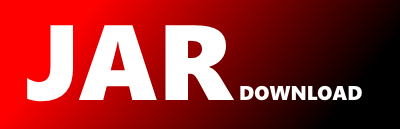
org.apfloat.calc.CalculatorParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apfloat-calc Show documentation
Show all versions of apfloat-calc Show documentation
Interactive arbitrary precision calculator application
The newest version!
/* CalculatorParser.java */
/* Generated By:JavaCC: Do not edit this line. CalculatorParser.java */
package org.apfloat.calc;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.io.Reader;
import java.io.Writer;
import java.util.List;
import java.util.ArrayList;
public class CalculatorParser implements CalculatorParserConstants {
public CalculatorParser(InputStream in, OutputStream out, CalculatorImpl calculatorImpl)
{
this(in);
this.out = new PrintWriter(new OutputStreamWriter(out), true);
this.calculatorImpl = calculatorImpl;
}
public CalculatorParser(Reader in, Writer out, CalculatorImpl calculatorImpl)
{
this(in);
this.out = new PrintWriter(out, true);
this.calculatorImpl = calculatorImpl;
}
public CalculatorParser(Reader in, PrintWriter out, CalculatorImpl calculatorImpl)
{
this(in);
this.out = out;
this.calculatorImpl = calculatorImpl;
}
private PrintWriter out;
private CalculatorImpl calculatorImpl;
final public boolean parseOneLine() throws ParseException {Number a;
if (jj_2_1(2)) {
a = expression();
this.out.println(this.calculatorImpl.format(a));
} else {
;
}
label_1:
while (true) {
if (jj_2_2(2)) {
;
} else {
break label_1;
}
jj_consume_token(DELIMITER);
a = expression();
this.out.println(this.calculatorImpl.format(a));
}
if (jj_2_3(2)) {
jj_consume_token(DELIMITER);
} else {
;
}
if (jj_2_4(2)) {
jj_consume_token(EOL);
{if ("" != null) return true;}
} else if (jj_2_5(2)) {
jj_consume_token(0);
{if ("" != null) return false;}
} else {
jj_consume_token(-1);
throw new ParseException();
}
throw new Error("Missing return statement in function");
}
final public Number expression() throws ParseException {Number a;
if (jj_2_6(2)) {
a = assignmentExpression();
} else if (jj_2_7(2)) {
a = additiveExpression();
} else {
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return a;}
throw new Error("Missing return statement in function");
}
final public Number assignmentExpression() throws ParseException {String v;
Number a;
v = variable();
if (jj_2_8(2)) {
jj_consume_token(11);
a = additiveExpression();
this.calculatorImpl.setVariable(v, a);
} else if (jj_2_9(2)) {
jj_consume_token(12);
a = additiveExpression();
this.calculatorImpl.setVariable(v, this.calculatorImpl.add(this.calculatorImpl.getVariable(v), a));
} else if (jj_2_10(2)) {
jj_consume_token(13);
a = additiveExpression();
this.calculatorImpl.setVariable(v, this.calculatorImpl.subtract(this.calculatorImpl.getVariable(v), a));
} else if (jj_2_11(2)) {
jj_consume_token(14);
a = additiveExpression();
this.calculatorImpl.setVariable(v, this.calculatorImpl.multiply(this.calculatorImpl.getVariable(v), a));
} else if (jj_2_12(2)) {
jj_consume_token(15);
a = additiveExpression();
this.calculatorImpl.setVariable(v, this.calculatorImpl.divide(this.calculatorImpl.getVariable(v), a));
} else if (jj_2_13(2)) {
jj_consume_token(16);
a = additiveExpression();
this.calculatorImpl.setVariable(v, this.calculatorImpl.mod(this.calculatorImpl.getVariable(v), a));
} else if (jj_2_14(2)) {
jj_consume_token(17);
a = additiveExpression();
this.calculatorImpl.setVariable(v, this.calculatorImpl.pow(this.calculatorImpl.getVariable(v), a));
} else {
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return this.calculatorImpl.getVariable(v);}
throw new Error("Missing return statement in function");
}
final public Number additiveExpression() throws ParseException {Number a;
Number b;
a = multiplicativeExpression();
label_2:
while (true) {
if (jj_2_15(2)) {
;
} else {
break label_2;
}
if (jj_2_16(2)) {
jj_consume_token(18);
b = multiplicativeExpression();
a = this.calculatorImpl.add(a, b);
} else if (jj_2_17(2)) {
jj_consume_token(19);
b = multiplicativeExpression();
a = this.calculatorImpl.subtract(a, b);
} else {
jj_consume_token(-1);
throw new ParseException();
}
}
{if ("" != null) return a;}
throw new Error("Missing return statement in function");
}
final public Number multiplicativeExpression() throws ParseException {Number a;
Number b;
a = unaryExpression();
label_3:
while (true) {
if (jj_2_18(2)) {
;
} else {
break label_3;
}
if (jj_2_19(2)) {
jj_consume_token(20);
b = unaryExpression();
a = this.calculatorImpl.multiply(a, b);
} else if (jj_2_20(2)) {
jj_consume_token(21);
b = unaryExpression();
a = this.calculatorImpl.divide(a, b);
} else if (jj_2_21(2)) {
jj_consume_token(22);
b = unaryExpression();
a = this.calculatorImpl.mod(a, b);
} else {
jj_consume_token(-1);
throw new ParseException();
}
}
{if ("" != null) return a;}
throw new Error("Missing return statement in function");
}
final public Number unaryExpression() throws ParseException {Number a;
if (jj_2_22(2)) {
jj_consume_token(19);
a = unaryExpression();
{if ("" != null) return this.calculatorImpl.negate(a);}
} else if (jj_2_23(2)) {
jj_consume_token(18);
a = unaryExpression();
{if ("" != null) return a;}
} else if (jj_2_24(2)) {
a = powerExpression();
{if ("" != null) return a;}
} else {
jj_consume_token(-1);
throw new ParseException();
}
throw new Error("Missing return statement in function");
}
final public Number powerExpression() throws ParseException {Number a;
Number b;
a = factorialExpression();
if (jj_2_25(2)) {
jj_consume_token(23);
b = unaryExpression();
a = this.calculatorImpl.pow(a, b);
} else {
;
}
{if ("" != null) return a;}
throw new Error("Missing return statement in function");
}
final public Number factorialExpression() throws ParseException {Number a;
a = element();
label_4:
while (true) {
if (jj_2_26(2)) {
;
} else {
break label_4;
}
if (jj_2_27(2)) {
jj_consume_token(24);
a = this.calculatorImpl.doubleFactorial(a);
} else if (jj_2_28(2)) {
jj_consume_token(25);
a = this.calculatorImpl.factorial(a);
} else {
jj_consume_token(-1);
throw new ParseException();
}
}
{if ("" != null) return a;}
throw new Error("Missing return statement in function");
}
final public Number element() throws ParseException {String v;
Number a;
if (jj_2_29(2)) {
a = constant();
{if ("" != null) return a;}
} else if (jj_2_30(2)) {
a = function();
{if ("" != null) return a;}
} else if (jj_2_31(2)) {
v = variable();
{if ("" != null) return this.calculatorImpl.getVariable(v);}
} else if (jj_2_32(2)) {
jj_consume_token(26);
a = expression();
jj_consume_token(27);
{if ("" != null) return a;}
} else {
jj_consume_token(-1);
throw new ParseException();
}
throw new Error("Missing return statement in function");
}
final public String variable() throws ParseException {String v;
v = identifier();
{if ("" != null) return v;}
throw new Error("Missing return statement in function");
}
final public Number function() throws ParseException {String v;
List l;
Number a;
v = identifier();
jj_consume_token(26);
l = argumentList();
jj_consume_token(27);
{if ("" != null) return this.calculatorImpl.function(v, l);}
throw new Error("Missing return statement in function");
}
final public List argumentList() throws ParseException {List list = new ArrayList();
Number a;
if (jj_2_34(2)) {
a = expression();
list.add(a);
label_5:
while (true) {
if (jj_2_33(2)) {
;
} else {
break label_5;
}
jj_consume_token(28);
a = expression();
list.add(a);
}
} else {
;
}
{if ("" != null) return list;}
throw new Error("Missing return statement in function");
}
final public String identifier() throws ParseException {Token t;
t = jj_consume_token(IDENTIFIER);
{if ("" != null) return t.toString();}
throw new Error("Missing return statement in function");
}
final public Number constant() throws ParseException {Token t;
if (jj_2_35(2)) {
t = jj_consume_token(INTEGER);
{if ("" != null) return this.calculatorImpl.parseInteger(t.toString());}
} else if (jj_2_36(2)) {
t = jj_consume_token(DECIMAL);
{if ("" != null) return this.calculatorImpl.parseDecimal(t.toString());}
} else {
jj_consume_token(-1);
throw new ParseException();
}
throw new Error("Missing return statement in function");
}
private boolean jj_2_1(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_1()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_2(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_2()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_3(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_3()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_4(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_4()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_5(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_5()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_6(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_6()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_7(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_7()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_8(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_8()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_9(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_9()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_10(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_10()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_11(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_11()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_12(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_12()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_13(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_13()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_14(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_14()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_15(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_15()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_16(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_16()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_17(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_17()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_18(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_18()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_19(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_19()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_20(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_20()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_21(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_21()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_22(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_22()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_23(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_23()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_24(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_24()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_25(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_25()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_26(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_26()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_27(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_27()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_28(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_28()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_29(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_29()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_30(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_30()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_31(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_31()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_32(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_32()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_33(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_33()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_34(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_34()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_35(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_35()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_2_36(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return (!jj_3_36()); }
catch(LookaheadSuccess ls) { return true; }
}
private boolean jj_3_4()
{
if (jj_scan_token(EOL)) return true;
return false;
}
private boolean jj_3_26()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3_27()) {
jj_scanpos = xsp;
if (jj_3_28()) return true;
}
return false;
}
private boolean jj_3_27()
{
if (jj_scan_token(24)) return true;
return false;
}
private boolean jj_3_3()
{
if (jj_scan_token(DELIMITER)) return true;
return false;
}
private boolean jj_3R_factorialExpression_178_5_15()
{
if (jj_3R_element_192_5_17()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3_26()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3_2()
{
if (jj_scan_token(DELIMITER)) return true;
if (jj_3R_expression_95_5_6()) return true;
return false;
}
private boolean jj_3_1()
{
if (jj_3R_expression_95_5_6()) return true;
return false;
}
private boolean jj_3_25()
{
if (jj_scan_token(23)) return true;
if (jj_3R_unaryExpression_155_5_10()) return true;
return false;
}
private boolean jj_3R_powerExpression_166_5_11()
{
if (jj_3R_factorialExpression_178_5_15()) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3_25()) jj_scanpos = xsp;
return false;
}
private boolean jj_3_24()
{
if (jj_3R_powerExpression_166_5_11()) return true;
return false;
}
private boolean jj_3_23()
{
if (jj_scan_token(18)) return true;
if (jj_3R_unaryExpression_155_5_10()) return true;
return false;
}
private boolean jj_3R_unaryExpression_155_5_10()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3_22()) {
jj_scanpos = xsp;
if (jj_3_23()) {
jj_scanpos = xsp;
if (jj_3_24()) return true;
}
}
return false;
}
private boolean jj_3_22()
{
if (jj_scan_token(19)) return true;
if (jj_3R_unaryExpression_155_5_10()) return true;
return false;
}
private boolean jj_3_36()
{
if (jj_scan_token(DECIMAL)) return true;
return false;
}
private boolean jj_3R_constant_245_5_12()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3_35()) {
jj_scanpos = xsp;
if (jj_3_36()) return true;
}
return false;
}
private boolean jj_3_35()
{
if (jj_scan_token(INTEGER)) return true;
return false;
}
private boolean jj_3_21()
{
if (jj_scan_token(22)) return true;
if (jj_3R_unaryExpression_155_5_10()) return true;
return false;
}
private boolean jj_3_20()
{
if (jj_scan_token(21)) return true;
if (jj_3R_unaryExpression_155_5_10()) return true;
return false;
}
private boolean jj_3_18()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3_19()) {
jj_scanpos = xsp;
if (jj_3_20()) {
jj_scanpos = xsp;
if (jj_3_21()) return true;
}
}
return false;
}
private boolean jj_3_19()
{
if (jj_scan_token(20)) return true;
if (jj_3R_unaryExpression_155_5_10()) return true;
return false;
}
private boolean jj_3R_identifier_237_5_16()
{
if (jj_scan_token(IDENTIFIER)) return true;
return false;
}
private boolean jj_3R_multiplicativeExpression_141_5_9()
{
if (jj_3R_unaryExpression_155_5_10()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3_18()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3_33()
{
if (jj_scan_token(28)) return true;
if (jj_3R_expression_95_5_6()) return true;
return false;
}
private boolean jj_3_17()
{
if (jj_scan_token(19)) return true;
if (jj_3R_multiplicativeExpression_141_5_9()) return true;
return false;
}
private boolean jj_3_16()
{
if (jj_scan_token(18)) return true;
if (jj_3R_multiplicativeExpression_141_5_9()) return true;
return false;
}
private boolean jj_3_15()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3_16()) {
jj_scanpos = xsp;
if (jj_3_17()) return true;
}
return false;
}
private boolean jj_3_34()
{
if (jj_3R_expression_95_5_6()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3_33()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_additiveExpression_127_5_8()
{
if (jj_3R_multiplicativeExpression_141_5_9()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3_15()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3_14()
{
if (jj_scan_token(17)) return true;
if (jj_3R_additiveExpression_127_5_8()) return true;
return false;
}
private boolean jj_3_13()
{
if (jj_scan_token(16)) return true;
if (jj_3R_additiveExpression_127_5_8()) return true;
return false;
}
private boolean jj_3R_function_213_5_13()
{
if (jj_3R_identifier_237_5_16()) return true;
if (jj_scan_token(26)) return true;
return false;
}
private boolean jj_3_12()
{
if (jj_scan_token(15)) return true;
if (jj_3R_additiveExpression_127_5_8()) return true;
return false;
}
private boolean jj_3_11()
{
if (jj_scan_token(14)) return true;
if (jj_3R_additiveExpression_127_5_8()) return true;
return false;
}
private boolean jj_3_10()
{
if (jj_scan_token(13)) return true;
if (jj_3R_additiveExpression_127_5_8()) return true;
return false;
}
private boolean jj_3_9()
{
if (jj_scan_token(12)) return true;
if (jj_3R_additiveExpression_127_5_8()) return true;
return false;
}
private boolean jj_3_8()
{
if (jj_scan_token(11)) return true;
if (jj_3R_additiveExpression_127_5_8()) return true;
return false;
}
private boolean jj_3R_variable_203_5_14()
{
if (jj_3R_identifier_237_5_16()) return true;
return false;
}
private boolean jj_3R_assignmentExpression_108_5_7()
{
if (jj_3R_variable_203_5_14()) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3_8()) {
jj_scanpos = xsp;
if (jj_3_9()) {
jj_scanpos = xsp;
if (jj_3_10()) {
jj_scanpos = xsp;
if (jj_3_11()) {
jj_scanpos = xsp;
if (jj_3_12()) {
jj_scanpos = xsp;
if (jj_3_13()) {
jj_scanpos = xsp;
if (jj_3_14()) return true;
}
}
}
}
}
}
return false;
}
private boolean jj_3_7()
{
if (jj_3R_additiveExpression_127_5_8()) return true;
return false;
}
private boolean jj_3_6()
{
if (jj_3R_assignmentExpression_108_5_7()) return true;
return false;
}
private boolean jj_3_32()
{
if (jj_scan_token(26)) return true;
if (jj_3R_expression_95_5_6()) return true;
return false;
}
private boolean jj_3_31()
{
if (jj_3R_variable_203_5_14()) return true;
return false;
}
private boolean jj_3_30()
{
if (jj_3R_function_213_5_13()) return true;
return false;
}
private boolean jj_3R_element_192_5_17()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3_29()) {
jj_scanpos = xsp;
if (jj_3_30()) {
jj_scanpos = xsp;
if (jj_3_31()) {
jj_scanpos = xsp;
if (jj_3_32()) return true;
}
}
}
return false;
}
private boolean jj_3_29()
{
if (jj_3R_constant_245_5_12()) return true;
return false;
}
private boolean jj_3R_expression_95_5_6()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3_6()) {
jj_scanpos = xsp;
if (jj_3_7()) return true;
}
return false;
}
private boolean jj_3_5()
{
if (jj_scan_token(0)) return true;
return false;
}
private boolean jj_3_28()
{
if (jj_scan_token(25)) return true;
return false;
}
/** Generated Token Manager. */
public CalculatorParserTokenManager token_source;
SimpleCharStream jj_input_stream;
/** Current token. */
public Token token;
/** Next token. */
public Token jj_nt;
private int jj_ntk;
private Token jj_scanpos, jj_lastpos;
private int jj_la;
/** Constructor with InputStream. */
public CalculatorParser(java.io.InputStream stream) {
this(stream, null);
}
/** Constructor with InputStream and supplied encoding */
public CalculatorParser(java.io.InputStream stream, String encoding) {
try { jj_input_stream = new SimpleCharStream(stream, encoding, 1, 1); } catch(java.io.UnsupportedEncodingException e) { throw new RuntimeException(e); }
token_source = new CalculatorParserTokenManager(jj_input_stream);
token = new Token();
jj_ntk = -1;
}
/** Reinitialise. */
public void ReInit(java.io.InputStream stream) {
ReInit(stream, null);
}
/** Reinitialise. */
public void ReInit(java.io.InputStream stream, String encoding) {
try { jj_input_stream.ReInit(stream, encoding, 1, 1); } catch(java.io.UnsupportedEncodingException e) { throw new RuntimeException(e); }
token_source.ReInit(jj_input_stream);
token = new Token();
jj_ntk = -1;
}
/** Constructor. */
public CalculatorParser(java.io.Reader stream) {
jj_input_stream = new SimpleCharStream(stream, 1, 1);
token_source = new CalculatorParserTokenManager(jj_input_stream);
token = new Token();
jj_ntk = -1;
}
/** Reinitialise. */
public void ReInit(java.io.Reader stream) {
if (jj_input_stream == null) {
jj_input_stream = new SimpleCharStream(stream, 1, 1);
} else {
jj_input_stream.ReInit(stream, 1, 1);
}
if (token_source == null) {
token_source = new CalculatorParserTokenManager(jj_input_stream);
}
token_source.ReInit(jj_input_stream);
token = new Token();
jj_ntk = -1;
}
/** Constructor with generated Token Manager. */
public CalculatorParser(CalculatorParserTokenManager tm) {
token_source = tm;
token = new Token();
jj_ntk = -1;
}
/** Reinitialise. */
public void ReInit(CalculatorParserTokenManager tm) {
token_source = tm;
token = new Token();
jj_ntk = -1;
}
private Token jj_consume_token(int kind) throws ParseException {
Token oldToken;
if ((oldToken = token).next != null) token = token.next;
else token = token.next = token_source.getNextToken();
jj_ntk = -1;
if (token.kind == kind) {
return token;
}
token = oldToken;
throw generateParseException();
}
@SuppressWarnings("serial")
static private final class LookaheadSuccess extends java.lang.Error {
@Override
public Throwable fillInStackTrace() {
return this;
}
}
static private final LookaheadSuccess jj_ls = new LookaheadSuccess();
private boolean jj_scan_token(int kind) {
if (jj_scanpos == jj_lastpos) {
jj_la--;
if (jj_scanpos.next == null) {
jj_lastpos = jj_scanpos = jj_scanpos.next = token_source.getNextToken();
} else {
jj_lastpos = jj_scanpos = jj_scanpos.next;
}
} else {
jj_scanpos = jj_scanpos.next;
}
if (jj_scanpos.kind != kind) return true;
if (jj_la == 0 && jj_scanpos == jj_lastpos) throw jj_ls;
return false;
}
/** Get the next Token. */
final public Token getNextToken() {
if (token.next != null) token = token.next;
else token = token.next = token_source.getNextToken();
jj_ntk = -1;
return token;
}
/** Get the specific Token. */
final public Token getToken(int index) {
Token t = token;
for (int i = 0; i < index; i++) {
if (t.next != null) t = t.next;
else t = t.next = token_source.getNextToken();
}
return t;
}
private int jj_ntk_f() {
if ((jj_nt=token.next) == null)
return (jj_ntk = (token.next=token_source.getNextToken()).kind);
else
return (jj_ntk = jj_nt.kind);
}
/** Generate ParseException. */
public ParseException generateParseException() {
Token errortok = token.next;
int line = errortok.beginLine, column = errortok.beginColumn;
String mess = (errortok.kind == 0) ? tokenImage[0] : errortok.image;
return new ParseException("Parse error at line " + line + ", column " + column + ". Encountered: " + mess);
}
private boolean trace_enabled;
/** Trace enabled. */
final public boolean trace_enabled() {
return trace_enabled;
}
/** Enable tracing. */
final public void enable_tracing() {
}
/** Disable tracing. */
final public void disable_tracing() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy