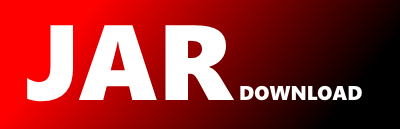
om.MapBuilder.vm Maven / Gradle / Ivy
## Licensed to the Apache Software Foundation (ASF) under one
## or more contributor license agreements. See the NOTICE file
## distributed with this work for additional information
## regarding copyright ownership. The ASF licenses this file
## to you under the Apache License, Version 2.0 (the
## "License"); you may not use this file except in compliance
## with the License. You may obtain a copy of the License at
##
## http://www.apache.org/licenses/LICENSE-2.0
##
## Unless required by applicable law or agreed to in writing,
## software distributed under the License is distributed on an
## "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
## KIND, either express or implied. See the License for the
## specific language governing permissions and limitations
## under the License.
package ${packageMap};
import java.util.Date;
import java.math.BigDecimal;
import org.apache.torque.Torque;
import org.apache.torque.TorqueException;
#if ($table.IdMethod == "native")
import org.apache.torque.adapter.DB;
#end
import org.apache.torque.map.MapBuilder;
import org.apache.torque.map.DatabaseMap;
import org.apache.torque.map.TableMap;
import org.apache.torque.map.ColumnMap;
import org.apache.torque.map.InheritanceMap;
/**
#if ($table.description)
* $!table.description
*
#end
#if ($addTimeStamp)
* This class was autogenerated by Torque on:
*
* [$now]
*
#end
*/
public class ${table.JavaName}MapBuilder implements MapBuilder
{
/**
* The name of this class
*/
public static final String CLASS_NAME =
"${packageMap}.${table.JavaName}MapBuilder";
/**
* The database map.
*/
private DatabaseMap dbMap = null;
/**
* Tells us if this DatabaseMapBuilder is built so that we
* don't have to re-build it every time.
*
* @return true if this DatabaseMapBuilder is built
*/
public boolean isBuilt()
{
return (dbMap != null);
}
/**
* Gets the databasemap this map builder built.
*
* @return the databasemap
*/
public DatabaseMap getDatabaseMap()
{
return this.dbMap;
}
/**
* The doBuild() method builds the DatabaseMap
*
* @throws TorqueException
*/
public synchronized void doBuild() throws TorqueException
{
if ( isBuilt() ) {
return;
}
dbMap = Torque.getDatabaseMap("$table.Database.Name");
dbMap.addTable("$table.Name");
TableMap tMap = dbMap.getTable("$table.Name");
tMap.setJavaName("$table.JavaName");
tMap.setOMClass( ${packageObject}.${table.JavaName}.class );
tMap.setPeerClass( ${packagePeer}.${table.JavaName}Peer.class );
#if ( $table.Description )
tMap.setDescription("$table.Description");
#end
#if ($table.IdMethod == "native")
tMap.setPrimaryKeyMethod(TableMap.NATIVE);
#elseif ($table.IdMethod == "idbroker")
tMap.setPrimaryKeyMethod(TableMap.ID_BROKER);
#else
tMap.setPrimaryKeyMethod("$table.IdMethod");
#end
#if ($table.IdMethodParameters)
// this might need upgrading based on what all the databases
// need, but for now assume one parameter.
#set ($imp = $table.IdMethodParameters.get(0) )
tMap.setPrimaryKeyMethodInfo("$imp.Value");
#elseif ($table.IdMethod == "idbroker")
tMap.setPrimaryKeyMethodInfo(tMap.getName());
#elseif ($table.IdMethod == "native")
DB dbAdapter = Torque.getDB("$table.Database.Name");
if (dbAdapter.getIDMethodType().equals(DB.SEQUENCE))
{
tMap.setPrimaryKeyMethodInfo("$table.SequenceName");
}
else if (dbAdapter.getIDMethodType().equals(DB.AUTO_INCREMENT))
{
tMap.setPrimaryKeyMethodInfo("$table.Name");
}
#end
#if ($useManagers && $table.PrimaryKey.size() > 0)
#set ($interfaceName = $table.JavaName)
#if ($table.Interface)
#set($lastdotpos = $table.Interface.lastIndexOf('.'))
#if($lastdotpos != -1)
#set ($lastdotpos = $lastdotpos + 1)
#set ($interfaceName = $table.Interface.substring($lastdotpos))
#else
#set ($interfaceName = $table.Interface)
#end
#end
tMap.setUseManager(true);
tMap.setManagerClass( ${packageManager}.${interfaceName}Manager.class );
#end
ColumnMap cMap = null;
#set ($tableUseInheritance = "false")
#set ($position = 1)
#foreach ($col in $table.Columns)
#set ( $cfc=$col.JavaName )
#set ( $cnm=$col.Name )
#if ( ${deprecatedUppercasePeer} )
#set ( $cnm=$col.Name.toUpperCase() )
#end
// ------------- Column: $cnm --------------------
cMap = new ColumnMap( "$cnm", tMap);
cMap.setType( $col.JavaObject );
cMap.setTorqueType( "$col.Domain.Type.Name" );
cMap.setUsePrimitive($col.UsePrimitive);
cMap.setPrimaryKey($col.isPrimaryKey());
cMap.setNotNull($col.isNotNull());
cMap.setJavaName( "$cfc" );
cMap.setAutoIncrement($col.AutoIncrement);
cMap.setProtected($col.Protected);
#if( $col.JavaType )
cMap.setJavaType( "${col.JavaType}" );
#end
#if( $col.Description )
cMap.setDescription("${col.Description}");
#end
#if( $col.DefaultValue )
cMap.setDefault("$col.DefaultValue");
#end
#if( $col.InheritanceType )
cMap.setInheritance("$col.InheritanceType");
#end
#if( $col.InputValidator )
cMap.setInputValidator("$col.InputValidator");
#end
#if( $col.JavaNamingMethod )
cMap.setJavaNamingMethod("$col.JavaNamingMethod");
#end
#if( $col.Precision )
cMap.setSize( $col.Precision );
#if( $col.Scale )
cMap.setScale( $col.Scale );
#end
#end
#if($col.isForeignKey())
cMap.setForeignKey("$col.RelatedTableName", "$col.RelatedColumnName");
#end
#if($col.isInheritance() )
#set($tableUseInheritance = "true");
cMap.setUseInheritance($col.Inheritance);
InheritanceMap iMap = null;
#foreach ($inh in $col.Children)
iMap = new InheritanceMap(cMap,"$inh.Key","$inh.ClassName","$inh.Ancestor");
cMap.addInheritanceMap(iMap);
#end
#end
cMap.setPosition($position);
#set ($position = $position + 1)
tMap.addColumn(cMap);
#end
tMap.setUseInheritance($tableUseInheritance);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy