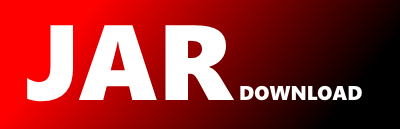
org.apache.webbeans.component.creation.ProducerMethodBeansBuilder Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.webbeans.component.creation;
import org.apache.webbeans.component.InjectionTargetBean;
import org.apache.webbeans.component.ProducerFieldBean;
import org.apache.webbeans.component.ProducerMethodBean;
import org.apache.webbeans.config.WebBeansContext;
import org.apache.webbeans.exception.WebBeansConfigurationException;
import org.apache.webbeans.util.AnnotationUtil;
import org.apache.webbeans.util.Asserts;
import org.apache.webbeans.util.WebBeansUtil;
import javax.enterprise.event.Observes;
import javax.enterprise.inject.Disposes;
import javax.enterprise.inject.Produces;
import javax.enterprise.inject.Specializes;
import javax.enterprise.inject.spi.AnnotatedMethod;
import javax.enterprise.inject.spi.AnnotatedType;
import javax.enterprise.inject.spi.BeanAttributes;
import javax.inject.Inject;
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
/**
* @param bean class type
*/
public class ProducerMethodBeansBuilder extends AbstractBeanBuilder
{
protected final WebBeansContext webBeansContext;
protected final AnnotatedType annotatedType;
/**
* Creates a new instance.
*
*/
public ProducerMethodBeansBuilder(WebBeansContext webBeansContext, AnnotatedType annotatedType)
{
Asserts.assertNotNull(webBeansContext, Asserts.PARAM_NAME_WEBBEANSCONTEXT);
Asserts.assertNotNull(annotatedType, "annotated type");
this.webBeansContext = webBeansContext;
this.annotatedType = annotatedType;
}
/**
* {@inheritDoc}
*/
public Set> defineProducerMethods(InjectionTargetBean bean, Set> producerFields)
{
final Set> producerBeans = new HashSet>();
final Set> annotatedMethods = webBeansContext.getAnnotatedElementFactory().getFilteredAnnotatedMethods(annotatedType);
final Collection> skipMethods = new HashSet>();
for(AnnotatedMethod super T> annotatedMethod: annotatedMethods)
{
final boolean isProducer = annotatedMethod.isAnnotationPresent(Produces.class);
if(isProducer &&
annotatedMethod.getJavaMember().getDeclaringClass().equals(annotatedType.getJavaClass()))
{
checkProducerMethodForDeployment(annotatedMethod);
boolean specialize = false;
if(annotatedMethod.isAnnotationPresent(Specializes.class))
{
if (annotatedMethod.isStatic())
{
throw new WebBeansConfigurationException("Specializing annotated producer method : " + annotatedMethod + " can not be static");
}
specialize = true;
}
final AnnotatedMethod method = (AnnotatedMethod) annotatedMethod;
final BeanAttributes beanAttributes = webBeansContext.getWebBeansUtil().fireProcessBeanAttributes(
annotatedMethod, annotatedMethod.getJavaMember().getReturnType(),
BeanAttributesBuilder.forContext(webBeansContext).newBeanAttibutes(method).build());
if (beanAttributes != null)
{
ProducerMethodBeanBuilder producerMethodBeanCreator = new ProducerMethodBeanBuilder(bean, annotatedMethod, beanAttributes);
ProducerMethodBean producerMethodBean = producerMethodBeanCreator.getBean();
//X TODO validateProxyable returns the exception, throw the returned exception??
webBeansContext.getDeploymentValidationService().validateProxyable(producerMethodBean);
producerMethodBeanCreator.validate();
if(specialize)
{
producerMethodBeanCreator.configureProducerSpecialization(producerMethodBean, (AnnotatedMethod) annotatedMethod);
}
producerMethodBean.setCreatorMethod(annotatedMethod.getJavaMember());
webBeansContext.getWebBeansUtil().setBeanEnableFlagForProducerBean(bean,
producerMethodBean,
AnnotationUtil.asArray(annotatedMethod.getAnnotations()));
WebBeansUtil.checkProducerGenericType(producerMethodBean, annotatedMethod.getJavaMember());
producerBeans.add(producerMethodBean);
}
}
else if (isProducer)
{
skipMethods.add(annotatedMethod);
}
}
// valid all @Disposes have a @Produces
validateNoDisposerWithoutProducer(annotatedMethods, producerBeans, producerFields, skipMethods);
return producerBeans;
}
/**
* Check producer method is ok for deployment.
*
* @param annotatedMethod producer method
*/
private void checkProducerMethodForDeployment(AnnotatedMethod super T> annotatedMethod)
{
Asserts.assertNotNull(annotatedMethod, "annotatedMethod argument");
if (annotatedMethod.isAnnotationPresent(Inject.class) ||
annotatedMethod.isAnnotationPresent(Disposes.class) ||
annotatedMethod.isAnnotationPresent(Observes.class))
{
throw new WebBeansConfigurationException("Producer annotated method : " + annotatedMethod + " can not be annotated with"
+ " @Initializer/@Destructor annotation or has a parameter annotated with @Disposes/@Observes");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy