\n\031CustomProcessorEventProt" +
"o\022\024\n\014user_payload\030\001 \001(\014\022\017\n\007version\030\002 \002(\005" +
"B3\n!org.apache.tez.runtime.api.eventsB\013E" +
"ventProtos\240\001\001"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_DataMovementEventProto_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_DataMovementEventProto_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_DataMovementEventProto_descriptor,
new java.lang.String[] { "SourceIndex", "TargetIndex", "UserPayload", "Version", });
internal_static_CompositeRoutedDataMovementEventProto_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_CompositeRoutedDataMovementEventProto_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_CompositeRoutedDataMovementEventProto_descriptor,
new java.lang.String[] { "SourceIndex", "TargetIndex", "Count", "UserPayload", "Version", });
internal_static_InputReadErrorEventProto_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_InputReadErrorEventProto_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_InputReadErrorEventProto_descriptor,
new java.lang.String[] { "Index", "Diagnostics", "Version", "IsLocalFetch", "IsDiskErrorAtSource", "DestinationLocalhostName", });
internal_static_InputFailedEventProto_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_InputFailedEventProto_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_InputFailedEventProto_descriptor,
new java.lang.String[] { "TargetIndex", "Version", });
internal_static_VertexManagerEventProto_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_VertexManagerEventProto_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_VertexManagerEventProto_descriptor,
new java.lang.String[] { "TargetVertexName", "UserPayload", });
internal_static_RootInputDataInformationEventProto_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_RootInputDataInformationEventProto_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_RootInputDataInformationEventProto_descriptor,
new java.lang.String[] { "SourceIndex", "TargetIndex", "UserPayload", });
internal_static_CompositeEventProto_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_CompositeEventProto_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_CompositeEventProto_descriptor,
new java.lang.String[] { "StartIndex", "Count", "UserPayload", "Version", });
internal_static_RootInputInitializerEventProto_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_RootInputInitializerEventProto_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_RootInputInitializerEventProto_descriptor,
new java.lang.String[] { "TargetVertexName", "TargetInputName", "UserPayload", });
internal_static_CustomProcessorEventProto_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_CustomProcessorEventProto_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_CustomProcessorEventProto_descriptor,
new java.lang.String[] { "UserPayload", "Version", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy