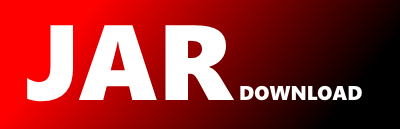
org.apache.tiles.servlet.context.ServletRequestScopeMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tiles-servlet Show documentation
Show all versions of tiles-servlet Show documentation
Tiles servlet support, to enable use of Tiles inside a Servlet environment.
/*
* $Id: ServletRequestScopeMap.java 581985 2007-10-04 18:57:15Z apetrelli $
*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.tiles.servlet.context;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Enumeration;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.servlet.ServletRequest;
import org.apache.tiles.context.MapEntry;
/**
* Private implementation of Map
for servlet request
* attributes.
*
* @version $Rev: 581985 $ $Date: 2007-10-04 20:57:15 +0200 (gio, 04 ott 2007) $
*/
final class ServletRequestScopeMap implements Map {
/**
* Constructor.
*
* @param request The request object to use.
*/
public ServletRequestScopeMap(ServletRequest request) {
this.request = request;
}
/**
* The request object to use.
*/
private ServletRequest request = null;
/** {@inheritDoc} */
public void clear() {
Iterator keys = keySet().iterator();
while (keys.hasNext()) {
request.removeAttribute(keys.next());
}
}
/** {@inheritDoc} */
public boolean containsKey(Object key) {
return (request.getAttribute(key(key)) != null);
}
/** {@inheritDoc} */
@SuppressWarnings("unchecked")
public boolean containsValue(Object value) {
if (value == null) {
return (false);
}
Enumeration keys = request.getAttributeNames();
while (keys.hasMoreElements()) {
Object next = request.getAttribute(keys.nextElement());
if (next == value) {
return (true);
}
}
return (false);
}
/** {@inheritDoc} */
@SuppressWarnings("unchecked")
public Set> entrySet() {
Set> set = new HashSet>();
Enumeration keys = request.getAttributeNames();
String key;
while (keys.hasMoreElements()) {
key = keys.nextElement();
set.add(new MapEntry(key,
request.getAttribute(key), true));
}
return (set);
}
/** {@inheritDoc} */
@SuppressWarnings("unchecked")
public boolean equals(Object o) {
ServletRequest otherRequest = ((ServletRequestScopeMap) o).request;
boolean retValue = true;
synchronized (request) {
for (Enumeration attribs = request.getAttributeNames(); attribs
.hasMoreElements()
&& retValue;) {
String attributeName = attribs.nextElement();
retValue = request.getAttribute(attributeName).equals(
otherRequest.getAttribute(attributeName));
}
}
return retValue;
}
/** {@inheritDoc} */
public Object get(Object key) {
return (request.getAttribute(key(key)));
}
/** {@inheritDoc} */
public int hashCode() {
return (request.hashCode());
}
/** {@inheritDoc} */
public boolean isEmpty() {
return (size() < 1);
}
/** {@inheritDoc} */
@SuppressWarnings("unchecked")
public Set keySet() {
Set set = new HashSet();
Enumeration keys = request.getAttributeNames();
while (keys.hasMoreElements()) {
set.add(keys.nextElement());
}
return (set);
}
/** {@inheritDoc} */
public Object put(String key, Object value) {
if (value == null) {
return (remove(key));
}
String skey = key(key);
Object previous = request.getAttribute(skey);
request.setAttribute(skey, value);
return (previous);
}
/** {@inheritDoc} */
public void putAll(Map extends String, ? extends Object> map) {
Iterator extends String> keys = map.keySet().iterator();
while (keys.hasNext()) {
String key = keys.next();
request.setAttribute(key, map.get(key));
}
}
/** {@inheritDoc} */
public Object remove(Object key) {
String skey = key(key);
Object previous = request.getAttribute(skey);
request.removeAttribute(skey);
return (previous);
}
/** {@inheritDoc} */
@SuppressWarnings("unchecked")
public int size() {
int n = 0;
Enumeration keys = request.getAttributeNames();
while (keys.hasMoreElements()) {
keys.nextElement();
n++;
}
return (n);
}
/** {@inheritDoc} */
@SuppressWarnings("unchecked")
public Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy