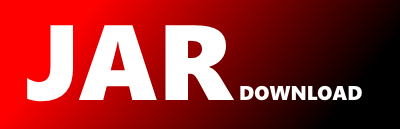
org.appconn.AppServer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appconn Show documentation
Show all versions of appconn Show documentation
Java library for easy and fast implementation of distributed applications, including, optimized
serialization, high availability administration and server resources management.
package org.appconn;
import org.appconn.channel.AbstractServer;
import org.appconn.channel.TcpServer;
import org.appconn.mgmt.Address;
import org.appconn.mgmt.AppServerService;
import org.appconn.mgmt.Server;
import org.appconn.mgmt.ServerProperties;
import java.io.IOException;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* appconn AppServer.
*/
final class AppServer extends Server implements AppServerService {
private final Integer backlog;
private final Integer selectorsLength;
private final Integer maximumPoolSize;
private final Integer queueCapacity;
private AbstractServer server;
private Interruptible interruptible;
private AppServer(int id, int mappingKey, Address address, byte state, Integer backlog, Integer selectorsLength, Integer maximumPoolSize, Integer queueCapacity) throws IOException {
super(id, mappingKey, address, STATE.OFFLINE.byteValue());
this.backlog = backlog;
this.selectorsLength = selectorsLength;
this.maximumPoolSize = maximumPoolSize;
this.queueCapacity = queueCapacity;
server = null;
interruptible = null;
setServerState(state);
}
AppServer(ServerProperties serverProperties) throws IOException {
this(serverProperties.getId(),
serverProperties.getMappingKey(),
serverProperties.getAddress(),
serverProperties.getState(),
serverProperties.getBacklog(),
serverProperties.getSelectorsLength(),
serverProperties.getMaximumPoolSize(),
serverProperties.getQueueCapacity());
}
@Override
public synchronized byte getState() {
return super.getState();
}
@Override
public synchronized void setState(byte state) {
try {
setServerState(state);
} catch (IOException e) {
Logger.getLogger("org.appconn").log(Level.WARNING, e, e::getMessage);
}
}
private void setServerState(byte state) throws IOException {
if (super.getState() != state) {
if (STATE.IS_ONLINE.or(STATE.IS_SPARE).test(state)) {
if (server == null) {
server = new TcpServer(getAddress().toSocketAddress(),
backlog != null ? backlog : TcpServer.DEFAULT_BACKLOG,
selectorsLength != null ? selectorsLength : 4,
maximumPoolSize != null ? maximumPoolSize : TcpServer.DEFAULT_MAXIMUM_POOL_SIZE,
queueCapacity != null ? queueCapacity : TcpServer.DEFAULT_QUEUE_CAPACITY);
}
super.setState(state);
} else if (STATE.IS_OFFLINE.test(state)) {
if (server != null) {
server.interrupt();
server = null;
}
super.setState(state);
if (interruptible != null) interruptible.interrupt();
}
Logger.getLogger("org.appconn").log(Level.CONFIG, super.toString());
}
}
/**
* @return the AbstractServer
*/
AbstractServer getServer() {
return server;
}
/**
* Sets the Interruptible implementation.
*/
void setInterruptible(Interruptible interruptible) {
this.interruptible = interruptible;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy