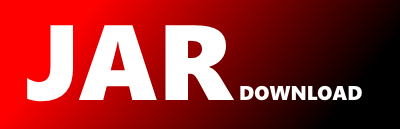
om.BaseManager.vm Maven / Gradle / Ivy
## Licensed to the Apache Software Foundation (ASF) under one
## or more contributor license agreements. See the NOTICE file
## distributed with this work for additional information
## regarding copyright ownership. The ASF licenses this file
## to you under the Apache License, Version 2.0 (the
## "License"); you may not use this file except in compliance
## with the License. You may obtain a copy of the License at
##
## http://www.apache.org/licenses/LICENSE-2.0
##
## Unless required by applicable law or agreed to in writing,
## software distributed under the License is distributed on an
## "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
## KIND, either express or implied. See the License for the
## specific language governing permissions and limitations
## under the License.
#*
* author John McNally
* author Thomas Vandahl
* version $Id: BaseManager.vm 503310 2007-02-03 21:05:45Z tv $
*#
#set ($interfaceName = $table.JavaName)
#if ($table.Interface)
#set($lastdotpos = $table.Interface.lastIndexOf('.'))
#if($lastdotpos != -1)
#set ($lastdotpos = $lastdotpos + 1)
#set ($interfaceName = $table.Interface.substring($lastdotpos))
#else
#set ($interfaceName = $table.Interface)
#end
#end
package ${packageBaseManager};
import java.math.BigDecimal;
import java.util.Date;
import java.util.List;
import org.apache.torque.Torque;
import org.apache.torque.TorqueException;
import org.apache.torque.manager.AbstractBaseManager;
import org.apache.torque.manager.CacheListener;
import org.apache.torque.manager.MethodResultCache;
import org.apache.torque.om.ObjectKey;
import org.apache.torque.om.SimpleKey;
import org.apache.torque.om.Persistent;
import org.apache.torque.util.Criteria;
#if ($packageBaseManager != $packageManager)
import ${packageManager}.*;
#end
#if ($packageBaseManager != $packagePeer)
import ${packagePeer}.*;
#end
#if ($packageBaseManager != $packageObject)
import ${packageObject}.*;
#end
#if ($table.Interface)
#set($lastdotpos = $table.Interface.lastIndexOf('.'))
#if($lastdotpos != -1)
import ${table.Interface};
#end
#end
/**
* This class manages $interfaceName objects.
#if ($table.description)
*
* $!table.description
*
#end
* This class was autogenerated by Torque #if ($addTimeStamp)on:
*
* [$now]
*
#end
*
* You should not use this class directly. It should not even be
* extended all references should be to ${interfaceName}Manager
*/
public abstract class $basePrefix${interfaceName}Manager
extends AbstractBaseManager
{
#if ($addTimeStamp)
/** Serial version */
private static final long serialVersionUID = ${now.Time}L;
#end
/** The name of the manager */
protected static final String MANAGED_CLASS = "${packageObject}.${table.JavaName}";
/** The name of our class to pass to Torque as the default manager. */
protected static final String DEFAULT_MANAGER_CLASS
= "${packageManager}.${interfaceName}Manager";
/**
* Retrieves an implementation of the manager, based on the settings in
* the configuration.
*
* @return an implementation of ${interfaceName}Manager.
*/
public static ${interfaceName}Manager getManager()
{
return (${interfaceName}Manager)
Torque.getManager(${interfaceName}Manager.MANAGED_CLASS,
${interfaceName}Manager.DEFAULT_MANAGER_CLASS);
}
/**
* Static accessor for the @see #getInstanceImpl().
*
* @return a ${interfaceName}
value
* @exception TorqueException if an error occurs
*/
public static ${interfaceName} getInstance()
throws TorqueException
{
return getManager().getInstanceImpl();
}
/**
* Static accessor for the @see #getInstanceImpl(ObjectKey).
*
* @param id an ObjectKey
value
* @return a ${interfaceName}
value
* @exception TorqueException if an error occurs
*/
public static ${interfaceName} getInstance(ObjectKey id)
throws TorqueException
{
return getManager().getInstanceImpl(id);
}
/**
* Static accessor for the @see #getCachedInstanceImpl(ObjectKey).
* Loads ${interfaceName}
from cache, returns
* null
, if instance is not in cache
*
* @param id an ObjectKey
value
* @return a ${interfaceName}
value
* @exception TorqueException if an error occurs
*/
public static ${interfaceName} getCachedInstance(ObjectKey id)
throws TorqueException
{
return getManager().getCachedInstanceImpl(id);
}
/**
* Static accessor for the @see #getInstanceImpl(ObjectKey, boolean).
*
* @param id an ObjectKey
value
* @param fromCache if true, look for cached ${interfaceName}s before loading
* from storage.
* @return a ${interfaceName}
value
* @exception TorqueException if an error occurs
*/
public static ${interfaceName} getInstance(ObjectKey id, boolean fromCache)
throws TorqueException
{
return getManager().getInstanceImpl(id, fromCache);
}
#set ($pks = $table.PrimaryKey)
#if ($pks.size() == 1)
#set ($pk = $pks.get(0))
/**
* Static accessor for the @see #getInstanceImpl(ObjectKey).
*
* @param id an ObjectKey
value
* @return a ${interfaceName}
value
* @exception TorqueException if an error occurs
*/
public static ${interfaceName} getInstance($pk.JavaNative id)
throws TorqueException
{
return getManager().getInstanceImpl(SimpleKey.keyFor(id));
}
/**
* Static accessor for the @see #getInstanceImpl(ObjectKey).
*
* @param id an ObjectKey
value
* @param fromCache if true, look for cached ${interfaceName}s before loading
* from storage.
* @return a ${interfaceName}
value
* @exception TorqueException if an error occurs
*/
public static ${interfaceName} getInstance($pk.JavaNative id, boolean fromCache)
throws TorqueException
{
return getManager().getInstanceImpl(SimpleKey.keyFor(id), fromCache);
}
#end
/**
* Static accessor for the @see #getInstancesImpl(List).
*
* @param ids a List
value
* @return a List
value
* @exception TorqueException if an error occurs
*/
public static List#if($enableJava5Features)<$interfaceName>#end getInstances(List#if($enableJava5Features)#end ids)
throws TorqueException
{
return getManager().getInstancesImpl(ids);
}
/**
* Static accessor for the @see #getInstancesImpl(List, boolean).
*
* @param ids a List
value
* @param fromCache if true, look for cached ${interfaceName}s before loading
* from storage.
* @return a List
value
* @exception TorqueException if an error occurs
*/
public static List#if($enableJava5Features)<$interfaceName>#end getInstances(List#if($enableJava5Features)#end ids, boolean fromCache)
throws TorqueException
{
return getManager().getInstancesImpl(ids, fromCache);
}
public static void putInstance(Persistent om)
throws TorqueException
{
getManager().putInstanceImpl(om);
}
public static void clear()
throws TorqueException
{
getManager().clearImpl();
}
public static boolean exists(${interfaceName} obj)
throws TorqueException
{
return getManager().existsImpl(obj);
}
public static MethodResultCache getMethodResult()
{
return getManager().getMethodResultCache();
}
public static void addCacheListener(CacheListener listener)
{
getManager().addCacheListenerImpl(listener);
}
/**
* Creates a new $basePrefix${interfaceName}Manager
instance.
*
* @exception TorqueException if an error occurs
*/
public $basePrefix${interfaceName}Manager()
throws TorqueException
{
setClassName($basePrefix${interfaceName}Manager.MANAGED_CLASS);
}
/**
* Get a fresh instance of a ${interfaceName}Manager
*/
protected ${interfaceName} getInstanceImpl()
throws TorqueException
{
${interfaceName} obj = null;
try
{
obj = (${interfaceName}) getOMInstance();
}
catch (Exception e)
{
throw new TorqueException(e);
}
return obj;
}
/**
* Get a ${interfaceName} with the given id.
*
* @param id ObjectKey
value
*/
protected ${interfaceName} getInstanceImpl(ObjectKey id)
throws TorqueException
{
return (${interfaceName}) getOMInstance(id);
}
/**
* Get a ${interfaceName} with the given id from the cache. Returns
* null
if instance is not in cache
*
* @param id ObjectKey
value
*/
protected ${interfaceName} getCachedInstanceImpl(ObjectKey id)
throws TorqueException
{
return (${interfaceName}) cacheGet(id);
}
/**
* Get a ${interfaceName} with the given id.
*
* @param id ObjectKey
value
* @param fromCache if true, look for cached ${interfaceName}s before loading
* from storage.
*/
protected ${interfaceName} getInstanceImpl(ObjectKey id, boolean fromCache)
throws TorqueException
{
return (${interfaceName}) getOMInstance(id, fromCache);
}
/**
* Gets a list of ${interfaceName}s based on id's.
*
* @param ids a List of ObjectKeys
value
* @return a List
of ${interfaceName}s
* @exception TorqueException if an error occurs
*/
protected List#if($enableJava5Features)<$interfaceName>#end getInstancesImpl(List#if($enableJava5Features)#end ids)
throws TorqueException
{
return getOMs(ids);
}
/**
* Gets a list of ${interfaceName}s based on id's.
*
* @param ids a List of ObjectKeys
value
* @param fromCache if true, look for cached ${interfaceName}s before loading
* from storage.
* @return a List
of ${interfaceName}s
* @exception TorqueException if an error occurs
*/
protected List#if($enableJava5Features)<$interfaceName>#end getInstancesImpl(List#if($enableJava5Features)#end ids, boolean fromCache)
throws TorqueException
{
return getOMs(ids, fromCache);
}
/**
* check for a duplicate project name
*/
protected boolean existsImpl(${interfaceName} om)
throws TorqueException
{
Criteria crit = ${table.JavaName}Peer
.buildCriteria((${table.JavaName})om);
return ${table.JavaName}Peer.doSelect(crit).size() > 0;
}
#if ($table.isAlias())
#set ($retrieveMethod = "retrieve${table.JavaName}ByPK")
#else
#set ($retrieveMethod = "retrieveByPK")
#end
protected Persistent retrieveStoredOM(ObjectKey id)
throws TorqueException
{
return ${table.JavaName}Peer.${retrieveMethod}(id);
}
/**
* Gets a list of ModuleEntities based on id's.
*
* @param ids a NumberKey[]
value
* @return a List
value
* @exception TorqueException if an error occurs
*/
protected List#if($enableJava5Features)<$table.JavaName>#end retrieveStoredOMs(List ids)
throws TorqueException
{
return ${table.JavaName}Peer.${retrieveMethod}s(ids);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy