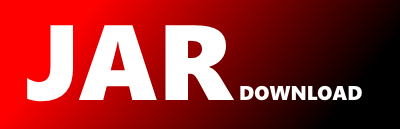
org.apfloat.ConcurrentWeakHashMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apfloat Show documentation
Show all versions of apfloat Show documentation
High performance arbitrary precision arithmetic library
package org.apfloat;
import java.lang.ref.ReferenceQueue;
import java.lang.ref.WeakReference;
import java.util.AbstractMap;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
/**
* Combination of WeakHashMap and ConcurrentHashMap,
* providing weak keys and non-blocking access.
*
* @since 1.5
* @version 1.5
* @author Mikko Tommila
*/
class ConcurrentWeakHashMap
extends AbstractMap
{
private static class Key
extends WeakReference
© 2015 - 2024 Weber Informatics LLC | Privacy Policy