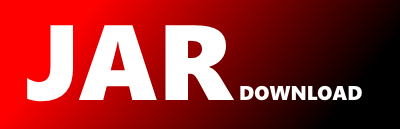
om.bean.Bean.vm Maven / Gradle / Ivy
## Licensed to the Apache Software Foundation (ASF) under one
## or more contributor license agreements. See the NOTICE file
## distributed with this work for additional information
## regarding copyright ownership. The ASF licenses this file
## to you under the Apache License, Version 2.0 (the
## "License"); you may not use this file except in compliance
## with the License. You may obtain a copy of the License at
##
## http://www.apache.org/licenses/LICENSE-2.0
##
## Unless required by applicable law or agreed to in writing,
## software distributed under the License is distributed on an
## "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
## KIND, either express or implied. See the License for the
## specific language governing permissions and limitations
## under the License.
#*
* author Thomas Fischer
* version $Id: Bean.vm 524492 2007-03-31 23:25:52Z gmonroe $
*#
package ${packageBaseBean};
import java.io.Serializable;
import java.math.BigDecimal;
import java.sql.Connection;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.List;
#if ($packageBaseBean != $packageBean)
import ${packageBean}.*;
#end
#set ($currentPackage = $table.Database.Package)
#foreach ($col in $table.Columns)
#if ($col.isForeignKey())
#set ($fkPackage = $table.Database.getTable($col.RelatedTableName).getPackage())
#if (!$fkPackage.equals($currentPackage) )
#if ($subpackageBean)
import ${fkPackage}.${subpackageBean}.${table.Database.getTable($col.RelatedTableName).JavaName}${beanSuffix};
#else
import ${fkPackage}.${table.Database.getTable($col.RelatedTableName).JavaName}${beanSuffix};
#end
#end
#end
#end
/**
#if ($table.description)
* $!table.description
*
#end
#if ($addTimeStamp)
* This class was autogenerated by Torque on:
*
* [$now]
*
#end
* You should not use this class directly. It should not even be
* extended; all references should be to ${table.JavaName}${beanSuffix}
*/
public abstract class ${basePrefix}${table.JavaName}${beanSuffix}
#if ( ${beanExtendsClass} )
extends ${beanExtendsClass}
#end
implements Serializable
{
## ----------------
## member variables
## ----------------
/**
* whether the bean or its underlying object has changed
* since last reading from the database
*/
private boolean modified = true;
/**
* false if the underlying object has been read from the database,
* true otherwise
*/
private boolean isNew = true;
#foreach ($col in $table.Columns)
#set ( $cjtype = $col.JavaNative )
#set ( $clo=$col.UncapitalisedJavaName )
#set ($defVal = "")
#if ($col.DefaultValue && !$col.DefaultValue.equalsIgnoreCase("NULL") )
#set ( $quote = '' )
#if ( $cjtype == "String" )
#set ( $quote = '"' )
#end
#set ( $defaultValue = $col.DefaultValue )
#if ( $cjtype == "boolean" || $cjtype == "Boolean" )
#if ( $defaultValue == "1" || $defaultValue == "Y" )
#set ( $defaultValue = "true" )
#elseif ( $defaultValue == "0" || $defaultValue == "N" )
#set ( $defaultValue = "false" )
#end
#end
#if ($cjtype == "BigDecimal")
#set ($defVal = "= new BigDecimal($defaultValue)")
#elseif ($cjtype == "NumberKey")
#set ( $quote = '"' )
#set ($defVal = "= new NumberKey($quote$defaultValue$quote)")
#elseif ($cjtype == "StringKey")
#set ( $quote = '"' )
#set ($defVal = "= new StringKey($quote$defaultValue$quote)")
#elseif ($cjtype == 'Byte')
#set ($defVal = "= new ${cjtype}((byte) $defaultValue)")
#elseif ($cjtype == "Short")
#set ( $quote = '"' )
#set ($defVal = "= new Short($quote$defaultValue$quote)")
#else
#if (!$col.isPrimitive() && $cjtype != "String")
#set ( $defaultValue = "new ${cjtype}($defaultValue)" )
#end
#set ($defVal = " = $quote$defaultValue$quote")
#end
#end
/** The value for the $clo field */
private $cjtype $clo$defVal;
#end
## -------------------------
## getter and setter methods
## -------------------------
/**
* sets whether the bean exists in the database
*/
public void setNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* returns whether the bean exists in the database
*/
public boolean isNew()
{
return this.isNew;
}
/**
* sets whether the bean or the object it was created from
* was modified since the object was last read from the database
*/
public void setModified(boolean isModified)
{
this.modified = isModified;
}
/**
* returns whether the bean or the object it was created from
* was modified since the object was last read from the database
*/
public boolean isModified()
{
return this.modified;
}
#foreach ($col in $table.Columns)
#set ( $cfc=$col.JavaName )
#set ( $clo=$col.UncapitalisedJavaName )
#set ( $cjtype = $col.JavaNative )
/**
* Get the $cfc
*
* @return $cjtype
*/
public $cjtype ${col.GetterName} ()
{
return $clo;
}
/**
* Set the value of $cfc
*
* @param v new value
*/
public void ${col.SetterName}($cjtype v)
{
#if (($cjtype == "NumberKey") || ($cjtype == "StringKey") || ($cjtype == "DateKey"))
if (v != null && v.getValue() == null)
{
// If this is an Objectkey than this set method is
// probably storing the id of this object or some
// associated object. If the objectKey value is null
// then we convert the parameter to null so that this
// property is consistently null to indicate that no
// object is associated or defined.
v = null;
}
#end
this.$clo = v;
setModified(true);
}
#end
#if ($complexObjectModel)
#set($pVars = []) ## Array of object set method names for later reference.
