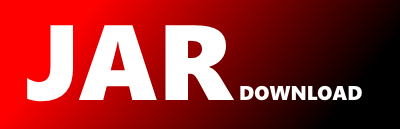
org.apache.zeppelin.interpreter.remote.RemoteInterpreter Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.zeppelin.interpreter.remote;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import org.apache.thrift.TException;
import org.apache.zeppelin.display.GUI;
import org.apache.zeppelin.interpreter.Interpreter;
import org.apache.zeppelin.interpreter.InterpreterContext;
import org.apache.zeppelin.interpreter.InterpreterContextRunner;
import org.apache.zeppelin.interpreter.InterpreterException;
import org.apache.zeppelin.interpreter.InterpreterGroup;
import org.apache.zeppelin.interpreter.InterpreterResult;
import org.apache.zeppelin.interpreter.InterpreterResult.Type;
import org.apache.zeppelin.interpreter.thrift.RemoteInterpreterContext;
import org.apache.zeppelin.interpreter.thrift.RemoteInterpreterResult;
import org.apache.zeppelin.interpreter.thrift.RemoteInterpreterService.Client;
import org.apache.zeppelin.scheduler.Scheduler;
import org.apache.zeppelin.scheduler.SchedulerFactory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.gson.Gson;
import com.google.gson.reflect.TypeToken;
/**
*
*/
public class RemoteInterpreter extends Interpreter {
Logger logger = LoggerFactory.getLogger(RemoteInterpreter.class);
Gson gson = new Gson();
private String interpreterRunner;
private String interpreterPath;
private String className;
FormType formType;
boolean initialized;
private Map env;
static Map interpreterGroupReference
= new HashMap();
private int connectTimeout;
public RemoteInterpreter(Properties property,
String className,
String interpreterRunner,
String interpreterPath,
int connectTimeout) {
super(property);
this.className = className;
initialized = false;
this.interpreterRunner = interpreterRunner;
this.interpreterPath = interpreterPath;
env = new HashMap();
this.connectTimeout = connectTimeout;
}
public RemoteInterpreter(Properties property,
String className,
String interpreterRunner,
String interpreterPath,
Map env,
int connectTimeout) {
super(property);
this.className = className;
this.interpreterRunner = interpreterRunner;
this.interpreterPath = interpreterPath;
this.env = env;
this.connectTimeout = connectTimeout;
}
@Override
public String getClassName() {
return className;
}
public RemoteInterpreterProcess getInterpreterProcess() {
synchronized (interpreterGroupReference) {
if (interpreterGroupReference.containsKey(getInterpreterGroupKey(getInterpreterGroup()))) {
RemoteInterpreterProcess interpreterProcess = interpreterGroupReference
.get(getInterpreterGroupKey(getInterpreterGroup()));
try {
return interpreterProcess;
} catch (Exception e) {
throw new InterpreterException(e);
}
} else {
throw new InterpreterException("Unexpected error");
}
}
}
private synchronized void init() {
if (initialized == true) {
return;
}
RemoteInterpreterProcess interpreterProcess = null;
synchronized (interpreterGroupReference) {
if (interpreterGroupReference.containsKey(getInterpreterGroupKey(getInterpreterGroup()))) {
interpreterProcess = interpreterGroupReference
.get(getInterpreterGroupKey(getInterpreterGroup()));
} else {
throw new InterpreterException("Unexpected error");
}
}
int rc = interpreterProcess.reference(getInterpreterGroup());
synchronized (interpreterProcess) {
// when first process created
if (rc == 1) {
// create all interpreter class in this interpreter group
Client client = null;
try {
client = interpreterProcess.getClient();
} catch (Exception e1) {
throw new InterpreterException(e1);
}
try {
for (Interpreter intp : this.getInterpreterGroup()) {
logger.info("Create remote interpreter {}", intp.getClassName());
client.createInterpreter(intp.getClassName(), (Map) property);
}
} catch (TException e) {
throw new InterpreterException(e);
} finally {
interpreterProcess.releaseClient(client);
}
}
}
initialized = true;
}
@Override
public void open() {
init();
}
@Override
public void close() {
RemoteInterpreterProcess interpreterProcess = getInterpreterProcess();
Client client = null;
try {
client = interpreterProcess.getClient();
} catch (Exception e1) {
throw new InterpreterException(e1);
}
try {
client.close(className);
} catch (TException e) {
throw new InterpreterException(e);
} finally {
interpreterProcess.releaseClient(client);
}
interpreterProcess.dereference();
}
@Override
public InterpreterResult interpret(String st, InterpreterContext context) {
FormType form = getFormType();
RemoteInterpreterProcess interpreterProcess = getInterpreterProcess();
Client client = null;
try {
client = interpreterProcess.getClient();
} catch (Exception e1) {
throw new InterpreterException(e1);
}
InterpreterContextRunnerPool interpreterContextRunnerPool = interpreterProcess
.getInterpreterContextRunnerPool();
List runners = context.getRunners();
if (runners != null && runners.size() != 0) {
// assume all runners in this InterpreterContext have the same note id
String noteId = runners.get(0).getNoteId();
interpreterContextRunnerPool.clear(noteId);
interpreterContextRunnerPool.addAll(noteId, runners);
}
try {
GUI settings = context.getGui();
RemoteInterpreterResult remoteResult = client.interpret(className, st, convert(context));
Map remoteConfig = (Map) gson.fromJson(
remoteResult.getConfig(), new TypeToken
© 2015 - 2025 Weber Informatics LLC | Privacy Policy