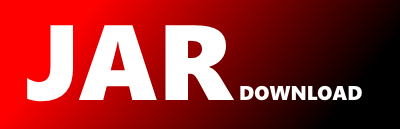
org.apache.thrift.protocol.TProtocol Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libthrift Show documentation
Show all versions of libthrift Show documentation
Thrift is a software framework for scalable cross-language services development.
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.thrift.protocol;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.IntFunction;
import org.apache.thrift.TException;
import org.apache.thrift.partial.TFieldData;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.transport.TTransport;
/** Protocol interface definition. */
public abstract class TProtocol implements TWriteProtocol, TReadProtocol {
/** Prevent direct instantiation */
@SuppressWarnings("unused")
private TProtocol() {}
/** Transport */
protected TTransport trans_;
/** Constructor */
protected TProtocol(TTransport trans) {
trans_ = trans;
}
/** Transport accessor */
public TTransport getTransport() {
return trans_;
}
protected void checkReadBytesAvailable(TMap map) throws TException {
long elemSize = getMinSerializedSize(map.keyType) + getMinSerializedSize(map.valueType);
trans_.checkReadBytesAvailable(map.size * elemSize);
}
protected void checkReadBytesAvailable(TList list) throws TException {
long size = list.getSize();
trans_.checkReadBytesAvailable(size * getMinSerializedSize(list.elemType));
}
protected void checkReadBytesAvailable(TSet set) throws TException {
long size = set.getSize();
trans_.checkReadBytesAvailable(size * getMinSerializedSize(set.elemType));
}
/**
* Return min serialized size in bytes
*
* @param type Returns the minimum amount of bytes needed to store the smallest possible instance
* of TType.
* @return min serialized size
* @throws TException when error happens
*/
public abstract int getMinSerializedSize(byte type) throws TException;
public interface WriteCallback {
void call(T e) throws TException;
}
public interface ReadCallback {
R accept(T t) throws TException;
}
public interface ReadCollectionCallback {
R call() throws TException;
}
public interface ReadMapEntryCallback {
K getKey() throws TException;
V getValue() throws TException;
}
public final void writeSet(byte elementType, Set set, WriteCallback callback)
throws TException {
writeSetBegin(new TSet(elementType, set.size()));
for (T t : set) {
callback.call(t);
}
writeSetEnd();
}
public final void writeList(byte elementType, List list, WriteCallback callback)
throws TException {
writeListBegin(new TList(elementType, list.size()));
for (T t : list) {
callback.call(t);
}
writeListEnd();
}
public final void writeMap(
byte keyType, byte valueType, Map map, WriteCallback> callback)
throws TException {
writeMapBegin(new TMap(keyType, valueType, map.size()));
for (Map.Entry entry : map.entrySet()) {
callback.call(entry);
}
writeMapEnd();
}
public final void writeField(TField field, WriteCallback callback) throws TException {
writeFieldBegin(field);
callback.call(null);
writeFieldEnd();
}
public final void writeStruct(TStruct struct, WriteCallback callback) throws TException {
writeStructBegin(struct);
callback.call(null);
writeStructEnd();
}
public final void writeMessage(TMessage message, WriteCallback callback) throws TException {
writeMessageBegin(message);
callback.call(null);
writeMessageEnd();
}
/**
* read a message by delegating to a callback, handles {@link #readMessageBegin() begin} and
* {@link #readMessageEnd() end} automatically.
*
* @param callback callback for actual reading
* @param result message type
* @return the message read
* @throws TException when any sub-operation failed
*/
public final T readMessage(ReadCallback callback) throws TException {
TMessage tMessage = readMessageBegin();
T t = callback.accept(tMessage);
readMessageEnd();
return t;
}
/**
* read a struct by delegating to a callback, handles {@link #readStructBegin() begin} and {@link
* #readStructEnd() end} automatically.
*
* @param callback callback for actual reading
* @param result struct type
* @return the struct read
* @throws TException when any sub-operation failed
*/
public final T readStruct(ReadCallback callback) throws TException {
TStruct tStruct = readStructBegin();
T t = callback.accept(tStruct);
readStructEnd();
return t;
}
/**
* read a field by delegating to a callback, handles {@link #readFieldBegin() begin} and {@link
* #readFieldEnd() end} automatically, and returns whether the {@link TType#STOP stop signal} was
* encountered. Because the value is not returned, you (the compiler generated code in most cases)
* are expected to set the field yourself within the callback.
*
* @param callback callback for reading a field
* @param result field type
* @return true if a stop signal was encountered, false otherwise
* @throws Exception when any sub-operation failed
*/
public final boolean readField(ReadCallback callback) throws Exception {
TField tField = readFieldBegin();
if (tField.type == org.apache.thrift.protocol.TType.STOP) {
return true;
}
callback.accept(tField);
readFieldEnd();
return false;
}
/**
* read a {@link Map} of elements by delegating to the callback, handles {@link #readMapBegin()
* begin} and {@link #readMapEnd() end} automatically.
*
* @param callback callback for reading the map
* @param result map type
* @return the map read
* @throws TException when any sub-operation fails
*/
public final > T readMap(ReadCallback callback) throws TException {
TMap tMap = readMapBegin();
T t = callback.accept(tMap);
readMapEnd();
return t;
}
/**
* read a {@link Map} of elements by delegating key and value reading to the callback, handles
* {@link #readMapBegin() begin} and {@link #readMapEnd() end} automatically.
*
* @param callback callback for reading keys and values, calls to {@link
* ReadMapEntryCallback#getKey()} and {@link ReadMapEntryCallback#getValue()} will be in
* alternating orders, i.e. k1, v1, k2, v2, .., k_n, v_n
* @param key type
* @param value type
* @return the map read
* @throws TException when any sub-operation fails
*/
public final Map readMap(ReadMapEntryCallback callback) throws TException {
return readMap(callback, HashMap::new);
}
/**
* read a {@link Map} of elements by delegating key and value reading to the callback, handles
* {@link #readMapBegin() begin} and {@link #readMapEnd() end} automatically, with a specialized
* map creator given the size hint.
*
* @param callback callback for reading keys and values, calls to {@link
* ReadMapEntryCallback#getKey()} and {@link ReadMapEntryCallback#getValue()} will be in
* alternating orders, i.e. k1, v1, k2, v2, .., k_n, v_n
* @param mapCreator map creator given the size hint
* @param key type
* @param value type
* @return the map read
* @throws TException when any sub-operation fails
*/
public final Map readMap(
ReadMapEntryCallback callback, IntFunction
© 2015 - 2024 Weber Informatics LLC | Privacy Policy