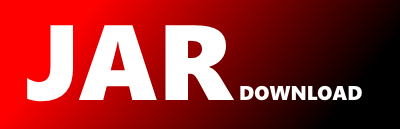
org.apache.wink.client.RestClient Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*
*******************************************************************************/
package org.apache.wink.client;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.util.Set;
import javax.ws.rs.core.Application;
import org.apache.wink.client.internal.ResourceImpl;
import org.apache.wink.common.WinkApplication;
import org.apache.wink.common.internal.application.ApplicationValidator;
import org.apache.wink.common.internal.i18n.Messages;
import org.apache.wink.common.internal.lifecycle.LifecycleManagersRegistry;
import org.apache.wink.common.internal.lifecycle.ScopeLifecycleManager;
import org.apache.wink.common.internal.registry.ProvidersRegistry;
import org.apache.wink.common.internal.registry.metadata.ProviderMetadataCollector;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* The RestClient is the entry point for all rest service operations. The
* RestClient is used to create instances of {@link Resource} classes that are
* used to make the actual invocations to the service. The client can be
* initialized with a user supplied configuration to specify custom Provider
* classes, in addition to other configuration options.
*
*
* // create the client
* RestClient client = new RestClient();
*
* // create the resource to make invocations on
* Resource resource = client.resource("http://myhost:80/my/service");
*
* // invoke GET on the resource and receive the response entity as a string
* String entity = resource.get(String.class);
* ...
*
*/
public class RestClient {
private static final Logger logger = LoggerFactory.getLogger(RestClient.class);
private ProvidersRegistry providersRegistry;
private ClientConfig config;
/**
* Construct a new RestClient using the default client configuration
*/
public RestClient() {
this(new ClientConfig());
}
/**
* Construct a new RestClient using the supplied configuration
*
* @param config the client configuration
*/
public RestClient(ClientConfig config) {
ClientConfig clone = config.clone();
this.config = clone.build();
initProvidersRegistry();
}
/**
* Get the unmodifiable client configuration
*
* @return the unmodifiable client configuration
*/
public ClientConfig getConfig() {
return config;
}
/**
* Create a new {@link Resource} instance
*
* @param uri uri of the resource to create
* @return a new {@link Resource} instance attached to the specified uri
*/
public Resource resource(URI uri) {
return new ResourceImpl(uri, config, providersRegistry);
}
/**
* Create a new {@link Resource} instance
*
* @param uri uri of the resource to create
* @return a new {@link Resource} instance attached to the specified uri
*/
public Resource resource(String uri) {
return resource(URI.create(uri));
}
/**
* Create a new {@link Resource} instance
*
* @param uri uri of the resource to create
* @param httpEncode boolean to declare whether the passed uri needs to be encoded (true) or not (false)
* @return a new {@link Resource} instance attached to the specified uri
*/
public Resource resource(String uri, boolean httpEncode) throws MalformedURLException, URISyntaxException {
if (httpEncode) {
URL url = new URL(uri);
// URI.toURL() will escape characters if we use one of the multi-param constructors
URI constructedURI = new URI(url.getProtocol(), url.getUserInfo(), url.getHost(), url.getPort(),url.getPath(), url.getQuery(), null);
return resource(constructedURI);
}
return resource(URI.create(uri));
}
private void initProvidersRegistry() {
// setup OFFactoryRegistry to support default and scope
LifecycleManagersRegistry ofFactoryRegistry = new LifecycleManagersRegistry();
ofFactoryRegistry.addFactoryFactory(new ScopeLifecycleManager
© 2015 - 2025 Weber Informatics LLC | Privacy Policy