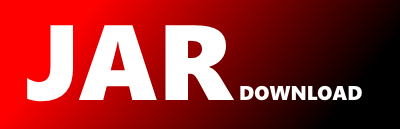
org.apichart.common.modules.context.WindowsServerInfos Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-certificate-starter Show documentation
Show all versions of spring-certificate-starter Show documentation
project for Spring Boot, to provide a reference for ensuring the stability of the system!
The newest version!
package org.apichart.common.modules.context;
import java.net.InetAddress;
import java.util.List;
import java.util.Scanner;
import java.util.stream.Collectors;
/**
* 用于获取客户Windows服务器的基本信息
*/
public class WindowsServerInfos extends AbstractServerInfos{
@Override
protected List getIpAddress() throws Exception {
List result = null;
//获取所有网络接口
List inetAddresses = getLocalAllInetAddress();
if(inetAddresses != null && inetAddresses.size() > 0){
result = inetAddresses.stream().map(InetAddress::getHostAddress).distinct().map(String::toLowerCase).collect(Collectors.toList());
}
return result;
}
@Override
protected List getMacAddress() throws Exception {
List result = null;
//1. 获取所有网络接口
List inetAddresses = getLocalAllInetAddress();
if(inetAddresses != null && inetAddresses.size() > 0){
//2. 获取所有网络接口的Mac地址
result = inetAddresses.stream().map(this::getMacByInetAddress).distinct().collect(Collectors.toList());
}
return result;
}
@Override
protected String getCPUSerial() throws Exception {
//序列号
String serialNumber = "";
//使用WMIC获取CPU序列号
Process process = Runtime.getRuntime().exec("wmic cpu get processorid");
process.getOutputStream().close();
Scanner scanner = new Scanner(process.getInputStream());
if(scanner != null && scanner.hasNext()){
scanner.next();
}
if(scanner.hasNext()){
serialNumber = scanner.next().trim();
}
scanner.close();
return serialNumber;
}
@Override
protected String getMainBoardSerial() throws Exception {
//序列号
String serialNumber = "";
//使用WMIC获取主板序列号
Process process = Runtime.getRuntime().exec("wmic baseboard get serialnumber");
process.getOutputStream().close();
Scanner scanner = new Scanner(process.getInputStream());
if(scanner != null && scanner.hasNext()){
scanner.next();
}
if(scanner.hasNext()){
serialNumber = scanner.next().trim();
}
scanner.close();
return serialNumber;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy