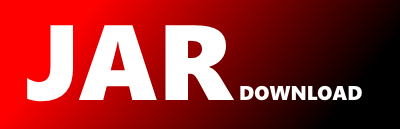
org.apereo.cas.jdbc.QueryAndEncodeDatabasePasswordEncoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cas-server-support-jdbc-authentication Show documentation
Show all versions of cas-server-support-jdbc-authentication Show documentation
cas-server-support-jdbc-authentication
The newest version!
package org.apereo.cas.jdbc;
import org.apereo.cas.configuration.model.support.jdbc.authn.QueryEncodeJdbcAuthenticationProperties;
import org.apereo.cas.util.DigestUtils;
import org.apereo.cas.util.function.FunctionUtils;
import lombok.RequiredArgsConstructor;
import lombok.val;
import org.apache.commons.lang3.ArrayUtils;
import java.nio.charset.StandardCharsets;
import java.util.Map;
/**
* This is {@link QueryAndEncodeDatabasePasswordEncoder}.
*
* @author Misagh Moayyed
* @since 7.0.0
*/
@RequiredArgsConstructor
public class QueryAndEncodeDatabasePasswordEncoder implements DatabasePasswordEncoder {
protected final QueryEncodeJdbcAuthenticationProperties properties;
@Override
public String encode(final String password, final Map queryValues) {
val iterations = getIterations(queryValues);
val dynaSalt = getDynamicSalt(queryValues);
val staticSalt = getStaticSalt(queryValues);
return DigestUtils.rawDigest(properties.getAlgorithmName(), staticSalt, dynaSalt, password, iterations);
}
protected int getIterations(final Map queryValues) {
var iterations = properties.getNumberOfIterations();
if (queryValues.containsKey(properties.getNumberOfIterationsFieldName())) {
val longAsStr = queryValues.get(properties.getNumberOfIterationsFieldName()).toString();
iterations = Integer.parseInt(longAsStr);
}
return iterations;
}
protected byte[] getStaticSalt(final Map queryValues) {
return FunctionUtils.doIfNotBlank(properties.getStaticSalt(),
() -> properties.getStaticSalt().getBytes(StandardCharsets.UTF_8),
() -> ArrayUtils.EMPTY_BYTE_ARRAY);
}
protected byte[] getDynamicSalt(final Map queryValues) {
return queryValues.containsKey(properties.getSaltFieldName())
? queryValues.get(properties.getSaltFieldName()).toString().getBytes(StandardCharsets.UTF_8)
: ArrayUtils.EMPTY_BYTE_ARRAY;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy