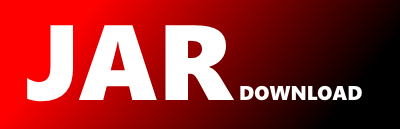
org.apache.ws.commons.schema.docpath.XmlSchemaStateMachineNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xmlschema-walker Show documentation
Show all versions of xmlschema-walker Show documentation
Code to walk an XML Schema and confirm an XML Document conforms to it.
/** * Licensed to the Apache Software Foundation (ASF) under one * or more contributor license agreements. See the NOTICE file * distributed with this work for additional information * regarding copyright ownership. The ASF licenses this file * to you under the Apache License, Version 2.0 (the * "License"); you may not use this file except in compliance * with the License. You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, * software distributed under the License is distributed on an * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY * KIND, either express or implied. See the License for the * specific language governing permissions and limitations * under the License. */ package org.apache.ws.commons.schema.docpath; import java.util.ArrayList; import java.util.List; import org.apache.ws.commons.schema.XmlSchemaAny; import org.apache.ws.commons.schema.XmlSchemaAttribute; import org.apache.ws.commons.schema.XmlSchemaElement; import org.apache.ws.commons.schema.walker.XmlSchemaAttrInfo; import org.apache.ws.commons.schema.walker.XmlSchemaTypeInfo; /** * This represents a node in the state machine used when parsing an XML * {@link org.w3c.dom.Document} based on its * {@link org.apache.ws.commons.schema.XmlSchema} and Avro * {@link org.apache.avro.Schema}. *
. * * @param next A node that could follow this one in the XML document. * @return Itself, for chaining. */ XmlSchemaStateMachineNode addPossibleNextState(XmlSchemaStateMachineNode next) { possibleNextStates.add(next); return this; } /** * Adds the set of possible states that could follow this ** A
SchemaStateMachineNode
represents one of: **
* As a {@link org.w3c.dom.Document} is traversed, the state machine is used to * determine how to process the current element. Two passes will be needed: the * first pass will determine the correct path through the document's schema in * order to properly parse the elements, and the second traversal will read the * elements while following that path. * */ public final class XmlSchemaStateMachineNode { private final Type nodeType; private final XmlSchemaElement element; private final List- An element, its type, and its attributes
*- An all group
*- A choice group
*- A sequence group
*- An <any> wildcard element
*- A substitution group
*attributes; private final XmlSchemaTypeInfo typeInfo; private final long minOccurs; private final long maxOccurs; private final XmlSchemaAny any; private List possibleNextStates; public enum Type { ELEMENT, SUBSTITUTION_GROUP, ALL, CHOICE, SEQUENCE, ANY } /** * Constructs a new SchemaStateMachineNode
for a group. * * @param nodeType The type of the group node ({@link Type#ALL}, * {@link Type#SUBSTITUTION_GROUP}, {@link Type#CHOICE}, * {@link Type#SEQUENCE}, or {@link Type#ANY}). * @param minOccurs The minimum number of occurrences of this group. * @param maxOccurs The maximum number of occurrences of this group. * @throws IllegalArgumentException if this constructor is used to define an * {@link Type#ELEMENT} or an {@link Type#ANY}. */ XmlSchemaStateMachineNode(Type nodeType, long minOccurs, long maxOccurs) { if (nodeType.equals(Type.ELEMENT)) { throw new IllegalArgumentException("This constructor cannot be used for elements."); } else if (nodeType.equals(Type.ANY)) { throw new IllegalArgumentException("This constructor cannot be used for wildcard elements."); } this.nodeType = nodeType; this.minOccurs = minOccurs; this.maxOccurs = maxOccurs; this.element = null; this.attributes = null; this.typeInfo = null; this.any = null; this.possibleNextStates = new ArrayList(); } /** * Constructs a new SchemaStateMachineNode
for an element. * * @param elem The {@link XmlSchemaElement} this node represents. * @param attrs The {@link XmlSchemaAttribute} contained by this element. An * empty {@link List} ornull
if none. * @param typeInfo The type information, if the element has simple content. *null
if not. */ XmlSchemaStateMachineNode(XmlSchemaElement elem, Listattrs, XmlSchemaTypeInfo typeInfo) { this.nodeType = Type.ELEMENT; this.element = elem; this.attributes = attrs; this.typeInfo = typeInfo; this.minOccurs = elem.getMinOccurs(); this.maxOccurs = elem.getMaxOccurs(); this.any = null; this.possibleNextStates = new ArrayList (); } /** * Constructs a {@link XmlSchemaStateMachineNode} from the * {@link XmlSchemaAny}. * * @param any The XmlSchemaAny
to construct the node from. */ XmlSchemaStateMachineNode(XmlSchemaAny any) { this.nodeType = Type.ANY; this.any = any; this.minOccurs = any.getMinOccurs(); this.maxOccurs = any.getMaxOccurs(); this.element = null; this.attributes = null; this.typeInfo = null; this.possibleNextStates = new ArrayList(); } /** * The XML Schema node {@link Type} this SchemaStateMachineNode
* represents. */ public Type getNodeType() { return nodeType; } /** * If thisSchemaStateMachineNode
represents an * {@link XmlSchemaElement}, theXmlSchemaElement
it * represents. */ public XmlSchemaElement getElement() { return element; } /** * If thisSchemaStateMachineNode
represents an * {@link XmlSchemaElement}, the {@link XmlSchemaTypeInfo} of the element it * represents. */ public XmlSchemaTypeInfo getElementType() { return typeInfo; } /** * If thisSchemaStateMachineNode
represents an * {@link XmlSchemaElement}, the set of {@link XmlSchemaAttrInfo}s * associated with the element it represents. */ public ListgetAttributes() { return attributes; } /** * The minimum number of times this SchemaStateMachineNode
may * appear in succession. */ public long getMinOccurs() { return minOccurs; } /** * The maximum number of times thisSchemaStateMachineNode
may * appear in succession. */ public long getMaxOccurs() { return maxOccurs; } /** * Returns the {@link XmlSchemaAny} associated with this node, or *null
SchemaStateMachineNodeSchemaStateMachineNode
. * * @param nextStates The set of possible nodes that could follow this one in * the XML document. * @return Itself, for chaining. */ XmlSchemaStateMachineNode addPossibleNextStates(java.util.CollectionnextStates) { possibleNextStates.addAll(nextStates); return this; } /** * All of the known possible states that could follow this one. */ public List getPossibleNextStates() { return possibleNextStates; } /** * Builds a {@link String} representing this * XmlSchemaStateMachineNode
. */ @Override public String toString() { StringBuilder name = new StringBuilder(nodeType.name()); switch (nodeType) { case ELEMENT: name.append(": ").append(element.getQName()).append(" ["); name.append(minOccurs).append(", "); name.append(maxOccurs).append("]"); break; case ANY: name.append(": NS: \"").append(any.getNamespace()).append("\", "); name.append("Processing: ").append(any.getProcessContent()); name.append(" [").append(minOccurs).append(", ").append(maxOccurs); name.append(']'); break; default: name.append(" [").append(minOccurs).append(", ").append(maxOccurs); name.append(']'); } return name.toString(); } }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy