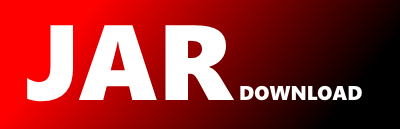
org.apache.tinkerpop.gremlin.process.AbstractGremlinProcessTest Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.tinkerpop.gremlin.process;
import org.apache.tinkerpop.gremlin.AbstractGremlinTest;
import org.apache.tinkerpop.gremlin.GraphManager;
import org.apache.tinkerpop.gremlin.process.traversal.Traversal;
import org.apache.tinkerpop.gremlin.process.traversal.TraversalEngine;
import org.apache.tinkerpop.gremlin.process.traversal.TraversalSideEffects;
import org.apache.tinkerpop.gremlin.process.traversal.step.util.MapHelper;
import org.apache.tinkerpop.gremlin.structure.util.StringFactory;
import org.junit.Before;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import java.util.stream.Collectors;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertFalse;
import static org.junit.Assert.assertTrue;
import static org.junit.Assume.assumeTrue;
/**
* Base test class for Gremlin Process tests.
*
* @author Stephen Mallette (http://stephen.genoprime.com)
*/
public abstract class AbstractGremlinProcessTest extends AbstractGremlinTest {
private static final Logger logger = LoggerFactory.getLogger(AbstractGremlinProcessTest.class);
/**
* Determines if a graph meets requirements for execution. All gremlin process tests should check this
* method as part of a call to {@code assumeTrue} to ensure that the test doesn't require the computer
* feature or if it does require the computer feature then ensure that the graph being tested supports it.
*/
protected boolean graphMeetsTestRequirements() {
return !hasGraphComputerRequirement() || graph.features().graph().supportsComputer();
}
/**
* Determines if this test suite has "computer" requirements.
*/
protected boolean hasGraphComputerRequirement() {
// do the negation of STANDARD as we expect a future type of REASONING that would infer COMPUTER support
return !GraphManager.getTraversalEngineType().equals(TraversalEngine.Type.STANDARD);
}
@Before
public void setupTest() {
assumeTrue(graphMeetsTestRequirements());
try {
// ignore tests that aren't supported by a specific TraversalEngine
final IgnoreEngine ignoreEngine = this.getClass().getMethod(name.getMethodName()).getAnnotation(IgnoreEngine.class);
if (ignoreEngine != null)
assumeTrue(String.format("This test is ignored for %s", ignoreEngine.value()), !ignoreEngine.value().equals(GraphManager.getTraversalEngineType()));
} catch (NoSuchMethodException nsme) {
throw new RuntimeException(String.format("Could not find test method %s in test case %s", name.getMethodName(), this.getClass().getName()));
}
}
public static void checkSideEffects(final TraversalSideEffects sideEffects, final Object... keysClasses) {
int counter = 0;
for (int i = 0; i < keysClasses.length; i = i + 2) {
counter++;
final String key = (String) keysClasses[i];
final Class clazz = (Class) keysClasses[i + 1];
assertTrue(sideEffects.keys().contains(key));
assertTrue(sideEffects.exists(key));
assertEquals(clazz, sideEffects.get((String) keysClasses[i]).getClass());
assertFalse(sideEffects.exists(UUID.randomUUID().toString()));
}
assertEquals(sideEffects.keys().size(), counter);
assertFalse(sideEffects.keys().contains(UUID.randomUUID().toString()));
assertEquals(StringFactory.traversalSideEffectsString(sideEffects), sideEffects.toString());
}
public static void checkResults(final List expectedResults, final Traversal, T> traversal) {
final List results = traversal.toList();
assertFalse(traversal.hasNext());
if (expectedResults.size() != results.size()) {
logger.error("Expected results: " + expectedResults);
logger.error("Actual results: " + results);
assertEquals("Checking result size", expectedResults.size(), results.size());
}
for (T t : results) {
if (t instanceof Map) {
assertTrue("Checking map result existence: " + t, expectedResults.stream().filter(e -> e instanceof Map).filter(e -> internalCheckMap((Map) e, (Map) t)).findAny().isPresent());
} else {
assertTrue("Checking result existence: " + t, expectedResults.contains(t));
}
}
final Map expectedResultsCount = new HashMap<>();
final Map resultsCount = new HashMap<>();
assertEquals("Checking indexing is equivalent", expectedResultsCount.size(), resultsCount.size());
expectedResults.forEach(t -> MapHelper.incr(expectedResultsCount, t, 1l));
results.forEach(t -> MapHelper.incr(resultsCount, t, 1l));
expectedResultsCount.forEach((k, v) -> assertEquals("Checking result group counts", v, resultsCount.get(k)));
assertFalse(traversal.hasNext());
}
public static void checkResults(final Map expectedResults, final Traversal, T> traversal) {
final List list = new ArrayList<>();
expectedResults.forEach((k, v) -> {
for (int i = 0; i < v; i++) {
list.add(k);
}
});
checkResults(list, traversal);
}
public static void checkMap(final Map expectedMap, final Map actualMap) {
final List> actualList = actualMap.entrySet().stream().sorted((a, b) -> a.getKey().toString().compareTo(b.getKey().toString())).collect(Collectors.toList());
final List> expectedList = expectedMap.entrySet().stream().sorted((a, b) -> a.getKey().toString().compareTo(b.getKey().toString())).collect(Collectors.toList());
assertEquals(expectedList.size(), actualList.size());
for (int i = 0; i < actualList.size(); i++) {
assertEquals(expectedList.get(i).getKey(), actualList.get(i).getKey());
assertEquals(expectedList.get(i).getValue(), actualList.get(i).getValue());
}
}
private static boolean internalCheckMap(final Map expectedMap, final Map actualMap) {
final List> actualList = actualMap.entrySet().stream().sorted((a, b) -> a.getKey().toString().compareTo(b.getKey().toString())).collect(Collectors.toList());
final List> expectedList = expectedMap.entrySet().stream().sorted((a, b) -> a.getKey().toString().compareTo(b.getKey().toString())).collect(Collectors.toList());
if (expectedList.size() != actualList.size()) {
return false;
}
for (int i = 0; i < actualList.size(); i++) {
if (!actualList.get(i).getKey().equals(expectedList.get(i).getKey())) {
return false;
}
if (!actualList.get(i).getValue().equals(expectedList.get(i).getValue())) {
return false;
}
}
return true;
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy