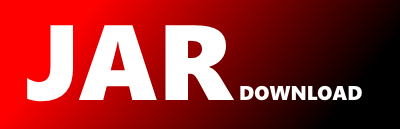
org.appconn.channel.AbstractServer Maven / Gradle / Ivy
package org.appconn.channel;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import java.util.concurrent.*;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* appconn AbstractServer.
*/
public abstract class AbstractServer extends Thread {
public static final int DEFAULT_MAXIMUM_POOL_SIZE = 32;
public static final int DEFAULT_QUEUE_CAPACITY = DEFAULT_MAXIMUM_POOL_SIZE << 2;
private static final int KEEP_ALIVE_TIME = 32;
final Map stubMap;
final ExecutorService executorService;
/**
* AbstractServer constructor.
* @param name the name of the thread
* @param maximumPoolSize the maximumPoolSize for ThreadPoolExecutor
* @param queueCapacity the queueCapacity for the pool waiting queue
*/
AbstractServer(String name, int maximumPoolSize, int queueCapacity) {
super(name);
stubMap = new HashMap<>();
executorService = new ThreadPoolExecutor(0, maximumPoolSize, KEEP_ALIVE_TIME, TimeUnit.SECONDS, new LinkedBlockingQueue<>(queueCapacity), new ServerThreadFactory(getClass().getName()));
}
@Override
public void interrupt() {
executorService.shutdown();
super.interrupt();
}
/**
* Puts the stub.
* @param key the stub key
* @param stub the MethodStub
*/
public void put(int key, MethodStub stub) {
if (Optional.ofNullable(stubMap.put(key, stub)).isPresent())
Logger.getLogger("org.appconn").log(Level.WARNING, "replace " + stub.getClass().getName() + "/" + key);
else Logger.getLogger("org.appconn").log(Level.CONFIG, stub.getClass().getName() + "/" + key);
}
private class ServerThreadFactory implements ThreadFactory {
private final ThreadGroup group;
private final AtomicInteger index;
private final String name;
private ServerThreadFactory(String name) {
SecurityManager securityManager = System.getSecurityManager();
group = (securityManager != null) ? securityManager.getThreadGroup() : Thread.currentThread().getThreadGroup();
index = new AtomicInteger(0);
this.name = name;
}
@Override
public Thread newThread(Runnable r) {
Thread t = new Thread(group, r, name + " " + index.getAndIncrement());
if (t.isDaemon()) t.setDaemon(false);
if (t.getPriority() != Thread.NORM_PRIORITY) t.setPriority(Thread.NORM_PRIORITY);
return t;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy