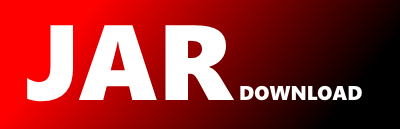
org.appdapter.gui.trigger.TriggerMouseAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.appdapter.lib.gui Show documentation
Show all versions of org.appdapter.lib.gui Show documentation
Appdapter Maven project including Java and Scala, produces jar, not bundle. Excludes concrete SLF4J binding.
package org.appdapter.gui.trigger;
import java.awt.Component;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.Graphics;
import java.awt.KeyboardFocusManager;
import java.awt.Point;
import java.awt.Toolkit;
import java.awt.datatransfer.Clipboard;
import java.awt.datatransfer.StringSelection;
import java.awt.event.ActionEvent;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.awt.image.BufferedImage;
import java.awt.image.RescaleOp;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import javax.accessibility.Accessible;
import javax.swing.AbstractAction;
import javax.swing.Action;
import javax.swing.CellEditor;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JComponent;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import javax.swing.JPopupMenu;
import javax.swing.JTabbedPane;
import javax.swing.JTable;
import javax.swing.JTextArea;
import javax.swing.JTree;
import javax.swing.KeyStroke;
import javax.swing.ListModel;
import javax.swing.SwingConstants;
import javax.swing.SwingUtilities;
import javax.swing.event.PopupMenuEvent;
import javax.swing.event.PopupMenuListener;
import javax.swing.plaf.basic.BasicArrowButton;
import javax.swing.plaf.basic.BasicComboBoxUI;
import javax.swing.plaf.basic.BasicComboPopup;
import javax.swing.table.TableModel;
import javax.swing.text.DefaultEditorKit;
import javax.swing.text.JTextComponent;
import javax.swing.text.TextAction;
import javax.swing.tree.DefaultMutableTreeNode;
import javax.swing.tree.TreePath;
import org.appdapter.core.jvm.CallableWithParameters;
import org.appdapter.core.jvm.GetObject;
import org.appdapter.gui.browse.Utility;
import org.appdapter.gui.swing.ObjectView;
import org.appdapter.gui.swing.SmallObjectView;
import com.jidesoft.swing.JideTabbedPane;
//import com.sun.java.swing.plaf.windows.WindowsComboBoxUI;
public class TriggerMouseAdapter extends MouseAdapter implements PopupMenuListener {
static boolean alreadyInstalled = false;
static Map comp2adapter = new HashMap();
static ImageIcon icon = null;
static Object installKBListenerLock = new Object();
static boolean lookedOnce = true;
public static boolean onlyTextComponents = true;
static {
installMouseListeners();
}
public static void addCopyText(JPopupMenu popup, final String txt) {
if (txt == null || txt.length() < 1)
return;
String str = "\"" + txt + "\"";
if (txt.length() > 30) {
int ind = txt.indexOf(' ', 29);
if (ind == -1) {
ind = txt.length();
} else {
str = "All \"" + txt.substring(0, ind) + "...\"";
}
}
JMenuItem item = new JMenuItem(new AbstractAction("Copy " + str) {
@Override public void actionPerformed(ActionEvent e) {
StringSelection stringSelection = new StringSelection(txt);
Clipboard clpbrd = Toolkit.getDefaultToolkit().getSystemClipboard();
clpbrd.setContents(stringSelection, null);
}
});
item.setEnabled(true);
item.getAction().putValue(Action.ACCELERATOR_KEY, KeyStroke.getKeyStroke("shift ctrl c"));
popup.add(item);
if (!Utility.isAppReady()) {
return;
}
Collection boxes = Utility.getTreeBoxCollection().findObjectsByName(txt, Object.class);
if (boxes != null) {
for (Object o : boxes)
TriggerMenuFactory.addTriggersToPopup(o, popup);
}
}
public static void addCutPaste(final JTextComponent component, final JPopupMenu menu) {
JMenuItem item;
item = new JMenuItem(new DefaultEditorKit.CopyAction());
item.setText("Copy");
item.setEnabled(component.getSelectionStart() != component.getSelectionEnd());
item.getAction().putValue(Action.ACCELERATOR_KEY, KeyStroke.getKeyStroke("ctrl C"));
menu.add(item);
item = new JMenuItem(new DefaultEditorKit.CutAction());
item.setText("Cut");
item.setEnabled(component.isEditable() && component.getSelectionStart() != component.getSelectionEnd());
item.getAction().putValue(Action.ACCELERATOR_KEY, KeyStroke.getKeyStroke("ctrl X"));
menu.add(item);
item = new JMenuItem(new DefaultEditorKit.PasteAction());
item.setText("Paste");
item.setEnabled(component.isEditable());
item.getAction().putValue(Action.ACCELERATOR_KEY, KeyStroke.getKeyStroke("ctrl V"));
menu.add(item);
menu.addSeparator();
menu.add(new AbstractAction("Select All") {
{
putValue(Action.ACCELERATOR_KEY, KeyStroke.getKeyStroke("ctrl A"));
}
public void actionPerformed(ActionEvent e) {
component.selectAll();
}
public boolean isEnabled() {
return component.isEnabled() && component.getText().length() > 0;
}
});
Action[] acts = component.getActions();
if (acts != null && acts.length > 0) {
menu.addSeparator();
for (Action act : acts) {
if (act instanceof TextAction)
continue;
menu.add(act);
}
menu.addSeparator();
}
}
public static Component addAdditionalTextItems(MouseEvent e, JPopupMenu popup) {
Component c = null;
if (c == null) {
c = e.getComponent();
}
if (c instanceof JTextComponent) {
addCutPaste((JTextComponent) c, popup);
}
final String txt = getTextValue(e, c, true);
if (txt != null && txt.length() > 0) {
addCopyText(popup, txt);
final String word = getTextValue(e, c, false);
if (word != null && word.length() > 0 && !word.equals(txt)) {
addCopyText(popup, word);
}
}
return c;
}
static boolean addWorkarrounds(JComboBox combo) {
if (lookedOnce && icon == null)
return true;
/*
* When tryig to run an osgi app outside of maven, I get:
* java.lang.NoClassDefFoundError: com/sun/java/swing/plaf/windows/WindowsComboBoxUI
* Commenting out the code below to temporarily fix this.
* -- Matt 2013-10-25
*/
// if (combo.getUI() instanceof WindowsComboBoxUI) {
// combo.setUI(new WindowsComboBoxUI() {
//
// @Override protected JButton createArrowButton() {
//
// if (icon == null && !lookedOnce) {
// lookedOnce = true;
// try {
// icon = new ImageIcon(getClass().getResource("14x14.png"));
// } catch (Exception e) {
//
// }
// }
// JButton button = new JButton(icon) {
// @Override public Dimension getPreferredSize() {
// return new Dimension(14, 14);
// }
// };
// button.setRolloverIcon(makeRolloverIcon(icon));
// button.setFocusPainted(false);
// button.setContentAreaFilled(false);
// return button;
// }
// });
// } else {
combo.setUI(new BasicComboBoxUI() {
@Override protected JButton createArrowButton() {
JButton button = super.createArrowButton();
((BasicArrowButton) button).setDirection(SwingConstants.EAST);
return button;
}
});
// }
return true;
}
public static String getTextValue(MouseEvent e, Component c, boolean useAllText) {
String allText = null;
if (c instanceof JTree) {
JTree tree = (JTree) c;
TreePath path = tree.getPathForLocation(e.getX(), e.getY());
if (path != null) {
DefaultMutableTreeNode treeNode = (DefaultMutableTreeNode) path.getLastPathComponent();
if (treeNode != null) {
allText = "" + treeNode;
}
}
}
if (c instanceof JTable) {
JTable theTable = (JTable) c;
int row = theTable.rowAtPoint(e.getPoint());
int col = theTable.columnAtPoint(e.getPoint());
CellEditor ce = theTable.getCellEditor(row, col);
Object val = null;
if (ce != null) {
val = ce.getCellEditorValue();
}
if (val == null)
val = theTable.getValueAt(row, col);
if (val == null) {
return null;
}
allText = "" + val;
}
if (allText == null)
allText = TriggerMenuFactory.getComponentLabelNoParent(c, 0);
if (useAllText)
return allText;
if (allText != null) {
int caret = -1;
if (c instanceof JTextArea) {
JTextArea textArea = (JTextArea) c;
textArea.setCaretPosition(textArea.viewToModel(e.getPoint()));
caret = textArea.getCaretPosition();
}
if (c instanceof JTextComponent) {
JTextComponent textArea = (JTextComponent) c;
String str = textArea.getSelectedText();
if (str == null || str.length() == 0) {
caret = textArea.viewToModel(e.getPoint());
textArea.setCaretPosition(caret);
caret = textArea.getCaretPosition();
} else {
caret = textArea.getSelectionStart();
}
}
return wordAt(caret, allText);
}
return null;
}
static public void installContextMenu(Component c) {
synchronized (comp2adapter) {
if (comp2adapter.containsKey(c))
return;
}
if (c instanceof JComponent) {
installMouseAdapter(c);
}
if (c instanceof Container) {
for (Component c1 : ((Container) c).getComponents()) {
installContextMenu(c1);
}
}
}
public static TriggerMouseAdapter installMouseAdapter(Component goc) {
synchronized (comp2adapter) {
Component comp = (Component) goc;
TriggerMouseAdapter ma = comp2adapter.get(comp);
if (ma == null) {
ma = new TriggerMouseAdapter(comp);
}
return ma;
}
}
public static TriggerMouseAdapter installMouseAdapter(Component comp, Object represented) {
TriggerMouseAdapter ma = installMouseAdapter(comp);
ma.setObject(represented);
return ma;
}
public static void installMouseListeners() {
if (alreadyInstalled)
return;
synchronized (installKBListenerLock) {
if (alreadyInstalled)
return;
alreadyInstalled = true;
}
KeyboardFocusManager focusManager = KeyboardFocusManager.getCurrentKeyboardFocusManager();
focusManager.addPropertyChangeListener("permanentFocusOwner", new PropertyChangeListener() {
@Override public void propertyChange(PropertyChangeEvent evt) {
TriggerMouseAdapter.noticeComponent(evt.getNewValue());
}
});
focusManager.addPropertyChangeListener("activeWindow", new PropertyChangeListener() {
@Override public void propertyChange(PropertyChangeEvent evt) {
TriggerMouseAdapter.noticeComponent(evt.getNewValue());
TriggerMouseAdapter.noticeComponent(evt.getOldValue());
}
});
}
public static ImageIcon makeRolloverIcon(ImageIcon srcIcon) {
RescaleOp op = new RescaleOp(new float[] { 1.2f, 1.2f, 1.2f, 1.0f }, new float[] { 0f, 0f, 0f, 0f }, null);
BufferedImage img = new BufferedImage(srcIcon.getIconWidth(), srcIcon.getIconHeight(), BufferedImage.TYPE_INT_ARGB);
Graphics g = img.getGraphics();
//g.drawImage(srcIcon.getImage(), 0, 0, null);
srcIcon.paintIcon(null, g, 0, 0);
g.dispose();
return new ImageIcon(op.filter(img, null));
}
public static Collection