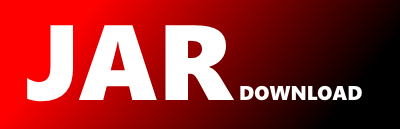
org.apfloat.internal.IntShortConvolutionStrategy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apfloat Show documentation
Show all versions of apfloat Show documentation
High performance arbitrary precision arithmetic library
package org.apfloat.internal;
import org.apfloat.ApfloatContext;
import org.apfloat.ApfloatRuntimeException;
import org.apfloat.spi.ConvolutionStrategy;
import org.apfloat.spi.DataStorageBuilder;
import org.apfloat.spi.DataStorage;
import org.apfloat.spi.ArrayAccess;
/**
* Short convolution strategy.
* Performs a simple multiplication when the size of one operand is 1.
*
* @version 1.1
* @author Mikko Tommila
*/
public class IntShortConvolutionStrategy
extends IntBaseMath
implements ConvolutionStrategy
{
// Implementation notes:
// - Assumes that the operands have been already truncated to match resultSize (the resultSize argument is ignored)
// - This class shouldn't be converted to a single class using generics because the performance impact is noticeable
/**
* Creates a convolution strategy using the specified radix.
*
* @param radix The radix that will be used.
*/
public IntShortConvolutionStrategy(int radix)
{
super(radix);
}
public DataStorage convolute(DataStorage x, DataStorage y, long resultSize)
throws ApfloatRuntimeException
{
DataStorage shortStorage, longStorage, resultStorage;
if (x.getSize() > 1)
{
shortStorage = y;
longStorage = x;
}
else
{
shortStorage = x;
longStorage = y;
}
assert (shortStorage.getSize() == 1);
long size = longStorage.getSize() + 1;
ArrayAccess arrayAccess = shortStorage.getArray(DataStorage.READ, 0, 1);
int factor = arrayAccess.getIntData()[arrayAccess.getOffset()];
arrayAccess.close();
ApfloatContext ctx = ApfloatContext.getContext();
DataStorageBuilder dataStorageBuilder = ctx.getBuilderFactory().getDataStorageBuilder();
resultStorage = dataStorageBuilder.createDataStorage(size * 4);
resultStorage.setSize(size);
DataStorage.Iterator src = longStorage.iterator(DataStorage.READ, size - 1, 0),
dst = resultStorage.iterator(DataStorage.WRITE, size, 0);
int carry = baseMultiplyAdd(src, null, factor, 0, dst, size - 1);
dst.setInt(carry);
dst.close();
return resultStorage;
}
private static final long serialVersionUID = 7238463434254768541L;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy