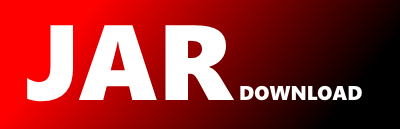
org.apache.tiles.template.PutAttributeModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tiles-template Show documentation
Show all versions of tiles-template Show documentation
Common code for integration of Tiles for different templating technologies.
/*
* $Id: PutAttributeModel.java 1305937 2012-03-27 18:15:15Z nlebas $
*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.tiles.template;
import java.io.IOException;
import java.util.Deque;
import org.apache.tiles.Attribute;
import org.apache.tiles.AttributeContext;
import org.apache.tiles.Expression;
import org.apache.tiles.TilesContainer;
import org.apache.tiles.access.TilesAccess;
import org.apache.tiles.autotag.core.runtime.ModelBody;
import org.apache.tiles.autotag.core.runtime.annotation.Parameter;
import org.apache.tiles.request.Request;
/**
*
* Put an attribute in enclosing attribute container tag.
*
*
* Enclosing attribute container tag can be :
*
* - <initContainer>
* - <definition>
* - <insertAttribute>
* - <insertDefinition>
* - <putListAttribute>
*
* (or any other tag which implements the PutAttributeTagParent
* interface. Exception is thrown if no appropriate tag can be found.
*
*
* Put tag can have following atributes :
*
* - name : Name of the attribute
* - value : value to put as attribute
* - type : value type. Possible type are : string (value is used as direct
* string), template (value is used as a page url to insert), definition (value
* is used as a definition name to insert), object (value is used as it is)
* - role : Role to check when 'insertAttribute' will be called.
*
*
*
* Value can also come from tag body. Tag body is taken into account only if
* value is not set by one of the tag attributes. In this case Attribute type is
* "string", unless tag body define another type.
*
*
* @version $Rev: 1305937 $ $Date: 2012-03-27 20:15:15 +0200 (Tue, 27 Mar 2012) $
* @since 2.2.0
*/
public class PutAttributeModel {
/**
* Executes the operation.
* @param name The name of the attribute to put.
* @param value The value of the attribute. Use this parameter, or
* expression, or body.
* @param expression The expression to calculate the value from. Use this
* parameter, or value, or body.
* @param role A comma-separated list of roles. If present, the attribute
* will be rendered only if the current user belongs to one of the roles.
* @param type The type (renderer) of the attribute.
* @param cascade If true
the attribute will be cascaded to all nested attributes.
* @param request The request.
* @param modelBody The body.
* @throws IOException If the body cannot be evaluated.
* @since 2.2.0
*/
public void execute(@Parameter(required = true) String name, Object value,
String expression, String role, String type, boolean cascade,
Request request, ModelBody modelBody) throws IOException {
Deque
© 2015 - 2024 Weber Informatics LLC | Privacy Policy