#set($aVars = []) ## Array of object field names for later reference.
#foreach ($fk in $table.ForeignKeys)
#set ( $tblFK = $table.Database.getTable($fk.ForeignTableName) )
#set ( $className = "${tblFK.JavaName}${beanSuffix}" )
#set ( $relCol = "" )
#foreach ($columnName in $fk.LocalColumns)
#set ( $column = $table.getColumn($columnName) )
#if ($column.isMultipleFK() || $fk.ForeignTableName.equals($table.Name))
#set ( $relCol = "$relCol$column.JavaName" )
#end
#end
#if ($relCol != "")
#set ( $relCol = "RelatedBy$relCol" )
#end
#set ( $pVarName = "$className$relCol" )
#set ( $varName = "a$pVarName" )
#set ( $retVal = $pVars.add($pVarName) )
#set ( $retVal = $aVars.add($varName) )
private $className $varName;
/**
* sets an associated $className object
*
* @param v $className
*/
public void set${pVarName}($className v)
{
#foreach ($columnName in $fk.LocalColumns)
#set ( $column = $table.getColumn($columnName) )
#set ( $colFKName = $fk.LocalForeignMapping.get($columnName) )
#set ( $colFK = $tblFK.getColumn($colFKName) )
#set ( $fktype = $colFK.JavaNative )
#set ( $casttype = "" )
if (v == null)
{
#if($colFK.Primitive)
#if ($fktype == "short")
#set ($casttype = "(short)")
#elseif($fktype == "byte")
#set ($casttype = "(byte)")
#end
#set ($coldefval = "0")
#set ($coldefval = $column.DefaultValue)
${column.SetterName}($casttype $coldefval);
#else
${column.SetterName}(($column.JavaNative) null);
#end
}
else
{
${column.SetterName}(v.${colFK.GetterName}());
}
#end
$varName = v;
}
#set ( $and = "" )
#set ( $comma = "" )
#set ( $conditional = "" )
#set ( $arglist = "" )
#set ( $argsize = 0 )
#foreach ($columnName in $fk.LocalColumns)
#set ( $column = $table.getColumn($columnName) )
#set ( $cjtype = $column.JavaNative )
#set ( $clo=$column.UncapitalisedJavaName )
#if ($cjtype == "short" || $cjtype == "int" || $cjtype == "long" || $cjtype == "byte" || $cjtype == "float" || $cjtype == "double")
#set ( $conditional = "$conditional${and}this.${clo} != 0" )
#else
#set ( $conditional = "$conditional${and}!ObjectUtils.equals(this.${clo}, null)" )
#end
#set ( $arglist = "$arglist${comma}this.$clo" )
#set ( $and = " && " )
#set ( $comma = ", " )
#set ( $argsize = $argsize + 1 )
#end
#set ( $pCollName = "${table.JavaName}s$relCol" )
/**
* Get the associated $className object
*
* @return the associated $className object
*/
public $className get${pVarName}()
{
return $varName;
}
#end
## ---------------------------
#if ($objectIsCaching)
#foreach ($fk in $table.Referrers)
#set ( $tblFK = $fk.Table )
#if ( !($tblFK.Name.equals($table.Name)) )
#set ( $className = $tblFK.JavaName )
#set ( $relatedByCol = "" )
#foreach ($columnName in $fk.LocalColumns)
#set ( $column = $tblFK.getColumn($columnName) )
#if ($column.isMultipleFK())
#set ($relatedByCol= "$relatedByCol$column.JavaName")
#end
#end
#if ($relatedByCol == "")
#set ( $relCol = "${className}${beanSuffix}s" )
#else
#set ( $relCol= "${className}${beanSuffix}sRelatedBy$relatedByCol" )
#end
#set ( $collName = "coll$relCol" )
/**
* Collection to store aggregation of $collName
*/
protected List#if($enableJava5Features)<${className}${beanSuffix}>#end $collName;
/**
* Returns the collection of ${relCol}
*/
public List#if($enableJava5Features)<${className}${beanSuffix}>#end get${relCol}()
{
return $collName;
}
/**
* Sets the collection of ${relCol} to the specified value
*/
public void set${relCol}(List#if($enableJava5Features)<${className}${beanSuffix}>#end list)
{
if (list == null)
{
$collName = null;
}
else
{
$collName = new ArrayList#if($enableJava5Features)<${className}${beanSuffix}>#end(list);
}
}
#end
#end
#end
#end
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy