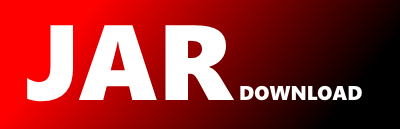
org.apereo.cas.webauthn.DynamoDbWebAuthnCredentialRepository Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cas-server-support-webauthn-dynamodb Show documentation
Show all versions of cas-server-support-webauthn-dynamodb Show documentation
cas-server-support-webauthn-dynamodb
package org.apereo.cas.webauthn;
import org.apereo.cas.configuration.CasConfigurationProperties;
import org.apereo.cas.util.crypto.CipherExecutor;
import org.apereo.cas.webauthn.storage.BaseWebAuthnCredentialRepository;
import com.fasterxml.jackson.core.type.TypeReference;
import com.yubico.data.CredentialRegistration;
import lombok.extern.slf4j.Slf4j;
import lombok.val;
import org.jooq.lambda.Unchecked;
import java.time.Clock;
import java.time.Instant;
import java.util.Collection;
import java.util.List;
import java.util.Locale;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* This is {@link DynamoDbWebAuthnCredentialRepository}.
*
* @author Misagh Moayyed
* @since 6.3.0
*/
@Slf4j
public class DynamoDbWebAuthnCredentialRepository extends BaseWebAuthnCredentialRepository {
private final DynamoDbWebAuthnFacilitator facilitator;
public DynamoDbWebAuthnCredentialRepository(final CasConfigurationProperties properties,
final CipherExecutor cipherExecutor,
final DynamoDbWebAuthnFacilitator facilitator) {
super(properties, cipherExecutor);
this.facilitator = facilitator;
}
@Override
public Collection getRegistrationsByUsername(final String username) {
return facilitator.getAccountsBy(username.trim().toLowerCase(Locale.ENGLISH))
.map(DynamoDbWebAuthnCredentialRegistration::getRecords)
.flatMap(List::stream)
.map(record -> getCipherExecutor().decode(record))
.map(Unchecked.function(record -> WebAuthnUtils.getObjectMapper().readValue(record, new TypeReference() {
})))
.collect(Collectors.toList());
}
@Override
public Stream stream() {
return facilitator.load()
.map(DynamoDbWebAuthnCredentialRegistration::getRecords)
.flatMap(List::stream)
.map(record -> getCipherExecutor().decode(record))
.map(Unchecked.function(record -> WebAuthnUtils.getObjectMapper().readValue(record, new TypeReference<>() {
})));
}
@Override
protected void update(final String username, final Collection records) {
if (records.isEmpty()) {
LOGGER.debug("No records are provided for [{}] so entry will be removed", username);
facilitator.remove(username.trim().toLowerCase(Locale.ENGLISH));
} else {
val jsonRecords = records.stream()
.map(record -> {
if (record.getRegistrationTime() == null) {
return record.withRegistrationTime(Instant.now(Clock.systemUTC()));
}
return record;
})
.map(Unchecked.function(record -> getCipherExecutor().encode(WebAuthnUtils.getObjectMapper().writeValueAsString(record))))
.collect(Collectors.toList());
val entry = DynamoDbWebAuthnCredentialRegistration.builder()
.records(jsonRecords)
.username(username.trim().toLowerCase(Locale.ENGLISH))
.build();
facilitator.save(entry);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy