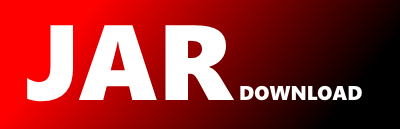
org.apache.wicket.util.string.StringEscapeUtils Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.wicket.util.string;
import java.io.IOException;
import java.io.StringWriter;
import java.io.Writer;
import java.util.Locale;
/**
*
* Escapes and unescapes String
s for Java, Java Script, HTML, XML, and SQL.
*
*
*
* #ThreadSafe#
*
*
* @author Apache Software Foundation
* @author Apache Jakarta Turbine
* @author Purple Technology
* @author Alexander Day Chaffee
* @author Antony Riley
* @author Helge Tesgaard
* @author Sean Brown
* @author Gary Gregory
* @author Phil Steitz
* @author Pete Gieser
* @since 2.0
* @version $Id$
*/
// Copy from commons-lang ver. 2.6. Non-html/xml methods were removed
class StringEscapeUtils
{
/**
*
* StringEscapeUtils
instances should NOT be constructed in standard programming.
*
*
*
* Instead, the class should be used as:
*
*
* StringEscapeUtils.escapeJava("foo");
*
*
*
*
*
* This constructor is public to permit tools that require a JavaBean instance to operate.
*
*/
public StringEscapeUtils()
{
super();
}
/**
*
* Returns an upper case hexadecimal String
for the given character.
*
*
* @param ch
* The character to convert.
* @return An upper case hexadecimal String
*/
private static String hex(char ch)
{
return Integer.toHexString(ch).toUpperCase(Locale.ENGLISH);
}
// HTML and XML
// --------------------------------------------------------------------------
/**
*
* Escapes the characters in a String
using HTML entities.
*
*
*
* For example:
*
*
* "bread" & "butter"
*
* becomes:
*
* "bread" & "butter"
.
*
*
*
* Supports all known HTML 4.0 entities, including funky accents. Note that the commonly used
* apostrophe escape character (') is not a legal entity and so is not supported).
*
*
* @param str
* the String
to escape, may be null
* @return a new escaped String
, null
if null string input
*
* @see #unescapeHtml(String)
* @see ISO
* Entities
* @see HTML 3.2 Character Entities for ISO
* Latin-1
* @see HTML 4.0 Character entity
* references
* @see HTML 4.01 Character
* References
* @see HTML 4.01 Code
* positions
*/
public static String escapeHtml(String str)
{
if (str == null)
{
return null;
}
try
{
StringWriter writer = new StringWriter((int)(str.length() * 1.5));
escapeHtml(writer, str);
return writer.toString();
}
catch (IOException ioe)
{
// should be impossible
throw new RuntimeException(ioe);
}
}
/**
*
* Escapes the characters in a String
using HTML entities and writes them to a
* Writer
.
*
*
*
* For example:
*
* "bread" & "butter"
*
* becomes:
*
* "bread" & "butter"
.
*
*
* Supports all known HTML 4.0 entities, including funky accents. Note that the commonly used
* apostrophe escape character (') is not a legal entity and so is not supported).
*
*
* @param writer
* the writer receiving the escaped string, not null
* @param string
* the String
to escape, may be null
* @throws IllegalArgumentException
* if the writer is null
* @throws IOException
* when Writer
passed throws the exception from calls to the
* {@link Writer#write(int)} methods.
*
* @see #escapeHtml(String)
* @see #unescapeHtml(String)
* @see ISO
* Entities
* @see HTML 3.2 Character Entities for ISO
* Latin-1
* @see HTML 4.0 Character entity
* references
* @see HTML 4.01 Character
* References
* @see HTML 4.01 Code
* positions
*/
public static void escapeHtml(Writer writer, String string) throws IOException
{
if (writer == null)
{
throw new IllegalArgumentException("The Writer must not be null.");
}
if (string == null)
{
return;
}
Entities.HTML40.escape(writer, string);
}
// -----------------------------------------------------------------------
/**
*
* Unescapes a string containing entity escapes to a string containing the actual Unicode
* characters corresponding to the escapes. Supports HTML 4.0 entities.
*
*
*
* For example, the string "<Français>" will become
* "<Français>"
*
*
*
* If an entity is unrecognized, it is left alone, and inserted verbatim into the result string.
* e.g. ">&zzzz;x" will become ">&zzzz;x".
*
*
* @param str
* the String
to unescape, may be null
* @return a new unescaped String
, null
if null string input
* @see #escapeHtml(Writer, String)
*/
public static String unescapeHtml(String str)
{
if (str == null)
{
return null;
}
try
{
StringWriter writer = new StringWriter((int)(str.length() * 1.5));
unescapeHtml(writer, str);
return writer.toString();
}
catch (IOException ioe)
{
// should be impossible
throw new RuntimeException(ioe);
}
}
/**
*
* Unescapes a string containing entity escapes to a string containing the actual Unicode
* characters corresponding to the escapes. Supports HTML 4.0 entities.
*
*
*
* For example, the string "<Français>" will become
* "<Français>"
*
*
*
* If an entity is unrecognized, it is left alone, and inserted verbatim into the result string.
* e.g. ">&zzzz;x" will become ">&zzzz;x".
*
*
* @param writer
* the writer receiving the unescaped string, not null
* @param string
* the String
to unescape, may be null
* @throws IllegalArgumentException
* if the writer is null
* @throws IOException
* if an IOException occurs
* @see #escapeHtml(String)
*/
public static void unescapeHtml(Writer writer, String string) throws IOException
{
if (writer == null)
{
throw new IllegalArgumentException("The Writer must not be null.");
}
if (string == null)
{
return;
}
Entities.HTML40.unescape(writer, string);
}
// -----------------------------------------------------------------------
/**
*
* Escapes the characters in a String
using XML entities.
*
*
*
* For example: "bread" & "butter" =>
* "bread" & "butter".
*
*
*
* Supports only the five basic XML entities (gt, lt, quot, amp, apos). Does not support DTDs or
* external entities.
*
*
*
* Note that unicode characters greater than 0x7f are currently escaped to their numerical \\u
* equivalent. This may change in future releases.
*
*
* @param writer
* the writer receiving the unescaped string, not null
* @param str
* the String
to escape, may be null
* @throws IllegalArgumentException
* if the writer is null
* @throws IOException
* if there is a problem writing
* @see #unescapeXml(java.lang.String)
*/
public static void escapeXml(Writer writer, String str) throws IOException
{
if (writer == null)
{
throw new IllegalArgumentException("The Writer must not be null.");
}
if (str == null)
{
return;
}
Entities.XML.escape(writer, str);
}
/**
*
* Escapes the characters in a String
using XML entities.
*
*
*
* For example: "bread" & "butter" =>
* "bread" & "butter".
*
*
*
* Supports only the five basic XML entities (gt, lt, quot, amp, apos). Does not support DTDs or
* external entities.
*
*
*
* Note that unicode characters greater than 0x7f are currently escaped to their numerical \\u
* equivalent. This may change in future releases.
*
*
* @param str
* the String
to escape, may be null
* @return a new escaped String
, null
if null string input
* @see #unescapeXml(java.lang.String)
*/
public static String escapeXml(String str)
{
if (str == null)
{
return null;
}
return Entities.XML.escape(str);
}
// -----------------------------------------------------------------------
/**
*
* Unescapes a string containing XML entity escapes to a string containing the actual Unicode
* characters corresponding to the escapes.
*
*
*
* Supports only the five basic XML entities (gt, lt, quot, amp, apos). Does not support DTDs or
* external entities.
*
*
*
* Note that numerical \\u unicode codes are unescaped to their respective unicode characters.
* This may change in future releases.
*
*
* @param writer
* the writer receiving the unescaped string, not null
* @param str
* the String
to unescape, may be null
* @throws IllegalArgumentException
* if the writer is null
* @throws IOException
* if there is a problem writing
* @see #escapeXml(String)
*/
public static void unescapeXml(Writer writer, String str) throws IOException
{
if (writer == null)
{
throw new IllegalArgumentException("The Writer must not be null.");
}
if (str == null)
{
return;
}
Entities.XML.unescape(writer, str);
}
/**
*
* Unescapes a string containing XML entity escapes to a string containing the actual Unicode
* characters corresponding to the escapes.
*
*
*
* Supports only the five basic XML entities (gt, lt, quot, amp, apos). Does not support DTDs or
* external entities.
*
*
*
* Note that numerical \\u unicode codes are unescaped to their respective unicode characters.
* This may change in future releases.
*
*
* @param str
* the String
to unescape, may be null
* @return a new unescaped String
, null
if null string input
* @see #escapeXml(String)
*/
public static String unescapeXml(String str)
{
if (str == null)
{
return null;
}
return Entities.XML.unescape(str);
}
}