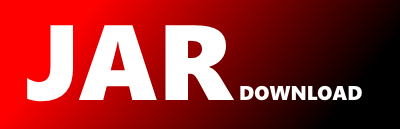
org.apache.woden.ant.CmHttpWriter Maven / Gradle / Ivy
The newest version!
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.woden.ant;
import java.util.Arrays;
import java.util.Comparator;
import org.apache.woden.wsdl20.extensions.http.HTTPAuthenticationScheme;
import org.apache.woden.wsdl20.extensions.http.HTTPBindingExtensions;
import org.apache.woden.wsdl20.extensions.http.HTTPBindingFaultExtensions;
import org.apache.woden.wsdl20.extensions.http.HTTPBindingMessageReferenceExtensions;
import org.apache.woden.wsdl20.extensions.http.HTTPBindingOperationExtensions;
import org.apache.woden.wsdl20.extensions.http.HTTPEndpointExtensions;
import org.apache.woden.wsdl20.extensions.http.HTTPErrorStatusCode;
import org.apache.woden.wsdl20.extensions.http.HTTPHeader;
import org.apache.woden.wsdl20.extensions.http.HTTPLocation;
/**
* @author Arthur Ryman ([email protected], [email protected])
*
*/
public class CmHttpWriter extends NamespaceWriter {
public final static String NS = "http://www.w3.org/2002/ws/desc/wsdl/component-http";
public final static String PREFIX = "cmhttp";
private CmBaseWriter cmbase;
public CmHttpWriter(XMLWriter out) {
super(out, NS, PREFIX);
cmbase = (CmBaseWriter) out.lookup(CmBaseWriter.NS);
}
public void httpBindingExtension(HTTPBindingExtensions http) {
if (http == null)
return;
out.beginElement(PREFIX + ":httpBindingExtension");
out.write(PREFIX + ":httpCookies", http.isHttpCookies());
out.write(PREFIX + ":httpMethodDefault", http.getHttpMethodDefault());
out.write(PREFIX + ":httpQueryParameterSeparatorDefault", http
.getHttpQueryParameterSeparatorDefault());
out.write(PREFIX + ":httpContentEncodingDefault", http
.getHttpContentEncodingDefault());
out.endElement();
}
public void httpBindingFaultExtension(HTTPBindingFaultExtensions http) {
if (http != null) {
out.beginElement(PREFIX + ":httpBindingFaultExtension");
write(PREFIX + ":httpErrorStatusCode", http
.getHttpErrorStatusCode());
write(PREFIX + ":httpHeaders", http.getHttpHeaders());
out.write(PREFIX + ":httpContentEncoding", http
.getHttpContentEncoding());
out.endElement();
}
}
public void httpBindingOperationExtension(
HTTPBindingOperationExtensions http) {
if (http == null)
return;
out.beginElement(PREFIX + ":httpBindingOperationExtension");
out.write(PREFIX + ":httpFaultSerialization", http
.getHttpFaultSerialization());
out.write(PREFIX + ":httpInputSerialization", http
.getHttpInputSerialization());
write(PREFIX + ":httpLocation", http.getHttpLocation());
out.write(PREFIX + ":httpLocationIgnoreUncited", http
.isHttpLocationIgnoreUncited());
out.write(PREFIX + ":httpMethod", http.getHttpMethod());
out.write(PREFIX + ":httpOutputSerialization", http
.getHttpOutputSerialization());
out.write(PREFIX + ":httpQueryParameterSeparator", http
.getHttpQueryParameterSeparator());
out.write(PREFIX + ":httpContentEncodingDefault", http
.getHttpContentEncodingDefault());
out.endElement();
}
public void httpBindingMessageReferenceExtension(
HTTPBindingMessageReferenceExtensions http) {
if (http == null)
return;
out.beginElement(PREFIX + ":httpBindingMessageReferenceExtension");
write(PREFIX + ":httpHeaders", http.getHttpHeaders());
out.write(PREFIX + ":httpContentEncoding", http.getHttpContentEncoding());
out.endElement();
}
public void httpEndpointExtension(HTTPEndpointExtensions http) {
if (http == null)
return;
out.beginElement(PREFIX + ":httpEndpointExtension");
out.write(PREFIX + ":httpAuthenticationRealm", http
.getHttpAuthenticationRealm());
write(PREFIX + ":httpAuthenticationScheme", http
.getHttpAuthenticationScheme());
out.endElement();
}
private void write(String tag, HTTPErrorStatusCode httpErrorStatusCode) {
if (httpErrorStatusCode == null)
return;
out.beginElement(tag);
if (httpErrorStatusCode.isCodeUsed()) {
out.write(PREFIX + ":code", httpErrorStatusCode.toString());
}
out.endElement();
}
public void write(String tag, HTTPHeader[] components) {
if (components.length == 0)
return;
Arrays.sort(components, new Comparator() {
public int compare(Object o1, Object o2) {
String n1 = ((HTTPHeader) o1).getName();
String n2 = ((HTTPHeader) o2).getName();
return n1.compareTo(n2);
}
});
out.beginElement(tag);
for (int i = 0; i < components.length; i++)
write(PREFIX + ":httpHeaderComponent", components[i]);
out.endElement();
}
private void write(String tag, HTTPHeader component) {
out.beginElement(tag, cmbase.idAttribute(component));
out.write(PREFIX + ":name", component.getName());
cmbase.writeRef(PREFIX + ":typeDefinition", component
.getTypeDefinition());
out.write(PREFIX + ":required", component.isRequired());
cmbase.parent(component.getParent());
out.endElement();
}
public void write(String tag, HTTPAuthenticationScheme scheme) {
if (scheme == null)
return;
out.write(tag, scheme.toString());
}
private void write(String tag, HTTPLocation location) {
if(location == null) {
return;
}
out.write(tag, location.getOriginalLocation());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy