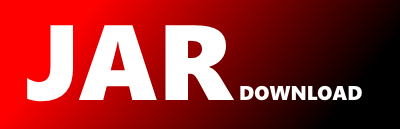
org.appdapter.gui.table.GenericBeansPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.appdapter.lib.gui Show documentation
Show all versions of org.appdapter.lib.gui Show documentation
Appdapter Maven project including Java and Scala, produces jar, not bundle. Excludes concrete SLF4J binding.
/*
* Copyright 2011 by The Appdapter Project (www.appdapter.org).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/*
* ModelMatrixPanel.java
*
* Created on Oct 25, 2010, 8:12:03 PM
*/
package org.appdapter.gui.table;
import static org.appdapter.core.log.Debuggable.printStackTrace;
import java.awt.Component;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JButton;
import javax.swing.JTable;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableModel;
import org.appdapter.api.trigger.Box;
import org.appdapter.core.jvm.CallableWithParameters;
import org.appdapter.core.convert.ToFromKeyConverter;
import org.appdapter.gui.api.WrapperValue;
import org.appdapter.gui.browse.Utility;
import org.appdapter.gui.swing.ScreenBoxPanel;
import org.appdapter.gui.swing.SimpleTextEditor;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* @author Stu B.
*/
abstract public class GenericBeansPanel extends ScreenBoxPanel implements CellConversions {
static Logger theLogger = LoggerFactory.getLogger(GenericBeansPanel.class);
public ToFromKeyConverter getCellConverter(Class valueClazz) {
return Utility.getToFromStringConverter(valueClazz);
}
protected String[] columnNamesPredefined;
protected CellConversions listFromH;
private List listOfRows;
protected Class rowClass;
protected Class matrixClass = null;
protected DefaultTableModel myModel;
private List actionComponents = new ArrayList();
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JPanel myActionPanel;
private com.jidesoft.swing.JideSplitPane myBottomSplitPane;
private javax.swing.JPanel myFilterPanel;
private javax.swing.JTable myTable;
private javax.swing.JScrollPane myTableScrollPane;
private javax.swing.JSplitPane myTopSplitPane;
// End of variables declaration//GEN-END:variables
private SimpleTextEditor textArea1;
public GenericBeansPanel(Class matrixClass) {
this(matrixClass, null, null, null);
}
/** Creates new form ModelMatrixPanel */
public GenericBeansPanel(Class matrixClass, Class listClass, CellConversions getList, String[] colNames) {
super(listClass);
this.matrixClass = matrixClass;
this.rowClass = listClass;
this.columnNamesPredefined = colNames;
this.listFromH = getList == null ? this : getList;
actionComponents.clear();
populateActionPanel(null, rowClass);
}
public GenericBeansPanel(WrapperValue wv) {
this(wv.getObjectClass());
setObject(wv.reallyGetValue());
}
@Override protected void completeSubClassGUI() {
}
@Override public void focusOnBox(Box b) {
setObject(b);
theLogger.info("Focusing on box: " + b);
}
@Override public Class getClassOfBox() {
return matrixClass;
}
public SafeJTable getTable() {
return (SafeJTable) myTable;
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
myTopSplitPane = new javax.swing.JSplitPane();
myFilterPanel = new javax.swing.JPanel();
textArea1 = new SimpleTextEditor();
myActionPanel = new javax.swing.JPanel();
myBottomSplitPane = new com.jidesoft.swing.JideSplitPane();
myTableScrollPane = new javax.swing.JScrollPane();
setLayout(new java.awt.BorderLayout());
myTopSplitPane.setDividerLocation(60);
myTopSplitPane.setOrientation(javax.swing.JSplitPane.VERTICAL_SPLIT);
myFilterPanel.setLayout(new java.awt.BorderLayout());
textArea1.setMinimumSize(new java.awt.Dimension(100, 5));
myFilterPanel.add(textArea1, java.awt.BorderLayout.CENTER);
myFilterPanel.add(myActionPanel, java.awt.BorderLayout.SOUTH);
myTopSplitPane.setTopComponent(myFilterPanel);
myBottomSplitPane.setOrientation(0);
myBottomSplitPane.setLayout(new java.awt.BorderLayout());
if (myModel == null)
myModel = new CustomTableModel(new Object[][] {
}, new String[] {
});
if (myTable == null)
myTable = new SafeJTable(myModel);
myTable.setAutoCreateRowSorter(true);
myTable.setModel(myModel);
myTableScrollPane.setViewportView(myTable);
myBottomSplitPane.add(myTableScrollPane, java.awt.BorderLayout.CENTER);
myTopSplitPane.setBottomComponent(myBottomSplitPane);
add(myTopSplitPane, java.awt.BorderLayout.CENTER);
}// //GEN-END:initComponents
private void tableReInit() {
TableModel myModel = this.myModel;
boolean replacedMyTable = false;
if (myTable != null) {
TableModel tm = myTable.getModel();
if (myModel == null || myModel == tm) {
myModel = tm;
} else {
}
}
if (!(myModel instanceof CustomTableModel))
myModel = new CustomTableModel(new Object[][] {
}, new String[] {
});
if (!(myTable instanceof SafeJTable)) {
myTable = new SafeJTable(myModel);
replacedMyTable = true;
}
this.myModel = (DefaultTableModel) myModel;
if (myTable != null) {
TableModel tm = myTable.getModel();
if (myModel == null || myModel == tm) {
myModel = tm;
} else {
theLogger.warn("replacing table model " + tm);
myTable.setModel(myModel);
}
}
myTable.setAutoCreateRowSorter(true);
myTable.setModel(myModel);
if (replacedMyTable) {
if (myTableScrollPane != null)
myTableScrollPane.setViewportView(myTable);
}
}
public void populateActionPanel(Object object, Class rowClass2) {
addAction("Properties (Selected)", new CallableWithParameters() {
@Override public Object call(Object box, Object... params) {
try {
Utility.showProperties(getModel());
return true;
} catch (Throwable e) {
printStackTrace(e);
return false;
}
}
});
addAction("Object Property", new CallableWithParameters() {
@Override public Object call(Object box, Object... params) {
try {
Utility.showProperties(getValue());
return true;
} catch (Throwable e) {
printStackTrace(e);
return false;
}
}
});
addAction("Reload", new CallableWithParameters() {
@Override public Object call(Object box, Object... params) {
try {
return reloadObjectGUI(getValue());
} catch (Throwable e) {
printStackTrace(e);
return false;
}
}
});
}
protected CustomTableModel getModel() {
return (CustomTableModel) getTable().getModel();
}
protected void rowObjectChanged(Object to) {
repopulateActionPanel(to, rowClass);
}
public void repopulateActionPanel(Object to, Class rowClass) {
actionComponents.clear();
if (myActionPanel != null) {
myActionPanel.removeAll();
}
populateActionPanel(to, rowClass);
}
protected Object getSelectedRowObject() {
return getTable().getRowObject(getTable().getSelectedRow());
}
public void addAction(String label, final CallableWithParameters callableWithParameters) {
JButton myAddButton = new JButton(label);
myAddButton.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
callableWithParameters.call(evt);
}
});
actionComponents.add(myAddButton);
if (myActionPanel != null) {
myActionPanel.add(myAddButton);
}
}
@Override protected void initSubclassGUI() throws Throwable {
tableReInit();
initComponents();
tableReInit();
Utility.makeTablePopupHandler(myTable);
}
@Override public boolean isObjectBoundGUI() {
return true;
}
public List listFromHolder(Object o) {
if (listFromH != null && listFromH != this) {
return listFromH.listFromHolder(o);
}
return Utility.recastCC(o, List.class);
}
@Override public void objectValueChanged(Object oldValue, Object newValue) {
try {
reloadObjectGUI(newValue);
} catch (Throwable e) {
printStackTrace(e);
}
}
@Override protected boolean reloadObjectGUI(Object obj) throws Throwable {
this.objectValue = obj;
JTable bl = getTable();
if (bl instanceof SafeJTable) {
SafeJTable sj = (SafeJTable) bl;
sj.setModelClasses(matrixClass, rowClass, this, columnNamesPredefined);
sj.setObject(obj);
}
repopulateActionPanel(getSelectedRowObject(), rowClass);
return true;
}
// means we render per cell instead
public boolean isRenderPerRow() {
return false;
}
@Override public Object convertWas(Object was, Object aValue, Class objNeedsToBe) {
return Utility.recastCC(aValue, objNeedsToBe);
}
}